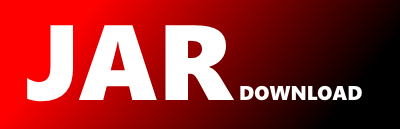
com.amazonaws.services.neptune.AmazonNeptuneAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-neptune Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.neptune;
import javax.annotation.Generated;
import com.amazonaws.services.neptune.model.*;
/**
* Interface for accessing Amazon Neptune asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.neptune.AbstractAmazonNeptuneAsync} instead.
*
*
* Amazon Neptune
*
* Amazon Neptune is a fast, reliable, fully-managed graph database service that makes it easy to build and run
* applications that work with highly connected datasets. The core of Amazon Neptune is a purpose-built,
* high-performance graph database engine optimized for storing billions of relationships and querying the graph with
* milliseconds latency. Amazon Neptune supports popular graph models Property Graph and W3C's RDF, and their respective
* query languages Apache TinkerPop Gremlin and SPARQL, allowing you to easily build queries that efficiently navigate
* highly connected datasets. Neptune powers graph use cases such as recommendation engines, fraud detection, knowledge
* graphs, drug discovery, and network security.
*
*
* This interface reference for Amazon Neptune contains documentation for a programming or command line interface you
* can use to manage Amazon Neptune. Note that Amazon Neptune is asynchronous, which means that some interfaces might
* require techniques such as polling or callback functions to determine when a command has been applied. In this
* reference, the parameter descriptions indicate whether a command is applied immediately, on the next instance reboot,
* or during the maintenance window. The reference structure is as follows, and we list following some related topics
* from the user guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonNeptuneAsync extends AmazonNeptune {
/**
*
* Associates an Identity and Access Management (IAM) role with an Neptune DB cluster.
*
*
* @param addRoleToDBClusterRequest
* @return A Java Future containing the result of the AddRoleToDBCluster operation returned by the service.
* @sample AmazonNeptuneAsync.AddRoleToDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addRoleToDBClusterAsync(AddRoleToDBClusterRequest addRoleToDBClusterRequest);
/**
*
* Associates an Identity and Access Management (IAM) role with an Neptune DB cluster.
*
*
* @param addRoleToDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddRoleToDBCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.AddRoleToDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addRoleToDBClusterAsync(AddRoleToDBClusterRequest addRoleToDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a source identifier to an existing event notification subscription.
*
*
* @param addSourceIdentifierToSubscriptionRequest
* @return A Java Future containing the result of the AddSourceIdentifierToSubscription operation returned by the
* service.
* @sample AmazonNeptuneAsync.AddSourceIdentifierToSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future addSourceIdentifierToSubscriptionAsync(
AddSourceIdentifierToSubscriptionRequest addSourceIdentifierToSubscriptionRequest);
/**
*
* Adds a source identifier to an existing event notification subscription.
*
*
* @param addSourceIdentifierToSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddSourceIdentifierToSubscription operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.AddSourceIdentifierToSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future addSourceIdentifierToSubscriptionAsync(
AddSourceIdentifierToSubscriptionRequest addSourceIdentifierToSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds metadata tags to an Amazon Neptune resource. These tags can also be used with cost allocation reporting to
* track cost associated with Amazon Neptune resources, or used in a Condition statement in an IAM policy for Amazon
* Neptune.
*
*
* @param addTagsToResourceRequest
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AmazonNeptuneAsync.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Adds metadata tags to an Amazon Neptune resource. These tags can also be used with cost allocation reporting to
* track cost associated with Amazon Neptune resources, or used in a Condition statement in an IAM policy for Amazon
* Neptune.
*
*
* @param addTagsToResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies a pending maintenance action to a resource (for example, to a DB instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* @sample AmazonNeptuneAsync.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future applyPendingMaintenanceActionAsync(
ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest);
/**
*
* Applies a pending maintenance action to a resource (for example, to a DB instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future applyPendingMaintenanceActionAsync(
ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified DB cluster parameter group.
*
*
* @param copyDBClusterParameterGroupRequest
* @return A Java Future containing the result of the CopyDBClusterParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsync.CopyDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future copyDBClusterParameterGroupAsync(CopyDBClusterParameterGroupRequest copyDBClusterParameterGroupRequest);
/**
*
* Copies the specified DB cluster parameter group.
*
*
* @param copyDBClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyDBClusterParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CopyDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future copyDBClusterParameterGroupAsync(
CopyDBClusterParameterGroupRequest copyDBClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies a snapshot of a DB cluster.
*
*
* To copy a DB cluster snapshot from a shared manual DB cluster snapshot,
* SourceDBClusterSnapshotIdentifier
must be the Amazon Resource Name (ARN) of the shared DB cluster
* snapshot.
*
*
* @param copyDBClusterSnapshotRequest
* @return A Java Future containing the result of the CopyDBClusterSnapshot operation returned by the service.
* @sample AmazonNeptuneAsync.CopyDBClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyDBClusterSnapshotAsync(CopyDBClusterSnapshotRequest copyDBClusterSnapshotRequest);
/**
*
* Copies a snapshot of a DB cluster.
*
*
* To copy a DB cluster snapshot from a shared manual DB cluster snapshot,
* SourceDBClusterSnapshotIdentifier
must be the Amazon Resource Name (ARN) of the shared DB cluster
* snapshot.
*
*
* @param copyDBClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyDBClusterSnapshot operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CopyDBClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyDBClusterSnapshotAsync(CopyDBClusterSnapshotRequest copyDBClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified DB parameter group.
*
*
* @param copyDBParameterGroupRequest
* @return A Java Future containing the result of the CopyDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsync.CopyDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyDBParameterGroupAsync(CopyDBParameterGroupRequest copyDBParameterGroupRequest);
/**
*
* Copies the specified DB parameter group.
*
*
* @param copyDBParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CopyDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyDBParameterGroupAsync(CopyDBParameterGroupRequest copyDBParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Neptune DB cluster.
*
*
* You can use the ReplicationSourceIdentifier
parameter to create the DB cluster as a Read Replica of
* another DB cluster or Amazon Neptune DB instance.
*
*
* Note that when you create a new cluster using CreateDBCluster
directly, deletion protection is
* disabled by default (when you create a new production cluster in the console, deletion protection is enabled by
* default). You can only delete a DB cluster if its DeletionProtection
field is set to
* false
.
*
*
* @param createDBClusterRequest
* @return A Java Future containing the result of the CreateDBCluster operation returned by the service.
* @sample AmazonNeptuneAsync.CreateDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBClusterAsync(CreateDBClusterRequest createDBClusterRequest);
/**
*
* Creates a new Amazon Neptune DB cluster.
*
*
* You can use the ReplicationSourceIdentifier
parameter to create the DB cluster as a Read Replica of
* another DB cluster or Amazon Neptune DB instance.
*
*
* Note that when you create a new cluster using CreateDBCluster
directly, deletion protection is
* disabled by default (when you create a new production cluster in the console, deletion protection is enabled by
* default). You can only delete a DB cluster if its DeletionProtection
field is set to
* false
.
*
*
* @param createDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CreateDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBClusterAsync(CreateDBClusterRequest createDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new custom endpoint and associates it with an Amazon Neptune DB cluster.
*
*
* @param createDBClusterEndpointRequest
* @return A Java Future containing the result of the CreateDBClusterEndpoint operation returned by the service.
* @sample AmazonNeptuneAsync.CreateDBClusterEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBClusterEndpointAsync(CreateDBClusterEndpointRequest createDBClusterEndpointRequest);
/**
*
* Creates a new custom endpoint and associates it with an Amazon Neptune DB cluster.
*
*
* @param createDBClusterEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBClusterEndpoint operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CreateDBClusterEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBClusterEndpointAsync(CreateDBClusterEndpointRequest createDBClusterEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB cluster parameter group.
*
*
* Parameters in a DB cluster parameter group apply to all of the instances in a DB cluster.
*
*
* A DB cluster parameter group is initially created with the default parameters for the database engine used by
* instances in the DB cluster. To provide custom values for any of the parameters, you must modify the group after
* creating it using ModifyDBClusterParameterGroup. Once you've created a DB cluster parameter group, you
* need to associate it with your DB cluster using ModifyDBCluster. When you associate a new DB cluster
* parameter group with a running DB cluster, you need to reboot the DB instances in the DB cluster without failover
* for the new DB cluster parameter group and associated settings to take effect.
*
*
*
* After you create a DB cluster parameter group, you should wait at least 5 minutes before creating your first DB
* cluster that uses that DB cluster parameter group as the default parameter group. This allows Amazon Neptune to
* fully complete the create action before the DB cluster parameter group is used as the default for a new DB
* cluster. This is especially important for parameters that are critical when creating the default database for a
* DB cluster, such as the character set for the default database defined by the character_set_database
* parameter. You can use the Parameter Groups option of the Amazon Neptune console or the DescribeDBClusterParameters
* command to verify that your DB cluster parameter group has been created or modified.
*
*
*
* @param createDBClusterParameterGroupRequest
* @return A Java Future containing the result of the CreateDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonNeptuneAsync.CreateDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBClusterParameterGroupAsync(
CreateDBClusterParameterGroupRequest createDBClusterParameterGroupRequest);
/**
*
* Creates a new DB cluster parameter group.
*
*
* Parameters in a DB cluster parameter group apply to all of the instances in a DB cluster.
*
*
* A DB cluster parameter group is initially created with the default parameters for the database engine used by
* instances in the DB cluster. To provide custom values for any of the parameters, you must modify the group after
* creating it using ModifyDBClusterParameterGroup. Once you've created a DB cluster parameter group, you
* need to associate it with your DB cluster using ModifyDBCluster. When you associate a new DB cluster
* parameter group with a running DB cluster, you need to reboot the DB instances in the DB cluster without failover
* for the new DB cluster parameter group and associated settings to take effect.
*
*
*
* After you create a DB cluster parameter group, you should wait at least 5 minutes before creating your first DB
* cluster that uses that DB cluster parameter group as the default parameter group. This allows Amazon Neptune to
* fully complete the create action before the DB cluster parameter group is used as the default for a new DB
* cluster. This is especially important for parameters that are critical when creating the default database for a
* DB cluster, such as the character set for the default database defined by the character_set_database
* parameter. You can use the Parameter Groups option of the Amazon Neptune console or the DescribeDBClusterParameters
* command to verify that your DB cluster parameter group has been created or modified.
*
*
*
* @param createDBClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.CreateDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBClusterParameterGroupAsync(
CreateDBClusterParameterGroupRequest createDBClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a snapshot of a DB cluster.
*
*
* @param createDBClusterSnapshotRequest
* @return A Java Future containing the result of the CreateDBClusterSnapshot operation returned by the service.
* @sample AmazonNeptuneAsync.CreateDBClusterSnapshot
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBClusterSnapshotAsync(CreateDBClusterSnapshotRequest createDBClusterSnapshotRequest);
/**
*
* Creates a snapshot of a DB cluster.
*
*
* @param createDBClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBClusterSnapshot operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CreateDBClusterSnapshot
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBClusterSnapshotAsync(CreateDBClusterSnapshotRequest createDBClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB instance.
*
*
* @param createDBInstanceRequest
* @return A Java Future containing the result of the CreateDBInstance operation returned by the service.
* @sample AmazonNeptuneAsync.CreateDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBInstanceAsync(CreateDBInstanceRequest createDBInstanceRequest);
/**
*
* Creates a new DB instance.
*
*
* @param createDBInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBInstance operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CreateDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBInstanceAsync(CreateDBInstanceRequest createDBInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB parameter group.
*
*
* A DB parameter group is initially created with the default parameters for the database engine used by the DB
* instance. To provide custom values for any of the parameters, you must modify the group after creating it using
* ModifyDBParameterGroup. Once you've created a DB parameter group, you need to associate it with your DB
* instance using ModifyDBInstance. When you associate a new DB parameter group with a running DB instance,
* you need to reboot the DB instance without failover for the new DB parameter group and associated settings to
* take effect.
*
*
*
* After you create a DB parameter group, you should wait at least 5 minutes before creating your first DB instance
* that uses that DB parameter group as the default parameter group. This allows Amazon Neptune to fully complete
* the create action before the parameter group is used as the default for a new DB instance. This is especially
* important for parameters that are critical when creating the default database for a DB instance, such as the
* character set for the default database defined by the character_set_database
parameter. You can use
* the Parameter Groups option of the Amazon Neptune console or the DescribeDBParameters command to
* verify that your DB parameter group has been created or modified.
*
*
*
* @param createDBParameterGroupRequest
* @return A Java Future containing the result of the CreateDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsync.CreateDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDBParameterGroupAsync(CreateDBParameterGroupRequest createDBParameterGroupRequest);
/**
*
* Creates a new DB parameter group.
*
*
* A DB parameter group is initially created with the default parameters for the database engine used by the DB
* instance. To provide custom values for any of the parameters, you must modify the group after creating it using
* ModifyDBParameterGroup. Once you've created a DB parameter group, you need to associate it with your DB
* instance using ModifyDBInstance. When you associate a new DB parameter group with a running DB instance,
* you need to reboot the DB instance without failover for the new DB parameter group and associated settings to
* take effect.
*
*
*
* After you create a DB parameter group, you should wait at least 5 minutes before creating your first DB instance
* that uses that DB parameter group as the default parameter group. This allows Amazon Neptune to fully complete
* the create action before the parameter group is used as the default for a new DB instance. This is especially
* important for parameters that are critical when creating the default database for a DB instance, such as the
* character set for the default database defined by the character_set_database
parameter. You can use
* the Parameter Groups option of the Amazon Neptune console or the DescribeDBParameters command to
* verify that your DB parameter group has been created or modified.
*
*
*
* @param createDBParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CreateDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDBParameterGroupAsync(CreateDBParameterGroupRequest createDBParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB subnet group. DB subnet groups must contain at least one subnet in at least two AZs in the
* Amazon Region.
*
*
* @param createDBSubnetGroupRequest
* @return A Java Future containing the result of the CreateDBSubnetGroup operation returned by the service.
* @sample AmazonNeptuneAsync.CreateDBSubnetGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDBSubnetGroupAsync(CreateDBSubnetGroupRequest createDBSubnetGroupRequest);
/**
*
* Creates a new DB subnet group. DB subnet groups must contain at least one subnet in at least two AZs in the
* Amazon Region.
*
*
* @param createDBSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBSubnetGroup operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CreateDBSubnetGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDBSubnetGroupAsync(CreateDBSubnetGroupRequest createDBSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an event notification subscription. This action requires a topic ARN (Amazon Resource Name) created by
* either the Neptune console, the SNS console, or the SNS API. To obtain an ARN with SNS, you must create a topic
* in Amazon SNS and subscribe to the topic. The ARN is displayed in the SNS console.
*
*
* You can specify the type of source (SourceType) you want to be notified of, provide a list of Neptune sources
* (SourceIds) that triggers the events, and provide a list of event categories (EventCategories) for events you
* want to be notified of. For example, you can specify SourceType = db-instance, SourceIds = mydbinstance1,
* mydbinstance2 and EventCategories = Availability, Backup.
*
*
* If you specify both the SourceType and SourceIds, such as SourceType = db-instance and SourceIdentifier =
* myDBInstance1, you are notified of all the db-instance events for the specified source. If you specify a
* SourceType but do not specify a SourceIdentifier, you receive notice of the events for that source type for all
* your Neptune sources. If you do not specify either the SourceType nor the SourceIdentifier, you are notified of
* events generated from all Neptune sources belonging to your customer account.
*
*
* @param createEventSubscriptionRequest
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AmazonNeptuneAsync.CreateEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest);
/**
*
* Creates an event notification subscription. This action requires a topic ARN (Amazon Resource Name) created by
* either the Neptune console, the SNS console, or the SNS API. To obtain an ARN with SNS, you must create a topic
* in Amazon SNS and subscribe to the topic. The ARN is displayed in the SNS console.
*
*
* You can specify the type of source (SourceType) you want to be notified of, provide a list of Neptune sources
* (SourceIds) that triggers the events, and provide a list of event categories (EventCategories) for events you
* want to be notified of. For example, you can specify SourceType = db-instance, SourceIds = mydbinstance1,
* mydbinstance2 and EventCategories = Availability, Backup.
*
*
* If you specify both the SourceType and SourceIds, such as SourceType = db-instance and SourceIdentifier =
* myDBInstance1, you are notified of all the db-instance events for the specified source. If you specify a
* SourceType but do not specify a SourceIdentifier, you receive notice of the events for that source type for all
* your Neptune sources. If you do not specify either the SourceType nor the SourceIdentifier, you are notified of
* events generated from all Neptune sources belonging to your customer account.
*
*
* @param createEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CreateEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Neptune global database spread across multiple Amazon Regions. The global database contains a single
* primary cluster with read-write capability, and read-only secondary clusters that receive data from the primary
* cluster through high-speed replication performed by the Neptune storage subsystem.
*
*
* You can create a global database that is initially empty, and then add a primary cluster and secondary clusters
* to it, or you can specify an existing Neptune cluster during the create operation to become the primary cluster
* of the global database.
*
*
* @param createGlobalClusterRequest
* @return A Java Future containing the result of the CreateGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsync.CreateGlobalCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGlobalClusterAsync(CreateGlobalClusterRequest createGlobalClusterRequest);
/**
*
* Creates a Neptune global database spread across multiple Amazon Regions. The global database contains a single
* primary cluster with read-write capability, and read-only secondary clusters that receive data from the primary
* cluster through high-speed replication performed by the Neptune storage subsystem.
*
*
* You can create a global database that is initially empty, and then add a primary cluster and secondary clusters
* to it, or you can specify an existing Neptune cluster during the create operation to become the primary cluster
* of the global database.
*
*
* @param createGlobalClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.CreateGlobalCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGlobalClusterAsync(CreateGlobalClusterRequest createGlobalClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteDBCluster action deletes a previously provisioned DB cluster. When you delete a DB cluster, all
* automated backups for that DB cluster are deleted and can't be recovered. Manual DB cluster snapshots of the
* specified DB cluster are not deleted.
*
*
* Note that the DB Cluster cannot be deleted if deletion protection is enabled. To delete it, you must first set
* its DeletionProtection
field to False
.
*
*
* @param deleteDBClusterRequest
* @return A Java Future containing the result of the DeleteDBCluster operation returned by the service.
* @sample AmazonNeptuneAsync.DeleteDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBClusterAsync(DeleteDBClusterRequest deleteDBClusterRequest);
/**
*
* The DeleteDBCluster action deletes a previously provisioned DB cluster. When you delete a DB cluster, all
* automated backups for that DB cluster are deleted and can't be recovered. Manual DB cluster snapshots of the
* specified DB cluster are not deleted.
*
*
* Note that the DB Cluster cannot be deleted if deletion protection is enabled. To delete it, you must first set
* its DeletionProtection
field to False
.
*
*
* @param deleteDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DeleteDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBClusterAsync(DeleteDBClusterRequest deleteDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom endpoint and removes it from an Amazon Neptune DB cluster.
*
*
* @param deleteDBClusterEndpointRequest
* @return A Java Future containing the result of the DeleteDBClusterEndpoint operation returned by the service.
* @sample AmazonNeptuneAsync.DeleteDBClusterEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBClusterEndpointAsync(DeleteDBClusterEndpointRequest deleteDBClusterEndpointRequest);
/**
*
* Deletes a custom endpoint and removes it from an Amazon Neptune DB cluster.
*
*
* @param deleteDBClusterEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBClusterEndpoint operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DeleteDBClusterEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBClusterEndpointAsync(DeleteDBClusterEndpointRequest deleteDBClusterEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specified DB cluster parameter group. The DB cluster parameter group to be deleted can't be associated
* with any DB clusters.
*
*
* @param deleteDBClusterParameterGroupRequest
* @return A Java Future containing the result of the DeleteDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonNeptuneAsync.DeleteDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBClusterParameterGroupAsync(
DeleteDBClusterParameterGroupRequest deleteDBClusterParameterGroupRequest);
/**
*
* Deletes a specified DB cluster parameter group. The DB cluster parameter group to be deleted can't be associated
* with any DB clusters.
*
*
* @param deleteDBClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.DeleteDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBClusterParameterGroupAsync(
DeleteDBClusterParameterGroupRequest deleteDBClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a DB cluster snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB cluster snapshot must be in the available
state to be deleted.
*
*
*
* @param deleteDBClusterSnapshotRequest
* @return A Java Future containing the result of the DeleteDBClusterSnapshot operation returned by the service.
* @sample AmazonNeptuneAsync.DeleteDBClusterSnapshot
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBClusterSnapshotAsync(DeleteDBClusterSnapshotRequest deleteDBClusterSnapshotRequest);
/**
*
* Deletes a DB cluster snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB cluster snapshot must be in the available
state to be deleted.
*
*
*
* @param deleteDBClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBClusterSnapshot operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DeleteDBClusterSnapshot
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBClusterSnapshotAsync(DeleteDBClusterSnapshotRequest deleteDBClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteDBInstance action deletes a previously provisioned DB instance. When you delete a DB instance, all
* automated backups for that instance are deleted and can't be recovered. Manual DB snapshots of the DB instance to
* be deleted by DeleteDBInstance
are not deleted.
*
*
* If you request a final DB snapshot the status of the Amazon Neptune DB instance is deleting
until
* the DB snapshot is created. The API action DescribeDBInstance
is used to monitor the status of this
* operation. The action can't be canceled or reverted once submitted.
*
*
* Note that when a DB instance is in a failure state and has a status of failed
,
* incompatible-restore
, or incompatible-network
, you can only delete it when the
* SkipFinalSnapshot
parameter is set to true
.
*
*
* You can't delete a DB instance if it is the only instance in the DB cluster, or if it has deletion protection
* enabled.
*
*
* @param deleteDBInstanceRequest
* @return A Java Future containing the result of the DeleteDBInstance operation returned by the service.
* @sample AmazonNeptuneAsync.DeleteDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBInstanceAsync(DeleteDBInstanceRequest deleteDBInstanceRequest);
/**
*
* The DeleteDBInstance action deletes a previously provisioned DB instance. When you delete a DB instance, all
* automated backups for that instance are deleted and can't be recovered. Manual DB snapshots of the DB instance to
* be deleted by DeleteDBInstance
are not deleted.
*
*
* If you request a final DB snapshot the status of the Amazon Neptune DB instance is deleting
until
* the DB snapshot is created. The API action DescribeDBInstance
is used to monitor the status of this
* operation. The action can't be canceled or reverted once submitted.
*
*
* Note that when a DB instance is in a failure state and has a status of failed
,
* incompatible-restore
, or incompatible-network
, you can only delete it when the
* SkipFinalSnapshot
parameter is set to true
.
*
*
* You can't delete a DB instance if it is the only instance in the DB cluster, or if it has deletion protection
* enabled.
*
*
* @param deleteDBInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBInstance operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DeleteDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBInstanceAsync(DeleteDBInstanceRequest deleteDBInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specified DBParameterGroup. The DBParameterGroup to be deleted can't be associated with any DB
* instances.
*
*
* @param deleteDBParameterGroupRequest
* @return A Java Future containing the result of the DeleteDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsync.DeleteDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDBParameterGroupAsync(DeleteDBParameterGroupRequest deleteDBParameterGroupRequest);
/**
*
* Deletes a specified DBParameterGroup. The DBParameterGroup to be deleted can't be associated with any DB
* instances.
*
*
* @param deleteDBParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DeleteDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDBParameterGroupAsync(DeleteDBParameterGroupRequest deleteDBParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a DB subnet group.
*
*
*
* The specified database subnet group must not be associated with any DB instances.
*
*
*
* @param deleteDBSubnetGroupRequest
* @return A Java Future containing the result of the DeleteDBSubnetGroup operation returned by the service.
* @sample AmazonNeptuneAsync.DeleteDBSubnetGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDBSubnetGroupAsync(DeleteDBSubnetGroupRequest deleteDBSubnetGroupRequest);
/**
*
* Deletes a DB subnet group.
*
*
*
* The specified database subnet group must not be associated with any DB instances.
*
*
*
* @param deleteDBSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBSubnetGroup operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DeleteDBSubnetGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDBSubnetGroupAsync(DeleteDBSubnetGroupRequest deleteDBSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AmazonNeptuneAsync.DeleteEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest);
/**
*
* Deletes an event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DeleteEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a global database. The primary and all secondary clusters must already be detached or deleted first.
*
*
* @param deleteGlobalClusterRequest
* @return A Java Future containing the result of the DeleteGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsync.DeleteGlobalCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteGlobalClusterAsync(DeleteGlobalClusterRequest deleteGlobalClusterRequest);
/**
*
* Deletes a global database. The primary and all secondary clusters must already be detached or deleted first.
*
*
* @param deleteGlobalClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DeleteGlobalCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteGlobalClusterAsync(DeleteGlobalClusterRequest deleteGlobalClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about endpoints for an Amazon Neptune DB cluster.
*
*
*
* This operation can also return information for Amazon RDS clusters and Amazon DocDB clusters.
*
*
*
* @param describeDBClusterEndpointsRequest
* @return A Java Future containing the result of the DescribeDBClusterEndpoints operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeDBClusterEndpoints
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterEndpointsAsync(
DescribeDBClusterEndpointsRequest describeDBClusterEndpointsRequest);
/**
*
* Returns information about endpoints for an Amazon Neptune DB cluster.
*
*
*
* This operation can also return information for Amazon RDS clusters and Amazon DocDB clusters.
*
*
*
* @param describeDBClusterEndpointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterEndpoints operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBClusterEndpoints
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterEndpointsAsync(
DescribeDBClusterEndpointsRequest describeDBClusterEndpointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* @param describeDBClusterParameterGroupsRequest
* @return A Java Future containing the result of the DescribeDBClusterParameterGroups operation returned by the
* service.
* @sample AmazonNeptuneAsync.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterParameterGroupsAsync(
DescribeDBClusterParameterGroupsRequest describeDBClusterParameterGroupsRequest);
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* @param describeDBClusterParameterGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterParameterGroups operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterParameterGroupsAsync(
DescribeDBClusterParameterGroupsRequest describeDBClusterParameterGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the detailed parameter list for a particular DB cluster parameter group.
*
*
* @param describeDBClusterParametersRequest
* @return A Java Future containing the result of the DescribeDBClusterParameters operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeDBClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterParametersAsync(
DescribeDBClusterParametersRequest describeDBClusterParametersRequest);
/**
*
* Returns the detailed parameter list for a particular DB cluster parameter group.
*
*
* @param describeDBClusterParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterParameters operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterParametersAsync(
DescribeDBClusterParametersRequest describeDBClusterParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DB cluster snapshot attribute names and values for a manual DB cluster snapshot.
*
*
* When sharing snapshots with other Amazon accounts, DescribeDBClusterSnapshotAttributes
returns the
* restore
attribute and a list of IDs for the Amazon accounts that are authorized to copy or restore
* the manual DB cluster snapshot. If all
is included in the list of values for the
* restore
attribute, then the manual DB cluster snapshot is public and can be copied or restored by
* all Amazon accounts.
*
*
* To add or remove access for an Amazon account to copy or restore a manual DB cluster snapshot, or to make the
* manual DB cluster snapshot public or private, use the ModifyDBClusterSnapshotAttribute API action.
*
*
* @param describeDBClusterSnapshotAttributesRequest
* @return A Java Future containing the result of the DescribeDBClusterSnapshotAttributes operation returned by the
* service.
* @sample AmazonNeptuneAsync.DescribeDBClusterSnapshotAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterSnapshotAttributesAsync(
DescribeDBClusterSnapshotAttributesRequest describeDBClusterSnapshotAttributesRequest);
/**
*
* Returns a list of DB cluster snapshot attribute names and values for a manual DB cluster snapshot.
*
*
* When sharing snapshots with other Amazon accounts, DescribeDBClusterSnapshotAttributes
returns the
* restore
attribute and a list of IDs for the Amazon accounts that are authorized to copy or restore
* the manual DB cluster snapshot. If all
is included in the list of values for the
* restore
attribute, then the manual DB cluster snapshot is public and can be copied or restored by
* all Amazon accounts.
*
*
* To add or remove access for an Amazon account to copy or restore a manual DB cluster snapshot, or to make the
* manual DB cluster snapshot public or private, use the ModifyDBClusterSnapshotAttribute API action.
*
*
* @param describeDBClusterSnapshotAttributesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterSnapshotAttributes operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBClusterSnapshotAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterSnapshotAttributesAsync(
DescribeDBClusterSnapshotAttributesRequest describeDBClusterSnapshotAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* @param describeDBClusterSnapshotsRequest
* @return A Java Future containing the result of the DescribeDBClusterSnapshots operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeDBClusterSnapshots
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterSnapshotsAsync(
DescribeDBClusterSnapshotsRequest describeDBClusterSnapshotsRequest);
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* @param describeDBClusterSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterSnapshots operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBClusterSnapshots
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterSnapshotsAsync(
DescribeDBClusterSnapshotsRequest describeDBClusterSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about provisioned DB clusters, and supports pagination.
*
*
*
* This operation can also return information for Amazon RDS clusters and Amazon DocDB clusters.
*
*
*
* @param describeDBClustersRequest
* @return A Java Future containing the result of the DescribeDBClusters operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeDBClusters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBClustersAsync(DescribeDBClustersRequest describeDBClustersRequest);
/**
*
* Returns information about provisioned DB clusters, and supports pagination.
*
*
*
* This operation can also return information for Amazon RDS clusters and Amazon DocDB clusters.
*
*
*
* @param describeDBClustersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusters operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBClusters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBClustersAsync(DescribeDBClustersRequest describeDBClustersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the available DB engines.
*
*
* @param describeDBEngineVersionsRequest
* @return A Java Future containing the result of the DescribeDBEngineVersions operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeDBEngineVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBEngineVersionsAsync(DescribeDBEngineVersionsRequest describeDBEngineVersionsRequest);
/**
*
* Returns a list of the available DB engines.
*
*
* @param describeDBEngineVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBEngineVersions operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBEngineVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBEngineVersionsAsync(DescribeDBEngineVersionsRequest describeDBEngineVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about provisioned instances, and supports pagination.
*
*
*
* This operation can also return information for Amazon RDS instances and Amazon DocDB instances.
*
*
*
* @param describeDBInstancesRequest
* @return A Java Future containing the result of the DescribeDBInstances operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeDBInstances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBInstancesAsync(DescribeDBInstancesRequest describeDBInstancesRequest);
/**
*
* Returns information about provisioned instances, and supports pagination.
*
*
*
* This operation can also return information for Amazon RDS instances and Amazon DocDB instances.
*
*
*
* @param describeDBInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBInstances operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBInstances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBInstancesAsync(DescribeDBInstancesRequest describeDBInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
* @param describeDBParameterGroupsRequest
* @return A Java Future containing the result of the DescribeDBParameterGroups operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeDBParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBParameterGroupsAsync(
DescribeDBParameterGroupsRequest describeDBParameterGroupsRequest);
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
* @param describeDBParameterGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBParameterGroups operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBParameterGroupsAsync(
DescribeDBParameterGroupsRequest describeDBParameterGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the detailed parameter list for a particular DB parameter group.
*
*
* @param describeDBParametersRequest
* @return A Java Future containing the result of the DescribeDBParameters operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeDBParameters
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBParametersAsync(DescribeDBParametersRequest describeDBParametersRequest);
/**
*
* Returns the detailed parameter list for a particular DB parameter group.
*
*
* @param describeDBParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBParameters operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBParameters
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBParametersAsync(DescribeDBParametersRequest describeDBParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName is specified, the list will contain only the
* descriptions of the specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
* @param describeDBSubnetGroupsRequest
* @return A Java Future containing the result of the DescribeDBSubnetGroups operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeDBSubnetGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBSubnetGroupsAsync(DescribeDBSubnetGroupsRequest describeDBSubnetGroupsRequest);
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName is specified, the list will contain only the
* descriptions of the specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
* @param describeDBSubnetGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBSubnetGroups operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeDBSubnetGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBSubnetGroupsAsync(DescribeDBSubnetGroupsRequest describeDBSubnetGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the default engine and system parameter information for the cluster database engine.
*
*
* @param describeEngineDefaultClusterParametersRequest
* @return A Java Future containing the result of the DescribeEngineDefaultClusterParameters operation returned by
* the service.
* @sample AmazonNeptuneAsync.DescribeEngineDefaultClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEngineDefaultClusterParametersAsync(
DescribeEngineDefaultClusterParametersRequest describeEngineDefaultClusterParametersRequest);
/**
*
* Returns the default engine and system parameter information for the cluster database engine.
*
*
* @param describeEngineDefaultClusterParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEngineDefaultClusterParameters operation returned by
* the service.
* @sample AmazonNeptuneAsyncHandler.DescribeEngineDefaultClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEngineDefaultClusterParametersAsync(
DescribeEngineDefaultClusterParametersRequest describeEngineDefaultClusterParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the default engine and system parameter information for the specified database engine.
*
*
* @param describeEngineDefaultParametersRequest
* @return A Java Future containing the result of the DescribeEngineDefaultParameters operation returned by the
* service.
* @sample AmazonNeptuneAsync.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEngineDefaultParametersAsync(
DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest);
/**
*
* Returns the default engine and system parameter information for the specified database engine.
*
*
* @param describeEngineDefaultParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEngineDefaultParameters operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEngineDefaultParametersAsync(
DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays a list of categories for all event source types, or, if specified, for a specified source type.
*
*
* @param describeEventCategoriesRequest
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeEventCategories
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest);
/**
*
* Displays a list of categories for all event source types, or, if specified, for a specified source type.
*
*
* @param describeEventCategoriesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeEventCategories
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the subscription descriptions for a customer account. The description for a subscription includes
* SubscriptionName, SNSTopicARN, CustomerID, SourceType, SourceID, CreationTime, and Status.
*
*
* If you specify a SubscriptionName, lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest
* @return A Java Future containing the result of the DescribeEventSubscriptions operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeEventSubscriptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest);
/**
*
* Lists all the subscription descriptions for a customer account. The description for a subscription includes
* SubscriptionName, SNSTopicARN, CustomerID, SourceType, SourceID, CreationTime, and Status.
*
*
* If you specify a SubscriptionName, lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventSubscriptions operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeEventSubscriptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns events related to DB instances, DB security groups, DB snapshots, and DB parameter groups for the past 14
* days. Events specific to a particular DB instance, DB security group, database snapshot, or DB parameter group
* can be obtained by providing the name as a parameter. By default, the past hour of events are returned.
*
*
* @param describeEventsRequest
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest);
/**
*
* Returns events related to DB instances, DB security groups, DB snapshots, and DB parameter groups for the past 14
* days. Events specific to a particular DB instance, DB security group, database snapshot, or DB parameter group
* can be obtained by providing the name as a parameter. By default, the past hour of events are returned.
*
*
* @param describeEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about Neptune global database clusters. This API supports pagination.
*
*
* @param describeGlobalClustersRequest
* @return A Java Future containing the result of the DescribeGlobalClusters operation returned by the service.
* @sample AmazonNeptuneAsync.DescribeGlobalClusters
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeGlobalClustersAsync(DescribeGlobalClustersRequest describeGlobalClustersRequest);
/**
*
* Returns information about Neptune global database clusters. This API supports pagination.
*
*
* @param describeGlobalClustersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGlobalClusters operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.DescribeGlobalClusters
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeGlobalClustersAsync(DescribeGlobalClustersRequest describeGlobalClustersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of orderable DB instance options for the specified engine.
*
*
* @param describeOrderableDBInstanceOptionsRequest
* @return A Java Future containing the result of the DescribeOrderableDBInstanceOptions operation returned by the
* service.
* @sample AmazonNeptuneAsync.DescribeOrderableDBInstanceOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrderableDBInstanceOptionsAsync(
DescribeOrderableDBInstanceOptionsRequest describeOrderableDBInstanceOptionsRequest);
/**
*
* Returns a list of orderable DB instance options for the specified engine.
*
*
* @param describeOrderableDBInstanceOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOrderableDBInstanceOptions operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.DescribeOrderableDBInstanceOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrderableDBInstanceOptionsAsync(
DescribeOrderableDBInstanceOptionsRequest describeOrderableDBInstanceOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of resources (for example, DB instances) that have at least one pending maintenance action.
*
*
* @param describePendingMaintenanceActionsRequest
* @return A Java Future containing the result of the DescribePendingMaintenanceActions operation returned by the
* service.
* @sample AmazonNeptuneAsync.DescribePendingMaintenanceActions
* @see AWS API Documentation
*/
java.util.concurrent.Future describePendingMaintenanceActionsAsync(
DescribePendingMaintenanceActionsRequest describePendingMaintenanceActionsRequest);
/**
*
* Returns a list of resources (for example, DB instances) that have at least one pending maintenance action.
*
*
* @param describePendingMaintenanceActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePendingMaintenanceActions operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.DescribePendingMaintenanceActions
* @see AWS API Documentation
*/
java.util.concurrent.Future describePendingMaintenanceActionsAsync(
DescribePendingMaintenanceActionsRequest describePendingMaintenanceActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* You can call DescribeValidDBInstanceModifications to learn what modifications you can make to your DB
* instance. You can use this information when you call ModifyDBInstance.
*
*
* @param describeValidDBInstanceModificationsRequest
* @return A Java Future containing the result of the DescribeValidDBInstanceModifications operation returned by the
* service.
* @sample AmazonNeptuneAsync.DescribeValidDBInstanceModifications
* @see AWS API Documentation
*/
java.util.concurrent.Future describeValidDBInstanceModificationsAsync(
DescribeValidDBInstanceModificationsRequest describeValidDBInstanceModificationsRequest);
/**
*
* You can call DescribeValidDBInstanceModifications to learn what modifications you can make to your DB
* instance. You can use this information when you call ModifyDBInstance.
*
*
* @param describeValidDBInstanceModificationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeValidDBInstanceModifications operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.DescribeValidDBInstanceModifications
* @see AWS API Documentation
*/
java.util.concurrent.Future describeValidDBInstanceModificationsAsync(
DescribeValidDBInstanceModificationsRequest describeValidDBInstanceModificationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Forces a failover for a DB cluster.
*
*
* A failover for a DB cluster promotes one of the Read Replicas (read-only instances) in the DB cluster to be the
* primary instance (the cluster writer).
*
*
* Amazon Neptune will automatically fail over to a Read Replica, if one exists, when the primary instance fails.
* You can force a failover when you want to simulate a failure of a primary instance for testing. Because each
* instance in a DB cluster has its own endpoint address, you will need to clean up and re-establish any existing
* connections that use those endpoint addresses when the failover is complete.
*
*
* @param failoverDBClusterRequest
* @return A Java Future containing the result of the FailoverDBCluster operation returned by the service.
* @sample AmazonNeptuneAsync.FailoverDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future failoverDBClusterAsync(FailoverDBClusterRequest failoverDBClusterRequest);
/**
*
* Forces a failover for a DB cluster.
*
*
* A failover for a DB cluster promotes one of the Read Replicas (read-only instances) in the DB cluster to be the
* primary instance (the cluster writer).
*
*
* Amazon Neptune will automatically fail over to a Read Replica, if one exists, when the primary instance fails.
* You can force a failover when you want to simulate a failure of a primary instance for testing. Because each
* instance in a DB cluster has its own endpoint address, you will need to clean up and re-establish any existing
* connections that use those endpoint addresses when the failover is complete.
*
*
* @param failoverDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the FailoverDBCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.FailoverDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future failoverDBClusterAsync(FailoverDBClusterRequest failoverDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates the failover process for a Neptune global database.
*
*
* A failover for a Neptune global database promotes one of secondary read-only DB clusters to be the primary DB
* cluster and demotes the primary DB cluster to being a secondary (read-only) DB cluster. In other words, the role
* of the current primary DB cluster and the selected target secondary DB cluster are switched. The selected
* secondary DB cluster assumes full read/write capabilities for the Neptune global database.
*
*
*
* This action applies only to Neptune global databases. This action is only intended for use on healthy
* Neptune global databases with healthy Neptune DB clusters and no region-wide outages, to test disaster recovery
* scenarios or to reconfigure the global database topology.
*
*
*
* @param failoverGlobalClusterRequest
* @return A Java Future containing the result of the FailoverGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsync.FailoverGlobalCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future failoverGlobalClusterAsync(FailoverGlobalClusterRequest failoverGlobalClusterRequest);
/**
*
* Initiates the failover process for a Neptune global database.
*
*
* A failover for a Neptune global database promotes one of secondary read-only DB clusters to be the primary DB
* cluster and demotes the primary DB cluster to being a secondary (read-only) DB cluster. In other words, the role
* of the current primary DB cluster and the selected target secondary DB cluster are switched. The selected
* secondary DB cluster assumes full read/write capabilities for the Neptune global database.
*
*
*
* This action applies only to Neptune global databases. This action is only intended for use on healthy
* Neptune global databases with healthy Neptune DB clusters and no region-wide outages, to test disaster recovery
* scenarios or to reconfigure the global database topology.
*
*
*
* @param failoverGlobalClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the FailoverGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.FailoverGlobalCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future failoverGlobalClusterAsync(FailoverGlobalClusterRequest failoverGlobalClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags on an Amazon Neptune resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonNeptuneAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all tags on an Amazon Neptune resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modify a setting for a DB cluster. You can change one or more database configuration parameters by specifying
* these parameters and the new values in the request.
*
*
* @param modifyDBClusterRequest
* @return A Java Future containing the result of the ModifyDBCluster operation returned by the service.
* @sample AmazonNeptuneAsync.ModifyDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyDBClusterAsync(ModifyDBClusterRequest modifyDBClusterRequest);
/**
*
* Modify a setting for a DB cluster. You can change one or more database configuration parameters by specifying
* these parameters and the new values in the request.
*
*
* @param modifyDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyDBCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.ModifyDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyDBClusterAsync(ModifyDBClusterRequest modifyDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the properties of an endpoint in an Amazon Neptune DB cluster.
*
*
* @param modifyDBClusterEndpointRequest
* @return A Java Future containing the result of the ModifyDBClusterEndpoint operation returned by the service.
* @sample AmazonNeptuneAsync.ModifyDBClusterEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyDBClusterEndpointAsync(ModifyDBClusterEndpointRequest modifyDBClusterEndpointRequest);
/**
*
* Modifies the properties of an endpoint in an Amazon Neptune DB cluster.
*
*
* @param modifyDBClusterEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyDBClusterEndpoint operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.ModifyDBClusterEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyDBClusterEndpointAsync(ModifyDBClusterEndpointRequest modifyDBClusterEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the parameters of a DB cluster parameter group. To modify more than one parameter, submit a list of the
* following: ParameterName
, ParameterValue
, and ApplyMethod
. A maximum of 20
* parameters can be modified in a single request.
*
*
*
* Changes to dynamic parameters are applied immediately. Changes to static parameters require a reboot without
* failover to the DB cluster associated with the parameter group before the change can take effect.
*
*
*
* After you create a DB cluster parameter group, you should wait at least 5 minutes before creating your first DB
* cluster that uses that DB cluster parameter group as the default parameter group. This allows Amazon Neptune to
* fully complete the create action before the parameter group is used as the default for a new DB cluster. This is
* especially important for parameters that are critical when creating the default database for a DB cluster, such
* as the character set for the default database defined by the character_set_database
parameter. You
* can use the Parameter Groups option of the Amazon Neptune console or the
* DescribeDBClusterParameters command to verify that your DB cluster parameter group has been created or
* modified.
*
*
*
* @param modifyDBClusterParameterGroupRequest
* @return A Java Future containing the result of the ModifyDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonNeptuneAsync.ModifyDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyDBClusterParameterGroupAsync(
ModifyDBClusterParameterGroupRequest modifyDBClusterParameterGroupRequest);
/**
*
* Modifies the parameters of a DB cluster parameter group. To modify more than one parameter, submit a list of the
* following: ParameterName
, ParameterValue
, and ApplyMethod
. A maximum of 20
* parameters can be modified in a single request.
*
*
*
* Changes to dynamic parameters are applied immediately. Changes to static parameters require a reboot without
* failover to the DB cluster associated with the parameter group before the change can take effect.
*
*
*
* After you create a DB cluster parameter group, you should wait at least 5 minutes before creating your first DB
* cluster that uses that DB cluster parameter group as the default parameter group. This allows Amazon Neptune to
* fully complete the create action before the parameter group is used as the default for a new DB cluster. This is
* especially important for parameters that are critical when creating the default database for a DB cluster, such
* as the character set for the default database defined by the character_set_database
parameter. You
* can use the Parameter Groups option of the Amazon Neptune console or the
* DescribeDBClusterParameters command to verify that your DB cluster parameter group has been created or
* modified.
*
*
*
* @param modifyDBClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.ModifyDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyDBClusterParameterGroupAsync(
ModifyDBClusterParameterGroupRequest modifyDBClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an attribute and values to, or removes an attribute and values from, a manual DB cluster snapshot.
*
*
* To share a manual DB cluster snapshot with other Amazon accounts, specify restore
as the
* AttributeName
and use the ValuesToAdd
parameter to add a list of IDs of the Amazon
* accounts that are authorized to restore the manual DB cluster snapshot. Use the value all
to make
* the manual DB cluster snapshot public, which means that it can be copied or restored by all Amazon accounts. Do
* not add the all
value for any manual DB cluster snapshots that contain private information that you
* don't want available to all Amazon accounts. If a manual DB cluster snapshot is encrypted, it can be shared, but
* only by specifying a list of authorized Amazon account IDs for the ValuesToAdd
parameter. You can't
* use all
as a value for that parameter in this case.
*
*
* To view which Amazon accounts have access to copy or restore a manual DB cluster snapshot, or whether a manual DB
* cluster snapshot public or private, use the DescribeDBClusterSnapshotAttributes API action.
*
*
* @param modifyDBClusterSnapshotAttributeRequest
* @return A Java Future containing the result of the ModifyDBClusterSnapshotAttribute operation returned by the
* service.
* @sample AmazonNeptuneAsync.ModifyDBClusterSnapshotAttribute
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyDBClusterSnapshotAttributeAsync(
ModifyDBClusterSnapshotAttributeRequest modifyDBClusterSnapshotAttributeRequest);
/**
*
* Adds an attribute and values to, or removes an attribute and values from, a manual DB cluster snapshot.
*
*
* To share a manual DB cluster snapshot with other Amazon accounts, specify restore
as the
* AttributeName
and use the ValuesToAdd
parameter to add a list of IDs of the Amazon
* accounts that are authorized to restore the manual DB cluster snapshot. Use the value all
to make
* the manual DB cluster snapshot public, which means that it can be copied or restored by all Amazon accounts. Do
* not add the all
value for any manual DB cluster snapshots that contain private information that you
* don't want available to all Amazon accounts. If a manual DB cluster snapshot is encrypted, it can be shared, but
* only by specifying a list of authorized Amazon account IDs for the ValuesToAdd
parameter. You can't
* use all
as a value for that parameter in this case.
*
*
* To view which Amazon accounts have access to copy or restore a manual DB cluster snapshot, or whether a manual DB
* cluster snapshot public or private, use the DescribeDBClusterSnapshotAttributes API action.
*
*
* @param modifyDBClusterSnapshotAttributeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyDBClusterSnapshotAttribute operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.ModifyDBClusterSnapshotAttribute
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyDBClusterSnapshotAttributeAsync(
ModifyDBClusterSnapshotAttributeRequest modifyDBClusterSnapshotAttributeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies settings for a DB instance. You can change one or more database configuration parameters by specifying
* these parameters and the new values in the request. To learn what modifications you can make to your DB instance,
* call DescribeValidDBInstanceModifications before you call ModifyDBInstance.
*
*
* @param modifyDBInstanceRequest
* @return A Java Future containing the result of the ModifyDBInstance operation returned by the service.
* @sample AmazonNeptuneAsync.ModifyDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyDBInstanceAsync(ModifyDBInstanceRequest modifyDBInstanceRequest);
/**
*
* Modifies settings for a DB instance. You can change one or more database configuration parameters by specifying
* these parameters and the new values in the request. To learn what modifications you can make to your DB instance,
* call DescribeValidDBInstanceModifications before you call ModifyDBInstance.
*
*
* @param modifyDBInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyDBInstance operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.ModifyDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyDBInstanceAsync(ModifyDBInstanceRequest modifyDBInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the parameters of a DB parameter group. To modify more than one parameter, submit a list of the
* following: ParameterName
, ParameterValue
, and ApplyMethod
. A maximum of 20
* parameters can be modified in a single request.
*
*
*
* Changes to dynamic parameters are applied immediately. Changes to static parameters require a reboot without
* failover to the DB instance associated with the parameter group before the change can take effect.
*
*
*
* After you modify a DB parameter group, you should wait at least 5 minutes before creating your first DB instance
* that uses that DB parameter group as the default parameter group. This allows Amazon Neptune to fully complete
* the modify action before the parameter group is used as the default for a new DB instance. This is especially
* important for parameters that are critical when creating the default database for a DB instance, such as the
* character set for the default database defined by the character_set_database
parameter. You can use
* the Parameter Groups option of the Amazon Neptune console or the DescribeDBParameters command to
* verify that your DB parameter group has been created or modified.
*
*
*
* @param modifyDBParameterGroupRequest
* @return A Java Future containing the result of the ModifyDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsync.ModifyDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyDBParameterGroupAsync(ModifyDBParameterGroupRequest modifyDBParameterGroupRequest);
/**
*
* Modifies the parameters of a DB parameter group. To modify more than one parameter, submit a list of the
* following: ParameterName
, ParameterValue
, and ApplyMethod
. A maximum of 20
* parameters can be modified in a single request.
*
*
*
* Changes to dynamic parameters are applied immediately. Changes to static parameters require a reboot without
* failover to the DB instance associated with the parameter group before the change can take effect.
*
*
*
* After you modify a DB parameter group, you should wait at least 5 minutes before creating your first DB instance
* that uses that DB parameter group as the default parameter group. This allows Amazon Neptune to fully complete
* the modify action before the parameter group is used as the default for a new DB instance. This is especially
* important for parameters that are critical when creating the default database for a DB instance, such as the
* character set for the default database defined by the character_set_database
parameter. You can use
* the Parameter Groups option of the Amazon Neptune console or the DescribeDBParameters command to
* verify that your DB parameter group has been created or modified.
*
*
*
* @param modifyDBParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.ModifyDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyDBParameterGroupAsync(ModifyDBParameterGroupRequest modifyDBParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies an existing DB subnet group. DB subnet groups must contain at least one subnet in at least two AZs in
* the Amazon Region.
*
*
* @param modifyDBSubnetGroupRequest
* @return A Java Future containing the result of the ModifyDBSubnetGroup operation returned by the service.
* @sample AmazonNeptuneAsync.ModifyDBSubnetGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyDBSubnetGroupAsync(ModifyDBSubnetGroupRequest modifyDBSubnetGroupRequest);
/**
*
* Modifies an existing DB subnet group. DB subnet groups must contain at least one subnet in at least two AZs in
* the Amazon Region.
*
*
* @param modifyDBSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyDBSubnetGroup operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.ModifyDBSubnetGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyDBSubnetGroupAsync(ModifyDBSubnetGroupRequest modifyDBSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies an existing event notification subscription. Note that you can't modify the source identifiers using
* this call; to change source identifiers for a subscription, use the AddSourceIdentifierToSubscription and
* RemoveSourceIdentifierFromSubscription calls.
*
*
* You can see a list of the event categories for a given SourceType by using the DescribeEventCategories
* action.
*
*
* @param modifyEventSubscriptionRequest
* @return A Java Future containing the result of the ModifyEventSubscription operation returned by the service.
* @sample AmazonNeptuneAsync.ModifyEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyEventSubscriptionAsync(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest);
/**
*
* Modifies an existing event notification subscription. Note that you can't modify the source identifiers using
* this call; to change source identifiers for a subscription, use the AddSourceIdentifierToSubscription and
* RemoveSourceIdentifierFromSubscription calls.
*
*
* You can see a list of the event categories for a given SourceType by using the DescribeEventCategories
* action.
*
*
* @param modifyEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyEventSubscription operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.ModifyEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyEventSubscriptionAsync(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modify a setting for an Amazon Neptune global cluster. You can change one or more database configuration
* parameters by specifying these parameters and their new values in the request.
*
*
* @param modifyGlobalClusterRequest
* @return A Java Future containing the result of the ModifyGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsync.ModifyGlobalCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyGlobalClusterAsync(ModifyGlobalClusterRequest modifyGlobalClusterRequest);
/**
*
* Modify a setting for an Amazon Neptune global cluster. You can change one or more database configuration
* parameters by specifying these parameters and their new values in the request.
*
*
* @param modifyGlobalClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.ModifyGlobalCluster
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyGlobalClusterAsync(ModifyGlobalClusterRequest modifyGlobalClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Not supported.
*
*
* @param promoteReadReplicaDBClusterRequest
* @return A Java Future containing the result of the PromoteReadReplicaDBCluster operation returned by the service.
* @sample AmazonNeptuneAsync.PromoteReadReplicaDBCluster
* @see AWS API Documentation
*/
java.util.concurrent.Future promoteReadReplicaDBClusterAsync(PromoteReadReplicaDBClusterRequest promoteReadReplicaDBClusterRequest);
/**
*
* Not supported.
*
*
* @param promoteReadReplicaDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PromoteReadReplicaDBCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.PromoteReadReplicaDBCluster
* @see AWS API Documentation
*/
java.util.concurrent.Future promoteReadReplicaDBClusterAsync(PromoteReadReplicaDBClusterRequest promoteReadReplicaDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* You might need to reboot your DB instance, usually for maintenance reasons. For example, if you make certain
* modifications, or if you change the DB parameter group associated with the DB instance, you must reboot the
* instance for the changes to take effect.
*
*
* Rebooting a DB instance restarts the database engine service. Rebooting a DB instance results in a momentary
* outage, during which the DB instance status is set to rebooting.
*
*
* @param rebootDBInstanceRequest
* @return A Java Future containing the result of the RebootDBInstance operation returned by the service.
* @sample AmazonNeptuneAsync.RebootDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future rebootDBInstanceAsync(RebootDBInstanceRequest rebootDBInstanceRequest);
/**
*
* You might need to reboot your DB instance, usually for maintenance reasons. For example, if you make certain
* modifications, or if you change the DB parameter group associated with the DB instance, you must reboot the
* instance for the changes to take effect.
*
*
* Rebooting a DB instance restarts the database engine service. Rebooting a DB instance results in a momentary
* outage, during which the DB instance status is set to rebooting.
*
*
* @param rebootDBInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RebootDBInstance operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.RebootDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future rebootDBInstanceAsync(RebootDBInstanceRequest rebootDBInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Detaches a Neptune DB cluster from a Neptune global database. A secondary cluster becomes a normal standalone
* cluster with read-write capability instead of being read-only, and no longer receives data from a the primary
* cluster.
*
*
* @param removeFromGlobalClusterRequest
* @return A Java Future containing the result of the RemoveFromGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsync.RemoveFromGlobalCluster
* @see AWS API Documentation
*/
java.util.concurrent.Future removeFromGlobalClusterAsync(RemoveFromGlobalClusterRequest removeFromGlobalClusterRequest);
/**
*
* Detaches a Neptune DB cluster from a Neptune global database. A secondary cluster becomes a normal standalone
* cluster with read-write capability instead of being read-only, and no longer receives data from a the primary
* cluster.
*
*
* @param removeFromGlobalClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveFromGlobalCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.RemoveFromGlobalCluster
* @see AWS API Documentation
*/
java.util.concurrent.Future removeFromGlobalClusterAsync(RemoveFromGlobalClusterRequest removeFromGlobalClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates an Identity and Access Management (IAM) role from a DB cluster.
*
*
* @param removeRoleFromDBClusterRequest
* @return A Java Future containing the result of the RemoveRoleFromDBCluster operation returned by the service.
* @sample AmazonNeptuneAsync.RemoveRoleFromDBCluster
* @see AWS API Documentation
*/
java.util.concurrent.Future removeRoleFromDBClusterAsync(RemoveRoleFromDBClusterRequest removeRoleFromDBClusterRequest);
/**
*
* Disassociates an Identity and Access Management (IAM) role from a DB cluster.
*
*
* @param removeRoleFromDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveRoleFromDBCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.RemoveRoleFromDBCluster
* @see AWS API Documentation
*/
java.util.concurrent.Future removeRoleFromDBClusterAsync(RemoveRoleFromDBClusterRequest removeRoleFromDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a source identifier from an existing event notification subscription.
*
*
* @param removeSourceIdentifierFromSubscriptionRequest
* @return A Java Future containing the result of the RemoveSourceIdentifierFromSubscription operation returned by
* the service.
* @sample AmazonNeptuneAsync.RemoveSourceIdentifierFromSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future removeSourceIdentifierFromSubscriptionAsync(
RemoveSourceIdentifierFromSubscriptionRequest removeSourceIdentifierFromSubscriptionRequest);
/**
*
* Removes a source identifier from an existing event notification subscription.
*
*
* @param removeSourceIdentifierFromSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveSourceIdentifierFromSubscription operation returned by
* the service.
* @sample AmazonNeptuneAsyncHandler.RemoveSourceIdentifierFromSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future removeSourceIdentifierFromSubscriptionAsync(
RemoveSourceIdentifierFromSubscriptionRequest removeSourceIdentifierFromSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes metadata tags from an Amazon Neptune resource.
*
*
* @param removeTagsFromResourceRequest
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* @sample AmazonNeptuneAsync.RemoveTagsFromResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future removeTagsFromResourceAsync(RemoveTagsFromResourceRequest removeTagsFromResourceRequest);
/**
*
* Removes metadata tags from an Amazon Neptune resource.
*
*
* @param removeTagsFromResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.RemoveTagsFromResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future removeTagsFromResourceAsync(RemoveTagsFromResourceRequest removeTagsFromResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the parameters of a DB cluster parameter group to the default value. To reset specific parameters submit
* a list of the following: ParameterName
and ApplyMethod
. To reset the entire DB cluster
* parameter group, specify the DBClusterParameterGroupName
and ResetAllParameters
* parameters.
*
*
* When resetting the entire group, dynamic parameters are updated immediately and static parameters are set to
* pending-reboot
to take effect on the next DB instance restart or RebootDBInstance request.
* You must call RebootDBInstance for every DB instance in your DB cluster that you want the updated static
* parameter to apply to.
*
*
* @param resetDBClusterParameterGroupRequest
* @return A Java Future containing the result of the ResetDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonNeptuneAsync.ResetDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future resetDBClusterParameterGroupAsync(
ResetDBClusterParameterGroupRequest resetDBClusterParameterGroupRequest);
/**
*
* Modifies the parameters of a DB cluster parameter group to the default value. To reset specific parameters submit
* a list of the following: ParameterName
and ApplyMethod
. To reset the entire DB cluster
* parameter group, specify the DBClusterParameterGroupName
and ResetAllParameters
* parameters.
*
*
* When resetting the entire group, dynamic parameters are updated immediately and static parameters are set to
* pending-reboot
to take effect on the next DB instance restart or RebootDBInstance request.
* You must call RebootDBInstance for every DB instance in your DB cluster that you want the updated static
* parameter to apply to.
*
*
* @param resetDBClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResetDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.ResetDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future resetDBClusterParameterGroupAsync(
ResetDBClusterParameterGroupRequest resetDBClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the parameters of a DB parameter group to the engine/system default value. To reset specific parameters,
* provide a list of the following: ParameterName
and ApplyMethod
. To reset the entire DB
* parameter group, specify the DBParameterGroup
name and ResetAllParameters
parameters.
* When resetting the entire group, dynamic parameters are updated immediately and static parameters are set to
* pending-reboot
to take effect on the next DB instance restart or RebootDBInstance
* request.
*
*
* @param resetDBParameterGroupRequest
* @return A Java Future containing the result of the ResetDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsync.ResetDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future resetDBParameterGroupAsync(ResetDBParameterGroupRequest resetDBParameterGroupRequest);
/**
*
* Modifies the parameters of a DB parameter group to the engine/system default value. To reset specific parameters,
* provide a list of the following: ParameterName
and ApplyMethod
. To reset the entire DB
* parameter group, specify the DBParameterGroup
name and ResetAllParameters
parameters.
* When resetting the entire group, dynamic parameters are updated immediately and static parameters are set to
* pending-reboot
to take effect on the next DB instance restart or RebootDBInstance
* request.
*
*
* @param resetDBParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResetDBParameterGroup operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.ResetDBParameterGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future resetDBParameterGroupAsync(ResetDBParameterGroupRequest resetDBParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB cluster from a DB snapshot or DB cluster snapshot.
*
*
* If a DB snapshot is specified, the target DB cluster is created from the source DB snapshot with a default
* configuration and default security group.
*
*
* If a DB cluster snapshot is specified, the target DB cluster is created from the source DB cluster restore point
* with the same configuration as the original source DB cluster, except that the new DB cluster is created with the
* default security group.
*
*
* @param restoreDBClusterFromSnapshotRequest
* @return A Java Future containing the result of the RestoreDBClusterFromSnapshot operation returned by the
* service.
* @sample AmazonNeptuneAsync.RestoreDBClusterFromSnapshot
* @see AWS API Documentation
*/
java.util.concurrent.Future restoreDBClusterFromSnapshotAsync(RestoreDBClusterFromSnapshotRequest restoreDBClusterFromSnapshotRequest);
/**
*
* Creates a new DB cluster from a DB snapshot or DB cluster snapshot.
*
*
* If a DB snapshot is specified, the target DB cluster is created from the source DB snapshot with a default
* configuration and default security group.
*
*
* If a DB cluster snapshot is specified, the target DB cluster is created from the source DB cluster restore point
* with the same configuration as the original source DB cluster, except that the new DB cluster is created with the
* default security group.
*
*
* @param restoreDBClusterFromSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RestoreDBClusterFromSnapshot operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.RestoreDBClusterFromSnapshot
* @see AWS API Documentation
*/
java.util.concurrent.Future restoreDBClusterFromSnapshotAsync(RestoreDBClusterFromSnapshotRequest restoreDBClusterFromSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Restores a DB cluster to an arbitrary point in time. Users can restore to any point in time before
* LatestRestorableTime
for up to BackupRetentionPeriod
days. The target DB cluster is
* created from the source DB cluster with the same configuration as the original DB cluster, except that the new DB
* cluster is created with the default DB security group.
*
*
*
* This action only restores the DB cluster, not the DB instances for that DB cluster. You must invoke the
* CreateDBInstance action to create DB instances for the restored DB cluster, specifying the identifier of
* the restored DB cluster in DBClusterIdentifier
. You can create DB instances only after the
* RestoreDBClusterToPointInTime
action has completed and the DB cluster is available.
*
*
*
* @param restoreDBClusterToPointInTimeRequest
* @return A Java Future containing the result of the RestoreDBClusterToPointInTime operation returned by the
* service.
* @sample AmazonNeptuneAsync.RestoreDBClusterToPointInTime
* @see AWS API Documentation
*/
java.util.concurrent.Future restoreDBClusterToPointInTimeAsync(RestoreDBClusterToPointInTimeRequest restoreDBClusterToPointInTimeRequest);
/**
*
* Restores a DB cluster to an arbitrary point in time. Users can restore to any point in time before
* LatestRestorableTime
for up to BackupRetentionPeriod
days. The target DB cluster is
* created from the source DB cluster with the same configuration as the original DB cluster, except that the new DB
* cluster is created with the default DB security group.
*
*
*
* This action only restores the DB cluster, not the DB instances for that DB cluster. You must invoke the
* CreateDBInstance action to create DB instances for the restored DB cluster, specifying the identifier of
* the restored DB cluster in DBClusterIdentifier
. You can create DB instances only after the
* RestoreDBClusterToPointInTime
action has completed and the DB cluster is available.
*
*
*
* @param restoreDBClusterToPointInTimeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RestoreDBClusterToPointInTime operation returned by the
* service.
* @sample AmazonNeptuneAsyncHandler.RestoreDBClusterToPointInTime
* @see AWS API Documentation
*/
java.util.concurrent.Future restoreDBClusterToPointInTimeAsync(RestoreDBClusterToPointInTimeRequest restoreDBClusterToPointInTimeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts an Amazon Neptune DB cluster that was stopped using the Amazon console, the Amazon CLI stop-db-cluster
* command, or the StopDBCluster API.
*
*
* @param startDBClusterRequest
* @return A Java Future containing the result of the StartDBCluster operation returned by the service.
* @sample AmazonNeptuneAsync.StartDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startDBClusterAsync(StartDBClusterRequest startDBClusterRequest);
/**
*
* Starts an Amazon Neptune DB cluster that was stopped using the Amazon console, the Amazon CLI stop-db-cluster
* command, or the StopDBCluster API.
*
*
* @param startDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartDBCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.StartDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startDBClusterAsync(StartDBClusterRequest startDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops an Amazon Neptune DB cluster. When you stop a DB cluster, Neptune retains the DB cluster's metadata,
* including its endpoints and DB parameter groups.
*
*
* Neptune also retains the transaction logs so you can do a point-in-time restore if necessary.
*
*
* @param stopDBClusterRequest
* @return A Java Future containing the result of the StopDBCluster operation returned by the service.
* @sample AmazonNeptuneAsync.StopDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopDBClusterAsync(StopDBClusterRequest stopDBClusterRequest);
/**
*
* Stops an Amazon Neptune DB cluster. When you stop a DB cluster, Neptune retains the DB cluster's metadata,
* including its endpoints and DB parameter groups.
*
*
* Neptune also retains the transaction logs so you can do a point-in-time restore if necessary.
*
*
* @param stopDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopDBCluster operation returned by the service.
* @sample AmazonNeptuneAsyncHandler.StopDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopDBClusterAsync(StopDBClusterRequest stopDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}