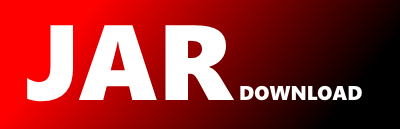
com.amazonaws.services.neptune.model.CreateDBClusterEndpointResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-neptune Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.neptune.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* This data type represents the information you need to connect to an Amazon Neptune DB cluster. This data type is used
* as a response element in the following actions:
*
*
* -
*
* CreateDBClusterEndpoint
*
*
* -
*
* DescribeDBClusterEndpoints
*
*
* -
*
* ModifyDBClusterEndpoint
*
*
* -
*
* DeleteDBClusterEndpoint
*
*
*
*
* For the data structure that represents Amazon Neptune DB instance endpoints, see Endpoint
.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateDBClusterEndpointResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The identifier associated with the endpoint. This parameter is stored as a lowercase string.
*
*/
private String dBClusterEndpointIdentifier;
/**
*
* The DB cluster identifier of the DB cluster associated with the endpoint. This parameter is stored as a lowercase
* string.
*
*/
private String dBClusterIdentifier;
/**
*
* A unique system-generated identifier for an endpoint. It remains the same for the whole life of the endpoint.
*
*/
private String dBClusterEndpointResourceIdentifier;
/**
*
* The DNS address of the endpoint.
*
*/
private String endpoint;
/**
*
* The current status of the endpoint. One of: creating
, available
, deleting
,
* inactive
, modifying
. The inactive
state applies to an endpoint that cannot
* be used for a certain kind of cluster, such as a writer
endpoint for a read-only secondary cluster
* in a global database.
*
*/
private String status;
/**
*
* The type of the endpoint. One of: READER
, WRITER
, CUSTOM
.
*
*/
private String endpointType;
/**
*
* The type associated with a custom endpoint. One of: READER
, WRITER
, ANY
.
*
*/
private String customEndpointType;
/**
*
* List of DB instance identifiers that are part of the custom endpoint group.
*
*/
private java.util.List staticMembers;
/**
*
* List of DB instance identifiers that aren't part of the custom endpoint group. All other eligible instances are
* reachable through the custom endpoint. Only relevant if the list of static members is empty.
*
*/
private java.util.List excludedMembers;
/**
*
* The Amazon Resource Name (ARN) for the endpoint.
*
*/
private String dBClusterEndpointArn;
/**
*
* The identifier associated with the endpoint. This parameter is stored as a lowercase string.
*
*
* @param dBClusterEndpointIdentifier
* The identifier associated with the endpoint. This parameter is stored as a lowercase string.
*/
public void setDBClusterEndpointIdentifier(String dBClusterEndpointIdentifier) {
this.dBClusterEndpointIdentifier = dBClusterEndpointIdentifier;
}
/**
*
* The identifier associated with the endpoint. This parameter is stored as a lowercase string.
*
*
* @return The identifier associated with the endpoint. This parameter is stored as a lowercase string.
*/
public String getDBClusterEndpointIdentifier() {
return this.dBClusterEndpointIdentifier;
}
/**
*
* The identifier associated with the endpoint. This parameter is stored as a lowercase string.
*
*
* @param dBClusterEndpointIdentifier
* The identifier associated with the endpoint. This parameter is stored as a lowercase string.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withDBClusterEndpointIdentifier(String dBClusterEndpointIdentifier) {
setDBClusterEndpointIdentifier(dBClusterEndpointIdentifier);
return this;
}
/**
*
* The DB cluster identifier of the DB cluster associated with the endpoint. This parameter is stored as a lowercase
* string.
*
*
* @param dBClusterIdentifier
* The DB cluster identifier of the DB cluster associated with the endpoint. This parameter is stored as a
* lowercase string.
*/
public void setDBClusterIdentifier(String dBClusterIdentifier) {
this.dBClusterIdentifier = dBClusterIdentifier;
}
/**
*
* The DB cluster identifier of the DB cluster associated with the endpoint. This parameter is stored as a lowercase
* string.
*
*
* @return The DB cluster identifier of the DB cluster associated with the endpoint. This parameter is stored as a
* lowercase string.
*/
public String getDBClusterIdentifier() {
return this.dBClusterIdentifier;
}
/**
*
* The DB cluster identifier of the DB cluster associated with the endpoint. This parameter is stored as a lowercase
* string.
*
*
* @param dBClusterIdentifier
* The DB cluster identifier of the DB cluster associated with the endpoint. This parameter is stored as a
* lowercase string.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withDBClusterIdentifier(String dBClusterIdentifier) {
setDBClusterIdentifier(dBClusterIdentifier);
return this;
}
/**
*
* A unique system-generated identifier for an endpoint. It remains the same for the whole life of the endpoint.
*
*
* @param dBClusterEndpointResourceIdentifier
* A unique system-generated identifier for an endpoint. It remains the same for the whole life of the
* endpoint.
*/
public void setDBClusterEndpointResourceIdentifier(String dBClusterEndpointResourceIdentifier) {
this.dBClusterEndpointResourceIdentifier = dBClusterEndpointResourceIdentifier;
}
/**
*
* A unique system-generated identifier for an endpoint. It remains the same for the whole life of the endpoint.
*
*
* @return A unique system-generated identifier for an endpoint. It remains the same for the whole life of the
* endpoint.
*/
public String getDBClusterEndpointResourceIdentifier() {
return this.dBClusterEndpointResourceIdentifier;
}
/**
*
* A unique system-generated identifier for an endpoint. It remains the same for the whole life of the endpoint.
*
*
* @param dBClusterEndpointResourceIdentifier
* A unique system-generated identifier for an endpoint. It remains the same for the whole life of the
* endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withDBClusterEndpointResourceIdentifier(String dBClusterEndpointResourceIdentifier) {
setDBClusterEndpointResourceIdentifier(dBClusterEndpointResourceIdentifier);
return this;
}
/**
*
* The DNS address of the endpoint.
*
*
* @param endpoint
* The DNS address of the endpoint.
*/
public void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
/**
*
* The DNS address of the endpoint.
*
*
* @return The DNS address of the endpoint.
*/
public String getEndpoint() {
return this.endpoint;
}
/**
*
* The DNS address of the endpoint.
*
*
* @param endpoint
* The DNS address of the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withEndpoint(String endpoint) {
setEndpoint(endpoint);
return this;
}
/**
*
* The current status of the endpoint. One of: creating
, available
, deleting
,
* inactive
, modifying
. The inactive
state applies to an endpoint that cannot
* be used for a certain kind of cluster, such as a writer
endpoint for a read-only secondary cluster
* in a global database.
*
*
* @param status
* The current status of the endpoint. One of: creating
, available
,
* deleting
, inactive
, modifying
. The inactive
state
* applies to an endpoint that cannot be used for a certain kind of cluster, such as a writer
* endpoint for a read-only secondary cluster in a global database.
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the endpoint. One of: creating
, available
, deleting
,
* inactive
, modifying
. The inactive
state applies to an endpoint that cannot
* be used for a certain kind of cluster, such as a writer
endpoint for a read-only secondary cluster
* in a global database.
*
*
* @return The current status of the endpoint. One of: creating
, available
,
* deleting
, inactive
, modifying
. The inactive
state
* applies to an endpoint that cannot be used for a certain kind of cluster, such as a writer
* endpoint for a read-only secondary cluster in a global database.
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the endpoint. One of: creating
, available
, deleting
,
* inactive
, modifying
. The inactive
state applies to an endpoint that cannot
* be used for a certain kind of cluster, such as a writer
endpoint for a read-only secondary cluster
* in a global database.
*
*
* @param status
* The current status of the endpoint. One of: creating
, available
,
* deleting
, inactive
, modifying
. The inactive
state
* applies to an endpoint that cannot be used for a certain kind of cluster, such as a writer
* endpoint for a read-only secondary cluster in a global database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The type of the endpoint. One of: READER
, WRITER
, CUSTOM
.
*
*
* @param endpointType
* The type of the endpoint. One of: READER
, WRITER
, CUSTOM
.
*/
public void setEndpointType(String endpointType) {
this.endpointType = endpointType;
}
/**
*
* The type of the endpoint. One of: READER
, WRITER
, CUSTOM
.
*
*
* @return The type of the endpoint. One of: READER
, WRITER
, CUSTOM
.
*/
public String getEndpointType() {
return this.endpointType;
}
/**
*
* The type of the endpoint. One of: READER
, WRITER
, CUSTOM
.
*
*
* @param endpointType
* The type of the endpoint. One of: READER
, WRITER
, CUSTOM
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withEndpointType(String endpointType) {
setEndpointType(endpointType);
return this;
}
/**
*
* The type associated with a custom endpoint. One of: READER
, WRITER
, ANY
.
*
*
* @param customEndpointType
* The type associated with a custom endpoint. One of: READER
, WRITER
,
* ANY
.
*/
public void setCustomEndpointType(String customEndpointType) {
this.customEndpointType = customEndpointType;
}
/**
*
* The type associated with a custom endpoint. One of: READER
, WRITER
, ANY
.
*
*
* @return The type associated with a custom endpoint. One of: READER
, WRITER
,
* ANY
.
*/
public String getCustomEndpointType() {
return this.customEndpointType;
}
/**
*
* The type associated with a custom endpoint. One of: READER
, WRITER
, ANY
.
*
*
* @param customEndpointType
* The type associated with a custom endpoint. One of: READER
, WRITER
,
* ANY
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withCustomEndpointType(String customEndpointType) {
setCustomEndpointType(customEndpointType);
return this;
}
/**
*
* List of DB instance identifiers that are part of the custom endpoint group.
*
*
* @return List of DB instance identifiers that are part of the custom endpoint group.
*/
public java.util.List getStaticMembers() {
return staticMembers;
}
/**
*
* List of DB instance identifiers that are part of the custom endpoint group.
*
*
* @param staticMembers
* List of DB instance identifiers that are part of the custom endpoint group.
*/
public void setStaticMembers(java.util.Collection staticMembers) {
if (staticMembers == null) {
this.staticMembers = null;
return;
}
this.staticMembers = new java.util.ArrayList(staticMembers);
}
/**
*
* List of DB instance identifiers that are part of the custom endpoint group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStaticMembers(java.util.Collection)} or {@link #withStaticMembers(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param staticMembers
* List of DB instance identifiers that are part of the custom endpoint group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withStaticMembers(String... staticMembers) {
if (this.staticMembers == null) {
setStaticMembers(new java.util.ArrayList(staticMembers.length));
}
for (String ele : staticMembers) {
this.staticMembers.add(ele);
}
return this;
}
/**
*
* List of DB instance identifiers that are part of the custom endpoint group.
*
*
* @param staticMembers
* List of DB instance identifiers that are part of the custom endpoint group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withStaticMembers(java.util.Collection staticMembers) {
setStaticMembers(staticMembers);
return this;
}
/**
*
* List of DB instance identifiers that aren't part of the custom endpoint group. All other eligible instances are
* reachable through the custom endpoint. Only relevant if the list of static members is empty.
*
*
* @return List of DB instance identifiers that aren't part of the custom endpoint group. All other eligible
* instances are reachable through the custom endpoint. Only relevant if the list of static members is
* empty.
*/
public java.util.List getExcludedMembers() {
return excludedMembers;
}
/**
*
* List of DB instance identifiers that aren't part of the custom endpoint group. All other eligible instances are
* reachable through the custom endpoint. Only relevant if the list of static members is empty.
*
*
* @param excludedMembers
* List of DB instance identifiers that aren't part of the custom endpoint group. All other eligible
* instances are reachable through the custom endpoint. Only relevant if the list of static members is empty.
*/
public void setExcludedMembers(java.util.Collection excludedMembers) {
if (excludedMembers == null) {
this.excludedMembers = null;
return;
}
this.excludedMembers = new java.util.ArrayList(excludedMembers);
}
/**
*
* List of DB instance identifiers that aren't part of the custom endpoint group. All other eligible instances are
* reachable through the custom endpoint. Only relevant if the list of static members is empty.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExcludedMembers(java.util.Collection)} or {@link #withExcludedMembers(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param excludedMembers
* List of DB instance identifiers that aren't part of the custom endpoint group. All other eligible
* instances are reachable through the custom endpoint. Only relevant if the list of static members is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withExcludedMembers(String... excludedMembers) {
if (this.excludedMembers == null) {
setExcludedMembers(new java.util.ArrayList(excludedMembers.length));
}
for (String ele : excludedMembers) {
this.excludedMembers.add(ele);
}
return this;
}
/**
*
* List of DB instance identifiers that aren't part of the custom endpoint group. All other eligible instances are
* reachable through the custom endpoint. Only relevant if the list of static members is empty.
*
*
* @param excludedMembers
* List of DB instance identifiers that aren't part of the custom endpoint group. All other eligible
* instances are reachable through the custom endpoint. Only relevant if the list of static members is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withExcludedMembers(java.util.Collection excludedMembers) {
setExcludedMembers(excludedMembers);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for the endpoint.
*
*
* @param dBClusterEndpointArn
* The Amazon Resource Name (ARN) for the endpoint.
*/
public void setDBClusterEndpointArn(String dBClusterEndpointArn) {
this.dBClusterEndpointArn = dBClusterEndpointArn;
}
/**
*
* The Amazon Resource Name (ARN) for the endpoint.
*
*
* @return The Amazon Resource Name (ARN) for the endpoint.
*/
public String getDBClusterEndpointArn() {
return this.dBClusterEndpointArn;
}
/**
*
* The Amazon Resource Name (ARN) for the endpoint.
*
*
* @param dBClusterEndpointArn
* The Amazon Resource Name (ARN) for the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDBClusterEndpointResult withDBClusterEndpointArn(String dBClusterEndpointArn) {
setDBClusterEndpointArn(dBClusterEndpointArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDBClusterEndpointIdentifier() != null)
sb.append("DBClusterEndpointIdentifier: ").append(getDBClusterEndpointIdentifier()).append(",");
if (getDBClusterIdentifier() != null)
sb.append("DBClusterIdentifier: ").append(getDBClusterIdentifier()).append(",");
if (getDBClusterEndpointResourceIdentifier() != null)
sb.append("DBClusterEndpointResourceIdentifier: ").append(getDBClusterEndpointResourceIdentifier()).append(",");
if (getEndpoint() != null)
sb.append("Endpoint: ").append(getEndpoint()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getEndpointType() != null)
sb.append("EndpointType: ").append(getEndpointType()).append(",");
if (getCustomEndpointType() != null)
sb.append("CustomEndpointType: ").append(getCustomEndpointType()).append(",");
if (getStaticMembers() != null)
sb.append("StaticMembers: ").append(getStaticMembers()).append(",");
if (getExcludedMembers() != null)
sb.append("ExcludedMembers: ").append(getExcludedMembers()).append(",");
if (getDBClusterEndpointArn() != null)
sb.append("DBClusterEndpointArn: ").append(getDBClusterEndpointArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDBClusterEndpointResult == false)
return false;
CreateDBClusterEndpointResult other = (CreateDBClusterEndpointResult) obj;
if (other.getDBClusterEndpointIdentifier() == null ^ this.getDBClusterEndpointIdentifier() == null)
return false;
if (other.getDBClusterEndpointIdentifier() != null && other.getDBClusterEndpointIdentifier().equals(this.getDBClusterEndpointIdentifier()) == false)
return false;
if (other.getDBClusterIdentifier() == null ^ this.getDBClusterIdentifier() == null)
return false;
if (other.getDBClusterIdentifier() != null && other.getDBClusterIdentifier().equals(this.getDBClusterIdentifier()) == false)
return false;
if (other.getDBClusterEndpointResourceIdentifier() == null ^ this.getDBClusterEndpointResourceIdentifier() == null)
return false;
if (other.getDBClusterEndpointResourceIdentifier() != null
&& other.getDBClusterEndpointResourceIdentifier().equals(this.getDBClusterEndpointResourceIdentifier()) == false)
return false;
if (other.getEndpoint() == null ^ this.getEndpoint() == null)
return false;
if (other.getEndpoint() != null && other.getEndpoint().equals(this.getEndpoint()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getEndpointType() == null ^ this.getEndpointType() == null)
return false;
if (other.getEndpointType() != null && other.getEndpointType().equals(this.getEndpointType()) == false)
return false;
if (other.getCustomEndpointType() == null ^ this.getCustomEndpointType() == null)
return false;
if (other.getCustomEndpointType() != null && other.getCustomEndpointType().equals(this.getCustomEndpointType()) == false)
return false;
if (other.getStaticMembers() == null ^ this.getStaticMembers() == null)
return false;
if (other.getStaticMembers() != null && other.getStaticMembers().equals(this.getStaticMembers()) == false)
return false;
if (other.getExcludedMembers() == null ^ this.getExcludedMembers() == null)
return false;
if (other.getExcludedMembers() != null && other.getExcludedMembers().equals(this.getExcludedMembers()) == false)
return false;
if (other.getDBClusterEndpointArn() == null ^ this.getDBClusterEndpointArn() == null)
return false;
if (other.getDBClusterEndpointArn() != null && other.getDBClusterEndpointArn().equals(this.getDBClusterEndpointArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDBClusterEndpointIdentifier() == null) ? 0 : getDBClusterEndpointIdentifier().hashCode());
hashCode = prime * hashCode + ((getDBClusterIdentifier() == null) ? 0 : getDBClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getDBClusterEndpointResourceIdentifier() == null) ? 0 : getDBClusterEndpointResourceIdentifier().hashCode());
hashCode = prime * hashCode + ((getEndpoint() == null) ? 0 : getEndpoint().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getEndpointType() == null) ? 0 : getEndpointType().hashCode());
hashCode = prime * hashCode + ((getCustomEndpointType() == null) ? 0 : getCustomEndpointType().hashCode());
hashCode = prime * hashCode + ((getStaticMembers() == null) ? 0 : getStaticMembers().hashCode());
hashCode = prime * hashCode + ((getExcludedMembers() == null) ? 0 : getExcludedMembers().hashCode());
hashCode = prime * hashCode + ((getDBClusterEndpointArn() == null) ? 0 : getDBClusterEndpointArn().hashCode());
return hashCode;
}
@Override
public CreateDBClusterEndpointResult clone() {
try {
return (CreateDBClusterEndpointResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}