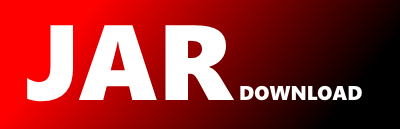
com.amazonaws.services.mgn.AWSmgn Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mgn Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mgn;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.mgn.model.*;
/**
* Interface for accessing mgn.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mgn.AbstractAWSmgn} instead.
*
*
*
* The Application Migration Service service.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSmgn {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "mgn";
/**
*
* Archive application.
*
*
* @param archiveApplicationRequest
* @return Result of the ArchiveApplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.ArchiveApplication
* @see AWS API
* Documentation
*/
ArchiveApplicationResult archiveApplication(ArchiveApplicationRequest archiveApplicationRequest);
/**
*
* Archive wave.
*
*
* @param archiveWaveRequest
* @return Result of the ArchiveWave operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.ArchiveWave
* @see AWS API
* Documentation
*/
ArchiveWaveResult archiveWave(ArchiveWaveRequest archiveWaveRequest);
/**
*
* Associate applications to wave.
*
*
* @param associateApplicationsRequest
* @return Result of the AssociateApplications operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.AssociateApplications
* @see AWS API
* Documentation
*/
AssociateApplicationsResult associateApplications(AssociateApplicationsRequest associateApplicationsRequest);
/**
*
* Associate source servers to application.
*
*
* @param associateSourceServersRequest
* @return Result of the AssociateSourceServers operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.AssociateSourceServers
* @see AWS API
* Documentation
*/
AssociateSourceServersResult associateSourceServers(AssociateSourceServersRequest associateSourceServersRequest);
/**
*
* Allows the user to set the SourceServer.LifeCycle.state property for specific Source Server IDs to one of the
* following: READY_FOR_TEST or READY_FOR_CUTOVER. This command only works if the Source Server is already
* launchable (dataReplicationInfo.lagDuration is not null.)
*
*
* @param changeServerLifeCycleStateRequest
* @return Result of the ChangeServerLifeCycleState operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.ChangeServerLifeCycleState
* @see AWS
* API Documentation
*/
ChangeServerLifeCycleStateResult changeServerLifeCycleState(ChangeServerLifeCycleStateRequest changeServerLifeCycleStateRequest);
/**
*
* Create application.
*
*
* @param createApplicationRequest
* @return Result of the CreateApplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.CreateApplication
* @see AWS API
* Documentation
*/
CreateApplicationResult createApplication(CreateApplicationRequest createApplicationRequest);
/**
*
* Create Connector.
*
*
* @param createConnectorRequest
* @return Result of the CreateConnector operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.CreateConnector
* @see AWS API
* Documentation
*/
CreateConnectorResult createConnector(CreateConnectorRequest createConnectorRequest);
/**
*
* Creates a new Launch Configuration Template.
*
*
* @param createLaunchConfigurationTemplateRequest
* @return Result of the CreateLaunchConfigurationTemplate operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @throws AccessDeniedException
* Operating denied due to a file permission or access check error.
* @sample AWSmgn.CreateLaunchConfigurationTemplate
* @see AWS API Documentation
*/
CreateLaunchConfigurationTemplateResult createLaunchConfigurationTemplate(CreateLaunchConfigurationTemplateRequest createLaunchConfigurationTemplateRequest);
/**
*
* Creates a new ReplicationConfigurationTemplate.
*
*
* @param createReplicationConfigurationTemplateRequest
* @return Result of the CreateReplicationConfigurationTemplate operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @throws AccessDeniedException
* Operating denied due to a file permission or access check error.
* @sample AWSmgn.CreateReplicationConfigurationTemplate
* @see AWS API Documentation
*/
CreateReplicationConfigurationTemplateResult createReplicationConfigurationTemplate(
CreateReplicationConfigurationTemplateRequest createReplicationConfigurationTemplateRequest);
/**
*
* Create wave.
*
*
* @param createWaveRequest
* @return Result of the CreateWave operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.CreateWave
* @see AWS API
* Documentation
*/
CreateWaveResult createWave(CreateWaveRequest createWaveRequest);
/**
*
* Delete application.
*
*
* @param deleteApplicationRequest
* @return Result of the DeleteApplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.DeleteApplication
* @see AWS API
* Documentation
*/
DeleteApplicationResult deleteApplication(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Delete Connector.
*
*
* @param deleteConnectorRequest
* @return Result of the DeleteConnector operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.DeleteConnector
* @see AWS API
* Documentation
*/
DeleteConnectorResult deleteConnector(DeleteConnectorRequest deleteConnectorRequest);
/**
*
* Deletes a single Job by ID.
*
*
* @param deleteJobRequest
* @return Result of the DeleteJob operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.DeleteJob
* @see AWS API
* Documentation
*/
DeleteJobResult deleteJob(DeleteJobRequest deleteJobRequest);
/**
*
* Deletes a single Launch Configuration Template by ID.
*
*
* @param deleteLaunchConfigurationTemplateRequest
* @return Result of the DeleteLaunchConfigurationTemplate operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.DeleteLaunchConfigurationTemplate
* @see AWS API Documentation
*/
DeleteLaunchConfigurationTemplateResult deleteLaunchConfigurationTemplate(DeleteLaunchConfigurationTemplateRequest deleteLaunchConfigurationTemplateRequest);
/**
*
* Deletes a single Replication Configuration Template by ID
*
*
* @param deleteReplicationConfigurationTemplateRequest
* @return Result of the DeleteReplicationConfigurationTemplate operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.DeleteReplicationConfigurationTemplate
* @see AWS API Documentation
*/
DeleteReplicationConfigurationTemplateResult deleteReplicationConfigurationTemplate(
DeleteReplicationConfigurationTemplateRequest deleteReplicationConfigurationTemplateRequest);
/**
*
* Deletes a single source server by ID.
*
*
* @param deleteSourceServerRequest
* @return Result of the DeleteSourceServer operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.DeleteSourceServer
* @see AWS API
* Documentation
*/
DeleteSourceServerResult deleteSourceServer(DeleteSourceServerRequest deleteSourceServerRequest);
/**
*
* Deletes a given vCenter client by ID.
*
*
* @param deleteVcenterClientRequest
* @return Result of the DeleteVcenterClient operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.DeleteVcenterClient
* @see AWS API
* Documentation
*/
DeleteVcenterClientResult deleteVcenterClient(DeleteVcenterClientRequest deleteVcenterClientRequest);
/**
*
* Delete wave.
*
*
* @param deleteWaveRequest
* @return Result of the DeleteWave operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.DeleteWave
* @see AWS API
* Documentation
*/
DeleteWaveResult deleteWave(DeleteWaveRequest deleteWaveRequest);
/**
*
* Retrieves detailed job log items with paging.
*
*
* @param describeJobLogItemsRequest
* @return Result of the DescribeJobLogItems operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.DescribeJobLogItems
* @see AWS API
* Documentation
*/
DescribeJobLogItemsResult describeJobLogItems(DescribeJobLogItemsRequest describeJobLogItemsRequest);
/**
*
* Returns a list of Jobs. Use the JobsID and fromDate and toData filters to limit which jobs are returned. The
* response is sorted by creationDataTime - latest date first. Jobs are normally created by the StartTest,
* StartCutover, and TerminateTargetInstances APIs. Jobs are also created by DiagnosticLaunch and
* TerminateDiagnosticInstances, which are APIs available only to *Support* and only used in response to relevant
* support tickets.
*
*
* @param describeJobsRequest
* @return Result of the DescribeJobs operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.DescribeJobs
* @see AWS API
* Documentation
*/
DescribeJobsResult describeJobs(DescribeJobsRequest describeJobsRequest);
/**
*
* Lists all Launch Configuration Templates, filtered by Launch Configuration Template IDs
*
*
* @param describeLaunchConfigurationTemplatesRequest
* @return Result of the DescribeLaunchConfigurationTemplates operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.DescribeLaunchConfigurationTemplates
* @see AWS API Documentation
*/
DescribeLaunchConfigurationTemplatesResult describeLaunchConfigurationTemplates(
DescribeLaunchConfigurationTemplatesRequest describeLaunchConfigurationTemplatesRequest);
/**
*
* Lists all ReplicationConfigurationTemplates, filtered by Source Server IDs.
*
*
* @param describeReplicationConfigurationTemplatesRequest
* @return Result of the DescribeReplicationConfigurationTemplates operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.DescribeReplicationConfigurationTemplates
* @see AWS API Documentation
*/
DescribeReplicationConfigurationTemplatesResult describeReplicationConfigurationTemplates(
DescribeReplicationConfigurationTemplatesRequest describeReplicationConfigurationTemplatesRequest);
/**
*
* Retrieves all SourceServers or multiple SourceServers by ID.
*
*
* @param describeSourceServersRequest
* @return Result of the DescribeSourceServers operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.DescribeSourceServers
* @see AWS API
* Documentation
*/
DescribeSourceServersResult describeSourceServers(DescribeSourceServersRequest describeSourceServersRequest);
/**
*
* Returns a list of the installed vCenter clients.
*
*
* @param describeVcenterClientsRequest
* @return Result of the DescribeVcenterClients operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.DescribeVcenterClients
* @see AWS API
* Documentation
*/
DescribeVcenterClientsResult describeVcenterClients(DescribeVcenterClientsRequest describeVcenterClientsRequest);
/**
*
* Disassociate applications from wave.
*
*
* @param disassociateApplicationsRequest
* @return Result of the DisassociateApplications operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.DisassociateApplications
* @see AWS
* API Documentation
*/
DisassociateApplicationsResult disassociateApplications(DisassociateApplicationsRequest disassociateApplicationsRequest);
/**
*
* Disassociate source servers from application.
*
*
* @param disassociateSourceServersRequest
* @return Result of the DisassociateSourceServers operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.DisassociateSourceServers
* @see AWS
* API Documentation
*/
DisassociateSourceServersResult disassociateSourceServers(DisassociateSourceServersRequest disassociateSourceServersRequest);
/**
*
* Disconnects specific Source Servers from Application Migration Service. Data replication is stopped immediately.
* All AWS resources created by Application Migration Service for enabling the replication of these source servers
* will be terminated / deleted within 90 minutes. Launched Test or Cutover instances will NOT be terminated. If the
* agent on the source server has not been prevented from communicating with the Application Migration Service
* service, then it will receive a command to uninstall itself (within approximately 10 minutes). The following
* properties of the SourceServer will be changed immediately: dataReplicationInfo.dataReplicationState will be set
* to DISCONNECTED; The totalStorageBytes property for each of dataReplicationInfo.replicatedDisks will be set to
* zero; dataReplicationInfo.lagDuration and dataReplicationInfo.lagDuration will be nullified.
*
*
* @param disconnectFromServiceRequest
* @return Result of the DisconnectFromService operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.DisconnectFromService
* @see AWS API
* Documentation
*/
DisconnectFromServiceResult disconnectFromService(DisconnectFromServiceRequest disconnectFromServiceRequest);
/**
*
* Finalizes the cutover immediately for specific Source Servers. All AWS resources created by Application Migration
* Service for enabling the replication of these source servers will be terminated / deleted within 90 minutes.
* Launched Test or Cutover instances will NOT be terminated. The AWS Replication Agent will receive a command to
* uninstall itself (within 10 minutes). The following properties of the SourceServer will be changed immediately:
* dataReplicationInfo.dataReplicationState will be changed to DISCONNECTED; The SourceServer.lifeCycle.state will
* be changed to CUTOVER; The totalStorageBytes property fo each of dataReplicationInfo.replicatedDisks will be set
* to zero; dataReplicationInfo.lagDuration and dataReplicationInfo.lagDuration will be nullified.
*
*
* @param finalizeCutoverRequest
* @return Result of the FinalizeCutover operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.FinalizeCutover
* @see AWS API
* Documentation
*/
FinalizeCutoverResult finalizeCutover(FinalizeCutoverRequest finalizeCutoverRequest);
/**
*
* Lists all LaunchConfigurations available, filtered by Source Server IDs.
*
*
* @param getLaunchConfigurationRequest
* @return Result of the GetLaunchConfiguration operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @sample AWSmgn.GetLaunchConfiguration
* @see AWS API
* Documentation
*/
GetLaunchConfigurationResult getLaunchConfiguration(GetLaunchConfigurationRequest getLaunchConfigurationRequest);
/**
*
* Lists all ReplicationConfigurations, filtered by Source Server ID.
*
*
* @param getReplicationConfigurationRequest
* @return Result of the GetReplicationConfiguration operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @sample AWSmgn.GetReplicationConfiguration
* @see AWS API Documentation
*/
GetReplicationConfigurationResult getReplicationConfiguration(GetReplicationConfigurationRequest getReplicationConfigurationRequest);
/**
*
* Initialize Application Migration Service.
*
*
* @param initializeServiceRequest
* @return Result of the InitializeService operation returned by the service.
* @throws ValidationException
* Validate exception.
* @throws AccessDeniedException
* Operating denied due to a file permission or access check error.
* @sample AWSmgn.InitializeService
* @see AWS API
* Documentation
*/
InitializeServiceResult initializeService(InitializeServiceRequest initializeServiceRequest);
/**
*
* Retrieves all applications or multiple applications by ID.
*
*
* @param listApplicationsRequest
* @return Result of the ListApplications operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @sample AWSmgn.ListApplications
* @see AWS API
* Documentation
*/
ListApplicationsResult listApplications(ListApplicationsRequest listApplicationsRequest);
/**
*
* List Connectors.
*
*
* @param listConnectorsRequest
* @return Result of the ListConnectors operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.ListConnectors
* @see AWS API
* Documentation
*/
ListConnectorsResult listConnectors(ListConnectorsRequest listConnectorsRequest);
/**
*
* List export errors.
*
*
* @param listExportErrorsRequest
* List export errors request.
* @return Result of the ListExportErrors operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.ListExportErrors
* @see AWS API
* Documentation
*/
ListExportErrorsResult listExportErrors(ListExportErrorsRequest listExportErrorsRequest);
/**
*
* List exports.
*
*
* @param listExportsRequest
* List export request.
* @return Result of the ListExports operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @sample AWSmgn.ListExports
* @see AWS API
* Documentation
*/
ListExportsResult listExports(ListExportsRequest listExportsRequest);
/**
*
* List import errors.
*
*
* @param listImportErrorsRequest
* List import errors request.
* @return Result of the ListImportErrors operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.ListImportErrors
* @see AWS API
* Documentation
*/
ListImportErrorsResult listImportErrors(ListImportErrorsRequest listImportErrorsRequest);
/**
*
* List imports.
*
*
* @param listImportsRequest
* List imports request.
* @return Result of the ListImports operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.ListImports
* @see AWS API
* Documentation
*/
ListImportsResult listImports(ListImportsRequest listImportsRequest);
/**
*
* List Managed Accounts.
*
*
* @param listManagedAccountsRequest
* List managed accounts request.
* @return Result of the ListManagedAccounts operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.ListManagedAccounts
* @see AWS API
* Documentation
*/
ListManagedAccountsResult listManagedAccounts(ListManagedAccountsRequest listManagedAccountsRequest);
/**
*
* List source server post migration custom actions.
*
*
* @param listSourceServerActionsRequest
* @return Result of the ListSourceServerActions operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @sample AWSmgn.ListSourceServerActions
* @see AWS
* API Documentation
*/
ListSourceServerActionsResult listSourceServerActions(ListSourceServerActionsRequest listSourceServerActionsRequest);
/**
*
* List all tags for your Application Migration Service resources.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ThrottlingException
* Reached throttling quota exception.
* @throws ValidationException
* Validate exception.
* @throws AccessDeniedException
* Operating denied due to a file permission or access check error.
* @throws InternalServerException
* The server encountered an unexpected condition that prevented it from fulfilling the request.
* @sample AWSmgn.ListTagsForResource
* @see AWS API
* Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List template post migration custom actions.
*
*
* @param listTemplateActionsRequest
* @return Result of the ListTemplateActions operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @sample AWSmgn.ListTemplateActions
* @see AWS API
* Documentation
*/
ListTemplateActionsResult listTemplateActions(ListTemplateActionsRequest listTemplateActionsRequest);
/**
*
* Retrieves all waves or multiple waves by ID.
*
*
* @param listWavesRequest
* @return Result of the ListWaves operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @sample AWSmgn.ListWaves
* @see AWS API
* Documentation
*/
ListWavesResult listWaves(ListWavesRequest listWavesRequest);
/**
*
* Archives specific Source Servers by setting the SourceServer.isArchived property to true for specified
* SourceServers by ID. This command only works for SourceServers with a lifecycle. state which equals DISCONNECTED
* or CUTOVER.
*
*
* @param markAsArchivedRequest
* @return Result of the MarkAsArchived operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.MarkAsArchived
* @see AWS API
* Documentation
*/
MarkAsArchivedResult markAsArchived(MarkAsArchivedRequest markAsArchivedRequest);
/**
*
* Pause Replication.
*
*
* @param pauseReplicationRequest
* @return Result of the PauseReplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.PauseReplication
* @see AWS API
* Documentation
*/
PauseReplicationResult pauseReplication(PauseReplicationRequest pauseReplicationRequest);
/**
*
* Put source server post migration custom action.
*
*
* @param putSourceServerActionRequest
* @return Result of the PutSourceServerAction operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.PutSourceServerAction
* @see AWS API
* Documentation
*/
PutSourceServerActionResult putSourceServerAction(PutSourceServerActionRequest putSourceServerActionRequest);
/**
*
* Put template post migration custom action.
*
*
* @param putTemplateActionRequest
* @return Result of the PutTemplateAction operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.PutTemplateAction
* @see AWS API
* Documentation
*/
PutTemplateActionResult putTemplateAction(PutTemplateActionRequest putTemplateActionRequest);
/**
*
* Remove source server post migration custom action.
*
*
* @param removeSourceServerActionRequest
* @return Result of the RemoveSourceServerAction operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.RemoveSourceServerAction
* @see AWS
* API Documentation
*/
RemoveSourceServerActionResult removeSourceServerAction(RemoveSourceServerActionRequest removeSourceServerActionRequest);
/**
*
* Remove template post migration custom action.
*
*
* @param removeTemplateActionRequest
* @return Result of the RemoveTemplateAction operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.RemoveTemplateAction
* @see AWS API
* Documentation
*/
RemoveTemplateActionResult removeTemplateAction(RemoveTemplateActionRequest removeTemplateActionRequest);
/**
*
* Resume Replication.
*
*
* @param resumeReplicationRequest
* @return Result of the ResumeReplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.ResumeReplication
* @see AWS API
* Documentation
*/
ResumeReplicationResult resumeReplication(ResumeReplicationRequest resumeReplicationRequest);
/**
*
* Causes the data replication initiation sequence to begin immediately upon next Handshake for specified
* SourceServer IDs, regardless of when the previous initiation started. This command will not work if the
* SourceServer is not stalled or is in a DISCONNECTED or STOPPED state.
*
*
* @param retryDataReplicationRequest
* @return Result of the RetryDataReplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.RetryDataReplication
* @see AWS API
* Documentation
*/
RetryDataReplicationResult retryDataReplication(RetryDataReplicationRequest retryDataReplicationRequest);
/**
*
* Launches a Cutover Instance for specific Source Servers. This command starts a LAUNCH job whose initiatedBy
* property is StartCutover and changes the SourceServer.lifeCycle.state property to CUTTING_OVER.
*
*
* @param startCutoverRequest
* @return Result of the StartCutover operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.StartCutover
* @see AWS API
* Documentation
*/
StartCutoverResult startCutover(StartCutoverRequest startCutoverRequest);
/**
*
* Start export.
*
*
* @param startExportRequest
* Start export request.
* @return Result of the StartExport operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @sample AWSmgn.StartExport
* @see AWS API
* Documentation
*/
StartExportResult startExport(StartExportRequest startExportRequest);
/**
*
* Start import.
*
*
* @param startImportRequest
* Start import request.
* @return Result of the StartImport operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.StartImport
* @see AWS API
* Documentation
*/
StartImportResult startImport(StartImportRequest startImportRequest);
/**
*
* Starts replication for SNAPSHOT_SHIPPING agents.
*
*
* @param startReplicationRequest
* @return Result of the StartReplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.StartReplication
* @see AWS API
* Documentation
*/
StartReplicationResult startReplication(StartReplicationRequest startReplicationRequest);
/**
*
* Launches a Test Instance for specific Source Servers. This command starts a LAUNCH job whose initiatedBy property
* is StartTest and changes the SourceServer.lifeCycle.state property to TESTING.
*
*
* @param startTestRequest
* @return Result of the StartTest operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.StartTest
* @see AWS API
* Documentation
*/
StartTestResult startTest(StartTestRequest startTestRequest);
/**
*
* Stop Replication.
*
*
* @param stopReplicationRequest
* @return Result of the StopReplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.StopReplication
* @see AWS API
* Documentation
*/
StopReplicationResult stopReplication(StopReplicationRequest stopReplicationRequest);
/**
*
* Adds or overwrites only the specified tags for the specified Application Migration Service resource or resources.
* When you specify an existing tag key, the value is overwritten with the new value. Each resource can have a
* maximum of 50 tags. Each tag consists of a key and optional value.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ThrottlingException
* Reached throttling quota exception.
* @throws ValidationException
* Validate exception.
* @throws AccessDeniedException
* Operating denied due to a file permission or access check error.
* @throws InternalServerException
* The server encountered an unexpected condition that prevented it from fulfilling the request.
* @sample AWSmgn.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Starts a job that terminates specific launched EC2 Test and Cutover instances. This command will not work for any
* Source Server with a lifecycle.state of TESTING, CUTTING_OVER, or CUTOVER.
*
*
* @param terminateTargetInstancesRequest
* @return Result of the TerminateTargetInstances operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ValidationException
* Validate exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.TerminateTargetInstances
* @see AWS
* API Documentation
*/
TerminateTargetInstancesResult terminateTargetInstances(TerminateTargetInstancesRequest terminateTargetInstancesRequest);
/**
*
* Unarchive application.
*
*
* @param unarchiveApplicationRequest
* @return Result of the UnarchiveApplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @sample AWSmgn.UnarchiveApplication
* @see AWS API
* Documentation
*/
UnarchiveApplicationResult unarchiveApplication(UnarchiveApplicationRequest unarchiveApplicationRequest);
/**
*
* Unarchive wave.
*
*
* @param unarchiveWaveRequest
* @return Result of the UnarchiveWave operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ServiceQuotaExceededException
* The request could not be completed because its exceeded the service quota.
* @sample AWSmgn.UnarchiveWave
* @see AWS API
* Documentation
*/
UnarchiveWaveResult unarchiveWave(UnarchiveWaveRequest unarchiveWaveRequest);
/**
*
* Deletes the specified set of tags from the specified set of Application Migration Service resources.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ThrottlingException
* Reached throttling quota exception.
* @throws ValidationException
* Validate exception.
* @throws AccessDeniedException
* Operating denied due to a file permission or access check error.
* @throws InternalServerException
* The server encountered an unexpected condition that prevented it from fulfilling the request.
* @sample AWSmgn.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Update application.
*
*
* @param updateApplicationRequest
* @return Result of the UpdateApplication operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.UpdateApplication
* @see AWS API
* Documentation
*/
UpdateApplicationResult updateApplication(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Update Connector.
*
*
* @param updateConnectorRequest
* @return Result of the UpdateConnector operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @sample AWSmgn.UpdateConnector
* @see AWS API
* Documentation
*/
UpdateConnectorResult updateConnector(UpdateConnectorRequest updateConnectorRequest);
/**
*
* Updates multiple LaunchConfigurations by Source Server ID.
*
*
*
* bootMode valid values are LEGACY_BIOS | UEFI
*
*
*
* @param updateLaunchConfigurationRequest
* @return Result of the UpdateLaunchConfiguration operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.UpdateLaunchConfiguration
* @see AWS
* API Documentation
*/
UpdateLaunchConfigurationResult updateLaunchConfiguration(UpdateLaunchConfigurationRequest updateLaunchConfigurationRequest);
/**
*
* Updates an existing Launch Configuration Template by ID.
*
*
* @param updateLaunchConfigurationTemplateRequest
* @return Result of the UpdateLaunchConfigurationTemplate operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws AccessDeniedException
* Operating denied due to a file permission or access check error.
* @sample AWSmgn.UpdateLaunchConfigurationTemplate
* @see AWS API Documentation
*/
UpdateLaunchConfigurationTemplateResult updateLaunchConfigurationTemplate(UpdateLaunchConfigurationTemplateRequest updateLaunchConfigurationTemplateRequest);
/**
*
* Allows you to update multiple ReplicationConfigurations by Source Server ID.
*
*
* @param updateReplicationConfigurationRequest
* @return Result of the UpdateReplicationConfiguration operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws AccessDeniedException
* Operating denied due to a file permission or access check error.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.UpdateReplicationConfiguration
* @see AWS API Documentation
*/
UpdateReplicationConfigurationResult updateReplicationConfiguration(UpdateReplicationConfigurationRequest updateReplicationConfigurationRequest);
/**
*
* Updates multiple ReplicationConfigurationTemplates by ID.
*
*
* @param updateReplicationConfigurationTemplateRequest
* @return Result of the UpdateReplicationConfigurationTemplate operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws AccessDeniedException
* Operating denied due to a file permission or access check error.
* @sample AWSmgn.UpdateReplicationConfigurationTemplate
* @see AWS API Documentation
*/
UpdateReplicationConfigurationTemplateResult updateReplicationConfigurationTemplate(
UpdateReplicationConfigurationTemplateRequest updateReplicationConfigurationTemplateRequest);
/**
*
* Update Source Server.
*
*
* @param updateSourceServerRequest
* @return Result of the UpdateSourceServer operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.UpdateSourceServer
* @see AWS API
* Documentation
*/
UpdateSourceServerResult updateSourceServer(UpdateSourceServerRequest updateSourceServerRequest);
/**
*
* Allows you to change between the AGENT_BASED replication type and the SNAPSHOT_SHIPPING replication type.
*
*
* @param updateSourceServerReplicationTypeRequest
* @return Result of the UpdateSourceServerReplicationType operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ValidationException
* Validate exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.UpdateSourceServerReplicationType
* @see AWS API Documentation
*/
UpdateSourceServerReplicationTypeResult updateSourceServerReplicationType(UpdateSourceServerReplicationTypeRequest updateSourceServerReplicationTypeRequest);
/**
*
* Update wave.
*
*
* @param updateWaveRequest
* @return Result of the UpdateWave operation returned by the service.
* @throws UninitializedAccountException
* Uninitialized account exception.
* @throws ResourceNotFoundException
* Resource not found exception.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSmgn.UpdateWave
* @see AWS API
* Documentation
*/
UpdateWaveResult updateWave(UpdateWaveRequest updateWaveRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}