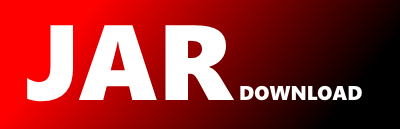
com.amazonaws.services.networkfirewall.AWSNetworkFirewallClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkfirewall Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkfirewall;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.networkfirewall.AWSNetworkFirewallClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.networkfirewall.model.*;
import com.amazonaws.services.networkfirewall.model.transform.*;
/**
* Client for accessing Network Firewall. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* This is the API Reference for Network Firewall. This guide is for developers who need detailed information about the
* Network Firewall API actions, data types, and errors.
*
*
* -
*
* The REST API requires you to handle connection details, such as calculating signatures, handling request retries, and
* error handling. For general information about using the Amazon Web Services REST APIs, see Amazon Web Services APIs.
*
*
* To access Network Firewall using the REST API endpoint:
* https://network-firewall.<region>.amazonaws.com
*
*
* -
*
* Alternatively, you can use one of the Amazon Web Services SDKs to access an API that's tailored to the programming
* language or platform that you're using. For more information, see Amazon
* Web Services SDKs.
*
*
* -
*
* For descriptions of Network Firewall features, including and step-by-step instructions on how to use them through the
* Network Firewall console, see the Network Firewall Developer Guide.
*
*
*
*
* Network Firewall is a stateful, managed, network firewall and intrusion detection and prevention service for Amazon
* Virtual Private Cloud (Amazon VPC). With Network Firewall, you can filter traffic at the perimeter of your VPC. This
* includes filtering traffic going to and coming from an internet gateway, NAT gateway, or over VPN or Direct Connect.
* Network Firewall uses rules that are compatible with Suricata, a free, open source network analysis and threat
* detection engine. Network Firewall supports Suricata version 6.0.9. For information about Suricata, see the Suricata website.
*
*
* You can use Network Firewall to monitor and protect your VPC traffic in a number of ways. The following are just a
* few examples:
*
*
* -
*
* Allow domains or IP addresses for known Amazon Web Services service endpoints, such as Amazon S3, and block all other
* forms of traffic.
*
*
* -
*
* Use custom lists of known bad domains to limit the types of domain names that your applications can access.
*
*
* -
*
* Perform deep packet inspection on traffic entering or leaving your VPC.
*
*
* -
*
* Use stateful protocol detection to filter protocols like HTTPS, regardless of the port used.
*
*
*
*
* To enable Network Firewall for your VPCs, you perform steps in both Amazon VPC and in Network Firewall. For
* information about using Amazon VPC, see Amazon VPC User
* Guide.
*
*
* To start using Network Firewall, do the following:
*
*
* -
*
* (Optional) If you don't already have a VPC that you want to protect, create it in Amazon VPC.
*
*
* -
*
* In Amazon VPC, in each Availability Zone where you want to have a firewall endpoint, create a subnet for the sole use
* of Network Firewall.
*
*
* -
*
* In Network Firewall, create stateless and stateful rule groups, to define the components of the network traffic
* filtering behavior that you want your firewall to have.
*
*
* -
*
* In Network Firewall, create a firewall policy that uses your rule groups and specifies additional default traffic
* filtering behavior.
*
*
* -
*
* In Network Firewall, create a firewall and specify your new firewall policy and VPC subnets. Network Firewall creates
* a firewall endpoint in each subnet that you specify, with the behavior that's defined in the firewall policy.
*
*
* -
*
* In Amazon VPC, use ingress routing enhancements to route traffic through the new firewall endpoints.
*
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSNetworkFirewallClient extends AmazonWebServiceClient implements AWSNetworkFirewall {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSNetworkFirewall.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "network-firewall";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.0")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidOperationException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.InvalidOperationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidRequestException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.InvalidRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LogDestinationPermissionException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.LogDestinationPermissionExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientCapacityException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.InsufficientCapacityExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidResourcePolicyException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.InvalidResourcePolicyExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTokenException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.InvalidTokenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceOwnerCheckException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.ResourceOwnerCheckExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedOperationException").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.UnsupportedOperationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerError").withExceptionUnmarshaller(
com.amazonaws.services.networkfirewall.model.transform.InternalServerErrorExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.networkfirewall.model.AWSNetworkFirewallException.class));
public static AWSNetworkFirewallClientBuilder builder() {
return AWSNetworkFirewallClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Network Firewall using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSNetworkFirewallClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Network Firewall using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSNetworkFirewallClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("network-firewall.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/networkfirewall/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/networkfirewall/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Associates a FirewallPolicy to a Firewall.
*
*
* A firewall policy defines how to monitor and manage your VPC network traffic, using a collection of inspection
* rule groups and other settings. Each firewall requires one firewall policy association, and you can use the same
* firewall policy for multiple firewalls.
*
*
* @param associateFirewallPolicyRequest
* @return Result of the AssociateFirewallPolicy operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @throws InvalidOperationException
* The operation failed because it's not valid. For example, you might have tried to delete a rule group or
* firewall policy that's in use.
* @sample AWSNetworkFirewall.AssociateFirewallPolicy
* @see AWS API Documentation
*/
@Override
public AssociateFirewallPolicyResult associateFirewallPolicy(AssociateFirewallPolicyRequest request) {
request = beforeClientExecution(request);
return executeAssociateFirewallPolicy(request);
}
@SdkInternalApi
final AssociateFirewallPolicyResult executeAssociateFirewallPolicy(AssociateFirewallPolicyRequest associateFirewallPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(associateFirewallPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateFirewallPolicyRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateFirewallPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateFirewallPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateFirewallPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates the specified subnets in the Amazon VPC to the firewall. You can specify one subnet for each of the
* Availability Zones that the VPC spans.
*
*
* This request creates an Network Firewall firewall endpoint in each of the subnets. To enable the firewall's
* protections, you must also modify the VPC's route tables for each subnet's Availability Zone, to redirect the
* traffic that's coming into and going out of the zone through the firewall endpoint.
*
*
* @param associateSubnetsRequest
* @return Result of the AssociateSubnets operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @throws InvalidOperationException
* The operation failed because it's not valid. For example, you might have tried to delete a rule group or
* firewall policy that's in use.
* @throws InsufficientCapacityException
* Amazon Web Services doesn't currently have enough available capacity to fulfill your request. Try your
* request later.
* @sample AWSNetworkFirewall.AssociateSubnets
* @see AWS API Documentation
*/
@Override
public AssociateSubnetsResult associateSubnets(AssociateSubnetsRequest request) {
request = beforeClientExecution(request);
return executeAssociateSubnets(request);
}
@SdkInternalApi
final AssociateSubnetsResult executeAssociateSubnets(AssociateSubnetsRequest associateSubnetsRequest) {
ExecutionContext executionContext = createExecutionContext(associateSubnetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateSubnetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associateSubnetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateSubnets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AssociateSubnetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Network Firewall Firewall and accompanying FirewallStatus for a VPC.
*
*
* The firewall defines the configuration settings for an Network Firewall firewall. The settings that you can
* define at creation include the firewall policy, the subnets in your VPC to use for the firewall endpoints, and
* any tags that are attached to the firewall Amazon Web Services resource.
*
*
* After you create a firewall, you can provide additional settings, like the logging configuration.
*
*
* To update the settings for a firewall, you use the operations that apply to the settings themselves, for example
* UpdateLoggingConfiguration, AssociateSubnets, and UpdateFirewallDeleteProtection.
*
*
* To manage a firewall's tags, use the standard Amazon Web Services resource tagging operations,
* ListTagsForResource, TagResource, and UntagResource.
*
*
* To retrieve information about firewalls, use ListFirewalls and DescribeFirewall.
*
*
* @param createFirewallRequest
* @return Result of the CreateFirewall operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws LimitExceededException
* Unable to perform the operation because doing so would violate a limit setting.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InsufficientCapacityException
* Amazon Web Services doesn't currently have enough available capacity to fulfill your request. Try your
* request later.
* @throws InvalidOperationException
* The operation failed because it's not valid. For example, you might have tried to delete a rule group or
* firewall policy that's in use.
* @sample AWSNetworkFirewall.CreateFirewall
* @see AWS API Documentation
*/
@Override
public CreateFirewallResult createFirewall(CreateFirewallRequest request) {
request = beforeClientExecution(request);
return executeCreateFirewall(request);
}
@SdkInternalApi
final CreateFirewallResult executeCreateFirewall(CreateFirewallRequest createFirewallRequest) {
ExecutionContext executionContext = createExecutionContext(createFirewallRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFirewallRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFirewallRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFirewall");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFirewallResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates the firewall policy for the firewall according to the specifications.
*
*
* An Network Firewall firewall policy defines the behavior of a firewall, in a collection of stateless and stateful
* rule groups and other settings. You can use one firewall policy for multiple firewalls.
*
*
* @param createFirewallPolicyRequest
* @return Result of the CreateFirewallPolicy operation returned by the service.
* @throws LimitExceededException
* Unable to perform the operation because doing so would violate a limit setting.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws InsufficientCapacityException
* Amazon Web Services doesn't currently have enough available capacity to fulfill your request. Try your
* request later.
* @sample AWSNetworkFirewall.CreateFirewallPolicy
* @see AWS API Documentation
*/
@Override
public CreateFirewallPolicyResult createFirewallPolicy(CreateFirewallPolicyRequest request) {
request = beforeClientExecution(request);
return executeCreateFirewallPolicy(request);
}
@SdkInternalApi
final CreateFirewallPolicyResult executeCreateFirewallPolicy(CreateFirewallPolicyRequest createFirewallPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(createFirewallPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFirewallPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFirewallPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFirewallPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFirewallPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates the specified stateless or stateful rule group, which includes the rules for network traffic inspection,
* a capacity setting, and tags.
*
*
* You provide your rule group specification in your request using either RuleGroup
or
* Rules
.
*
*
* @param createRuleGroupRequest
* @return Result of the CreateRuleGroup operation returned by the service.
* @throws LimitExceededException
* Unable to perform the operation because doing so would violate a limit setting.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws InsufficientCapacityException
* Amazon Web Services doesn't currently have enough available capacity to fulfill your request. Try your
* request later.
* @sample AWSNetworkFirewall.CreateRuleGroup
* @see AWS API Documentation
*/
@Override
public CreateRuleGroupResult createRuleGroup(CreateRuleGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateRuleGroup(request);
}
@SdkInternalApi
final CreateRuleGroupResult executeCreateRuleGroup(CreateRuleGroupRequest createRuleGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createRuleGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRuleGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createRuleGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRuleGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateRuleGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Network Firewall TLS inspection configuration. Network Firewall uses TLS inspection configurations to
* decrypt your firewall's inbound and outbound SSL/TLS traffic. After decryption, Network Firewall inspects the
* traffic according to your firewall policy's stateful rules, and then re-encrypts it before sending it to its
* destination. You can enable inspection of your firewall's inbound traffic, outbound traffic, or both. To use TLS
* inspection with your firewall, you must first import or provision certificates using ACM, create a TLS inspection
* configuration, add that configuration to a new firewall policy, and then associate that policy with your
* firewall.
*
*
* To update the settings for a TLS inspection configuration, use UpdateTLSInspectionConfiguration.
*
*
* To manage a TLS inspection configuration's tags, use the standard Amazon Web Services resource tagging
* operations, ListTagsForResource, TagResource, and UntagResource.
*
*
* To retrieve information about TLS inspection configurations, use ListTLSInspectionConfigurations and
* DescribeTLSInspectionConfiguration.
*
*
* For more information about TLS inspection configurations, see Inspecting SSL/TLS
* traffic with TLS inspection configurations in the Network Firewall Developer Guide.
*
*
* @param createTLSInspectionConfigurationRequest
* @return Result of the CreateTLSInspectionConfiguration operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws LimitExceededException
* Unable to perform the operation because doing so would violate a limit setting.
* @throws InsufficientCapacityException
* Amazon Web Services doesn't currently have enough available capacity to fulfill your request. Try your
* request later.
* @sample AWSNetworkFirewall.CreateTLSInspectionConfiguration
* @see AWS API Documentation
*/
@Override
public CreateTLSInspectionConfigurationResult createTLSInspectionConfiguration(CreateTLSInspectionConfigurationRequest request) {
request = beforeClientExecution(request);
return executeCreateTLSInspectionConfiguration(request);
}
@SdkInternalApi
final CreateTLSInspectionConfigurationResult executeCreateTLSInspectionConfiguration(
CreateTLSInspectionConfigurationRequest createTLSInspectionConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(createTLSInspectionConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTLSInspectionConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createTLSInspectionConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateTLSInspectionConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateTLSInspectionConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Firewall and its FirewallStatus. This operation requires the firewall's
* DeleteProtection
flag to be FALSE
. You can't revert this operation.
*
*
* You can check whether a firewall is in use by reviewing the route tables for the Availability Zones where you
* have firewall subnet mappings. Retrieve the subnet mappings by calling DescribeFirewall. You define and
* update the route tables through Amazon VPC. As needed, update the route tables for the zones to remove the
* firewall endpoints. When the route tables no longer use the firewall endpoints, you can remove the firewall
* safely.
*
*
* To delete a firewall, remove the delete protection if you need to using UpdateFirewallDeleteProtection,
* then delete the firewall by calling DeleteFirewall.
*
*
* @param deleteFirewallRequest
* @return Result of the DeleteFirewall operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws UnsupportedOperationException
* The operation you requested isn't supported by Network Firewall.
* @throws InvalidOperationException
* The operation failed because it's not valid. For example, you might have tried to delete a rule group or
* firewall policy that's in use.
* @sample AWSNetworkFirewall.DeleteFirewall
* @see AWS API Documentation
*/
@Override
public DeleteFirewallResult deleteFirewall(DeleteFirewallRequest request) {
request = beforeClientExecution(request);
return executeDeleteFirewall(request);
}
@SdkInternalApi
final DeleteFirewallResult executeDeleteFirewall(DeleteFirewallRequest deleteFirewallRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFirewallRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFirewallRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFirewallRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFirewall");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFirewallResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified FirewallPolicy.
*
*
* @param deleteFirewallPolicyRequest
* @return Result of the DeleteFirewallPolicy operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws UnsupportedOperationException
* The operation you requested isn't supported by Network Firewall.
* @throws InvalidOperationException
* The operation failed because it's not valid. For example, you might have tried to delete a rule group or
* firewall policy that's in use.
* @sample AWSNetworkFirewall.DeleteFirewallPolicy
* @see AWS API Documentation
*/
@Override
public DeleteFirewallPolicyResult deleteFirewallPolicy(DeleteFirewallPolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteFirewallPolicy(request);
}
@SdkInternalApi
final DeleteFirewallPolicyResult executeDeleteFirewallPolicy(DeleteFirewallPolicyRequest deleteFirewallPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFirewallPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFirewallPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFirewallPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFirewallPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFirewallPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a resource policy that you created in a PutResourcePolicy request.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidResourcePolicyException
* The policy statement failed validation.
* @sample AWSNetworkFirewall.DeleteResourcePolicy
* @see AWS API Documentation
*/
@Override
public DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteResourcePolicy(request);
}
@SdkInternalApi
final DeleteResourcePolicyResult executeDeleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified RuleGroup.
*
*
* @param deleteRuleGroupRequest
* @return Result of the DeleteRuleGroup operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws UnsupportedOperationException
* The operation you requested isn't supported by Network Firewall.
* @throws InvalidOperationException
* The operation failed because it's not valid. For example, you might have tried to delete a rule group or
* firewall policy that's in use.
* @sample AWSNetworkFirewall.DeleteRuleGroup
* @see AWS API Documentation
*/
@Override
public DeleteRuleGroupResult deleteRuleGroup(DeleteRuleGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteRuleGroup(request);
}
@SdkInternalApi
final DeleteRuleGroupResult executeDeleteRuleGroup(DeleteRuleGroupRequest deleteRuleGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRuleGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRuleGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteRuleGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRuleGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteRuleGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified TLSInspectionConfiguration.
*
*
* @param deleteTLSInspectionConfigurationRequest
* @return Result of the DeleteTLSInspectionConfiguration operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidOperationException
* The operation failed because it's not valid. For example, you might have tried to delete a rule group or
* firewall policy that's in use.
* @sample AWSNetworkFirewall.DeleteTLSInspectionConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteTLSInspectionConfigurationResult deleteTLSInspectionConfiguration(DeleteTLSInspectionConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteTLSInspectionConfiguration(request);
}
@SdkInternalApi
final DeleteTLSInspectionConfigurationResult executeDeleteTLSInspectionConfiguration(
DeleteTLSInspectionConfigurationRequest deleteTLSInspectionConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTLSInspectionConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteTLSInspectionConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteTLSInspectionConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteTLSInspectionConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteTLSInspectionConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the data objects for the specified firewall.
*
*
* @param describeFirewallRequest
* @return Result of the DescribeFirewall operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @sample AWSNetworkFirewall.DescribeFirewall
* @see AWS API Documentation
*/
@Override
public DescribeFirewallResult describeFirewall(DescribeFirewallRequest request) {
request = beforeClientExecution(request);
return executeDescribeFirewall(request);
}
@SdkInternalApi
final DescribeFirewallResult executeDescribeFirewall(DescribeFirewallRequest describeFirewallRequest) {
ExecutionContext executionContext = createExecutionContext(describeFirewallRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFirewallRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeFirewallRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFirewall");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeFirewallResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the data objects for the specified firewall policy.
*
*
* @param describeFirewallPolicyRequest
* @return Result of the DescribeFirewallPolicy operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @sample AWSNetworkFirewall.DescribeFirewallPolicy
* @see AWS API Documentation
*/
@Override
public DescribeFirewallPolicyResult describeFirewallPolicy(DescribeFirewallPolicyRequest request) {
request = beforeClientExecution(request);
return executeDescribeFirewallPolicy(request);
}
@SdkInternalApi
final DescribeFirewallPolicyResult executeDescribeFirewallPolicy(DescribeFirewallPolicyRequest describeFirewallPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(describeFirewallPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFirewallPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeFirewallPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFirewallPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeFirewallPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the logging configuration for the specified firewall.
*
*
* @param describeLoggingConfigurationRequest
* @return Result of the DescribeLoggingConfiguration operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @sample AWSNetworkFirewall.DescribeLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeLoggingConfigurationResult describeLoggingConfiguration(DescribeLoggingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDescribeLoggingConfiguration(request);
}
@SdkInternalApi
final DescribeLoggingConfigurationResult executeDescribeLoggingConfiguration(DescribeLoggingConfigurationRequest describeLoggingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(describeLoggingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLoggingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeLoggingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeLoggingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeLoggingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a resource policy that you created in a PutResourcePolicy request.
*
*
* @param describeResourcePolicyRequest
* @return Result of the DescribeResourcePolicy operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @sample AWSNetworkFirewall.DescribeResourcePolicy
* @see AWS API Documentation
*/
@Override
public DescribeResourcePolicyResult describeResourcePolicy(DescribeResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeDescribeResourcePolicy(request);
}
@SdkInternalApi
final DescribeResourcePolicyResult executeDescribeResourcePolicy(DescribeResourcePolicyRequest describeResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(describeResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the data objects for the specified rule group.
*
*
* @param describeRuleGroupRequest
* @return Result of the DescribeRuleGroup operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @sample AWSNetworkFirewall.DescribeRuleGroup
* @see AWS API Documentation
*/
@Override
public DescribeRuleGroupResult describeRuleGroup(DescribeRuleGroupRequest request) {
request = beforeClientExecution(request);
return executeDescribeRuleGroup(request);
}
@SdkInternalApi
final DescribeRuleGroupResult executeDescribeRuleGroup(DescribeRuleGroupRequest describeRuleGroupRequest) {
ExecutionContext executionContext = createExecutionContext(describeRuleGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRuleGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRuleGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRuleGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeRuleGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* High-level information about a rule group, returned by operations like create and describe. You can use the
* information provided in the metadata to retrieve and manage a rule group. You can retrieve all objects for a rule
* group by calling DescribeRuleGroup.
*
*
* @param describeRuleGroupMetadataRequest
* @return Result of the DescribeRuleGroupMetadata operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @sample AWSNetworkFirewall.DescribeRuleGroupMetadata
* @see AWS API Documentation
*/
@Override
public DescribeRuleGroupMetadataResult describeRuleGroupMetadata(DescribeRuleGroupMetadataRequest request) {
request = beforeClientExecution(request);
return executeDescribeRuleGroupMetadata(request);
}
@SdkInternalApi
final DescribeRuleGroupMetadataResult executeDescribeRuleGroupMetadata(DescribeRuleGroupMetadataRequest describeRuleGroupMetadataRequest) {
ExecutionContext executionContext = createExecutionContext(describeRuleGroupMetadataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRuleGroupMetadataRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeRuleGroupMetadataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRuleGroupMetadata");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeRuleGroupMetadataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the data objects for the specified TLS inspection configuration.
*
*
* @param describeTLSInspectionConfigurationRequest
* @return Result of the DescribeTLSInspectionConfiguration operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @sample AWSNetworkFirewall.DescribeTLSInspectionConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeTLSInspectionConfigurationResult describeTLSInspectionConfiguration(DescribeTLSInspectionConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDescribeTLSInspectionConfiguration(request);
}
@SdkInternalApi
final DescribeTLSInspectionConfigurationResult executeDescribeTLSInspectionConfiguration(
DescribeTLSInspectionConfigurationRequest describeTLSInspectionConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(describeTLSInspectionConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTLSInspectionConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeTLSInspectionConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeTLSInspectionConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeTLSInspectionConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified subnet associations from the firewall. This removes the firewall endpoints from the subnets
* and removes any network filtering protections that the endpoints were providing.
*
*
* @param disassociateSubnetsRequest
* @return Result of the DisassociateSubnets operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @throws InvalidOperationException
* The operation failed because it's not valid. For example, you might have tried to delete a rule group or
* firewall policy that's in use.
* @sample AWSNetworkFirewall.DisassociateSubnets
* @see AWS API Documentation
*/
@Override
public DisassociateSubnetsResult disassociateSubnets(DisassociateSubnetsRequest request) {
request = beforeClientExecution(request);
return executeDisassociateSubnets(request);
}
@SdkInternalApi
final DisassociateSubnetsResult executeDisassociateSubnets(DisassociateSubnetsRequest disassociateSubnetsRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateSubnetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateSubnetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disassociateSubnetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateSubnets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisassociateSubnetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the metadata for the firewall policies that you have defined. Depending on your setting for max results
* and the number of firewall policies, a single call might not return the full list.
*
*
* @param listFirewallPoliciesRequest
* @return Result of the ListFirewallPolicies operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @sample AWSNetworkFirewall.ListFirewallPolicies
* @see AWS API Documentation
*/
@Override
public ListFirewallPoliciesResult listFirewallPolicies(ListFirewallPoliciesRequest request) {
request = beforeClientExecution(request);
return executeListFirewallPolicies(request);
}
@SdkInternalApi
final ListFirewallPoliciesResult executeListFirewallPolicies(ListFirewallPoliciesRequest listFirewallPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(listFirewallPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFirewallPoliciesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFirewallPoliciesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFirewallPolicies");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFirewallPoliciesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the metadata for the firewalls that you have defined. If you provide VPC identifiers in your request,
* this returns only the firewalls for those VPCs.
*
*
* Depending on your setting for max results and the number of firewalls, a single call might not return the full
* list.
*
*
* @param listFirewallsRequest
* @return Result of the ListFirewalls operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @sample AWSNetworkFirewall.ListFirewalls
* @see AWS
* API Documentation
*/
@Override
public ListFirewallsResult listFirewalls(ListFirewallsRequest request) {
request = beforeClientExecution(request);
return executeListFirewalls(request);
}
@SdkInternalApi
final ListFirewallsResult executeListFirewalls(ListFirewallsRequest listFirewallsRequest) {
ExecutionContext executionContext = createExecutionContext(listFirewallsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFirewallsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFirewallsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFirewalls");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFirewallsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the metadata for the rule groups that you have defined. Depending on your setting for max results and
* the number of rule groups, a single call might not return the full list.
*
*
* @param listRuleGroupsRequest
* @return Result of the ListRuleGroups operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @sample AWSNetworkFirewall.ListRuleGroups
* @see AWS API Documentation
*/
@Override
public ListRuleGroupsResult listRuleGroups(ListRuleGroupsRequest request) {
request = beforeClientExecution(request);
return executeListRuleGroups(request);
}
@SdkInternalApi
final ListRuleGroupsResult executeListRuleGroups(ListRuleGroupsRequest listRuleGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listRuleGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRuleGroupsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRuleGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRuleGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListRuleGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the metadata for the TLS inspection configurations that you have defined. Depending on your setting for
* max results and the number of TLS inspection configurations, a single call might not return the full list.
*
*
* @param listTLSInspectionConfigurationsRequest
* @return Result of the ListTLSInspectionConfigurations operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @sample AWSNetworkFirewall.ListTLSInspectionConfigurations
* @see AWS API Documentation
*/
@Override
public ListTLSInspectionConfigurationsResult listTLSInspectionConfigurations(ListTLSInspectionConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeListTLSInspectionConfigurations(request);
}
@SdkInternalApi
final ListTLSInspectionConfigurationsResult executeListTLSInspectionConfigurations(
ListTLSInspectionConfigurationsRequest listTLSInspectionConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(listTLSInspectionConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTLSInspectionConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listTLSInspectionConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTLSInspectionConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListTLSInspectionConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the tags associated with the specified resource. Tags are key:value pairs that you can use to
* categorize and manage your resources, for purposes like billing. For example, you might set the tag key to
* "customer" and the value to the customer name or ID. You can specify one or more tags to add to each Amazon Web
* Services resource, up to 50 tags for a resource.
*
*
* You can tag the Amazon Web Services resources that you manage through Network Firewall: firewalls, firewall
* policies, and rule groups.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @sample AWSNetworkFirewall.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates or updates an IAM policy for your rule group or firewall policy. Use this to share rule groups and
* firewall policies between accounts. This operation works in conjunction with the Amazon Web Services Resource
* Access Manager (RAM) service to manage resource sharing for Network Firewall.
*
*
* Use this operation to create or update a resource policy for your rule group or firewall policy. In the policy,
* you specify the accounts that you want to share the resource with and the operations that you want the accounts
* to be able to perform.
*
*
* When you add an account in the resource policy, you then run the following Resource Access Manager (RAM)
* operations to access and accept the shared rule group or firewall policy.
*
*
* -
*
*
* GetResourceShareInvitations - Returns the Amazon Resource Names (ARNs) of the resource share invitations.
*
*
* -
*
*
* AcceptResourceShareInvitation - Accepts the share invitation for a specified resource share.
*
*
*
*
* For additional information about resource sharing using RAM, see Resource Access Manager User Guide.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidResourcePolicyException
* The policy statement failed validation.
* @sample AWSNetworkFirewall.PutResourcePolicy
* @see AWS API Documentation
*/
@Override
public PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executePutResourcePolicy(request);
}
@SdkInternalApi
final PutResourcePolicyResult executePutResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds the specified tags to the specified resource. Tags are key:value pairs that you can use to categorize and
* manage your resources, for purposes like billing. For example, you might set the tag key to "customer" and the
* value to the customer name or ID. You can specify one or more tags to add to each Amazon Web Services resource,
* up to 50 tags for a resource.
*
*
* You can tag the Amazon Web Services resources that you manage through Network Firewall: firewalls, firewall
* policies, and rule groups.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @sample AWSNetworkFirewall.TagResource
* @see AWS
* API Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the tags with the specified keys from the specified resource. Tags are key:value pairs that you can use
* to categorize and manage your resources, for purposes like billing. For example, you might set the tag key to
* "customer" and the value to the customer name or ID. You can specify one or more tags to add to each Amazon Web
* Services resource, up to 50 tags for a resource.
*
*
* You can manage tags for the Amazon Web Services resources that you manage through Network Firewall: firewalls,
* firewall policies, and rule groups.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @sample AWSNetworkFirewall.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the flag, DeleteProtection
, which indicates whether it is possible to delete the firewall.
* If the flag is set to TRUE
, the firewall is protected against deletion. This setting helps protect
* against accidentally deleting a firewall that's in use.
*
*
* @param updateFirewallDeleteProtectionRequest
* @return Result of the UpdateFirewallDeleteProtection operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @throws ResourceOwnerCheckException
* Unable to change the resource because your account doesn't own it.
* @sample AWSNetworkFirewall.UpdateFirewallDeleteProtection
* @see AWS API Documentation
*/
@Override
public UpdateFirewallDeleteProtectionResult updateFirewallDeleteProtection(UpdateFirewallDeleteProtectionRequest request) {
request = beforeClientExecution(request);
return executeUpdateFirewallDeleteProtection(request);
}
@SdkInternalApi
final UpdateFirewallDeleteProtectionResult executeUpdateFirewallDeleteProtection(UpdateFirewallDeleteProtectionRequest updateFirewallDeleteProtectionRequest) {
ExecutionContext executionContext = createExecutionContext(updateFirewallDeleteProtectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFirewallDeleteProtectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateFirewallDeleteProtectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFirewallDeleteProtection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateFirewallDeleteProtectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the description for the specified firewall. Use the description to help you identify the firewall when
* you're working with it.
*
*
* @param updateFirewallDescriptionRequest
* @return Result of the UpdateFirewallDescription operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @sample AWSNetworkFirewall.UpdateFirewallDescription
* @see AWS API Documentation
*/
@Override
public UpdateFirewallDescriptionResult updateFirewallDescription(UpdateFirewallDescriptionRequest request) {
request = beforeClientExecution(request);
return executeUpdateFirewallDescription(request);
}
@SdkInternalApi
final UpdateFirewallDescriptionResult executeUpdateFirewallDescription(UpdateFirewallDescriptionRequest updateFirewallDescriptionRequest) {
ExecutionContext executionContext = createExecutionContext(updateFirewallDescriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFirewallDescriptionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateFirewallDescriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFirewallDescription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateFirewallDescriptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* A complex type that contains settings for encryption of your firewall resources.
*
*
* @param updateFirewallEncryptionConfigurationRequest
* @return Result of the UpdateFirewallEncryptionConfiguration operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @throws ResourceOwnerCheckException
* Unable to change the resource because your account doesn't own it.
* @sample AWSNetworkFirewall.UpdateFirewallEncryptionConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateFirewallEncryptionConfigurationResult updateFirewallEncryptionConfiguration(UpdateFirewallEncryptionConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateFirewallEncryptionConfiguration(request);
}
@SdkInternalApi
final UpdateFirewallEncryptionConfigurationResult executeUpdateFirewallEncryptionConfiguration(
UpdateFirewallEncryptionConfigurationRequest updateFirewallEncryptionConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateFirewallEncryptionConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFirewallEncryptionConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateFirewallEncryptionConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFirewallEncryptionConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateFirewallEncryptionConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the properties of the specified firewall policy.
*
*
* @param updateFirewallPolicyRequest
* @return Result of the UpdateFirewallPolicy operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @sample AWSNetworkFirewall.UpdateFirewallPolicy
* @see AWS API Documentation
*/
@Override
public UpdateFirewallPolicyResult updateFirewallPolicy(UpdateFirewallPolicyRequest request) {
request = beforeClientExecution(request);
return executeUpdateFirewallPolicy(request);
}
@SdkInternalApi
final UpdateFirewallPolicyResult executeUpdateFirewallPolicy(UpdateFirewallPolicyRequest updateFirewallPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(updateFirewallPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFirewallPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateFirewallPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFirewallPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateFirewallPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the flag, ChangeProtection
, which indicates whether it is possible to change the firewall.
* If the flag is set to TRUE
, the firewall is protected from changes. This setting helps protect
* against accidentally changing a firewall that's in use.
*
*
* @param updateFirewallPolicyChangeProtectionRequest
* @return Result of the UpdateFirewallPolicyChangeProtection operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @throws ResourceOwnerCheckException
* Unable to change the resource because your account doesn't own it.
* @sample AWSNetworkFirewall.UpdateFirewallPolicyChangeProtection
* @see AWS API Documentation
*/
@Override
public UpdateFirewallPolicyChangeProtectionResult updateFirewallPolicyChangeProtection(UpdateFirewallPolicyChangeProtectionRequest request) {
request = beforeClientExecution(request);
return executeUpdateFirewallPolicyChangeProtection(request);
}
@SdkInternalApi
final UpdateFirewallPolicyChangeProtectionResult executeUpdateFirewallPolicyChangeProtection(
UpdateFirewallPolicyChangeProtectionRequest updateFirewallPolicyChangeProtectionRequest) {
ExecutionContext executionContext = createExecutionContext(updateFirewallPolicyChangeProtectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFirewallPolicyChangeProtectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateFirewallPolicyChangeProtectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFirewallPolicyChangeProtection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateFirewallPolicyChangeProtectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sets the logging configuration for the specified firewall.
*
*
* To change the logging configuration, retrieve the LoggingConfiguration by calling
* DescribeLoggingConfiguration, then change it and provide the modified object to this update call. You must
* change the logging configuration one LogDestinationConfig at a time inside the retrieved
* LoggingConfiguration object.
*
*
* You can perform only one of the following actions in any call to UpdateLoggingConfiguration
:
*
*
* -
*
* Create a new log destination object by adding a single LogDestinationConfig
array element to
* LogDestinationConfigs
.
*
*
* -
*
* Delete a log destination object by removing a single LogDestinationConfig
array element from
* LogDestinationConfigs
.
*
*
* -
*
* Change the LogDestination
setting in a single LogDestinationConfig
array element.
*
*
*
*
* You can't change the LogDestinationType
or LogType
in a
* LogDestinationConfig
. To change these settings, delete the existing
* LogDestinationConfig
object and create a new one, using two separate calls to this update operation.
*
*
* @param updateLoggingConfigurationRequest
* @return Result of the UpdateLoggingConfiguration operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @throws LogDestinationPermissionException
* Unable to send logs to a configured logging destination.
* @sample AWSNetworkFirewall.UpdateLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateLoggingConfigurationResult updateLoggingConfiguration(UpdateLoggingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateLoggingConfiguration(request);
}
@SdkInternalApi
final UpdateLoggingConfigurationResult executeUpdateLoggingConfiguration(UpdateLoggingConfigurationRequest updateLoggingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateLoggingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateLoggingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateLoggingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateLoggingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateLoggingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the rule settings for the specified rule group. You use a rule group by reference in one or more firewall
* policies. When you modify a rule group, you modify all firewall policies that use the rule group.
*
*
* To update a rule group, first call DescribeRuleGroup to retrieve the current RuleGroup object,
* update the object as needed, and then provide the updated object to this call.
*
*
* @param updateRuleGroupRequest
* @return Result of the UpdateRuleGroup operation returned by the service.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @sample AWSNetworkFirewall.UpdateRuleGroup
* @see AWS API Documentation
*/
@Override
public UpdateRuleGroupResult updateRuleGroup(UpdateRuleGroupRequest request) {
request = beforeClientExecution(request);
return executeUpdateRuleGroup(request);
}
@SdkInternalApi
final UpdateRuleGroupResult executeUpdateRuleGroup(UpdateRuleGroupRequest updateRuleGroupRequest) {
ExecutionContext executionContext = createExecutionContext(updateRuleGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateRuleGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateRuleGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateRuleGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateRuleGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* @param updateSubnetChangeProtectionRequest
* @return Result of the UpdateSubnetChangeProtection operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @throws ResourceOwnerCheckException
* Unable to change the resource because your account doesn't own it.
* @sample AWSNetworkFirewall.UpdateSubnetChangeProtection
* @see AWS API Documentation
*/
@Override
public UpdateSubnetChangeProtectionResult updateSubnetChangeProtection(UpdateSubnetChangeProtectionRequest request) {
request = beforeClientExecution(request);
return executeUpdateSubnetChangeProtection(request);
}
@SdkInternalApi
final UpdateSubnetChangeProtectionResult executeUpdateSubnetChangeProtection(UpdateSubnetChangeProtectionRequest updateSubnetChangeProtectionRequest) {
ExecutionContext executionContext = createExecutionContext(updateSubnetChangeProtectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSubnetChangeProtectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateSubnetChangeProtectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateSubnetChangeProtection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateSubnetChangeProtectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the TLS inspection configuration settings for the specified TLS inspection configuration. You use a TLS
* inspection configuration by referencing it in one or more firewall policies. When you modify a TLS inspection
* configuration, you modify all firewall policies that use the TLS inspection configuration.
*
*
* To update a TLS inspection configuration, first call DescribeTLSInspectionConfiguration to retrieve the
* current TLSInspectionConfiguration object, update the object as needed, and then provide the updated
* object to this call.
*
*
* @param updateTLSInspectionConfigurationRequest
* @return Result of the UpdateTLSInspectionConfiguration operation returned by the service.
* @throws InvalidRequestException
* The operation failed because of a problem with your request. Examples include:
*
* -
*
* You specified an unsupported parameter name or value.
*
*
* -
*
* You tried to update a property with a value that isn't among the available types.
*
*
* -
*
* Your request references an ARN that is malformed, or corresponds to a resource that isn't valid in the
* context of the request.
*
*
* @throws ResourceNotFoundException
* Unable to locate a resource using the parameters that you provided.
* @throws ThrottlingException
* Unable to process the request due to throttling limitations.
* @throws InternalServerErrorException
* Your request is valid, but Network Firewall couldn't perform the operation because of a system problem.
* Retry your request.
* @throws InvalidTokenException
* The token you provided is stale or isn't valid for the operation.
* @sample AWSNetworkFirewall.UpdateTLSInspectionConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateTLSInspectionConfigurationResult updateTLSInspectionConfiguration(UpdateTLSInspectionConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateTLSInspectionConfiguration(request);
}
@SdkInternalApi
final UpdateTLSInspectionConfigurationResult executeUpdateTLSInspectionConfiguration(
UpdateTLSInspectionConfigurationRequest updateTLSInspectionConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateTLSInspectionConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateTLSInspectionConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateTLSInspectionConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Network Firewall");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateTLSInspectionConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateTLSInspectionConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}