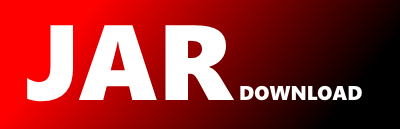
com.amazonaws.services.networkmanager.AWSNetworkManager Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkmanager Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkmanager;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.networkmanager.model.*;
/**
* Interface for accessing NetworkManager.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.networkmanager.AbstractAWSNetworkManager} instead.
*
*
*
* Amazon Web Services enables you to centrally manage your Amazon Web Services Cloud WAN core network and your Transit
* Gateway network across Amazon Web Services accounts, Regions, and on-premises locations.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSNetworkManager {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "networkmanager";
/**
*
* Accepts a core network attachment request.
*
*
* Once the attachment request is accepted by a core network owner, the attachment is created and connected to a
* core network.
*
*
* @param acceptAttachmentRequest
* @return Result of the AcceptAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AcceptAttachment
* @see AWS API Documentation
*/
AcceptAttachmentResult acceptAttachment(AcceptAttachmentRequest acceptAttachmentRequest);
/**
*
* Associates a core network Connect peer with a device and optionally, with a link.
*
*
* If you specify a link, it must be associated with the specified device. You can only associate core network
* Connect peers that have been created on a core network Connect attachment on a core network.
*
*
* @param associateConnectPeerRequest
* @return Result of the AssociateConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AssociateConnectPeer
* @see AWS API Documentation
*/
AssociateConnectPeerResult associateConnectPeer(AssociateConnectPeerRequest associateConnectPeerRequest);
/**
*
* Associates a customer gateway with a device and optionally, with a link. If you specify a link, it must be
* associated with the specified device.
*
*
* You can only associate customer gateways that are connected to a VPN attachment on a transit gateway or core
* network registered in your global network. When you register a transit gateway or core network, customer gateways
* that are connected to the transit gateway are automatically included in the global network. To list customer
* gateways that are connected to a transit gateway, use the DescribeVpnConnections EC2 API and filter by transit-gateway-id
.
*
*
* You cannot associate a customer gateway with more than one device and link.
*
*
* @param associateCustomerGatewayRequest
* @return Result of the AssociateCustomerGateway operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AssociateCustomerGateway
* @see AWS API Documentation
*/
AssociateCustomerGatewayResult associateCustomerGateway(AssociateCustomerGatewayRequest associateCustomerGatewayRequest);
/**
*
* Associates a link to a device. A device can be associated to multiple links and a link can be associated to
* multiple devices. The device and link must be in the same global network and the same site.
*
*
* @param associateLinkRequest
* @return Result of the AssociateLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AssociateLink
* @see AWS
* API Documentation
*/
AssociateLinkResult associateLink(AssociateLinkRequest associateLinkRequest);
/**
*
* Associates a transit gateway Connect peer with a device, and optionally, with a link. If you specify a link, it
* must be associated with the specified device.
*
*
* You can only associate transit gateway Connect peers that have been created on a transit gateway that's
* registered in your global network.
*
*
* You cannot associate a transit gateway Connect peer with more than one device and link.
*
*
* @param associateTransitGatewayConnectPeerRequest
* @return Result of the AssociateTransitGatewayConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AssociateTransitGatewayConnectPeer
* @see AWS API Documentation
*/
AssociateTransitGatewayConnectPeerResult associateTransitGatewayConnectPeer(
AssociateTransitGatewayConnectPeerRequest associateTransitGatewayConnectPeerRequest);
/**
*
* Creates a core network Connect attachment from a specified core network attachment.
*
*
* A core network Connect attachment is a GRE-based tunnel attachment that you can use to establish a connection
* between a core network and an appliance. A core network Connect attachment uses an existing VPC attachment as the
* underlying transport mechanism.
*
*
* @param createConnectAttachmentRequest
* @return Result of the CreateConnectAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateConnectAttachment
* @see AWS API Documentation
*/
CreateConnectAttachmentResult createConnectAttachment(CreateConnectAttachmentRequest createConnectAttachmentRequest);
/**
*
* Creates a core network Connect peer for a specified core network connect attachment between a core network and an
* appliance. The peer address and transit gateway address must be the same IP address family (IPv4 or IPv6).
*
*
* @param createConnectPeerRequest
* @return Result of the CreateConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateConnectPeer
* @see AWS API Documentation
*/
CreateConnectPeerResult createConnectPeer(CreateConnectPeerRequest createConnectPeerRequest);
/**
*
* Creates a connection between two devices. The devices can be a physical or virtual appliance that connects to a
* third-party appliance in a VPC, or a physical appliance that connects to another physical appliance in an
* on-premises network.
*
*
* @param createConnectionRequest
* @return Result of the CreateConnection operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateConnection
* @see AWS API Documentation
*/
CreateConnectionResult createConnection(CreateConnectionRequest createConnectionRequest);
/**
*
* Creates a core network as part of your global network, and optionally, with a core network policy.
*
*
* @param createCoreNetworkRequest
* @return Result of the CreateCoreNetwork operation returned by the service.
* @throws CoreNetworkPolicyException
* Describes a core network policy exception.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateCoreNetwork
* @see AWS API Documentation
*/
CreateCoreNetworkResult createCoreNetwork(CreateCoreNetworkRequest createCoreNetworkRequest);
/**
*
* Creates a new device in a global network. If you specify both a site ID and a location, the location of the site
* is used for visualization in the Network Manager console.
*
*
* @param createDeviceRequest
* @return Result of the CreateDevice operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateDevice
* @see AWS
* API Documentation
*/
CreateDeviceResult createDevice(CreateDeviceRequest createDeviceRequest);
/**
*
* Creates a new, empty global network.
*
*
* @param createGlobalNetworkRequest
* @return Result of the CreateGlobalNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateGlobalNetwork
* @see AWS API Documentation
*/
CreateGlobalNetworkResult createGlobalNetwork(CreateGlobalNetworkRequest createGlobalNetworkRequest);
/**
*
* Creates a new link for a specified site.
*
*
* @param createLinkRequest
* @return Result of the CreateLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateLink
* @see AWS API
* Documentation
*/
CreateLinkResult createLink(CreateLinkRequest createLinkRequest);
/**
*
* Creates a new site in a global network.
*
*
* @param createSiteRequest
* @return Result of the CreateSite operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateSite
* @see AWS API
* Documentation
*/
CreateSiteResult createSite(CreateSiteRequest createSiteRequest);
/**
*
* Creates an Amazon Web Services site-to-site VPN attachment on an edge location of a core network.
*
*
* @param createSiteToSiteVpnAttachmentRequest
* @return Result of the CreateSiteToSiteVpnAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateSiteToSiteVpnAttachment
* @see AWS API Documentation
*/
CreateSiteToSiteVpnAttachmentResult createSiteToSiteVpnAttachment(CreateSiteToSiteVpnAttachmentRequest createSiteToSiteVpnAttachmentRequest);
/**
*
* Creates a VPC attachment on an edge location of a core network.
*
*
* @param createVpcAttachmentRequest
* @return Result of the CreateVpcAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateVpcAttachment
* @see AWS API Documentation
*/
CreateVpcAttachmentResult createVpcAttachment(CreateVpcAttachmentRequest createVpcAttachmentRequest);
/**
*
* Deletes an attachment. Supports all attachment types.
*
*
* @param deleteAttachmentRequest
* @return Result of the DeleteAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteAttachment
* @see AWS API Documentation
*/
DeleteAttachmentResult deleteAttachment(DeleteAttachmentRequest deleteAttachmentRequest);
/**
*
* Deletes a Connect peer.
*
*
* @param deleteConnectPeerRequest
* @return Result of the DeleteConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteConnectPeer
* @see AWS API Documentation
*/
DeleteConnectPeerResult deleteConnectPeer(DeleteConnectPeerRequest deleteConnectPeerRequest);
/**
*
* Deletes the specified connection in your global network.
*
*
* @param deleteConnectionRequest
* @return Result of the DeleteConnection operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteConnection
* @see AWS API Documentation
*/
DeleteConnectionResult deleteConnection(DeleteConnectionRequest deleteConnectionRequest);
/**
*
* Deletes a core network along with all core network policies. This can only be done if there are no attachments on
* a core network.
*
*
* @param deleteCoreNetworkRequest
* @return Result of the DeleteCoreNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteCoreNetwork
* @see AWS API Documentation
*/
DeleteCoreNetworkResult deleteCoreNetwork(DeleteCoreNetworkRequest deleteCoreNetworkRequest);
/**
*
* Deletes a policy version from a core network. You can't delete the current LIVE policy.
*
*
* @param deleteCoreNetworkPolicyVersionRequest
* @return Result of the DeleteCoreNetworkPolicyVersion operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @sample AWSNetworkManager.DeleteCoreNetworkPolicyVersion
* @see AWS API Documentation
*/
DeleteCoreNetworkPolicyVersionResult deleteCoreNetworkPolicyVersion(DeleteCoreNetworkPolicyVersionRequest deleteCoreNetworkPolicyVersionRequest);
/**
*
* Deletes an existing device. You must first disassociate the device from any links and customer gateways.
*
*
* @param deleteDeviceRequest
* @return Result of the DeleteDevice operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteDevice
* @see AWS
* API Documentation
*/
DeleteDeviceResult deleteDevice(DeleteDeviceRequest deleteDeviceRequest);
/**
*
* Deletes an existing global network. You must first delete all global network objects (devices, links, and sites),
* deregister all transit gateways, and delete any core networks.
*
*
* @param deleteGlobalNetworkRequest
* @return Result of the DeleteGlobalNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteGlobalNetwork
* @see AWS API Documentation
*/
DeleteGlobalNetworkResult deleteGlobalNetwork(DeleteGlobalNetworkRequest deleteGlobalNetworkRequest);
/**
*
* Deletes an existing link. You must first disassociate the link from any devices and customer gateways.
*
*
* @param deleteLinkRequest
* @return Result of the DeleteLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteLink
* @see AWS API
* Documentation
*/
DeleteLinkResult deleteLink(DeleteLinkRequest deleteLinkRequest);
/**
*
* Deletes a resource policy for the specified resource. This revokes the access of the principals specified in the
* resource policy.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteResourcePolicy
* @see AWS API Documentation
*/
DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Deletes an existing site. The site cannot be associated with any device or link.
*
*
* @param deleteSiteRequest
* @return Result of the DeleteSite operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteSite
* @see AWS API
* Documentation
*/
DeleteSiteResult deleteSite(DeleteSiteRequest deleteSiteRequest);
/**
*
* Deregisters a transit gateway from your global network. This action does not delete your transit gateway, or
* modify any of its attachments. This action removes any customer gateway associations.
*
*
* @param deregisterTransitGatewayRequest
* @return Result of the DeregisterTransitGateway operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeregisterTransitGateway
* @see AWS API Documentation
*/
DeregisterTransitGatewayResult deregisterTransitGateway(DeregisterTransitGatewayRequest deregisterTransitGatewayRequest);
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
* @param describeGlobalNetworksRequest
* @return Result of the DescribeGlobalNetworks operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DescribeGlobalNetworks
* @see AWS API Documentation
*/
DescribeGlobalNetworksResult describeGlobalNetworks(DescribeGlobalNetworksRequest describeGlobalNetworksRequest);
/**
*
* Disassociates a core network Connect peer from a device and a link.
*
*
* @param disassociateConnectPeerRequest
* @return Result of the DisassociateConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DisassociateConnectPeer
* @see AWS API Documentation
*/
DisassociateConnectPeerResult disassociateConnectPeer(DisassociateConnectPeerRequest disassociateConnectPeerRequest);
/**
*
* Disassociates a customer gateway from a device and a link.
*
*
* @param disassociateCustomerGatewayRequest
* @return Result of the DisassociateCustomerGateway operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DisassociateCustomerGateway
* @see AWS API Documentation
*/
DisassociateCustomerGatewayResult disassociateCustomerGateway(DisassociateCustomerGatewayRequest disassociateCustomerGatewayRequest);
/**
*
* Disassociates an existing device from a link. You must first disassociate any customer gateways that are
* associated with the link.
*
*
* @param disassociateLinkRequest
* @return Result of the DisassociateLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DisassociateLink
* @see AWS API Documentation
*/
DisassociateLinkResult disassociateLink(DisassociateLinkRequest disassociateLinkRequest);
/**
*
* Disassociates a transit gateway Connect peer from a device and link.
*
*
* @param disassociateTransitGatewayConnectPeerRequest
* @return Result of the DisassociateTransitGatewayConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DisassociateTransitGatewayConnectPeer
* @see AWS API Documentation
*/
DisassociateTransitGatewayConnectPeerResult disassociateTransitGatewayConnectPeer(
DisassociateTransitGatewayConnectPeerRequest disassociateTransitGatewayConnectPeerRequest);
/**
*
* Executes a change set on your core network. Deploys changes globally based on the policy submitted..
*
*
* @param executeCoreNetworkChangeSetRequest
* @return Result of the ExecuteCoreNetworkChangeSet operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @sample AWSNetworkManager.ExecuteCoreNetworkChangeSet
* @see AWS API Documentation
*/
ExecuteCoreNetworkChangeSetResult executeCoreNetworkChangeSet(ExecuteCoreNetworkChangeSetRequest executeCoreNetworkChangeSetRequest);
/**
*
* Returns information about a core network Connect attachment.
*
*
* @param getConnectAttachmentRequest
* @return Result of the GetConnectAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetConnectAttachment
* @see AWS API Documentation
*/
GetConnectAttachmentResult getConnectAttachment(GetConnectAttachmentRequest getConnectAttachmentRequest);
/**
*
* Returns information about a core network Connect peer.
*
*
* @param getConnectPeerRequest
* @return Result of the GetConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetConnectPeer
* @see AWS
* API Documentation
*/
GetConnectPeerResult getConnectPeer(GetConnectPeerRequest getConnectPeerRequest);
/**
*
* Returns information about a core network Connect peer associations.
*
*
* @param getConnectPeerAssociationsRequest
* @return Result of the GetConnectPeerAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetConnectPeerAssociations
* @see AWS API Documentation
*/
GetConnectPeerAssociationsResult getConnectPeerAssociations(GetConnectPeerAssociationsRequest getConnectPeerAssociationsRequest);
/**
*
* Gets information about one or more of your connections in a global network.
*
*
* @param getConnectionsRequest
* @return Result of the GetConnections operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetConnections
* @see AWS
* API Documentation
*/
GetConnectionsResult getConnections(GetConnectionsRequest getConnectionsRequest);
/**
*
* Returns information about the LIVE policy for a core network.
*
*
* @param getCoreNetworkRequest
* @return Result of the GetCoreNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetCoreNetwork
* @see AWS
* API Documentation
*/
GetCoreNetworkResult getCoreNetwork(GetCoreNetworkRequest getCoreNetworkRequest);
/**
*
* Returns a change set between the LIVE core network policy and a submitted policy.
*
*
* @param getCoreNetworkChangeSetRequest
* @return Result of the GetCoreNetworkChangeSet operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetCoreNetworkChangeSet
* @see AWS API Documentation
*/
GetCoreNetworkChangeSetResult getCoreNetworkChangeSet(GetCoreNetworkChangeSetRequest getCoreNetworkChangeSetRequest);
/**
*
* Gets details about a core network policy. You can get details about your current live policy or any previous
* policy version.
*
*
* @param getCoreNetworkPolicyRequest
* @return Result of the GetCoreNetworkPolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetCoreNetworkPolicy
* @see AWS API Documentation
*/
GetCoreNetworkPolicyResult getCoreNetworkPolicy(GetCoreNetworkPolicyRequest getCoreNetworkPolicyRequest);
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
* @param getCustomerGatewayAssociationsRequest
* @return Result of the GetCustomerGatewayAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
GetCustomerGatewayAssociationsResult getCustomerGatewayAssociations(GetCustomerGatewayAssociationsRequest getCustomerGatewayAssociationsRequest);
/**
*
* Gets information about one or more of your devices in a global network.
*
*
* @param getDevicesRequest
* @return Result of the GetDevices operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetDevices
* @see AWS API
* Documentation
*/
GetDevicesResult getDevices(GetDevicesRequest getDevicesRequest);
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
* @param getLinkAssociationsRequest
* @return Result of the GetLinkAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetLinkAssociations
* @see AWS API Documentation
*/
GetLinkAssociationsResult getLinkAssociations(GetLinkAssociationsRequest getLinkAssociationsRequest);
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
* @param getLinksRequest
* @return Result of the GetLinks operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetLinks
* @see AWS API
* Documentation
*/
GetLinksResult getLinks(GetLinksRequest getLinksRequest);
/**
*
* Gets the count of network resources, by resource type, for the specified global network.
*
*
* @param getNetworkResourceCountsRequest
* @return Result of the GetNetworkResourceCounts operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkResourceCounts
* @see AWS API Documentation
*/
GetNetworkResourceCountsResult getNetworkResourceCounts(GetNetworkResourceCountsRequest getNetworkResourceCountsRequest);
/**
*
* Gets the network resource relationships for the specified global network.
*
*
* @param getNetworkResourceRelationshipsRequest
* @return Result of the GetNetworkResourceRelationships operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkResourceRelationships
* @see AWS API Documentation
*/
GetNetworkResourceRelationshipsResult getNetworkResourceRelationships(GetNetworkResourceRelationshipsRequest getNetworkResourceRelationshipsRequest);
/**
*
* Describes the network resources for the specified global network.
*
*
* The results include information from the corresponding Describe call for the resource, minus any sensitive
* information such as pre-shared keys.
*
*
* @param getNetworkResourcesRequest
* @return Result of the GetNetworkResources operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkResources
* @see AWS API Documentation
*/
GetNetworkResourcesResult getNetworkResources(GetNetworkResourcesRequest getNetworkResourcesRequest);
/**
*
* Gets the network routes of the specified global network.
*
*
* @param getNetworkRoutesRequest
* @return Result of the GetNetworkRoutes operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkRoutes
* @see AWS API Documentation
*/
GetNetworkRoutesResult getNetworkRoutes(GetNetworkRoutesRequest getNetworkRoutesRequest);
/**
*
* Gets the network telemetry of the specified global network.
*
*
* @param getNetworkTelemetryRequest
* @return Result of the GetNetworkTelemetry operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkTelemetry
* @see AWS API Documentation
*/
GetNetworkTelemetryResult getNetworkTelemetry(GetNetworkTelemetryRequest getNetworkTelemetryRequest);
/**
*
* Returns information about a resource policy.
*
*
* @param getResourcePolicyRequest
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetResourcePolicy
* @see AWS API Documentation
*/
GetResourcePolicyResult getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest);
/**
*
* Gets information about the specified route analysis.
*
*
* @param getRouteAnalysisRequest
* @return Result of the GetRouteAnalysis operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetRouteAnalysis
* @see AWS API Documentation
*/
GetRouteAnalysisResult getRouteAnalysis(GetRouteAnalysisRequest getRouteAnalysisRequest);
/**
*
* Returns information about a site-to-site VPN attachment.
*
*
* @param getSiteToSiteVpnAttachmentRequest
* @return Result of the GetSiteToSiteVpnAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetSiteToSiteVpnAttachment
* @see AWS API Documentation
*/
GetSiteToSiteVpnAttachmentResult getSiteToSiteVpnAttachment(GetSiteToSiteVpnAttachmentRequest getSiteToSiteVpnAttachmentRequest);
/**
*
* Gets information about one or more of your sites in a global network.
*
*
* @param getSitesRequest
* @return Result of the GetSites operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetSites
* @see AWS API
* Documentation
*/
GetSitesResult getSites(GetSitesRequest getSitesRequest);
/**
*
* Gets information about one or more of your transit gateway Connect peer associations in a global network.
*
*
* @param getTransitGatewayConnectPeerAssociationsRequest
* @return Result of the GetTransitGatewayConnectPeerAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetTransitGatewayConnectPeerAssociations
* @see AWS API Documentation
*/
GetTransitGatewayConnectPeerAssociationsResult getTransitGatewayConnectPeerAssociations(
GetTransitGatewayConnectPeerAssociationsRequest getTransitGatewayConnectPeerAssociationsRequest);
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
* @param getTransitGatewayRegistrationsRequest
* @return Result of the GetTransitGatewayRegistrations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
GetTransitGatewayRegistrationsResult getTransitGatewayRegistrations(GetTransitGatewayRegistrationsRequest getTransitGatewayRegistrationsRequest);
/**
*
* Returns information about a VPC attachment.
*
*
* @param getVpcAttachmentRequest
* @return Result of the GetVpcAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetVpcAttachment
* @see AWS API Documentation
*/
GetVpcAttachmentResult getVpcAttachment(GetVpcAttachmentRequest getVpcAttachmentRequest);
/**
*
* Returns a list of core network attachments.
*
*
* @param listAttachmentsRequest
* @return Result of the ListAttachments operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListAttachments
* @see AWS
* API Documentation
*/
ListAttachmentsResult listAttachments(ListAttachmentsRequest listAttachmentsRequest);
/**
*
* Returns a list of core network Connect peers.
*
*
* @param listConnectPeersRequest
* @return Result of the ListConnectPeers operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListConnectPeers
* @see AWS API Documentation
*/
ListConnectPeersResult listConnectPeers(ListConnectPeersRequest listConnectPeersRequest);
/**
*
* Returns a list of core network policy versions.
*
*
* @param listCoreNetworkPolicyVersionsRequest
* @return Result of the ListCoreNetworkPolicyVersions operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListCoreNetworkPolicyVersions
* @see AWS API Documentation
*/
ListCoreNetworkPolicyVersionsResult listCoreNetworkPolicyVersions(ListCoreNetworkPolicyVersionsRequest listCoreNetworkPolicyVersionsRequest);
/**
*
* Returns a list of owned and shared core networks.
*
*
* @param listCoreNetworksRequest
* @return Result of the ListCoreNetworks operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListCoreNetworks
* @see AWS API Documentation
*/
ListCoreNetworksResult listCoreNetworks(ListCoreNetworksRequest listCoreNetworksRequest);
/**
* @param listOrganizationServiceAccessStatusRequest
* @return Result of the ListOrganizationServiceAccessStatus operation returned by the service.
* @sample AWSNetworkManager.ListOrganizationServiceAccessStatus
* @see AWS API Documentation
*/
ListOrganizationServiceAccessStatusResult listOrganizationServiceAccessStatus(
ListOrganizationServiceAccessStatusRequest listOrganizationServiceAccessStatusRequest);
/**
*
* Lists the tags for a specified resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Creates a new, immutable version of a core network policy. A subsequent change set is created showing the
* differences between the LIVE policy and the submitted policy.
*
*
* @param putCoreNetworkPolicyRequest
* @return Result of the PutCoreNetworkPolicy operation returned by the service.
* @throws CoreNetworkPolicyException
* Describes a core network policy exception.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @sample AWSNetworkManager.PutCoreNetworkPolicy
* @see AWS API Documentation
*/
PutCoreNetworkPolicyResult putCoreNetworkPolicy(PutCoreNetworkPolicyRequest putCoreNetworkPolicyRequest);
/**
*
* Creates or updates a resource policy.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.PutResourcePolicy
* @see AWS API Documentation
*/
PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest);
/**
*
* Registers a transit gateway in your global network. The transit gateway can be in any Amazon Web Services Region,
* but it must be owned by the same Amazon Web Services account that owns the global network. You cannot register a
* transit gateway in more than one global network.
*
*
* @param registerTransitGatewayRequest
* @return Result of the RegisterTransitGateway operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.RegisterTransitGateway
* @see AWS API Documentation
*/
RegisterTransitGatewayResult registerTransitGateway(RegisterTransitGatewayRequest registerTransitGatewayRequest);
/**
*
* Rejects a core network attachment request.
*
*
* @param rejectAttachmentRequest
* @return Result of the RejectAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.RejectAttachment
* @see AWS API Documentation
*/
RejectAttachmentResult rejectAttachment(RejectAttachmentRequest rejectAttachmentRequest);
/**
*
* Restores a previous policy version as a new, immutable version of a core network policy. A subsequent change set
* is created showing the differences between the LIVE policy and restored policy.
*
*
* @param restoreCoreNetworkPolicyVersionRequest
* @return Result of the RestoreCoreNetworkPolicyVersion operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @sample AWSNetworkManager.RestoreCoreNetworkPolicyVersion
* @see AWS API Documentation
*/
RestoreCoreNetworkPolicyVersionResult restoreCoreNetworkPolicyVersion(RestoreCoreNetworkPolicyVersionRequest restoreCoreNetworkPolicyVersionRequest);
/**
* @param startOrganizationServiceAccessUpdateRequest
* @return Result of the StartOrganizationServiceAccessUpdate operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.StartOrganizationServiceAccessUpdate
* @see AWS API Documentation
*/
StartOrganizationServiceAccessUpdateResult startOrganizationServiceAccessUpdate(
StartOrganizationServiceAccessUpdateRequest startOrganizationServiceAccessUpdateRequest);
/**
*
* Starts analyzing the routing path between the specified source and destination. For more information, see Route Analyzer.
*
*
* @param startRouteAnalysisRequest
* @return Result of the StartRouteAnalysis operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.StartRouteAnalysis
* @see AWS API Documentation
*/
StartRouteAnalysisResult startRouteAnalysis(StartRouteAnalysisRequest startRouteAnalysisRequest);
/**
*
* Tags a specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from a specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the information for an existing connection. To remove information for any of the parameters, specify an
* empty string.
*
*
* @param updateConnectionRequest
* @return Result of the UpdateConnection operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateConnection
* @see AWS API Documentation
*/
UpdateConnectionResult updateConnection(UpdateConnectionRequest updateConnectionRequest);
/**
*
* Updates the description of a core network.
*
*
* @param updateCoreNetworkRequest
* @return Result of the UpdateCoreNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateCoreNetwork
* @see AWS API Documentation
*/
UpdateCoreNetworkResult updateCoreNetwork(UpdateCoreNetworkRequest updateCoreNetworkRequest);
/**
*
* Updates the details for an existing device. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateDeviceRequest
* @return Result of the UpdateDevice operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateDevice
* @see AWS
* API Documentation
*/
UpdateDeviceResult updateDevice(UpdateDeviceRequest updateDeviceRequest);
/**
*
* Updates an existing global network. To remove information for any of the parameters, specify an empty string.
*
*
* @param updateGlobalNetworkRequest
* @return Result of the UpdateGlobalNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateGlobalNetwork
* @see AWS API Documentation
*/
UpdateGlobalNetworkResult updateGlobalNetwork(UpdateGlobalNetworkRequest updateGlobalNetworkRequest);
/**
*
* Updates the details for an existing link. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateLinkRequest
* @return Result of the UpdateLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateLink
* @see AWS API
* Documentation
*/
UpdateLinkResult updateLink(UpdateLinkRequest updateLinkRequest);
/**
*
* Updates the resource metadata for the specified global network.
*
*
* @param updateNetworkResourceMetadataRequest
* @return Result of the UpdateNetworkResourceMetadata operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateNetworkResourceMetadata
* @see AWS API Documentation
*/
UpdateNetworkResourceMetadataResult updateNetworkResourceMetadata(UpdateNetworkResourceMetadataRequest updateNetworkResourceMetadataRequest);
/**
*
* Updates the information for an existing site. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateSiteRequest
* @return Result of the UpdateSite operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateSite
* @see AWS API
* Documentation
*/
UpdateSiteResult updateSite(UpdateSiteRequest updateSiteRequest);
/**
*
* Updates a VPC attachment.
*
*
* @param updateVpcAttachmentRequest
* @return Result of the UpdateVpcAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateVpcAttachment
* @see AWS API Documentation
*/
UpdateVpcAttachmentResult updateVpcAttachment(UpdateVpcAttachmentRequest updateVpcAttachmentRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}