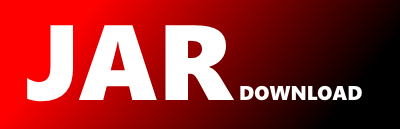
com.amazonaws.services.memorydb.AmazonMemoryDBAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-memorydb Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.memorydb;
import javax.annotation.Generated;
import com.amazonaws.services.memorydb.model.*;
/**
* Interface for accessing Amazon MemoryDB asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.memorydb.AbstractAmazonMemoryDBAsync} instead.
*
*
*
* MemoryDB is a fully managed, Redis OSS-compatible, in-memory database that delivers ultra-fast performance and
* Multi-AZ durability for modern applications built using microservices architectures. MemoryDB stores the entire
* database in-memory, enabling low latency and high throughput data access. It is compatible with Redis OSS, a popular
* open source data store, enabling you to leverage Redis OSS’ flexible and friendly data structures, APIs, and
* commands.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonMemoryDBAsync extends AmazonMemoryDB {
/**
*
* Apply the service update to a list of clusters supplied. For more information on service updates and applying
* them, see Applying the
* service updates.
*
*
* @param batchUpdateClusterRequest
* @return A Java Future containing the result of the BatchUpdateCluster operation returned by the service.
* @sample AmazonMemoryDBAsync.BatchUpdateCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchUpdateClusterAsync(BatchUpdateClusterRequest batchUpdateClusterRequest);
/**
*
* Apply the service update to a list of clusters supplied. For more information on service updates and applying
* them, see Applying the
* service updates.
*
*
* @param batchUpdateClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUpdateCluster operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.BatchUpdateCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchUpdateClusterAsync(BatchUpdateClusterRequest batchUpdateClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Makes a copy of an existing snapshot.
*
*
* @param copySnapshotRequest
* @return A Java Future containing the result of the CopySnapshot operation returned by the service.
* @sample AmazonMemoryDBAsync.CopySnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copySnapshotAsync(CopySnapshotRequest copySnapshotRequest);
/**
*
* Makes a copy of an existing snapshot.
*
*
* @param copySnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopySnapshot operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.CopySnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copySnapshotAsync(CopySnapshotRequest copySnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Access Control List. For more information, see Authenticating users with Access
* Contol Lists (ACLs).
*
*
* @param createACLRequest
* @return A Java Future containing the result of the CreateACL operation returned by the service.
* @sample AmazonMemoryDBAsync.CreateACL
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createACLAsync(CreateACLRequest createACLRequest);
/**
*
* Creates an Access Control List. For more information, see Authenticating users with Access
* Contol Lists (ACLs).
*
*
* @param createACLRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateACL operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.CreateACL
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createACLAsync(CreateACLRequest createACLRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a cluster. All nodes in the cluster run the same protocol-compliant engine software.
*
*
* @param createClusterRequest
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* @sample AmazonMemoryDBAsync.CreateCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createClusterAsync(CreateClusterRequest createClusterRequest);
/**
*
* Creates a cluster. All nodes in the cluster run the same protocol-compliant engine software.
*
*
* @param createClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.CreateCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createClusterAsync(CreateClusterRequest createClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new MemoryDB parameter group. A parameter group is a collection of parameters and their values that are
* applied to all of the nodes in any cluster. For more information, see Configuring engine parameters
* using parameter groups.
*
*
* @param createParameterGroupRequest
* @return A Java Future containing the result of the CreateParameterGroup operation returned by the service.
* @sample AmazonMemoryDBAsync.CreateParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createParameterGroupAsync(CreateParameterGroupRequest createParameterGroupRequest);
/**
*
* Creates a new MemoryDB parameter group. A parameter group is a collection of parameters and their values that are
* applied to all of the nodes in any cluster. For more information, see Configuring engine parameters
* using parameter groups.
*
*
* @param createParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateParameterGroup operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.CreateParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createParameterGroupAsync(CreateParameterGroupRequest createParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a copy of an entire cluster at a specific moment in time.
*
*
* @param createSnapshotRequest
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AmazonMemoryDBAsync.CreateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest);
/**
*
* Creates a copy of an entire cluster at a specific moment in time.
*
*
* @param createSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.CreateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a subnet group. A subnet group is a collection of subnets (typically private) that you can designate for
* your clusters running in an Amazon Virtual Private Cloud (VPC) environment. When you create a cluster in an
* Amazon VPC, you must specify a subnet group. MemoryDB uses that subnet group to choose a subnet and IP addresses
* within that subnet to associate with your nodes. For more information, see Subnets and subnet groups.
*
*
* @param createSubnetGroupRequest
* @return A Java Future containing the result of the CreateSubnetGroup operation returned by the service.
* @sample AmazonMemoryDBAsync.CreateSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSubnetGroupAsync(CreateSubnetGroupRequest createSubnetGroupRequest);
/**
*
* Creates a subnet group. A subnet group is a collection of subnets (typically private) that you can designate for
* your clusters running in an Amazon Virtual Private Cloud (VPC) environment. When you create a cluster in an
* Amazon VPC, you must specify a subnet group. MemoryDB uses that subnet group to choose a subnet and IP addresses
* within that subnet to associate with your nodes. For more information, see Subnets and subnet groups.
*
*
* @param createSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSubnetGroup operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.CreateSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSubnetGroupAsync(CreateSubnetGroupRequest createSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a MemoryDB user. For more information, see Authenticating users with Access
* Contol Lists (ACLs).
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* @sample AmazonMemoryDBAsync.CreateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUserAsync(CreateUserRequest createUserRequest);
/**
*
* Creates a MemoryDB user. For more information, see Authenticating users with Access
* Contol Lists (ACLs).
*
*
* @param createUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.CreateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUserAsync(CreateUserRequest createUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Access Control List. The ACL must first be disassociated from the cluster before it can be deleted.
* For more information, see Authenticating users with Access
* Contol Lists (ACLs).
*
*
* @param deleteACLRequest
* @return A Java Future containing the result of the DeleteACL operation returned by the service.
* @sample AmazonMemoryDBAsync.DeleteACL
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteACLAsync(DeleteACLRequest deleteACLRequest);
/**
*
* Deletes an Access Control List. The ACL must first be disassociated from the cluster before it can be deleted.
* For more information, see Authenticating users with Access
* Contol Lists (ACLs).
*
*
* @param deleteACLRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteACL operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DeleteACL
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteACLAsync(DeleteACLRequest deleteACLRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a cluster. It also deletes all associated nodes and node endpoints
*
*
* @param deleteClusterRequest
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* @sample AmazonMemoryDBAsync.DeleteCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteClusterAsync(DeleteClusterRequest deleteClusterRequest);
/**
*
* Deletes a cluster. It also deletes all associated nodes and node endpoints
*
*
* @param deleteClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DeleteCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteClusterAsync(DeleteClusterRequest deleteClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified parameter group. You cannot delete a parameter group if it is associated with any clusters.
* You cannot delete the default parameter groups in your account.
*
*
* @param deleteParameterGroupRequest
* @return A Java Future containing the result of the DeleteParameterGroup operation returned by the service.
* @sample AmazonMemoryDBAsync.DeleteParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteParameterGroupAsync(DeleteParameterGroupRequest deleteParameterGroupRequest);
/**
*
* Deletes the specified parameter group. You cannot delete a parameter group if it is associated with any clusters.
* You cannot delete the default parameter groups in your account.
*
*
* @param deleteParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteParameterGroup operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DeleteParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteParameterGroupAsync(DeleteParameterGroupRequest deleteParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing snapshot. When you receive a successful response from this operation, MemoryDB immediately
* begins deleting the snapshot; you cannot cancel or revert this operation.
*
*
* @param deleteSnapshotRequest
* @return A Java Future containing the result of the DeleteSnapshot operation returned by the service.
* @sample AmazonMemoryDBAsync.DeleteSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSnapshotAsync(DeleteSnapshotRequest deleteSnapshotRequest);
/**
*
* Deletes an existing snapshot. When you receive a successful response from this operation, MemoryDB immediately
* begins deleting the snapshot; you cannot cancel or revert this operation.
*
*
* @param deleteSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSnapshot operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DeleteSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSnapshotAsync(DeleteSnapshotRequest deleteSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a subnet group. You cannot delete a default subnet group or one that is associated with any clusters.
*
*
* @param deleteSubnetGroupRequest
* @return A Java Future containing the result of the DeleteSubnetGroup operation returned by the service.
* @sample AmazonMemoryDBAsync.DeleteSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSubnetGroupAsync(DeleteSubnetGroupRequest deleteSubnetGroupRequest);
/**
*
* Deletes a subnet group. You cannot delete a default subnet group or one that is associated with any clusters.
*
*
* @param deleteSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSubnetGroup operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DeleteSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSubnetGroupAsync(DeleteSubnetGroupRequest deleteSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a user. The user will be removed from all ACLs and in turn removed from all clusters.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* @sample AmazonMemoryDBAsync.DeleteUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUserAsync(DeleteUserRequest deleteUserRequest);
/**
*
* Deletes a user. The user will be removed from all ACLs and in turn removed from all clusters.
*
*
* @param deleteUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DeleteUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUserAsync(DeleteUserRequest deleteUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of ACLs
*
*
* @param describeACLsRequest
* @return A Java Future containing the result of the DescribeACLs operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeACLs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeACLsAsync(DescribeACLsRequest describeACLsRequest);
/**
*
* Returns a list of ACLs
*
*
* @param describeACLsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeACLs operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeACLs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeACLsAsync(DescribeACLsRequest describeACLsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about all provisioned clusters if no cluster identifier is specified, or about a specific
* cluster if a cluster name is supplied.
*
*
* @param describeClustersRequest
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeClusters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeClustersAsync(DescribeClustersRequest describeClustersRequest);
/**
*
* Returns information about all provisioned clusters if no cluster identifier is specified, or about a specific
* cluster if a cluster name is supplied.
*
*
* @param describeClustersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeClusters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeClustersAsync(DescribeClustersRequest describeClustersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the available Redis OSS engine versions.
*
*
* @param describeEngineVersionsRequest
* @return A Java Future containing the result of the DescribeEngineVersions operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeEngineVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEngineVersionsAsync(DescribeEngineVersionsRequest describeEngineVersionsRequest);
/**
*
* Returns a list of the available Redis OSS engine versions.
*
*
* @param describeEngineVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEngineVersions operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeEngineVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEngineVersionsAsync(DescribeEngineVersionsRequest describeEngineVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns events related to clusters, security groups, and parameter groups. You can obtain events specific to a
* particular cluster, security group, or parameter group by providing the name as a parameter. By default, only the
* events occurring within the last hour are returned; however, you can retrieve up to 14 days' worth of events if
* necessary.
*
*
* @param describeEventsRequest
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest);
/**
*
* Returns events related to clusters, security groups, and parameter groups. You can obtain events specific to a
* particular cluster, security group, or parameter group by providing the name as a parameter. By default, only the
* events occurring within the last hour are returned; however, you can retrieve up to 14 days' worth of events if
* necessary.
*
*
* @param describeEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of parameter group descriptions. If a parameter group name is specified, the list contains only
* the descriptions for that group.
*
*
* @param describeParameterGroupsRequest
* @return A Java Future containing the result of the DescribeParameterGroups operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeParameterGroupsAsync(DescribeParameterGroupsRequest describeParameterGroupsRequest);
/**
*
* Returns a list of parameter group descriptions. If a parameter group name is specified, the list contains only
* the descriptions for that group.
*
*
* @param describeParameterGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeParameterGroups operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeParameterGroupsAsync(DescribeParameterGroupsRequest describeParameterGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the detailed parameter list for a particular parameter group.
*
*
* @param describeParametersRequest
* @return A Java Future containing the result of the DescribeParameters operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeParameters
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeParametersAsync(DescribeParametersRequest describeParametersRequest);
/**
*
* Returns the detailed parameter list for a particular parameter group.
*
*
* @param describeParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeParameters operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeParameters
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeParametersAsync(DescribeParametersRequest describeParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about reserved nodes for this account, or about a specified reserved node.
*
*
* @param describeReservedNodesRequest
* @return A Java Future containing the result of the DescribeReservedNodes operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeReservedNodes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeReservedNodesAsync(DescribeReservedNodesRequest describeReservedNodesRequest);
/**
*
* Returns information about reserved nodes for this account, or about a specified reserved node.
*
*
* @param describeReservedNodesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReservedNodes operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeReservedNodes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeReservedNodesAsync(DescribeReservedNodesRequest describeReservedNodesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists available reserved node offerings.
*
*
* @param describeReservedNodesOfferingsRequest
* @return A Java Future containing the result of the DescribeReservedNodesOfferings operation returned by the
* service.
* @sample AmazonMemoryDBAsync.DescribeReservedNodesOfferings
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReservedNodesOfferingsAsync(
DescribeReservedNodesOfferingsRequest describeReservedNodesOfferingsRequest);
/**
*
* Lists available reserved node offerings.
*
*
* @param describeReservedNodesOfferingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReservedNodesOfferings operation returned by the
* service.
* @sample AmazonMemoryDBAsyncHandler.DescribeReservedNodesOfferings
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReservedNodesOfferingsAsync(
DescribeReservedNodesOfferingsRequest describeReservedNodesOfferingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns details of the service updates
*
*
* @param describeServiceUpdatesRequest
* @return A Java Future containing the result of the DescribeServiceUpdates operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeServiceUpdates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeServiceUpdatesAsync(DescribeServiceUpdatesRequest describeServiceUpdatesRequest);
/**
*
* Returns details of the service updates
*
*
* @param describeServiceUpdatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeServiceUpdates operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeServiceUpdates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeServiceUpdatesAsync(DescribeServiceUpdatesRequest describeServiceUpdatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about cluster snapshots. By default, DescribeSnapshots lists all of your snapshots; it can
* optionally describe a single snapshot, or just the snapshots associated with a particular cluster.
*
*
* @param describeSnapshotsRequest
* @return A Java Future containing the result of the DescribeSnapshots operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSnapshotsAsync(DescribeSnapshotsRequest describeSnapshotsRequest);
/**
*
* Returns information about cluster snapshots. By default, DescribeSnapshots lists all of your snapshots; it can
* optionally describe a single snapshot, or just the snapshots associated with a particular cluster.
*
*
* @param describeSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSnapshots operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSnapshotsAsync(DescribeSnapshotsRequest describeSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of subnet group descriptions. If a subnet group name is specified, the list contains only the
* description of that group.
*
*
* @param describeSubnetGroupsRequest
* @return A Java Future containing the result of the DescribeSubnetGroups operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeSubnetGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeSubnetGroupsAsync(DescribeSubnetGroupsRequest describeSubnetGroupsRequest);
/**
*
* Returns a list of subnet group descriptions. If a subnet group name is specified, the list contains only the
* description of that group.
*
*
* @param describeSubnetGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSubnetGroups operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeSubnetGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeSubnetGroupsAsync(DescribeSubnetGroupsRequest describeSubnetGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of users.
*
*
* @param describeUsersRequest
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* @sample AmazonMemoryDBAsync.DescribeUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeUsersAsync(DescribeUsersRequest describeUsersRequest);
/**
*
* Returns a list of users.
*
*
* @param describeUsersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.DescribeUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeUsersAsync(DescribeUsersRequest describeUsersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to failover a shard. This API is designed for testing the behavior of your application in case of MemoryDB
* failover. It is not designed to be used as a production-level tool for initiating a failover to overcome a
* problem you may have with the cluster. Moreover, in certain conditions such as large scale operational events,
* Amazon may block this API.
*
*
* @param failoverShardRequest
* @return A Java Future containing the result of the FailoverShard operation returned by the service.
* @sample AmazonMemoryDBAsync.FailoverShard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future failoverShardAsync(FailoverShardRequest failoverShardRequest);
/**
*
* Used to failover a shard. This API is designed for testing the behavior of your application in case of MemoryDB
* failover. It is not designed to be used as a production-level tool for initiating a failover to overcome a
* problem you may have with the cluster. Moreover, in certain conditions such as large scale operational events,
* Amazon may block this API.
*
*
* @param failoverShardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the FailoverShard operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.FailoverShard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future failoverShardAsync(FailoverShardRequest failoverShardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all available node types that you can scale to from your cluster's current node type. When you use the
* UpdateCluster operation to scale your cluster, the value of the NodeType parameter must be one of the node types
* returned by this operation.
*
*
* @param listAllowedNodeTypeUpdatesRequest
* @return A Java Future containing the result of the ListAllowedNodeTypeUpdates operation returned by the service.
* @sample AmazonMemoryDBAsync.ListAllowedNodeTypeUpdates
* @see AWS API Documentation
*/
java.util.concurrent.Future listAllowedNodeTypeUpdatesAsync(
ListAllowedNodeTypeUpdatesRequest listAllowedNodeTypeUpdatesRequest);
/**
*
* Lists all available node types that you can scale to from your cluster's current node type. When you use the
* UpdateCluster operation to scale your cluster, the value of the NodeType parameter must be one of the node types
* returned by this operation.
*
*
* @param listAllowedNodeTypeUpdatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAllowedNodeTypeUpdates operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.ListAllowedNodeTypeUpdates
* @see AWS API Documentation
*/
java.util.concurrent.Future listAllowedNodeTypeUpdatesAsync(
ListAllowedNodeTypeUpdatesRequest listAllowedNodeTypeUpdatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags currently on a named resource. A tag is a key-value pair where the key and value are
* case-sensitive. You can use tags to categorize and track your MemoryDB resources. For more information, see Tagging your MemoryDB
* resources
*
*
* @param listTagsRequest
* @return A Java Future containing the result of the ListTags operation returned by the service.
* @sample AmazonMemoryDBAsync.ListTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsAsync(ListTagsRequest listTagsRequest);
/**
*
* Lists all tags currently on a named resource. A tag is a key-value pair where the key and value are
* case-sensitive. You can use tags to categorize and track your MemoryDB resources. For more information, see Tagging your MemoryDB
* resources
*
*
* @param listTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTags operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.ListTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsAsync(ListTagsRequest listTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allows you to purchase a reserved node offering. Reserved nodes are not eligible for cancellation and are
* non-refundable.
*
*
* @param purchaseReservedNodesOfferingRequest
* @return A Java Future containing the result of the PurchaseReservedNodesOffering operation returned by the
* service.
* @sample AmazonMemoryDBAsync.PurchaseReservedNodesOffering
* @see AWS API Documentation
*/
java.util.concurrent.Future purchaseReservedNodesOfferingAsync(
PurchaseReservedNodesOfferingRequest purchaseReservedNodesOfferingRequest);
/**
*
* Allows you to purchase a reserved node offering. Reserved nodes are not eligible for cancellation and are
* non-refundable.
*
*
* @param purchaseReservedNodesOfferingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PurchaseReservedNodesOffering operation returned by the
* service.
* @sample AmazonMemoryDBAsyncHandler.PurchaseReservedNodesOffering
* @see AWS API Documentation
*/
java.util.concurrent.Future purchaseReservedNodesOfferingAsync(
PurchaseReservedNodesOfferingRequest purchaseReservedNodesOfferingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the parameters of a parameter group to the engine or system default value. You can reset specific
* parameters by submitting a list of parameter names. To reset the entire parameter group, specify the
* AllParameters and ParameterGroupName parameters.
*
*
* @param resetParameterGroupRequest
* @return A Java Future containing the result of the ResetParameterGroup operation returned by the service.
* @sample AmazonMemoryDBAsync.ResetParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future resetParameterGroupAsync(ResetParameterGroupRequest resetParameterGroupRequest);
/**
*
* Modifies the parameters of a parameter group to the engine or system default value. You can reset specific
* parameters by submitting a list of parameter names. To reset the entire parameter group, specify the
* AllParameters and ParameterGroupName parameters.
*
*
* @param resetParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResetParameterGroup operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.ResetParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future resetParameterGroupAsync(ResetParameterGroupRequest resetParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your MemoryDB resources. When you add or remove tags on clusters, those actions will be replicated to all
* nodes in the cluster. For more information, see Resource-level
* permissions.
*
*
* For example, you can use cost-allocation tags to your MemoryDB resources, Amazon generates a cost allocation
* report as a comma-separated value (CSV) file with your usage and costs aggregated by your tags. You can apply
* tags that represent business categories (such as cost centers, application names, or owners) to organize your
* costs across multiple services. For more information, see Using Cost Allocation Tags.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonMemoryDBAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your MemoryDB resources. When you add or remove tags on clusters, those actions will be replicated to all
* nodes in the cluster. For more information, see Resource-level
* permissions.
*
*
* For example, you can use cost-allocation tags to your MemoryDB resources, Amazon generates a cost allocation
* report as a comma-separated value (CSV) file with your usage and costs aggregated by your tags. You can apply
* tags that represent business categories (such as cost centers, application names, or owners) to organize your
* costs across multiple services. For more information, see Using Cost Allocation Tags.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use this operation to remove tags on a resource
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonMemoryDBAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Use this operation to remove tags on a resource
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes the list of users that belong to the Access Control List.
*
*
* @param updateACLRequest
* @return A Java Future containing the result of the UpdateACL operation returned by the service.
* @sample AmazonMemoryDBAsync.UpdateACL
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateACLAsync(UpdateACLRequest updateACLRequest);
/**
*
* Changes the list of users that belong to the Access Control List.
*
*
* @param updateACLRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateACL operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.UpdateACL
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateACLAsync(UpdateACLRequest updateACLRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the settings for a cluster. You can use this operation to change one or more cluster configuration
* settings by specifying the settings and the new values.
*
*
* @param updateClusterRequest
* @return A Java Future containing the result of the UpdateCluster operation returned by the service.
* @sample AmazonMemoryDBAsync.UpdateCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateClusterAsync(UpdateClusterRequest updateClusterRequest);
/**
*
* Modifies the settings for a cluster. You can use this operation to change one or more cluster configuration
* settings by specifying the settings and the new values.
*
*
* @param updateClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateCluster operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.UpdateCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateClusterAsync(UpdateClusterRequest updateClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the parameters of a parameter group. You can modify up to 20 parameters in a single request by submitting
* a list parameter name and value pairs.
*
*
* @param updateParameterGroupRequest
* @return A Java Future containing the result of the UpdateParameterGroup operation returned by the service.
* @sample AmazonMemoryDBAsync.UpdateParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateParameterGroupAsync(UpdateParameterGroupRequest updateParameterGroupRequest);
/**
*
* Updates the parameters of a parameter group. You can modify up to 20 parameters in a single request by submitting
* a list parameter name and value pairs.
*
*
* @param updateParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateParameterGroup operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.UpdateParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateParameterGroupAsync(UpdateParameterGroupRequest updateParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a subnet group. For more information, see Updating a subnet
* group
*
*
* @param updateSubnetGroupRequest
* @return A Java Future containing the result of the UpdateSubnetGroup operation returned by the service.
* @sample AmazonMemoryDBAsync.UpdateSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSubnetGroupAsync(UpdateSubnetGroupRequest updateSubnetGroupRequest);
/**
*
* Updates a subnet group. For more information, see Updating a subnet
* group
*
*
* @param updateSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSubnetGroup operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.UpdateSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSubnetGroupAsync(UpdateSubnetGroupRequest updateSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes user password(s) and/or access string.
*
*
* @param updateUserRequest
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* @sample AmazonMemoryDBAsync.UpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateUserAsync(UpdateUserRequest updateUserRequest);
/**
*
* Changes user password(s) and/or access string.
*
*
* @param updateUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* @sample AmazonMemoryDBAsyncHandler.UpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateUserAsync(UpdateUserRequest updateUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}