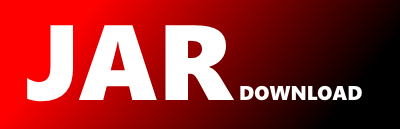
com.amazonaws.services.medicalimaging.AWSMedicalImagingAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-medicalimaging Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.medicalimaging;
import javax.annotation.Generated;
import com.amazonaws.services.medicalimaging.model.*;
/**
* Interface for accessing AWS Health Imaging asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.medicalimaging.AbstractAWSMedicalImagingAsync} instead.
*
*
*
* This is the AWS HealthImaging API Reference. AWS HealthImaging is a HIPAA eligible service that empowers
* healthcare providers, life science organizations, and their software partners to store, analyze, and share medical
* images in the cloud at petabyte scale. For an introduction to the service, see the AWS HealthImaging Developer
* Guide .
*
*
*
* We recommend using one of the AWS Software Development Kits (SDKs) for your programming language, as they take care
* of request authentication, serialization, and connection management. For more information, see Tools to build on AWS.
*
*
*
* The following sections list AWS HealthImaging API actions categorized according to functionality. Links are provided
* to actions within this Reference, along with links back to corresponding sections in the AWS HealthImaging
* Developer Guide where you can view tested code examples.
*
*
* Data store actions
*
*
* -
*
* CreateDatastore
* – See Creating a data
* store.
*
*
* -
*
* GetDatastore – See
* Getting data store
* properties.
*
*
* -
*
* ListDatastores –
* See Listing data
* stores.
*
*
* -
*
* DeleteDatastore
* – See Deleting a data
* store.
*
*
*
*
* Import job actions
*
*
* -
*
*
* StartDICOMImportJob – See Starting an import
* job.
*
*
* -
*
* GetDICOMImportJob
* – See Getting import
* job properties.
*
*
* -
*
*
* ListDICOMImportJobs – See Listing import jobs.
*
*
*
*
* Image set access actions
*
*
* -
*
* SearchImageSets
* – See Searching image
* sets.
*
*
* -
*
* GetImageSet – See Getting image set
* properties.
*
*
* -
*
*
* GetImageSetMetadata – See Getting image set
* metadata.
*
*
* -
*
* GetImageFrame –
* See Getting image set pixel
* data.
*
*
*
*
* Image set modification actions
*
*
* -
*
*
* ListImageSetVersions – See Listing image set
* versions.
*
*
* -
*
*
* UpdateImageSetMetadata – See Updating image set
* metadata.
*
*
* -
*
* CopyImageSet – See
* Copying an image set.
*
*
* -
*
* DeleteImageSet –
* See Deleting an image
* set.
*
*
*
*
* Tagging actions
*
*
* -
*
* TagResource – See Tagging a resource.
*
*
* -
*
*
* ListTagsForResource – See Listing tags for a
* resource.
*
*
* -
*
* UntagResource –
* See Untagging a resource.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMedicalImagingAsync extends AWSMedicalImaging {
/**
*
* Copy an image set.
*
*
* @param copyImageSetRequest
* @return A Java Future containing the result of the CopyImageSet operation returned by the service.
* @sample AWSMedicalImagingAsync.CopyImageSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyImageSetAsync(CopyImageSetRequest copyImageSetRequest);
/**
*
* Copy an image set.
*
*
* @param copyImageSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyImageSet operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.CopyImageSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyImageSetAsync(CopyImageSetRequest copyImageSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a data store.
*
*
* @param createDatastoreRequest
* @return A Java Future containing the result of the CreateDatastore operation returned by the service.
* @sample AWSMedicalImagingAsync.CreateDatastore
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatastoreAsync(CreateDatastoreRequest createDatastoreRequest);
/**
*
* Create a data store.
*
*
* @param createDatastoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDatastore operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.CreateDatastore
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatastoreAsync(CreateDatastoreRequest createDatastoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a data store.
*
*
*
* Before a data store can be deleted, you must first delete all image sets within it.
*
*
*
* @param deleteDatastoreRequest
* @return A Java Future containing the result of the DeleteDatastore operation returned by the service.
* @sample AWSMedicalImagingAsync.DeleteDatastore
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatastoreAsync(DeleteDatastoreRequest deleteDatastoreRequest);
/**
*
* Delete a data store.
*
*
*
* Before a data store can be deleted, you must first delete all image sets within it.
*
*
*
* @param deleteDatastoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDatastore operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.DeleteDatastore
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatastoreAsync(DeleteDatastoreRequest deleteDatastoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an image set.
*
*
* @param deleteImageSetRequest
* @return A Java Future containing the result of the DeleteImageSet operation returned by the service.
* @sample AWSMedicalImagingAsync.DeleteImageSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteImageSetAsync(DeleteImageSetRequest deleteImageSetRequest);
/**
*
* Delete an image set.
*
*
* @param deleteImageSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteImageSet operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.DeleteImageSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteImageSetAsync(DeleteImageSetRequest deleteImageSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the import job properties to learn more about the job or job progress.
*
*
*
* The jobStatus
refers to the execution of the import job. Therefore, an import job can return a
* jobStatus
as COMPLETED
even if validation issues are discovered during the import
* process. If a jobStatus
returns as COMPLETED
, we still recommend you review the output
* manifests written to S3, as they provide details on the success or failure of individual P10 object imports.
*
*
*
* @param getDICOMImportJobRequest
* @return A Java Future containing the result of the GetDICOMImportJob operation returned by the service.
* @sample AWSMedicalImagingAsync.GetDICOMImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getDICOMImportJobAsync(GetDICOMImportJobRequest getDICOMImportJobRequest);
/**
*
* Get the import job properties to learn more about the job or job progress.
*
*
*
* The jobStatus
refers to the execution of the import job. Therefore, an import job can return a
* jobStatus
as COMPLETED
even if validation issues are discovered during the import
* process. If a jobStatus
returns as COMPLETED
, we still recommend you review the output
* manifests written to S3, as they provide details on the success or failure of individual P10 object imports.
*
*
*
* @param getDICOMImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDICOMImportJob operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.GetDICOMImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getDICOMImportJobAsync(GetDICOMImportJobRequest getDICOMImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get data store properties.
*
*
* @param getDatastoreRequest
* @return A Java Future containing the result of the GetDatastore operation returned by the service.
* @sample AWSMedicalImagingAsync.GetDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDatastoreAsync(GetDatastoreRequest getDatastoreRequest);
/**
*
* Get data store properties.
*
*
* @param getDatastoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDatastore operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.GetDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDatastoreAsync(GetDatastoreRequest getDatastoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get an image frame (pixel data) for an image set.
*
*
* @param getImageFrameRequest
* @return A Java Future containing the result of the GetImageFrame operation returned by the service.
* @sample AWSMedicalImagingAsync.GetImageFrame
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getImageFrameAsync(GetImageFrameRequest getImageFrameRequest);
/**
*
* Get an image frame (pixel data) for an image set.
*
*
* @param getImageFrameRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetImageFrame operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.GetImageFrame
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getImageFrameAsync(GetImageFrameRequest getImageFrameRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get image set properties.
*
*
* @param getImageSetRequest
* @return A Java Future containing the result of the GetImageSet operation returned by the service.
* @sample AWSMedicalImagingAsync.GetImageSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getImageSetAsync(GetImageSetRequest getImageSetRequest);
/**
*
* Get image set properties.
*
*
* @param getImageSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetImageSet operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.GetImageSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getImageSetAsync(GetImageSetRequest getImageSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get metadata attributes for an image set.
*
*
* @param getImageSetMetadataRequest
* @return A Java Future containing the result of the GetImageSetMetadata operation returned by the service.
* @sample AWSMedicalImagingAsync.GetImageSetMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getImageSetMetadataAsync(GetImageSetMetadataRequest getImageSetMetadataRequest);
/**
*
* Get metadata attributes for an image set.
*
*
* @param getImageSetMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetImageSetMetadata operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.GetImageSetMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getImageSetMetadataAsync(GetImageSetMetadataRequest getImageSetMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List import jobs created for a specific data store.
*
*
* @param listDICOMImportJobsRequest
* @return A Java Future containing the result of the ListDICOMImportJobs operation returned by the service.
* @sample AWSMedicalImagingAsync.ListDICOMImportJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listDICOMImportJobsAsync(ListDICOMImportJobsRequest listDICOMImportJobsRequest);
/**
*
* List import jobs created for a specific data store.
*
*
* @param listDICOMImportJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDICOMImportJobs operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.ListDICOMImportJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listDICOMImportJobsAsync(ListDICOMImportJobsRequest listDICOMImportJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List data stores.
*
*
* @param listDatastoresRequest
* @return A Java Future containing the result of the ListDatastores operation returned by the service.
* @sample AWSMedicalImagingAsync.ListDatastores
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDatastoresAsync(ListDatastoresRequest listDatastoresRequest);
/**
*
* List data stores.
*
*
* @param listDatastoresRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDatastores operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.ListDatastores
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDatastoresAsync(ListDatastoresRequest listDatastoresRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List image set versions.
*
*
* @param listImageSetVersionsRequest
* @return A Java Future containing the result of the ListImageSetVersions operation returned by the service.
* @sample AWSMedicalImagingAsync.ListImageSetVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listImageSetVersionsAsync(ListImageSetVersionsRequest listImageSetVersionsRequest);
/**
*
* List image set versions.
*
*
* @param listImageSetVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListImageSetVersions operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.ListImageSetVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listImageSetVersionsAsync(ListImageSetVersionsRequest listImageSetVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags associated with a medical imaging resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMedicalImagingAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all tags associated with a medical imaging resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Search image sets based on defined input attributes.
*
*
*
* SearchImageSets
accepts a single search query parameter and returns a paginated response of all
* image sets that have the matching criteria. All date range queries must be input as
* (lowerBound, upperBound)
.
*
*
* By default, SearchImageSets
uses the updatedAt
field for sorting in descending order
* from newest to oldest.
*
*
*
* @param searchImageSetsRequest
* @return A Java Future containing the result of the SearchImageSets operation returned by the service.
* @sample AWSMedicalImagingAsync.SearchImageSets
* @see AWS API Documentation
*/
java.util.concurrent.Future searchImageSetsAsync(SearchImageSetsRequest searchImageSetsRequest);
/**
*
* Search image sets based on defined input attributes.
*
*
*
* SearchImageSets
accepts a single search query parameter and returns a paginated response of all
* image sets that have the matching criteria. All date range queries must be input as
* (lowerBound, upperBound)
.
*
*
* By default, SearchImageSets
uses the updatedAt
field for sorting in descending order
* from newest to oldest.
*
*
*
* @param searchImageSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchImageSets operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.SearchImageSets
* @see AWS API Documentation
*/
java.util.concurrent.Future searchImageSetsAsync(SearchImageSetsRequest searchImageSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Start importing bulk data into an ACTIVE
data store. The import job imports DICOM P10 files found in
* the S3 prefix specified by the inputS3Uri
parameter. The import job stores processing results in the
* file specified by the outputS3Uri
parameter.
*
*
* @param startDICOMImportJobRequest
* @return A Java Future containing the result of the StartDICOMImportJob operation returned by the service.
* @sample AWSMedicalImagingAsync.StartDICOMImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startDICOMImportJobAsync(StartDICOMImportJobRequest startDICOMImportJobRequest);
/**
*
* Start importing bulk data into an ACTIVE
data store. The import job imports DICOM P10 files found in
* the S3 prefix specified by the inputS3Uri
parameter. The import job stores processing results in the
* file specified by the outputS3Uri
parameter.
*
*
* @param startDICOMImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartDICOMImportJob operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.StartDICOMImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startDICOMImportJobAsync(StartDICOMImportJobRequest startDICOMImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a user-specifed key and value tag to a medical imaging resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMedicalImagingAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds a user-specifed key and value tag to a medical imaging resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from a medical imaging resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMedicalImagingAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from a medical imaging resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update image set metadata attributes.
*
*
* @param updateImageSetMetadataRequest
* @return A Java Future containing the result of the UpdateImageSetMetadata operation returned by the service.
* @sample AWSMedicalImagingAsync.UpdateImageSetMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future updateImageSetMetadataAsync(UpdateImageSetMetadataRequest updateImageSetMetadataRequest);
/**
*
* Update image set metadata attributes.
*
*
* @param updateImageSetMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateImageSetMetadata operation returned by the service.
* @sample AWSMedicalImagingAsyncHandler.UpdateImageSetMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future updateImageSetMetadataAsync(UpdateImageSetMetadataRequest updateImageSetMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}