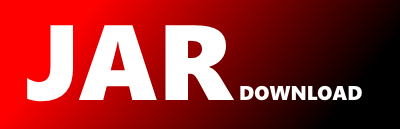
com.amazonaws.services.medicalimaging.AWSMedicalImagingClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-medicalimaging Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.medicalimaging;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.medicalimaging.AWSMedicalImagingClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.medicalimaging.model.*;
import com.amazonaws.services.medicalimaging.model.transform.*;
/**
* Client for accessing AWS Health Imaging. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* This is the AWS HealthImaging API Reference. AWS HealthImaging is a HIPAA eligible service that empowers
* healthcare providers, life science organizations, and their software partners to store, analyze, and share medical
* images in the cloud at petabyte scale. For an introduction to the service, see the AWS HealthImaging Developer
* Guide .
*
*
*
* We recommend using one of the AWS Software Development Kits (SDKs) for your programming language, as they take care
* of request authentication, serialization, and connection management. For more information, see Tools to build on AWS.
*
*
*
* The following sections list AWS HealthImaging API actions categorized according to functionality. Links are provided
* to actions within this Reference, along with links back to corresponding sections in the AWS HealthImaging
* Developer Guide where you can view tested code examples.
*
*
* Data store actions
*
*
* -
*
* CreateDatastore
* – See Creating a data
* store.
*
*
* -
*
* GetDatastore – See
* Getting data store
* properties.
*
*
* -
*
* ListDatastores –
* See Listing data
* stores.
*
*
* -
*
* DeleteDatastore
* – See Deleting a data
* store.
*
*
*
*
* Import job actions
*
*
* -
*
*
* StartDICOMImportJob – See Starting an import
* job.
*
*
* -
*
* GetDICOMImportJob
* – See Getting import
* job properties.
*
*
* -
*
*
* ListDICOMImportJobs – See Listing import jobs.
*
*
*
*
* Image set access actions
*
*
* -
*
* SearchImageSets
* – See Searching image
* sets.
*
*
* -
*
* GetImageSet – See Getting image set
* properties.
*
*
* -
*
*
* GetImageSetMetadata – See Getting image set
* metadata.
*
*
* -
*
* GetImageFrame –
* See Getting image set pixel
* data.
*
*
*
*
* Image set modification actions
*
*
* -
*
*
* ListImageSetVersions – See Listing image set
* versions.
*
*
* -
*
*
* UpdateImageSetMetadata – See Updating image set
* metadata.
*
*
* -
*
* CopyImageSet – See
* Copying an image set.
*
*
* -
*
* DeleteImageSet –
* See Deleting an image
* set.
*
*
*
*
* Tagging actions
*
*
* -
*
* TagResource – See Tagging a resource.
*
*
* -
*
*
* ListTagsForResource – See Listing tags for a
* resource.
*
*
* -
*
* UntagResource –
* See Untagging a resource.
*
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSMedicalImagingClient extends AmazonWebServiceClient implements AWSMedicalImaging {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSMedicalImaging.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "medical-imaging";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.medicalimaging.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.medicalimaging.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.medicalimaging.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.medicalimaging.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.medicalimaging.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.medicalimaging.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.medicalimaging.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.medicalimaging.model.AWSMedicalImagingException.class));
public static AWSMedicalImagingClientBuilder builder() {
return AWSMedicalImagingClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS Health Imaging using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSMedicalImagingClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS Health Imaging using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSMedicalImagingClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("medical-imaging.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/medicalimaging/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/medicalimaging/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Copy an image set.
*
*
* @param copyImageSetRequest
* @return Result of the CopyImageSet operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @sample AWSMedicalImaging.CopyImageSet
* @see AWS
* API Documentation
*/
@Override
public CopyImageSetResult copyImageSet(CopyImageSetRequest request) {
request = beforeClientExecution(request);
return executeCopyImageSet(request);
}
@SdkInternalApi
final CopyImageSetResult executeCopyImageSet(CopyImageSetRequest copyImageSetRequest) {
ExecutionContext executionContext = createExecutionContext(copyImageSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CopyImageSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(copyImageSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CopyImageSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "runtime-";
String resolvedHostPrefix = String.format("runtime-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CopyImageSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a data store.
*
*
* @param createDatastoreRequest
* @return Result of the CreateDatastore operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @sample AWSMedicalImaging.CreateDatastore
* @see AWS API Documentation
*/
@Override
public CreateDatastoreResult createDatastore(CreateDatastoreRequest request) {
request = beforeClientExecution(request);
return executeCreateDatastore(request);
}
@SdkInternalApi
final CreateDatastoreResult executeCreateDatastore(CreateDatastoreRequest createDatastoreRequest) {
ExecutionContext executionContext = createExecutionContext(createDatastoreRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDatastoreRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDatastoreRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDatastore");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDatastoreResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete a data store.
*
*
*
* Before a data store can be deleted, you must first delete all image sets within it.
*
*
*
* @param deleteDatastoreRequest
* @return Result of the DeleteDatastore operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.DeleteDatastore
* @see AWS API Documentation
*/
@Override
public DeleteDatastoreResult deleteDatastore(DeleteDatastoreRequest request) {
request = beforeClientExecution(request);
return executeDeleteDatastore(request);
}
@SdkInternalApi
final DeleteDatastoreResult executeDeleteDatastore(DeleteDatastoreRequest deleteDatastoreRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDatastoreRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDatastoreRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDatastoreRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDatastore");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDatastoreResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an image set.
*
*
* @param deleteImageSetRequest
* @return Result of the DeleteImageSet operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.DeleteImageSet
* @see AWS
* API Documentation
*/
@Override
public DeleteImageSetResult deleteImageSet(DeleteImageSetRequest request) {
request = beforeClientExecution(request);
return executeDeleteImageSet(request);
}
@SdkInternalApi
final DeleteImageSetResult executeDeleteImageSet(DeleteImageSetRequest deleteImageSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteImageSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteImageSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteImageSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteImageSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "runtime-";
String resolvedHostPrefix = String.format("runtime-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteImageSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the import job properties to learn more about the job or job progress.
*
*
*
* The jobStatus
refers to the execution of the import job. Therefore, an import job can return a
* jobStatus
as COMPLETED
even if validation issues are discovered during the import
* process. If a jobStatus
returns as COMPLETED
, we still recommend you review the output
* manifests written to S3, as they provide details on the success or failure of individual P10 object imports.
*
*
*
* @param getDICOMImportJobRequest
* @return Result of the GetDICOMImportJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.GetDICOMImportJob
* @see AWS API Documentation
*/
@Override
public GetDICOMImportJobResult getDICOMImportJob(GetDICOMImportJobRequest request) {
request = beforeClientExecution(request);
return executeGetDICOMImportJob(request);
}
@SdkInternalApi
final GetDICOMImportJobResult executeGetDICOMImportJob(GetDICOMImportJobRequest getDICOMImportJobRequest) {
ExecutionContext executionContext = createExecutionContext(getDICOMImportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDICOMImportJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDICOMImportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDICOMImportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDICOMImportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get data store properties.
*
*
* @param getDatastoreRequest
* @return Result of the GetDatastore operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.GetDatastore
* @see AWS
* API Documentation
*/
@Override
public GetDatastoreResult getDatastore(GetDatastoreRequest request) {
request = beforeClientExecution(request);
return executeGetDatastore(request);
}
@SdkInternalApi
final GetDatastoreResult executeGetDatastore(GetDatastoreRequest getDatastoreRequest) {
ExecutionContext executionContext = createExecutionContext(getDatastoreRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDatastoreRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDatastoreRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDatastore");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDatastoreResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
* @param getImageFrameRequest
* @return Result of the GetImageFrame operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.GetImageFrame
* @see AWS
* API Documentation
*/
@Override
public GetImageFrameResult getImageFrame(GetImageFrameRequest request) {
request = beforeClientExecution(request);
return executeGetImageFrame(request);
}
@SdkInternalApi
final GetImageFrameResult executeGetImageFrame(GetImageFrameRequest getImageFrameRequest) {
ExecutionContext executionContext = createExecutionContext(getImageFrameRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetImageFrameRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getImageFrameRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetImageFrame");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "runtime-";
String resolvedHostPrefix = String.format("runtime-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(false).withHasStreamingSuccessResponse(true), new GetImageFrameResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
request.addHandlerContext(HandlerContextKey.HAS_STREAMING_OUTPUT, Boolean.TRUE);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get image set properties.
*
*
* @param getImageSetRequest
* @return Result of the GetImageSet operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.GetImageSet
* @see AWS
* API Documentation
*/
@Override
public GetImageSetResult getImageSet(GetImageSetRequest request) {
request = beforeClientExecution(request);
return executeGetImageSet(request);
}
@SdkInternalApi
final GetImageSetResult executeGetImageSet(GetImageSetRequest getImageSetRequest) {
ExecutionContext executionContext = createExecutionContext(getImageSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetImageSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getImageSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetImageSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "runtime-";
String resolvedHostPrefix = String.format("runtime-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetImageSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get metadata attributes for an image set.
*
*
* @param getImageSetMetadataRequest
* @return Result of the GetImageSetMetadata operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.GetImageSetMetadata
* @see AWS API Documentation
*/
@Override
public GetImageSetMetadataResult getImageSetMetadata(GetImageSetMetadataRequest request) {
request = beforeClientExecution(request);
return executeGetImageSetMetadata(request);
}
@SdkInternalApi
final GetImageSetMetadataResult executeGetImageSetMetadata(GetImageSetMetadataRequest getImageSetMetadataRequest) {
ExecutionContext executionContext = createExecutionContext(getImageSetMetadataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetImageSetMetadataRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getImageSetMetadataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetImageSetMetadata");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "runtime-";
String resolvedHostPrefix = String.format("runtime-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(false).withHasStreamingSuccessResponse(true), new GetImageSetMetadataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
request.addHandlerContext(HandlerContextKey.HAS_STREAMING_OUTPUT, Boolean.TRUE);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List import jobs created for a specific data store.
*
*
* @param listDICOMImportJobsRequest
* @return Result of the ListDICOMImportJobs operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.ListDICOMImportJobs
* @see AWS API Documentation
*/
@Override
public ListDICOMImportJobsResult listDICOMImportJobs(ListDICOMImportJobsRequest request) {
request = beforeClientExecution(request);
return executeListDICOMImportJobs(request);
}
@SdkInternalApi
final ListDICOMImportJobsResult executeListDICOMImportJobs(ListDICOMImportJobsRequest listDICOMImportJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listDICOMImportJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDICOMImportJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDICOMImportJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDICOMImportJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDICOMImportJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List data stores.
*
*
* @param listDatastoresRequest
* @return Result of the ListDatastores operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @sample AWSMedicalImaging.ListDatastores
* @see AWS
* API Documentation
*/
@Override
public ListDatastoresResult listDatastores(ListDatastoresRequest request) {
request = beforeClientExecution(request);
return executeListDatastores(request);
}
@SdkInternalApi
final ListDatastoresResult executeListDatastores(ListDatastoresRequest listDatastoresRequest) {
ExecutionContext executionContext = createExecutionContext(listDatastoresRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDatastoresRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDatastoresRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDatastores");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDatastoresResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List image set versions.
*
*
* @param listImageSetVersionsRequest
* @return Result of the ListImageSetVersions operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.ListImageSetVersions
* @see AWS API Documentation
*/
@Override
public ListImageSetVersionsResult listImageSetVersions(ListImageSetVersionsRequest request) {
request = beforeClientExecution(request);
return executeListImageSetVersions(request);
}
@SdkInternalApi
final ListImageSetVersionsResult executeListImageSetVersions(ListImageSetVersionsRequest listImageSetVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(listImageSetVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListImageSetVersionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listImageSetVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListImageSetVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "runtime-";
String resolvedHostPrefix = String.format("runtime-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListImageSetVersionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all tags associated with a medical imaging resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Search image sets based on defined input attributes.
*
*
*
* SearchImageSets
accepts a single search query parameter and returns a paginated response of all
* image sets that have the matching criteria. All date range queries must be input as
* (lowerBound, upperBound)
.
*
*
* By default, SearchImageSets
uses the updatedAt
field for sorting in descending order
* from newest to oldest.
*
*
*
* @param searchImageSetsRequest
* @return Result of the SearchImageSets operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.SearchImageSets
* @see AWS API Documentation
*/
@Override
public SearchImageSetsResult searchImageSets(SearchImageSetsRequest request) {
request = beforeClientExecution(request);
return executeSearchImageSets(request);
}
@SdkInternalApi
final SearchImageSetsResult executeSearchImageSets(SearchImageSetsRequest searchImageSetsRequest) {
ExecutionContext executionContext = createExecutionContext(searchImageSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchImageSetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(searchImageSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchImageSets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "runtime-";
String resolvedHostPrefix = String.format("runtime-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new SearchImageSetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Start importing bulk data into an ACTIVE
data store. The import job imports DICOM P10 files found in
* the S3 prefix specified by the inputS3Uri
parameter. The import job stores processing results in the
* file specified by the outputS3Uri
parameter.
*
*
* @param startDICOMImportJobRequest
* @return Result of the StartDICOMImportJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @sample AWSMedicalImaging.StartDICOMImportJob
* @see AWS API Documentation
*/
@Override
public StartDICOMImportJobResult startDICOMImportJob(StartDICOMImportJobRequest request) {
request = beforeClientExecution(request);
return executeStartDICOMImportJob(request);
}
@SdkInternalApi
final StartDICOMImportJobResult executeStartDICOMImportJob(StartDICOMImportJobRequest startDICOMImportJobRequest) {
ExecutionContext executionContext = createExecutionContext(startDICOMImportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartDICOMImportJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startDICOMImportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartDICOMImportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartDICOMImportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a user-specifed key and value tag to a medical imaging resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.TagResource
* @see AWS
* API Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes tags from a medical imaging resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @sample AWSMedicalImaging.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update image set metadata attributes.
*
*
* @param updateImageSetMetadataRequest
* @return Result of the UpdateImageSetMetadata operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints set by the service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @sample AWSMedicalImaging.UpdateImageSetMetadata
* @see AWS API Documentation
*/
@Override
public UpdateImageSetMetadataResult updateImageSetMetadata(UpdateImageSetMetadataRequest request) {
request = beforeClientExecution(request);
return executeUpdateImageSetMetadata(request);
}
@SdkInternalApi
final UpdateImageSetMetadataResult executeUpdateImageSetMetadata(UpdateImageSetMetadataRequest updateImageSetMetadataRequest) {
ExecutionContext executionContext = createExecutionContext(updateImageSetMetadataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateImageSetMetadataRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateImageSetMetadataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Medical Imaging");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateImageSetMetadata");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "runtime-";
String resolvedHostPrefix = String.format("runtime-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateImageSetMetadataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}