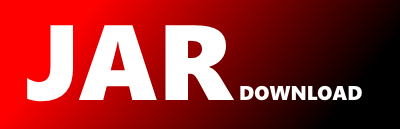
com.amazonaws.services.mediatailor.AWSMediaTailorAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediatailor Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediatailor;
import javax.annotation.Generated;
import com.amazonaws.services.mediatailor.model.*;
/**
* Interface for accessing MediaTailor asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mediatailor.AbstractAWSMediaTailorAsync} instead.
*
*
*
* Use the AWS Elemental MediaTailor SDKs and CLI to configure scalable ad insertion and linear channels. With
* MediaTailor, you can assemble existing content into a linear stream and serve targeted ads to viewers while
* maintaining broadcast quality in over-the-top (OTT) video applications. For information about using the service,
* including detailed information about the settings covered in this guide, see the AWS Elemental MediaTailor User Guide.
*
*
* Through the SDKs and the CLI you manage AWS Elemental MediaTailor configurations and channels the same as you do
* through the console. For example, you specify ad insertion behavior and mapping information for the origin server and
* the ad decision server (ADS).
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMediaTailorAsync extends AWSMediaTailor {
/**
*
* Configures Amazon CloudWatch log settings for a channel.
*
*
* @param configureLogsForChannelRequest
* @return A Java Future containing the result of the ConfigureLogsForChannel operation returned by the service.
* @sample AWSMediaTailorAsync.ConfigureLogsForChannel
* @see AWS API Documentation
*/
java.util.concurrent.Future configureLogsForChannelAsync(ConfigureLogsForChannelRequest configureLogsForChannelRequest);
/**
*
* Configures Amazon CloudWatch log settings for a channel.
*
*
* @param configureLogsForChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ConfigureLogsForChannel operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.ConfigureLogsForChannel
* @see AWS API Documentation
*/
java.util.concurrent.Future configureLogsForChannelAsync(ConfigureLogsForChannelRequest configureLogsForChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Amazon CloudWatch log settings for a playback configuration.
*
*
* @param configureLogsForPlaybackConfigurationRequest
* Configures Amazon CloudWatch log settings for a playback configuration.
* @return A Java Future containing the result of the ConfigureLogsForPlaybackConfiguration operation returned by
* the service.
* @sample AWSMediaTailorAsync.ConfigureLogsForPlaybackConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future configureLogsForPlaybackConfigurationAsync(
ConfigureLogsForPlaybackConfigurationRequest configureLogsForPlaybackConfigurationRequest);
/**
*
* Amazon CloudWatch log settings for a playback configuration.
*
*
* @param configureLogsForPlaybackConfigurationRequest
* Configures Amazon CloudWatch log settings for a playback configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ConfigureLogsForPlaybackConfiguration operation returned by
* the service.
* @sample AWSMediaTailorAsyncHandler.ConfigureLogsForPlaybackConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future configureLogsForPlaybackConfigurationAsync(
ConfigureLogsForPlaybackConfigurationRequest configureLogsForPlaybackConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param createChannelRequest
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* @sample AWSMediaTailorAsync.CreateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createChannelAsync(CreateChannelRequest createChannelRequest);
/**
*
* Creates a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param createChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.CreateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createChannelAsync(CreateChannelRequest createChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The live source configuration.
*
*
* @param createLiveSourceRequest
* @return A Java Future containing the result of the CreateLiveSource operation returned by the service.
* @sample AWSMediaTailorAsync.CreateLiveSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLiveSourceAsync(CreateLiveSourceRequest createLiveSourceRequest);
/**
*
* The live source configuration.
*
*
* @param createLiveSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLiveSource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.CreateLiveSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLiveSourceAsync(CreateLiveSourceRequest createLiveSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a prefetch schedule for a playback configuration. A prefetch schedule allows you to tell MediaTailor to
* fetch and prepare certain ads before an ad break happens. For more information about ad prefetching, see Using ad prefetching in the
* MediaTailor User Guide.
*
*
* @param createPrefetchScheduleRequest
* @return A Java Future containing the result of the CreatePrefetchSchedule operation returned by the service.
* @sample AWSMediaTailorAsync.CreatePrefetchSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future createPrefetchScheduleAsync(CreatePrefetchScheduleRequest createPrefetchScheduleRequest);
/**
*
* Creates a prefetch schedule for a playback configuration. A prefetch schedule allows you to tell MediaTailor to
* fetch and prepare certain ads before an ad break happens. For more information about ad prefetching, see Using ad prefetching in the
* MediaTailor User Guide.
*
*
* @param createPrefetchScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePrefetchSchedule operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.CreatePrefetchSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future createPrefetchScheduleAsync(CreatePrefetchScheduleRequest createPrefetchScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a program within a channel. For information about programs, see Working with programs
* in the MediaTailor User Guide.
*
*
* @param createProgramRequest
* @return A Java Future containing the result of the CreateProgram operation returned by the service.
* @sample AWSMediaTailorAsync.CreateProgram
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createProgramAsync(CreateProgramRequest createProgramRequest);
/**
*
* Creates a program within a channel. For information about programs, see Working with programs
* in the MediaTailor User Guide.
*
*
* @param createProgramRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProgram operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.CreateProgram
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createProgramAsync(CreateProgramRequest createProgramRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param createSourceLocationRequest
* @return A Java Future containing the result of the CreateSourceLocation operation returned by the service.
* @sample AWSMediaTailorAsync.CreateSourceLocation
* @see AWS API Documentation
*/
java.util.concurrent.Future createSourceLocationAsync(CreateSourceLocationRequest createSourceLocationRequest);
/**
*
* Creates a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param createSourceLocationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSourceLocation operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.CreateSourceLocation
* @see AWS API Documentation
*/
java.util.concurrent.Future createSourceLocationAsync(CreateSourceLocationRequest createSourceLocationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The VOD source configuration parameters.
*
*
* @param createVodSourceRequest
* @return A Java Future containing the result of the CreateVodSource operation returned by the service.
* @sample AWSMediaTailorAsync.CreateVodSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVodSourceAsync(CreateVodSourceRequest createVodSourceRequest);
/**
*
* The VOD source configuration parameters.
*
*
* @param createVodSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVodSource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.CreateVodSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVodSourceAsync(CreateVodSourceRequest createVodSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param deleteChannelRequest
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* @sample AWSMediaTailorAsync.DeleteChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteChannelAsync(DeleteChannelRequest deleteChannelRequest);
/**
*
* Deletes a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param deleteChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DeleteChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteChannelAsync(DeleteChannelRequest deleteChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The channel policy to delete.
*
*
* @param deleteChannelPolicyRequest
* @return A Java Future containing the result of the DeleteChannelPolicy operation returned by the service.
* @sample AWSMediaTailorAsync.DeleteChannelPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteChannelPolicyAsync(DeleteChannelPolicyRequest deleteChannelPolicyRequest);
/**
*
* The channel policy to delete.
*
*
* @param deleteChannelPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChannelPolicy operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DeleteChannelPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteChannelPolicyAsync(DeleteChannelPolicyRequest deleteChannelPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The live source to delete.
*
*
* @param deleteLiveSourceRequest
* @return A Java Future containing the result of the DeleteLiveSource operation returned by the service.
* @sample AWSMediaTailorAsync.DeleteLiveSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteLiveSourceAsync(DeleteLiveSourceRequest deleteLiveSourceRequest);
/**
*
* The live source to delete.
*
*
* @param deleteLiveSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLiveSource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DeleteLiveSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteLiveSourceAsync(DeleteLiveSourceRequest deleteLiveSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a playback configuration. For information about MediaTailor configurations, see Working with configurations in AWS
* Elemental MediaTailor.
*
*
* @param deletePlaybackConfigurationRequest
* @return A Java Future containing the result of the DeletePlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailorAsync.DeletePlaybackConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePlaybackConfigurationAsync(
DeletePlaybackConfigurationRequest deletePlaybackConfigurationRequest);
/**
*
* Deletes a playback configuration. For information about MediaTailor configurations, see Working with configurations in AWS
* Elemental MediaTailor.
*
*
* @param deletePlaybackConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DeletePlaybackConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePlaybackConfigurationAsync(
DeletePlaybackConfigurationRequest deletePlaybackConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a prefetch schedule for a specific playback configuration. If you call
* DeletePrefetchSchedule
on an expired prefetch schedule, MediaTailor returns an HTTP 404 status code.
* For more information about ad prefetching, see Using ad prefetching in the
* MediaTailor User Guide.
*
*
* @param deletePrefetchScheduleRequest
* @return A Java Future containing the result of the DeletePrefetchSchedule operation returned by the service.
* @sample AWSMediaTailorAsync.DeletePrefetchSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePrefetchScheduleAsync(DeletePrefetchScheduleRequest deletePrefetchScheduleRequest);
/**
*
* Deletes a prefetch schedule for a specific playback configuration. If you call
* DeletePrefetchSchedule
on an expired prefetch schedule, MediaTailor returns an HTTP 404 status code.
* For more information about ad prefetching, see Using ad prefetching in the
* MediaTailor User Guide.
*
*
* @param deletePrefetchScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePrefetchSchedule operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DeletePrefetchSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePrefetchScheduleAsync(DeletePrefetchScheduleRequest deletePrefetchScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a program within a channel. For information about programs, see Working with programs
* in the MediaTailor User Guide.
*
*
* @param deleteProgramRequest
* @return A Java Future containing the result of the DeleteProgram operation returned by the service.
* @sample AWSMediaTailorAsync.DeleteProgram
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteProgramAsync(DeleteProgramRequest deleteProgramRequest);
/**
*
* Deletes a program within a channel. For information about programs, see Working with programs
* in the MediaTailor User Guide.
*
*
* @param deleteProgramRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProgram operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DeleteProgram
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteProgramAsync(DeleteProgramRequest deleteProgramRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param deleteSourceLocationRequest
* @return A Java Future containing the result of the DeleteSourceLocation operation returned by the service.
* @sample AWSMediaTailorAsync.DeleteSourceLocation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSourceLocationAsync(DeleteSourceLocationRequest deleteSourceLocationRequest);
/**
*
* Deletes a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param deleteSourceLocationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSourceLocation operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DeleteSourceLocation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSourceLocationAsync(DeleteSourceLocationRequest deleteSourceLocationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The video on demand (VOD) source to delete.
*
*
* @param deleteVodSourceRequest
* @return A Java Future containing the result of the DeleteVodSource operation returned by the service.
* @sample AWSMediaTailorAsync.DeleteVodSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVodSourceAsync(DeleteVodSourceRequest deleteVodSourceRequest);
/**
*
* The video on demand (VOD) source to delete.
*
*
* @param deleteVodSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVodSource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DeleteVodSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVodSourceAsync(DeleteVodSourceRequest deleteVodSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param describeChannelRequest
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* @sample AWSMediaTailorAsync.DescribeChannel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeChannelAsync(DescribeChannelRequest describeChannelRequest);
/**
*
* Describes a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param describeChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DescribeChannel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeChannelAsync(DescribeChannelRequest describeChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The live source to describe.
*
*
* @param describeLiveSourceRequest
* @return A Java Future containing the result of the DescribeLiveSource operation returned by the service.
* @sample AWSMediaTailorAsync.DescribeLiveSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLiveSourceAsync(DescribeLiveSourceRequest describeLiveSourceRequest);
/**
*
* The live source to describe.
*
*
* @param describeLiveSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLiveSource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DescribeLiveSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLiveSourceAsync(DescribeLiveSourceRequest describeLiveSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a program within a channel. For information about programs, see Working with programs
* in the MediaTailor User Guide.
*
*
* @param describeProgramRequest
* @return A Java Future containing the result of the DescribeProgram operation returned by the service.
* @sample AWSMediaTailorAsync.DescribeProgram
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeProgramAsync(DescribeProgramRequest describeProgramRequest);
/**
*
* Describes a program within a channel. For information about programs, see Working with programs
* in the MediaTailor User Guide.
*
*
* @param describeProgramRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProgram operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DescribeProgram
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeProgramAsync(DescribeProgramRequest describeProgramRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param describeSourceLocationRequest
* @return A Java Future containing the result of the DescribeSourceLocation operation returned by the service.
* @sample AWSMediaTailorAsync.DescribeSourceLocation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSourceLocationAsync(DescribeSourceLocationRequest describeSourceLocationRequest);
/**
*
* Describes a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param describeSourceLocationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSourceLocation operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DescribeSourceLocation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSourceLocationAsync(DescribeSourceLocationRequest describeSourceLocationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides details about a specific video on demand (VOD) source in a specific source location.
*
*
* @param describeVodSourceRequest
* @return A Java Future containing the result of the DescribeVodSource operation returned by the service.
* @sample AWSMediaTailorAsync.DescribeVodSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVodSourceAsync(DescribeVodSourceRequest describeVodSourceRequest);
/**
*
* Provides details about a specific video on demand (VOD) source in a specific source location.
*
*
* @param describeVodSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVodSource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.DescribeVodSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVodSourceAsync(DescribeVodSourceRequest describeVodSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the channel's IAM policy. IAM policies are used to control access to your channel.
*
*
* @param getChannelPolicyRequest
* @return A Java Future containing the result of the GetChannelPolicy operation returned by the service.
* @sample AWSMediaTailorAsync.GetChannelPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getChannelPolicyAsync(GetChannelPolicyRequest getChannelPolicyRequest);
/**
*
* Returns the channel's IAM policy. IAM policies are used to control access to your channel.
*
*
* @param getChannelPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetChannelPolicy operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.GetChannelPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getChannelPolicyAsync(GetChannelPolicyRequest getChannelPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about your channel's schedule.
*
*
* @param getChannelScheduleRequest
* @return A Java Future containing the result of the GetChannelSchedule operation returned by the service.
* @sample AWSMediaTailorAsync.GetChannelSchedule
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getChannelScheduleAsync(GetChannelScheduleRequest getChannelScheduleRequest);
/**
*
* Retrieves information about your channel's schedule.
*
*
* @param getChannelScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetChannelSchedule operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.GetChannelSchedule
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getChannelScheduleAsync(GetChannelScheduleRequest getChannelScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a playback configuration. For information about MediaTailor configurations, see Working with configurations in AWS
* Elemental MediaTailor.
*
*
* @param getPlaybackConfigurationRequest
* @return A Java Future containing the result of the GetPlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailorAsync.GetPlaybackConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getPlaybackConfigurationAsync(GetPlaybackConfigurationRequest getPlaybackConfigurationRequest);
/**
*
* Retrieves a playback configuration. For information about MediaTailor configurations, see Working with configurations in AWS
* Elemental MediaTailor.
*
*
* @param getPlaybackConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.GetPlaybackConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getPlaybackConfigurationAsync(GetPlaybackConfigurationRequest getPlaybackConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a prefetch schedule for a playback configuration. A prefetch schedule allows you to tell MediaTailor to
* fetch and prepare certain ads before an ad break happens. For more information about ad prefetching, see Using ad prefetching in the
* MediaTailor User Guide.
*
*
* @param getPrefetchScheduleRequest
* @return A Java Future containing the result of the GetPrefetchSchedule operation returned by the service.
* @sample AWSMediaTailorAsync.GetPrefetchSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future getPrefetchScheduleAsync(GetPrefetchScheduleRequest getPrefetchScheduleRequest);
/**
*
* Retrieves a prefetch schedule for a playback configuration. A prefetch schedule allows you to tell MediaTailor to
* fetch and prepare certain ads before an ad break happens. For more information about ad prefetching, see Using ad prefetching in the
* MediaTailor User Guide.
*
*
* @param getPrefetchScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPrefetchSchedule operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.GetPrefetchSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future getPrefetchScheduleAsync(GetPrefetchScheduleRequest getPrefetchScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the alerts that are associated with a MediaTailor channel assembly resource.
*
*
* @param listAlertsRequest
* @return A Java Future containing the result of the ListAlerts operation returned by the service.
* @sample AWSMediaTailorAsync.ListAlerts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAlertsAsync(ListAlertsRequest listAlertsRequest);
/**
*
* Lists the alerts that are associated with a MediaTailor channel assembly resource.
*
*
* @param listAlertsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAlerts operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.ListAlerts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAlertsAsync(ListAlertsRequest listAlertsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the channels that are associated with the current AWS account.
*
*
* @param listChannelsRequest
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* @sample AWSMediaTailorAsync.ListChannels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listChannelsAsync(ListChannelsRequest listChannelsRequest);
/**
*
* Retrieves information about the channels that are associated with the current AWS account.
*
*
* @param listChannelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.ListChannels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listChannelsAsync(ListChannelsRequest listChannelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the live sources contained in a source location. A source represents a piece of content.
*
*
* @param listLiveSourcesRequest
* @return A Java Future containing the result of the ListLiveSources operation returned by the service.
* @sample AWSMediaTailorAsync.ListLiveSources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLiveSourcesAsync(ListLiveSourcesRequest listLiveSourcesRequest);
/**
*
* Lists the live sources contained in a source location. A source represents a piece of content.
*
*
* @param listLiveSourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLiveSources operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.ListLiveSources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLiveSourcesAsync(ListLiveSourcesRequest listLiveSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves existing playback configurations. For information about MediaTailor configurations, see Working with Configurations in AWS
* Elemental MediaTailor.
*
*
* @param listPlaybackConfigurationsRequest
* @return A Java Future containing the result of the ListPlaybackConfigurations operation returned by the service.
* @sample AWSMediaTailorAsync.ListPlaybackConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listPlaybackConfigurationsAsync(
ListPlaybackConfigurationsRequest listPlaybackConfigurationsRequest);
/**
*
* Retrieves existing playback configurations. For information about MediaTailor configurations, see Working with Configurations in AWS
* Elemental MediaTailor.
*
*
* @param listPlaybackConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPlaybackConfigurations operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.ListPlaybackConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listPlaybackConfigurationsAsync(
ListPlaybackConfigurationsRequest listPlaybackConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the prefetch schedules for a playback configuration.
*
*
* @param listPrefetchSchedulesRequest
* @return A Java Future containing the result of the ListPrefetchSchedules operation returned by the service.
* @sample AWSMediaTailorAsync.ListPrefetchSchedules
* @see AWS API Documentation
*/
java.util.concurrent.Future listPrefetchSchedulesAsync(ListPrefetchSchedulesRequest listPrefetchSchedulesRequest);
/**
*
* Lists the prefetch schedules for a playback configuration.
*
*
* @param listPrefetchSchedulesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPrefetchSchedules operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.ListPrefetchSchedules
* @see AWS API Documentation
*/
java.util.concurrent.Future listPrefetchSchedulesAsync(ListPrefetchSchedulesRequest listPrefetchSchedulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the source locations for a channel. A source location defines the host server URL, and contains a list of
* sources.
*
*
* @param listSourceLocationsRequest
* @return A Java Future containing the result of the ListSourceLocations operation returned by the service.
* @sample AWSMediaTailorAsync.ListSourceLocations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSourceLocationsAsync(ListSourceLocationsRequest listSourceLocationsRequest);
/**
*
* Lists the source locations for a channel. A source location defines the host server URL, and contains a list of
* sources.
*
*
* @param listSourceLocationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSourceLocations operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.ListSourceLocations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSourceLocationsAsync(ListSourceLocationsRequest listSourceLocationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A list of tags that are associated with this resource. Tags are key-value pairs that you can associate with
* Amazon resources to help with organization, access control, and cost tracking. For more information, see Tagging AWS Elemental MediaTailor
* Resources.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMediaTailorAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* A list of tags that are associated with this resource. Tags are key-value pairs that you can associate with
* Amazon resources to help with organization, access control, and cost tracking. For more information, see Tagging AWS Elemental MediaTailor
* Resources.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the VOD sources contained in a source location. A source represents a piece of content.
*
*
* @param listVodSourcesRequest
* @return A Java Future containing the result of the ListVodSources operation returned by the service.
* @sample AWSMediaTailorAsync.ListVodSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVodSourcesAsync(ListVodSourcesRequest listVodSourcesRequest);
/**
*
* Lists the VOD sources contained in a source location. A source represents a piece of content.
*
*
* @param listVodSourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVodSources operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.ListVodSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVodSourcesAsync(ListVodSourcesRequest listVodSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an IAM policy for the channel. IAM policies are used to control access to your channel.
*
*
* @param putChannelPolicyRequest
* @return A Java Future containing the result of the PutChannelPolicy operation returned by the service.
* @sample AWSMediaTailorAsync.PutChannelPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putChannelPolicyAsync(PutChannelPolicyRequest putChannelPolicyRequest);
/**
*
* Creates an IAM policy for the channel. IAM policies are used to control access to your channel.
*
*
* @param putChannelPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutChannelPolicy operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.PutChannelPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putChannelPolicyAsync(PutChannelPolicyRequest putChannelPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a playback configuration. For information about MediaTailor configurations, see Working with configurations in AWS
* Elemental MediaTailor.
*
*
* @param putPlaybackConfigurationRequest
* @return A Java Future containing the result of the PutPlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailorAsync.PutPlaybackConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putPlaybackConfigurationAsync(PutPlaybackConfigurationRequest putPlaybackConfigurationRequest);
/**
*
* Creates a playback configuration. For information about MediaTailor configurations, see Working with configurations in AWS
* Elemental MediaTailor.
*
*
* @param putPlaybackConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutPlaybackConfiguration operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.PutPlaybackConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putPlaybackConfigurationAsync(PutPlaybackConfigurationRequest putPlaybackConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param startChannelRequest
* @return A Java Future containing the result of the StartChannel operation returned by the service.
* @sample AWSMediaTailorAsync.StartChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startChannelAsync(StartChannelRequest startChannelRequest);
/**
*
* Starts a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param startChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartChannel operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.StartChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startChannelAsync(StartChannelRequest startChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param stopChannelRequest
* @return A Java Future containing the result of the StopChannel operation returned by the service.
* @sample AWSMediaTailorAsync.StopChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopChannelAsync(StopChannelRequest stopChannelRequest);
/**
*
* Stops a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param stopChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopChannel operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.StopChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopChannelAsync(StopChannelRequest stopChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The resource to tag. Tags are key-value pairs that you can associate with Amazon resources to help with
* organization, access control, and cost tracking. For more information, see Tagging AWS Elemental MediaTailor
* Resources.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMediaTailorAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* The resource to tag. Tags are key-value pairs that you can associate with Amazon resources to help with
* organization, access control, and cost tracking. For more information, see Tagging AWS Elemental MediaTailor
* Resources.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The resource to untag.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMediaTailorAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* The resource to untag.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param updateChannelRequest
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* @sample AWSMediaTailorAsync.UpdateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateChannelAsync(UpdateChannelRequest updateChannelRequest);
/**
*
* Updates a channel. For information about MediaTailor channels, see Working with channels
* in the MediaTailor User Guide.
*
*
* @param updateChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.UpdateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateChannelAsync(UpdateChannelRequest updateChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a live source's configuration.
*
*
* @param updateLiveSourceRequest
* @return A Java Future containing the result of the UpdateLiveSource operation returned by the service.
* @sample AWSMediaTailorAsync.UpdateLiveSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateLiveSourceAsync(UpdateLiveSourceRequest updateLiveSourceRequest);
/**
*
* Updates a live source's configuration.
*
*
* @param updateLiveSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLiveSource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.UpdateLiveSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateLiveSourceAsync(UpdateLiveSourceRequest updateLiveSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a program within a channel.
*
*
* @param updateProgramRequest
* @return A Java Future containing the result of the UpdateProgram operation returned by the service.
* @sample AWSMediaTailorAsync.UpdateProgram
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateProgramAsync(UpdateProgramRequest updateProgramRequest);
/**
*
* Updates a program within a channel.
*
*
* @param updateProgramRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateProgram operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.UpdateProgram
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateProgramAsync(UpdateProgramRequest updateProgramRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param updateSourceLocationRequest
* @return A Java Future containing the result of the UpdateSourceLocation operation returned by the service.
* @sample AWSMediaTailorAsync.UpdateSourceLocation
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSourceLocationAsync(UpdateSourceLocationRequest updateSourceLocationRequest);
/**
*
* Updates a source location. A source location is a container for sources. For more information about source
* locations, see Working with
* source locations in the MediaTailor User Guide.
*
*
* @param updateSourceLocationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSourceLocation operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.UpdateSourceLocation
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSourceLocationAsync(UpdateSourceLocationRequest updateSourceLocationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a VOD source's configuration.
*
*
* @param updateVodSourceRequest
* @return A Java Future containing the result of the UpdateVodSource operation returned by the service.
* @sample AWSMediaTailorAsync.UpdateVodSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVodSourceAsync(UpdateVodSourceRequest updateVodSourceRequest);
/**
*
* Updates a VOD source's configuration.
*
*
* @param updateVodSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVodSource operation returned by the service.
* @sample AWSMediaTailorAsyncHandler.UpdateVodSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVodSourceAsync(UpdateVodSourceRequest updateVodSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}