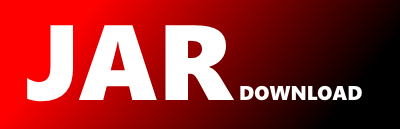
com.amazonaws.services.neptune.model.ModifyDBClusterRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-neptune Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.neptune.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyDBClusterRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The DB cluster identifier for the cluster being modified. This parameter is not case-sensitive.
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBCluster.
*
*
*
*/
private String dBClusterIdentifier;
/**
*
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a lowercase
* string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens
*
*
* -
*
* The first character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*
*/
private String newDBClusterIdentifier;
/**
*
* A value that specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is set to false
, changes to the DB cluster are applied during the next
* maintenance window.
*
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If you set
* the ApplyImmediately
parameter value to false, then changes to NewDBClusterIdentifier
* values are applied during the next maintenance window. All other changes are applied immediately, regardless of
* the value of the ApplyImmediately
parameter.
*
*
* Default: false
*
*/
private Boolean applyImmediately;
/**
*
* The number of days for which automated backups are retained. You must specify a minimum value of 1.
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35
*
*
*
*/
private Integer backupRetentionPeriod;
/**
*
* The name of the DB cluster parameter group to use for the DB cluster.
*
*/
private String dBClusterParameterGroupName;
/**
*
* A list of VPC security groups that the DB cluster will belong to.
*
*/
private java.util.List vpcSecurityGroupIds;
/**
*
* The port number on which the DB cluster accepts connections.
*
*
* Constraints: Value must be 1150-65535
*
*
* Default: The same port as the original DB cluster.
*
*/
private Integer port;
/**
*
* Not supported by Neptune.
*
*/
private String masterUserPassword;
/**
*
* Not supported by Neptune.
*
*/
private String optionGroupName;
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, using the
* BackupRetentionPeriod
parameter.
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*/
private String preferredBackupWindow;
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun.
*
*
* Constraints: Minimum 30-minute window.
*
*/
private String preferredMaintenanceWindow;
/**
*
* True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
*
* Default: false
*
*/
private Boolean enableIAMDatabaseAuthentication;
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster. See Using the
* CLI to publish Neptune audit logs to CloudWatch Logs.
*
*/
private CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration;
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter results in an
* outage. The change is applied during the next maintenance window unless the ApplyImmediately
* parameter is set to true.
*
*
* For a list of valid engine versions, see Engine Releases for Amazon
* Neptune, or call DescribeDBEngineVersions.
*
*/
private String engineVersion;
/**
*
* A value that indicates whether upgrades between different major versions are allowed.
*
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an EngineVersion
* parameter that uses a different major version than the DB cluster's current version.
*
*/
private Boolean allowMajorVersionUpgrade;
/**
*
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*
*
* When you apply a parameter group using DBInstanceParameterGroupName
, parameter changes aren't
* applied during the next maintenance window but instead are applied immediately.
*
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as the target DB cluster version.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is only valid in combination with the
* AllowMajorVersionUpgrade
parameter.
*
*
*
*/
private String dBInstanceParameterGroupName;
/**
*
* A value that indicates whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection is disabled.
*
*/
private Boolean deletionProtection;
/**
*
* If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*
*/
private Boolean copyTagsToSnapshot;
/**
*
* Contains the scaling configuration of a Neptune Serverless DB cluster.
*
*
* For more information, see Using Amazon Neptune
* Serverless in the Amazon Neptune User Guide.
*
*/
private ServerlessV2ScalingConfiguration serverlessV2ScalingConfiguration;
/**
*
* The storage type to associate with the DB cluster.
*
*
* Valid Values:
*
*
* -
*
* standard | iopt1
*
*
*
*
* Default:
*
*
* -
*
* standard
*
*
*
*/
private String storageType;
/**
*
* The DB cluster identifier for the cluster being modified. This parameter is not case-sensitive.
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBCluster.
*
*
*
*
* @param dBClusterIdentifier
* The DB cluster identifier for the cluster being modified. This parameter is not case-sensitive.
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBCluster.
*
*
*/
public void setDBClusterIdentifier(String dBClusterIdentifier) {
this.dBClusterIdentifier = dBClusterIdentifier;
}
/**
*
* The DB cluster identifier for the cluster being modified. This parameter is not case-sensitive.
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBCluster.
*
*
*
*
* @return The DB cluster identifier for the cluster being modified. This parameter is not case-sensitive.
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBCluster.
*
*
*/
public String getDBClusterIdentifier() {
return this.dBClusterIdentifier;
}
/**
*
* The DB cluster identifier for the cluster being modified. This parameter is not case-sensitive.
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBCluster.
*
*
*
*
* @param dBClusterIdentifier
* The DB cluster identifier for the cluster being modified. This parameter is not case-sensitive.
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBCluster.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDBClusterIdentifier(String dBClusterIdentifier) {
setDBClusterIdentifier(dBClusterIdentifier);
return this;
}
/**
*
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a lowercase
* string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens
*
*
* -
*
* The first character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*
*
* @param newDBClusterIdentifier
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a
* lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens
*
*
* -
*
* The first character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*/
public void setNewDBClusterIdentifier(String newDBClusterIdentifier) {
this.newDBClusterIdentifier = newDBClusterIdentifier;
}
/**
*
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a lowercase
* string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens
*
*
* -
*
* The first character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*
*
* @return The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a
* lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens
*
*
* -
*
* The first character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*/
public String getNewDBClusterIdentifier() {
return this.newDBClusterIdentifier;
}
/**
*
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a lowercase
* string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens
*
*
* -
*
* The first character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*
*
* @param newDBClusterIdentifier
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a
* lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens
*
*
* -
*
* The first character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withNewDBClusterIdentifier(String newDBClusterIdentifier) {
setNewDBClusterIdentifier(newDBClusterIdentifier);
return this;
}
/**
*
* A value that specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is set to false
, changes to the DB cluster are applied during the next
* maintenance window.
*
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If you set
* the ApplyImmediately
parameter value to false, then changes to NewDBClusterIdentifier
* values are applied during the next maintenance window. All other changes are applied immediately, regardless of
* the value of the ApplyImmediately
parameter.
*
*
* Default: false
*
*
* @param applyImmediately
* A value that specifies whether the modifications in this request and any pending modifications are
* asynchronously applied as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the DB cluster. If this parameter is set to false
, changes to the DB cluster are
* applied during the next maintenance window.
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If
* you set the ApplyImmediately
parameter value to false, then changes to
* NewDBClusterIdentifier
values are applied during the next maintenance window. All other
* changes are applied immediately, regardless of the value of the ApplyImmediately
parameter.
*
*
* Default: false
*/
public void setApplyImmediately(Boolean applyImmediately) {
this.applyImmediately = applyImmediately;
}
/**
*
* A value that specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is set to false
, changes to the DB cluster are applied during the next
* maintenance window.
*
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If you set
* the ApplyImmediately
parameter value to false, then changes to NewDBClusterIdentifier
* values are applied during the next maintenance window. All other changes are applied immediately, regardless of
* the value of the ApplyImmediately
parameter.
*
*
* Default: false
*
*
* @return A value that specifies whether the modifications in this request and any pending modifications are
* asynchronously applied as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the DB cluster. If this parameter is set to false
, changes to the DB cluster are
* applied during the next maintenance window.
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If
* you set the ApplyImmediately
parameter value to false, then changes to
* NewDBClusterIdentifier
values are applied during the next maintenance window. All other
* changes are applied immediately, regardless of the value of the ApplyImmediately
parameter.
*
*
* Default: false
*/
public Boolean getApplyImmediately() {
return this.applyImmediately;
}
/**
*
* A value that specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is set to false
, changes to the DB cluster are applied during the next
* maintenance window.
*
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If you set
* the ApplyImmediately
parameter value to false, then changes to NewDBClusterIdentifier
* values are applied during the next maintenance window. All other changes are applied immediately, regardless of
* the value of the ApplyImmediately
parameter.
*
*
* Default: false
*
*
* @param applyImmediately
* A value that specifies whether the modifications in this request and any pending modifications are
* asynchronously applied as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the DB cluster. If this parameter is set to false
, changes to the DB cluster are
* applied during the next maintenance window.
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If
* you set the ApplyImmediately
parameter value to false, then changes to
* NewDBClusterIdentifier
values are applied during the next maintenance window. All other
* changes are applied immediately, regardless of the value of the ApplyImmediately
parameter.
*
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withApplyImmediately(Boolean applyImmediately) {
setApplyImmediately(applyImmediately);
return this;
}
/**
*
* A value that specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is set to false
, changes to the DB cluster are applied during the next
* maintenance window.
*
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If you set
* the ApplyImmediately
parameter value to false, then changes to NewDBClusterIdentifier
* values are applied during the next maintenance window. All other changes are applied immediately, regardless of
* the value of the ApplyImmediately
parameter.
*
*
* Default: false
*
*
* @return A value that specifies whether the modifications in this request and any pending modifications are
* asynchronously applied as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the DB cluster. If this parameter is set to false
, changes to the DB cluster are
* applied during the next maintenance window.
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If
* you set the ApplyImmediately
parameter value to false, then changes to
* NewDBClusterIdentifier
values are applied during the next maintenance window. All other
* changes are applied immediately, regardless of the value of the ApplyImmediately
parameter.
*
*
* Default: false
*/
public Boolean isApplyImmediately() {
return this.applyImmediately;
}
/**
*
* The number of days for which automated backups are retained. You must specify a minimum value of 1.
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35
*
*
*
*
* @param backupRetentionPeriod
* The number of days for which automated backups are retained. You must specify a minimum value of 1.
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35
*
*
*/
public void setBackupRetentionPeriod(Integer backupRetentionPeriod) {
this.backupRetentionPeriod = backupRetentionPeriod;
}
/**
*
* The number of days for which automated backups are retained. You must specify a minimum value of 1.
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35
*
*
*
*
* @return The number of days for which automated backups are retained. You must specify a minimum value of 1.
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35
*
*
*/
public Integer getBackupRetentionPeriod() {
return this.backupRetentionPeriod;
}
/**
*
* The number of days for which automated backups are retained. You must specify a minimum value of 1.
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35
*
*
*
*
* @param backupRetentionPeriod
* The number of days for which automated backups are retained. You must specify a minimum value of 1.
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withBackupRetentionPeriod(Integer backupRetentionPeriod) {
setBackupRetentionPeriod(backupRetentionPeriod);
return this;
}
/**
*
* The name of the DB cluster parameter group to use for the DB cluster.
*
*
* @param dBClusterParameterGroupName
* The name of the DB cluster parameter group to use for the DB cluster.
*/
public void setDBClusterParameterGroupName(String dBClusterParameterGroupName) {
this.dBClusterParameterGroupName = dBClusterParameterGroupName;
}
/**
*
* The name of the DB cluster parameter group to use for the DB cluster.
*
*
* @return The name of the DB cluster parameter group to use for the DB cluster.
*/
public String getDBClusterParameterGroupName() {
return this.dBClusterParameterGroupName;
}
/**
*
* The name of the DB cluster parameter group to use for the DB cluster.
*
*
* @param dBClusterParameterGroupName
* The name of the DB cluster parameter group to use for the DB cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDBClusterParameterGroupName(String dBClusterParameterGroupName) {
setDBClusterParameterGroupName(dBClusterParameterGroupName);
return this;
}
/**
*
* A list of VPC security groups that the DB cluster will belong to.
*
*
* @return A list of VPC security groups that the DB cluster will belong to.
*/
public java.util.List getVpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
/**
*
* A list of VPC security groups that the DB cluster will belong to.
*
*
* @param vpcSecurityGroupIds
* A list of VPC security groups that the DB cluster will belong to.
*/
public void setVpcSecurityGroupIds(java.util.Collection vpcSecurityGroupIds) {
if (vpcSecurityGroupIds == null) {
this.vpcSecurityGroupIds = null;
return;
}
this.vpcSecurityGroupIds = new java.util.ArrayList(vpcSecurityGroupIds);
}
/**
*
* A list of VPC security groups that the DB cluster will belong to.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVpcSecurityGroupIds(java.util.Collection)} or {@link #withVpcSecurityGroupIds(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param vpcSecurityGroupIds
* A list of VPC security groups that the DB cluster will belong to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withVpcSecurityGroupIds(String... vpcSecurityGroupIds) {
if (this.vpcSecurityGroupIds == null) {
setVpcSecurityGroupIds(new java.util.ArrayList(vpcSecurityGroupIds.length));
}
for (String ele : vpcSecurityGroupIds) {
this.vpcSecurityGroupIds.add(ele);
}
return this;
}
/**
*
* A list of VPC security groups that the DB cluster will belong to.
*
*
* @param vpcSecurityGroupIds
* A list of VPC security groups that the DB cluster will belong to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withVpcSecurityGroupIds(java.util.Collection vpcSecurityGroupIds) {
setVpcSecurityGroupIds(vpcSecurityGroupIds);
return this;
}
/**
*
* The port number on which the DB cluster accepts connections.
*
*
* Constraints: Value must be 1150-65535
*
*
* Default: The same port as the original DB cluster.
*
*
* @param port
* The port number on which the DB cluster accepts connections.
*
* Constraints: Value must be 1150-65535
*
*
* Default: The same port as the original DB cluster.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* The port number on which the DB cluster accepts connections.
*
*
* Constraints: Value must be 1150-65535
*
*
* Default: The same port as the original DB cluster.
*
*
* @return The port number on which the DB cluster accepts connections.
*
* Constraints: Value must be 1150-65535
*
*
* Default: The same port as the original DB cluster.
*/
public Integer getPort() {
return this.port;
}
/**
*
* The port number on which the DB cluster accepts connections.
*
*
* Constraints: Value must be 1150-65535
*
*
* Default: The same port as the original DB cluster.
*
*
* @param port
* The port number on which the DB cluster accepts connections.
*
* Constraints: Value must be 1150-65535
*
*
* Default: The same port as the original DB cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* Not supported by Neptune.
*
*
* @param masterUserPassword
* Not supported by Neptune.
*/
public void setMasterUserPassword(String masterUserPassword) {
this.masterUserPassword = masterUserPassword;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public String getMasterUserPassword() {
return this.masterUserPassword;
}
/**
*
* Not supported by Neptune.
*
*
* @param masterUserPassword
* Not supported by Neptune.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withMasterUserPassword(String masterUserPassword) {
setMasterUserPassword(masterUserPassword);
return this;
}
/**
*
* Not supported by Neptune.
*
*
* @param optionGroupName
* Not supported by Neptune.
*/
public void setOptionGroupName(String optionGroupName) {
this.optionGroupName = optionGroupName;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public String getOptionGroupName() {
return this.optionGroupName;
}
/**
*
* Not supported by Neptune.
*
*
* @param optionGroupName
* Not supported by Neptune.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withOptionGroupName(String optionGroupName) {
setOptionGroupName(optionGroupName);
return this;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, using the
* BackupRetentionPeriod
parameter.
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @param preferredBackupWindow
* The daily time range during which automated backups are created if automated backups are enabled, using
* the BackupRetentionPeriod
parameter.
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*/
public void setPreferredBackupWindow(String preferredBackupWindow) {
this.preferredBackupWindow = preferredBackupWindow;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, using the
* BackupRetentionPeriod
parameter.
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @return The daily time range during which automated backups are created if automated backups are enabled, using
* the BackupRetentionPeriod
parameter.
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*/
public String getPreferredBackupWindow() {
return this.preferredBackupWindow;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, using the
* BackupRetentionPeriod
parameter.
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @param preferredBackupWindow
* The daily time range during which automated backups are created if automated backups are enabled, using
* the BackupRetentionPeriod
parameter.
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withPreferredBackupWindow(String preferredBackupWindow) {
setPreferredBackupWindow(preferredBackupWindow);
return this;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun.
*
*
* Constraints: Minimum 30-minute window.
*
*
* @param preferredMaintenanceWindow
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun.
*
*
* Constraints: Minimum 30-minute window.
*/
public void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun.
*
*
* Constraints: Minimum 30-minute window.
*
*
* @return The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun.
*
*
* Constraints: Minimum 30-minute window.
*/
public String getPreferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun.
*
*
* Constraints: Minimum 30-minute window.
*
*
* @param preferredMaintenanceWindow
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun.
*
*
* Constraints: Minimum 30-minute window.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
setPreferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
*
* True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
*
* Default: false
*
*
* @param enableIAMDatabaseAuthentication
* True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
* Default: false
*/
public void setEnableIAMDatabaseAuthentication(Boolean enableIAMDatabaseAuthentication) {
this.enableIAMDatabaseAuthentication = enableIAMDatabaseAuthentication;
}
/**
*
* True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
*
* Default: false
*
*
* @return True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
* Default: false
*/
public Boolean getEnableIAMDatabaseAuthentication() {
return this.enableIAMDatabaseAuthentication;
}
/**
*
* True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
*
* Default: false
*
*
* @param enableIAMDatabaseAuthentication
* True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEnableIAMDatabaseAuthentication(Boolean enableIAMDatabaseAuthentication) {
setEnableIAMDatabaseAuthentication(enableIAMDatabaseAuthentication);
return this;
}
/**
*
* True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
*
* Default: false
*
*
* @return True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
* Default: false
*/
public Boolean isEnableIAMDatabaseAuthentication() {
return this.enableIAMDatabaseAuthentication;
}
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster. See Using the
* CLI to publish Neptune audit logs to CloudWatch Logs.
*
*
* @param cloudwatchLogsExportConfiguration
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster. See Using
* the CLI to publish Neptune audit logs to CloudWatch Logs.
*/
public void setCloudwatchLogsExportConfiguration(CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration) {
this.cloudwatchLogsExportConfiguration = cloudwatchLogsExportConfiguration;
}
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster. See Using the
* CLI to publish Neptune audit logs to CloudWatch Logs.
*
*
* @return The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster. See Using the CLI to publish Neptune audit logs to CloudWatch Logs.
*/
public CloudwatchLogsExportConfiguration getCloudwatchLogsExportConfiguration() {
return this.cloudwatchLogsExportConfiguration;
}
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster. See Using the
* CLI to publish Neptune audit logs to CloudWatch Logs.
*
*
* @param cloudwatchLogsExportConfiguration
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster. See Using
* the CLI to publish Neptune audit logs to CloudWatch Logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withCloudwatchLogsExportConfiguration(CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration) {
setCloudwatchLogsExportConfiguration(cloudwatchLogsExportConfiguration);
return this;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter results in an
* outage. The change is applied during the next maintenance window unless the ApplyImmediately
* parameter is set to true.
*
*
* For a list of valid engine versions, see Engine Releases for Amazon
* Neptune, or call DescribeDBEngineVersions.
*
*
* @param engineVersion
* The version number of the database engine to which you want to upgrade. Changing this parameter results in
* an outage. The change is applied during the next maintenance window unless the
* ApplyImmediately
parameter is set to true.
*
* For a list of valid engine versions, see Engine Releases for
* Amazon Neptune, or call DescribeDBEngineVersions.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter results in an
* outage. The change is applied during the next maintenance window unless the ApplyImmediately
* parameter is set to true.
*
*
* For a list of valid engine versions, see Engine Releases for Amazon
* Neptune, or call DescribeDBEngineVersions.
*
*
* @return The version number of the database engine to which you want to upgrade. Changing this parameter results
* in an outage. The change is applied during the next maintenance window unless the
* ApplyImmediately
parameter is set to true.
*
* For a list of valid engine versions, see Engine Releases for
* Amazon Neptune, or call DescribeDBEngineVersions.
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter results in an
* outage. The change is applied during the next maintenance window unless the ApplyImmediately
* parameter is set to true.
*
*
* For a list of valid engine versions, see Engine Releases for Amazon
* Neptune, or call DescribeDBEngineVersions.
*
*
* @param engineVersion
* The version number of the database engine to which you want to upgrade. Changing this parameter results in
* an outage. The change is applied during the next maintenance window unless the
* ApplyImmediately
parameter is set to true.
*
* For a list of valid engine versions, see Engine Releases for
* Amazon Neptune, or call DescribeDBEngineVersions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* A value that indicates whether upgrades between different major versions are allowed.
*
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an EngineVersion
* parameter that uses a different major version than the DB cluster's current version.
*
*
* @param allowMajorVersionUpgrade
* A value that indicates whether upgrades between different major versions are allowed.
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an
* EngineVersion
parameter that uses a different major version than the DB cluster's current
* version.
*/
public void setAllowMajorVersionUpgrade(Boolean allowMajorVersionUpgrade) {
this.allowMajorVersionUpgrade = allowMajorVersionUpgrade;
}
/**
*
* A value that indicates whether upgrades between different major versions are allowed.
*
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an EngineVersion
* parameter that uses a different major version than the DB cluster's current version.
*
*
* @return A value that indicates whether upgrades between different major versions are allowed.
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an
* EngineVersion
parameter that uses a different major version than the DB cluster's current
* version.
*/
public Boolean getAllowMajorVersionUpgrade() {
return this.allowMajorVersionUpgrade;
}
/**
*
* A value that indicates whether upgrades between different major versions are allowed.
*
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an EngineVersion
* parameter that uses a different major version than the DB cluster's current version.
*
*
* @param allowMajorVersionUpgrade
* A value that indicates whether upgrades between different major versions are allowed.
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an
* EngineVersion
parameter that uses a different major version than the DB cluster's current
* version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withAllowMajorVersionUpgrade(Boolean allowMajorVersionUpgrade) {
setAllowMajorVersionUpgrade(allowMajorVersionUpgrade);
return this;
}
/**
*
* A value that indicates whether upgrades between different major versions are allowed.
*
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an EngineVersion
* parameter that uses a different major version than the DB cluster's current version.
*
*
* @return A value that indicates whether upgrades between different major versions are allowed.
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an
* EngineVersion
parameter that uses a different major version than the DB cluster's current
* version.
*/
public Boolean isAllowMajorVersionUpgrade() {
return this.allowMajorVersionUpgrade;
}
/**
*
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*
*
* When you apply a parameter group using DBInstanceParameterGroupName
, parameter changes aren't
* applied during the next maintenance window but instead are applied immediately.
*
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as the target DB cluster version.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is only valid in combination with the
* AllowMajorVersionUpgrade
parameter.
*
*
*
*
* @param dBInstanceParameterGroupName
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
* When you apply a parameter group using DBInstanceParameterGroupName
, parameter changes aren't
* applied during the next maintenance window but instead are applied immediately.
*
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as the target DB cluster version.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is only valid in combination with the
* AllowMajorVersionUpgrade
parameter.
*
*
*/
public void setDBInstanceParameterGroupName(String dBInstanceParameterGroupName) {
this.dBInstanceParameterGroupName = dBInstanceParameterGroupName;
}
/**
*
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*
*
* When you apply a parameter group using DBInstanceParameterGroupName
, parameter changes aren't
* applied during the next maintenance window but instead are applied immediately.
*
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as the target DB cluster version.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is only valid in combination with the
* AllowMajorVersionUpgrade
parameter.
*
*
*
*
* @return The name of the DB parameter group to apply to all instances of the DB cluster.
*
* When you apply a parameter group using DBInstanceParameterGroupName
, parameter changes
* aren't applied during the next maintenance window but instead are applied immediately.
*
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as the target DB cluster version.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is only valid in combination with the
* AllowMajorVersionUpgrade
parameter.
*
*
*/
public String getDBInstanceParameterGroupName() {
return this.dBInstanceParameterGroupName;
}
/**
*
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*
*
* When you apply a parameter group using DBInstanceParameterGroupName
, parameter changes aren't
* applied during the next maintenance window but instead are applied immediately.
*
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as the target DB cluster version.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is only valid in combination with the
* AllowMajorVersionUpgrade
parameter.
*
*
*
*
* @param dBInstanceParameterGroupName
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
* When you apply a parameter group using DBInstanceParameterGroupName
, parameter changes aren't
* applied during the next maintenance window but instead are applied immediately.
*
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as the target DB cluster version.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is only valid in combination with the
* AllowMajorVersionUpgrade
parameter.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDBInstanceParameterGroupName(String dBInstanceParameterGroupName) {
setDBInstanceParameterGroupName(dBInstanceParameterGroupName);
return this;
}
/**
*
* A value that indicates whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection is disabled.
*
*
* @param deletionProtection
* A value that indicates whether the DB cluster has deletion protection enabled. The database can't be
* deleted when deletion protection is enabled. By default, deletion protection is disabled.
*/
public void setDeletionProtection(Boolean deletionProtection) {
this.deletionProtection = deletionProtection;
}
/**
*
* A value that indicates whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection is disabled.
*
*
* @return A value that indicates whether the DB cluster has deletion protection enabled. The database can't be
* deleted when deletion protection is enabled. By default, deletion protection is disabled.
*/
public Boolean getDeletionProtection() {
return this.deletionProtection;
}
/**
*
* A value that indicates whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection is disabled.
*
*
* @param deletionProtection
* A value that indicates whether the DB cluster has deletion protection enabled. The database can't be
* deleted when deletion protection is enabled. By default, deletion protection is disabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDeletionProtection(Boolean deletionProtection) {
setDeletionProtection(deletionProtection);
return this;
}
/**
*
* A value that indicates whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection is disabled.
*
*
* @return A value that indicates whether the DB cluster has deletion protection enabled. The database can't be
* deleted when deletion protection is enabled. By default, deletion protection is disabled.
*/
public Boolean isDeletionProtection() {
return this.deletionProtection;
}
/**
*
* If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*
*
* @param copyTagsToSnapshot
* If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*/
public void setCopyTagsToSnapshot(Boolean copyTagsToSnapshot) {
this.copyTagsToSnapshot = copyTagsToSnapshot;
}
/**
*
* If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*
*
* @return If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*/
public Boolean getCopyTagsToSnapshot() {
return this.copyTagsToSnapshot;
}
/**
*
* If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*
*
* @param copyTagsToSnapshot
* If set to true
, tags are copied to any snapshot of the DB cluster that is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withCopyTagsToSnapshot(Boolean copyTagsToSnapshot) {
setCopyTagsToSnapshot(copyTagsToSnapshot);
return this;
}
/**
*
* If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*
*
* @return If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*/
public Boolean isCopyTagsToSnapshot() {
return this.copyTagsToSnapshot;
}
/**
*
* Contains the scaling configuration of a Neptune Serverless DB cluster.
*
*
* For more information, see Using Amazon Neptune
* Serverless in the Amazon Neptune User Guide.
*
*
* @param serverlessV2ScalingConfiguration
* Contains the scaling configuration of a Neptune Serverless DB cluster.
*
* For more information, see Using Amazon
* Neptune Serverless in the Amazon Neptune User Guide.
*/
public void setServerlessV2ScalingConfiguration(ServerlessV2ScalingConfiguration serverlessV2ScalingConfiguration) {
this.serverlessV2ScalingConfiguration = serverlessV2ScalingConfiguration;
}
/**
*
* Contains the scaling configuration of a Neptune Serverless DB cluster.
*
*
* For more information, see Using Amazon Neptune
* Serverless in the Amazon Neptune User Guide.
*
*
* @return Contains the scaling configuration of a Neptune Serverless DB cluster.
*
* For more information, see Using Amazon
* Neptune Serverless in the Amazon Neptune User Guide.
*/
public ServerlessV2ScalingConfiguration getServerlessV2ScalingConfiguration() {
return this.serverlessV2ScalingConfiguration;
}
/**
*
* Contains the scaling configuration of a Neptune Serverless DB cluster.
*
*
* For more information, see Using Amazon Neptune
* Serverless in the Amazon Neptune User Guide.
*
*
* @param serverlessV2ScalingConfiguration
* Contains the scaling configuration of a Neptune Serverless DB cluster.
*
* For more information, see Using Amazon
* Neptune Serverless in the Amazon Neptune User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withServerlessV2ScalingConfiguration(ServerlessV2ScalingConfiguration serverlessV2ScalingConfiguration) {
setServerlessV2ScalingConfiguration(serverlessV2ScalingConfiguration);
return this;
}
/**
*
* The storage type to associate with the DB cluster.
*
*
* Valid Values:
*
*
* -
*
* standard | iopt1
*
*
*
*
* Default:
*
*
* -
*
* standard
*
*
*
*
* @param storageType
* The storage type to associate with the DB cluster.
*
* Valid Values:
*
*
* -
*
* standard | iopt1
*
*
*
*
* Default:
*
*
* -
*
* standard
*
*
*/
public void setStorageType(String storageType) {
this.storageType = storageType;
}
/**
*
* The storage type to associate with the DB cluster.
*
*
* Valid Values:
*
*
* -
*
* standard | iopt1
*
*
*
*
* Default:
*
*
* -
*
* standard
*
*
*
*
* @return The storage type to associate with the DB cluster.
*
* Valid Values:
*
*
* -
*
* standard | iopt1
*
*
*
*
* Default:
*
*
* -
*
* standard
*
*
*/
public String getStorageType() {
return this.storageType;
}
/**
*
* The storage type to associate with the DB cluster.
*
*
* Valid Values:
*
*
* -
*
* standard | iopt1
*
*
*
*
* Default:
*
*
* -
*
* standard
*
*
*
*
* @param storageType
* The storage type to associate with the DB cluster.
*
* Valid Values:
*
*
* -
*
* standard | iopt1
*
*
*
*
* Default:
*
*
* -
*
* standard
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withStorageType(String storageType) {
setStorageType(storageType);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDBClusterIdentifier() != null)
sb.append("DBClusterIdentifier: ").append(getDBClusterIdentifier()).append(",");
if (getNewDBClusterIdentifier() != null)
sb.append("NewDBClusterIdentifier: ").append(getNewDBClusterIdentifier()).append(",");
if (getApplyImmediately() != null)
sb.append("ApplyImmediately: ").append(getApplyImmediately()).append(",");
if (getBackupRetentionPeriod() != null)
sb.append("BackupRetentionPeriod: ").append(getBackupRetentionPeriod()).append(",");
if (getDBClusterParameterGroupName() != null)
sb.append("DBClusterParameterGroupName: ").append(getDBClusterParameterGroupName()).append(",");
if (getVpcSecurityGroupIds() != null)
sb.append("VpcSecurityGroupIds: ").append(getVpcSecurityGroupIds()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getMasterUserPassword() != null)
sb.append("MasterUserPassword: ").append(getMasterUserPassword()).append(",");
if (getOptionGroupName() != null)
sb.append("OptionGroupName: ").append(getOptionGroupName()).append(",");
if (getPreferredBackupWindow() != null)
sb.append("PreferredBackupWindow: ").append(getPreferredBackupWindow()).append(",");
if (getPreferredMaintenanceWindow() != null)
sb.append("PreferredMaintenanceWindow: ").append(getPreferredMaintenanceWindow()).append(",");
if (getEnableIAMDatabaseAuthentication() != null)
sb.append("EnableIAMDatabaseAuthentication: ").append(getEnableIAMDatabaseAuthentication()).append(",");
if (getCloudwatchLogsExportConfiguration() != null)
sb.append("CloudwatchLogsExportConfiguration: ").append(getCloudwatchLogsExportConfiguration()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getAllowMajorVersionUpgrade() != null)
sb.append("AllowMajorVersionUpgrade: ").append(getAllowMajorVersionUpgrade()).append(",");
if (getDBInstanceParameterGroupName() != null)
sb.append("DBInstanceParameterGroupName: ").append(getDBInstanceParameterGroupName()).append(",");
if (getDeletionProtection() != null)
sb.append("DeletionProtection: ").append(getDeletionProtection()).append(",");
if (getCopyTagsToSnapshot() != null)
sb.append("CopyTagsToSnapshot: ").append(getCopyTagsToSnapshot()).append(",");
if (getServerlessV2ScalingConfiguration() != null)
sb.append("ServerlessV2ScalingConfiguration: ").append(getServerlessV2ScalingConfiguration()).append(",");
if (getStorageType() != null)
sb.append("StorageType: ").append(getStorageType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyDBClusterRequest == false)
return false;
ModifyDBClusterRequest other = (ModifyDBClusterRequest) obj;
if (other.getDBClusterIdentifier() == null ^ this.getDBClusterIdentifier() == null)
return false;
if (other.getDBClusterIdentifier() != null && other.getDBClusterIdentifier().equals(this.getDBClusterIdentifier()) == false)
return false;
if (other.getNewDBClusterIdentifier() == null ^ this.getNewDBClusterIdentifier() == null)
return false;
if (other.getNewDBClusterIdentifier() != null && other.getNewDBClusterIdentifier().equals(this.getNewDBClusterIdentifier()) == false)
return false;
if (other.getApplyImmediately() == null ^ this.getApplyImmediately() == null)
return false;
if (other.getApplyImmediately() != null && other.getApplyImmediately().equals(this.getApplyImmediately()) == false)
return false;
if (other.getBackupRetentionPeriod() == null ^ this.getBackupRetentionPeriod() == null)
return false;
if (other.getBackupRetentionPeriod() != null && other.getBackupRetentionPeriod().equals(this.getBackupRetentionPeriod()) == false)
return false;
if (other.getDBClusterParameterGroupName() == null ^ this.getDBClusterParameterGroupName() == null)
return false;
if (other.getDBClusterParameterGroupName() != null && other.getDBClusterParameterGroupName().equals(this.getDBClusterParameterGroupName()) == false)
return false;
if (other.getVpcSecurityGroupIds() == null ^ this.getVpcSecurityGroupIds() == null)
return false;
if (other.getVpcSecurityGroupIds() != null && other.getVpcSecurityGroupIds().equals(this.getVpcSecurityGroupIds()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getMasterUserPassword() == null ^ this.getMasterUserPassword() == null)
return false;
if (other.getMasterUserPassword() != null && other.getMasterUserPassword().equals(this.getMasterUserPassword()) == false)
return false;
if (other.getOptionGroupName() == null ^ this.getOptionGroupName() == null)
return false;
if (other.getOptionGroupName() != null && other.getOptionGroupName().equals(this.getOptionGroupName()) == false)
return false;
if (other.getPreferredBackupWindow() == null ^ this.getPreferredBackupWindow() == null)
return false;
if (other.getPreferredBackupWindow() != null && other.getPreferredBackupWindow().equals(this.getPreferredBackupWindow()) == false)
return false;
if (other.getPreferredMaintenanceWindow() == null ^ this.getPreferredMaintenanceWindow() == null)
return false;
if (other.getPreferredMaintenanceWindow() != null && other.getPreferredMaintenanceWindow().equals(this.getPreferredMaintenanceWindow()) == false)
return false;
if (other.getEnableIAMDatabaseAuthentication() == null ^ this.getEnableIAMDatabaseAuthentication() == null)
return false;
if (other.getEnableIAMDatabaseAuthentication() != null
&& other.getEnableIAMDatabaseAuthentication().equals(this.getEnableIAMDatabaseAuthentication()) == false)
return false;
if (other.getCloudwatchLogsExportConfiguration() == null ^ this.getCloudwatchLogsExportConfiguration() == null)
return false;
if (other.getCloudwatchLogsExportConfiguration() != null
&& other.getCloudwatchLogsExportConfiguration().equals(this.getCloudwatchLogsExportConfiguration()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getAllowMajorVersionUpgrade() == null ^ this.getAllowMajorVersionUpgrade() == null)
return false;
if (other.getAllowMajorVersionUpgrade() != null && other.getAllowMajorVersionUpgrade().equals(this.getAllowMajorVersionUpgrade()) == false)
return false;
if (other.getDBInstanceParameterGroupName() == null ^ this.getDBInstanceParameterGroupName() == null)
return false;
if (other.getDBInstanceParameterGroupName() != null && other.getDBInstanceParameterGroupName().equals(this.getDBInstanceParameterGroupName()) == false)
return false;
if (other.getDeletionProtection() == null ^ this.getDeletionProtection() == null)
return false;
if (other.getDeletionProtection() != null && other.getDeletionProtection().equals(this.getDeletionProtection()) == false)
return false;
if (other.getCopyTagsToSnapshot() == null ^ this.getCopyTagsToSnapshot() == null)
return false;
if (other.getCopyTagsToSnapshot() != null && other.getCopyTagsToSnapshot().equals(this.getCopyTagsToSnapshot()) == false)
return false;
if (other.getServerlessV2ScalingConfiguration() == null ^ this.getServerlessV2ScalingConfiguration() == null)
return false;
if (other.getServerlessV2ScalingConfiguration() != null
&& other.getServerlessV2ScalingConfiguration().equals(this.getServerlessV2ScalingConfiguration()) == false)
return false;
if (other.getStorageType() == null ^ this.getStorageType() == null)
return false;
if (other.getStorageType() != null && other.getStorageType().equals(this.getStorageType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDBClusterIdentifier() == null) ? 0 : getDBClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getNewDBClusterIdentifier() == null) ? 0 : getNewDBClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getApplyImmediately() == null) ? 0 : getApplyImmediately().hashCode());
hashCode = prime * hashCode + ((getBackupRetentionPeriod() == null) ? 0 : getBackupRetentionPeriod().hashCode());
hashCode = prime * hashCode + ((getDBClusterParameterGroupName() == null) ? 0 : getDBClusterParameterGroupName().hashCode());
hashCode = prime * hashCode + ((getVpcSecurityGroupIds() == null) ? 0 : getVpcSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getMasterUserPassword() == null) ? 0 : getMasterUserPassword().hashCode());
hashCode = prime * hashCode + ((getOptionGroupName() == null) ? 0 : getOptionGroupName().hashCode());
hashCode = prime * hashCode + ((getPreferredBackupWindow() == null) ? 0 : getPreferredBackupWindow().hashCode());
hashCode = prime * hashCode + ((getPreferredMaintenanceWindow() == null) ? 0 : getPreferredMaintenanceWindow().hashCode());
hashCode = prime * hashCode + ((getEnableIAMDatabaseAuthentication() == null) ? 0 : getEnableIAMDatabaseAuthentication().hashCode());
hashCode = prime * hashCode + ((getCloudwatchLogsExportConfiguration() == null) ? 0 : getCloudwatchLogsExportConfiguration().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getAllowMajorVersionUpgrade() == null) ? 0 : getAllowMajorVersionUpgrade().hashCode());
hashCode = prime * hashCode + ((getDBInstanceParameterGroupName() == null) ? 0 : getDBInstanceParameterGroupName().hashCode());
hashCode = prime * hashCode + ((getDeletionProtection() == null) ? 0 : getDeletionProtection().hashCode());
hashCode = prime * hashCode + ((getCopyTagsToSnapshot() == null) ? 0 : getCopyTagsToSnapshot().hashCode());
hashCode = prime * hashCode + ((getServerlessV2ScalingConfiguration() == null) ? 0 : getServerlessV2ScalingConfiguration().hashCode());
hashCode = prime * hashCode + ((getStorageType() == null) ? 0 : getStorageType().hashCode());
return hashCode;
}
@Override
public ModifyDBClusterRequest clone() {
return (ModifyDBClusterRequest) super.clone();
}
}