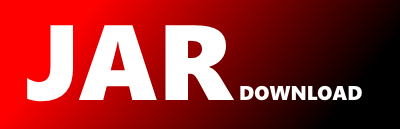
com.amazonaws.services.neptunedata.AmazonNeptunedata Maven / Gradle / Ivy
Show all versions of aws-java-sdk-neptunedata Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.neptunedata;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.neptunedata.model.*;
/**
* Interface for accessing Amazon NeptuneData.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.neptunedata.AbstractAmazonNeptunedata} instead.
*
*
*
* Neptune Data API
*
* The Amazon Neptune data API provides SDK support for more than 40 of Neptune's data operations, including data
* loading, query execution, data inquiry, and machine learning. It supports the Gremlin and openCypher query languages,
* and is available in all SDK languages. It automatically signs API requests and greatly simplifies integrating Neptune
* into your applications.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonNeptunedata {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "neptune-db";
/**
*
* Cancels a Gremlin query. See Gremlin query
* cancellation for more information.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:CancelQuery IAM action in that cluster.
*
*
* @param cancelGremlinQueryRequest
* @return Result of the CancelGremlinQuery operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.CancelGremlinQuery
* @see AWS
* API Documentation
*/
CancelGremlinQueryResult cancelGremlinQuery(CancelGremlinQueryRequest cancelGremlinQueryRequest);
/**
*
* Cancels a specified load job. This is an HTTP DELETE
request. See Neptune Loader
* Get-Status API for more information.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:CancelLoaderJob IAM action in that cluster..
*
*
* @param cancelLoaderJobRequest
* @return Result of the CancelLoaderJob operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws BulkLoadIdNotFoundException
* Raised when a specified bulk-load job ID cannot be found.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws LoadUrlAccessDeniedException
* Raised when access is denied to a specified load URL.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws InternalFailureException
* Raised when the processing of the request failed unexpectedly.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.CancelLoaderJob
* @see AWS
* API Documentation
*/
CancelLoaderJobResult cancelLoaderJob(CancelLoaderJobRequest cancelLoaderJobRequest);
/**
*
* Cancels a Neptune ML data processing job. See The
* dataprocessing
command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:CancelMLDataProcessingJob IAM action in that cluster.
*
*
* @param cancelMLDataProcessingJobRequest
* @return Result of the CancelMLDataProcessingJob operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.CancelMLDataProcessingJob
* @see AWS API Documentation
*/
CancelMLDataProcessingJobResult cancelMLDataProcessingJob(CancelMLDataProcessingJobRequest cancelMLDataProcessingJobRequest);
/**
*
* Cancels a Neptune ML model training job. See Model
* training using the modeltraining
command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:CancelMLModelTrainingJob IAM action in that cluster.
*
*
* @param cancelMLModelTrainingJobRequest
* @return Result of the CancelMLModelTrainingJob operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.CancelMLModelTrainingJob
* @see AWS API Documentation
*/
CancelMLModelTrainingJobResult cancelMLModelTrainingJob(CancelMLModelTrainingJobRequest cancelMLModelTrainingJobRequest);
/**
*
* Cancels a specified model transform job. See Use a trained
* model to generate new model artifacts.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:CancelMLModelTransformJob IAM action in that cluster.
*
*
* @param cancelMLModelTransformJobRequest
* @return Result of the CancelMLModelTransformJob operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.CancelMLModelTransformJob
* @see AWS API Documentation
*/
CancelMLModelTransformJobResult cancelMLModelTransformJob(CancelMLModelTransformJobRequest cancelMLModelTransformJobRequest);
/**
*
* Cancels a specified openCypher query. See Neptune
* openCypher status endpoint for more information.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:CancelQuery IAM action in that cluster.
*
*
* @param cancelOpenCypherQueryRequest
* @return Result of the CancelOpenCypherQuery operation returned by the service.
* @throws InvalidNumericDataException
* Raised when invalid numerical data is encountered when servicing a request.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.CancelOpenCypherQuery
* @see AWS API Documentation
*/
CancelOpenCypherQueryResult cancelOpenCypherQuery(CancelOpenCypherQueryRequest cancelOpenCypherQueryRequest);
/**
*
* Creates a new Neptune ML inference endpoint that lets you query one specific model that the model-training
* process constructed. See Managing
* inference endpoints using the endpoints command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:CreateMLEndpoint IAM action in that cluster.
*
*
* @param createMLEndpointRequest
* @return Result of the CreateMLEndpoint operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.CreateMLEndpoint
* @see AWS
* API Documentation
*/
CreateMLEndpointResult createMLEndpoint(CreateMLEndpointRequest createMLEndpointRequest);
/**
*
* Cancels the creation of a Neptune ML inference endpoint. See Managing
* inference endpoints using the endpoints command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:DeleteMLEndpoint IAM action in that cluster.
*
*
* @param deleteMLEndpointRequest
* @return Result of the DeleteMLEndpoint operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.DeleteMLEndpoint
* @see AWS
* API Documentation
*/
DeleteMLEndpointResult deleteMLEndpoint(DeleteMLEndpointRequest deleteMLEndpointRequest);
/**
*
* Deletes statistics for Gremlin and openCypher (property graph) data.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:DeleteStatistics IAM action in that cluster.
*
*
* @param deletePropertygraphStatisticsRequest
* @return Result of the DeletePropertygraphStatistics operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws StatisticsNotAvailableException
* Raised when statistics needed to satisfy a request are not available.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.DeletePropertygraphStatistics
* @see AWS API Documentation
*/
DeletePropertygraphStatisticsResult deletePropertygraphStatistics(DeletePropertygraphStatisticsRequest deletePropertygraphStatisticsRequest);
/**
*
* Deletes SPARQL statistics
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:DeleteStatistics IAM action in that cluster.
*
*
* @param deleteSparqlStatisticsRequest
* @return Result of the DeleteSparqlStatistics operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws StatisticsNotAvailableException
* Raised when statistics needed to satisfy a request are not available.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.DeleteSparqlStatistics
* @see AWS API Documentation
*/
DeleteSparqlStatisticsResult deleteSparqlStatistics(DeleteSparqlStatisticsRequest deleteSparqlStatisticsRequest);
/**
*
* The fast reset REST API lets you reset a Neptune graph quicky and easily, removing all of its data.
*
*
* Neptune fast reset is a two-step process. First you call ExecuteFastReset
with action
* set to initiateDatabaseReset
. This returns a UUID token which you then include when calling
* ExecuteFastReset
again with action
set to performDatabaseReset
. See Empty an Amazon
* Neptune DB cluster using the fast reset API.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:ResetDatabase IAM action in that cluster.
*
*
* @param executeFastResetRequest
* @return Result of the ExecuteFastReset operation returned by the service.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws ServerShutdownException
* Raised when the server shuts down while processing a request.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws MethodNotAllowedException
* Raised when the HTTP method used by a request is not supported by the endpoint being used.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.ExecuteFastReset
* @see AWS
* API Documentation
*/
ExecuteFastResetResult executeFastReset(ExecuteFastResetRequest executeFastResetRequest);
/**
*
* Executes a Gremlin Explain query.
*
*
* Amazon Neptune has added a Gremlin feature named explain
that provides is a self-service tool for
* understanding the execution approach being taken by the Neptune engine for the query. You invoke it by adding an
* explain
parameter to an HTTP call that submits a Gremlin query.
*
*
* The explain feature provides information about the logical structure of query execution plans. You can use this
* information to identify potential evaluation and execution bottlenecks and to tune your query, as explained in Tuning Gremlin
* queries. You can also use query hints to improve query execution plans.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows one of the following IAM actions in that cluster,
* depending on the query:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* Note that the neptune-db:QueryLanguage:Gremlin IAM condition key can be used in the policy document to restrict the use of
* Gremlin queries (see Condition keys available
* in Neptune IAM data-access policy statements).
*
*
* @param executeGremlinExplainQueryRequest
* @return Result of the ExecuteGremlinExplainQuery operation returned by the service.
* @throws QueryTooLargeException
* Raised when the body of a query is too large.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws QueryLimitExceededException
* Raised when the number of active queries exceeds what the server can process. The query in question can
* be retried when the system is less busy.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws QueryLimitException
* Raised when the size of a query exceeds the system limit.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws CancelledByUserException
* Raised when a user cancelled a request.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws MemoryLimitExceededException
* Raised when a request fails because of insufficient memory resources. The request can be retried.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws MalformedQueryException
* Raised when a query is submitted that is syntactically incorrect or does not pass additional validation.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.ExecuteGremlinExplainQuery
* @see AWS API Documentation
*/
ExecuteGremlinExplainQueryResult executeGremlinExplainQuery(ExecuteGremlinExplainQueryRequest executeGremlinExplainQueryRequest);
/**
*
* Executes a Gremlin Profile query, which runs a specified traversal, collects various metrics about the run, and
* produces a profile report as output. See Gremlin profile API in
* Neptune for details.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:ReadDataViaQuery IAM action in that cluster.
*
*
* Note that the neptune-db:QueryLanguage:Gremlin IAM condition key can be used in the policy document to restrict the use of
* Gremlin queries (see Condition keys available
* in Neptune IAM data-access policy statements).
*
*
* @param executeGremlinProfileQueryRequest
* @return Result of the ExecuteGremlinProfileQuery operation returned by the service.
* @throws QueryTooLargeException
* Raised when the body of a query is too large.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws QueryLimitExceededException
* Raised when the number of active queries exceeds what the server can process. The query in question can
* be retried when the system is less busy.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws QueryLimitException
* Raised when the size of a query exceeds the system limit.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws CancelledByUserException
* Raised when a user cancelled a request.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws MemoryLimitExceededException
* Raised when a request fails because of insufficient memory resources. The request can be retried.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws MalformedQueryException
* Raised when a query is submitted that is syntactically incorrect or does not pass additional validation.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.ExecuteGremlinProfileQuery
* @see AWS API Documentation
*/
ExecuteGremlinProfileQueryResult executeGremlinProfileQuery(ExecuteGremlinProfileQueryRequest executeGremlinProfileQueryRequest);
/**
*
* This commands executes a Gremlin query. Amazon Neptune is compatible with Apache TinkerPop3 and Gremlin, so you
* can use the Gremlin traversal language to query the graph, as described under The Graph in the Apache TinkerPop3
* documentation. More details can also be found in Accessing a Neptune graph
* with Gremlin.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that enables one of the following IAM actions in that cluster,
* depending on the query:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* Note that the neptune-db:QueryLanguage:Gremlin IAM condition key can be used in the policy document to restrict the use of
* Gremlin queries (see Condition keys available
* in Neptune IAM data-access policy statements).
*
*
* @param executeGremlinQueryRequest
* @return Result of the ExecuteGremlinQuery operation returned by the service.
* @throws QueryTooLargeException
* Raised when the body of a query is too large.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws QueryLimitExceededException
* Raised when the number of active queries exceeds what the server can process. The query in question can
* be retried when the system is less busy.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws QueryLimitException
* Raised when the size of a query exceeds the system limit.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws CancelledByUserException
* Raised when a user cancelled a request.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws MemoryLimitExceededException
* Raised when a request fails because of insufficient memory resources. The request can be retried.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws MalformedQueryException
* Raised when a query is submitted that is syntactically incorrect or does not pass additional validation.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.ExecuteGremlinQuery
* @see AWS API Documentation
*/
ExecuteGremlinQueryResult executeGremlinQuery(ExecuteGremlinQueryRequest executeGremlinQueryRequest);
/**
*
* Executes an openCypher explain
request. See The openCypher
* explain feature for more information.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:ReadDataViaQuery IAM action in that cluster.
*
*
* Note that the neptune-db:QueryLanguage:OpenCypher IAM condition key can be used in the policy document to restrict the use
* of openCypher queries (see Condition keys available
* in Neptune IAM data-access policy statements).
*
*
* @param executeOpenCypherExplainQueryRequest
* @return Result of the ExecuteOpenCypherExplainQuery operation returned by the service.
* @throws QueryTooLargeException
* Raised when the body of a query is too large.
* @throws InvalidNumericDataException
* Raised when invalid numerical data is encountered when servicing a request.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws QueryLimitExceededException
* Raised when the number of active queries exceeds what the server can process. The query in question can
* be retried when the system is less busy.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws QueryLimitException
* Raised when the size of a query exceeds the system limit.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws CancelledByUserException
* Raised when a user cancelled a request.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws MemoryLimitExceededException
* Raised when a request fails because of insufficient memory resources. The request can be retried.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws MalformedQueryException
* Raised when a query is submitted that is syntactically incorrect or does not pass additional validation.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.ExecuteOpenCypherExplainQuery
* @see AWS API Documentation
*/
ExecuteOpenCypherExplainQueryResult executeOpenCypherExplainQuery(ExecuteOpenCypherExplainQueryRequest executeOpenCypherExplainQueryRequest);
/**
*
* Retrieves the status of the graph database on the host.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetEngineStatus IAM action in that cluster.
*
*
* @param getEngineStatusRequest
* @return Result of the GetEngineStatus operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws InternalFailureException
* Raised when the processing of the request failed unexpectedly.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.GetEngineStatus
* @see AWS
* API Documentation
*/
GetEngineStatusResult getEngineStatus(GetEngineStatusRequest getEngineStatusRequest);
/**
*
* Gets the status of a specified Gremlin query.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetQueryStatus IAM action in that cluster.
*
*
* Note that the neptune-db:QueryLanguage:Gremlin IAM condition key can be used in the policy document to restrict the use of
* Gremlin queries (see Condition keys available
* in Neptune IAM data-access policy statements).
*
*
* @param getGremlinQueryStatusRequest
* @return Result of the GetGremlinQueryStatus operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.GetGremlinQueryStatus
* @see AWS API Documentation
*/
GetGremlinQueryStatusResult getGremlinQueryStatus(GetGremlinQueryStatusRequest getGremlinQueryStatusRequest);
/**
*
* Retrieves information about a specified data processing job. See The
* dataprocessing
command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:neptune-db:GetMLDataProcessingJobStatus IAM action in that cluster.
*
*
* @param getMLDataProcessingJobRequest
* @return Result of the GetMLDataProcessingJob operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.GetMLDataProcessingJob
* @see AWS API Documentation
*/
GetMLDataProcessingJobResult getMLDataProcessingJob(GetMLDataProcessingJobRequest getMLDataProcessingJobRequest);
/**
*
* Retrieves details about an inference endpoint. See Managing
* inference endpoints using the endpoints command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetMLEndpointStatus IAM action in that cluster.
*
*
* @param getMLEndpointRequest
* @return Result of the GetMLEndpoint operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.GetMLEndpoint
* @see AWS API
* Documentation
*/
GetMLEndpointResult getMLEndpoint(GetMLEndpointRequest getMLEndpointRequest);
/**
*
* Retrieves information about a Neptune ML model training job. See Model
* training using the modeltraining
command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetMLModelTrainingJobStatus IAM action in that cluster.
*
*
* @param getMLModelTrainingJobRequest
* @return Result of the GetMLModelTrainingJob operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.GetMLModelTrainingJob
* @see AWS API Documentation
*/
GetMLModelTrainingJobResult getMLModelTrainingJob(GetMLModelTrainingJobRequest getMLModelTrainingJobRequest);
/**
*
* Gets information about a specified model transform job. See Use a trained
* model to generate new model artifacts.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetMLModelTransformJobStatus IAM action in that cluster.
*
*
* @param getMLModelTransformJobRequest
* @return Result of the GetMLModelTransformJob operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.GetMLModelTransformJob
* @see AWS API Documentation
*/
GetMLModelTransformJobResult getMLModelTransformJob(GetMLModelTransformJobRequest getMLModelTransformJobRequest);
/**
*
* Retrieves the status of a specified openCypher query.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetQueryStatus IAM action in that cluster.
*
*
* Note that the neptune-db:QueryLanguage:OpenCypher IAM condition key can be used in the policy document to restrict the use
* of openCypher queries (see Condition keys available
* in Neptune IAM data-access policy statements).
*
*
* @param getOpenCypherQueryStatusRequest
* @return Result of the GetOpenCypherQueryStatus operation returned by the service.
* @throws InvalidNumericDataException
* Raised when invalid numerical data is encountered when servicing a request.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.GetOpenCypherQueryStatus
* @see AWS API Documentation
*/
GetOpenCypherQueryStatusResult getOpenCypherQueryStatus(GetOpenCypherQueryStatusRequest getOpenCypherQueryStatusRequest);
/**
*
* Gets property graph statistics (Gremlin and openCypher).
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetStatisticsStatus IAM action in that cluster.
*
*
* @param getPropertygraphStatisticsRequest
* @return Result of the GetPropertygraphStatistics operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws StatisticsNotAvailableException
* Raised when statistics needed to satisfy a request are not available.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.GetPropertygraphStatistics
* @see AWS API Documentation
*/
GetPropertygraphStatisticsResult getPropertygraphStatistics(GetPropertygraphStatisticsRequest getPropertygraphStatisticsRequest);
/**
*
* Gets a graph summary for a property graph.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetGraphSummary IAM action in that cluster.
*
*
* @param getPropertygraphSummaryRequest
* @return Result of the GetPropertygraphSummary operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws StatisticsNotAvailableException
* Raised when statistics needed to satisfy a request are not available.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.GetPropertygraphSummary
* @see AWS API Documentation
*/
GetPropertygraphSummaryResult getPropertygraphSummary(GetPropertygraphSummaryRequest getPropertygraphSummaryRequest);
/**
*
* Gets a graph summary for an RDF graph.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetGraphSummary IAM action in that cluster.
*
*
* @param getRDFGraphSummaryRequest
* @return Result of the GetRDFGraphSummary operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws StatisticsNotAvailableException
* Raised when statistics needed to satisfy a request are not available.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.GetRDFGraphSummary
* @see AWS
* API Documentation
*/
GetRDFGraphSummaryResult getRDFGraphSummary(GetRDFGraphSummaryRequest getRDFGraphSummaryRequest);
/**
*
* Gets RDF statistics (SPARQL).
*
*
* @param getSparqlStatisticsRequest
* @return Result of the GetSparqlStatistics operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws StatisticsNotAvailableException
* Raised when statistics needed to satisfy a request are not available.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.GetSparqlStatistics
* @see AWS API Documentation
*/
GetSparqlStatisticsResult getSparqlStatistics(GetSparqlStatisticsRequest getSparqlStatisticsRequest);
/**
*
* Gets a stream for an RDF graph.
*
*
* With the Neptune Streams feature, you can generate a complete sequence of change-log entries that record every
* change made to your graph data as it happens. GetSparqlStream
lets you collect these change-log
* entries for an RDF graph.
*
*
* The Neptune streams feature needs to be enabled on your Neptune DBcluster. To enable streams, set the neptune_streams DB cluster parameter to 1
.
*
*
* See Capturing graph changes in real
* time using Neptune streams.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetStreamRecords IAM action in that cluster.
*
*
* Note that the neptune-db:QueryLanguage:Sparql IAM condition key can be used in the policy document to restrict the use of
* SPARQL queries (see Condition keys available
* in Neptune IAM data-access policy statements).
*
*
* @param getSparqlStreamRequest
* @return Result of the GetSparqlStream operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws ExpiredStreamException
* Raised when a request attempts to access an stream that has expired.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws MemoryLimitExceededException
* Raised when a request fails because of insufficient memory resources. The request can be retried.
* @throws StreamRecordsNotFoundException
* Raised when stream records requested by a query cannot be found.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ThrottlingException
* Raised when the rate of requests exceeds the maximum throughput. Requests can be retried after
* encountering this exception.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.GetSparqlStream
* @see AWS
* API Documentation
*/
GetSparqlStreamResult getSparqlStream(GetSparqlStreamRequest getSparqlStreamRequest);
/**
*
* Lists active Gremlin queries. See Gremlin query status API
* for details about the output.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetQueryStatus IAM action in that cluster.
*
*
* Note that the neptune-db:QueryLanguage:Gremlin IAM condition key can be used in the policy document to restrict the use of
* Gremlin queries (see Condition keys available
* in Neptune IAM data-access policy statements).
*
*
* @param listGremlinQueriesRequest
* @return Result of the ListGremlinQueries operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.ListGremlinQueries
* @see AWS
* API Documentation
*/
ListGremlinQueriesResult listGremlinQueries(ListGremlinQueriesRequest listGremlinQueriesRequest);
/**
*
* Retrieves a list of the loadIds
for all active loader jobs.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:ListLoaderJobs IAM action in that cluster..
*
*
* @param listLoaderJobsRequest
* @return Result of the ListLoaderJobs operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws BulkLoadIdNotFoundException
* Raised when a specified bulk-load job ID cannot be found.
* @throws InternalFailureException
* Raised when the processing of the request failed unexpectedly.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws LoadUrlAccessDeniedException
* Raised when access is denied to a specified load URL.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.ListLoaderJobs
* @see AWS API
* Documentation
*/
ListLoaderJobsResult listLoaderJobs(ListLoaderJobsRequest listLoaderJobsRequest);
/**
*
* Returns a list of Neptune ML data processing jobs. See Listing active data-processing jobs using the Neptune ML dataprocessing command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:ListMLDataProcessingJobs IAM action in that cluster.
*
*
* @param listMLDataProcessingJobsRequest
* @return Result of the ListMLDataProcessingJobs operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.ListMLDataProcessingJobs
* @see AWS API Documentation
*/
ListMLDataProcessingJobsResult listMLDataProcessingJobs(ListMLDataProcessingJobsRequest listMLDataProcessingJobsRequest);
/**
*
* Lists existing inference endpoints. See Managing
* inference endpoints using the endpoints command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:ListMLEndpoints IAM action in that cluster.
*
*
* @param listMLEndpointsRequest
* @return Result of the ListMLEndpoints operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.ListMLEndpoints
* @see AWS
* API Documentation
*/
ListMLEndpointsResult listMLEndpoints(ListMLEndpointsRequest listMLEndpointsRequest);
/**
*
* Lists Neptune ML model-training jobs. See Model
* training using the modeltraining
command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:neptune-db:ListMLModelTrainingJobs IAM action in that cluster.
*
*
* @param listMLModelTrainingJobsRequest
* @return Result of the ListMLModelTrainingJobs operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.ListMLModelTrainingJobs
* @see AWS API Documentation
*/
ListMLModelTrainingJobsResult listMLModelTrainingJobs(ListMLModelTrainingJobsRequest listMLModelTrainingJobsRequest);
/**
*
* Returns a list of model transform job IDs. See Use a trained
* model to generate new model artifacts.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:ListMLModelTransformJobs IAM action in that cluster.
*
*
* @param listMLModelTransformJobsRequest
* @return Result of the ListMLModelTransformJobs operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.ListMLModelTransformJobs
* @see AWS API Documentation
*/
ListMLModelTransformJobsResult listMLModelTransformJobs(ListMLModelTransformJobsRequest listMLModelTransformJobsRequest);
/**
*
* Lists active openCypher queries. See Neptune
* openCypher status endpoint for more information.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:GetQueryStatus IAM action in that cluster.
*
*
* Note that the neptune-db:QueryLanguage:OpenCypher IAM condition key can be used in the policy document to restrict the use
* of openCypher queries (see Condition keys available
* in Neptune IAM data-access policy statements).
*
*
* @param listOpenCypherQueriesRequest
* @return Result of the ListOpenCypherQueries operation returned by the service.
* @throws InvalidNumericDataException
* Raised when invalid numerical data is encountered when servicing a request.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws FailureByQueryException
* Raised when a request fails.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ParsingException
* Raised when a parsing issue is encountered.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws TimeLimitExceededException
* Raised when the an operation exceeds the time limit allowed for it.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws ConcurrentModificationException
* Raised when a request attempts to modify data that is concurrently being modified by another process.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.ListOpenCypherQueries
* @see AWS API Documentation
*/
ListOpenCypherQueriesResult listOpenCypherQueries(ListOpenCypherQueriesRequest listOpenCypherQueriesRequest);
/**
*
* Manages the generation and use of property graph statistics.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:ManageStatistics IAM action in that cluster.
*
*
* @param managePropertygraphStatisticsRequest
* @return Result of the ManagePropertygraphStatistics operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws StatisticsNotAvailableException
* Raised when statistics needed to satisfy a request are not available.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.ManagePropertygraphStatistics
* @see AWS API Documentation
*/
ManagePropertygraphStatisticsResult managePropertygraphStatistics(ManagePropertygraphStatisticsRequest managePropertygraphStatisticsRequest);
/**
*
* Manages the generation and use of RDF graph statistics.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:ManageStatistics IAM action in that cluster.
*
*
* @param manageSparqlStatisticsRequest
* @return Result of the ManageSparqlStatistics operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws StatisticsNotAvailableException
* Raised when statistics needed to satisfy a request are not available.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws AccessDeniedException
* Raised in case of an authentication or authorization failure.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ReadOnlyViolationException
* Raised when a request attempts to write to a read-only resource.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.ManageSparqlStatistics
* @see AWS API Documentation
*/
ManageSparqlStatisticsResult manageSparqlStatistics(ManageSparqlStatisticsRequest manageSparqlStatisticsRequest);
/**
*
* Starts a Neptune bulk loader job to load data from an Amazon S3 bucket into a Neptune DB instance. See Using the Amazon Neptune Bulk Loader
* to Ingest Data.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:StartLoaderJob IAM action in that cluster.
*
*
* @param startLoaderJobRequest
* @return Result of the StartLoaderJob operation returned by the service.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws BulkLoadIdNotFoundException
* Raised when a specified bulk-load job ID cannot be found.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws LoadUrlAccessDeniedException
* Raised when access is denied to a specified load URL.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws InternalFailureException
* Raised when the processing of the request failed unexpectedly.
* @throws S3Exception
* Raised when there is a problem accessing Amazon S3.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @sample AmazonNeptunedata.StartLoaderJob
* @see AWS API
* Documentation
*/
StartLoaderJobResult startLoaderJob(StartLoaderJobRequest startLoaderJobRequest);
/**
*
* Creates a new Neptune ML data processing job for processing the graph data exported from Neptune for training.
* See The
* dataprocessing
command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:StartMLModelDataProcessingJob IAM action in that cluster.
*
*
* @param startMLDataProcessingJobRequest
* @return Result of the StartMLDataProcessingJob operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.StartMLDataProcessingJob
* @see AWS API Documentation
*/
StartMLDataProcessingJobResult startMLDataProcessingJob(StartMLDataProcessingJobRequest startMLDataProcessingJobRequest);
/**
*
* Creates a new Neptune ML model training job. See Model
* training using the modeltraining
command.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:StartMLModelTrainingJob IAM action in that cluster.
*
*
* @param startMLModelTrainingJobRequest
* @return Result of the StartMLModelTrainingJob operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.StartMLModelTrainingJob
* @see AWS API Documentation
*/
StartMLModelTrainingJobResult startMLModelTrainingJob(StartMLModelTrainingJobRequest startMLModelTrainingJobRequest);
/**
*
* Creates a new model transform job. See Use a trained
* model to generate new model artifacts.
*
*
* When invoking this operation in a Neptune cluster that has IAM authentication enabled, the IAM user or role
* making the request must have a policy attached that allows the neptune-db:StartMLModelTransformJob IAM action in that cluster.
*
*
* @param startMLModelTransformJobRequest
* @return Result of the StartMLModelTransformJob operation returned by the service.
* @throws UnsupportedOperationException
* Raised when a request attempts to initiate an operation that is not supported.
* @throws BadRequestException
* Raised when a request is submitted that cannot be processed.
* @throws MLResourceNotFoundException
* Raised when a specified machine-learning resource could not be found.
* @throws InvalidParameterException
* Raised when a parameter value is not valid.
* @throws ClientTimeoutException
* Raised when a request timed out in the client.
* @throws PreconditionsFailedException
* Raised when a precondition for processing a request is not satisfied.
* @throws ConstraintViolationException
* Raised when a value in a request field did not satisfy required constraints.
* @throws InvalidArgumentException
* Raised when an argument in a request has an invalid value.
* @throws MissingParameterException
* Raised when a required parameter is missing.
* @throws IllegalArgumentException
* Raised when an argument in a request is not supported.
* @throws TooManyRequestsException
* Raised when the number of requests being processed exceeds the limit.
* @sample AmazonNeptunedata.StartMLModelTransformJob
* @see AWS API Documentation
*/
StartMLModelTransformJobResult startMLModelTransformJob(StartMLModelTransformJobRequest startMLModelTransformJobRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}