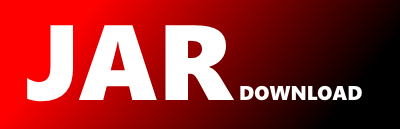
com.amazonaws.services.networkmanager.AWSNetworkManagerClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkmanager Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkmanager;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.networkmanager.AWSNetworkManagerClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.networkmanager.model.*;
import com.amazonaws.services.networkmanager.model.transform.*;
/**
* Client for accessing NetworkManager. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
*
* Amazon Web Services enables you to centrally manage your Amazon Web Services Cloud WAN core network and your Transit
* Gateway network across Amazon Web Services accounts, Regions, and on-premises locations.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSNetworkManagerClient extends AmazonWebServiceClient implements AWSNetworkManager {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSNetworkManager.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "networkmanager";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.networkmanager.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.networkmanager.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.networkmanager.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.networkmanager.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.networkmanager.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.networkmanager.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.networkmanager.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CoreNetworkPolicyException").withExceptionUnmarshaller(
com.amazonaws.services.networkmanager.model.transform.CoreNetworkPolicyExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.networkmanager.model.AWSNetworkManagerException.class));
public static AWSNetworkManagerClientBuilder builder() {
return AWSNetworkManagerClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on NetworkManager using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSNetworkManagerClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on NetworkManager using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSNetworkManagerClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("networkmanager.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/networkmanager/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/networkmanager/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Accepts a core network attachment request.
*
*
* Once the attachment request is accepted by a core network owner, the attachment is created and connected to a
* core network.
*
*
* @param acceptAttachmentRequest
* @return Result of the AcceptAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AcceptAttachment
* @see AWS API Documentation
*/
@Override
public AcceptAttachmentResult acceptAttachment(AcceptAttachmentRequest request) {
request = beforeClientExecution(request);
return executeAcceptAttachment(request);
}
@SdkInternalApi
final AcceptAttachmentResult executeAcceptAttachment(AcceptAttachmentRequest acceptAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(acceptAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AcceptAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(acceptAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AcceptAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AcceptAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a core network Connect peer with a device and optionally, with a link.
*
*
* If you specify a link, it must be associated with the specified device. You can only associate core network
* Connect peers that have been created on a core network Connect attachment on a core network.
*
*
* @param associateConnectPeerRequest
* @return Result of the AssociateConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AssociateConnectPeer
* @see AWS API Documentation
*/
@Override
public AssociateConnectPeerResult associateConnectPeer(AssociateConnectPeerRequest request) {
request = beforeClientExecution(request);
return executeAssociateConnectPeer(request);
}
@SdkInternalApi
final AssociateConnectPeerResult executeAssociateConnectPeer(AssociateConnectPeerRequest associateConnectPeerRequest) {
ExecutionContext executionContext = createExecutionContext(associateConnectPeerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateConnectPeerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associateConnectPeerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateConnectPeer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AssociateConnectPeerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a customer gateway with a device and optionally, with a link. If you specify a link, it must be
* associated with the specified device.
*
*
* You can only associate customer gateways that are connected to a VPN attachment on a transit gateway or core
* network registered in your global network. When you register a transit gateway or core network, customer gateways
* that are connected to the transit gateway are automatically included in the global network. To list customer
* gateways that are connected to a transit gateway, use the DescribeVpnConnections EC2 API and filter by transit-gateway-id
.
*
*
* You cannot associate a customer gateway with more than one device and link.
*
*
* @param associateCustomerGatewayRequest
* @return Result of the AssociateCustomerGateway operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AssociateCustomerGateway
* @see AWS API Documentation
*/
@Override
public AssociateCustomerGatewayResult associateCustomerGateway(AssociateCustomerGatewayRequest request) {
request = beforeClientExecution(request);
return executeAssociateCustomerGateway(request);
}
@SdkInternalApi
final AssociateCustomerGatewayResult executeAssociateCustomerGateway(AssociateCustomerGatewayRequest associateCustomerGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(associateCustomerGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateCustomerGatewayRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateCustomerGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateCustomerGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateCustomerGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a link to a device. A device can be associated to multiple links and a link can be associated to
* multiple devices. The device and link must be in the same global network and the same site.
*
*
* @param associateLinkRequest
* @return Result of the AssociateLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AssociateLink
* @see AWS
* API Documentation
*/
@Override
public AssociateLinkResult associateLink(AssociateLinkRequest request) {
request = beforeClientExecution(request);
return executeAssociateLink(request);
}
@SdkInternalApi
final AssociateLinkResult executeAssociateLink(AssociateLinkRequest associateLinkRequest) {
ExecutionContext executionContext = createExecutionContext(associateLinkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateLinkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associateLinkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateLink");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AssociateLinkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a transit gateway Connect peer with a device, and optionally, with a link. If you specify a link, it
* must be associated with the specified device.
*
*
* You can only associate transit gateway Connect peers that have been created on a transit gateway that's
* registered in your global network.
*
*
* You cannot associate a transit gateway Connect peer with more than one device and link.
*
*
* @param associateTransitGatewayConnectPeerRequest
* @return Result of the AssociateTransitGatewayConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.AssociateTransitGatewayConnectPeer
* @see AWS API Documentation
*/
@Override
public AssociateTransitGatewayConnectPeerResult associateTransitGatewayConnectPeer(AssociateTransitGatewayConnectPeerRequest request) {
request = beforeClientExecution(request);
return executeAssociateTransitGatewayConnectPeer(request);
}
@SdkInternalApi
final AssociateTransitGatewayConnectPeerResult executeAssociateTransitGatewayConnectPeer(
AssociateTransitGatewayConnectPeerRequest associateTransitGatewayConnectPeerRequest) {
ExecutionContext executionContext = createExecutionContext(associateTransitGatewayConnectPeerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateTransitGatewayConnectPeerRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateTransitGatewayConnectPeerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateTransitGatewayConnectPeer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateTransitGatewayConnectPeerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a core network Connect attachment from a specified core network attachment.
*
*
* A core network Connect attachment is a GRE-based tunnel attachment that you can use to establish a connection
* between a core network and an appliance. A core network Connect attachment uses an existing VPC attachment as the
* underlying transport mechanism.
*
*
* @param createConnectAttachmentRequest
* @return Result of the CreateConnectAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateConnectAttachment
* @see AWS API Documentation
*/
@Override
public CreateConnectAttachmentResult createConnectAttachment(CreateConnectAttachmentRequest request) {
request = beforeClientExecution(request);
return executeCreateConnectAttachment(request);
}
@SdkInternalApi
final CreateConnectAttachmentResult executeCreateConnectAttachment(CreateConnectAttachmentRequest createConnectAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(createConnectAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConnectAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createConnectAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateConnectAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateConnectAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a core network Connect peer for a specified core network connect attachment between a core network and an
* appliance. The peer address and transit gateway address must be the same IP address family (IPv4 or IPv6).
*
*
* @param createConnectPeerRequest
* @return Result of the CreateConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateConnectPeer
* @see AWS API Documentation
*/
@Override
public CreateConnectPeerResult createConnectPeer(CreateConnectPeerRequest request) {
request = beforeClientExecution(request);
return executeCreateConnectPeer(request);
}
@SdkInternalApi
final CreateConnectPeerResult executeCreateConnectPeer(CreateConnectPeerRequest createConnectPeerRequest) {
ExecutionContext executionContext = createExecutionContext(createConnectPeerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConnectPeerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createConnectPeerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateConnectPeer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateConnectPeerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a connection between two devices. The devices can be a physical or virtual appliance that connects to a
* third-party appliance in a VPC, or a physical appliance that connects to another physical appliance in an
* on-premises network.
*
*
* @param createConnectionRequest
* @return Result of the CreateConnection operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateConnection
* @see AWS API Documentation
*/
@Override
public CreateConnectionResult createConnection(CreateConnectionRequest request) {
request = beforeClientExecution(request);
return executeCreateConnection(request);
}
@SdkInternalApi
final CreateConnectionResult executeCreateConnection(CreateConnectionRequest createConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(createConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConnectionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a core network as part of your global network, and optionally, with a core network policy.
*
*
* @param createCoreNetworkRequest
* @return Result of the CreateCoreNetwork operation returned by the service.
* @throws CoreNetworkPolicyException
* Describes a core network policy exception.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateCoreNetwork
* @see AWS API Documentation
*/
@Override
public CreateCoreNetworkResult createCoreNetwork(CreateCoreNetworkRequest request) {
request = beforeClientExecution(request);
return executeCreateCoreNetwork(request);
}
@SdkInternalApi
final CreateCoreNetworkResult executeCreateCoreNetwork(CreateCoreNetworkRequest createCoreNetworkRequest) {
ExecutionContext executionContext = createExecutionContext(createCoreNetworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCoreNetworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createCoreNetworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCoreNetwork");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateCoreNetworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new device in a global network. If you specify both a site ID and a location, the location of the site
* is used for visualization in the Network Manager console.
*
*
* @param createDeviceRequest
* @return Result of the CreateDevice operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateDevice
* @see AWS
* API Documentation
*/
@Override
public CreateDeviceResult createDevice(CreateDeviceRequest request) {
request = beforeClientExecution(request);
return executeCreateDevice(request);
}
@SdkInternalApi
final CreateDeviceResult executeCreateDevice(CreateDeviceRequest createDeviceRequest) {
ExecutionContext executionContext = createExecutionContext(createDeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDeviceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDevice");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDeviceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new, empty global network.
*
*
* @param createGlobalNetworkRequest
* @return Result of the CreateGlobalNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateGlobalNetwork
* @see AWS API Documentation
*/
@Override
public CreateGlobalNetworkResult createGlobalNetwork(CreateGlobalNetworkRequest request) {
request = beforeClientExecution(request);
return executeCreateGlobalNetwork(request);
}
@SdkInternalApi
final CreateGlobalNetworkResult executeCreateGlobalNetwork(CreateGlobalNetworkRequest createGlobalNetworkRequest) {
ExecutionContext executionContext = createExecutionContext(createGlobalNetworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateGlobalNetworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createGlobalNetworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateGlobalNetwork");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateGlobalNetworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new link for a specified site.
*
*
* @param createLinkRequest
* @return Result of the CreateLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateLink
* @see AWS API
* Documentation
*/
@Override
public CreateLinkResult createLink(CreateLinkRequest request) {
request = beforeClientExecution(request);
return executeCreateLink(request);
}
@SdkInternalApi
final CreateLinkResult executeCreateLink(CreateLinkRequest createLinkRequest) {
ExecutionContext executionContext = createExecutionContext(createLinkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLinkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createLinkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateLink");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateLinkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new site in a global network.
*
*
* @param createSiteRequest
* @return Result of the CreateSite operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateSite
* @see AWS API
* Documentation
*/
@Override
public CreateSiteResult createSite(CreateSiteRequest request) {
request = beforeClientExecution(request);
return executeCreateSite(request);
}
@SdkInternalApi
final CreateSiteResult executeCreateSite(CreateSiteRequest createSiteRequest) {
ExecutionContext executionContext = createExecutionContext(createSiteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSiteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSiteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSite");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSiteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Web Services site-to-site VPN attachment on an edge location of a core network.
*
*
* @param createSiteToSiteVpnAttachmentRequest
* @return Result of the CreateSiteToSiteVpnAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateSiteToSiteVpnAttachment
* @see AWS API Documentation
*/
@Override
public CreateSiteToSiteVpnAttachmentResult createSiteToSiteVpnAttachment(CreateSiteToSiteVpnAttachmentRequest request) {
request = beforeClientExecution(request);
return executeCreateSiteToSiteVpnAttachment(request);
}
@SdkInternalApi
final CreateSiteToSiteVpnAttachmentResult executeCreateSiteToSiteVpnAttachment(CreateSiteToSiteVpnAttachmentRequest createSiteToSiteVpnAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(createSiteToSiteVpnAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSiteToSiteVpnAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createSiteToSiteVpnAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSiteToSiteVpnAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateSiteToSiteVpnAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a transit gateway peering connection.
*
*
* @param createTransitGatewayPeeringRequest
* @return Result of the CreateTransitGatewayPeering operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateTransitGatewayPeering
* @see AWS API Documentation
*/
@Override
public CreateTransitGatewayPeeringResult createTransitGatewayPeering(CreateTransitGatewayPeeringRequest request) {
request = beforeClientExecution(request);
return executeCreateTransitGatewayPeering(request);
}
@SdkInternalApi
final CreateTransitGatewayPeeringResult executeCreateTransitGatewayPeering(CreateTransitGatewayPeeringRequest createTransitGatewayPeeringRequest) {
ExecutionContext executionContext = createExecutionContext(createTransitGatewayPeeringRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTransitGatewayPeeringRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createTransitGatewayPeeringRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateTransitGatewayPeering");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateTransitGatewayPeeringResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a transit gateway route table attachment.
*
*
* @param createTransitGatewayRouteTableAttachmentRequest
* @return Result of the CreateTransitGatewayRouteTableAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateTransitGatewayRouteTableAttachment
* @see AWS API Documentation
*/
@Override
public CreateTransitGatewayRouteTableAttachmentResult createTransitGatewayRouteTableAttachment(CreateTransitGatewayRouteTableAttachmentRequest request) {
request = beforeClientExecution(request);
return executeCreateTransitGatewayRouteTableAttachment(request);
}
@SdkInternalApi
final CreateTransitGatewayRouteTableAttachmentResult executeCreateTransitGatewayRouteTableAttachment(
CreateTransitGatewayRouteTableAttachmentRequest createTransitGatewayRouteTableAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(createTransitGatewayRouteTableAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTransitGatewayRouteTableAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createTransitGatewayRouteTableAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateTransitGatewayRouteTableAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateTransitGatewayRouteTableAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a VPC attachment on an edge location of a core network.
*
*
* @param createVpcAttachmentRequest
* @return Result of the CreateVpcAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.CreateVpcAttachment
* @see AWS API Documentation
*/
@Override
public CreateVpcAttachmentResult createVpcAttachment(CreateVpcAttachmentRequest request) {
request = beforeClientExecution(request);
return executeCreateVpcAttachment(request);
}
@SdkInternalApi
final CreateVpcAttachmentResult executeCreateVpcAttachment(CreateVpcAttachmentRequest createVpcAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(createVpcAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVpcAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVpcAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVpcAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVpcAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an attachment. Supports all attachment types.
*
*
* @param deleteAttachmentRequest
* @return Result of the DeleteAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteAttachment
* @see AWS API Documentation
*/
@Override
public DeleteAttachmentResult deleteAttachment(DeleteAttachmentRequest request) {
request = beforeClientExecution(request);
return executeDeleteAttachment(request);
}
@SdkInternalApi
final DeleteAttachmentResult executeDeleteAttachment(DeleteAttachmentRequest deleteAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Connect peer.
*
*
* @param deleteConnectPeerRequest
* @return Result of the DeleteConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteConnectPeer
* @see AWS API Documentation
*/
@Override
public DeleteConnectPeerResult deleteConnectPeer(DeleteConnectPeerRequest request) {
request = beforeClientExecution(request);
return executeDeleteConnectPeer(request);
}
@SdkInternalApi
final DeleteConnectPeerResult executeDeleteConnectPeer(DeleteConnectPeerRequest deleteConnectPeerRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConnectPeerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConnectPeerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteConnectPeerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteConnectPeer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteConnectPeerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified connection in your global network.
*
*
* @param deleteConnectionRequest
* @return Result of the DeleteConnection operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteConnection
* @see AWS API Documentation
*/
@Override
public DeleteConnectionResult deleteConnection(DeleteConnectionRequest request) {
request = beforeClientExecution(request);
return executeDeleteConnection(request);
}
@SdkInternalApi
final DeleteConnectionResult executeDeleteConnection(DeleteConnectionRequest deleteConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConnectionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a core network along with all core network policies. This can only be done if there are no attachments on
* a core network.
*
*
* @param deleteCoreNetworkRequest
* @return Result of the DeleteCoreNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteCoreNetwork
* @see AWS API Documentation
*/
@Override
public DeleteCoreNetworkResult deleteCoreNetwork(DeleteCoreNetworkRequest request) {
request = beforeClientExecution(request);
return executeDeleteCoreNetwork(request);
}
@SdkInternalApi
final DeleteCoreNetworkResult executeDeleteCoreNetwork(DeleteCoreNetworkRequest deleteCoreNetworkRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCoreNetworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCoreNetworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteCoreNetworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCoreNetwork");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteCoreNetworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a policy version from a core network. You can't delete the current LIVE policy.
*
*
* @param deleteCoreNetworkPolicyVersionRequest
* @return Result of the DeleteCoreNetworkPolicyVersion operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @sample AWSNetworkManager.DeleteCoreNetworkPolicyVersion
* @see AWS API Documentation
*/
@Override
public DeleteCoreNetworkPolicyVersionResult deleteCoreNetworkPolicyVersion(DeleteCoreNetworkPolicyVersionRequest request) {
request = beforeClientExecution(request);
return executeDeleteCoreNetworkPolicyVersion(request);
}
@SdkInternalApi
final DeleteCoreNetworkPolicyVersionResult executeDeleteCoreNetworkPolicyVersion(DeleteCoreNetworkPolicyVersionRequest deleteCoreNetworkPolicyVersionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCoreNetworkPolicyVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCoreNetworkPolicyVersionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteCoreNetworkPolicyVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCoreNetworkPolicyVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteCoreNetworkPolicyVersionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing device. You must first disassociate the device from any links and customer gateways.
*
*
* @param deleteDeviceRequest
* @return Result of the DeleteDevice operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteDevice
* @see AWS
* API Documentation
*/
@Override
public DeleteDeviceResult deleteDevice(DeleteDeviceRequest request) {
request = beforeClientExecution(request);
return executeDeleteDevice(request);
}
@SdkInternalApi
final DeleteDeviceResult executeDeleteDevice(DeleteDeviceRequest deleteDeviceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDeviceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDevice");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDeviceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing global network. You must first delete all global network objects (devices, links, and sites),
* deregister all transit gateways, and delete any core networks.
*
*
* @param deleteGlobalNetworkRequest
* @return Result of the DeleteGlobalNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteGlobalNetwork
* @see AWS API Documentation
*/
@Override
public DeleteGlobalNetworkResult deleteGlobalNetwork(DeleteGlobalNetworkRequest request) {
request = beforeClientExecution(request);
return executeDeleteGlobalNetwork(request);
}
@SdkInternalApi
final DeleteGlobalNetworkResult executeDeleteGlobalNetwork(DeleteGlobalNetworkRequest deleteGlobalNetworkRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGlobalNetworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteGlobalNetworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteGlobalNetworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteGlobalNetwork");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteGlobalNetworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing link. You must first disassociate the link from any devices and customer gateways.
*
*
* @param deleteLinkRequest
* @return Result of the DeleteLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteLink
* @see AWS API
* Documentation
*/
@Override
public DeleteLinkResult deleteLink(DeleteLinkRequest request) {
request = beforeClientExecution(request);
return executeDeleteLink(request);
}
@SdkInternalApi
final DeleteLinkResult executeDeleteLink(DeleteLinkRequest deleteLinkRequest) {
ExecutionContext executionContext = createExecutionContext(deleteLinkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteLinkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteLinkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteLink");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteLinkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing peering connection.
*
*
* @param deletePeeringRequest
* @return Result of the DeletePeering operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeletePeering
* @see AWS
* API Documentation
*/
@Override
public DeletePeeringResult deletePeering(DeletePeeringRequest request) {
request = beforeClientExecution(request);
return executeDeletePeering(request);
}
@SdkInternalApi
final DeletePeeringResult executeDeletePeering(DeletePeeringRequest deletePeeringRequest) {
ExecutionContext executionContext = createExecutionContext(deletePeeringRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePeeringRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deletePeeringRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePeering");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeletePeeringResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a resource policy for the specified resource. This revokes the access of the principals specified in the
* resource policy.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteResourcePolicy
* @see AWS API Documentation
*/
@Override
public DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteResourcePolicy(request);
}
@SdkInternalApi
final DeleteResourcePolicyResult executeDeleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing site. The site cannot be associated with any device or link.
*
*
* @param deleteSiteRequest
* @return Result of the DeleteSite operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeleteSite
* @see AWS API
* Documentation
*/
@Override
public DeleteSiteResult deleteSite(DeleteSiteRequest request) {
request = beforeClientExecution(request);
return executeDeleteSite(request);
}
@SdkInternalApi
final DeleteSiteResult executeDeleteSite(DeleteSiteRequest deleteSiteRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSiteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSiteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSiteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSite");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSiteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deregisters a transit gateway from your global network. This action does not delete your transit gateway, or
* modify any of its attachments. This action removes any customer gateway associations.
*
*
* @param deregisterTransitGatewayRequest
* @return Result of the DeregisterTransitGateway operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DeregisterTransitGateway
* @see AWS API Documentation
*/
@Override
public DeregisterTransitGatewayResult deregisterTransitGateway(DeregisterTransitGatewayRequest request) {
request = beforeClientExecution(request);
return executeDeregisterTransitGateway(request);
}
@SdkInternalApi
final DeregisterTransitGatewayResult executeDeregisterTransitGateway(DeregisterTransitGatewayRequest deregisterTransitGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterTransitGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterTransitGatewayRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deregisterTransitGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeregisterTransitGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeregisterTransitGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
* @param describeGlobalNetworksRequest
* @return Result of the DescribeGlobalNetworks operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DescribeGlobalNetworks
* @see AWS API Documentation
*/
@Override
public DescribeGlobalNetworksResult describeGlobalNetworks(DescribeGlobalNetworksRequest request) {
request = beforeClientExecution(request);
return executeDescribeGlobalNetworks(request);
}
@SdkInternalApi
final DescribeGlobalNetworksResult executeDescribeGlobalNetworks(DescribeGlobalNetworksRequest describeGlobalNetworksRequest) {
ExecutionContext executionContext = createExecutionContext(describeGlobalNetworksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeGlobalNetworksRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeGlobalNetworksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeGlobalNetworks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeGlobalNetworksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a core network Connect peer from a device and a link.
*
*
* @param disassociateConnectPeerRequest
* @return Result of the DisassociateConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DisassociateConnectPeer
* @see AWS API Documentation
*/
@Override
public DisassociateConnectPeerResult disassociateConnectPeer(DisassociateConnectPeerRequest request) {
request = beforeClientExecution(request);
return executeDisassociateConnectPeer(request);
}
@SdkInternalApi
final DisassociateConnectPeerResult executeDisassociateConnectPeer(DisassociateConnectPeerRequest disassociateConnectPeerRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateConnectPeerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateConnectPeerRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateConnectPeerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateConnectPeer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateConnectPeerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a customer gateway from a device and a link.
*
*
* @param disassociateCustomerGatewayRequest
* @return Result of the DisassociateCustomerGateway operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DisassociateCustomerGateway
* @see AWS API Documentation
*/
@Override
public DisassociateCustomerGatewayResult disassociateCustomerGateway(DisassociateCustomerGatewayRequest request) {
request = beforeClientExecution(request);
return executeDisassociateCustomerGateway(request);
}
@SdkInternalApi
final DisassociateCustomerGatewayResult executeDisassociateCustomerGateway(DisassociateCustomerGatewayRequest disassociateCustomerGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateCustomerGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateCustomerGatewayRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateCustomerGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateCustomerGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateCustomerGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates an existing device from a link. You must first disassociate any customer gateways that are
* associated with the link.
*
*
* @param disassociateLinkRequest
* @return Result of the DisassociateLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DisassociateLink
* @see AWS API Documentation
*/
@Override
public DisassociateLinkResult disassociateLink(DisassociateLinkRequest request) {
request = beforeClientExecution(request);
return executeDisassociateLink(request);
}
@SdkInternalApi
final DisassociateLinkResult executeDisassociateLink(DisassociateLinkRequest disassociateLinkRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateLinkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateLinkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disassociateLinkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateLink");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisassociateLinkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a transit gateway Connect peer from a device and link.
*
*
* @param disassociateTransitGatewayConnectPeerRequest
* @return Result of the DisassociateTransitGatewayConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.DisassociateTransitGatewayConnectPeer
* @see AWS API Documentation
*/
@Override
public DisassociateTransitGatewayConnectPeerResult disassociateTransitGatewayConnectPeer(DisassociateTransitGatewayConnectPeerRequest request) {
request = beforeClientExecution(request);
return executeDisassociateTransitGatewayConnectPeer(request);
}
@SdkInternalApi
final DisassociateTransitGatewayConnectPeerResult executeDisassociateTransitGatewayConnectPeer(
DisassociateTransitGatewayConnectPeerRequest disassociateTransitGatewayConnectPeerRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateTransitGatewayConnectPeerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateTransitGatewayConnectPeerRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateTransitGatewayConnectPeerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateTransitGatewayConnectPeer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateTransitGatewayConnectPeerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Executes a change set on your core network. Deploys changes globally based on the policy submitted..
*
*
* @param executeCoreNetworkChangeSetRequest
* @return Result of the ExecuteCoreNetworkChangeSet operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @sample AWSNetworkManager.ExecuteCoreNetworkChangeSet
* @see AWS API Documentation
*/
@Override
public ExecuteCoreNetworkChangeSetResult executeCoreNetworkChangeSet(ExecuteCoreNetworkChangeSetRequest request) {
request = beforeClientExecution(request);
return executeExecuteCoreNetworkChangeSet(request);
}
@SdkInternalApi
final ExecuteCoreNetworkChangeSetResult executeExecuteCoreNetworkChangeSet(ExecuteCoreNetworkChangeSetRequest executeCoreNetworkChangeSetRequest) {
ExecutionContext executionContext = createExecutionContext(executeCoreNetworkChangeSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ExecuteCoreNetworkChangeSetRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(executeCoreNetworkChangeSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ExecuteCoreNetworkChangeSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ExecuteCoreNetworkChangeSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a core network Connect attachment.
*
*
* @param getConnectAttachmentRequest
* @return Result of the GetConnectAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetConnectAttachment
* @see AWS API Documentation
*/
@Override
public GetConnectAttachmentResult getConnectAttachment(GetConnectAttachmentRequest request) {
request = beforeClientExecution(request);
return executeGetConnectAttachment(request);
}
@SdkInternalApi
final GetConnectAttachmentResult executeGetConnectAttachment(GetConnectAttachmentRequest getConnectAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(getConnectAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetConnectAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getConnectAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetConnectAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetConnectAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a core network Connect peer.
*
*
* @param getConnectPeerRequest
* @return Result of the GetConnectPeer operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetConnectPeer
* @see AWS
* API Documentation
*/
@Override
public GetConnectPeerResult getConnectPeer(GetConnectPeerRequest request) {
request = beforeClientExecution(request);
return executeGetConnectPeer(request);
}
@SdkInternalApi
final GetConnectPeerResult executeGetConnectPeer(GetConnectPeerRequest getConnectPeerRequest) {
ExecutionContext executionContext = createExecutionContext(getConnectPeerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetConnectPeerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getConnectPeerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetConnectPeer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetConnectPeerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a core network Connect peer associations.
*
*
* @param getConnectPeerAssociationsRequest
* @return Result of the GetConnectPeerAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetConnectPeerAssociations
* @see AWS API Documentation
*/
@Override
public GetConnectPeerAssociationsResult getConnectPeerAssociations(GetConnectPeerAssociationsRequest request) {
request = beforeClientExecution(request);
return executeGetConnectPeerAssociations(request);
}
@SdkInternalApi
final GetConnectPeerAssociationsResult executeGetConnectPeerAssociations(GetConnectPeerAssociationsRequest getConnectPeerAssociationsRequest) {
ExecutionContext executionContext = createExecutionContext(getConnectPeerAssociationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetConnectPeerAssociationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getConnectPeerAssociationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetConnectPeerAssociations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetConnectPeerAssociationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about one or more of your connections in a global network.
*
*
* @param getConnectionsRequest
* @return Result of the GetConnections operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetConnections
* @see AWS
* API Documentation
*/
@Override
public GetConnectionsResult getConnections(GetConnectionsRequest request) {
request = beforeClientExecution(request);
return executeGetConnections(request);
}
@SdkInternalApi
final GetConnectionsResult executeGetConnections(GetConnectionsRequest getConnectionsRequest) {
ExecutionContext executionContext = createExecutionContext(getConnectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetConnectionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getConnectionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetConnections");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetConnectionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about the LIVE policy for a core network.
*
*
* @param getCoreNetworkRequest
* @return Result of the GetCoreNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetCoreNetwork
* @see AWS
* API Documentation
*/
@Override
public GetCoreNetworkResult getCoreNetwork(GetCoreNetworkRequest request) {
request = beforeClientExecution(request);
return executeGetCoreNetwork(request);
}
@SdkInternalApi
final GetCoreNetworkResult executeGetCoreNetwork(GetCoreNetworkRequest getCoreNetworkRequest) {
ExecutionContext executionContext = createExecutionContext(getCoreNetworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCoreNetworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getCoreNetworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCoreNetwork");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetCoreNetworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a core network change event.
*
*
* @param getCoreNetworkChangeEventsRequest
* @return Result of the GetCoreNetworkChangeEvents operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetCoreNetworkChangeEvents
* @see AWS API Documentation
*/
@Override
public GetCoreNetworkChangeEventsResult getCoreNetworkChangeEvents(GetCoreNetworkChangeEventsRequest request) {
request = beforeClientExecution(request);
return executeGetCoreNetworkChangeEvents(request);
}
@SdkInternalApi
final GetCoreNetworkChangeEventsResult executeGetCoreNetworkChangeEvents(GetCoreNetworkChangeEventsRequest getCoreNetworkChangeEventsRequest) {
ExecutionContext executionContext = createExecutionContext(getCoreNetworkChangeEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCoreNetworkChangeEventsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getCoreNetworkChangeEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCoreNetworkChangeEvents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetCoreNetworkChangeEventsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a change set between the LIVE core network policy and a submitted policy.
*
*
* @param getCoreNetworkChangeSetRequest
* @return Result of the GetCoreNetworkChangeSet operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetCoreNetworkChangeSet
* @see AWS API Documentation
*/
@Override
public GetCoreNetworkChangeSetResult getCoreNetworkChangeSet(GetCoreNetworkChangeSetRequest request) {
request = beforeClientExecution(request);
return executeGetCoreNetworkChangeSet(request);
}
@SdkInternalApi
final GetCoreNetworkChangeSetResult executeGetCoreNetworkChangeSet(GetCoreNetworkChangeSetRequest getCoreNetworkChangeSetRequest) {
ExecutionContext executionContext = createExecutionContext(getCoreNetworkChangeSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCoreNetworkChangeSetRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getCoreNetworkChangeSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCoreNetworkChangeSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetCoreNetworkChangeSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns details about a core network policy. You can get details about your current live policy or any previous
* policy version.
*
*
* @param getCoreNetworkPolicyRequest
* @return Result of the GetCoreNetworkPolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetCoreNetworkPolicy
* @see AWS API Documentation
*/
@Override
public GetCoreNetworkPolicyResult getCoreNetworkPolicy(GetCoreNetworkPolicyRequest request) {
request = beforeClientExecution(request);
return executeGetCoreNetworkPolicy(request);
}
@SdkInternalApi
final GetCoreNetworkPolicyResult executeGetCoreNetworkPolicy(GetCoreNetworkPolicyRequest getCoreNetworkPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getCoreNetworkPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCoreNetworkPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getCoreNetworkPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCoreNetworkPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetCoreNetworkPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
* @param getCustomerGatewayAssociationsRequest
* @return Result of the GetCustomerGatewayAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
@Override
public GetCustomerGatewayAssociationsResult getCustomerGatewayAssociations(GetCustomerGatewayAssociationsRequest request) {
request = beforeClientExecution(request);
return executeGetCustomerGatewayAssociations(request);
}
@SdkInternalApi
final GetCustomerGatewayAssociationsResult executeGetCustomerGatewayAssociations(GetCustomerGatewayAssociationsRequest getCustomerGatewayAssociationsRequest) {
ExecutionContext executionContext = createExecutionContext(getCustomerGatewayAssociationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCustomerGatewayAssociationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getCustomerGatewayAssociationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCustomerGatewayAssociations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetCustomerGatewayAssociationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about one or more of your devices in a global network.
*
*
* @param getDevicesRequest
* @return Result of the GetDevices operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetDevices
* @see AWS API
* Documentation
*/
@Override
public GetDevicesResult getDevices(GetDevicesRequest request) {
request = beforeClientExecution(request);
return executeGetDevices(request);
}
@SdkInternalApi
final GetDevicesResult executeGetDevices(GetDevicesRequest getDevicesRequest) {
ExecutionContext executionContext = createExecutionContext(getDevicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDevicesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDevicesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDevices");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDevicesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
* @param getLinkAssociationsRequest
* @return Result of the GetLinkAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetLinkAssociations
* @see AWS API Documentation
*/
@Override
public GetLinkAssociationsResult getLinkAssociations(GetLinkAssociationsRequest request) {
request = beforeClientExecution(request);
return executeGetLinkAssociations(request);
}
@SdkInternalApi
final GetLinkAssociationsResult executeGetLinkAssociations(GetLinkAssociationsRequest getLinkAssociationsRequest) {
ExecutionContext executionContext = createExecutionContext(getLinkAssociationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetLinkAssociationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getLinkAssociationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetLinkAssociations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetLinkAssociationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
* @param getLinksRequest
* @return Result of the GetLinks operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetLinks
* @see AWS API
* Documentation
*/
@Override
public GetLinksResult getLinks(GetLinksRequest request) {
request = beforeClientExecution(request);
return executeGetLinks(request);
}
@SdkInternalApi
final GetLinksResult executeGetLinks(GetLinksRequest getLinksRequest) {
ExecutionContext executionContext = createExecutionContext(getLinksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetLinksRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getLinksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetLinks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetLinksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the count of network resources, by resource type, for the specified global network.
*
*
* @param getNetworkResourceCountsRequest
* @return Result of the GetNetworkResourceCounts operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkResourceCounts
* @see AWS API Documentation
*/
@Override
public GetNetworkResourceCountsResult getNetworkResourceCounts(GetNetworkResourceCountsRequest request) {
request = beforeClientExecution(request);
return executeGetNetworkResourceCounts(request);
}
@SdkInternalApi
final GetNetworkResourceCountsResult executeGetNetworkResourceCounts(GetNetworkResourceCountsRequest getNetworkResourceCountsRequest) {
ExecutionContext executionContext = createExecutionContext(getNetworkResourceCountsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetNetworkResourceCountsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getNetworkResourceCountsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetNetworkResourceCounts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetNetworkResourceCountsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the network resource relationships for the specified global network.
*
*
* @param getNetworkResourceRelationshipsRequest
* @return Result of the GetNetworkResourceRelationships operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkResourceRelationships
* @see AWS API Documentation
*/
@Override
public GetNetworkResourceRelationshipsResult getNetworkResourceRelationships(GetNetworkResourceRelationshipsRequest request) {
request = beforeClientExecution(request);
return executeGetNetworkResourceRelationships(request);
}
@SdkInternalApi
final GetNetworkResourceRelationshipsResult executeGetNetworkResourceRelationships(
GetNetworkResourceRelationshipsRequest getNetworkResourceRelationshipsRequest) {
ExecutionContext executionContext = createExecutionContext(getNetworkResourceRelationshipsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetNetworkResourceRelationshipsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getNetworkResourceRelationshipsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetNetworkResourceRelationships");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetNetworkResourceRelationshipsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the network resources for the specified global network.
*
*
* The results include information from the corresponding Describe call for the resource, minus any sensitive
* information such as pre-shared keys.
*
*
* @param getNetworkResourcesRequest
* @return Result of the GetNetworkResources operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkResources
* @see AWS API Documentation
*/
@Override
public GetNetworkResourcesResult getNetworkResources(GetNetworkResourcesRequest request) {
request = beforeClientExecution(request);
return executeGetNetworkResources(request);
}
@SdkInternalApi
final GetNetworkResourcesResult executeGetNetworkResources(GetNetworkResourcesRequest getNetworkResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(getNetworkResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetNetworkResourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getNetworkResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetNetworkResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetNetworkResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the network routes of the specified global network.
*
*
* @param getNetworkRoutesRequest
* @return Result of the GetNetworkRoutes operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkRoutes
* @see AWS API Documentation
*/
@Override
public GetNetworkRoutesResult getNetworkRoutes(GetNetworkRoutesRequest request) {
request = beforeClientExecution(request);
return executeGetNetworkRoutes(request);
}
@SdkInternalApi
final GetNetworkRoutesResult executeGetNetworkRoutes(GetNetworkRoutesRequest getNetworkRoutesRequest) {
ExecutionContext executionContext = createExecutionContext(getNetworkRoutesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetNetworkRoutesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getNetworkRoutesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetNetworkRoutes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetNetworkRoutesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the network telemetry of the specified global network.
*
*
* @param getNetworkTelemetryRequest
* @return Result of the GetNetworkTelemetry operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetNetworkTelemetry
* @see AWS API Documentation
*/
@Override
public GetNetworkTelemetryResult getNetworkTelemetry(GetNetworkTelemetryRequest request) {
request = beforeClientExecution(request);
return executeGetNetworkTelemetry(request);
}
@SdkInternalApi
final GetNetworkTelemetryResult executeGetNetworkTelemetry(GetNetworkTelemetryRequest getNetworkTelemetryRequest) {
ExecutionContext executionContext = createExecutionContext(getNetworkTelemetryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetNetworkTelemetryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getNetworkTelemetryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetNetworkTelemetry");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetNetworkTelemetryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a resource policy.
*
*
* @param getResourcePolicyRequest
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetResourcePolicy
* @see AWS API Documentation
*/
@Override
public GetResourcePolicyResult getResourcePolicy(GetResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeGetResourcePolicy(request);
}
@SdkInternalApi
final GetResourcePolicyResult executeGetResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified route analysis.
*
*
* @param getRouteAnalysisRequest
* @return Result of the GetRouteAnalysis operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetRouteAnalysis
* @see AWS API Documentation
*/
@Override
public GetRouteAnalysisResult getRouteAnalysis(GetRouteAnalysisRequest request) {
request = beforeClientExecution(request);
return executeGetRouteAnalysis(request);
}
@SdkInternalApi
final GetRouteAnalysisResult executeGetRouteAnalysis(GetRouteAnalysisRequest getRouteAnalysisRequest) {
ExecutionContext executionContext = createExecutionContext(getRouteAnalysisRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRouteAnalysisRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getRouteAnalysisRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRouteAnalysis");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetRouteAnalysisResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a site-to-site VPN attachment.
*
*
* @param getSiteToSiteVpnAttachmentRequest
* @return Result of the GetSiteToSiteVpnAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetSiteToSiteVpnAttachment
* @see AWS API Documentation
*/
@Override
public GetSiteToSiteVpnAttachmentResult getSiteToSiteVpnAttachment(GetSiteToSiteVpnAttachmentRequest request) {
request = beforeClientExecution(request);
return executeGetSiteToSiteVpnAttachment(request);
}
@SdkInternalApi
final GetSiteToSiteVpnAttachmentResult executeGetSiteToSiteVpnAttachment(GetSiteToSiteVpnAttachmentRequest getSiteToSiteVpnAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(getSiteToSiteVpnAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSiteToSiteVpnAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSiteToSiteVpnAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSiteToSiteVpnAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSiteToSiteVpnAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about one or more of your sites in a global network.
*
*
* @param getSitesRequest
* @return Result of the GetSites operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetSites
* @see AWS API
* Documentation
*/
@Override
public GetSitesResult getSites(GetSitesRequest request) {
request = beforeClientExecution(request);
return executeGetSites(request);
}
@SdkInternalApi
final GetSitesResult executeGetSites(GetSitesRequest getSitesRequest) {
ExecutionContext executionContext = createExecutionContext(getSitesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSitesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSitesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSites");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSitesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about one or more of your transit gateway Connect peer associations in a global network.
*
*
* @param getTransitGatewayConnectPeerAssociationsRequest
* @return Result of the GetTransitGatewayConnectPeerAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetTransitGatewayConnectPeerAssociations
* @see AWS API Documentation
*/
@Override
public GetTransitGatewayConnectPeerAssociationsResult getTransitGatewayConnectPeerAssociations(GetTransitGatewayConnectPeerAssociationsRequest request) {
request = beforeClientExecution(request);
return executeGetTransitGatewayConnectPeerAssociations(request);
}
@SdkInternalApi
final GetTransitGatewayConnectPeerAssociationsResult executeGetTransitGatewayConnectPeerAssociations(
GetTransitGatewayConnectPeerAssociationsRequest getTransitGatewayConnectPeerAssociationsRequest) {
ExecutionContext executionContext = createExecutionContext(getTransitGatewayConnectPeerAssociationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTransitGatewayConnectPeerAssociationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getTransitGatewayConnectPeerAssociationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTransitGatewayConnectPeerAssociations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetTransitGatewayConnectPeerAssociationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a transit gateway peer.
*
*
* @param getTransitGatewayPeeringRequest
* @return Result of the GetTransitGatewayPeering operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetTransitGatewayPeering
* @see AWS API Documentation
*/
@Override
public GetTransitGatewayPeeringResult getTransitGatewayPeering(GetTransitGatewayPeeringRequest request) {
request = beforeClientExecution(request);
return executeGetTransitGatewayPeering(request);
}
@SdkInternalApi
final GetTransitGatewayPeeringResult executeGetTransitGatewayPeering(GetTransitGatewayPeeringRequest getTransitGatewayPeeringRequest) {
ExecutionContext executionContext = createExecutionContext(getTransitGatewayPeeringRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTransitGatewayPeeringRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getTransitGatewayPeeringRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTransitGatewayPeering");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetTransitGatewayPeeringResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
* @param getTransitGatewayRegistrationsRequest
* @return Result of the GetTransitGatewayRegistrations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
@Override
public GetTransitGatewayRegistrationsResult getTransitGatewayRegistrations(GetTransitGatewayRegistrationsRequest request) {
request = beforeClientExecution(request);
return executeGetTransitGatewayRegistrations(request);
}
@SdkInternalApi
final GetTransitGatewayRegistrationsResult executeGetTransitGatewayRegistrations(GetTransitGatewayRegistrationsRequest getTransitGatewayRegistrationsRequest) {
ExecutionContext executionContext = createExecutionContext(getTransitGatewayRegistrationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTransitGatewayRegistrationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getTransitGatewayRegistrationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTransitGatewayRegistrations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetTransitGatewayRegistrationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a transit gateway route table attachment.
*
*
* @param getTransitGatewayRouteTableAttachmentRequest
* @return Result of the GetTransitGatewayRouteTableAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetTransitGatewayRouteTableAttachment
* @see AWS API Documentation
*/
@Override
public GetTransitGatewayRouteTableAttachmentResult getTransitGatewayRouteTableAttachment(GetTransitGatewayRouteTableAttachmentRequest request) {
request = beforeClientExecution(request);
return executeGetTransitGatewayRouteTableAttachment(request);
}
@SdkInternalApi
final GetTransitGatewayRouteTableAttachmentResult executeGetTransitGatewayRouteTableAttachment(
GetTransitGatewayRouteTableAttachmentRequest getTransitGatewayRouteTableAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(getTransitGatewayRouteTableAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTransitGatewayRouteTableAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getTransitGatewayRouteTableAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTransitGatewayRouteTableAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetTransitGatewayRouteTableAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a VPC attachment.
*
*
* @param getVpcAttachmentRequest
* @return Result of the GetVpcAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.GetVpcAttachment
* @see AWS API Documentation
*/
@Override
public GetVpcAttachmentResult getVpcAttachment(GetVpcAttachmentRequest request) {
request = beforeClientExecution(request);
return executeGetVpcAttachment(request);
}
@SdkInternalApi
final GetVpcAttachmentResult executeGetVpcAttachment(GetVpcAttachmentRequest getVpcAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(getVpcAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVpcAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getVpcAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVpcAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetVpcAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of core network attachments.
*
*
* @param listAttachmentsRequest
* @return Result of the ListAttachments operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListAttachments
* @see AWS
* API Documentation
*/
@Override
public ListAttachmentsResult listAttachments(ListAttachmentsRequest request) {
request = beforeClientExecution(request);
return executeListAttachments(request);
}
@SdkInternalApi
final ListAttachmentsResult executeListAttachments(ListAttachmentsRequest listAttachmentsRequest) {
ExecutionContext executionContext = createExecutionContext(listAttachmentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAttachmentsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAttachmentsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAttachments");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAttachmentsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of core network Connect peers.
*
*
* @param listConnectPeersRequest
* @return Result of the ListConnectPeers operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListConnectPeers
* @see AWS API Documentation
*/
@Override
public ListConnectPeersResult listConnectPeers(ListConnectPeersRequest request) {
request = beforeClientExecution(request);
return executeListConnectPeers(request);
}
@SdkInternalApi
final ListConnectPeersResult executeListConnectPeers(ListConnectPeersRequest listConnectPeersRequest) {
ExecutionContext executionContext = createExecutionContext(listConnectPeersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListConnectPeersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listConnectPeersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListConnectPeers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListConnectPeersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of core network policy versions.
*
*
* @param listCoreNetworkPolicyVersionsRequest
* @return Result of the ListCoreNetworkPolicyVersions operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListCoreNetworkPolicyVersions
* @see AWS API Documentation
*/
@Override
public ListCoreNetworkPolicyVersionsResult listCoreNetworkPolicyVersions(ListCoreNetworkPolicyVersionsRequest request) {
request = beforeClientExecution(request);
return executeListCoreNetworkPolicyVersions(request);
}
@SdkInternalApi
final ListCoreNetworkPolicyVersionsResult executeListCoreNetworkPolicyVersions(ListCoreNetworkPolicyVersionsRequest listCoreNetworkPolicyVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(listCoreNetworkPolicyVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCoreNetworkPolicyVersionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listCoreNetworkPolicyVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCoreNetworkPolicyVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListCoreNetworkPolicyVersionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of owned and shared core networks.
*
*
* @param listCoreNetworksRequest
* @return Result of the ListCoreNetworks operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListCoreNetworks
* @see AWS API Documentation
*/
@Override
public ListCoreNetworksResult listCoreNetworks(ListCoreNetworksRequest request) {
request = beforeClientExecution(request);
return executeListCoreNetworks(request);
}
@SdkInternalApi
final ListCoreNetworksResult executeListCoreNetworks(ListCoreNetworksRequest listCoreNetworksRequest) {
ExecutionContext executionContext = createExecutionContext(listCoreNetworksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCoreNetworksRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listCoreNetworksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCoreNetworks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListCoreNetworksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the status of the Service Linked Role (SLR) deployment for the accounts in a given Amazon Web Services
* Organization.
*
*
* @param listOrganizationServiceAccessStatusRequest
* @return Result of the ListOrganizationServiceAccessStatus operation returned by the service.
* @sample AWSNetworkManager.ListOrganizationServiceAccessStatus
* @see AWS API Documentation
*/
@Override
public ListOrganizationServiceAccessStatusResult listOrganizationServiceAccessStatus(ListOrganizationServiceAccessStatusRequest request) {
request = beforeClientExecution(request);
return executeListOrganizationServiceAccessStatus(request);
}
@SdkInternalApi
final ListOrganizationServiceAccessStatusResult executeListOrganizationServiceAccessStatus(
ListOrganizationServiceAccessStatusRequest listOrganizationServiceAccessStatusRequest) {
ExecutionContext executionContext = createExecutionContext(listOrganizationServiceAccessStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListOrganizationServiceAccessStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listOrganizationServiceAccessStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListOrganizationServiceAccessStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListOrganizationServiceAccessStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the peerings for a core network.
*
*
* @param listPeeringsRequest
* @return Result of the ListPeerings operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListPeerings
* @see AWS
* API Documentation
*/
@Override
public ListPeeringsResult listPeerings(ListPeeringsRequest request) {
request = beforeClientExecution(request);
return executeListPeerings(request);
}
@SdkInternalApi
final ListPeeringsResult executeListPeerings(ListPeeringsRequest listPeeringsRequest) {
ExecutionContext executionContext = createExecutionContext(listPeeringsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPeeringsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPeeringsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPeerings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPeeringsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the tags for a specified resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new, immutable version of a core network policy. A subsequent change set is created showing the
* differences between the LIVE policy and the submitted policy.
*
*
* @param putCoreNetworkPolicyRequest
* @return Result of the PutCoreNetworkPolicy operation returned by the service.
* @throws CoreNetworkPolicyException
* Describes a core network policy exception.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @sample AWSNetworkManager.PutCoreNetworkPolicy
* @see AWS API Documentation
*/
@Override
public PutCoreNetworkPolicyResult putCoreNetworkPolicy(PutCoreNetworkPolicyRequest request) {
request = beforeClientExecution(request);
return executePutCoreNetworkPolicy(request);
}
@SdkInternalApi
final PutCoreNetworkPolicyResult executePutCoreNetworkPolicy(PutCoreNetworkPolicyRequest putCoreNetworkPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putCoreNetworkPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutCoreNetworkPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putCoreNetworkPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutCoreNetworkPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutCoreNetworkPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates or updates a resource policy.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.PutResourcePolicy
* @see AWS API Documentation
*/
@Override
public PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executePutResourcePolicy(request);
}
@SdkInternalApi
final PutResourcePolicyResult executePutResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Registers a transit gateway in your global network. Not all Regions support transit gateways for global networks.
* For a list of the supported Regions, see Region Availability in the Amazon Web Services Transit Gateways for Global Networks User Guide. The
* transit gateway can be in any of the supported Amazon Web Services Regions, but it must be owned by the same
* Amazon Web Services account that owns the global network. You cannot register a transit gateway in more than one
* global network.
*
*
* @param registerTransitGatewayRequest
* @return Result of the RegisterTransitGateway operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.RegisterTransitGateway
* @see AWS API Documentation
*/
@Override
public RegisterTransitGatewayResult registerTransitGateway(RegisterTransitGatewayRequest request) {
request = beforeClientExecution(request);
return executeRegisterTransitGateway(request);
}
@SdkInternalApi
final RegisterTransitGatewayResult executeRegisterTransitGateway(RegisterTransitGatewayRequest registerTransitGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(registerTransitGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterTransitGatewayRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(registerTransitGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RegisterTransitGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RegisterTransitGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Rejects a core network attachment request.
*
*
* @param rejectAttachmentRequest
* @return Result of the RejectAttachment operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.RejectAttachment
* @see AWS API Documentation
*/
@Override
public RejectAttachmentResult rejectAttachment(RejectAttachmentRequest request) {
request = beforeClientExecution(request);
return executeRejectAttachment(request);
}
@SdkInternalApi
final RejectAttachmentResult executeRejectAttachment(RejectAttachmentRequest rejectAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(rejectAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RejectAttachmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(rejectAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RejectAttachment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RejectAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Restores a previous policy version as a new, immutable version of a core network policy. A subsequent change set
* is created showing the differences between the LIVE policy and restored policy.
*
*
* @param restoreCoreNetworkPolicyVersionRequest
* @return Result of the RestoreCoreNetworkPolicyVersion operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @sample AWSNetworkManager.RestoreCoreNetworkPolicyVersion
* @see AWS API Documentation
*/
@Override
public RestoreCoreNetworkPolicyVersionResult restoreCoreNetworkPolicyVersion(RestoreCoreNetworkPolicyVersionRequest request) {
request = beforeClientExecution(request);
return executeRestoreCoreNetworkPolicyVersion(request);
}
@SdkInternalApi
final RestoreCoreNetworkPolicyVersionResult executeRestoreCoreNetworkPolicyVersion(
RestoreCoreNetworkPolicyVersionRequest restoreCoreNetworkPolicyVersionRequest) {
ExecutionContext executionContext = createExecutionContext(restoreCoreNetworkPolicyVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RestoreCoreNetworkPolicyVersionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(restoreCoreNetworkPolicyVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RestoreCoreNetworkPolicyVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RestoreCoreNetworkPolicyVersionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables the Network Manager service for an Amazon Web Services Organization. This can only be called by a
* management account within the organization.
*
*
* @param startOrganizationServiceAccessUpdateRequest
* @return Result of the StartOrganizationServiceAccessUpdate operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.StartOrganizationServiceAccessUpdate
* @see AWS API Documentation
*/
@Override
public StartOrganizationServiceAccessUpdateResult startOrganizationServiceAccessUpdate(StartOrganizationServiceAccessUpdateRequest request) {
request = beforeClientExecution(request);
return executeStartOrganizationServiceAccessUpdate(request);
}
@SdkInternalApi
final StartOrganizationServiceAccessUpdateResult executeStartOrganizationServiceAccessUpdate(
StartOrganizationServiceAccessUpdateRequest startOrganizationServiceAccessUpdateRequest) {
ExecutionContext executionContext = createExecutionContext(startOrganizationServiceAccessUpdateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartOrganizationServiceAccessUpdateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(startOrganizationServiceAccessUpdateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartOrganizationServiceAccessUpdate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartOrganizationServiceAccessUpdateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts analyzing the routing path between the specified source and destination. For more information, see Route Analyzer.
*
*
* @param startRouteAnalysisRequest
* @return Result of the StartRouteAnalysis operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.StartRouteAnalysis
* @see AWS API Documentation
*/
@Override
public StartRouteAnalysisResult startRouteAnalysis(StartRouteAnalysisRequest request) {
request = beforeClientExecution(request);
return executeStartRouteAnalysis(request);
}
@SdkInternalApi
final StartRouteAnalysisResult executeStartRouteAnalysis(StartRouteAnalysisRequest startRouteAnalysisRequest) {
ExecutionContext executionContext = createExecutionContext(startRouteAnalysisRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartRouteAnalysisRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startRouteAnalysisRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartRouteAnalysis");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartRouteAnalysisResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Tags a specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes tags from a specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the information for an existing connection. To remove information for any of the parameters, specify an
* empty string.
*
*
* @param updateConnectionRequest
* @return Result of the UpdateConnection operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateConnection
* @see AWS API Documentation
*/
@Override
public UpdateConnectionResult updateConnection(UpdateConnectionRequest request) {
request = beforeClientExecution(request);
return executeUpdateConnection(request);
}
@SdkInternalApi
final UpdateConnectionResult executeUpdateConnection(UpdateConnectionRequest updateConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(updateConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateConnectionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the description of a core network.
*
*
* @param updateCoreNetworkRequest
* @return Result of the UpdateCoreNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateCoreNetwork
* @see AWS API Documentation
*/
@Override
public UpdateCoreNetworkResult updateCoreNetwork(UpdateCoreNetworkRequest request) {
request = beforeClientExecution(request);
return executeUpdateCoreNetwork(request);
}
@SdkInternalApi
final UpdateCoreNetworkResult executeUpdateCoreNetwork(UpdateCoreNetworkRequest updateCoreNetworkRequest) {
ExecutionContext executionContext = createExecutionContext(updateCoreNetworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateCoreNetworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateCoreNetworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateCoreNetwork");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateCoreNetworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the details for an existing device. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateDeviceRequest
* @return Result of the UpdateDevice operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateDevice
* @see AWS
* API Documentation
*/
@Override
public UpdateDeviceResult updateDevice(UpdateDeviceRequest request) {
request = beforeClientExecution(request);
return executeUpdateDevice(request);
}
@SdkInternalApi
final UpdateDeviceResult executeUpdateDevice(UpdateDeviceRequest updateDeviceRequest) {
ExecutionContext executionContext = createExecutionContext(updateDeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDeviceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateDeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDevice");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateDeviceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing global network. To remove information for any of the parameters, specify an empty string.
*
*
* @param updateGlobalNetworkRequest
* @return Result of the UpdateGlobalNetwork operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateGlobalNetwork
* @see AWS API Documentation
*/
@Override
public UpdateGlobalNetworkResult updateGlobalNetwork(UpdateGlobalNetworkRequest request) {
request = beforeClientExecution(request);
return executeUpdateGlobalNetwork(request);
}
@SdkInternalApi
final UpdateGlobalNetworkResult executeUpdateGlobalNetwork(UpdateGlobalNetworkRequest updateGlobalNetworkRequest) {
ExecutionContext executionContext = createExecutionContext(updateGlobalNetworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateGlobalNetworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateGlobalNetworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateGlobalNetwork");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateGlobalNetworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the details for an existing link. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateLinkRequest
* @return Result of the UpdateLink operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws ServiceQuotaExceededException
* A service limit was exceeded.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateLink
* @see AWS API
* Documentation
*/
@Override
public UpdateLinkResult updateLink(UpdateLinkRequest request) {
request = beforeClientExecution(request);
return executeUpdateLink(request);
}
@SdkInternalApi
final UpdateLinkResult executeUpdateLink(UpdateLinkRequest updateLinkRequest) {
ExecutionContext executionContext = createExecutionContext(updateLinkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateLinkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateLinkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateLink");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateLinkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the resource metadata for the specified global network.
*
*
* @param updateNetworkResourceMetadataRequest
* @return Result of the UpdateNetworkResourceMetadata operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateNetworkResourceMetadata
* @see AWS API Documentation
*/
@Override
public UpdateNetworkResourceMetadataResult updateNetworkResourceMetadata(UpdateNetworkResourceMetadataRequest request) {
request = beforeClientExecution(request);
return executeUpdateNetworkResourceMetadata(request);
}
@SdkInternalApi
final UpdateNetworkResourceMetadataResult executeUpdateNetworkResourceMetadata(UpdateNetworkResourceMetadataRequest updateNetworkResourceMetadataRequest) {
ExecutionContext executionContext = createExecutionContext(updateNetworkResourceMetadataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateNetworkResourceMetadataRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateNetworkResourceMetadataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "NetworkManager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateNetworkResourceMetadata");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateNetworkResourceMetadataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the information for an existing site. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateSiteRequest
* @return Result of the UpdateSite operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ConflictException
* There was a conflict processing the request. Updating or deleting the resource can cause an inconsistent
* state.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request has failed due to an internal error.
* @sample AWSNetworkManager.UpdateSite
* @see AWS API
* Documentation
*/
@Override
public UpdateSiteResult updateSite(UpdateSiteRequest request) {
request = beforeClientExecution(request);
return executeUpdateSite(request);
}
@SdkInternalApi
final UpdateSiteResult executeUpdateSite(UpdateSiteRequest updateSiteRequest) {
ExecutionContext executionContext = createExecutionContext(updateSiteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response