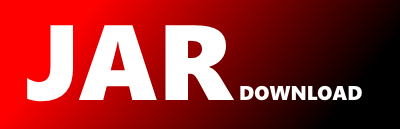
com.amazonaws.services.mediatailor.model.AvailSuppression Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediatailor Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediatailor.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The configuration for avail suppression, also known as ad suppression. For more information about ad suppression, see
* Ad Suppression.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AvailSuppression implements Serializable, Cloneable, StructuredPojo {
/**
*
* Defines the policy to apply to the avail suppression mode. BEHIND_LIVE_EDGE
will always use the full
* avail suppression policy. AFTER_LIVE_EDGE
mode can be used to invoke partial ad break fills when a
* session starts mid-break.
*
*/
private String fillPolicy;
/**
*
* Sets the ad suppression mode. By default, ad suppression is off and all ad breaks are filled with ads or slate.
* When Mode is set to BEHIND_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks
* on or behind the ad suppression Value time in the manifest lookback window. When Mode is set to
* AFTER_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks that are within the
* live edge plus the avail suppression value.
*
*/
private String mode;
/**
*
* A live edge offset time in HH:MM:SS. MediaTailor won't fill ad breaks on or behind this time in the manifest
* lookback window. If Value is set to 00:00:00, it is in sync with the live edge, and MediaTailor won't fill any ad
* breaks on or behind the live edge. If you set a Value time, MediaTailor won't fill any ad breaks on or behind
* this time in the manifest lookback window. For example, if you set 00:45:00, then MediaTailor will fill ad breaks
* that occur within 45 minutes behind the live edge, but won't fill ad breaks on or behind 45 minutes behind the
* live edge.
*
*/
private String value;
/**
*
* Defines the policy to apply to the avail suppression mode. BEHIND_LIVE_EDGE
will always use the full
* avail suppression policy. AFTER_LIVE_EDGE
mode can be used to invoke partial ad break fills when a
* session starts mid-break.
*
*
* @param fillPolicy
* Defines the policy to apply to the avail suppression mode. BEHIND_LIVE_EDGE
will always use
* the full avail suppression policy. AFTER_LIVE_EDGE
mode can be used to invoke partial ad
* break fills when a session starts mid-break.
* @see FillPolicy
*/
public void setFillPolicy(String fillPolicy) {
this.fillPolicy = fillPolicy;
}
/**
*
* Defines the policy to apply to the avail suppression mode. BEHIND_LIVE_EDGE
will always use the full
* avail suppression policy. AFTER_LIVE_EDGE
mode can be used to invoke partial ad break fills when a
* session starts mid-break.
*
*
* @return Defines the policy to apply to the avail suppression mode. BEHIND_LIVE_EDGE
will always use
* the full avail suppression policy. AFTER_LIVE_EDGE
mode can be used to invoke partial ad
* break fills when a session starts mid-break.
* @see FillPolicy
*/
public String getFillPolicy() {
return this.fillPolicy;
}
/**
*
* Defines the policy to apply to the avail suppression mode. BEHIND_LIVE_EDGE
will always use the full
* avail suppression policy. AFTER_LIVE_EDGE
mode can be used to invoke partial ad break fills when a
* session starts mid-break.
*
*
* @param fillPolicy
* Defines the policy to apply to the avail suppression mode. BEHIND_LIVE_EDGE
will always use
* the full avail suppression policy. AFTER_LIVE_EDGE
mode can be used to invoke partial ad
* break fills when a session starts mid-break.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FillPolicy
*/
public AvailSuppression withFillPolicy(String fillPolicy) {
setFillPolicy(fillPolicy);
return this;
}
/**
*
* Defines the policy to apply to the avail suppression mode. BEHIND_LIVE_EDGE
will always use the full
* avail suppression policy. AFTER_LIVE_EDGE
mode can be used to invoke partial ad break fills when a
* session starts mid-break.
*
*
* @param fillPolicy
* Defines the policy to apply to the avail suppression mode. BEHIND_LIVE_EDGE
will always use
* the full avail suppression policy. AFTER_LIVE_EDGE
mode can be used to invoke partial ad
* break fills when a session starts mid-break.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FillPolicy
*/
public AvailSuppression withFillPolicy(FillPolicy fillPolicy) {
this.fillPolicy = fillPolicy.toString();
return this;
}
/**
*
* Sets the ad suppression mode. By default, ad suppression is off and all ad breaks are filled with ads or slate.
* When Mode is set to BEHIND_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks
* on or behind the ad suppression Value time in the manifest lookback window. When Mode is set to
* AFTER_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks that are within the
* live edge plus the avail suppression value.
*
*
* @param mode
* Sets the ad suppression mode. By default, ad suppression is off and all ad breaks are filled with ads or
* slate. When Mode is set to BEHIND_LIVE_EDGE
, ad suppression is active and MediaTailor won't
* fill ad breaks on or behind the ad suppression Value time in the manifest lookback window. When Mode is
* set to AFTER_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks that
* are within the live edge plus the avail suppression value.
* @see Mode
*/
public void setMode(String mode) {
this.mode = mode;
}
/**
*
* Sets the ad suppression mode. By default, ad suppression is off and all ad breaks are filled with ads or slate.
* When Mode is set to BEHIND_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks
* on or behind the ad suppression Value time in the manifest lookback window. When Mode is set to
* AFTER_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks that are within the
* live edge plus the avail suppression value.
*
*
* @return Sets the ad suppression mode. By default, ad suppression is off and all ad breaks are filled with ads or
* slate. When Mode is set to BEHIND_LIVE_EDGE
, ad suppression is active and MediaTailor won't
* fill ad breaks on or behind the ad suppression Value time in the manifest lookback window. When Mode is
* set to AFTER_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks that
* are within the live edge plus the avail suppression value.
* @see Mode
*/
public String getMode() {
return this.mode;
}
/**
*
* Sets the ad suppression mode. By default, ad suppression is off and all ad breaks are filled with ads or slate.
* When Mode is set to BEHIND_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks
* on or behind the ad suppression Value time in the manifest lookback window. When Mode is set to
* AFTER_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks that are within the
* live edge plus the avail suppression value.
*
*
* @param mode
* Sets the ad suppression mode. By default, ad suppression is off and all ad breaks are filled with ads or
* slate. When Mode is set to BEHIND_LIVE_EDGE
, ad suppression is active and MediaTailor won't
* fill ad breaks on or behind the ad suppression Value time in the manifest lookback window. When Mode is
* set to AFTER_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks that
* are within the live edge plus the avail suppression value.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Mode
*/
public AvailSuppression withMode(String mode) {
setMode(mode);
return this;
}
/**
*
* Sets the ad suppression mode. By default, ad suppression is off and all ad breaks are filled with ads or slate.
* When Mode is set to BEHIND_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks
* on or behind the ad suppression Value time in the manifest lookback window. When Mode is set to
* AFTER_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks that are within the
* live edge plus the avail suppression value.
*
*
* @param mode
* Sets the ad suppression mode. By default, ad suppression is off and all ad breaks are filled with ads or
* slate. When Mode is set to BEHIND_LIVE_EDGE
, ad suppression is active and MediaTailor won't
* fill ad breaks on or behind the ad suppression Value time in the manifest lookback window. When Mode is
* set to AFTER_LIVE_EDGE
, ad suppression is active and MediaTailor won't fill ad breaks that
* are within the live edge plus the avail suppression value.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Mode
*/
public AvailSuppression withMode(Mode mode) {
this.mode = mode.toString();
return this;
}
/**
*
* A live edge offset time in HH:MM:SS. MediaTailor won't fill ad breaks on or behind this time in the manifest
* lookback window. If Value is set to 00:00:00, it is in sync with the live edge, and MediaTailor won't fill any ad
* breaks on or behind the live edge. If you set a Value time, MediaTailor won't fill any ad breaks on or behind
* this time in the manifest lookback window. For example, if you set 00:45:00, then MediaTailor will fill ad breaks
* that occur within 45 minutes behind the live edge, but won't fill ad breaks on or behind 45 minutes behind the
* live edge.
*
*
* @param value
* A live edge offset time in HH:MM:SS. MediaTailor won't fill ad breaks on or behind this time in the
* manifest lookback window. If Value is set to 00:00:00, it is in sync with the live edge, and MediaTailor
* won't fill any ad breaks on or behind the live edge. If you set a Value time, MediaTailor won't fill any
* ad breaks on or behind this time in the manifest lookback window. For example, if you set 00:45:00, then
* MediaTailor will fill ad breaks that occur within 45 minutes behind the live edge, but won't fill ad
* breaks on or behind 45 minutes behind the live edge.
*/
public void setValue(String value) {
this.value = value;
}
/**
*
* A live edge offset time in HH:MM:SS. MediaTailor won't fill ad breaks on or behind this time in the manifest
* lookback window. If Value is set to 00:00:00, it is in sync with the live edge, and MediaTailor won't fill any ad
* breaks on or behind the live edge. If you set a Value time, MediaTailor won't fill any ad breaks on or behind
* this time in the manifest lookback window. For example, if you set 00:45:00, then MediaTailor will fill ad breaks
* that occur within 45 minutes behind the live edge, but won't fill ad breaks on or behind 45 minutes behind the
* live edge.
*
*
* @return A live edge offset time in HH:MM:SS. MediaTailor won't fill ad breaks on or behind this time in the
* manifest lookback window. If Value is set to 00:00:00, it is in sync with the live edge, and MediaTailor
* won't fill any ad breaks on or behind the live edge. If you set a Value time, MediaTailor won't fill any
* ad breaks on or behind this time in the manifest lookback window. For example, if you set 00:45:00, then
* MediaTailor will fill ad breaks that occur within 45 minutes behind the live edge, but won't fill ad
* breaks on or behind 45 minutes behind the live edge.
*/
public String getValue() {
return this.value;
}
/**
*
* A live edge offset time in HH:MM:SS. MediaTailor won't fill ad breaks on or behind this time in the manifest
* lookback window. If Value is set to 00:00:00, it is in sync with the live edge, and MediaTailor won't fill any ad
* breaks on or behind the live edge. If you set a Value time, MediaTailor won't fill any ad breaks on or behind
* this time in the manifest lookback window. For example, if you set 00:45:00, then MediaTailor will fill ad breaks
* that occur within 45 minutes behind the live edge, but won't fill ad breaks on or behind 45 minutes behind the
* live edge.
*
*
* @param value
* A live edge offset time in HH:MM:SS. MediaTailor won't fill ad breaks on or behind this time in the
* manifest lookback window. If Value is set to 00:00:00, it is in sync with the live edge, and MediaTailor
* won't fill any ad breaks on or behind the live edge. If you set a Value time, MediaTailor won't fill any
* ad breaks on or behind this time in the manifest lookback window. For example, if you set 00:45:00, then
* MediaTailor will fill ad breaks that occur within 45 minutes behind the live edge, but won't fill ad
* breaks on or behind 45 minutes behind the live edge.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AvailSuppression withValue(String value) {
setValue(value);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFillPolicy() != null)
sb.append("FillPolicy: ").append(getFillPolicy()).append(",");
if (getMode() != null)
sb.append("Mode: ").append(getMode()).append(",");
if (getValue() != null)
sb.append("Value: ").append(getValue());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AvailSuppression == false)
return false;
AvailSuppression other = (AvailSuppression) obj;
if (other.getFillPolicy() == null ^ this.getFillPolicy() == null)
return false;
if (other.getFillPolicy() != null && other.getFillPolicy().equals(this.getFillPolicy()) == false)
return false;
if (other.getMode() == null ^ this.getMode() == null)
return false;
if (other.getMode() != null && other.getMode().equals(this.getMode()) == false)
return false;
if (other.getValue() == null ^ this.getValue() == null)
return false;
if (other.getValue() != null && other.getValue().equals(this.getValue()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFillPolicy() == null) ? 0 : getFillPolicy().hashCode());
hashCode = prime * hashCode + ((getMode() == null) ? 0 : getMode().hashCode());
hashCode = prime * hashCode + ((getValue() == null) ? 0 : getValue().hashCode());
return hashCode;
}
@Override
public AvailSuppression clone() {
try {
return (AvailSuppression) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.mediatailor.model.transform.AvailSuppressionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}