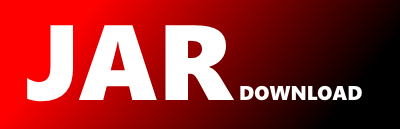
com.amazonaws.services.neptune.model.ModifyGlobalClusterRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-neptune Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.neptune.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyGlobalClusterRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The DB cluster identifier for the global cluster being modified. This parameter is not case-sensitive.
*
*
* Constraints: Must match the identifier of an existing global database cluster.
*
*/
private String globalClusterIdentifier;
/**
*
* A new cluster identifier to assign to the global database. This value is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*
*/
private String newGlobalClusterIdentifier;
/**
*
* Indicates whether the global database has deletion protection enabled. The global database cannot be deleted when
* deletion protection is enabled.
*
*/
private Boolean deletionProtection;
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter will result in an
* outage. The change is applied during the next maintenance window unless ApplyImmediately
is enabled.
*
*
* To list all of the available Neptune engine versions, use the following command:
*
*/
private String engineVersion;
/**
*
* A value that indicates whether major version upgrades are allowed.
*
*
* Constraints: You must allow major version upgrades if you specify a value for the EngineVersion
* parameter that is a different major version than the DB cluster's current version.
*
*
* If you upgrade the major version of a global database, the cluster and DB instance parameter groups are set to
* the default parameter groups for the new version, so you will need to apply any custom parameter groups after
* completing the upgrade.
*
*/
private Boolean allowMajorVersionUpgrade;
/**
*
* The DB cluster identifier for the global cluster being modified. This parameter is not case-sensitive.
*
*
* Constraints: Must match the identifier of an existing global database cluster.
*
*
* @param globalClusterIdentifier
* The DB cluster identifier for the global cluster being modified. This parameter is not case-sensitive.
*
* Constraints: Must match the identifier of an existing global database cluster.
*/
public void setGlobalClusterIdentifier(String globalClusterIdentifier) {
this.globalClusterIdentifier = globalClusterIdentifier;
}
/**
*
* The DB cluster identifier for the global cluster being modified. This parameter is not case-sensitive.
*
*
* Constraints: Must match the identifier of an existing global database cluster.
*
*
* @return The DB cluster identifier for the global cluster being modified. This parameter is not
* case-sensitive.
*
* Constraints: Must match the identifier of an existing global database cluster.
*/
public String getGlobalClusterIdentifier() {
return this.globalClusterIdentifier;
}
/**
*
* The DB cluster identifier for the global cluster being modified. This parameter is not case-sensitive.
*
*
* Constraints: Must match the identifier of an existing global database cluster.
*
*
* @param globalClusterIdentifier
* The DB cluster identifier for the global cluster being modified. This parameter is not case-sensitive.
*
* Constraints: Must match the identifier of an existing global database cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyGlobalClusterRequest withGlobalClusterIdentifier(String globalClusterIdentifier) {
setGlobalClusterIdentifier(globalClusterIdentifier);
return this;
}
/**
*
* A new cluster identifier to assign to the global database. This value is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*
*
* @param newGlobalClusterIdentifier
* A new cluster identifier to assign to the global database. This value is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*/
public void setNewGlobalClusterIdentifier(String newGlobalClusterIdentifier) {
this.newGlobalClusterIdentifier = newGlobalClusterIdentifier;
}
/**
*
* A new cluster identifier to assign to the global database. This value is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*
*
* @return A new cluster identifier to assign to the global database. This value is stored as a lowercase
* string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*/
public String getNewGlobalClusterIdentifier() {
return this.newGlobalClusterIdentifier;
}
/**
*
* A new cluster identifier to assign to the global database. This value is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*
*
* @param newGlobalClusterIdentifier
* A new cluster identifier to assign to the global database. This value is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyGlobalClusterRequest withNewGlobalClusterIdentifier(String newGlobalClusterIdentifier) {
setNewGlobalClusterIdentifier(newGlobalClusterIdentifier);
return this;
}
/**
*
* Indicates whether the global database has deletion protection enabled. The global database cannot be deleted when
* deletion protection is enabled.
*
*
* @param deletionProtection
* Indicates whether the global database has deletion protection enabled. The global database cannot be
* deleted when deletion protection is enabled.
*/
public void setDeletionProtection(Boolean deletionProtection) {
this.deletionProtection = deletionProtection;
}
/**
*
* Indicates whether the global database has deletion protection enabled. The global database cannot be deleted when
* deletion protection is enabled.
*
*
* @return Indicates whether the global database has deletion protection enabled. The global database cannot be
* deleted when deletion protection is enabled.
*/
public Boolean getDeletionProtection() {
return this.deletionProtection;
}
/**
*
* Indicates whether the global database has deletion protection enabled. The global database cannot be deleted when
* deletion protection is enabled.
*
*
* @param deletionProtection
* Indicates whether the global database has deletion protection enabled. The global database cannot be
* deleted when deletion protection is enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyGlobalClusterRequest withDeletionProtection(Boolean deletionProtection) {
setDeletionProtection(deletionProtection);
return this;
}
/**
*
* Indicates whether the global database has deletion protection enabled. The global database cannot be deleted when
* deletion protection is enabled.
*
*
* @return Indicates whether the global database has deletion protection enabled. The global database cannot be
* deleted when deletion protection is enabled.
*/
public Boolean isDeletionProtection() {
return this.deletionProtection;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter will result in an
* outage. The change is applied during the next maintenance window unless ApplyImmediately
is enabled.
*
*
* To list all of the available Neptune engine versions, use the following command:
*
*
* @param engineVersion
* The version number of the database engine to which you want to upgrade. Changing this parameter will
* result in an outage. The change is applied during the next maintenance window unless
* ApplyImmediately
is enabled.
*
* To list all of the available Neptune engine versions, use the following command:
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter will result in an
* outage. The change is applied during the next maintenance window unless ApplyImmediately
is enabled.
*
*
* To list all of the available Neptune engine versions, use the following command:
*
*
* @return The version number of the database engine to which you want to upgrade. Changing this parameter will
* result in an outage. The change is applied during the next maintenance window unless
* ApplyImmediately
is enabled.
*
* To list all of the available Neptune engine versions, use the following command:
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter will result in an
* outage. The change is applied during the next maintenance window unless ApplyImmediately
is enabled.
*
*
* To list all of the available Neptune engine versions, use the following command:
*
*
* @param engineVersion
* The version number of the database engine to which you want to upgrade. Changing this parameter will
* result in an outage. The change is applied during the next maintenance window unless
* ApplyImmediately
is enabled.
*
* To list all of the available Neptune engine versions, use the following command:
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyGlobalClusterRequest withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* A value that indicates whether major version upgrades are allowed.
*
*
* Constraints: You must allow major version upgrades if you specify a value for the EngineVersion
* parameter that is a different major version than the DB cluster's current version.
*
*
* If you upgrade the major version of a global database, the cluster and DB instance parameter groups are set to
* the default parameter groups for the new version, so you will need to apply any custom parameter groups after
* completing the upgrade.
*
*
* @param allowMajorVersionUpgrade
* A value that indicates whether major version upgrades are allowed.
*
* Constraints: You must allow major version upgrades if you specify a value for the
* EngineVersion
parameter that is a different major version than the DB cluster's current
* version.
*
*
* If you upgrade the major version of a global database, the cluster and DB instance parameter groups are
* set to the default parameter groups for the new version, so you will need to apply any custom parameter
* groups after completing the upgrade.
*/
public void setAllowMajorVersionUpgrade(Boolean allowMajorVersionUpgrade) {
this.allowMajorVersionUpgrade = allowMajorVersionUpgrade;
}
/**
*
* A value that indicates whether major version upgrades are allowed.
*
*
* Constraints: You must allow major version upgrades if you specify a value for the EngineVersion
* parameter that is a different major version than the DB cluster's current version.
*
*
* If you upgrade the major version of a global database, the cluster and DB instance parameter groups are set to
* the default parameter groups for the new version, so you will need to apply any custom parameter groups after
* completing the upgrade.
*
*
* @return A value that indicates whether major version upgrades are allowed.
*
* Constraints: You must allow major version upgrades if you specify a value for the
* EngineVersion
parameter that is a different major version than the DB cluster's current
* version.
*
*
* If you upgrade the major version of a global database, the cluster and DB instance parameter groups are
* set to the default parameter groups for the new version, so you will need to apply any custom parameter
* groups after completing the upgrade.
*/
public Boolean getAllowMajorVersionUpgrade() {
return this.allowMajorVersionUpgrade;
}
/**
*
* A value that indicates whether major version upgrades are allowed.
*
*
* Constraints: You must allow major version upgrades if you specify a value for the EngineVersion
* parameter that is a different major version than the DB cluster's current version.
*
*
* If you upgrade the major version of a global database, the cluster and DB instance parameter groups are set to
* the default parameter groups for the new version, so you will need to apply any custom parameter groups after
* completing the upgrade.
*
*
* @param allowMajorVersionUpgrade
* A value that indicates whether major version upgrades are allowed.
*
* Constraints: You must allow major version upgrades if you specify a value for the
* EngineVersion
parameter that is a different major version than the DB cluster's current
* version.
*
*
* If you upgrade the major version of a global database, the cluster and DB instance parameter groups are
* set to the default parameter groups for the new version, so you will need to apply any custom parameter
* groups after completing the upgrade.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyGlobalClusterRequest withAllowMajorVersionUpgrade(Boolean allowMajorVersionUpgrade) {
setAllowMajorVersionUpgrade(allowMajorVersionUpgrade);
return this;
}
/**
*
* A value that indicates whether major version upgrades are allowed.
*
*
* Constraints: You must allow major version upgrades if you specify a value for the EngineVersion
* parameter that is a different major version than the DB cluster's current version.
*
*
* If you upgrade the major version of a global database, the cluster and DB instance parameter groups are set to
* the default parameter groups for the new version, so you will need to apply any custom parameter groups after
* completing the upgrade.
*
*
* @return A value that indicates whether major version upgrades are allowed.
*
* Constraints: You must allow major version upgrades if you specify a value for the
* EngineVersion
parameter that is a different major version than the DB cluster's current
* version.
*
*
* If you upgrade the major version of a global database, the cluster and DB instance parameter groups are
* set to the default parameter groups for the new version, so you will need to apply any custom parameter
* groups after completing the upgrade.
*/
public Boolean isAllowMajorVersionUpgrade() {
return this.allowMajorVersionUpgrade;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getGlobalClusterIdentifier() != null)
sb.append("GlobalClusterIdentifier: ").append(getGlobalClusterIdentifier()).append(",");
if (getNewGlobalClusterIdentifier() != null)
sb.append("NewGlobalClusterIdentifier: ").append(getNewGlobalClusterIdentifier()).append(",");
if (getDeletionProtection() != null)
sb.append("DeletionProtection: ").append(getDeletionProtection()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getAllowMajorVersionUpgrade() != null)
sb.append("AllowMajorVersionUpgrade: ").append(getAllowMajorVersionUpgrade());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyGlobalClusterRequest == false)
return false;
ModifyGlobalClusterRequest other = (ModifyGlobalClusterRequest) obj;
if (other.getGlobalClusterIdentifier() == null ^ this.getGlobalClusterIdentifier() == null)
return false;
if (other.getGlobalClusterIdentifier() != null && other.getGlobalClusterIdentifier().equals(this.getGlobalClusterIdentifier()) == false)
return false;
if (other.getNewGlobalClusterIdentifier() == null ^ this.getNewGlobalClusterIdentifier() == null)
return false;
if (other.getNewGlobalClusterIdentifier() != null && other.getNewGlobalClusterIdentifier().equals(this.getNewGlobalClusterIdentifier()) == false)
return false;
if (other.getDeletionProtection() == null ^ this.getDeletionProtection() == null)
return false;
if (other.getDeletionProtection() != null && other.getDeletionProtection().equals(this.getDeletionProtection()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getAllowMajorVersionUpgrade() == null ^ this.getAllowMajorVersionUpgrade() == null)
return false;
if (other.getAllowMajorVersionUpgrade() != null && other.getAllowMajorVersionUpgrade().equals(this.getAllowMajorVersionUpgrade()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getGlobalClusterIdentifier() == null) ? 0 : getGlobalClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getNewGlobalClusterIdentifier() == null) ? 0 : getNewGlobalClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getDeletionProtection() == null) ? 0 : getDeletionProtection().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getAllowMajorVersionUpgrade() == null) ? 0 : getAllowMajorVersionUpgrade().hashCode());
return hashCode;
}
@Override
public ModifyGlobalClusterRequest clone() {
return (ModifyGlobalClusterRequest) super.clone();
}
}