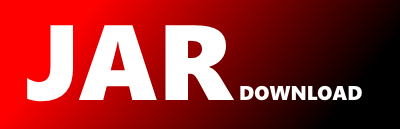
com.amazonaws.services.networkfirewall.model.RuleGroupResponse Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkfirewall Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkfirewall.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The high-level properties of a rule group. This, along with the RuleGroup, define the rule group. You can
* retrieve all objects for a rule group by calling DescribeRuleGroup.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RuleGroupResponse implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the rule group.
*
*
*
* If this response is for a create request that had DryRun
set to TRUE
, then this ARN is
* a placeholder that isn't attached to a valid resource.
*
*
*/
private String ruleGroupArn;
/**
*
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*
*/
private String ruleGroupName;
/**
*
* The unique identifier for the rule group.
*
*/
private String ruleGroupId;
/**
*
* A description of the rule group.
*
*/
private String description;
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*/
private String type;
/**
*
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation. When you
* update a rule group, you are limited to this capacity. When you reference a rule group from a firewall policy,
* Network Firewall reserves this capacity for the rule group.
*
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by calling
* CreateRuleGroup with DryRun
set to TRUE
.
*
*/
private Integer capacity;
/**
*
* Detailed information about the current status of a rule group.
*
*/
private String ruleGroupStatus;
/**
*
* The key:value pairs to associate with the resource.
*
*/
private java.util.List tags;
/**
*
* The number of capacity units currently consumed by the rule group rules.
*
*/
private Integer consumedCapacity;
/**
*
* The number of firewall policies that use this rule group.
*
*/
private Integer numberOfAssociations;
/**
*
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your rule group.
*
*/
private EncryptionConfiguration encryptionConfiguration;
/**
*
* A complex type that contains metadata about the rule group that your own rule group is copied from. You can use
* the metadata to track the version updates made to the originating rule group.
*
*/
private SourceMetadata sourceMetadata;
/**
*
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to record changes
* to the managed rule group. You can subscribe to the SNS topic to receive notifications when the managed rule
* group is modified, such as for new versions and for version expiration. For more information, see the Amazon Simple Notification Service Developer
* Guide..
*
*/
private String snsTopic;
/**
*
* The last time that the rule group was changed.
*
*/
private java.util.Date lastModifiedTime;
/**
*
* The list of analysis results for AnalyzeRuleGroup
. If you set AnalyzeRuleGroup
to
* TRUE
in CreateRuleGroup, UpdateRuleGroup, or DescribeRuleGroup, Network
* Firewall analyzes the rule group and identifies the rules that might adversely effect your firewall's
* functionality. For example, if Network Firewall detects a rule that's routing traffic asymmetrically, which
* impacts the service's ability to properly process traffic, the service includes the rule in the list of analysis
* results.
*
*/
private java.util.List analysisResults;
/**
*
* The Amazon Resource Name (ARN) of the rule group.
*
*
*
* If this response is for a create request that had DryRun
set to TRUE
, then this ARN is
* a placeholder that isn't attached to a valid resource.
*
*
*
* @param ruleGroupArn
* The Amazon Resource Name (ARN) of the rule group.
*
* If this response is for a create request that had DryRun
set to TRUE
, then this
* ARN is a placeholder that isn't attached to a valid resource.
*
*/
public void setRuleGroupArn(String ruleGroupArn) {
this.ruleGroupArn = ruleGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the rule group.
*
*
*
* If this response is for a create request that had DryRun
set to TRUE
, then this ARN is
* a placeholder that isn't attached to a valid resource.
*
*
*
* @return The Amazon Resource Name (ARN) of the rule group.
*
* If this response is for a create request that had DryRun
set to TRUE
, then this
* ARN is a placeholder that isn't attached to a valid resource.
*
*/
public String getRuleGroupArn() {
return this.ruleGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the rule group.
*
*
*
* If this response is for a create request that had DryRun
set to TRUE
, then this ARN is
* a placeholder that isn't attached to a valid resource.
*
*
*
* @param ruleGroupArn
* The Amazon Resource Name (ARN) of the rule group.
*
* If this response is for a create request that had DryRun
set to TRUE
, then this
* ARN is a placeholder that isn't attached to a valid resource.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withRuleGroupArn(String ruleGroupArn) {
setRuleGroupArn(ruleGroupArn);
return this;
}
/**
*
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*
*
* @param ruleGroupName
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*/
public void setRuleGroupName(String ruleGroupName) {
this.ruleGroupName = ruleGroupName;
}
/**
*
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*
*
* @return The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*/
public String getRuleGroupName() {
return this.ruleGroupName;
}
/**
*
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
*
*
* @param ruleGroupName
* The descriptive name of the rule group. You can't change the name of a rule group after you create it.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withRuleGroupName(String ruleGroupName) {
setRuleGroupName(ruleGroupName);
return this;
}
/**
*
* The unique identifier for the rule group.
*
*
* @param ruleGroupId
* The unique identifier for the rule group.
*/
public void setRuleGroupId(String ruleGroupId) {
this.ruleGroupId = ruleGroupId;
}
/**
*
* The unique identifier for the rule group.
*
*
* @return The unique identifier for the rule group.
*/
public String getRuleGroupId() {
return this.ruleGroupId;
}
/**
*
* The unique identifier for the rule group.
*
*
* @param ruleGroupId
* The unique identifier for the rule group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withRuleGroupId(String ruleGroupId) {
setRuleGroupId(ruleGroupId);
return this;
}
/**
*
* A description of the rule group.
*
*
* @param description
* A description of the rule group.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the rule group.
*
*
* @return A description of the rule group.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the rule group.
*
*
* @param description
* A description of the rule group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*
* @param type
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains
* stateless rules. If it is stateful, it contains stateful rules.
* @see RuleGroupType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*
* @return Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains
* stateless rules. If it is stateful, it contains stateful rules.
* @see RuleGroupType
*/
public String getType() {
return this.type;
}
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*
* @param type
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains
* stateless rules. If it is stateful, it contains stateful rules.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RuleGroupType
*/
public RuleGroupResponse withType(String type) {
setType(type);
return this;
}
/**
*
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains stateless
* rules. If it is stateful, it contains stateful rules.
*
*
* @param type
* Indicates whether the rule group is stateless or stateful. If the rule group is stateless, it contains
* stateless rules. If it is stateful, it contains stateful rules.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RuleGroupType
*/
public RuleGroupResponse withType(RuleGroupType type) {
this.type = type.toString();
return this;
}
/**
*
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation. When you
* update a rule group, you are limited to this capacity. When you reference a rule group from a firewall policy,
* Network Firewall reserves this capacity for the rule group.
*
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by calling
* CreateRuleGroup with DryRun
set to TRUE
.
*
*
* @param capacity
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation.
* When you update a rule group, you are limited to this capacity. When you reference a rule group from a
* firewall policy, Network Firewall reserves this capacity for the rule group.
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by
* calling CreateRuleGroup with DryRun
set to TRUE
.
*/
public void setCapacity(Integer capacity) {
this.capacity = capacity;
}
/**
*
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation. When you
* update a rule group, you are limited to this capacity. When you reference a rule group from a firewall policy,
* Network Firewall reserves this capacity for the rule group.
*
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by calling
* CreateRuleGroup with DryRun
set to TRUE
.
*
*
* @return The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation.
* When you update a rule group, you are limited to this capacity. When you reference a rule group from a
* firewall policy, Network Firewall reserves this capacity for the rule group.
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by
* calling CreateRuleGroup with DryRun
set to TRUE
.
*/
public Integer getCapacity() {
return this.capacity;
}
/**
*
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation. When you
* update a rule group, you are limited to this capacity. When you reference a rule group from a firewall policy,
* Network Firewall reserves this capacity for the rule group.
*
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by calling
* CreateRuleGroup with DryRun
set to TRUE
.
*
*
* @param capacity
* The maximum operating resources that this rule group can use. Rule group capacity is fixed at creation.
* When you update a rule group, you are limited to this capacity. When you reference a rule group from a
* firewall policy, Network Firewall reserves this capacity for the rule group.
*
* You can retrieve the capacity that would be required for a rule group before you create the rule group by
* calling CreateRuleGroup with DryRun
set to TRUE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withCapacity(Integer capacity) {
setCapacity(capacity);
return this;
}
/**
*
* Detailed information about the current status of a rule group.
*
*
* @param ruleGroupStatus
* Detailed information about the current status of a rule group.
* @see ResourceStatus
*/
public void setRuleGroupStatus(String ruleGroupStatus) {
this.ruleGroupStatus = ruleGroupStatus;
}
/**
*
* Detailed information about the current status of a rule group.
*
*
* @return Detailed information about the current status of a rule group.
* @see ResourceStatus
*/
public String getRuleGroupStatus() {
return this.ruleGroupStatus;
}
/**
*
* Detailed information about the current status of a rule group.
*
*
* @param ruleGroupStatus
* Detailed information about the current status of a rule group.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceStatus
*/
public RuleGroupResponse withRuleGroupStatus(String ruleGroupStatus) {
setRuleGroupStatus(ruleGroupStatus);
return this;
}
/**
*
* Detailed information about the current status of a rule group.
*
*
* @param ruleGroupStatus
* Detailed information about the current status of a rule group.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceStatus
*/
public RuleGroupResponse withRuleGroupStatus(ResourceStatus ruleGroupStatus) {
this.ruleGroupStatus = ruleGroupStatus.toString();
return this;
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* @return The key:value pairs to associate with the resource.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* @param tags
* The key:value pairs to associate with the resource.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The key:value pairs to associate with the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The key:value pairs to associate with the resource.
*
*
* @param tags
* The key:value pairs to associate with the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The number of capacity units currently consumed by the rule group rules.
*
*
* @param consumedCapacity
* The number of capacity units currently consumed by the rule group rules.
*/
public void setConsumedCapacity(Integer consumedCapacity) {
this.consumedCapacity = consumedCapacity;
}
/**
*
* The number of capacity units currently consumed by the rule group rules.
*
*
* @return The number of capacity units currently consumed by the rule group rules.
*/
public Integer getConsumedCapacity() {
return this.consumedCapacity;
}
/**
*
* The number of capacity units currently consumed by the rule group rules.
*
*
* @param consumedCapacity
* The number of capacity units currently consumed by the rule group rules.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withConsumedCapacity(Integer consumedCapacity) {
setConsumedCapacity(consumedCapacity);
return this;
}
/**
*
* The number of firewall policies that use this rule group.
*
*
* @param numberOfAssociations
* The number of firewall policies that use this rule group.
*/
public void setNumberOfAssociations(Integer numberOfAssociations) {
this.numberOfAssociations = numberOfAssociations;
}
/**
*
* The number of firewall policies that use this rule group.
*
*
* @return The number of firewall policies that use this rule group.
*/
public Integer getNumberOfAssociations() {
return this.numberOfAssociations;
}
/**
*
* The number of firewall policies that use this rule group.
*
*
* @param numberOfAssociations
* The number of firewall policies that use this rule group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withNumberOfAssociations(Integer numberOfAssociations) {
setNumberOfAssociations(numberOfAssociations);
return this;
}
/**
*
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your rule group.
*
*
* @param encryptionConfiguration
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your rule
* group.
*/
public void setEncryptionConfiguration(EncryptionConfiguration encryptionConfiguration) {
this.encryptionConfiguration = encryptionConfiguration;
}
/**
*
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your rule group.
*
*
* @return A complex type that contains the Amazon Web Services KMS encryption configuration settings for your rule
* group.
*/
public EncryptionConfiguration getEncryptionConfiguration() {
return this.encryptionConfiguration;
}
/**
*
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your rule group.
*
*
* @param encryptionConfiguration
* A complex type that contains the Amazon Web Services KMS encryption configuration settings for your rule
* group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withEncryptionConfiguration(EncryptionConfiguration encryptionConfiguration) {
setEncryptionConfiguration(encryptionConfiguration);
return this;
}
/**
*
* A complex type that contains metadata about the rule group that your own rule group is copied from. You can use
* the metadata to track the version updates made to the originating rule group.
*
*
* @param sourceMetadata
* A complex type that contains metadata about the rule group that your own rule group is copied from. You
* can use the metadata to track the version updates made to the originating rule group.
*/
public void setSourceMetadata(SourceMetadata sourceMetadata) {
this.sourceMetadata = sourceMetadata;
}
/**
*
* A complex type that contains metadata about the rule group that your own rule group is copied from. You can use
* the metadata to track the version updates made to the originating rule group.
*
*
* @return A complex type that contains metadata about the rule group that your own rule group is copied from. You
* can use the metadata to track the version updates made to the originating rule group.
*/
public SourceMetadata getSourceMetadata() {
return this.sourceMetadata;
}
/**
*
* A complex type that contains metadata about the rule group that your own rule group is copied from. You can use
* the metadata to track the version updates made to the originating rule group.
*
*
* @param sourceMetadata
* A complex type that contains metadata about the rule group that your own rule group is copied from. You
* can use the metadata to track the version updates made to the originating rule group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withSourceMetadata(SourceMetadata sourceMetadata) {
setSourceMetadata(sourceMetadata);
return this;
}
/**
*
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to record changes
* to the managed rule group. You can subscribe to the SNS topic to receive notifications when the managed rule
* group is modified, such as for new versions and for version expiration. For more information, see the Amazon Simple Notification Service Developer
* Guide..
*
*
* @param snsTopic
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to record
* changes to the managed rule group. You can subscribe to the SNS topic to receive notifications when the
* managed rule group is modified, such as for new versions and for version expiration. For more information,
* see the Amazon Simple Notification
* Service Developer Guide..
*/
public void setSnsTopic(String snsTopic) {
this.snsTopic = snsTopic;
}
/**
*
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to record changes
* to the managed rule group. You can subscribe to the SNS topic to receive notifications when the managed rule
* group is modified, such as for new versions and for version expiration. For more information, see the Amazon Simple Notification Service Developer
* Guide..
*
*
* @return The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to record
* changes to the managed rule group. You can subscribe to the SNS topic to receive notifications when the
* managed rule group is modified, such as for new versions and for version expiration. For more
* information, see the Amazon Simple
* Notification Service Developer Guide..
*/
public String getSnsTopic() {
return this.snsTopic;
}
/**
*
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to record changes
* to the managed rule group. You can subscribe to the SNS topic to receive notifications when the managed rule
* group is modified, such as for new versions and for version expiration. For more information, see the Amazon Simple Notification Service Developer
* Guide..
*
*
* @param snsTopic
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to record
* changes to the managed rule group. You can subscribe to the SNS topic to receive notifications when the
* managed rule group is modified, such as for new versions and for version expiration. For more information,
* see the Amazon Simple Notification
* Service Developer Guide..
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withSnsTopic(String snsTopic) {
setSnsTopic(snsTopic);
return this;
}
/**
*
* The last time that the rule group was changed.
*
*
* @param lastModifiedTime
* The last time that the rule group was changed.
*/
public void setLastModifiedTime(java.util.Date lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
/**
*
* The last time that the rule group was changed.
*
*
* @return The last time that the rule group was changed.
*/
public java.util.Date getLastModifiedTime() {
return this.lastModifiedTime;
}
/**
*
* The last time that the rule group was changed.
*
*
* @param lastModifiedTime
* The last time that the rule group was changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withLastModifiedTime(java.util.Date lastModifiedTime) {
setLastModifiedTime(lastModifiedTime);
return this;
}
/**
*
* The list of analysis results for AnalyzeRuleGroup
. If you set AnalyzeRuleGroup
to
* TRUE
in CreateRuleGroup, UpdateRuleGroup, or DescribeRuleGroup, Network
* Firewall analyzes the rule group and identifies the rules that might adversely effect your firewall's
* functionality. For example, if Network Firewall detects a rule that's routing traffic asymmetrically, which
* impacts the service's ability to properly process traffic, the service includes the rule in the list of analysis
* results.
*
*
* @return The list of analysis results for AnalyzeRuleGroup
. If you set AnalyzeRuleGroup
* to TRUE
in CreateRuleGroup, UpdateRuleGroup, or DescribeRuleGroup,
* Network Firewall analyzes the rule group and identifies the rules that might adversely effect your
* firewall's functionality. For example, if Network Firewall detects a rule that's routing traffic
* asymmetrically, which impacts the service's ability to properly process traffic, the service includes the
* rule in the list of analysis results.
*/
public java.util.List getAnalysisResults() {
return analysisResults;
}
/**
*
* The list of analysis results for AnalyzeRuleGroup
. If you set AnalyzeRuleGroup
to
* TRUE
in CreateRuleGroup, UpdateRuleGroup, or DescribeRuleGroup, Network
* Firewall analyzes the rule group and identifies the rules that might adversely effect your firewall's
* functionality. For example, if Network Firewall detects a rule that's routing traffic asymmetrically, which
* impacts the service's ability to properly process traffic, the service includes the rule in the list of analysis
* results.
*
*
* @param analysisResults
* The list of analysis results for AnalyzeRuleGroup
. If you set AnalyzeRuleGroup
* to TRUE
in CreateRuleGroup, UpdateRuleGroup, or DescribeRuleGroup,
* Network Firewall analyzes the rule group and identifies the rules that might adversely effect your
* firewall's functionality. For example, if Network Firewall detects a rule that's routing traffic
* asymmetrically, which impacts the service's ability to properly process traffic, the service includes the
* rule in the list of analysis results.
*/
public void setAnalysisResults(java.util.Collection analysisResults) {
if (analysisResults == null) {
this.analysisResults = null;
return;
}
this.analysisResults = new java.util.ArrayList(analysisResults);
}
/**
*
* The list of analysis results for AnalyzeRuleGroup
. If you set AnalyzeRuleGroup
to
* TRUE
in CreateRuleGroup, UpdateRuleGroup, or DescribeRuleGroup, Network
* Firewall analyzes the rule group and identifies the rules that might adversely effect your firewall's
* functionality. For example, if Network Firewall detects a rule that's routing traffic asymmetrically, which
* impacts the service's ability to properly process traffic, the service includes the rule in the list of analysis
* results.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAnalysisResults(java.util.Collection)} or {@link #withAnalysisResults(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param analysisResults
* The list of analysis results for AnalyzeRuleGroup
. If you set AnalyzeRuleGroup
* to TRUE
in CreateRuleGroup, UpdateRuleGroup, or DescribeRuleGroup,
* Network Firewall analyzes the rule group and identifies the rules that might adversely effect your
* firewall's functionality. For example, if Network Firewall detects a rule that's routing traffic
* asymmetrically, which impacts the service's ability to properly process traffic, the service includes the
* rule in the list of analysis results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withAnalysisResults(AnalysisResult... analysisResults) {
if (this.analysisResults == null) {
setAnalysisResults(new java.util.ArrayList(analysisResults.length));
}
for (AnalysisResult ele : analysisResults) {
this.analysisResults.add(ele);
}
return this;
}
/**
*
* The list of analysis results for AnalyzeRuleGroup
. If you set AnalyzeRuleGroup
to
* TRUE
in CreateRuleGroup, UpdateRuleGroup, or DescribeRuleGroup, Network
* Firewall analyzes the rule group and identifies the rules that might adversely effect your firewall's
* functionality. For example, if Network Firewall detects a rule that's routing traffic asymmetrically, which
* impacts the service's ability to properly process traffic, the service includes the rule in the list of analysis
* results.
*
*
* @param analysisResults
* The list of analysis results for AnalyzeRuleGroup
. If you set AnalyzeRuleGroup
* to TRUE
in CreateRuleGroup, UpdateRuleGroup, or DescribeRuleGroup,
* Network Firewall analyzes the rule group and identifies the rules that might adversely effect your
* firewall's functionality. For example, if Network Firewall detects a rule that's routing traffic
* asymmetrically, which impacts the service's ability to properly process traffic, the service includes the
* rule in the list of analysis results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleGroupResponse withAnalysisResults(java.util.Collection analysisResults) {
setAnalysisResults(analysisResults);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRuleGroupArn() != null)
sb.append("RuleGroupArn: ").append(getRuleGroupArn()).append(",");
if (getRuleGroupName() != null)
sb.append("RuleGroupName: ").append(getRuleGroupName()).append(",");
if (getRuleGroupId() != null)
sb.append("RuleGroupId: ").append(getRuleGroupId()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getCapacity() != null)
sb.append("Capacity: ").append(getCapacity()).append(",");
if (getRuleGroupStatus() != null)
sb.append("RuleGroupStatus: ").append(getRuleGroupStatus()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getConsumedCapacity() != null)
sb.append("ConsumedCapacity: ").append(getConsumedCapacity()).append(",");
if (getNumberOfAssociations() != null)
sb.append("NumberOfAssociations: ").append(getNumberOfAssociations()).append(",");
if (getEncryptionConfiguration() != null)
sb.append("EncryptionConfiguration: ").append(getEncryptionConfiguration()).append(",");
if (getSourceMetadata() != null)
sb.append("SourceMetadata: ").append(getSourceMetadata()).append(",");
if (getSnsTopic() != null)
sb.append("SnsTopic: ").append(getSnsTopic()).append(",");
if (getLastModifiedTime() != null)
sb.append("LastModifiedTime: ").append(getLastModifiedTime()).append(",");
if (getAnalysisResults() != null)
sb.append("AnalysisResults: ").append(getAnalysisResults());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RuleGroupResponse == false)
return false;
RuleGroupResponse other = (RuleGroupResponse) obj;
if (other.getRuleGroupArn() == null ^ this.getRuleGroupArn() == null)
return false;
if (other.getRuleGroupArn() != null && other.getRuleGroupArn().equals(this.getRuleGroupArn()) == false)
return false;
if (other.getRuleGroupName() == null ^ this.getRuleGroupName() == null)
return false;
if (other.getRuleGroupName() != null && other.getRuleGroupName().equals(this.getRuleGroupName()) == false)
return false;
if (other.getRuleGroupId() == null ^ this.getRuleGroupId() == null)
return false;
if (other.getRuleGroupId() != null && other.getRuleGroupId().equals(this.getRuleGroupId()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getCapacity() == null ^ this.getCapacity() == null)
return false;
if (other.getCapacity() != null && other.getCapacity().equals(this.getCapacity()) == false)
return false;
if (other.getRuleGroupStatus() == null ^ this.getRuleGroupStatus() == null)
return false;
if (other.getRuleGroupStatus() != null && other.getRuleGroupStatus().equals(this.getRuleGroupStatus()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getConsumedCapacity() == null ^ this.getConsumedCapacity() == null)
return false;
if (other.getConsumedCapacity() != null && other.getConsumedCapacity().equals(this.getConsumedCapacity()) == false)
return false;
if (other.getNumberOfAssociations() == null ^ this.getNumberOfAssociations() == null)
return false;
if (other.getNumberOfAssociations() != null && other.getNumberOfAssociations().equals(this.getNumberOfAssociations()) == false)
return false;
if (other.getEncryptionConfiguration() == null ^ this.getEncryptionConfiguration() == null)
return false;
if (other.getEncryptionConfiguration() != null && other.getEncryptionConfiguration().equals(this.getEncryptionConfiguration()) == false)
return false;
if (other.getSourceMetadata() == null ^ this.getSourceMetadata() == null)
return false;
if (other.getSourceMetadata() != null && other.getSourceMetadata().equals(this.getSourceMetadata()) == false)
return false;
if (other.getSnsTopic() == null ^ this.getSnsTopic() == null)
return false;
if (other.getSnsTopic() != null && other.getSnsTopic().equals(this.getSnsTopic()) == false)
return false;
if (other.getLastModifiedTime() == null ^ this.getLastModifiedTime() == null)
return false;
if (other.getLastModifiedTime() != null && other.getLastModifiedTime().equals(this.getLastModifiedTime()) == false)
return false;
if (other.getAnalysisResults() == null ^ this.getAnalysisResults() == null)
return false;
if (other.getAnalysisResults() != null && other.getAnalysisResults().equals(this.getAnalysisResults()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRuleGroupArn() == null) ? 0 : getRuleGroupArn().hashCode());
hashCode = prime * hashCode + ((getRuleGroupName() == null) ? 0 : getRuleGroupName().hashCode());
hashCode = prime * hashCode + ((getRuleGroupId() == null) ? 0 : getRuleGroupId().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getCapacity() == null) ? 0 : getCapacity().hashCode());
hashCode = prime * hashCode + ((getRuleGroupStatus() == null) ? 0 : getRuleGroupStatus().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getConsumedCapacity() == null) ? 0 : getConsumedCapacity().hashCode());
hashCode = prime * hashCode + ((getNumberOfAssociations() == null) ? 0 : getNumberOfAssociations().hashCode());
hashCode = prime * hashCode + ((getEncryptionConfiguration() == null) ? 0 : getEncryptionConfiguration().hashCode());
hashCode = prime * hashCode + ((getSourceMetadata() == null) ? 0 : getSourceMetadata().hashCode());
hashCode = prime * hashCode + ((getSnsTopic() == null) ? 0 : getSnsTopic().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTime() == null) ? 0 : getLastModifiedTime().hashCode());
hashCode = prime * hashCode + ((getAnalysisResults() == null) ? 0 : getAnalysisResults().hashCode());
return hashCode;
}
@Override
public RuleGroupResponse clone() {
try {
return (RuleGroupResponse) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.networkfirewall.model.transform.RuleGroupResponseMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}