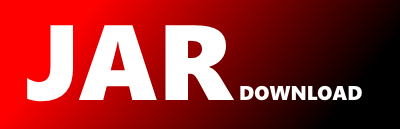
com.amazonaws.services.networkfirewall.model.CreateRuleGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkfirewall Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkfirewall.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateRuleGroupRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*
*/
private String ruleGroupName;
/**
*
* An object that defines the rule group rules.
*
*
*
* You must provide either this rule group setting or a Rules
setting, but not both.
*
*
*/
private RuleGroup ruleGroup;
/**
*
* A string containing stateful rule group rules specifications in Suricata flat format, with one rule per line. Use
* this to import your existing Suricata compatible rule groups.
*
*
*
* You must provide either this rules setting or a populated RuleGroup
setting, but not both.
*
*
*
* You can provide your rule group specification in Suricata flat format through this setting when you create or
* update your rule group. The call response returns a RuleGroup object that Network Firewall has populated
* from your string.
*
*/
private String rules;
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*/
private String type;
/**
*
* A description of the rule group.
*
*/
private String description;
/**
*
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation. When you
* update a rule group, you are limited to this capacity. When you reference a rule group from a firewall policy,
* Network Firewall reserves this capacity for the rule group.
*
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by calling
* CreateRuleGroup with DryRun
set to TRUE
.
*
*
*
* You can't change or exceed this capacity when you update the rule group, so leave room for your rule group to
* grow.
*
*
*
* Capacity for a stateless rule group
*
*
* For a stateless rule group, the capacity required is the sum of the capacity requirements of the individual rules
* that you expect to have in the rule group.
*
*
* To calculate the capacity requirement of a single rule, multiply the capacity requirement values of each of the
* rule's match settings:
*
*
* -
*
* A match setting with no criteria specified has a value of 1.
*
*
* -
*
* A match setting with Any
specified has a value of 1.
*
*
* -
*
* All other match settings have a value equal to the number of elements provided in the setting. For example, a
* protocol setting ["UDP"] and a source setting ["10.0.0.0/24"] each have a value of 1. A protocol setting
* ["UDP","TCP"] has a value of 2. A source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"] has a value of 3.
*
*
*
*
* A rule with no criteria specified in any of its match settings has a capacity requirement of 1. A rule with
* protocol setting ["UDP","TCP"], source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"], and a single
* specification or no specification for each of the other match settings has a capacity requirement of 6.
*
*
* Capacity for a stateful rule group
*
*
* For a stateful rule group, the minimum capacity required is the number of individual rules that you expect to
* have in the rule group.
*
*/
private Integer capacity;
/**
*
* The key:value pairs to associate with the resource.
*
*/
private java.util.List tags;
/**
*
* Indicates whether you want Network Firewall to just check the validity of the request, rather than run the
* request.
*
*
* If set to TRUE
, Network Firewall checks whether the request can run successfully, but doesn't
* actually make the requested changes. The call returns the value that the request would return if you ran it with
* dry run set to FALSE
, but doesn't make additions or changes to your resources. This option allows
* you to make sure that you have the required permissions to run the request and that your request parameters are
* valid.
*
*
* If set to FALSE
, Network Firewall makes the requested changes to your resources.
*
*/
private Boolean dryRun;
/**
*
* A complex type that contains settings for encryption of your rule group resources.
*
*/
private EncryptionConfiguration encryptionConfiguration;
/**
*
* A complex type that contains metadata about the rule group that your own rule group is copied from. You can use
* the metadata to keep track of updates made to the originating rule group.
*
*/
private SourceMetadata sourceMetadata;
/**
*
* Indicates whether you want Network Firewall to analyze the stateless rules in the rule group for rule behavior
* such as asymmetric routing. If set to TRUE
, Network Firewall runs the analysis and then creates the
* rule group for you. To run the stateless rule group analyzer without creating the rule group, set
* DryRun
to TRUE
.
*
*/
private Boolean analyzeRuleGroup;
/**
*
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*
*
* @param ruleGroupName
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*/
public void setRuleGroupName(String ruleGroupName) {
this.ruleGroupName = ruleGroupName;
}
/**
*
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*
*
* @return The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*/
public String getRuleGroupName() {
return this.ruleGroupName;
}
/**
*
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*
*
* @param ruleGroupName
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withRuleGroupName(String ruleGroupName) {
setRuleGroupName(ruleGroupName);
return this;
}
/**
*
* An object that defines the rule group rules.
*
*
*
* You must provide either this rule group setting or a Rules
setting, but not both.
*
*
*
* @param ruleGroup
* An object that defines the rule group rules.
*
* You must provide either this rule group setting or a Rules
setting, but not both.
*
*/
public void setRuleGroup(RuleGroup ruleGroup) {
this.ruleGroup = ruleGroup;
}
/**
*
* An object that defines the rule group rules.
*
*
*
* You must provide either this rule group setting or a Rules
setting, but not both.
*
*
*
* @return An object that defines the rule group rules.
*
* You must provide either this rule group setting or a Rules
setting, but not both.
*
*/
public RuleGroup getRuleGroup() {
return this.ruleGroup;
}
/**
*
* An object that defines the rule group rules.
*
*
*
* You must provide either this rule group setting or a Rules
setting, but not both.
*
*
*
* @param ruleGroup
* An object that defines the rule group rules.
*
* You must provide either this rule group setting or a Rules
setting, but not both.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withRuleGroup(RuleGroup ruleGroup) {
setRuleGroup(ruleGroup);
return this;
}
/**
*
* A string containing stateful rule group rules specifications in Suricata flat format, with one rule per line. Use
* this to import your existing Suricata compatible rule groups.
*
*
*
* You must provide either this rules setting or a populated RuleGroup
setting, but not both.
*
*
*
* You can provide your rule group specification in Suricata flat format through this setting when you create or
* update your rule group. The call response returns a RuleGroup object that Network Firewall has populated
* from your string.
*
*
* @param rules
* A string containing stateful rule group rules specifications in Suricata flat format, with one rule per
* line. Use this to import your existing Suricata compatible rule groups.
*
* You must provide either this rules setting or a populated RuleGroup
setting, but not both.
*
*
*
* You can provide your rule group specification in Suricata flat format through this setting when you create
* or update your rule group. The call response returns a RuleGroup object that Network Firewall has
* populated from your string.
*/
public void setRules(String rules) {
this.rules = rules;
}
/**
*
* A string containing stateful rule group rules specifications in Suricata flat format, with one rule per line. Use
* this to import your existing Suricata compatible rule groups.
*
*
*
* You must provide either this rules setting or a populated RuleGroup
setting, but not both.
*
*
*
* You can provide your rule group specification in Suricata flat format through this setting when you create or
* update your rule group. The call response returns a RuleGroup object that Network Firewall has populated
* from your string.
*
*
* @return A string containing stateful rule group rules specifications in Suricata flat format, with one rule per
* line. Use this to import your existing Suricata compatible rule groups.
*
* You must provide either this rules setting or a populated RuleGroup
setting, but not both.
*
*
*
* You can provide your rule group specification in Suricata flat format through this setting when you
* create or update your rule group. The call response returns a RuleGroup object that Network
* Firewall has populated from your string.
*/
public String getRules() {
return this.rules;
}
/**
*
* A string containing stateful rule group rules specifications in Suricata flat format, with one rule per line. Use
* this to import your existing Suricata compatible rule groups.
*
*
*
* You must provide either this rules setting or a populated RuleGroup
setting, but not both.
*
*
*
* You can provide your rule group specification in Suricata flat format through this setting when you create or
* update your rule group. The call response returns a RuleGroup object that Network Firewall has populated
* from your string.
*
*
* @param rules
* A string containing stateful rule group rules specifications in Suricata flat format, with one rule per
* line. Use this to import your existing Suricata compatible rule groups.
*
* You must provide either this rules setting or a populated RuleGroup
setting, but not both.
*
*
*
* You can provide your rule group specification in Suricata flat format through this setting when you create
* or update your rule group. The call response returns a RuleGroup object that Network Firewall has
* populated from your string.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withRules(String rules) {
setRules(rules);
return this;
}
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*
* @param type
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains
* stateless rules. If it is stateful, it contains stateful rules.
* @see RuleGroupType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*
* @return Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains
* stateless rules. If it is stateful, it contains stateful rules.
* @see RuleGroupType
*/
public String getType() {
return this.type;
}
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*
* @param type
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains
* stateless rules. If it is stateful, it contains stateful rules.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RuleGroupType
*/
public CreateRuleGroupRequest withType(String type) {
setType(type);
return this;
}
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*
* @param type
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains
* stateless rules. If it is stateful, it contains stateful rules.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RuleGroupType
*/
public CreateRuleGroupRequest withType(RuleGroupType type) {
this.type = type.toString();
return this;
}
/**
*
* A description of the rule group.
*
*
* @param description
* A description of the rule group.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the rule group.
*
*
* @return A description of the rule group.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the rule group.
*
*
* @param description
* A description of the rule group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation. When you
* update a rule group, you are limited to this capacity. When you reference a rule group from a firewall policy,
* Network Firewall reserves this capacity for the rule group.
*
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by calling
* CreateRuleGroup with DryRun
set to TRUE
.
*
*
*
* You can't change or exceed this capacity when you update the rule group, so leave room for your rule group to
* grow.
*
*
*
* Capacity for a stateless rule group
*
*
* For a stateless rule group, the capacity required is the sum of the capacity requirements of the individual rules
* that you expect to have in the rule group.
*
*
* To calculate the capacity requirement of a single rule, multiply the capacity requirement values of each of the
* rule's match settings:
*
*
* -
*
* A match setting with no criteria specified has a value of 1.
*
*
* -
*
* A match setting with Any
specified has a value of 1.
*
*
* -
*
* All other match settings have a value equal to the number of elements provided in the setting. For example, a
* protocol setting ["UDP"] and a source setting ["10.0.0.0/24"] each have a value of 1. A protocol setting
* ["UDP","TCP"] has a value of 2. A source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"] has a value of 3.
*
*
*
*
* A rule with no criteria specified in any of its match settings has a capacity requirement of 1. A rule with
* protocol setting ["UDP","TCP"], source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"], and a single
* specification or no specification for each of the other match settings has a capacity requirement of 6.
*
*
* Capacity for a stateful rule group
*
*
* For a stateful rule group, the minimum capacity required is the number of individual rules that you expect to
* have in the rule group.
*
*
* @param capacity
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation.
* When you update a rule group, you are limited to this capacity. When you reference a rule group from a
* firewall policy, Network Firewall reserves this capacity for the rule group.
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by
* calling CreateRuleGroup with DryRun
set to TRUE
.
*
*
*
* You can't change or exceed this capacity when you update the rule group, so leave room for your rule group
* to grow.
*
*
*
* Capacity for a stateless rule group
*
*
* For a stateless rule group, the capacity required is the sum of the capacity requirements of the
* individual rules that you expect to have in the rule group.
*
*
* To calculate the capacity requirement of a single rule, multiply the capacity requirement values of each
* of the rule's match settings:
*
*
* -
*
* A match setting with no criteria specified has a value of 1.
*
*
* -
*
* A match setting with Any
specified has a value of 1.
*
*
* -
*
* All other match settings have a value equal to the number of elements provided in the setting. For
* example, a protocol setting ["UDP"] and a source setting ["10.0.0.0/24"] each have a value of 1. A
* protocol setting ["UDP","TCP"] has a value of 2. A source setting
* ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"] has a value of 3.
*
*
*
*
* A rule with no criteria specified in any of its match settings has a capacity requirement of 1. A rule
* with protocol setting ["UDP","TCP"], source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"], and a
* single specification or no specification for each of the other match settings has a capacity requirement
* of 6.
*
*
* Capacity for a stateful rule group
*
*
* For a stateful rule group, the minimum capacity required is the number of individual rules that you expect
* to have in the rule group.
*/
public void setCapacity(Integer capacity) {
this.capacity = capacity;
}
/**
*
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation. When you
* update a rule group, you are limited to this capacity. When you reference a rule group from a firewall policy,
* Network Firewall reserves this capacity for the rule group.
*
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by calling
* CreateRuleGroup with DryRun
set to TRUE
.
*
*
*
* You can't change or exceed this capacity when you update the rule group, so leave room for your rule group to
* grow.
*
*
*
* Capacity for a stateless rule group
*
*
* For a stateless rule group, the capacity required is the sum of the capacity requirements of the individual rules
* that you expect to have in the rule group.
*
*
* To calculate the capacity requirement of a single rule, multiply the capacity requirement values of each of the
* rule's match settings:
*
*
* -
*
* A match setting with no criteria specified has a value of 1.
*
*
* -
*
* A match setting with Any
specified has a value of 1.
*
*
* -
*
* All other match settings have a value equal to the number of elements provided in the setting. For example, a
* protocol setting ["UDP"] and a source setting ["10.0.0.0/24"] each have a value of 1. A protocol setting
* ["UDP","TCP"] has a value of 2. A source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"] has a value of 3.
*
*
*
*
* A rule with no criteria specified in any of its match settings has a capacity requirement of 1. A rule with
* protocol setting ["UDP","TCP"], source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"], and a single
* specification or no specification for each of the other match settings has a capacity requirement of 6.
*
*
* Capacity for a stateful rule group
*
*
* For a stateful rule group, the minimum capacity required is the number of individual rules that you expect to
* have in the rule group.
*
*
* @return The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation.
* When you update a rule group, you are limited to this capacity. When you reference a rule group from a
* firewall policy, Network Firewall reserves this capacity for the rule group.
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by
* calling CreateRuleGroup with DryRun
set to TRUE
.
*
*
*
* You can't change or exceed this capacity when you update the rule group, so leave room for your rule
* group to grow.
*
*
*
* Capacity for a stateless rule group
*
*
* For a stateless rule group, the capacity required is the sum of the capacity requirements of the
* individual rules that you expect to have in the rule group.
*
*
* To calculate the capacity requirement of a single rule, multiply the capacity requirement values of each
* of the rule's match settings:
*
*
* -
*
* A match setting with no criteria specified has a value of 1.
*
*
* -
*
* A match setting with Any
specified has a value of 1.
*
*
* -
*
* All other match settings have a value equal to the number of elements provided in the setting. For
* example, a protocol setting ["UDP"] and a source setting ["10.0.0.0/24"] each have a value of 1. A
* protocol setting ["UDP","TCP"] has a value of 2. A source setting
* ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"] has a value of 3.
*
*
*
*
* A rule with no criteria specified in any of its match settings has a capacity requirement of 1. A rule
* with protocol setting ["UDP","TCP"], source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"], and a
* single specification or no specification for each of the other match settings has a capacity requirement
* of 6.
*
*
* Capacity for a stateful rule group
*
*
* For a stateful rule group, the minimum capacity required is the number of individual rules that you
* expect to have in the rule group.
*/
public Integer getCapacity() {
return this.capacity;
}
/**
*
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation. When you
* update a rule group, you are limited to this capacity. When you reference a rule group from a firewall policy,
* Network Firewall reserves this capacity for the rule group.
*
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by calling
* CreateRuleGroup with DryRun
set to TRUE
.
*
*
*
* You can't change or exceed this capacity when you update the rule group, so leave room for your rule group to
* grow.
*
*
*
* Capacity for a stateless rule group
*
*
* For a stateless rule group, the capacity required is the sum of the capacity requirements of the individual rules
* that you expect to have in the rule group.
*
*
* To calculate the capacity requirement of a single rule, multiply the capacity requirement values of each of the
* rule's match settings:
*
*
* -
*
* A match setting with no criteria specified has a value of 1.
*
*
* -
*
* A match setting with Any
specified has a value of 1.
*
*
* -
*
* All other match settings have a value equal to the number of elements provided in the setting. For example, a
* protocol setting ["UDP"] and a source setting ["10.0.0.0/24"] each have a value of 1. A protocol setting
* ["UDP","TCP"] has a value of 2. A source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"] has a value of 3.
*
*
*
*
* A rule with no criteria specified in any of its match settings has a capacity requirement of 1. A rule with
* protocol setting ["UDP","TCP"], source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"], and a single
* specification or no specification for each of the other match settings has a capacity requirement of 6.
*
*
* Capacity for a stateful rule group
*
*
* For a stateful rule group, the minimum capacity required is the number of individual rules that you expect to
* have in the rule group.
*
*
* @param capacity
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation.
* When you update a rule group, you are limited to this capacity. When you reference a rule group from a
* firewall policy, Network Firewall reserves this capacity for the rule group.
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by
* calling CreateRuleGroup with DryRun
set to TRUE
.
*
*
*
* You can't change or exceed this capacity when you update the rule group, so leave room for your rule group
* to grow.
*
*
*
* Capacity for a stateless rule group
*
*
* For a stateless rule group, the capacity required is the sum of the capacity requirements of the
* individual rules that you expect to have in the rule group.
*
*
* To calculate the capacity requirement of a single rule, multiply the capacity requirement values of each
* of the rule's match settings:
*
*
* -
*
* A match setting with no criteria specified has a value of 1.
*
*
* -
*
* A match setting with Any
specified has a value of 1.
*
*
* -
*
* All other match settings have a value equal to the number of elements provided in the setting. For
* example, a protocol setting ["UDP"] and a source setting ["10.0.0.0/24"] each have a value of 1. A
* protocol setting ["UDP","TCP"] has a value of 2. A source setting
* ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"] has a value of 3.
*
*
*
*
* A rule with no criteria specified in any of its match settings has a capacity requirement of 1. A rule
* with protocol setting ["UDP","TCP"], source setting ["10.0.0.0/24","10.0.0.1/24","10.0.0.2/24"], and a
* single specification or no specification for each of the other match settings has a capacity requirement
* of 6.
*
*
* Capacity for a stateful rule group
*
*
* For a stateful rule group, the minimum capacity required is the number of individual rules that you expect
* to have in the rule group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withCapacity(Integer capacity) {
setCapacity(capacity);
return this;
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* @return The key:value pairs to associate with the resource.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* @param tags
* The key:value pairs to associate with the resource.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The key:value pairs to associate with the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* @param tags
* The key:value pairs to associate with the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Indicates whether you want Network Firewall to just check the validity of the request, rather than run the
* request.
*
*
* If set to TRUE
, Network Firewall checks whether the request can run successfully, but doesn't
* actually make the requested changes. The call returns the value that the request would return if you ran it with
* dry run set to FALSE
, but doesn't make additions or changes to your resources. This option allows
* you to make sure that you have the required permissions to run the request and that your request parameters are
* valid.
*
*
* If set to FALSE
, Network Firewall makes the requested changes to your resources.
*
*
* @param dryRun
* Indicates whether you want Network Firewall to just check the validity of the request, rather than run the
* request.
*
* If set to TRUE
, Network Firewall checks whether the request can run successfully, but doesn't
* actually make the requested changes. The call returns the value that the request would return if you ran
* it with dry run set to FALSE
, but doesn't make additions or changes to your resources. This
* option allows you to make sure that you have the required permissions to run the request and that your
* request parameters are valid.
*
*
* If set to FALSE
, Network Firewall makes the requested changes to your resources.
*/
public void setDryRun(Boolean dryRun) {
this.dryRun = dryRun;
}
/**
*
* Indicates whether you want Network Firewall to just check the validity of the request, rather than run the
* request.
*
*
* If set to TRUE
, Network Firewall checks whether the request can run successfully, but doesn't
* actually make the requested changes. The call returns the value that the request would return if you ran it with
* dry run set to FALSE
, but doesn't make additions or changes to your resources. This option allows
* you to make sure that you have the required permissions to run the request and that your request parameters are
* valid.
*
*
* If set to FALSE
, Network Firewall makes the requested changes to your resources.
*
*
* @return Indicates whether you want Network Firewall to just check the validity of the request, rather than run
* the request.
*
* If set to TRUE
, Network Firewall checks whether the request can run successfully, but
* doesn't actually make the requested changes. The call returns the value that the request would return if
* you ran it with dry run set to FALSE
, but doesn't make additions or changes to your
* resources. This option allows you to make sure that you have the required permissions to run the request
* and that your request parameters are valid.
*
*
* If set to FALSE
, Network Firewall makes the requested changes to your resources.
*/
public Boolean getDryRun() {
return this.dryRun;
}
/**
*
* Indicates whether you want Network Firewall to just check the validity of the request, rather than run the
* request.
*
*
* If set to TRUE
, Network Firewall checks whether the request can run successfully, but doesn't
* actually make the requested changes. The call returns the value that the request would return if you ran it with
* dry run set to FALSE
, but doesn't make additions or changes to your resources. This option allows
* you to make sure that you have the required permissions to run the request and that your request parameters are
* valid.
*
*
* If set to FALSE
, Network Firewall makes the requested changes to your resources.
*
*
* @param dryRun
* Indicates whether you want Network Firewall to just check the validity of the request, rather than run the
* request.
*
* If set to TRUE
, Network Firewall checks whether the request can run successfully, but doesn't
* actually make the requested changes. The call returns the value that the request would return if you ran
* it with dry run set to FALSE
, but doesn't make additions or changes to your resources. This
* option allows you to make sure that you have the required permissions to run the request and that your
* request parameters are valid.
*
*
* If set to FALSE
, Network Firewall makes the requested changes to your resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withDryRun(Boolean dryRun) {
setDryRun(dryRun);
return this;
}
/**
*
* Indicates whether you want Network Firewall to just check the validity of the request, rather than run the
* request.
*
*
* If set to TRUE
, Network Firewall checks whether the request can run successfully, but doesn't
* actually make the requested changes. The call returns the value that the request would return if you ran it with
* dry run set to FALSE
, but doesn't make additions or changes to your resources. This option allows
* you to make sure that you have the required permissions to run the request and that your request parameters are
* valid.
*
*
* If set to FALSE
, Network Firewall makes the requested changes to your resources.
*
*
* @return Indicates whether you want Network Firewall to just check the validity of the request, rather than run
* the request.
*
* If set to TRUE
, Network Firewall checks whether the request can run successfully, but
* doesn't actually make the requested changes. The call returns the value that the request would return if
* you ran it with dry run set to FALSE
, but doesn't make additions or changes to your
* resources. This option allows you to make sure that you have the required permissions to run the request
* and that your request parameters are valid.
*
*
* If set to FALSE
, Network Firewall makes the requested changes to your resources.
*/
public Boolean isDryRun() {
return this.dryRun;
}
/**
*
* A complex type that contains settings for encryption of your rule group resources.
*
*
* @param encryptionConfiguration
* A complex type that contains settings for encryption of your rule group resources.
*/
public void setEncryptionConfiguration(EncryptionConfiguration encryptionConfiguration) {
this.encryptionConfiguration = encryptionConfiguration;
}
/**
*
* A complex type that contains settings for encryption of your rule group resources.
*
*
* @return A complex type that contains settings for encryption of your rule group resources.
*/
public EncryptionConfiguration getEncryptionConfiguration() {
return this.encryptionConfiguration;
}
/**
*
* A complex type that contains settings for encryption of your rule group resources.
*
*
* @param encryptionConfiguration
* A complex type that contains settings for encryption of your rule group resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withEncryptionConfiguration(EncryptionConfiguration encryptionConfiguration) {
setEncryptionConfiguration(encryptionConfiguration);
return this;
}
/**
*
* A complex type that contains metadata about the rule group that your own rule group is copied from. You can use
* the metadata to keep track of updates made to the originating rule group.
*
*
* @param sourceMetadata
* A complex type that contains metadata about the rule group that your own rule group is copied from. You
* can use the metadata to keep track of updates made to the originating rule group.
*/
public void setSourceMetadata(SourceMetadata sourceMetadata) {
this.sourceMetadata = sourceMetadata;
}
/**
*
* A complex type that contains metadata about the rule group that your own rule group is copied from. You can use
* the metadata to keep track of updates made to the originating rule group.
*
*
* @return A complex type that contains metadata about the rule group that your own rule group is copied from. You
* can use the metadata to keep track of updates made to the originating rule group.
*/
public SourceMetadata getSourceMetadata() {
return this.sourceMetadata;
}
/**
*
* A complex type that contains metadata about the rule group that your own rule group is copied from. You can use
* the metadata to keep track of updates made to the originating rule group.
*
*
* @param sourceMetadata
* A complex type that contains metadata about the rule group that your own rule group is copied from. You
* can use the metadata to keep track of updates made to the originating rule group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withSourceMetadata(SourceMetadata sourceMetadata) {
setSourceMetadata(sourceMetadata);
return this;
}
/**
*
* Indicates whether you want Network Firewall to analyze the stateless rules in the rule group for rule behavior
* such as asymmetric routing. If set to TRUE
, Network Firewall runs the analysis and then creates the
* rule group for you. To run the stateless rule group analyzer without creating the rule group, set
* DryRun
to TRUE
.
*
*
* @param analyzeRuleGroup
* Indicates whether you want Network Firewall to analyze the stateless rules in the rule group for rule
* behavior such as asymmetric routing. If set to TRUE
, Network Firewall runs the analysis and
* then creates the rule group for you. To run the stateless rule group analyzer without creating the rule
* group, set DryRun
to TRUE
.
*/
public void setAnalyzeRuleGroup(Boolean analyzeRuleGroup) {
this.analyzeRuleGroup = analyzeRuleGroup;
}
/**
*
* Indicates whether you want Network Firewall to analyze the stateless rules in the rule group for rule behavior
* such as asymmetric routing. If set to TRUE
, Network Firewall runs the analysis and then creates the
* rule group for you. To run the stateless rule group analyzer without creating the rule group, set
* DryRun
to TRUE
.
*
*
* @return Indicates whether you want Network Firewall to analyze the stateless rules in the rule group for rule
* behavior such as asymmetric routing. If set to TRUE
, Network Firewall runs the analysis and
* then creates the rule group for you. To run the stateless rule group analyzer without creating the rule
* group, set DryRun
to TRUE
.
*/
public Boolean getAnalyzeRuleGroup() {
return this.analyzeRuleGroup;
}
/**
*
* Indicates whether you want Network Firewall to analyze the stateless rules in the rule group for rule behavior
* such as asymmetric routing. If set to TRUE
, Network Firewall runs the analysis and then creates the
* rule group for you. To run the stateless rule group analyzer without creating the rule group, set
* DryRun
to TRUE
.
*
*
* @param analyzeRuleGroup
* Indicates whether you want Network Firewall to analyze the stateless rules in the rule group for rule
* behavior such as asymmetric routing. If set to TRUE
, Network Firewall runs the analysis and
* then creates the rule group for you. To run the stateless rule group analyzer without creating the rule
* group, set DryRun
to TRUE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withAnalyzeRuleGroup(Boolean analyzeRuleGroup) {
setAnalyzeRuleGroup(analyzeRuleGroup);
return this;
}
/**
*
* Indicates whether you want Network Firewall to analyze the stateless rules in the rule group for rule behavior
* such as asymmetric routing. If set to TRUE
, Network Firewall runs the analysis and then creates the
* rule group for you. To run the stateless rule group analyzer without creating the rule group, set
* DryRun
to TRUE
.
*
*
* @return Indicates whether you want Network Firewall to analyze the stateless rules in the rule group for rule
* behavior such as asymmetric routing. If set to TRUE
, Network Firewall runs the analysis and
* then creates the rule group for you. To run the stateless rule group analyzer without creating the rule
* group, set DryRun
to TRUE
.
*/
public Boolean isAnalyzeRuleGroup() {
return this.analyzeRuleGroup;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRuleGroupName() != null)
sb.append("RuleGroupName: ").append(getRuleGroupName()).append(",");
if (getRuleGroup() != null)
sb.append("RuleGroup: ").append(getRuleGroup()).append(",");
if (getRules() != null)
sb.append("Rules: ").append(getRules()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getCapacity() != null)
sb.append("Capacity: ").append(getCapacity()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getDryRun() != null)
sb.append("DryRun: ").append(getDryRun()).append(",");
if (getEncryptionConfiguration() != null)
sb.append("EncryptionConfiguration: ").append(getEncryptionConfiguration()).append(",");
if (getSourceMetadata() != null)
sb.append("SourceMetadata: ").append(getSourceMetadata()).append(",");
if (getAnalyzeRuleGroup() != null)
sb.append("AnalyzeRuleGroup: ").append(getAnalyzeRuleGroup());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateRuleGroupRequest == false)
return false;
CreateRuleGroupRequest other = (CreateRuleGroupRequest) obj;
if (other.getRuleGroupName() == null ^ this.getRuleGroupName() == null)
return false;
if (other.getRuleGroupName() != null && other.getRuleGroupName().equals(this.getRuleGroupName()) == false)
return false;
if (other.getRuleGroup() == null ^ this.getRuleGroup() == null)
return false;
if (other.getRuleGroup() != null && other.getRuleGroup().equals(this.getRuleGroup()) == false)
return false;
if (other.getRules() == null ^ this.getRules() == null)
return false;
if (other.getRules() != null && other.getRules().equals(this.getRules()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getCapacity() == null ^ this.getCapacity() == null)
return false;
if (other.getCapacity() != null && other.getCapacity().equals(this.getCapacity()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getDryRun() == null ^ this.getDryRun() == null)
return false;
if (other.getDryRun() != null && other.getDryRun().equals(this.getDryRun()) == false)
return false;
if (other.getEncryptionConfiguration() == null ^ this.getEncryptionConfiguration() == null)
return false;
if (other.getEncryptionConfiguration() != null && other.getEncryptionConfiguration().equals(this.getEncryptionConfiguration()) == false)
return false;
if (other.getSourceMetadata() == null ^ this.getSourceMetadata() == null)
return false;
if (other.getSourceMetadata() != null && other.getSourceMetadata().equals(this.getSourceMetadata()) == false)
return false;
if (other.getAnalyzeRuleGroup() == null ^ this.getAnalyzeRuleGroup() == null)
return false;
if (other.getAnalyzeRuleGroup() != null && other.getAnalyzeRuleGroup().equals(this.getAnalyzeRuleGroup()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRuleGroupName() == null) ? 0 : getRuleGroupName().hashCode());
hashCode = prime * hashCode + ((getRuleGroup() == null) ? 0 : getRuleGroup().hashCode());
hashCode = prime * hashCode + ((getRules() == null) ? 0 : getRules().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getCapacity() == null) ? 0 : getCapacity().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getDryRun() == null) ? 0 : getDryRun().hashCode());
hashCode = prime * hashCode + ((getEncryptionConfiguration() == null) ? 0 : getEncryptionConfiguration().hashCode());
hashCode = prime * hashCode + ((getSourceMetadata() == null) ? 0 : getSourceMetadata().hashCode());
hashCode = prime * hashCode + ((getAnalyzeRuleGroup() == null) ? 0 : getAnalyzeRuleGroup().hashCode());
return hashCode;
}
@Override
public CreateRuleGroupRequest clone() {
return (CreateRuleGroupRequest) super.clone();
}
}