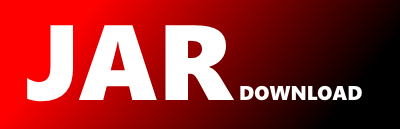
com.amazonaws.services.mgn.AWSmgnAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mgn Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mgn;
import javax.annotation.Generated;
import com.amazonaws.services.mgn.model.*;
/**
* Interface for accessing mgn asynchronously. Each asynchronous method will return a Java Future object representing
* the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive notification when
* an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mgn.AbstractAWSmgnAsync} instead.
*
*
*
* The Application Migration Service service.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSmgnAsync extends AWSmgn {
/**
*
* Archive application.
*
*
* @param archiveApplicationRequest
* @return A Java Future containing the result of the ArchiveApplication operation returned by the service.
* @sample AWSmgnAsync.ArchiveApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future archiveApplicationAsync(ArchiveApplicationRequest archiveApplicationRequest);
/**
*
* Archive application.
*
*
* @param archiveApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ArchiveApplication operation returned by the service.
* @sample AWSmgnAsyncHandler.ArchiveApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future archiveApplicationAsync(ArchiveApplicationRequest archiveApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Archive wave.
*
*
* @param archiveWaveRequest
* @return A Java Future containing the result of the ArchiveWave operation returned by the service.
* @sample AWSmgnAsync.ArchiveWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future archiveWaveAsync(ArchiveWaveRequest archiveWaveRequest);
/**
*
* Archive wave.
*
*
* @param archiveWaveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ArchiveWave operation returned by the service.
* @sample AWSmgnAsyncHandler.ArchiveWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future archiveWaveAsync(ArchiveWaveRequest archiveWaveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate applications to wave.
*
*
* @param associateApplicationsRequest
* @return A Java Future containing the result of the AssociateApplications operation returned by the service.
* @sample AWSmgnAsync.AssociateApplications
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateApplicationsAsync(AssociateApplicationsRequest associateApplicationsRequest);
/**
*
* Associate applications to wave.
*
*
* @param associateApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateApplications operation returned by the service.
* @sample AWSmgnAsyncHandler.AssociateApplications
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateApplicationsAsync(AssociateApplicationsRequest associateApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate source servers to application.
*
*
* @param associateSourceServersRequest
* @return A Java Future containing the result of the AssociateSourceServers operation returned by the service.
* @sample AWSmgnAsync.AssociateSourceServers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateSourceServersAsync(AssociateSourceServersRequest associateSourceServersRequest);
/**
*
* Associate source servers to application.
*
*
* @param associateSourceServersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateSourceServers operation returned by the service.
* @sample AWSmgnAsyncHandler.AssociateSourceServers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateSourceServersAsync(AssociateSourceServersRequest associateSourceServersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allows the user to set the SourceServer.LifeCycle.state property for specific Source Server IDs to one of the
* following: READY_FOR_TEST or READY_FOR_CUTOVER. This command only works if the Source Server is already
* launchable (dataReplicationInfo.lagDuration is not null.)
*
*
* @param changeServerLifeCycleStateRequest
* @return A Java Future containing the result of the ChangeServerLifeCycleState operation returned by the service.
* @sample AWSmgnAsync.ChangeServerLifeCycleState
* @see AWS
* API Documentation
*/
java.util.concurrent.Future changeServerLifeCycleStateAsync(
ChangeServerLifeCycleStateRequest changeServerLifeCycleStateRequest);
/**
*
* Allows the user to set the SourceServer.LifeCycle.state property for specific Source Server IDs to one of the
* following: READY_FOR_TEST or READY_FOR_CUTOVER. This command only works if the Source Server is already
* launchable (dataReplicationInfo.lagDuration is not null.)
*
*
* @param changeServerLifeCycleStateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ChangeServerLifeCycleState operation returned by the service.
* @sample AWSmgnAsyncHandler.ChangeServerLifeCycleState
* @see AWS
* API Documentation
*/
java.util.concurrent.Future changeServerLifeCycleStateAsync(
ChangeServerLifeCycleStateRequest changeServerLifeCycleStateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create application.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AWSmgnAsync.CreateApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest);
/**
*
* Create application.
*
*
* @param createApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AWSmgnAsyncHandler.CreateApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create Connector.
*
*
* @param createConnectorRequest
* @return A Java Future containing the result of the CreateConnector operation returned by the service.
* @sample AWSmgnAsync.CreateConnector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createConnectorAsync(CreateConnectorRequest createConnectorRequest);
/**
*
* Create Connector.
*
*
* @param createConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConnector operation returned by the service.
* @sample AWSmgnAsyncHandler.CreateConnector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createConnectorAsync(CreateConnectorRequest createConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Launch Configuration Template.
*
*
* @param createLaunchConfigurationTemplateRequest
* @return A Java Future containing the result of the CreateLaunchConfigurationTemplate operation returned by the
* service.
* @sample AWSmgnAsync.CreateLaunchConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createLaunchConfigurationTemplateAsync(
CreateLaunchConfigurationTemplateRequest createLaunchConfigurationTemplateRequest);
/**
*
* Creates a new Launch Configuration Template.
*
*
* @param createLaunchConfigurationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLaunchConfigurationTemplate operation returned by the
* service.
* @sample AWSmgnAsyncHandler.CreateLaunchConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createLaunchConfigurationTemplateAsync(
CreateLaunchConfigurationTemplateRequest createLaunchConfigurationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new ReplicationConfigurationTemplate.
*
*
* @param createReplicationConfigurationTemplateRequest
* @return A Java Future containing the result of the CreateReplicationConfigurationTemplate operation returned by
* the service.
* @sample AWSmgnAsync.CreateReplicationConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createReplicationConfigurationTemplateAsync(
CreateReplicationConfigurationTemplateRequest createReplicationConfigurationTemplateRequest);
/**
*
* Creates a new ReplicationConfigurationTemplate.
*
*
* @param createReplicationConfigurationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReplicationConfigurationTemplate operation returned by
* the service.
* @sample AWSmgnAsyncHandler.CreateReplicationConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createReplicationConfigurationTemplateAsync(
CreateReplicationConfigurationTemplateRequest createReplicationConfigurationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create wave.
*
*
* @param createWaveRequest
* @return A Java Future containing the result of the CreateWave operation returned by the service.
* @sample AWSmgnAsync.CreateWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createWaveAsync(CreateWaveRequest createWaveRequest);
/**
*
* Create wave.
*
*
* @param createWaveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWave operation returned by the service.
* @sample AWSmgnAsyncHandler.CreateWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createWaveAsync(CreateWaveRequest createWaveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete application.
*
*
* @param deleteApplicationRequest
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AWSmgnAsync.DeleteApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Delete application.
*
*
* @param deleteApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AWSmgnAsyncHandler.DeleteApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete Connector.
*
*
* @param deleteConnectorRequest
* @return A Java Future containing the result of the DeleteConnector operation returned by the service.
* @sample AWSmgnAsync.DeleteConnector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteConnectorAsync(DeleteConnectorRequest deleteConnectorRequest);
/**
*
* Delete Connector.
*
*
* @param deleteConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConnector operation returned by the service.
* @sample AWSmgnAsyncHandler.DeleteConnector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteConnectorAsync(DeleteConnectorRequest deleteConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a single Job by ID.
*
*
* @param deleteJobRequest
* @return A Java Future containing the result of the DeleteJob operation returned by the service.
* @sample AWSmgnAsync.DeleteJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteJobAsync(DeleteJobRequest deleteJobRequest);
/**
*
* Deletes a single Job by ID.
*
*
* @param deleteJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteJob operation returned by the service.
* @sample AWSmgnAsyncHandler.DeleteJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteJobAsync(DeleteJobRequest deleteJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a single Launch Configuration Template by ID.
*
*
* @param deleteLaunchConfigurationTemplateRequest
* @return A Java Future containing the result of the DeleteLaunchConfigurationTemplate operation returned by the
* service.
* @sample AWSmgnAsync.DeleteLaunchConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteLaunchConfigurationTemplateAsync(
DeleteLaunchConfigurationTemplateRequest deleteLaunchConfigurationTemplateRequest);
/**
*
* Deletes a single Launch Configuration Template by ID.
*
*
* @param deleteLaunchConfigurationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLaunchConfigurationTemplate operation returned by the
* service.
* @sample AWSmgnAsyncHandler.DeleteLaunchConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteLaunchConfigurationTemplateAsync(
DeleteLaunchConfigurationTemplateRequest deleteLaunchConfigurationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a single Replication Configuration Template by ID
*
*
* @param deleteReplicationConfigurationTemplateRequest
* @return A Java Future containing the result of the DeleteReplicationConfigurationTemplate operation returned by
* the service.
* @sample AWSmgnAsync.DeleteReplicationConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteReplicationConfigurationTemplateAsync(
DeleteReplicationConfigurationTemplateRequest deleteReplicationConfigurationTemplateRequest);
/**
*
* Deletes a single Replication Configuration Template by ID
*
*
* @param deleteReplicationConfigurationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationConfigurationTemplate operation returned by
* the service.
* @sample AWSmgnAsyncHandler.DeleteReplicationConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteReplicationConfigurationTemplateAsync(
DeleteReplicationConfigurationTemplateRequest deleteReplicationConfigurationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a single source server by ID.
*
*
* @param deleteSourceServerRequest
* @return A Java Future containing the result of the DeleteSourceServer operation returned by the service.
* @sample AWSmgnAsync.DeleteSourceServer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSourceServerAsync(DeleteSourceServerRequest deleteSourceServerRequest);
/**
*
* Deletes a single source server by ID.
*
*
* @param deleteSourceServerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSourceServer operation returned by the service.
* @sample AWSmgnAsyncHandler.DeleteSourceServer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSourceServerAsync(DeleteSourceServerRequest deleteSourceServerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a given vCenter client by ID.
*
*
* @param deleteVcenterClientRequest
* @return A Java Future containing the result of the DeleteVcenterClient operation returned by the service.
* @sample AWSmgnAsync.DeleteVcenterClient
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteVcenterClientAsync(DeleteVcenterClientRequest deleteVcenterClientRequest);
/**
*
* Deletes a given vCenter client by ID.
*
*
* @param deleteVcenterClientRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVcenterClient operation returned by the service.
* @sample AWSmgnAsyncHandler.DeleteVcenterClient
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteVcenterClientAsync(DeleteVcenterClientRequest deleteVcenterClientRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete wave.
*
*
* @param deleteWaveRequest
* @return A Java Future containing the result of the DeleteWave operation returned by the service.
* @sample AWSmgnAsync.DeleteWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteWaveAsync(DeleteWaveRequest deleteWaveRequest);
/**
*
* Delete wave.
*
*
* @param deleteWaveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteWave operation returned by the service.
* @sample AWSmgnAsyncHandler.DeleteWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteWaveAsync(DeleteWaveRequest deleteWaveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves detailed job log items with paging.
*
*
* @param describeJobLogItemsRequest
* @return A Java Future containing the result of the DescribeJobLogItems operation returned by the service.
* @sample AWSmgnAsync.DescribeJobLogItems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeJobLogItemsAsync(DescribeJobLogItemsRequest describeJobLogItemsRequest);
/**
*
* Retrieves detailed job log items with paging.
*
*
* @param describeJobLogItemsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeJobLogItems operation returned by the service.
* @sample AWSmgnAsyncHandler.DescribeJobLogItems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeJobLogItemsAsync(DescribeJobLogItemsRequest describeJobLogItemsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of Jobs. Use the JobsID and fromDate and toData filters to limit which jobs are returned. The
* response is sorted by creationDataTime - latest date first. Jobs are normally created by the StartTest,
* StartCutover, and TerminateTargetInstances APIs. Jobs are also created by DiagnosticLaunch and
* TerminateDiagnosticInstances, which are APIs available only to *Support* and only used in response to relevant
* support tickets.
*
*
* @param describeJobsRequest
* @return A Java Future containing the result of the DescribeJobs operation returned by the service.
* @sample AWSmgnAsync.DescribeJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeJobsAsync(DescribeJobsRequest describeJobsRequest);
/**
*
* Returns a list of Jobs. Use the JobsID and fromDate and toData filters to limit which jobs are returned. The
* response is sorted by creationDataTime - latest date first. Jobs are normally created by the StartTest,
* StartCutover, and TerminateTargetInstances APIs. Jobs are also created by DiagnosticLaunch and
* TerminateDiagnosticInstances, which are APIs available only to *Support* and only used in response to relevant
* support tickets.
*
*
* @param describeJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeJobs operation returned by the service.
* @sample AWSmgnAsyncHandler.DescribeJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeJobsAsync(DescribeJobsRequest describeJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all Launch Configuration Templates, filtered by Launch Configuration Template IDs
*
*
* @param describeLaunchConfigurationTemplatesRequest
* @return A Java Future containing the result of the DescribeLaunchConfigurationTemplates operation returned by the
* service.
* @sample AWSmgnAsync.DescribeLaunchConfigurationTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLaunchConfigurationTemplatesAsync(
DescribeLaunchConfigurationTemplatesRequest describeLaunchConfigurationTemplatesRequest);
/**
*
* Lists all Launch Configuration Templates, filtered by Launch Configuration Template IDs
*
*
* @param describeLaunchConfigurationTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLaunchConfigurationTemplates operation returned by the
* service.
* @sample AWSmgnAsyncHandler.DescribeLaunchConfigurationTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLaunchConfigurationTemplatesAsync(
DescribeLaunchConfigurationTemplatesRequest describeLaunchConfigurationTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all ReplicationConfigurationTemplates, filtered by Source Server IDs.
*
*
* @param describeReplicationConfigurationTemplatesRequest
* @return A Java Future containing the result of the DescribeReplicationConfigurationTemplates operation returned
* by the service.
* @sample AWSmgnAsync.DescribeReplicationConfigurationTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationConfigurationTemplatesAsync(
DescribeReplicationConfigurationTemplatesRequest describeReplicationConfigurationTemplatesRequest);
/**
*
* Lists all ReplicationConfigurationTemplates, filtered by Source Server IDs.
*
*
* @param describeReplicationConfigurationTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationConfigurationTemplates operation returned
* by the service.
* @sample AWSmgnAsyncHandler.DescribeReplicationConfigurationTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationConfigurationTemplatesAsync(
DescribeReplicationConfigurationTemplatesRequest describeReplicationConfigurationTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves all SourceServers or multiple SourceServers by ID.
*
*
* @param describeSourceServersRequest
* @return A Java Future containing the result of the DescribeSourceServers operation returned by the service.
* @sample AWSmgnAsync.DescribeSourceServers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSourceServersAsync(DescribeSourceServersRequest describeSourceServersRequest);
/**
*
* Retrieves all SourceServers or multiple SourceServers by ID.
*
*
* @param describeSourceServersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSourceServers operation returned by the service.
* @sample AWSmgnAsyncHandler.DescribeSourceServers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSourceServersAsync(DescribeSourceServersRequest describeSourceServersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the installed vCenter clients.
*
*
* @param describeVcenterClientsRequest
* @return A Java Future containing the result of the DescribeVcenterClients operation returned by the service.
* @sample AWSmgnAsync.DescribeVcenterClients
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeVcenterClientsAsync(DescribeVcenterClientsRequest describeVcenterClientsRequest);
/**
*
* Returns a list of the installed vCenter clients.
*
*
* @param describeVcenterClientsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVcenterClients operation returned by the service.
* @sample AWSmgnAsyncHandler.DescribeVcenterClients
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeVcenterClientsAsync(DescribeVcenterClientsRequest describeVcenterClientsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociate applications from wave.
*
*
* @param disassociateApplicationsRequest
* @return A Java Future containing the result of the DisassociateApplications operation returned by the service.
* @sample AWSmgnAsync.DisassociateApplications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateApplicationsAsync(DisassociateApplicationsRequest disassociateApplicationsRequest);
/**
*
* Disassociate applications from wave.
*
*
* @param disassociateApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateApplications operation returned by the service.
* @sample AWSmgnAsyncHandler.DisassociateApplications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateApplicationsAsync(DisassociateApplicationsRequest disassociateApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociate source servers from application.
*
*
* @param disassociateSourceServersRequest
* @return A Java Future containing the result of the DisassociateSourceServers operation returned by the service.
* @sample AWSmgnAsync.DisassociateSourceServers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateSourceServersAsync(
DisassociateSourceServersRequest disassociateSourceServersRequest);
/**
*
* Disassociate source servers from application.
*
*
* @param disassociateSourceServersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateSourceServers operation returned by the service.
* @sample AWSmgnAsyncHandler.DisassociateSourceServers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateSourceServersAsync(
DisassociateSourceServersRequest disassociateSourceServersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disconnects specific Source Servers from Application Migration Service. Data replication is stopped immediately.
* All AWS resources created by Application Migration Service for enabling the replication of these source servers
* will be terminated / deleted within 90 minutes. Launched Test or Cutover instances will NOT be terminated. If the
* agent on the source server has not been prevented from communicating with the Application Migration Service
* service, then it will receive a command to uninstall itself (within approximately 10 minutes). The following
* properties of the SourceServer will be changed immediately: dataReplicationInfo.dataReplicationState will be set
* to DISCONNECTED; The totalStorageBytes property for each of dataReplicationInfo.replicatedDisks will be set to
* zero; dataReplicationInfo.lagDuration and dataReplicationInfo.lagDuration will be nullified.
*
*
* @param disconnectFromServiceRequest
* @return A Java Future containing the result of the DisconnectFromService operation returned by the service.
* @sample AWSmgnAsync.DisconnectFromService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disconnectFromServiceAsync(DisconnectFromServiceRequest disconnectFromServiceRequest);
/**
*
* Disconnects specific Source Servers from Application Migration Service. Data replication is stopped immediately.
* All AWS resources created by Application Migration Service for enabling the replication of these source servers
* will be terminated / deleted within 90 minutes. Launched Test or Cutover instances will NOT be terminated. If the
* agent on the source server has not been prevented from communicating with the Application Migration Service
* service, then it will receive a command to uninstall itself (within approximately 10 minutes). The following
* properties of the SourceServer will be changed immediately: dataReplicationInfo.dataReplicationState will be set
* to DISCONNECTED; The totalStorageBytes property for each of dataReplicationInfo.replicatedDisks will be set to
* zero; dataReplicationInfo.lagDuration and dataReplicationInfo.lagDuration will be nullified.
*
*
* @param disconnectFromServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisconnectFromService operation returned by the service.
* @sample AWSmgnAsyncHandler.DisconnectFromService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disconnectFromServiceAsync(DisconnectFromServiceRequest disconnectFromServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Finalizes the cutover immediately for specific Source Servers. All AWS resources created by Application Migration
* Service for enabling the replication of these source servers will be terminated / deleted within 90 minutes.
* Launched Test or Cutover instances will NOT be terminated. The AWS Replication Agent will receive a command to
* uninstall itself (within 10 minutes). The following properties of the SourceServer will be changed immediately:
* dataReplicationInfo.dataReplicationState will be changed to DISCONNECTED; The SourceServer.lifeCycle.state will
* be changed to CUTOVER; The totalStorageBytes property fo each of dataReplicationInfo.replicatedDisks will be set
* to zero; dataReplicationInfo.lagDuration and dataReplicationInfo.lagDuration will be nullified.
*
*
* @param finalizeCutoverRequest
* @return A Java Future containing the result of the FinalizeCutover operation returned by the service.
* @sample AWSmgnAsync.FinalizeCutover
* @see AWS API
* Documentation
*/
java.util.concurrent.Future finalizeCutoverAsync(FinalizeCutoverRequest finalizeCutoverRequest);
/**
*
* Finalizes the cutover immediately for specific Source Servers. All AWS resources created by Application Migration
* Service for enabling the replication of these source servers will be terminated / deleted within 90 minutes.
* Launched Test or Cutover instances will NOT be terminated. The AWS Replication Agent will receive a command to
* uninstall itself (within 10 minutes). The following properties of the SourceServer will be changed immediately:
* dataReplicationInfo.dataReplicationState will be changed to DISCONNECTED; The SourceServer.lifeCycle.state will
* be changed to CUTOVER; The totalStorageBytes property fo each of dataReplicationInfo.replicatedDisks will be set
* to zero; dataReplicationInfo.lagDuration and dataReplicationInfo.lagDuration will be nullified.
*
*
* @param finalizeCutoverRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the FinalizeCutover operation returned by the service.
* @sample AWSmgnAsyncHandler.FinalizeCutover
* @see AWS API
* Documentation
*/
java.util.concurrent.Future finalizeCutoverAsync(FinalizeCutoverRequest finalizeCutoverRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all LaunchConfigurations available, filtered by Source Server IDs.
*
*
* @param getLaunchConfigurationRequest
* @return A Java Future containing the result of the GetLaunchConfiguration operation returned by the service.
* @sample AWSmgnAsync.GetLaunchConfiguration
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLaunchConfigurationAsync(GetLaunchConfigurationRequest getLaunchConfigurationRequest);
/**
*
* Lists all LaunchConfigurations available, filtered by Source Server IDs.
*
*
* @param getLaunchConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLaunchConfiguration operation returned by the service.
* @sample AWSmgnAsyncHandler.GetLaunchConfiguration
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLaunchConfigurationAsync(GetLaunchConfigurationRequest getLaunchConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all ReplicationConfigurations, filtered by Source Server ID.
*
*
* @param getReplicationConfigurationRequest
* @return A Java Future containing the result of the GetReplicationConfiguration operation returned by the service.
* @sample AWSmgnAsync.GetReplicationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getReplicationConfigurationAsync(
GetReplicationConfigurationRequest getReplicationConfigurationRequest);
/**
*
* Lists all ReplicationConfigurations, filtered by Source Server ID.
*
*
* @param getReplicationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetReplicationConfiguration operation returned by the service.
* @sample AWSmgnAsyncHandler.GetReplicationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getReplicationConfigurationAsync(
GetReplicationConfigurationRequest getReplicationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initialize Application Migration Service.
*
*
* @param initializeServiceRequest
* @return A Java Future containing the result of the InitializeService operation returned by the service.
* @sample AWSmgnAsync.InitializeService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future initializeServiceAsync(InitializeServiceRequest initializeServiceRequest);
/**
*
* Initialize Application Migration Service.
*
*
* @param initializeServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the InitializeService operation returned by the service.
* @sample AWSmgnAsyncHandler.InitializeService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future initializeServiceAsync(InitializeServiceRequest initializeServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves all applications or multiple applications by ID.
*
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AWSmgnAsync.ListApplications
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest);
/**
*
* Retrieves all applications or multiple applications by ID.
*
*
* @param listApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AWSmgnAsyncHandler.ListApplications
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List Connectors.
*
*
* @param listConnectorsRequest
* @return A Java Future containing the result of the ListConnectors operation returned by the service.
* @sample AWSmgnAsync.ListConnectors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listConnectorsAsync(ListConnectorsRequest listConnectorsRequest);
/**
*
* List Connectors.
*
*
* @param listConnectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListConnectors operation returned by the service.
* @sample AWSmgnAsyncHandler.ListConnectors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listConnectorsAsync(ListConnectorsRequest listConnectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List export errors.
*
*
* @param listExportErrorsRequest
* List export errors request.
* @return A Java Future containing the result of the ListExportErrors operation returned by the service.
* @sample AWSmgnAsync.ListExportErrors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listExportErrorsAsync(ListExportErrorsRequest listExportErrorsRequest);
/**
*
* List export errors.
*
*
* @param listExportErrorsRequest
* List export errors request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListExportErrors operation returned by the service.
* @sample AWSmgnAsyncHandler.ListExportErrors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listExportErrorsAsync(ListExportErrorsRequest listExportErrorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List exports.
*
*
* @param listExportsRequest
* List export request.
* @return A Java Future containing the result of the ListExports operation returned by the service.
* @sample AWSmgnAsync.ListExports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listExportsAsync(ListExportsRequest listExportsRequest);
/**
*
* List exports.
*
*
* @param listExportsRequest
* List export request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListExports operation returned by the service.
* @sample AWSmgnAsyncHandler.ListExports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listExportsAsync(ListExportsRequest listExportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List import errors.
*
*
* @param listImportErrorsRequest
* List import errors request.
* @return A Java Future containing the result of the ListImportErrors operation returned by the service.
* @sample AWSmgnAsync.ListImportErrors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportErrorsAsync(ListImportErrorsRequest listImportErrorsRequest);
/**
*
* List import errors.
*
*
* @param listImportErrorsRequest
* List import errors request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListImportErrors operation returned by the service.
* @sample AWSmgnAsyncHandler.ListImportErrors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportErrorsAsync(ListImportErrorsRequest listImportErrorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List imports.
*
*
* @param listImportsRequest
* List imports request.
* @return A Java Future containing the result of the ListImports operation returned by the service.
* @sample AWSmgnAsync.ListImports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportsAsync(ListImportsRequest listImportsRequest);
/**
*
* List imports.
*
*
* @param listImportsRequest
* List imports request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListImports operation returned by the service.
* @sample AWSmgnAsyncHandler.ListImports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportsAsync(ListImportsRequest listImportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List Managed Accounts.
*
*
* @param listManagedAccountsRequest
* List managed accounts request.
* @return A Java Future containing the result of the ListManagedAccounts operation returned by the service.
* @sample AWSmgnAsync.ListManagedAccounts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listManagedAccountsAsync(ListManagedAccountsRequest listManagedAccountsRequest);
/**
*
* List Managed Accounts.
*
*
* @param listManagedAccountsRequest
* List managed accounts request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListManagedAccounts operation returned by the service.
* @sample AWSmgnAsyncHandler.ListManagedAccounts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listManagedAccountsAsync(ListManagedAccountsRequest listManagedAccountsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List source server post migration custom actions.
*
*
* @param listSourceServerActionsRequest
* @return A Java Future containing the result of the ListSourceServerActions operation returned by the service.
* @sample AWSmgnAsync.ListSourceServerActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSourceServerActionsAsync(ListSourceServerActionsRequest listSourceServerActionsRequest);
/**
*
* List source server post migration custom actions.
*
*
* @param listSourceServerActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSourceServerActions operation returned by the service.
* @sample AWSmgnAsyncHandler.ListSourceServerActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSourceServerActionsAsync(ListSourceServerActionsRequest listSourceServerActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all tags for your Application Migration Service resources.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSmgnAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List all tags for your Application Migration Service resources.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSmgnAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List template post migration custom actions.
*
*
* @param listTemplateActionsRequest
* @return A Java Future containing the result of the ListTemplateActions operation returned by the service.
* @sample AWSmgnAsync.ListTemplateActions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTemplateActionsAsync(ListTemplateActionsRequest listTemplateActionsRequest);
/**
*
* List template post migration custom actions.
*
*
* @param listTemplateActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTemplateActions operation returned by the service.
* @sample AWSmgnAsyncHandler.ListTemplateActions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTemplateActionsAsync(ListTemplateActionsRequest listTemplateActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves all waves or multiple waves by ID.
*
*
* @param listWavesRequest
* @return A Java Future containing the result of the ListWaves operation returned by the service.
* @sample AWSmgnAsync.ListWaves
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listWavesAsync(ListWavesRequest listWavesRequest);
/**
*
* Retrieves all waves or multiple waves by ID.
*
*
* @param listWavesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWaves operation returned by the service.
* @sample AWSmgnAsyncHandler.ListWaves
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listWavesAsync(ListWavesRequest listWavesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Archives specific Source Servers by setting the SourceServer.isArchived property to true for specified
* SourceServers by ID. This command only works for SourceServers with a lifecycle. state which equals DISCONNECTED
* or CUTOVER.
*
*
* @param markAsArchivedRequest
* @return A Java Future containing the result of the MarkAsArchived operation returned by the service.
* @sample AWSmgnAsync.MarkAsArchived
* @see AWS API
* Documentation
*/
java.util.concurrent.Future markAsArchivedAsync(MarkAsArchivedRequest markAsArchivedRequest);
/**
*
* Archives specific Source Servers by setting the SourceServer.isArchived property to true for specified
* SourceServers by ID. This command only works for SourceServers with a lifecycle. state which equals DISCONNECTED
* or CUTOVER.
*
*
* @param markAsArchivedRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the MarkAsArchived operation returned by the service.
* @sample AWSmgnAsyncHandler.MarkAsArchived
* @see AWS API
* Documentation
*/
java.util.concurrent.Future markAsArchivedAsync(MarkAsArchivedRequest markAsArchivedRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Pause Replication.
*
*
* @param pauseReplicationRequest
* @return A Java Future containing the result of the PauseReplication operation returned by the service.
* @sample AWSmgnAsync.PauseReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future pauseReplicationAsync(PauseReplicationRequest pauseReplicationRequest);
/**
*
* Pause Replication.
*
*
* @param pauseReplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PauseReplication operation returned by the service.
* @sample AWSmgnAsyncHandler.PauseReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future pauseReplicationAsync(PauseReplicationRequest pauseReplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Put source server post migration custom action.
*
*
* @param putSourceServerActionRequest
* @return A Java Future containing the result of the PutSourceServerAction operation returned by the service.
* @sample AWSmgnAsync.PutSourceServerAction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putSourceServerActionAsync(PutSourceServerActionRequest putSourceServerActionRequest);
/**
*
* Put source server post migration custom action.
*
*
* @param putSourceServerActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutSourceServerAction operation returned by the service.
* @sample AWSmgnAsyncHandler.PutSourceServerAction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putSourceServerActionAsync(PutSourceServerActionRequest putSourceServerActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Put template post migration custom action.
*
*
* @param putTemplateActionRequest
* @return A Java Future containing the result of the PutTemplateAction operation returned by the service.
* @sample AWSmgnAsync.PutTemplateAction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTemplateActionAsync(PutTemplateActionRequest putTemplateActionRequest);
/**
*
* Put template post migration custom action.
*
*
* @param putTemplateActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutTemplateAction operation returned by the service.
* @sample AWSmgnAsyncHandler.PutTemplateAction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTemplateActionAsync(PutTemplateActionRequest putTemplateActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove source server post migration custom action.
*
*
* @param removeSourceServerActionRequest
* @return A Java Future containing the result of the RemoveSourceServerAction operation returned by the service.
* @sample AWSmgnAsync.RemoveSourceServerAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future removeSourceServerActionAsync(RemoveSourceServerActionRequest removeSourceServerActionRequest);
/**
*
* Remove source server post migration custom action.
*
*
* @param removeSourceServerActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveSourceServerAction operation returned by the service.
* @sample AWSmgnAsyncHandler.RemoveSourceServerAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future removeSourceServerActionAsync(RemoveSourceServerActionRequest removeSourceServerActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove template post migration custom action.
*
*
* @param removeTemplateActionRequest
* @return A Java Future containing the result of the RemoveTemplateAction operation returned by the service.
* @sample AWSmgnAsync.RemoveTemplateAction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTemplateActionAsync(RemoveTemplateActionRequest removeTemplateActionRequest);
/**
*
* Remove template post migration custom action.
*
*
* @param removeTemplateActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTemplateAction operation returned by the service.
* @sample AWSmgnAsyncHandler.RemoveTemplateAction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTemplateActionAsync(RemoveTemplateActionRequest removeTemplateActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Resume Replication.
*
*
* @param resumeReplicationRequest
* @return A Java Future containing the result of the ResumeReplication operation returned by the service.
* @sample AWSmgnAsync.ResumeReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resumeReplicationAsync(ResumeReplicationRequest resumeReplicationRequest);
/**
*
* Resume Replication.
*
*
* @param resumeReplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResumeReplication operation returned by the service.
* @sample AWSmgnAsyncHandler.ResumeReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resumeReplicationAsync(ResumeReplicationRequest resumeReplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Causes the data replication initiation sequence to begin immediately upon next Handshake for specified
* SourceServer IDs, regardless of when the previous initiation started. This command will not work if the
* SourceServer is not stalled or is in a DISCONNECTED or STOPPED state.
*
*
* @param retryDataReplicationRequest
* @return A Java Future containing the result of the RetryDataReplication operation returned by the service.
* @sample AWSmgnAsync.RetryDataReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future retryDataReplicationAsync(RetryDataReplicationRequest retryDataReplicationRequest);
/**
*
* Causes the data replication initiation sequence to begin immediately upon next Handshake for specified
* SourceServer IDs, regardless of when the previous initiation started. This command will not work if the
* SourceServer is not stalled or is in a DISCONNECTED or STOPPED state.
*
*
* @param retryDataReplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RetryDataReplication operation returned by the service.
* @sample AWSmgnAsyncHandler.RetryDataReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future retryDataReplicationAsync(RetryDataReplicationRequest retryDataReplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Launches a Cutover Instance for specific Source Servers. This command starts a LAUNCH job whose initiatedBy
* property is StartCutover and changes the SourceServer.lifeCycle.state property to CUTTING_OVER.
*
*
* @param startCutoverRequest
* @return A Java Future containing the result of the StartCutover operation returned by the service.
* @sample AWSmgnAsync.StartCutover
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startCutoverAsync(StartCutoverRequest startCutoverRequest);
/**
*
* Launches a Cutover Instance for specific Source Servers. This command starts a LAUNCH job whose initiatedBy
* property is StartCutover and changes the SourceServer.lifeCycle.state property to CUTTING_OVER.
*
*
* @param startCutoverRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartCutover operation returned by the service.
* @sample AWSmgnAsyncHandler.StartCutover
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startCutoverAsync(StartCutoverRequest startCutoverRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Start export.
*
*
* @param startExportRequest
* Start export request.
* @return A Java Future containing the result of the StartExport operation returned by the service.
* @sample AWSmgnAsync.StartExport
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startExportAsync(StartExportRequest startExportRequest);
/**
*
* Start export.
*
*
* @param startExportRequest
* Start export request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartExport operation returned by the service.
* @sample AWSmgnAsyncHandler.StartExport
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startExportAsync(StartExportRequest startExportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Start import.
*
*
* @param startImportRequest
* Start import request.
* @return A Java Future containing the result of the StartImport operation returned by the service.
* @sample AWSmgnAsync.StartImport
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startImportAsync(StartImportRequest startImportRequest);
/**
*
* Start import.
*
*
* @param startImportRequest
* Start import request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartImport operation returned by the service.
* @sample AWSmgnAsyncHandler.StartImport
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startImportAsync(StartImportRequest startImportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts replication for SNAPSHOT_SHIPPING agents.
*
*
* @param startReplicationRequest
* @return A Java Future containing the result of the StartReplication operation returned by the service.
* @sample AWSmgnAsync.StartReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startReplicationAsync(StartReplicationRequest startReplicationRequest);
/**
*
* Starts replication for SNAPSHOT_SHIPPING agents.
*
*
* @param startReplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartReplication operation returned by the service.
* @sample AWSmgnAsyncHandler.StartReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startReplicationAsync(StartReplicationRequest startReplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Launches a Test Instance for specific Source Servers. This command starts a LAUNCH job whose initiatedBy property
* is StartTest and changes the SourceServer.lifeCycle.state property to TESTING.
*
*
* @param startTestRequest
* @return A Java Future containing the result of the StartTest operation returned by the service.
* @sample AWSmgnAsync.StartTest
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startTestAsync(StartTestRequest startTestRequest);
/**
*
* Launches a Test Instance for specific Source Servers. This command starts a LAUNCH job whose initiatedBy property
* is StartTest and changes the SourceServer.lifeCycle.state property to TESTING.
*
*
* @param startTestRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartTest operation returned by the service.
* @sample AWSmgnAsyncHandler.StartTest
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startTestAsync(StartTestRequest startTestRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stop Replication.
*
*
* @param stopReplicationRequest
* @return A Java Future containing the result of the StopReplication operation returned by the service.
* @sample AWSmgnAsync.StopReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopReplicationAsync(StopReplicationRequest stopReplicationRequest);
/**
*
* Stop Replication.
*
*
* @param stopReplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopReplication operation returned by the service.
* @sample AWSmgnAsyncHandler.StopReplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopReplicationAsync(StopReplicationRequest stopReplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or overwrites only the specified tags for the specified Application Migration Service resource or resources.
* When you specify an existing tag key, the value is overwritten with the new value. Each resource can have a
* maximum of 50 tags. Each tag consists of a key and optional value.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSmgnAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds or overwrites only the specified tags for the specified Application Migration Service resource or resources.
* When you specify an existing tag key, the value is overwritten with the new value. Each resource can have a
* maximum of 50 tags. Each tag consists of a key and optional value.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSmgnAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a job that terminates specific launched EC2 Test and Cutover instances. This command will not work for any
* Source Server with a lifecycle.state of TESTING, CUTTING_OVER, or CUTOVER.
*
*
* @param terminateTargetInstancesRequest
* @return A Java Future containing the result of the TerminateTargetInstances operation returned by the service.
* @sample AWSmgnAsync.TerminateTargetInstances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future terminateTargetInstancesAsync(TerminateTargetInstancesRequest terminateTargetInstancesRequest);
/**
*
* Starts a job that terminates specific launched EC2 Test and Cutover instances. This command will not work for any
* Source Server with a lifecycle.state of TESTING, CUTTING_OVER, or CUTOVER.
*
*
* @param terminateTargetInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TerminateTargetInstances operation returned by the service.
* @sample AWSmgnAsyncHandler.TerminateTargetInstances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future terminateTargetInstancesAsync(TerminateTargetInstancesRequest terminateTargetInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Unarchive application.
*
*
* @param unarchiveApplicationRequest
* @return A Java Future containing the result of the UnarchiveApplication operation returned by the service.
* @sample AWSmgnAsync.UnarchiveApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future unarchiveApplicationAsync(UnarchiveApplicationRequest unarchiveApplicationRequest);
/**
*
* Unarchive application.
*
*
* @param unarchiveApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UnarchiveApplication operation returned by the service.
* @sample AWSmgnAsyncHandler.UnarchiveApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future unarchiveApplicationAsync(UnarchiveApplicationRequest unarchiveApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Unarchive wave.
*
*
* @param unarchiveWaveRequest
* @return A Java Future containing the result of the UnarchiveWave operation returned by the service.
* @sample AWSmgnAsync.UnarchiveWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future unarchiveWaveAsync(UnarchiveWaveRequest unarchiveWaveRequest);
/**
*
* Unarchive wave.
*
*
* @param unarchiveWaveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UnarchiveWave operation returned by the service.
* @sample AWSmgnAsyncHandler.UnarchiveWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future unarchiveWaveAsync(UnarchiveWaveRequest unarchiveWaveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified set of tags from the specified set of Application Migration Service resources.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSmgnAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Deletes the specified set of tags from the specified set of Application Migration Service resources.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSmgnAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update application.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AWSmgnAsync.UpdateApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Update application.
*
*
* @param updateApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AWSmgnAsyncHandler.UpdateApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update Connector.
*
*
* @param updateConnectorRequest
* @return A Java Future containing the result of the UpdateConnector operation returned by the service.
* @sample AWSmgnAsync.UpdateConnector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateConnectorAsync(UpdateConnectorRequest updateConnectorRequest);
/**
*
* Update Connector.
*
*
* @param updateConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateConnector operation returned by the service.
* @sample AWSmgnAsyncHandler.UpdateConnector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateConnectorAsync(UpdateConnectorRequest updateConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates multiple LaunchConfigurations by Source Server ID.
*
*
*
* bootMode valid values are LEGACY_BIOS | UEFI
*
*
*
* @param updateLaunchConfigurationRequest
* @return A Java Future containing the result of the UpdateLaunchConfiguration operation returned by the service.
* @sample AWSmgnAsync.UpdateLaunchConfiguration
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateLaunchConfigurationAsync(
UpdateLaunchConfigurationRequest updateLaunchConfigurationRequest);
/**
*
* Updates multiple LaunchConfigurations by Source Server ID.
*
*
*
* bootMode valid values are LEGACY_BIOS | UEFI
*
*
*
* @param updateLaunchConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLaunchConfiguration operation returned by the service.
* @sample AWSmgnAsyncHandler.UpdateLaunchConfiguration
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateLaunchConfigurationAsync(
UpdateLaunchConfigurationRequest updateLaunchConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing Launch Configuration Template by ID.
*
*
* @param updateLaunchConfigurationTemplateRequest
* @return A Java Future containing the result of the UpdateLaunchConfigurationTemplate operation returned by the
* service.
* @sample AWSmgnAsync.UpdateLaunchConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLaunchConfigurationTemplateAsync(
UpdateLaunchConfigurationTemplateRequest updateLaunchConfigurationTemplateRequest);
/**
*
* Updates an existing Launch Configuration Template by ID.
*
*
* @param updateLaunchConfigurationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLaunchConfigurationTemplate operation returned by the
* service.
* @sample AWSmgnAsyncHandler.UpdateLaunchConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLaunchConfigurationTemplateAsync(
UpdateLaunchConfigurationTemplateRequest updateLaunchConfigurationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allows you to update multiple ReplicationConfigurations by Source Server ID.
*
*
* @param updateReplicationConfigurationRequest
* @return A Java Future containing the result of the UpdateReplicationConfiguration operation returned by the
* service.
* @sample AWSmgnAsync.UpdateReplicationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateReplicationConfigurationAsync(
UpdateReplicationConfigurationRequest updateReplicationConfigurationRequest);
/**
*
* Allows you to update multiple ReplicationConfigurations by Source Server ID.
*
*
* @param updateReplicationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateReplicationConfiguration operation returned by the
* service.
* @sample AWSmgnAsyncHandler.UpdateReplicationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateReplicationConfigurationAsync(
UpdateReplicationConfigurationRequest updateReplicationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates multiple ReplicationConfigurationTemplates by ID.
*
*
* @param updateReplicationConfigurationTemplateRequest
* @return A Java Future containing the result of the UpdateReplicationConfigurationTemplate operation returned by
* the service.
* @sample AWSmgnAsync.UpdateReplicationConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateReplicationConfigurationTemplateAsync(
UpdateReplicationConfigurationTemplateRequest updateReplicationConfigurationTemplateRequest);
/**
*
* Updates multiple ReplicationConfigurationTemplates by ID.
*
*
* @param updateReplicationConfigurationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateReplicationConfigurationTemplate operation returned by
* the service.
* @sample AWSmgnAsyncHandler.UpdateReplicationConfigurationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateReplicationConfigurationTemplateAsync(
UpdateReplicationConfigurationTemplateRequest updateReplicationConfigurationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update Source Server.
*
*
* @param updateSourceServerRequest
* @return A Java Future containing the result of the UpdateSourceServer operation returned by the service.
* @sample AWSmgnAsync.UpdateSourceServer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSourceServerAsync(UpdateSourceServerRequest updateSourceServerRequest);
/**
*
* Update Source Server.
*
*
* @param updateSourceServerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSourceServer operation returned by the service.
* @sample AWSmgnAsyncHandler.UpdateSourceServer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSourceServerAsync(UpdateSourceServerRequest updateSourceServerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allows you to change between the AGENT_BASED replication type and the SNAPSHOT_SHIPPING replication type.
*
*
* @param updateSourceServerReplicationTypeRequest
* @return A Java Future containing the result of the UpdateSourceServerReplicationType operation returned by the
* service.
* @sample AWSmgnAsync.UpdateSourceServerReplicationType
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSourceServerReplicationTypeAsync(
UpdateSourceServerReplicationTypeRequest updateSourceServerReplicationTypeRequest);
/**
*
* Allows you to change between the AGENT_BASED replication type and the SNAPSHOT_SHIPPING replication type.
*
*
* @param updateSourceServerReplicationTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSourceServerReplicationType operation returned by the
* service.
* @sample AWSmgnAsyncHandler.UpdateSourceServerReplicationType
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSourceServerReplicationTypeAsync(
UpdateSourceServerReplicationTypeRequest updateSourceServerReplicationTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update wave.
*
*
* @param updateWaveRequest
* @return A Java Future containing the result of the UpdateWave operation returned by the service.
* @sample AWSmgnAsync.UpdateWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateWaveAsync(UpdateWaveRequest updateWaveRequest);
/**
*
* Update wave.
*
*
* @param updateWaveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateWave operation returned by the service.
* @sample AWSmgnAsyncHandler.UpdateWave
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateWaveAsync(UpdateWaveRequest updateWaveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}