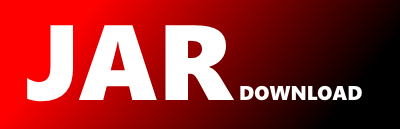
com.amazonaws.services.mediatailor.model.AdBreak Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediatailor Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediatailor.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Ad break configuration parameters.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AdBreak implements Serializable, Cloneable, StructuredPojo {
/**
*
* Defines a list of key/value pairs that MediaTailor generates within the EXT-X-ASSET
tag for
* SCTE35_ENHANCED
output.
*
*/
private java.util.List adBreakMetadata;
/**
*
* The SCTE-35 ad insertion type. Accepted value: SPLICE_INSERT
, TIME_SIGNAL
.
*
*/
private String messageType;
/**
*
* How long (in milliseconds) after the beginning of the program that an ad starts. This value must fall within
* 100ms of a segment boundary, otherwise the ad break will be skipped.
*
*/
private Long offsetMillis;
/**
*
* Ad break slate configuration.
*
*/
private SlateSource slate;
/**
*
* This defines the SCTE-35 splice_insert()
message inserted around the ad. For information about using
* splice_insert()
, see the SCTE-35 specficiaiton, section 9.7.3.1.
*
*/
private SpliceInsertMessage spliceInsertMessage;
/**
*
* Defines the SCTE-35 time_signal
message inserted around the ad.
*
*
* Programs on a channel's schedule can be configured with one or more ad breaks. You can attach a
* splice_insert
SCTE-35 message to the ad break. This message provides basic metadata about the ad
* break.
*
*
* See section 9.7.4 of the 2022 SCTE-35 specification for more information.
*
*/
private TimeSignalMessage timeSignalMessage;
/**
*
* Defines a list of key/value pairs that MediaTailor generates within the EXT-X-ASSET
tag for
* SCTE35_ENHANCED
output.
*
*
* @return Defines a list of key/value pairs that MediaTailor generates within the EXT-X-ASSET
tag for
* SCTE35_ENHANCED
output.
*/
public java.util.List getAdBreakMetadata() {
return adBreakMetadata;
}
/**
*
* Defines a list of key/value pairs that MediaTailor generates within the EXT-X-ASSET
tag for
* SCTE35_ENHANCED
output.
*
*
* @param adBreakMetadata
* Defines a list of key/value pairs that MediaTailor generates within the EXT-X-ASSET
tag for
* SCTE35_ENHANCED
output.
*/
public void setAdBreakMetadata(java.util.Collection adBreakMetadata) {
if (adBreakMetadata == null) {
this.adBreakMetadata = null;
return;
}
this.adBreakMetadata = new java.util.ArrayList(adBreakMetadata);
}
/**
*
* Defines a list of key/value pairs that MediaTailor generates within the EXT-X-ASSET
tag for
* SCTE35_ENHANCED
output.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdBreakMetadata(java.util.Collection)} or {@link #withAdBreakMetadata(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param adBreakMetadata
* Defines a list of key/value pairs that MediaTailor generates within the EXT-X-ASSET
tag for
* SCTE35_ENHANCED
output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdBreak withAdBreakMetadata(KeyValuePair... adBreakMetadata) {
if (this.adBreakMetadata == null) {
setAdBreakMetadata(new java.util.ArrayList(adBreakMetadata.length));
}
for (KeyValuePair ele : adBreakMetadata) {
this.adBreakMetadata.add(ele);
}
return this;
}
/**
*
* Defines a list of key/value pairs that MediaTailor generates within the EXT-X-ASSET
tag for
* SCTE35_ENHANCED
output.
*
*
* @param adBreakMetadata
* Defines a list of key/value pairs that MediaTailor generates within the EXT-X-ASSET
tag for
* SCTE35_ENHANCED
output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdBreak withAdBreakMetadata(java.util.Collection adBreakMetadata) {
setAdBreakMetadata(adBreakMetadata);
return this;
}
/**
*
* The SCTE-35 ad insertion type. Accepted value: SPLICE_INSERT
, TIME_SIGNAL
.
*
*
* @param messageType
* The SCTE-35 ad insertion type. Accepted value: SPLICE_INSERT
, TIME_SIGNAL
.
* @see MessageType
*/
public void setMessageType(String messageType) {
this.messageType = messageType;
}
/**
*
* The SCTE-35 ad insertion type. Accepted value: SPLICE_INSERT
, TIME_SIGNAL
.
*
*
* @return The SCTE-35 ad insertion type. Accepted value: SPLICE_INSERT
, TIME_SIGNAL
.
* @see MessageType
*/
public String getMessageType() {
return this.messageType;
}
/**
*
* The SCTE-35 ad insertion type. Accepted value: SPLICE_INSERT
, TIME_SIGNAL
.
*
*
* @param messageType
* The SCTE-35 ad insertion type. Accepted value: SPLICE_INSERT
, TIME_SIGNAL
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MessageType
*/
public AdBreak withMessageType(String messageType) {
setMessageType(messageType);
return this;
}
/**
*
* The SCTE-35 ad insertion type. Accepted value: SPLICE_INSERT
, TIME_SIGNAL
.
*
*
* @param messageType
* The SCTE-35 ad insertion type. Accepted value: SPLICE_INSERT
, TIME_SIGNAL
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MessageType
*/
public AdBreak withMessageType(MessageType messageType) {
this.messageType = messageType.toString();
return this;
}
/**
*
* How long (in milliseconds) after the beginning of the program that an ad starts. This value must fall within
* 100ms of a segment boundary, otherwise the ad break will be skipped.
*
*
* @param offsetMillis
* How long (in milliseconds) after the beginning of the program that an ad starts. This value must fall
* within 100ms of a segment boundary, otherwise the ad break will be skipped.
*/
public void setOffsetMillis(Long offsetMillis) {
this.offsetMillis = offsetMillis;
}
/**
*
* How long (in milliseconds) after the beginning of the program that an ad starts. This value must fall within
* 100ms of a segment boundary, otherwise the ad break will be skipped.
*
*
* @return How long (in milliseconds) after the beginning of the program that an ad starts. This value must fall
* within 100ms of a segment boundary, otherwise the ad break will be skipped.
*/
public Long getOffsetMillis() {
return this.offsetMillis;
}
/**
*
* How long (in milliseconds) after the beginning of the program that an ad starts. This value must fall within
* 100ms of a segment boundary, otherwise the ad break will be skipped.
*
*
* @param offsetMillis
* How long (in milliseconds) after the beginning of the program that an ad starts. This value must fall
* within 100ms of a segment boundary, otherwise the ad break will be skipped.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdBreak withOffsetMillis(Long offsetMillis) {
setOffsetMillis(offsetMillis);
return this;
}
/**
*
* Ad break slate configuration.
*
*
* @param slate
* Ad break slate configuration.
*/
public void setSlate(SlateSource slate) {
this.slate = slate;
}
/**
*
* Ad break slate configuration.
*
*
* @return Ad break slate configuration.
*/
public SlateSource getSlate() {
return this.slate;
}
/**
*
* Ad break slate configuration.
*
*
* @param slate
* Ad break slate configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdBreak withSlate(SlateSource slate) {
setSlate(slate);
return this;
}
/**
*
* This defines the SCTE-35 splice_insert()
message inserted around the ad. For information about using
* splice_insert()
, see the SCTE-35 specficiaiton, section 9.7.3.1.
*
*
* @param spliceInsertMessage
* This defines the SCTE-35 splice_insert()
message inserted around the ad. For information
* about using splice_insert()
, see the SCTE-35 specficiaiton, section 9.7.3.1.
*/
public void setSpliceInsertMessage(SpliceInsertMessage spliceInsertMessage) {
this.spliceInsertMessage = spliceInsertMessage;
}
/**
*
* This defines the SCTE-35 splice_insert()
message inserted around the ad. For information about using
* splice_insert()
, see the SCTE-35 specficiaiton, section 9.7.3.1.
*
*
* @return This defines the SCTE-35 splice_insert()
message inserted around the ad. For information
* about using splice_insert()
, see the SCTE-35 specficiaiton, section 9.7.3.1.
*/
public SpliceInsertMessage getSpliceInsertMessage() {
return this.spliceInsertMessage;
}
/**
*
* This defines the SCTE-35 splice_insert()
message inserted around the ad. For information about using
* splice_insert()
, see the SCTE-35 specficiaiton, section 9.7.3.1.
*
*
* @param spliceInsertMessage
* This defines the SCTE-35 splice_insert()
message inserted around the ad. For information
* about using splice_insert()
, see the SCTE-35 specficiaiton, section 9.7.3.1.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdBreak withSpliceInsertMessage(SpliceInsertMessage spliceInsertMessage) {
setSpliceInsertMessage(spliceInsertMessage);
return this;
}
/**
*
* Defines the SCTE-35 time_signal
message inserted around the ad.
*
*
* Programs on a channel's schedule can be configured with one or more ad breaks. You can attach a
* splice_insert
SCTE-35 message to the ad break. This message provides basic metadata about the ad
* break.
*
*
* See section 9.7.4 of the 2022 SCTE-35 specification for more information.
*
*
* @param timeSignalMessage
* Defines the SCTE-35 time_signal
message inserted around the ad.
*
* Programs on a channel's schedule can be configured with one or more ad breaks. You can attach a
* splice_insert
SCTE-35 message to the ad break. This message provides basic metadata about the
* ad break.
*
*
* See section 9.7.4 of the 2022 SCTE-35 specification for more information.
*/
public void setTimeSignalMessage(TimeSignalMessage timeSignalMessage) {
this.timeSignalMessage = timeSignalMessage;
}
/**
*
* Defines the SCTE-35 time_signal
message inserted around the ad.
*
*
* Programs on a channel's schedule can be configured with one or more ad breaks. You can attach a
* splice_insert
SCTE-35 message to the ad break. This message provides basic metadata about the ad
* break.
*
*
* See section 9.7.4 of the 2022 SCTE-35 specification for more information.
*
*
* @return Defines the SCTE-35 time_signal
message inserted around the ad.
*
* Programs on a channel's schedule can be configured with one or more ad breaks. You can attach a
* splice_insert
SCTE-35 message to the ad break. This message provides basic metadata about
* the ad break.
*
*
* See section 9.7.4 of the 2022 SCTE-35 specification for more information.
*/
public TimeSignalMessage getTimeSignalMessage() {
return this.timeSignalMessage;
}
/**
*
* Defines the SCTE-35 time_signal
message inserted around the ad.
*
*
* Programs on a channel's schedule can be configured with one or more ad breaks. You can attach a
* splice_insert
SCTE-35 message to the ad break. This message provides basic metadata about the ad
* break.
*
*
* See section 9.7.4 of the 2022 SCTE-35 specification for more information.
*
*
* @param timeSignalMessage
* Defines the SCTE-35 time_signal
message inserted around the ad.
*
* Programs on a channel's schedule can be configured with one or more ad breaks. You can attach a
* splice_insert
SCTE-35 message to the ad break. This message provides basic metadata about the
* ad break.
*
*
* See section 9.7.4 of the 2022 SCTE-35 specification for more information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdBreak withTimeSignalMessage(TimeSignalMessage timeSignalMessage) {
setTimeSignalMessage(timeSignalMessage);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAdBreakMetadata() != null)
sb.append("AdBreakMetadata: ").append(getAdBreakMetadata()).append(",");
if (getMessageType() != null)
sb.append("MessageType: ").append(getMessageType()).append(",");
if (getOffsetMillis() != null)
sb.append("OffsetMillis: ").append(getOffsetMillis()).append(",");
if (getSlate() != null)
sb.append("Slate: ").append(getSlate()).append(",");
if (getSpliceInsertMessage() != null)
sb.append("SpliceInsertMessage: ").append(getSpliceInsertMessage()).append(",");
if (getTimeSignalMessage() != null)
sb.append("TimeSignalMessage: ").append(getTimeSignalMessage());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AdBreak == false)
return false;
AdBreak other = (AdBreak) obj;
if (other.getAdBreakMetadata() == null ^ this.getAdBreakMetadata() == null)
return false;
if (other.getAdBreakMetadata() != null && other.getAdBreakMetadata().equals(this.getAdBreakMetadata()) == false)
return false;
if (other.getMessageType() == null ^ this.getMessageType() == null)
return false;
if (other.getMessageType() != null && other.getMessageType().equals(this.getMessageType()) == false)
return false;
if (other.getOffsetMillis() == null ^ this.getOffsetMillis() == null)
return false;
if (other.getOffsetMillis() != null && other.getOffsetMillis().equals(this.getOffsetMillis()) == false)
return false;
if (other.getSlate() == null ^ this.getSlate() == null)
return false;
if (other.getSlate() != null && other.getSlate().equals(this.getSlate()) == false)
return false;
if (other.getSpliceInsertMessage() == null ^ this.getSpliceInsertMessage() == null)
return false;
if (other.getSpliceInsertMessage() != null && other.getSpliceInsertMessage().equals(this.getSpliceInsertMessage()) == false)
return false;
if (other.getTimeSignalMessage() == null ^ this.getTimeSignalMessage() == null)
return false;
if (other.getTimeSignalMessage() != null && other.getTimeSignalMessage().equals(this.getTimeSignalMessage()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAdBreakMetadata() == null) ? 0 : getAdBreakMetadata().hashCode());
hashCode = prime * hashCode + ((getMessageType() == null) ? 0 : getMessageType().hashCode());
hashCode = prime * hashCode + ((getOffsetMillis() == null) ? 0 : getOffsetMillis().hashCode());
hashCode = prime * hashCode + ((getSlate() == null) ? 0 : getSlate().hashCode());
hashCode = prime * hashCode + ((getSpliceInsertMessage() == null) ? 0 : getSpliceInsertMessage().hashCode());
hashCode = prime * hashCode + ((getTimeSignalMessage() == null) ? 0 : getTimeSignalMessage().hashCode());
return hashCode;
}
@Override
public AdBreak clone() {
try {
return (AdBreak) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.mediatailor.model.transform.AdBreakMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}