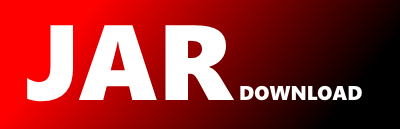
com.amazonaws.services.neptune.model.CopyDBParameterGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-neptune Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.neptune.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CopyDBParameterGroupRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The identifier or ARN for the source DB parameter group. For information about creating an ARN, see
* Constructing an Amazon Resource Name (ARN).
*
*
* Constraints:
*
*
* -
*
* Must specify a valid DB parameter group.
*
*
* -
*
* Must specify a valid DB parameter group identifier, for example my-db-param-group
, or a valid ARN.
*
*
*
*/
private String sourceDBParameterGroupIdentifier;
/**
*
* The identifier for the copied DB parameter group.
*
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-db-parameter-group
*
*/
private String targetDBParameterGroupIdentifier;
/**
*
* A description for the copied DB parameter group.
*
*/
private String targetDBParameterGroupDescription;
/**
*
* The tags to be assigned to the copied DB parameter group.
*
*/
private java.util.List tags;
/**
*
* The identifier or ARN for the source DB parameter group. For information about creating an ARN, see
* Constructing an Amazon Resource Name (ARN).
*
*
* Constraints:
*
*
* -
*
* Must specify a valid DB parameter group.
*
*
* -
*
* Must specify a valid DB parameter group identifier, for example my-db-param-group
, or a valid ARN.
*
*
*
*
* @param sourceDBParameterGroupIdentifier
* The identifier or ARN for the source DB parameter group. For information about creating an ARN, see
* Constructing an Amazon Resource Name (ARN).
*
* Constraints:
*
*
* -
*
* Must specify a valid DB parameter group.
*
*
* -
*
* Must specify a valid DB parameter group identifier, for example my-db-param-group
, or a valid
* ARN.
*
*
*/
public void setSourceDBParameterGroupIdentifier(String sourceDBParameterGroupIdentifier) {
this.sourceDBParameterGroupIdentifier = sourceDBParameterGroupIdentifier;
}
/**
*
* The identifier or ARN for the source DB parameter group. For information about creating an ARN, see
* Constructing an Amazon Resource Name (ARN).
*
*
* Constraints:
*
*
* -
*
* Must specify a valid DB parameter group.
*
*
* -
*
* Must specify a valid DB parameter group identifier, for example my-db-param-group
, or a valid ARN.
*
*
*
*
* @return The identifier or ARN for the source DB parameter group. For information about creating an ARN, see
* Constructing an Amazon Resource Name (ARN).
*
* Constraints:
*
*
* -
*
* Must specify a valid DB parameter group.
*
*
* -
*
* Must specify a valid DB parameter group identifier, for example my-db-param-group
, or a
* valid ARN.
*
*
*/
public String getSourceDBParameterGroupIdentifier() {
return this.sourceDBParameterGroupIdentifier;
}
/**
*
* The identifier or ARN for the source DB parameter group. For information about creating an ARN, see
* Constructing an Amazon Resource Name (ARN).
*
*
* Constraints:
*
*
* -
*
* Must specify a valid DB parameter group.
*
*
* -
*
* Must specify a valid DB parameter group identifier, for example my-db-param-group
, or a valid ARN.
*
*
*
*
* @param sourceDBParameterGroupIdentifier
* The identifier or ARN for the source DB parameter group. For information about creating an ARN, see
* Constructing an Amazon Resource Name (ARN).
*
* Constraints:
*
*
* -
*
* Must specify a valid DB parameter group.
*
*
* -
*
* Must specify a valid DB parameter group identifier, for example my-db-param-group
, or a valid
* ARN.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBParameterGroupRequest withSourceDBParameterGroupIdentifier(String sourceDBParameterGroupIdentifier) {
setSourceDBParameterGroupIdentifier(sourceDBParameterGroupIdentifier);
return this;
}
/**
*
* The identifier for the copied DB parameter group.
*
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-db-parameter-group
*
*
* @param targetDBParameterGroupIdentifier
* The identifier for the copied DB parameter group.
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-db-parameter-group
*/
public void setTargetDBParameterGroupIdentifier(String targetDBParameterGroupIdentifier) {
this.targetDBParameterGroupIdentifier = targetDBParameterGroupIdentifier;
}
/**
*
* The identifier for the copied DB parameter group.
*
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-db-parameter-group
*
*
* @return The identifier for the copied DB parameter group.
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-db-parameter-group
*/
public String getTargetDBParameterGroupIdentifier() {
return this.targetDBParameterGroupIdentifier;
}
/**
*
* The identifier for the copied DB parameter group.
*
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-db-parameter-group
*
*
* @param targetDBParameterGroupIdentifier
* The identifier for the copied DB parameter group.
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-db-parameter-group
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBParameterGroupRequest withTargetDBParameterGroupIdentifier(String targetDBParameterGroupIdentifier) {
setTargetDBParameterGroupIdentifier(targetDBParameterGroupIdentifier);
return this;
}
/**
*
* A description for the copied DB parameter group.
*
*
* @param targetDBParameterGroupDescription
* A description for the copied DB parameter group.
*/
public void setTargetDBParameterGroupDescription(String targetDBParameterGroupDescription) {
this.targetDBParameterGroupDescription = targetDBParameterGroupDescription;
}
/**
*
* A description for the copied DB parameter group.
*
*
* @return A description for the copied DB parameter group.
*/
public String getTargetDBParameterGroupDescription() {
return this.targetDBParameterGroupDescription;
}
/**
*
* A description for the copied DB parameter group.
*
*
* @param targetDBParameterGroupDescription
* A description for the copied DB parameter group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBParameterGroupRequest withTargetDBParameterGroupDescription(String targetDBParameterGroupDescription) {
setTargetDBParameterGroupDescription(targetDBParameterGroupDescription);
return this;
}
/**
*
* The tags to be assigned to the copied DB parameter group.
*
*
* @return The tags to be assigned to the copied DB parameter group.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The tags to be assigned to the copied DB parameter group.
*
*
* @param tags
* The tags to be assigned to the copied DB parameter group.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The tags to be assigned to the copied DB parameter group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The tags to be assigned to the copied DB parameter group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBParameterGroupRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The tags to be assigned to the copied DB parameter group.
*
*
* @param tags
* The tags to be assigned to the copied DB parameter group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBParameterGroupRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSourceDBParameterGroupIdentifier() != null)
sb.append("SourceDBParameterGroupIdentifier: ").append(getSourceDBParameterGroupIdentifier()).append(",");
if (getTargetDBParameterGroupIdentifier() != null)
sb.append("TargetDBParameterGroupIdentifier: ").append(getTargetDBParameterGroupIdentifier()).append(",");
if (getTargetDBParameterGroupDescription() != null)
sb.append("TargetDBParameterGroupDescription: ").append(getTargetDBParameterGroupDescription()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CopyDBParameterGroupRequest == false)
return false;
CopyDBParameterGroupRequest other = (CopyDBParameterGroupRequest) obj;
if (other.getSourceDBParameterGroupIdentifier() == null ^ this.getSourceDBParameterGroupIdentifier() == null)
return false;
if (other.getSourceDBParameterGroupIdentifier() != null
&& other.getSourceDBParameterGroupIdentifier().equals(this.getSourceDBParameterGroupIdentifier()) == false)
return false;
if (other.getTargetDBParameterGroupIdentifier() == null ^ this.getTargetDBParameterGroupIdentifier() == null)
return false;
if (other.getTargetDBParameterGroupIdentifier() != null
&& other.getTargetDBParameterGroupIdentifier().equals(this.getTargetDBParameterGroupIdentifier()) == false)
return false;
if (other.getTargetDBParameterGroupDescription() == null ^ this.getTargetDBParameterGroupDescription() == null)
return false;
if (other.getTargetDBParameterGroupDescription() != null
&& other.getTargetDBParameterGroupDescription().equals(this.getTargetDBParameterGroupDescription()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSourceDBParameterGroupIdentifier() == null) ? 0 : getSourceDBParameterGroupIdentifier().hashCode());
hashCode = prime * hashCode + ((getTargetDBParameterGroupIdentifier() == null) ? 0 : getTargetDBParameterGroupIdentifier().hashCode());
hashCode = prime * hashCode + ((getTargetDBParameterGroupDescription() == null) ? 0 : getTargetDBParameterGroupDescription().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CopyDBParameterGroupRequest clone() {
return (CopyDBParameterGroupRequest) super.clone();
}
}