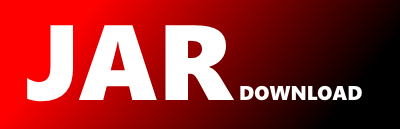
com.amazonaws.services.networkfirewall.model.MatchAttributes Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkfirewall Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkfirewall.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Criteria for Network Firewall to use to inspect an individual packet in stateless rule inspection. Each match
* attributes set can include one or more items such as IP address, CIDR range, port number, protocol, and TCP flags.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MatchAttributes implements Serializable, Cloneable, StructuredPojo {
/**
*
* The source IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches with
* any source address.
*
*/
private java.util.List sources;
/**
*
* The destination IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches
* with any destination address.
*
*/
private java.util.List destinations;
/**
*
* The source ports to inspect for. If not specified, this matches with any source port. This setting is only used
* for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*/
private java.util.List sourcePorts;
/**
*
* The destination ports to inspect for. If not specified, this matches with any destination port. This setting is
* only used for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*/
private java.util.List destinationPorts;
/**
*
* The protocols to inspect for, specified using each protocol's assigned internet protocol number (IANA). If not
* specified, this matches with any protocol.
*
*/
private java.util.List protocols;
/**
*
* The TCP flags and masks to inspect for. If not specified, this matches with any settings. This setting is only
* used for protocol 6 (TCP).
*
*/
private java.util.List tCPFlags;
/**
*
* The source IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches with
* any source address.
*
*
* @return The source IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this
* matches with any source address.
*/
public java.util.List getSources() {
return sources;
}
/**
*
* The source IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches with
* any source address.
*
*
* @param sources
* The source IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this
* matches with any source address.
*/
public void setSources(java.util.Collection sources) {
if (sources == null) {
this.sources = null;
return;
}
this.sources = new java.util.ArrayList(sources);
}
/**
*
* The source IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches with
* any source address.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSources(java.util.Collection)} or {@link #withSources(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param sources
* The source IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this
* matches with any source address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withSources(Address... sources) {
if (this.sources == null) {
setSources(new java.util.ArrayList(sources.length));
}
for (Address ele : sources) {
this.sources.add(ele);
}
return this;
}
/**
*
* The source IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches with
* any source address.
*
*
* @param sources
* The source IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this
* matches with any source address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withSources(java.util.Collection sources) {
setSources(sources);
return this;
}
/**
*
* The destination IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches
* with any destination address.
*
*
* @return The destination IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this
* matches with any destination address.
*/
public java.util.List getDestinations() {
return destinations;
}
/**
*
* The destination IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches
* with any destination address.
*
*
* @param destinations
* The destination IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this
* matches with any destination address.
*/
public void setDestinations(java.util.Collection destinations) {
if (destinations == null) {
this.destinations = null;
return;
}
this.destinations = new java.util.ArrayList(destinations);
}
/**
*
* The destination IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches
* with any destination address.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDestinations(java.util.Collection)} or {@link #withDestinations(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param destinations
* The destination IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this
* matches with any destination address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withDestinations(Address... destinations) {
if (this.destinations == null) {
setDestinations(new java.util.ArrayList(destinations.length));
}
for (Address ele : destinations) {
this.destinations.add(ele);
}
return this;
}
/**
*
* The destination IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this matches
* with any destination address.
*
*
* @param destinations
* The destination IP addresses and address ranges to inspect for, in CIDR notation. If not specified, this
* matches with any destination address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withDestinations(java.util.Collection destinations) {
setDestinations(destinations);
return this;
}
/**
*
* The source ports to inspect for. If not specified, this matches with any source port. This setting is only used
* for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*
* @return The source ports to inspect for. If not specified, this matches with any source port. This setting is
* only used for protocols 6 (TCP) and 17 (UDP).
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for
* example 1990:1994
.
*/
public java.util.List getSourcePorts() {
return sourcePorts;
}
/**
*
* The source ports to inspect for. If not specified, this matches with any source port. This setting is only used
* for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*
* @param sourcePorts
* The source ports to inspect for. If not specified, this matches with any source port. This setting is only
* used for protocols 6 (TCP) and 17 (UDP).
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for
* example 1990:1994
.
*/
public void setSourcePorts(java.util.Collection sourcePorts) {
if (sourcePorts == null) {
this.sourcePorts = null;
return;
}
this.sourcePorts = new java.util.ArrayList(sourcePorts);
}
/**
*
* The source ports to inspect for. If not specified, this matches with any source port. This setting is only used
* for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSourcePorts(java.util.Collection)} or {@link #withSourcePorts(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param sourcePorts
* The source ports to inspect for. If not specified, this matches with any source port. This setting is only
* used for protocols 6 (TCP) and 17 (UDP).
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for
* example 1990:1994
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withSourcePorts(PortRange... sourcePorts) {
if (this.sourcePorts == null) {
setSourcePorts(new java.util.ArrayList(sourcePorts.length));
}
for (PortRange ele : sourcePorts) {
this.sourcePorts.add(ele);
}
return this;
}
/**
*
* The source ports to inspect for. If not specified, this matches with any source port. This setting is only used
* for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*
* @param sourcePorts
* The source ports to inspect for. If not specified, this matches with any source port. This setting is only
* used for protocols 6 (TCP) and 17 (UDP).
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for
* example 1990:1994
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withSourcePorts(java.util.Collection sourcePorts) {
setSourcePorts(sourcePorts);
return this;
}
/**
*
* The destination ports to inspect for. If not specified, this matches with any destination port. This setting is
* only used for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*
* @return The destination ports to inspect for. If not specified, this matches with any destination port. This
* setting is only used for protocols 6 (TCP) and 17 (UDP).
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for
* example 1990:1994
.
*/
public java.util.List getDestinationPorts() {
return destinationPorts;
}
/**
*
* The destination ports to inspect for. If not specified, this matches with any destination port. This setting is
* only used for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*
* @param destinationPorts
* The destination ports to inspect for. If not specified, this matches with any destination port. This
* setting is only used for protocols 6 (TCP) and 17 (UDP).
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for
* example 1990:1994
.
*/
public void setDestinationPorts(java.util.Collection destinationPorts) {
if (destinationPorts == null) {
this.destinationPorts = null;
return;
}
this.destinationPorts = new java.util.ArrayList(destinationPorts);
}
/**
*
* The destination ports to inspect for. If not specified, this matches with any destination port. This setting is
* only used for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDestinationPorts(java.util.Collection)} or {@link #withDestinationPorts(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param destinationPorts
* The destination ports to inspect for. If not specified, this matches with any destination port. This
* setting is only used for protocols 6 (TCP) and 17 (UDP).
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for
* example 1990:1994
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withDestinationPorts(PortRange... destinationPorts) {
if (this.destinationPorts == null) {
setDestinationPorts(new java.util.ArrayList(destinationPorts.length));
}
for (PortRange ele : destinationPorts) {
this.destinationPorts.add(ele);
}
return this;
}
/**
*
* The destination ports to inspect for. If not specified, this matches with any destination port. This setting is
* only used for protocols 6 (TCP) and 17 (UDP).
*
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for example
* 1990:1994
.
*
*
* @param destinationPorts
* The destination ports to inspect for. If not specified, this matches with any destination port. This
* setting is only used for protocols 6 (TCP) and 17 (UDP).
*
* You can specify individual ports, for example 1994
and you can specify port ranges, for
* example 1990:1994
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withDestinationPorts(java.util.Collection destinationPorts) {
setDestinationPorts(destinationPorts);
return this;
}
/**
*
* The protocols to inspect for, specified using each protocol's assigned internet protocol number (IANA). If not
* specified, this matches with any protocol.
*
*
* @return The protocols to inspect for, specified using each protocol's assigned internet protocol number (IANA).
* If not specified, this matches with any protocol.
*/
public java.util.List getProtocols() {
return protocols;
}
/**
*
* The protocols to inspect for, specified using each protocol's assigned internet protocol number (IANA). If not
* specified, this matches with any protocol.
*
*
* @param protocols
* The protocols to inspect for, specified using each protocol's assigned internet protocol number (IANA). If
* not specified, this matches with any protocol.
*/
public void setProtocols(java.util.Collection protocols) {
if (protocols == null) {
this.protocols = null;
return;
}
this.protocols = new java.util.ArrayList(protocols);
}
/**
*
* The protocols to inspect for, specified using each protocol's assigned internet protocol number (IANA). If not
* specified, this matches with any protocol.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setProtocols(java.util.Collection)} or {@link #withProtocols(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param protocols
* The protocols to inspect for, specified using each protocol's assigned internet protocol number (IANA). If
* not specified, this matches with any protocol.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withProtocols(Integer... protocols) {
if (this.protocols == null) {
setProtocols(new java.util.ArrayList(protocols.length));
}
for (Integer ele : protocols) {
this.protocols.add(ele);
}
return this;
}
/**
*
* The protocols to inspect for, specified using each protocol's assigned internet protocol number (IANA). If not
* specified, this matches with any protocol.
*
*
* @param protocols
* The protocols to inspect for, specified using each protocol's assigned internet protocol number (IANA). If
* not specified, this matches with any protocol.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withProtocols(java.util.Collection protocols) {
setProtocols(protocols);
return this;
}
/**
*
* The TCP flags and masks to inspect for. If not specified, this matches with any settings. This setting is only
* used for protocol 6 (TCP).
*
*
* @return The TCP flags and masks to inspect for. If not specified, this matches with any settings. This setting is
* only used for protocol 6 (TCP).
*/
public java.util.List getTCPFlags() {
return tCPFlags;
}
/**
*
* The TCP flags and masks to inspect for. If not specified, this matches with any settings. This setting is only
* used for protocol 6 (TCP).
*
*
* @param tCPFlags
* The TCP flags and masks to inspect for. If not specified, this matches with any settings. This setting is
* only used for protocol 6 (TCP).
*/
public void setTCPFlags(java.util.Collection tCPFlags) {
if (tCPFlags == null) {
this.tCPFlags = null;
return;
}
this.tCPFlags = new java.util.ArrayList(tCPFlags);
}
/**
*
* The TCP flags and masks to inspect for. If not specified, this matches with any settings. This setting is only
* used for protocol 6 (TCP).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTCPFlags(java.util.Collection)} or {@link #withTCPFlags(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param tCPFlags
* The TCP flags and masks to inspect for. If not specified, this matches with any settings. This setting is
* only used for protocol 6 (TCP).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withTCPFlags(TCPFlagField... tCPFlags) {
if (this.tCPFlags == null) {
setTCPFlags(new java.util.ArrayList(tCPFlags.length));
}
for (TCPFlagField ele : tCPFlags) {
this.tCPFlags.add(ele);
}
return this;
}
/**
*
* The TCP flags and masks to inspect for. If not specified, this matches with any settings. This setting is only
* used for protocol 6 (TCP).
*
*
* @param tCPFlags
* The TCP flags and masks to inspect for. If not specified, this matches with any settings. This setting is
* only used for protocol 6 (TCP).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchAttributes withTCPFlags(java.util.Collection tCPFlags) {
setTCPFlags(tCPFlags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSources() != null)
sb.append("Sources: ").append(getSources()).append(",");
if (getDestinations() != null)
sb.append("Destinations: ").append(getDestinations()).append(",");
if (getSourcePorts() != null)
sb.append("SourcePorts: ").append(getSourcePorts()).append(",");
if (getDestinationPorts() != null)
sb.append("DestinationPorts: ").append(getDestinationPorts()).append(",");
if (getProtocols() != null)
sb.append("Protocols: ").append(getProtocols()).append(",");
if (getTCPFlags() != null)
sb.append("TCPFlags: ").append(getTCPFlags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MatchAttributes == false)
return false;
MatchAttributes other = (MatchAttributes) obj;
if (other.getSources() == null ^ this.getSources() == null)
return false;
if (other.getSources() != null && other.getSources().equals(this.getSources()) == false)
return false;
if (other.getDestinations() == null ^ this.getDestinations() == null)
return false;
if (other.getDestinations() != null && other.getDestinations().equals(this.getDestinations()) == false)
return false;
if (other.getSourcePorts() == null ^ this.getSourcePorts() == null)
return false;
if (other.getSourcePorts() != null && other.getSourcePorts().equals(this.getSourcePorts()) == false)
return false;
if (other.getDestinationPorts() == null ^ this.getDestinationPorts() == null)
return false;
if (other.getDestinationPorts() != null && other.getDestinationPorts().equals(this.getDestinationPorts()) == false)
return false;
if (other.getProtocols() == null ^ this.getProtocols() == null)
return false;
if (other.getProtocols() != null && other.getProtocols().equals(this.getProtocols()) == false)
return false;
if (other.getTCPFlags() == null ^ this.getTCPFlags() == null)
return false;
if (other.getTCPFlags() != null && other.getTCPFlags().equals(this.getTCPFlags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSources() == null) ? 0 : getSources().hashCode());
hashCode = prime * hashCode + ((getDestinations() == null) ? 0 : getDestinations().hashCode());
hashCode = prime * hashCode + ((getSourcePorts() == null) ? 0 : getSourcePorts().hashCode());
hashCode = prime * hashCode + ((getDestinationPorts() == null) ? 0 : getDestinationPorts().hashCode());
hashCode = prime * hashCode + ((getProtocols() == null) ? 0 : getProtocols().hashCode());
hashCode = prime * hashCode + ((getTCPFlags() == null) ? 0 : getTCPFlags().hashCode());
return hashCode;
}
@Override
public MatchAttributes clone() {
try {
return (MatchAttributes) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.networkfirewall.model.transform.MatchAttributesMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}