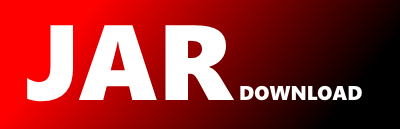
com.amazonaws.services.networkfirewall.model.StatefulRule Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkfirewall Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkfirewall.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A single Suricata rules specification, for use in a stateful rule group. Use this option to specify a simple Suricata
* rule with protocol, source and destination, ports, direction, and rule options. For information about the Suricata
* Rules
format, see Rules
* Format.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StatefulRule implements Serializable, Cloneable, StructuredPojo {
/**
*
* Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the stateful
* rule criteria. For all actions, Network Firewall performs the specified action and discontinues stateful
* inspection of the traffic flow.
*
*
* The actions for a stateful rule are defined as follows:
*
*
* -
*
* PASS - Permits the packets to go to the intended destination.
*
*
* -
*
* DROP - Blocks the packets from going to the intended destination and sends an alert log message, if alert
* logging is configured in the Firewall LoggingConfiguration.
*
*
* -
*
* ALERT - Sends an alert log message, if alert logging is configured in the Firewall
* LoggingConfiguration.
*
*
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule with
* ALERT
action, verify in the logs that the rule is filtering as you want, then change the action to
* DROP
.
*
*
* -
*
* REJECT - Drops traffic that matches the conditions of the stateful rule, and sends a TCP reset packet back
* to sender of the packet. A TCP reset packet is a packet with no payload and an RST bit contained in the TCP
* header flags. REJECT is available only for TCP traffic. This option doesn't support FTP or IMAP protocols.
*
*
*
*/
private String action;
/**
*
* The stateful inspection criteria for this rule, used to inspect traffic flows.
*
*/
private Header header;
/**
*
* Additional options for the rule. These are the Suricata RuleOptions
settings.
*
*/
private java.util.List ruleOptions;
/**
*
* Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the stateful
* rule criteria. For all actions, Network Firewall performs the specified action and discontinues stateful
* inspection of the traffic flow.
*
*
* The actions for a stateful rule are defined as follows:
*
*
* -
*
* PASS - Permits the packets to go to the intended destination.
*
*
* -
*
* DROP - Blocks the packets from going to the intended destination and sends an alert log message, if alert
* logging is configured in the Firewall LoggingConfiguration.
*
*
* -
*
* ALERT - Sends an alert log message, if alert logging is configured in the Firewall
* LoggingConfiguration.
*
*
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule with
* ALERT
action, verify in the logs that the rule is filtering as you want, then change the action to
* DROP
.
*
*
* -
*
* REJECT - Drops traffic that matches the conditions of the stateful rule, and sends a TCP reset packet back
* to sender of the packet. A TCP reset packet is a packet with no payload and an RST bit contained in the TCP
* header flags. REJECT is available only for TCP traffic. This option doesn't support FTP or IMAP protocols.
*
*
*
*
* @param action
* Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the
* stateful rule criteria. For all actions, Network Firewall performs the specified action and discontinues
* stateful inspection of the traffic flow.
*
* The actions for a stateful rule are defined as follows:
*
*
* -
*
* PASS - Permits the packets to go to the intended destination.
*
*
* -
*
* DROP - Blocks the packets from going to the intended destination and sends an alert log message, if
* alert logging is configured in the Firewall LoggingConfiguration.
*
*
* -
*
* ALERT - Sends an alert log message, if alert logging is configured in the Firewall
* LoggingConfiguration.
*
*
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule
* with ALERT
action, verify in the logs that the rule is filtering as you want, then change the
* action to DROP
.
*
*
* -
*
* REJECT - Drops traffic that matches the conditions of the stateful rule, and sends a TCP reset
* packet back to sender of the packet. A TCP reset packet is a packet with no payload and an RST bit
* contained in the TCP header flags. REJECT is available only for TCP traffic. This option doesn't support
* FTP or IMAP protocols.
*
*
* @see StatefulAction
*/
public void setAction(String action) {
this.action = action;
}
/**
*
* Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the stateful
* rule criteria. For all actions, Network Firewall performs the specified action and discontinues stateful
* inspection of the traffic flow.
*
*
* The actions for a stateful rule are defined as follows:
*
*
* -
*
* PASS - Permits the packets to go to the intended destination.
*
*
* -
*
* DROP - Blocks the packets from going to the intended destination and sends an alert log message, if alert
* logging is configured in the Firewall LoggingConfiguration.
*
*
* -
*
* ALERT - Sends an alert log message, if alert logging is configured in the Firewall
* LoggingConfiguration.
*
*
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule with
* ALERT
action, verify in the logs that the rule is filtering as you want, then change the action to
* DROP
.
*
*
* -
*
* REJECT - Drops traffic that matches the conditions of the stateful rule, and sends a TCP reset packet back
* to sender of the packet. A TCP reset packet is a packet with no payload and an RST bit contained in the TCP
* header flags. REJECT is available only for TCP traffic. This option doesn't support FTP or IMAP protocols.
*
*
*
*
* @return Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the
* stateful rule criteria. For all actions, Network Firewall performs the specified action and discontinues
* stateful inspection of the traffic flow.
*
* The actions for a stateful rule are defined as follows:
*
*
* -
*
* PASS - Permits the packets to go to the intended destination.
*
*
* -
*
* DROP - Blocks the packets from going to the intended destination and sends an alert log message,
* if alert logging is configured in the Firewall LoggingConfiguration.
*
*
* -
*
* ALERT - Sends an alert log message, if alert logging is configured in the Firewall
* LoggingConfiguration.
*
*
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule
* with ALERT
action, verify in the logs that the rule is filtering as you want, then change
* the action to DROP
.
*
*
* -
*
* REJECT - Drops traffic that matches the conditions of the stateful rule, and sends a TCP reset
* packet back to sender of the packet. A TCP reset packet is a packet with no payload and an RST bit
* contained in the TCP header flags. REJECT is available only for TCP traffic. This option doesn't support
* FTP or IMAP protocols.
*
*
* @see StatefulAction
*/
public String getAction() {
return this.action;
}
/**
*
* Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the stateful
* rule criteria. For all actions, Network Firewall performs the specified action and discontinues stateful
* inspection of the traffic flow.
*
*
* The actions for a stateful rule are defined as follows:
*
*
* -
*
* PASS - Permits the packets to go to the intended destination.
*
*
* -
*
* DROP - Blocks the packets from going to the intended destination and sends an alert log message, if alert
* logging is configured in the Firewall LoggingConfiguration.
*
*
* -
*
* ALERT - Sends an alert log message, if alert logging is configured in the Firewall
* LoggingConfiguration.
*
*
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule with
* ALERT
action, verify in the logs that the rule is filtering as you want, then change the action to
* DROP
.
*
*
* -
*
* REJECT - Drops traffic that matches the conditions of the stateful rule, and sends a TCP reset packet back
* to sender of the packet. A TCP reset packet is a packet with no payload and an RST bit contained in the TCP
* header flags. REJECT is available only for TCP traffic. This option doesn't support FTP or IMAP protocols.
*
*
*
*
* @param action
* Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the
* stateful rule criteria. For all actions, Network Firewall performs the specified action and discontinues
* stateful inspection of the traffic flow.
*
* The actions for a stateful rule are defined as follows:
*
*
* -
*
* PASS - Permits the packets to go to the intended destination.
*
*
* -
*
* DROP - Blocks the packets from going to the intended destination and sends an alert log message, if
* alert logging is configured in the Firewall LoggingConfiguration.
*
*
* -
*
* ALERT - Sends an alert log message, if alert logging is configured in the Firewall
* LoggingConfiguration.
*
*
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule
* with ALERT
action, verify in the logs that the rule is filtering as you want, then change the
* action to DROP
.
*
*
* -
*
* REJECT - Drops traffic that matches the conditions of the stateful rule, and sends a TCP reset
* packet back to sender of the packet. A TCP reset packet is a packet with no payload and an RST bit
* contained in the TCP header flags. REJECT is available only for TCP traffic. This option doesn't support
* FTP or IMAP protocols.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StatefulAction
*/
public StatefulRule withAction(String action) {
setAction(action);
return this;
}
/**
*
* Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the stateful
* rule criteria. For all actions, Network Firewall performs the specified action and discontinues stateful
* inspection of the traffic flow.
*
*
* The actions for a stateful rule are defined as follows:
*
*
* -
*
* PASS - Permits the packets to go to the intended destination.
*
*
* -
*
* DROP - Blocks the packets from going to the intended destination and sends an alert log message, if alert
* logging is configured in the Firewall LoggingConfiguration.
*
*
* -
*
* ALERT - Sends an alert log message, if alert logging is configured in the Firewall
* LoggingConfiguration.
*
*
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule with
* ALERT
action, verify in the logs that the rule is filtering as you want, then change the action to
* DROP
.
*
*
* -
*
* REJECT - Drops traffic that matches the conditions of the stateful rule, and sends a TCP reset packet back
* to sender of the packet. A TCP reset packet is a packet with no payload and an RST bit contained in the TCP
* header flags. REJECT is available only for TCP traffic. This option doesn't support FTP or IMAP protocols.
*
*
*
*
* @param action
* Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the
* stateful rule criteria. For all actions, Network Firewall performs the specified action and discontinues
* stateful inspection of the traffic flow.
*
* The actions for a stateful rule are defined as follows:
*
*
* -
*
* PASS - Permits the packets to go to the intended destination.
*
*
* -
*
* DROP - Blocks the packets from going to the intended destination and sends an alert log message, if
* alert logging is configured in the Firewall LoggingConfiguration.
*
*
* -
*
* ALERT - Sends an alert log message, if alert logging is configured in the Firewall
* LoggingConfiguration.
*
*
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule
* with ALERT
action, verify in the logs that the rule is filtering as you want, then change the
* action to DROP
.
*
*
* -
*
* REJECT - Drops traffic that matches the conditions of the stateful rule, and sends a TCP reset
* packet back to sender of the packet. A TCP reset packet is a packet with no payload and an RST bit
* contained in the TCP header flags. REJECT is available only for TCP traffic. This option doesn't support
* FTP or IMAP protocols.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StatefulAction
*/
public StatefulRule withAction(StatefulAction action) {
this.action = action.toString();
return this;
}
/**
*
* The stateful inspection criteria for this rule, used to inspect traffic flows.
*
*
* @param header
* The stateful inspection criteria for this rule, used to inspect traffic flows.
*/
public void setHeader(Header header) {
this.header = header;
}
/**
*
* The stateful inspection criteria for this rule, used to inspect traffic flows.
*
*
* @return The stateful inspection criteria for this rule, used to inspect traffic flows.
*/
public Header getHeader() {
return this.header;
}
/**
*
* The stateful inspection criteria for this rule, used to inspect traffic flows.
*
*
* @param header
* The stateful inspection criteria for this rule, used to inspect traffic flows.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatefulRule withHeader(Header header) {
setHeader(header);
return this;
}
/**
*
* Additional options for the rule. These are the Suricata RuleOptions
settings.
*
*
* @return Additional options for the rule. These are the Suricata RuleOptions
settings.
*/
public java.util.List getRuleOptions() {
return ruleOptions;
}
/**
*
* Additional options for the rule. These are the Suricata RuleOptions
settings.
*
*
* @param ruleOptions
* Additional options for the rule. These are the Suricata RuleOptions
settings.
*/
public void setRuleOptions(java.util.Collection ruleOptions) {
if (ruleOptions == null) {
this.ruleOptions = null;
return;
}
this.ruleOptions = new java.util.ArrayList(ruleOptions);
}
/**
*
* Additional options for the rule. These are the Suricata RuleOptions
settings.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRuleOptions(java.util.Collection)} or {@link #withRuleOptions(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param ruleOptions
* Additional options for the rule. These are the Suricata RuleOptions
settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatefulRule withRuleOptions(RuleOption... ruleOptions) {
if (this.ruleOptions == null) {
setRuleOptions(new java.util.ArrayList(ruleOptions.length));
}
for (RuleOption ele : ruleOptions) {
this.ruleOptions.add(ele);
}
return this;
}
/**
*
* Additional options for the rule. These are the Suricata RuleOptions
settings.
*
*
* @param ruleOptions
* Additional options for the rule. These are the Suricata RuleOptions
settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatefulRule withRuleOptions(java.util.Collection ruleOptions) {
setRuleOptions(ruleOptions);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAction() != null)
sb.append("Action: ").append(getAction()).append(",");
if (getHeader() != null)
sb.append("Header: ").append(getHeader()).append(",");
if (getRuleOptions() != null)
sb.append("RuleOptions: ").append(getRuleOptions());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StatefulRule == false)
return false;
StatefulRule other = (StatefulRule) obj;
if (other.getAction() == null ^ this.getAction() == null)
return false;
if (other.getAction() != null && other.getAction().equals(this.getAction()) == false)
return false;
if (other.getHeader() == null ^ this.getHeader() == null)
return false;
if (other.getHeader() != null && other.getHeader().equals(this.getHeader()) == false)
return false;
if (other.getRuleOptions() == null ^ this.getRuleOptions() == null)
return false;
if (other.getRuleOptions() != null && other.getRuleOptions().equals(this.getRuleOptions()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAction() == null) ? 0 : getAction().hashCode());
hashCode = prime * hashCode + ((getHeader() == null) ? 0 : getHeader().hashCode());
hashCode = prime * hashCode + ((getRuleOptions() == null) ? 0 : getRuleOptions().hashCode());
return hashCode;
}
@Override
public StatefulRule clone() {
try {
return (StatefulRule) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.networkfirewall.model.transform.StatefulRuleMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}