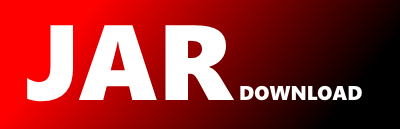
com.amazonaws.services.networkfirewall.model.TLSInspectionConfigurationResponse Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkfirewall Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkfirewall.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The high-level properties of a TLS inspection configuration. This, along with the
* TLSInspectionConfiguration
, define the TLS inspection configuration. You can retrieve all objects for a
* TLS inspection configuration by calling DescribeTLSInspectionConfiguration
.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TLSInspectionConfigurationResponse implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the TLS inspection configuration.
*
*/
private String tLSInspectionConfigurationArn;
/**
*
* The descriptive name of the TLS inspection configuration. You can't change the name of a TLS inspection
* configuration after you create it.
*
*/
private String tLSInspectionConfigurationName;
/**
*
* A unique identifier for the TLS inspection configuration. This ID is returned in the responses to create and list
* commands. You provide it to operations such as update and delete.
*
*/
private String tLSInspectionConfigurationId;
/**
*
* Detailed information about the current status of a TLSInspectionConfiguration. You can retrieve this for a
* TLS inspection configuration by calling DescribeTLSInspectionConfiguration and providing the TLS
* inspection configuration name and ARN.
*
*/
private String tLSInspectionConfigurationStatus;
/**
*
* A description of the TLS inspection configuration.
*
*/
private String description;
/**
*
* The key:value pairs to associate with the resource.
*
*/
private java.util.List tags;
/**
*
* The last time that the TLS inspection configuration was changed.
*
*/
private java.util.Date lastModifiedTime;
/**
*
* The number of firewall policies that use this TLS inspection configuration.
*
*/
private Integer numberOfAssociations;
/**
*
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your TLS
* inspection configuration.
*
*/
private EncryptionConfiguration encryptionConfiguration;
/**
*
* A list of the certificates associated with the TLS inspection configuration.
*
*/
private java.util.List certificates;
private TlsCertificateData certificateAuthority;
/**
*
* The Amazon Resource Name (ARN) of the TLS inspection configuration.
*
*
* @param tLSInspectionConfigurationArn
* The Amazon Resource Name (ARN) of the TLS inspection configuration.
*/
public void setTLSInspectionConfigurationArn(String tLSInspectionConfigurationArn) {
this.tLSInspectionConfigurationArn = tLSInspectionConfigurationArn;
}
/**
*
* The Amazon Resource Name (ARN) of the TLS inspection configuration.
*
*
* @return The Amazon Resource Name (ARN) of the TLS inspection configuration.
*/
public String getTLSInspectionConfigurationArn() {
return this.tLSInspectionConfigurationArn;
}
/**
*
* The Amazon Resource Name (ARN) of the TLS inspection configuration.
*
*
* @param tLSInspectionConfigurationArn
* The Amazon Resource Name (ARN) of the TLS inspection configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withTLSInspectionConfigurationArn(String tLSInspectionConfigurationArn) {
setTLSInspectionConfigurationArn(tLSInspectionConfigurationArn);
return this;
}
/**
*
* The descriptive name of the TLS inspection configuration. You can't change the name of a TLS inspection
* configuration after you create it.
*
*
* @param tLSInspectionConfigurationName
* The descriptive name of the TLS inspection configuration. You can't change the name of a TLS inspection
* configuration after you create it.
*/
public void setTLSInspectionConfigurationName(String tLSInspectionConfigurationName) {
this.tLSInspectionConfigurationName = tLSInspectionConfigurationName;
}
/**
*
* The descriptive name of the TLS inspection configuration. You can't change the name of a TLS inspection
* configuration after you create it.
*
*
* @return The descriptive name of the TLS inspection configuration. You can't change the name of a TLS inspection
* configuration after you create it.
*/
public String getTLSInspectionConfigurationName() {
return this.tLSInspectionConfigurationName;
}
/**
*
* The descriptive name of the TLS inspection configuration. You can't change the name of a TLS inspection
* configuration after you create it.
*
*
* @param tLSInspectionConfigurationName
* The descriptive name of the TLS inspection configuration. You can't change the name of a TLS inspection
* configuration after you create it.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withTLSInspectionConfigurationName(String tLSInspectionConfigurationName) {
setTLSInspectionConfigurationName(tLSInspectionConfigurationName);
return this;
}
/**
*
* A unique identifier for the TLS inspection configuration. This ID is returned in the responses to create and list
* commands. You provide it to operations such as update and delete.
*
*
* @param tLSInspectionConfigurationId
* A unique identifier for the TLS inspection configuration. This ID is returned in the responses to create
* and list commands. You provide it to operations such as update and delete.
*/
public void setTLSInspectionConfigurationId(String tLSInspectionConfigurationId) {
this.tLSInspectionConfigurationId = tLSInspectionConfigurationId;
}
/**
*
* A unique identifier for the TLS inspection configuration. This ID is returned in the responses to create and list
* commands. You provide it to operations such as update and delete.
*
*
* @return A unique identifier for the TLS inspection configuration. This ID is returned in the responses to create
* and list commands. You provide it to operations such as update and delete.
*/
public String getTLSInspectionConfigurationId() {
return this.tLSInspectionConfigurationId;
}
/**
*
* A unique identifier for the TLS inspection configuration. This ID is returned in the responses to create and list
* commands. You provide it to operations such as update and delete.
*
*
* @param tLSInspectionConfigurationId
* A unique identifier for the TLS inspection configuration. This ID is returned in the responses to create
* and list commands. You provide it to operations such as update and delete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withTLSInspectionConfigurationId(String tLSInspectionConfigurationId) {
setTLSInspectionConfigurationId(tLSInspectionConfigurationId);
return this;
}
/**
*
* Detailed information about the current status of a TLSInspectionConfiguration. You can retrieve this for a
* TLS inspection configuration by calling DescribeTLSInspectionConfiguration and providing the TLS
* inspection configuration name and ARN.
*
*
* @param tLSInspectionConfigurationStatus
* Detailed information about the current status of a TLSInspectionConfiguration. You can retrieve
* this for a TLS inspection configuration by calling DescribeTLSInspectionConfiguration and providing
* the TLS inspection configuration name and ARN.
* @see ResourceStatus
*/
public void setTLSInspectionConfigurationStatus(String tLSInspectionConfigurationStatus) {
this.tLSInspectionConfigurationStatus = tLSInspectionConfigurationStatus;
}
/**
*
* Detailed information about the current status of a TLSInspectionConfiguration. You can retrieve this for a
* TLS inspection configuration by calling DescribeTLSInspectionConfiguration and providing the TLS
* inspection configuration name and ARN.
*
*
* @return Detailed information about the current status of a TLSInspectionConfiguration. You can retrieve
* this for a TLS inspection configuration by calling DescribeTLSInspectionConfiguration and
* providing the TLS inspection configuration name and ARN.
* @see ResourceStatus
*/
public String getTLSInspectionConfigurationStatus() {
return this.tLSInspectionConfigurationStatus;
}
/**
*
* Detailed information about the current status of a TLSInspectionConfiguration. You can retrieve this for a
* TLS inspection configuration by calling DescribeTLSInspectionConfiguration and providing the TLS
* inspection configuration name and ARN.
*
*
* @param tLSInspectionConfigurationStatus
* Detailed information about the current status of a TLSInspectionConfiguration. You can retrieve
* this for a TLS inspection configuration by calling DescribeTLSInspectionConfiguration and providing
* the TLS inspection configuration name and ARN.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceStatus
*/
public TLSInspectionConfigurationResponse withTLSInspectionConfigurationStatus(String tLSInspectionConfigurationStatus) {
setTLSInspectionConfigurationStatus(tLSInspectionConfigurationStatus);
return this;
}
/**
*
* Detailed information about the current status of a TLSInspectionConfiguration. You can retrieve this for a
* TLS inspection configuration by calling DescribeTLSInspectionConfiguration and providing the TLS
* inspection configuration name and ARN.
*
*
* @param tLSInspectionConfigurationStatus
* Detailed information about the current status of a TLSInspectionConfiguration. You can retrieve
* this for a TLS inspection configuration by calling DescribeTLSInspectionConfiguration and providing
* the TLS inspection configuration name and ARN.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceStatus
*/
public TLSInspectionConfigurationResponse withTLSInspectionConfigurationStatus(ResourceStatus tLSInspectionConfigurationStatus) {
this.tLSInspectionConfigurationStatus = tLSInspectionConfigurationStatus.toString();
return this;
}
/**
*
* A description of the TLS inspection configuration.
*
*
* @param description
* A description of the TLS inspection configuration.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the TLS inspection configuration.
*
*
* @return A description of the TLS inspection configuration.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the TLS inspection configuration.
*
*
* @param description
* A description of the TLS inspection configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* @return The key:value pairs to associate with the resource.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* @param tags
* The key:value pairs to associate with the resource.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The key:value pairs to associate with the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* @param tags
* The key:value pairs to associate with the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The last time that the TLS inspection configuration was changed.
*
*
* @param lastModifiedTime
* The last time that the TLS inspection configuration was changed.
*/
public void setLastModifiedTime(java.util.Date lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
/**
*
* The last time that the TLS inspection configuration was changed.
*
*
* @return The last time that the TLS inspection configuration was changed.
*/
public java.util.Date getLastModifiedTime() {
return this.lastModifiedTime;
}
/**
*
* The last time that the TLS inspection configuration was changed.
*
*
* @param lastModifiedTime
* The last time that the TLS inspection configuration was changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withLastModifiedTime(java.util.Date lastModifiedTime) {
setLastModifiedTime(lastModifiedTime);
return this;
}
/**
*
* The number of firewall policies that use this TLS inspection configuration.
*
*
* @param numberOfAssociations
* The number of firewall policies that use this TLS inspection configuration.
*/
public void setNumberOfAssociations(Integer numberOfAssociations) {
this.numberOfAssociations = numberOfAssociations;
}
/**
*
* The number of firewall policies that use this TLS inspection configuration.
*
*
* @return The number of firewall policies that use this TLS inspection configuration.
*/
public Integer getNumberOfAssociations() {
return this.numberOfAssociations;
}
/**
*
* The number of firewall policies that use this TLS inspection configuration.
*
*
* @param numberOfAssociations
* The number of firewall policies that use this TLS inspection configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withNumberOfAssociations(Integer numberOfAssociations) {
setNumberOfAssociations(numberOfAssociations);
return this;
}
/**
*
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your TLS
* inspection configuration.
*
*
* @param encryptionConfiguration
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your TLS
* inspection configuration.
*/
public void setEncryptionConfiguration(EncryptionConfiguration encryptionConfiguration) {
this.encryptionConfiguration = encryptionConfiguration;
}
/**
*
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your TLS
* inspection configuration.
*
*
* @return A complex type that contains the Amazon Web Services KMS encryption configuration settings for your TLS
* inspection configuration.
*/
public EncryptionConfiguration getEncryptionConfiguration() {
return this.encryptionConfiguration;
}
/**
*
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your TLS
* inspection configuration.
*
*
* @param encryptionConfiguration
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your TLS
* inspection configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withEncryptionConfiguration(EncryptionConfiguration encryptionConfiguration) {
setEncryptionConfiguration(encryptionConfiguration);
return this;
}
/**
*
* A list of the certificates associated with the TLS inspection configuration.
*
*
* @return A list of the certificates associated with the TLS inspection configuration.
*/
public java.util.List getCertificates() {
return certificates;
}
/**
*
* A list of the certificates associated with the TLS inspection configuration.
*
*
* @param certificates
* A list of the certificates associated with the TLS inspection configuration.
*/
public void setCertificates(java.util.Collection certificates) {
if (certificates == null) {
this.certificates = null;
return;
}
this.certificates = new java.util.ArrayList(certificates);
}
/**
*
* A list of the certificates associated with the TLS inspection configuration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCertificates(java.util.Collection)} or {@link #withCertificates(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param certificates
* A list of the certificates associated with the TLS inspection configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withCertificates(TlsCertificateData... certificates) {
if (this.certificates == null) {
setCertificates(new java.util.ArrayList(certificates.length));
}
for (TlsCertificateData ele : certificates) {
this.certificates.add(ele);
}
return this;
}
/**
*
* A list of the certificates associated with the TLS inspection configuration.
*
*
* @param certificates
* A list of the certificates associated with the TLS inspection configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withCertificates(java.util.Collection certificates) {
setCertificates(certificates);
return this;
}
/**
* @param certificateAuthority
*/
public void setCertificateAuthority(TlsCertificateData certificateAuthority) {
this.certificateAuthority = certificateAuthority;
}
/**
* @return
*/
public TlsCertificateData getCertificateAuthority() {
return this.certificateAuthority;
}
/**
* @param certificateAuthority
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TLSInspectionConfigurationResponse withCertificateAuthority(TlsCertificateData certificateAuthority) {
setCertificateAuthority(certificateAuthority);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTLSInspectionConfigurationArn() != null)
sb.append("TLSInspectionConfigurationArn: ").append(getTLSInspectionConfigurationArn()).append(",");
if (getTLSInspectionConfigurationName() != null)
sb.append("TLSInspectionConfigurationName: ").append(getTLSInspectionConfigurationName()).append(",");
if (getTLSInspectionConfigurationId() != null)
sb.append("TLSInspectionConfigurationId: ").append(getTLSInspectionConfigurationId()).append(",");
if (getTLSInspectionConfigurationStatus() != null)
sb.append("TLSInspectionConfigurationStatus: ").append(getTLSInspectionConfigurationStatus()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getLastModifiedTime() != null)
sb.append("LastModifiedTime: ").append(getLastModifiedTime()).append(",");
if (getNumberOfAssociations() != null)
sb.append("NumberOfAssociations: ").append(getNumberOfAssociations()).append(",");
if (getEncryptionConfiguration() != null)
sb.append("EncryptionConfiguration: ").append(getEncryptionConfiguration()).append(",");
if (getCertificates() != null)
sb.append("Certificates: ").append(getCertificates()).append(",");
if (getCertificateAuthority() != null)
sb.append("CertificateAuthority: ").append(getCertificateAuthority());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TLSInspectionConfigurationResponse == false)
return false;
TLSInspectionConfigurationResponse other = (TLSInspectionConfigurationResponse) obj;
if (other.getTLSInspectionConfigurationArn() == null ^ this.getTLSInspectionConfigurationArn() == null)
return false;
if (other.getTLSInspectionConfigurationArn() != null
&& other.getTLSInspectionConfigurationArn().equals(this.getTLSInspectionConfigurationArn()) == false)
return false;
if (other.getTLSInspectionConfigurationName() == null ^ this.getTLSInspectionConfigurationName() == null)
return false;
if (other.getTLSInspectionConfigurationName() != null
&& other.getTLSInspectionConfigurationName().equals(this.getTLSInspectionConfigurationName()) == false)
return false;
if (other.getTLSInspectionConfigurationId() == null ^ this.getTLSInspectionConfigurationId() == null)
return false;
if (other.getTLSInspectionConfigurationId() != null && other.getTLSInspectionConfigurationId().equals(this.getTLSInspectionConfigurationId()) == false)
return false;
if (other.getTLSInspectionConfigurationStatus() == null ^ this.getTLSInspectionConfigurationStatus() == null)
return false;
if (other.getTLSInspectionConfigurationStatus() != null
&& other.getTLSInspectionConfigurationStatus().equals(this.getTLSInspectionConfigurationStatus()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getLastModifiedTime() == null ^ this.getLastModifiedTime() == null)
return false;
if (other.getLastModifiedTime() != null && other.getLastModifiedTime().equals(this.getLastModifiedTime()) == false)
return false;
if (other.getNumberOfAssociations() == null ^ this.getNumberOfAssociations() == null)
return false;
if (other.getNumberOfAssociations() != null && other.getNumberOfAssociations().equals(this.getNumberOfAssociations()) == false)
return false;
if (other.getEncryptionConfiguration() == null ^ this.getEncryptionConfiguration() == null)
return false;
if (other.getEncryptionConfiguration() != null && other.getEncryptionConfiguration().equals(this.getEncryptionConfiguration()) == false)
return false;
if (other.getCertificates() == null ^ this.getCertificates() == null)
return false;
if (other.getCertificates() != null && other.getCertificates().equals(this.getCertificates()) == false)
return false;
if (other.getCertificateAuthority() == null ^ this.getCertificateAuthority() == null)
return false;
if (other.getCertificateAuthority() != null && other.getCertificateAuthority().equals(this.getCertificateAuthority()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTLSInspectionConfigurationArn() == null) ? 0 : getTLSInspectionConfigurationArn().hashCode());
hashCode = prime * hashCode + ((getTLSInspectionConfigurationName() == null) ? 0 : getTLSInspectionConfigurationName().hashCode());
hashCode = prime * hashCode + ((getTLSInspectionConfigurationId() == null) ? 0 : getTLSInspectionConfigurationId().hashCode());
hashCode = prime * hashCode + ((getTLSInspectionConfigurationStatus() == null) ? 0 : getTLSInspectionConfigurationStatus().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTime() == null) ? 0 : getLastModifiedTime().hashCode());
hashCode = prime * hashCode + ((getNumberOfAssociations() == null) ? 0 : getNumberOfAssociations().hashCode());
hashCode = prime * hashCode + ((getEncryptionConfiguration() == null) ? 0 : getEncryptionConfiguration().hashCode());
hashCode = prime * hashCode + ((getCertificates() == null) ? 0 : getCertificates().hashCode());
hashCode = prime * hashCode + ((getCertificateAuthority() == null) ? 0 : getCertificateAuthority().hashCode());
return hashCode;
}
@Override
public TLSInspectionConfigurationResponse clone() {
try {
return (TLSInspectionConfigurationResponse) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.networkfirewall.model.transform.TLSInspectionConfigurationResponseMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}