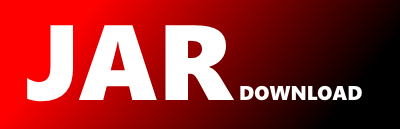
com.amazonaws.services.networkfirewall.AWSNetworkFirewallAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkfirewall Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkfirewall;
import javax.annotation.Generated;
import com.amazonaws.services.networkfirewall.model.*;
/**
* Interface for accessing Network Firewall asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.networkfirewall.AbstractAWSNetworkFirewallAsync} instead.
*
*
*
* This is the API Reference for Network Firewall. This guide is for developers who need detailed information about the
* Network Firewall API actions, data types, and errors.
*
*
* -
*
* The REST API requires you to handle connection details, such as calculating signatures, handling request retries, and
* error handling. For general information about using the Amazon Web Services REST APIs, see Amazon Web Services APIs.
*
*
* To access Network Firewall using the REST API endpoint:
* https://network-firewall.<region>.amazonaws.com
*
*
* -
*
* Alternatively, you can use one of the Amazon Web Services SDKs to access an API that's tailored to the programming
* language or platform that you're using. For more information, see Amazon
* Web Services SDKs.
*
*
* -
*
* For descriptions of Network Firewall features, including and step-by-step instructions on how to use them through the
* Network Firewall console, see the Network Firewall Developer Guide.
*
*
*
*
* Network Firewall is a stateful, managed, network firewall and intrusion detection and prevention service for Amazon
* Virtual Private Cloud (Amazon VPC). With Network Firewall, you can filter traffic at the perimeter of your VPC. This
* includes filtering traffic going to and coming from an internet gateway, NAT gateway, or over VPN or Direct Connect.
* Network Firewall uses rules that are compatible with Suricata, a free, open source network analysis and threat
* detection engine. Network Firewall supports Suricata version 6.0.9. For information about Suricata, see the Suricata website.
*
*
* You can use Network Firewall to monitor and protect your VPC traffic in a number of ways. The following are just a
* few examples:
*
*
* -
*
* Allow domains or IP addresses for known Amazon Web Services service endpoints, such as Amazon S3, and block all other
* forms of traffic.
*
*
* -
*
* Use custom lists of known bad domains to limit the types of domain names that your applications can access.
*
*
* -
*
* Perform deep packet inspection on traffic entering or leaving your VPC.
*
*
* -
*
* Use stateful protocol detection to filter protocols like HTTPS, regardless of the port used.
*
*
*
*
* To enable Network Firewall for your VPCs, you perform steps in both Amazon VPC and in Network Firewall. For
* information about using Amazon VPC, see Amazon VPC User
* Guide.
*
*
* To start using Network Firewall, do the following:
*
*
* -
*
* (Optional) If you don't already have a VPC that you want to protect, create it in Amazon VPC.
*
*
* -
*
* In Amazon VPC, in each Availability Zone where you want to have a firewall endpoint, create a subnet for the sole use
* of Network Firewall.
*
*
* -
*
* In Network Firewall, create stateless and stateful rule groups, to define the components of the network traffic
* filtering behavior that you want your firewall to have.
*
*
* -
*
* In Network Firewall, create a firewall policy that uses your rule groups and specifies additional default traffic
* filtering behavior.
*
*
* -
*
* In Network Firewall, create a firewall and specify your new firewall policy and VPC subnets. Network Firewall creates
* a firewall endpoint in each subnet that you specify, with the behavior that's defined in the firewall policy.
*
*
* -
*
* In Amazon VPC, use ingress routing enhancements to route traffic through the new firewall endpoints.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSNetworkFirewallAsync extends AWSNetworkFirewall {
/**
*
* Associates a FirewallPolicy to a Firewall.
*
*
* A firewall policy defines how to monitor and manage your VPC network traffic, using a collection of inspection
* rule groups and other settings. Each firewall requires one firewall policy association, and you can use the same
* firewall policy for multiple firewalls.
*
*
* @param associateFirewallPolicyRequest
* @return A Java Future containing the result of the AssociateFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsync.AssociateFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future associateFirewallPolicyAsync(AssociateFirewallPolicyRequest associateFirewallPolicyRequest);
/**
*
* Associates a FirewallPolicy to a Firewall.
*
*
* A firewall policy defines how to monitor and manage your VPC network traffic, using a collection of inspection
* rule groups and other settings. Each firewall requires one firewall policy association, and you can use the same
* firewall policy for multiple firewalls.
*
*
* @param associateFirewallPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.AssociateFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future associateFirewallPolicyAsync(AssociateFirewallPolicyRequest associateFirewallPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified subnets in the Amazon VPC to the firewall. You can specify one subnet for each of the
* Availability Zones that the VPC spans.
*
*
* This request creates an Network Firewall firewall endpoint in each of the subnets. To enable the firewall's
* protections, you must also modify the VPC's route tables for each subnet's Availability Zone, to redirect the
* traffic that's coming into and going out of the zone through the firewall endpoint.
*
*
* @param associateSubnetsRequest
* @return A Java Future containing the result of the AssociateSubnets operation returned by the service.
* @sample AWSNetworkFirewallAsync.AssociateSubnets
* @see AWS API Documentation
*/
java.util.concurrent.Future associateSubnetsAsync(AssociateSubnetsRequest associateSubnetsRequest);
/**
*
* Associates the specified subnets in the Amazon VPC to the firewall. You can specify one subnet for each of the
* Availability Zones that the VPC spans.
*
*
* This request creates an Network Firewall firewall endpoint in each of the subnets. To enable the firewall's
* protections, you must also modify the VPC's route tables for each subnet's Availability Zone, to redirect the
* traffic that's coming into and going out of the zone through the firewall endpoint.
*
*
* @param associateSubnetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateSubnets operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.AssociateSubnets
* @see AWS API Documentation
*/
java.util.concurrent.Future associateSubnetsAsync(AssociateSubnetsRequest associateSubnetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Network Firewall Firewall and accompanying FirewallStatus for a VPC.
*
*
* The firewall defines the configuration settings for an Network Firewall firewall. The settings that you can
* define at creation include the firewall policy, the subnets in your VPC to use for the firewall endpoints, and
* any tags that are attached to the firewall Amazon Web Services resource.
*
*
* After you create a firewall, you can provide additional settings, like the logging configuration.
*
*
* To update the settings for a firewall, you use the operations that apply to the settings themselves, for example
* UpdateLoggingConfiguration, AssociateSubnets, and UpdateFirewallDeleteProtection.
*
*
* To manage a firewall's tags, use the standard Amazon Web Services resource tagging operations,
* ListTagsForResource, TagResource, and UntagResource.
*
*
* To retrieve information about firewalls, use ListFirewalls and DescribeFirewall.
*
*
* @param createFirewallRequest
* @return A Java Future containing the result of the CreateFirewall operation returned by the service.
* @sample AWSNetworkFirewallAsync.CreateFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future createFirewallAsync(CreateFirewallRequest createFirewallRequest);
/**
*
* Creates an Network Firewall Firewall and accompanying FirewallStatus for a VPC.
*
*
* The firewall defines the configuration settings for an Network Firewall firewall. The settings that you can
* define at creation include the firewall policy, the subnets in your VPC to use for the firewall endpoints, and
* any tags that are attached to the firewall Amazon Web Services resource.
*
*
* After you create a firewall, you can provide additional settings, like the logging configuration.
*
*
* To update the settings for a firewall, you use the operations that apply to the settings themselves, for example
* UpdateLoggingConfiguration, AssociateSubnets, and UpdateFirewallDeleteProtection.
*
*
* To manage a firewall's tags, use the standard Amazon Web Services resource tagging operations,
* ListTagsForResource, TagResource, and UntagResource.
*
*
* To retrieve information about firewalls, use ListFirewalls and DescribeFirewall.
*
*
* @param createFirewallRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFirewall operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.CreateFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future createFirewallAsync(CreateFirewallRequest createFirewallRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the firewall policy for the firewall according to the specifications.
*
*
* An Network Firewall firewall policy defines the behavior of a firewall, in a collection of stateless and stateful
* rule groups and other settings. You can use one firewall policy for multiple firewalls.
*
*
* @param createFirewallPolicyRequest
* @return A Java Future containing the result of the CreateFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsync.CreateFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createFirewallPolicyAsync(CreateFirewallPolicyRequest createFirewallPolicyRequest);
/**
*
* Creates the firewall policy for the firewall according to the specifications.
*
*
* An Network Firewall firewall policy defines the behavior of a firewall, in a collection of stateless and stateful
* rule groups and other settings. You can use one firewall policy for multiple firewalls.
*
*
* @param createFirewallPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.CreateFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createFirewallPolicyAsync(CreateFirewallPolicyRequest createFirewallPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the specified stateless or stateful rule group, which includes the rules for network traffic inspection,
* a capacity setting, and tags.
*
*
* You provide your rule group specification in your request using either RuleGroup
or
* Rules
.
*
*
* @param createRuleGroupRequest
* @return A Java Future containing the result of the CreateRuleGroup operation returned by the service.
* @sample AWSNetworkFirewallAsync.CreateRuleGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createRuleGroupAsync(CreateRuleGroupRequest createRuleGroupRequest);
/**
*
* Creates the specified stateless or stateful rule group, which includes the rules for network traffic inspection,
* a capacity setting, and tags.
*
*
* You provide your rule group specification in your request using either RuleGroup
or
* Rules
.
*
*
* @param createRuleGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRuleGroup operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.CreateRuleGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createRuleGroupAsync(CreateRuleGroupRequest createRuleGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Network Firewall TLS inspection configuration. Network Firewall uses TLS inspection configurations to
* decrypt your firewall's inbound and outbound SSL/TLS traffic. After decryption, Network Firewall inspects the
* traffic according to your firewall policy's stateful rules, and then re-encrypts it before sending it to its
* destination. You can enable inspection of your firewall's inbound traffic, outbound traffic, or both. To use TLS
* inspection with your firewall, you must first import or provision certificates using ACM, create a TLS inspection
* configuration, add that configuration to a new firewall policy, and then associate that policy with your
* firewall.
*
*
* To update the settings for a TLS inspection configuration, use UpdateTLSInspectionConfiguration.
*
*
* To manage a TLS inspection configuration's tags, use the standard Amazon Web Services resource tagging
* operations, ListTagsForResource, TagResource, and UntagResource.
*
*
* To retrieve information about TLS inspection configurations, use ListTLSInspectionConfigurations and
* DescribeTLSInspectionConfiguration.
*
*
* For more information about TLS inspection configurations, see Inspecting SSL/TLS
* traffic with TLS inspection configurations in the Network Firewall Developer Guide.
*
*
* @param createTLSInspectionConfigurationRequest
* @return A Java Future containing the result of the CreateTLSInspectionConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsync.CreateTLSInspectionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createTLSInspectionConfigurationAsync(
CreateTLSInspectionConfigurationRequest createTLSInspectionConfigurationRequest);
/**
*
* Creates an Network Firewall TLS inspection configuration. Network Firewall uses TLS inspection configurations to
* decrypt your firewall's inbound and outbound SSL/TLS traffic. After decryption, Network Firewall inspects the
* traffic according to your firewall policy's stateful rules, and then re-encrypts it before sending it to its
* destination. You can enable inspection of your firewall's inbound traffic, outbound traffic, or both. To use TLS
* inspection with your firewall, you must first import or provision certificates using ACM, create a TLS inspection
* configuration, add that configuration to a new firewall policy, and then associate that policy with your
* firewall.
*
*
* To update the settings for a TLS inspection configuration, use UpdateTLSInspectionConfiguration.
*
*
* To manage a TLS inspection configuration's tags, use the standard Amazon Web Services resource tagging
* operations, ListTagsForResource, TagResource, and UntagResource.
*
*
* To retrieve information about TLS inspection configurations, use ListTLSInspectionConfigurations and
* DescribeTLSInspectionConfiguration.
*
*
* For more information about TLS inspection configurations, see Inspecting SSL/TLS
* traffic with TLS inspection configurations in the Network Firewall Developer Guide.
*
*
* @param createTLSInspectionConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTLSInspectionConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsyncHandler.CreateTLSInspectionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createTLSInspectionConfigurationAsync(
CreateTLSInspectionConfigurationRequest createTLSInspectionConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Firewall and its FirewallStatus. This operation requires the firewall's
* DeleteProtection
flag to be FALSE
. You can't revert this operation.
*
*
* You can check whether a firewall is in use by reviewing the route tables for the Availability Zones where you
* have firewall subnet mappings. Retrieve the subnet mappings by calling DescribeFirewall. You define and
* update the route tables through Amazon VPC. As needed, update the route tables for the zones to remove the
* firewall endpoints. When the route tables no longer use the firewall endpoints, you can remove the firewall
* safely.
*
*
* To delete a firewall, remove the delete protection if you need to using UpdateFirewallDeleteProtection,
* then delete the firewall by calling DeleteFirewall.
*
*
* @param deleteFirewallRequest
* @return A Java Future containing the result of the DeleteFirewall operation returned by the service.
* @sample AWSNetworkFirewallAsync.DeleteFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFirewallAsync(DeleteFirewallRequest deleteFirewallRequest);
/**
*
* Deletes the specified Firewall and its FirewallStatus. This operation requires the firewall's
* DeleteProtection
flag to be FALSE
. You can't revert this operation.
*
*
* You can check whether a firewall is in use by reviewing the route tables for the Availability Zones where you
* have firewall subnet mappings. Retrieve the subnet mappings by calling DescribeFirewall. You define and
* update the route tables through Amazon VPC. As needed, update the route tables for the zones to remove the
* firewall endpoints. When the route tables no longer use the firewall endpoints, you can remove the firewall
* safely.
*
*
* To delete a firewall, remove the delete protection if you need to using UpdateFirewallDeleteProtection,
* then delete the firewall by calling DeleteFirewall.
*
*
* @param deleteFirewallRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFirewall operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DeleteFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFirewallAsync(DeleteFirewallRequest deleteFirewallRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified FirewallPolicy.
*
*
* @param deleteFirewallPolicyRequest
* @return A Java Future containing the result of the DeleteFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsync.DeleteFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFirewallPolicyAsync(DeleteFirewallPolicyRequest deleteFirewallPolicyRequest);
/**
*
* Deletes the specified FirewallPolicy.
*
*
* @param deleteFirewallPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DeleteFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFirewallPolicyAsync(DeleteFirewallPolicyRequest deleteFirewallPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a resource policy that you created in a PutResourcePolicy request.
*
*
* @param deleteResourcePolicyRequest
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* @sample AWSNetworkFirewallAsync.DeleteResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteResourcePolicyAsync(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Deletes a resource policy that you created in a PutResourcePolicy request.
*
*
* @param deleteResourcePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DeleteResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteResourcePolicyAsync(DeleteResourcePolicyRequest deleteResourcePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified RuleGroup.
*
*
* @param deleteRuleGroupRequest
* @return A Java Future containing the result of the DeleteRuleGroup operation returned by the service.
* @sample AWSNetworkFirewallAsync.DeleteRuleGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRuleGroupAsync(DeleteRuleGroupRequest deleteRuleGroupRequest);
/**
*
* Deletes the specified RuleGroup.
*
*
* @param deleteRuleGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRuleGroup operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DeleteRuleGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRuleGroupAsync(DeleteRuleGroupRequest deleteRuleGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified TLSInspectionConfiguration.
*
*
* @param deleteTLSInspectionConfigurationRequest
* @return A Java Future containing the result of the DeleteTLSInspectionConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsync.DeleteTLSInspectionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTLSInspectionConfigurationAsync(
DeleteTLSInspectionConfigurationRequest deleteTLSInspectionConfigurationRequest);
/**
*
* Deletes the specified TLSInspectionConfiguration.
*
*
* @param deleteTLSInspectionConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTLSInspectionConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsyncHandler.DeleteTLSInspectionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTLSInspectionConfigurationAsync(
DeleteTLSInspectionConfigurationRequest deleteTLSInspectionConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the data objects for the specified firewall.
*
*
* @param describeFirewallRequest
* @return A Java Future containing the result of the DescribeFirewall operation returned by the service.
* @sample AWSNetworkFirewallAsync.DescribeFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFirewallAsync(DescribeFirewallRequest describeFirewallRequest);
/**
*
* Returns the data objects for the specified firewall.
*
*
* @param describeFirewallRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFirewall operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DescribeFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFirewallAsync(DescribeFirewallRequest describeFirewallRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the data objects for the specified firewall policy.
*
*
* @param describeFirewallPolicyRequest
* @return A Java Future containing the result of the DescribeFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsync.DescribeFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFirewallPolicyAsync(DescribeFirewallPolicyRequest describeFirewallPolicyRequest);
/**
*
* Returns the data objects for the specified firewall policy.
*
*
* @param describeFirewallPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DescribeFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFirewallPolicyAsync(DescribeFirewallPolicyRequest describeFirewallPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the logging configuration for the specified firewall.
*
*
* @param describeLoggingConfigurationRequest
* @return A Java Future containing the result of the DescribeLoggingConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsync.DescribeLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLoggingConfigurationAsync(
DescribeLoggingConfigurationRequest describeLoggingConfigurationRequest);
/**
*
* Returns the logging configuration for the specified firewall.
*
*
* @param describeLoggingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLoggingConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsyncHandler.DescribeLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLoggingConfigurationAsync(
DescribeLoggingConfigurationRequest describeLoggingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a resource policy that you created in a PutResourcePolicy request.
*
*
* @param describeResourcePolicyRequest
* @return A Java Future containing the result of the DescribeResourcePolicy operation returned by the service.
* @sample AWSNetworkFirewallAsync.DescribeResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future describeResourcePolicyAsync(DescribeResourcePolicyRequest describeResourcePolicyRequest);
/**
*
* Retrieves a resource policy that you created in a PutResourcePolicy request.
*
*
* @param describeResourcePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeResourcePolicy operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DescribeResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future describeResourcePolicyAsync(DescribeResourcePolicyRequest describeResourcePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the data objects for the specified rule group.
*
*
* @param describeRuleGroupRequest
* @return A Java Future containing the result of the DescribeRuleGroup operation returned by the service.
* @sample AWSNetworkFirewallAsync.DescribeRuleGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRuleGroupAsync(DescribeRuleGroupRequest describeRuleGroupRequest);
/**
*
* Returns the data objects for the specified rule group.
*
*
* @param describeRuleGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRuleGroup operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DescribeRuleGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRuleGroupAsync(DescribeRuleGroupRequest describeRuleGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* High-level information about a rule group, returned by operations like create and describe. You can use the
* information provided in the metadata to retrieve and manage a rule group. You can retrieve all objects for a rule
* group by calling DescribeRuleGroup.
*
*
* @param describeRuleGroupMetadataRequest
* @return A Java Future containing the result of the DescribeRuleGroupMetadata operation returned by the service.
* @sample AWSNetworkFirewallAsync.DescribeRuleGroupMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRuleGroupMetadataAsync(
DescribeRuleGroupMetadataRequest describeRuleGroupMetadataRequest);
/**
*
* High-level information about a rule group, returned by operations like create and describe. You can use the
* information provided in the metadata to retrieve and manage a rule group. You can retrieve all objects for a rule
* group by calling DescribeRuleGroup.
*
*
* @param describeRuleGroupMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRuleGroupMetadata operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DescribeRuleGroupMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRuleGroupMetadataAsync(
DescribeRuleGroupMetadataRequest describeRuleGroupMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the data objects for the specified TLS inspection configuration.
*
*
* @param describeTLSInspectionConfigurationRequest
* @return A Java Future containing the result of the DescribeTLSInspectionConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsync.DescribeTLSInspectionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTLSInspectionConfigurationAsync(
DescribeTLSInspectionConfigurationRequest describeTLSInspectionConfigurationRequest);
/**
*
* Returns the data objects for the specified TLS inspection configuration.
*
*
* @param describeTLSInspectionConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTLSInspectionConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsyncHandler.DescribeTLSInspectionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTLSInspectionConfigurationAsync(
DescribeTLSInspectionConfigurationRequest describeTLSInspectionConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified subnet associations from the firewall. This removes the firewall endpoints from the subnets
* and removes any network filtering protections that the endpoints were providing.
*
*
* @param disassociateSubnetsRequest
* @return A Java Future containing the result of the DisassociateSubnets operation returned by the service.
* @sample AWSNetworkFirewallAsync.DisassociateSubnets
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateSubnetsAsync(DisassociateSubnetsRequest disassociateSubnetsRequest);
/**
*
* Removes the specified subnet associations from the firewall. This removes the firewall endpoints from the subnets
* and removes any network filtering protections that the endpoints were providing.
*
*
* @param disassociateSubnetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateSubnets operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.DisassociateSubnets
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateSubnetsAsync(DisassociateSubnetsRequest disassociateSubnetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the metadata for the firewall policies that you have defined. Depending on your setting for max results
* and the number of firewall policies, a single call might not return the full list.
*
*
* @param listFirewallPoliciesRequest
* @return A Java Future containing the result of the ListFirewallPolicies operation returned by the service.
* @sample AWSNetworkFirewallAsync.ListFirewallPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listFirewallPoliciesAsync(ListFirewallPoliciesRequest listFirewallPoliciesRequest);
/**
*
* Retrieves the metadata for the firewall policies that you have defined. Depending on your setting for max results
* and the number of firewall policies, a single call might not return the full list.
*
*
* @param listFirewallPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFirewallPolicies operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.ListFirewallPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listFirewallPoliciesAsync(ListFirewallPoliciesRequest listFirewallPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the metadata for the firewalls that you have defined. If you provide VPC identifiers in your request,
* this returns only the firewalls for those VPCs.
*
*
* Depending on your setting for max results and the number of firewalls, a single call might not return the full
* list.
*
*
* @param listFirewallsRequest
* @return A Java Future containing the result of the ListFirewalls operation returned by the service.
* @sample AWSNetworkFirewallAsync.ListFirewalls
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listFirewallsAsync(ListFirewallsRequest listFirewallsRequest);
/**
*
* Retrieves the metadata for the firewalls that you have defined. If you provide VPC identifiers in your request,
* this returns only the firewalls for those VPCs.
*
*
* Depending on your setting for max results and the number of firewalls, a single call might not return the full
* list.
*
*
* @param listFirewallsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFirewalls operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.ListFirewalls
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listFirewallsAsync(ListFirewallsRequest listFirewallsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the metadata for the rule groups that you have defined. Depending on your setting for max results and
* the number of rule groups, a single call might not return the full list.
*
*
* @param listRuleGroupsRequest
* @return A Java Future containing the result of the ListRuleGroups operation returned by the service.
* @sample AWSNetworkFirewallAsync.ListRuleGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listRuleGroupsAsync(ListRuleGroupsRequest listRuleGroupsRequest);
/**
*
* Retrieves the metadata for the rule groups that you have defined. Depending on your setting for max results and
* the number of rule groups, a single call might not return the full list.
*
*
* @param listRuleGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRuleGroups operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.ListRuleGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listRuleGroupsAsync(ListRuleGroupsRequest listRuleGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the metadata for the TLS inspection configurations that you have defined. Depending on your setting for
* max results and the number of TLS inspection configurations, a single call might not return the full list.
*
*
* @param listTLSInspectionConfigurationsRequest
* @return A Java Future containing the result of the ListTLSInspectionConfigurations operation returned by the
* service.
* @sample AWSNetworkFirewallAsync.ListTLSInspectionConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listTLSInspectionConfigurationsAsync(
ListTLSInspectionConfigurationsRequest listTLSInspectionConfigurationsRequest);
/**
*
* Retrieves the metadata for the TLS inspection configurations that you have defined. Depending on your setting for
* max results and the number of TLS inspection configurations, a single call might not return the full list.
*
*
* @param listTLSInspectionConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTLSInspectionConfigurations operation returned by the
* service.
* @sample AWSNetworkFirewallAsyncHandler.ListTLSInspectionConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listTLSInspectionConfigurationsAsync(
ListTLSInspectionConfigurationsRequest listTLSInspectionConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the tags associated with the specified resource. Tags are key:value pairs that you can use to
* categorize and manage your resources, for purposes like billing. For example, you might set the tag key to
* "customer" and the value to the customer name or ID. You can specify one or more tags to add to each Amazon Web
* Services resource, up to 50 tags for a resource.
*
*
* You can tag the Amazon Web Services resources that you manage through Network Firewall: firewalls, firewall
* policies, and rule groups.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSNetworkFirewallAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves the tags associated with the specified resource. Tags are key:value pairs that you can use to
* categorize and manage your resources, for purposes like billing. For example, you might set the tag key to
* "customer" and the value to the customer name or ID. You can specify one or more tags to add to each Amazon Web
* Services resource, up to 50 tags for a resource.
*
*
* You can tag the Amazon Web Services resources that you manage through Network Firewall: firewalls, firewall
* policies, and rule groups.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates an IAM policy for your rule group or firewall policy. Use this to share rule groups and
* firewall policies between accounts. This operation works in conjunction with the Amazon Web Services Resource
* Access Manager (RAM) service to manage resource sharing for Network Firewall.
*
*
* Use this operation to create or update a resource policy for your rule group or firewall policy. In the policy,
* you specify the accounts that you want to share the resource with and the operations that you want the accounts
* to be able to perform.
*
*
* When you add an account in the resource policy, you then run the following Resource Access Manager (RAM)
* operations to access and accept the shared rule group or firewall policy.
*
*
* -
*
*
* GetResourceShareInvitations - Returns the Amazon Resource Names (ARNs) of the resource share invitations.
*
*
* -
*
*
* AcceptResourceShareInvitation - Accepts the share invitation for a specified resource share.
*
*
*
*
* For additional information about resource sharing using RAM, see Resource Access Manager User Guide.
*
*
* @param putResourcePolicyRequest
* @return A Java Future containing the result of the PutResourcePolicy operation returned by the service.
* @sample AWSNetworkFirewallAsync.PutResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putResourcePolicyAsync(PutResourcePolicyRequest putResourcePolicyRequest);
/**
*
* Creates or updates an IAM policy for your rule group or firewall policy. Use this to share rule groups and
* firewall policies between accounts. This operation works in conjunction with the Amazon Web Services Resource
* Access Manager (RAM) service to manage resource sharing for Network Firewall.
*
*
* Use this operation to create or update a resource policy for your rule group or firewall policy. In the policy,
* you specify the accounts that you want to share the resource with and the operations that you want the accounts
* to be able to perform.
*
*
* When you add an account in the resource policy, you then run the following Resource Access Manager (RAM)
* operations to access and accept the shared rule group or firewall policy.
*
*
* -
*
*
* GetResourceShareInvitations - Returns the Amazon Resource Names (ARNs) of the resource share invitations.
*
*
* -
*
*
* AcceptResourceShareInvitation - Accepts the share invitation for a specified resource share.
*
*
*
*
* For additional information about resource sharing using RAM, see Resource Access Manager User Guide.
*
*
* @param putResourcePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutResourcePolicy operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.PutResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putResourcePolicyAsync(PutResourcePolicyRequest putResourcePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified tags to the specified resource. Tags are key:value pairs that you can use to categorize and
* manage your resources, for purposes like billing. For example, you might set the tag key to "customer" and the
* value to the customer name or ID. You can specify one or more tags to add to each Amazon Web Services resource,
* up to 50 tags for a resource.
*
*
* You can tag the Amazon Web Services resources that you manage through Network Firewall: firewalls, firewall
* policies, and rule groups.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSNetworkFirewallAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds the specified tags to the specified resource. Tags are key:value pairs that you can use to categorize and
* manage your resources, for purposes like billing. For example, you might set the tag key to "customer" and the
* value to the customer name or ID. You can specify one or more tags to add to each Amazon Web Services resource,
* up to 50 tags for a resource.
*
*
* You can tag the Amazon Web Services resources that you manage through Network Firewall: firewalls, firewall
* policies, and rule groups.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the tags with the specified keys from the specified resource. Tags are key:value pairs that you can use
* to categorize and manage your resources, for purposes like billing. For example, you might set the tag key to
* "customer" and the value to the customer name or ID. You can specify one or more tags to add to each Amazon Web
* Services resource, up to 50 tags for a resource.
*
*
* You can manage tags for the Amazon Web Services resources that you manage through Network Firewall: firewalls,
* firewall policies, and rule groups.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSNetworkFirewallAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes the tags with the specified keys from the specified resource. Tags are key:value pairs that you can use
* to categorize and manage your resources, for purposes like billing. For example, you might set the tag key to
* "customer" and the value to the customer name or ID. You can specify one or more tags to add to each Amazon Web
* Services resource, up to 50 tags for a resource.
*
*
* You can manage tags for the Amazon Web Services resources that you manage through Network Firewall: firewalls,
* firewall policies, and rule groups.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the flag, DeleteProtection
, which indicates whether it is possible to delete the firewall.
* If the flag is set to TRUE
, the firewall is protected against deletion. This setting helps protect
* against accidentally deleting a firewall that's in use.
*
*
* @param updateFirewallDeleteProtectionRequest
* @return A Java Future containing the result of the UpdateFirewallDeleteProtection operation returned by the
* service.
* @sample AWSNetworkFirewallAsync.UpdateFirewallDeleteProtection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallDeleteProtectionAsync(
UpdateFirewallDeleteProtectionRequest updateFirewallDeleteProtectionRequest);
/**
*
* Modifies the flag, DeleteProtection
, which indicates whether it is possible to delete the firewall.
* If the flag is set to TRUE
, the firewall is protected against deletion. This setting helps protect
* against accidentally deleting a firewall that's in use.
*
*
* @param updateFirewallDeleteProtectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFirewallDeleteProtection operation returned by the
* service.
* @sample AWSNetworkFirewallAsyncHandler.UpdateFirewallDeleteProtection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallDeleteProtectionAsync(
UpdateFirewallDeleteProtectionRequest updateFirewallDeleteProtectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the description for the specified firewall. Use the description to help you identify the firewall when
* you're working with it.
*
*
* @param updateFirewallDescriptionRequest
* @return A Java Future containing the result of the UpdateFirewallDescription operation returned by the service.
* @sample AWSNetworkFirewallAsync.UpdateFirewallDescription
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallDescriptionAsync(
UpdateFirewallDescriptionRequest updateFirewallDescriptionRequest);
/**
*
* Modifies the description for the specified firewall. Use the description to help you identify the firewall when
* you're working with it.
*
*
* @param updateFirewallDescriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFirewallDescription operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.UpdateFirewallDescription
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallDescriptionAsync(
UpdateFirewallDescriptionRequest updateFirewallDescriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A complex type that contains settings for encryption of your firewall resources.
*
*
* @param updateFirewallEncryptionConfigurationRequest
* @return A Java Future containing the result of the UpdateFirewallEncryptionConfiguration operation returned by
* the service.
* @sample AWSNetworkFirewallAsync.UpdateFirewallEncryptionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallEncryptionConfigurationAsync(
UpdateFirewallEncryptionConfigurationRequest updateFirewallEncryptionConfigurationRequest);
/**
*
* A complex type that contains settings for encryption of your firewall resources.
*
*
* @param updateFirewallEncryptionConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFirewallEncryptionConfiguration operation returned by
* the service.
* @sample AWSNetworkFirewallAsyncHandler.UpdateFirewallEncryptionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallEncryptionConfigurationAsync(
UpdateFirewallEncryptionConfigurationRequest updateFirewallEncryptionConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the properties of the specified firewall policy.
*
*
* @param updateFirewallPolicyRequest
* @return A Java Future containing the result of the UpdateFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsync.UpdateFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallPolicyAsync(UpdateFirewallPolicyRequest updateFirewallPolicyRequest);
/**
*
* Updates the properties of the specified firewall policy.
*
*
* @param updateFirewallPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFirewallPolicy operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.UpdateFirewallPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallPolicyAsync(UpdateFirewallPolicyRequest updateFirewallPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the flag, ChangeProtection
, which indicates whether it is possible to change the firewall.
* If the flag is set to TRUE
, the firewall is protected from changes. This setting helps protect
* against accidentally changing a firewall that's in use.
*
*
* @param updateFirewallPolicyChangeProtectionRequest
* @return A Java Future containing the result of the UpdateFirewallPolicyChangeProtection operation returned by the
* service.
* @sample AWSNetworkFirewallAsync.UpdateFirewallPolicyChangeProtection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallPolicyChangeProtectionAsync(
UpdateFirewallPolicyChangeProtectionRequest updateFirewallPolicyChangeProtectionRequest);
/**
*
* Modifies the flag, ChangeProtection
, which indicates whether it is possible to change the firewall.
* If the flag is set to TRUE
, the firewall is protected from changes. This setting helps protect
* against accidentally changing a firewall that's in use.
*
*
* @param updateFirewallPolicyChangeProtectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFirewallPolicyChangeProtection operation returned by the
* service.
* @sample AWSNetworkFirewallAsyncHandler.UpdateFirewallPolicyChangeProtection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFirewallPolicyChangeProtectionAsync(
UpdateFirewallPolicyChangeProtectionRequest updateFirewallPolicyChangeProtectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the logging configuration for the specified firewall.
*
*
* To change the logging configuration, retrieve the LoggingConfiguration by calling
* DescribeLoggingConfiguration, then change it and provide the modified object to this update call. You must
* change the logging configuration one LogDestinationConfig at a time inside the retrieved
* LoggingConfiguration object.
*
*
* You can perform only one of the following actions in any call to UpdateLoggingConfiguration
:
*
*
* -
*
* Create a new log destination object by adding a single LogDestinationConfig
array element to
* LogDestinationConfigs
.
*
*
* -
*
* Delete a log destination object by removing a single LogDestinationConfig
array element from
* LogDestinationConfigs
.
*
*
* -
*
* Change the LogDestination
setting in a single LogDestinationConfig
array element.
*
*
*
*
* You can't change the LogDestinationType
or LogType
in a
* LogDestinationConfig
. To change these settings, delete the existing
* LogDestinationConfig
object and create a new one, using two separate calls to this update operation.
*
*
* @param updateLoggingConfigurationRequest
* @return A Java Future containing the result of the UpdateLoggingConfiguration operation returned by the service.
* @sample AWSNetworkFirewallAsync.UpdateLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLoggingConfigurationAsync(
UpdateLoggingConfigurationRequest updateLoggingConfigurationRequest);
/**
*
* Sets the logging configuration for the specified firewall.
*
*
* To change the logging configuration, retrieve the LoggingConfiguration by calling
* DescribeLoggingConfiguration, then change it and provide the modified object to this update call. You must
* change the logging configuration one LogDestinationConfig at a time inside the retrieved
* LoggingConfiguration object.
*
*
* You can perform only one of the following actions in any call to UpdateLoggingConfiguration
:
*
*
* -
*
* Create a new log destination object by adding a single LogDestinationConfig
array element to
* LogDestinationConfigs
.
*
*
* -
*
* Delete a log destination object by removing a single LogDestinationConfig
array element from
* LogDestinationConfigs
.
*
*
* -
*
* Change the LogDestination
setting in a single LogDestinationConfig
array element.
*
*
*
*
* You can't change the LogDestinationType
or LogType
in a
* LogDestinationConfig
. To change these settings, delete the existing
* LogDestinationConfig
object and create a new one, using two separate calls to this update operation.
*
*
* @param updateLoggingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLoggingConfiguration operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.UpdateLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLoggingConfigurationAsync(
UpdateLoggingConfigurationRequest updateLoggingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the rule settings for the specified rule group. You use a rule group by reference in one or more firewall
* policies. When you modify a rule group, you modify all firewall policies that use the rule group.
*
*
* To update a rule group, first call DescribeRuleGroup to retrieve the current RuleGroup object,
* update the object as needed, and then provide the updated object to this call.
*
*
* @param updateRuleGroupRequest
* @return A Java Future containing the result of the UpdateRuleGroup operation returned by the service.
* @sample AWSNetworkFirewallAsync.UpdateRuleGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRuleGroupAsync(UpdateRuleGroupRequest updateRuleGroupRequest);
/**
*
* Updates the rule settings for the specified rule group. You use a rule group by reference in one or more firewall
* policies. When you modify a rule group, you modify all firewall policies that use the rule group.
*
*
* To update a rule group, first call DescribeRuleGroup to retrieve the current RuleGroup object,
* update the object as needed, and then provide the updated object to this call.
*
*
* @param updateRuleGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRuleGroup operation returned by the service.
* @sample AWSNetworkFirewallAsyncHandler.UpdateRuleGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRuleGroupAsync(UpdateRuleGroupRequest updateRuleGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* @param updateSubnetChangeProtectionRequest
* @return A Java Future containing the result of the UpdateSubnetChangeProtection operation returned by the
* service.
* @sample AWSNetworkFirewallAsync.UpdateSubnetChangeProtection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSubnetChangeProtectionAsync(
UpdateSubnetChangeProtectionRequest updateSubnetChangeProtectionRequest);
/**
*
*
* @param updateSubnetChangeProtectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSubnetChangeProtection operation returned by the
* service.
* @sample AWSNetworkFirewallAsyncHandler.UpdateSubnetChangeProtection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSubnetChangeProtectionAsync(
UpdateSubnetChangeProtectionRequest updateSubnetChangeProtectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the TLS inspection configuration settings for the specified TLS inspection configuration. You use a TLS
* inspection configuration by referencing it in one or more firewall policies. When you modify a TLS inspection
* configuration, you modify all firewall policies that use the TLS inspection configuration.
*
*
* To update a TLS inspection configuration, first call DescribeTLSInspectionConfiguration to retrieve the
* current TLSInspectionConfiguration object, update the object as needed, and then provide the updated
* object to this call.
*
*
* @param updateTLSInspectionConfigurationRequest
* @return A Java Future containing the result of the UpdateTLSInspectionConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsync.UpdateTLSInspectionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTLSInspectionConfigurationAsync(
UpdateTLSInspectionConfigurationRequest updateTLSInspectionConfigurationRequest);
/**
*
* Updates the TLS inspection configuration settings for the specified TLS inspection configuration. You use a TLS
* inspection configuration by referencing it in one or more firewall policies. When you modify a TLS inspection
* configuration, you modify all firewall policies that use the TLS inspection configuration.
*
*
* To update a TLS inspection configuration, first call DescribeTLSInspectionConfiguration to retrieve the
* current TLSInspectionConfiguration object, update the object as needed, and then provide the updated
* object to this call.
*
*
* @param updateTLSInspectionConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTLSInspectionConfiguration operation returned by the
* service.
* @sample AWSNetworkFirewallAsyncHandler.UpdateTLSInspectionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTLSInspectionConfigurationAsync(
UpdateTLSInspectionConfigurationRequest updateTLSInspectionConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}