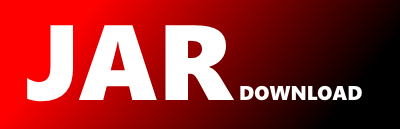
com.amazonaws.services.mediatailor.AWSMediaTailor Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediatailor Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediatailor;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.mediatailor.model.*;
/**
* Interface for accessing MediaTailor.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mediatailor.AbstractAWSMediaTailor} instead.
*
*
*
* Use the AWS Elemental MediaTailor SDKs and CLI to configure scalable ad insertion and linear channels. With
* MediaTailor, you can assemble existing content into a linear stream and serve targeted ads to viewers while
* maintaining broadcast quality in over-the-top (OTT) video applications. For information about using the service,
* including detailed information about the settings covered in this guide, see the AWS Elemental MediaTailor User Guide.
*
*
* Through the SDKs and the CLI you manage AWS Elemental MediaTailor configurations and channels the same as you do
* through the console. For example, you specify ad insertion behavior and mapping information for the origin server and
* the ad decision server (ADS).
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMediaTailor {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "api.mediatailor";
/**
*
* Configures Amazon CloudWatch log settings for a channel.
*
*
* @param configureLogsForChannelRequest
* @return Result of the ConfigureLogsForChannel operation returned by the service.
* @sample AWSMediaTailor.ConfigureLogsForChannel
* @see AWS API Documentation
*/
ConfigureLogsForChannelResult configureLogsForChannel(ConfigureLogsForChannelRequest configureLogsForChannelRequest);
/**
*
* Amazon CloudWatch log settings for a playback configuration.
*
*
* @param configureLogsForPlaybackConfigurationRequest
* Configures Amazon CloudWatch log settings for a playback configuration.
* @return Result of the ConfigureLogsForPlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailor.ConfigureLogsForPlaybackConfiguration
* @see AWS API Documentation
*/
ConfigureLogsForPlaybackConfigurationResult configureLogsForPlaybackConfiguration(
ConfigureLogsForPlaybackConfigurationRequest configureLogsForPlaybackConfigurationRequest);
/**
*
* Creates a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param createChannelRequest
* @return Result of the CreateChannel operation returned by the service.
* @sample AWSMediaTailor.CreateChannel
* @see AWS API
* Documentation
*/
CreateChannelResult createChannel(CreateChannelRequest createChannelRequest);
/**
*
* The live source configuration.
*
*
* @param createLiveSourceRequest
* @return Result of the CreateLiveSource operation returned by the service.
* @sample AWSMediaTailor.CreateLiveSource
* @see AWS
* API Documentation
*/
CreateLiveSourceResult createLiveSource(CreateLiveSourceRequest createLiveSourceRequest);
/**
*
* Creates a prefetch schedule for a playback configuration. A prefetch schedule allows you to tell MediaTailor to
* fetch and prepare certain ads before an ad break happens. For more information about ad prefetching, see Using ad prefetching in the
* MediaTailor User Guide.
*
*
* @param createPrefetchScheduleRequest
* @return Result of the CreatePrefetchSchedule operation returned by the service.
* @sample AWSMediaTailor.CreatePrefetchSchedule
* @see AWS API Documentation
*/
CreatePrefetchScheduleResult createPrefetchSchedule(CreatePrefetchScheduleRequest createPrefetchScheduleRequest);
/**
*
* Creates a program within a channel. For information about programs, see Working with programs
* in the MediaTailor User Guide.
*
*
* @param createProgramRequest
* @return Result of the CreateProgram operation returned by the service.
* @sample AWSMediaTailor.CreateProgram
* @see AWS API
* Documentation
*/
CreateProgramResult createProgram(CreateProgramRequest createProgramRequest);
/**
*
* Creates a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param createSourceLocationRequest
* @return Result of the CreateSourceLocation operation returned by the service.
* @sample AWSMediaTailor.CreateSourceLocation
* @see AWS API Documentation
*/
CreateSourceLocationResult createSourceLocation(CreateSourceLocationRequest createSourceLocationRequest);
/**
*
* The VOD source configuration parameters.
*
*
* @param createVodSourceRequest
* @return Result of the CreateVodSource operation returned by the service.
* @sample AWSMediaTailor.CreateVodSource
* @see AWS
* API Documentation
*/
CreateVodSourceResult createVodSource(CreateVodSourceRequest createVodSourceRequest);
/**
*
* Deletes a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param deleteChannelRequest
* @return Result of the DeleteChannel operation returned by the service.
* @sample AWSMediaTailor.DeleteChannel
* @see AWS API
* Documentation
*/
DeleteChannelResult deleteChannel(DeleteChannelRequest deleteChannelRequest);
/**
*
* The channel policy to delete.
*
*
* @param deleteChannelPolicyRequest
* @return Result of the DeleteChannelPolicy operation returned by the service.
* @sample AWSMediaTailor.DeleteChannelPolicy
* @see AWS API Documentation
*/
DeleteChannelPolicyResult deleteChannelPolicy(DeleteChannelPolicyRequest deleteChannelPolicyRequest);
/**
*
* The live source to delete.
*
*
* @param deleteLiveSourceRequest
* @return Result of the DeleteLiveSource operation returned by the service.
* @sample AWSMediaTailor.DeleteLiveSource
* @see AWS
* API Documentation
*/
DeleteLiveSourceResult deleteLiveSource(DeleteLiveSourceRequest deleteLiveSourceRequest);
/**
*
* Deletes a playback configuration. For information about MediaTailor configurations, see Working with configurations in AWS
* Elemental MediaTailor.
*
*
* @param deletePlaybackConfigurationRequest
* @return Result of the DeletePlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailor.DeletePlaybackConfiguration
* @see AWS API Documentation
*/
DeletePlaybackConfigurationResult deletePlaybackConfiguration(DeletePlaybackConfigurationRequest deletePlaybackConfigurationRequest);
/**
*
* Deletes a prefetch schedule for a specific playback configuration. If you call
* DeletePrefetchSchedule
on an expired prefetch schedule, MediaTailor returns an HTTP 404 status code.
* For more information about ad prefetching, see Using ad prefetching in the
* MediaTailor User Guide.
*
*
* @param deletePrefetchScheduleRequest
* @return Result of the DeletePrefetchSchedule operation returned by the service.
* @sample AWSMediaTailor.DeletePrefetchSchedule
* @see AWS API Documentation
*/
DeletePrefetchScheduleResult deletePrefetchSchedule(DeletePrefetchScheduleRequest deletePrefetchScheduleRequest);
/**
*
* Deletes a program within a channel. For information about programs, see Working with programs
* in the MediaTailor User Guide.
*
*
* @param deleteProgramRequest
* @return Result of the DeleteProgram operation returned by the service.
* @sample AWSMediaTailor.DeleteProgram
* @see AWS API
* Documentation
*/
DeleteProgramResult deleteProgram(DeleteProgramRequest deleteProgramRequest);
/**
*
* Deletes a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param deleteSourceLocationRequest
* @return Result of the DeleteSourceLocation operation returned by the service.
* @sample AWSMediaTailor.DeleteSourceLocation
* @see AWS API Documentation
*/
DeleteSourceLocationResult deleteSourceLocation(DeleteSourceLocationRequest deleteSourceLocationRequest);
/**
*
* The video on demand (VOD) source to delete.
*
*
* @param deleteVodSourceRequest
* @return Result of the DeleteVodSource operation returned by the service.
* @sample AWSMediaTailor.DeleteVodSource
* @see AWS
* API Documentation
*/
DeleteVodSourceResult deleteVodSource(DeleteVodSourceRequest deleteVodSourceRequest);
/**
*
* Describes a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param describeChannelRequest
* @return Result of the DescribeChannel operation returned by the service.
* @sample AWSMediaTailor.DescribeChannel
* @see AWS
* API Documentation
*/
DescribeChannelResult describeChannel(DescribeChannelRequest describeChannelRequest);
/**
*
* The live source to describe.
*
*
* @param describeLiveSourceRequest
* @return Result of the DescribeLiveSource operation returned by the service.
* @sample AWSMediaTailor.DescribeLiveSource
* @see AWS
* API Documentation
*/
DescribeLiveSourceResult describeLiveSource(DescribeLiveSourceRequest describeLiveSourceRequest);
/**
*
* Describes a program within a channel. For information about programs, see Working with programs
* in the MediaTailor User Guide.
*
*
* @param describeProgramRequest
* @return Result of the DescribeProgram operation returned by the service.
* @sample AWSMediaTailor.DescribeProgram
* @see AWS
* API Documentation
*/
DescribeProgramResult describeProgram(DescribeProgramRequest describeProgramRequest);
/**
*
* Describes a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param describeSourceLocationRequest
* @return Result of the DescribeSourceLocation operation returned by the service.
* @sample AWSMediaTailor.DescribeSourceLocation
* @see AWS API Documentation
*/
DescribeSourceLocationResult describeSourceLocation(DescribeSourceLocationRequest describeSourceLocationRequest);
/**
*
* Provides details about a specific video on demand (VOD) source in a specific source location.
*
*
* @param describeVodSourceRequest
* @return Result of the DescribeVodSource operation returned by the service.
* @sample AWSMediaTailor.DescribeVodSource
* @see AWS
* API Documentation
*/
DescribeVodSourceResult describeVodSource(DescribeVodSourceRequest describeVodSourceRequest);
/**
*
* Returns the channel's IAM policy. IAM policies are used to control access to your channel.
*
*
* @param getChannelPolicyRequest
* @return Result of the GetChannelPolicy operation returned by the service.
* @sample AWSMediaTailor.GetChannelPolicy
* @see AWS
* API Documentation
*/
GetChannelPolicyResult getChannelPolicy(GetChannelPolicyRequest getChannelPolicyRequest);
/**
*
* Retrieves information about your channel's schedule.
*
*
* @param getChannelScheduleRequest
* @return Result of the GetChannelSchedule operation returned by the service.
* @sample AWSMediaTailor.GetChannelSchedule
* @see AWS
* API Documentation
*/
GetChannelScheduleResult getChannelSchedule(GetChannelScheduleRequest getChannelScheduleRequest);
/**
*
* Retrieves a playback configuration. For information about MediaTailor configurations, see Working with configurations in AWS
* Elemental MediaTailor.
*
*
* @param getPlaybackConfigurationRequest
* @return Result of the GetPlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailor.GetPlaybackConfiguration
* @see AWS API Documentation
*/
GetPlaybackConfigurationResult getPlaybackConfiguration(GetPlaybackConfigurationRequest getPlaybackConfigurationRequest);
/**
*
* Retrieves a prefetch schedule for a playback configuration. A prefetch schedule allows you to tell MediaTailor to
* fetch and prepare certain ads before an ad break happens. For more information about ad prefetching, see Using ad prefetching in the
* MediaTailor User Guide.
*
*
* @param getPrefetchScheduleRequest
* @return Result of the GetPrefetchSchedule operation returned by the service.
* @sample AWSMediaTailor.GetPrefetchSchedule
* @see AWS API Documentation
*/
GetPrefetchScheduleResult getPrefetchSchedule(GetPrefetchScheduleRequest getPrefetchScheduleRequest);
/**
*
* Lists the alerts that are associated with a MediaTailor channel assembly resource.
*
*
* @param listAlertsRequest
* @return Result of the ListAlerts operation returned by the service.
* @sample AWSMediaTailor.ListAlerts
* @see AWS API
* Documentation
*/
ListAlertsResult listAlerts(ListAlertsRequest listAlertsRequest);
/**
*
* Retrieves information about the channels that are associated with the current AWS account.
*
*
* @param listChannelsRequest
* @return Result of the ListChannels operation returned by the service.
* @sample AWSMediaTailor.ListChannels
* @see AWS API
* Documentation
*/
ListChannelsResult listChannels(ListChannelsRequest listChannelsRequest);
/**
*
* Lists the live sources contained in a source location. A source represents a piece of content.
*
*
* @param listLiveSourcesRequest
* @return Result of the ListLiveSources operation returned by the service.
* @sample AWSMediaTailor.ListLiveSources
* @see AWS
* API Documentation
*/
ListLiveSourcesResult listLiveSources(ListLiveSourcesRequest listLiveSourcesRequest);
/**
*
* Retrieves existing playback configurations. For information about MediaTailor configurations, see Working with Configurations in AWS
* Elemental MediaTailor.
*
*
* @param listPlaybackConfigurationsRequest
* @return Result of the ListPlaybackConfigurations operation returned by the service.
* @sample AWSMediaTailor.ListPlaybackConfigurations
* @see AWS API Documentation
*/
ListPlaybackConfigurationsResult listPlaybackConfigurations(ListPlaybackConfigurationsRequest listPlaybackConfigurationsRequest);
/**
*
* Lists the prefetch schedules for a playback configuration.
*
*
* @param listPrefetchSchedulesRequest
* @return Result of the ListPrefetchSchedules operation returned by the service.
* @sample AWSMediaTailor.ListPrefetchSchedules
* @see AWS API Documentation
*/
ListPrefetchSchedulesResult listPrefetchSchedules(ListPrefetchSchedulesRequest listPrefetchSchedulesRequest);
/**
*
* Lists the source locations for a channel. A source location defines the host server URL, and contains a list of
* sources.
*
*
* @param listSourceLocationsRequest
* @return Result of the ListSourceLocations operation returned by the service.
* @sample AWSMediaTailor.ListSourceLocations
* @see AWS API Documentation
*/
ListSourceLocationsResult listSourceLocations(ListSourceLocationsRequest listSourceLocationsRequest);
/**
*
* A list of tags that are associated with this resource. Tags are key-value pairs that you can associate with
* Amazon resources to help with organization, access control, and cost tracking. For more information, see Tagging AWS Elemental MediaTailor
* Resources.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* A request contains unexpected data.
* @sample AWSMediaTailor.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the VOD sources contained in a source location. A source represents a piece of content.
*
*
* @param listVodSourcesRequest
* @return Result of the ListVodSources operation returned by the service.
* @sample AWSMediaTailor.ListVodSources
* @see AWS API
* Documentation
*/
ListVodSourcesResult listVodSources(ListVodSourcesRequest listVodSourcesRequest);
/**
*
* Creates an IAM policy for the channel. IAM policies are used to control access to your channel.
*
*
* @param putChannelPolicyRequest
* @return Result of the PutChannelPolicy operation returned by the service.
* @sample AWSMediaTailor.PutChannelPolicy
* @see AWS
* API Documentation
*/
PutChannelPolicyResult putChannelPolicy(PutChannelPolicyRequest putChannelPolicyRequest);
/**
*
* Creates a playback configuration. For information about MediaTailor configurations, see Working with configurations in AWS
* Elemental MediaTailor.
*
*
* @param putPlaybackConfigurationRequest
* @return Result of the PutPlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailor.PutPlaybackConfiguration
* @see AWS API Documentation
*/
PutPlaybackConfigurationResult putPlaybackConfiguration(PutPlaybackConfigurationRequest putPlaybackConfigurationRequest);
/**
*
* Starts a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param startChannelRequest
* @return Result of the StartChannel operation returned by the service.
* @sample AWSMediaTailor.StartChannel
* @see AWS API
* Documentation
*/
StartChannelResult startChannel(StartChannelRequest startChannelRequest);
/**
*
* Stops a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param stopChannelRequest
* @return Result of the StopChannel operation returned by the service.
* @sample AWSMediaTailor.StopChannel
* @see AWS API
* Documentation
*/
StopChannelResult stopChannel(StopChannelRequest stopChannelRequest);
/**
*
* The resource to tag. Tags are key-value pairs that you can associate with Amazon resources to help with
* organization, access control, and cost tracking. For more information, see Tagging AWS Elemental MediaTailor
* Resources.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* A request contains unexpected data.
* @sample AWSMediaTailor.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* The resource to untag.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* A request contains unexpected data.
* @sample AWSMediaTailor.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param updateChannelRequest
* @return Result of the UpdateChannel operation returned by the service.
* @sample AWSMediaTailor.UpdateChannel
* @see AWS API
* Documentation
*/
UpdateChannelResult updateChannel(UpdateChannelRequest updateChannelRequest);
/**
*
* Updates a live source's configuration.
*
*
* @param updateLiveSourceRequest
* @return Result of the UpdateLiveSource operation returned by the service.
* @sample AWSMediaTailor.UpdateLiveSource
* @see AWS
* API Documentation
*/
UpdateLiveSourceResult updateLiveSource(UpdateLiveSourceRequest updateLiveSourceRequest);
/**
*
* Updates a program within a channel.
*
*
* @param updateProgramRequest
* @return Result of the UpdateProgram operation returned by the service.
* @sample AWSMediaTailor.UpdateProgram
* @see AWS API
* Documentation
*/
UpdateProgramResult updateProgram(UpdateProgramRequest updateProgramRequest);
/**
*
* Updates a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param updateSourceLocationRequest
* @return Result of the UpdateSourceLocation operation returned by the service.
* @sample AWSMediaTailor.UpdateSourceLocation
* @see AWS API Documentation
*/
UpdateSourceLocationResult updateSourceLocation(UpdateSourceLocationRequest updateSourceLocationRequest);
/**
*
* Updates a VOD source's configuration.
*
*
* @param updateVodSourceRequest
* @return Result of the UpdateVodSource operation returned by the service.
* @sample AWSMediaTailor.UpdateVodSource
* @see AWS
* API Documentation
*/
UpdateVodSourceResult updateVodSource(UpdateVodSourceRequest updateVodSourceRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}