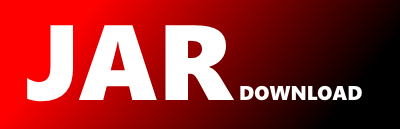
com.amazonaws.services.networkmanager.model.Peering Maven / Gradle / Ivy
Show all versions of aws-java-sdk-networkmanager Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.networkmanager.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a peering connection.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Peering implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the core network for the peering request.
*
*/
private String coreNetworkId;
/**
*
* The ARN of a core network.
*
*/
private String coreNetworkArn;
/**
*
* The ID of the peering attachment.
*
*/
private String peeringId;
/**
*
* The ID of the account owner.
*
*/
private String ownerAccountId;
/**
*
* The type of peering. This will be TRANSIT_GATEWAY
.
*
*/
private String peeringType;
/**
*
* The current state of the peering connection.
*
*/
private String state;
/**
*
* The edge location for the peer.
*
*/
private String edgeLocation;
/**
*
* The resource ARN of the peer.
*
*/
private String resourceArn;
/**
*
* The list of key-value tags associated with the peering.
*
*/
private java.util.List tags;
/**
*
* The timestamp when the attachment peer was created.
*
*/
private java.util.Date createdAt;
/**
*
* Describes the error associated with the Connect peer request.
*
*/
private java.util.List lastModificationErrors;
/**
*
* The ID of the core network for the peering request.
*
*
* @param coreNetworkId
* The ID of the core network for the peering request.
*/
public void setCoreNetworkId(String coreNetworkId) {
this.coreNetworkId = coreNetworkId;
}
/**
*
* The ID of the core network for the peering request.
*
*
* @return The ID of the core network for the peering request.
*/
public String getCoreNetworkId() {
return this.coreNetworkId;
}
/**
*
* The ID of the core network for the peering request.
*
*
* @param coreNetworkId
* The ID of the core network for the peering request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withCoreNetworkId(String coreNetworkId) {
setCoreNetworkId(coreNetworkId);
return this;
}
/**
*
* The ARN of a core network.
*
*
* @param coreNetworkArn
* The ARN of a core network.
*/
public void setCoreNetworkArn(String coreNetworkArn) {
this.coreNetworkArn = coreNetworkArn;
}
/**
*
* The ARN of a core network.
*
*
* @return The ARN of a core network.
*/
public String getCoreNetworkArn() {
return this.coreNetworkArn;
}
/**
*
* The ARN of a core network.
*
*
* @param coreNetworkArn
* The ARN of a core network.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withCoreNetworkArn(String coreNetworkArn) {
setCoreNetworkArn(coreNetworkArn);
return this;
}
/**
*
* The ID of the peering attachment.
*
*
* @param peeringId
* The ID of the peering attachment.
*/
public void setPeeringId(String peeringId) {
this.peeringId = peeringId;
}
/**
*
* The ID of the peering attachment.
*
*
* @return The ID of the peering attachment.
*/
public String getPeeringId() {
return this.peeringId;
}
/**
*
* The ID of the peering attachment.
*
*
* @param peeringId
* The ID of the peering attachment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withPeeringId(String peeringId) {
setPeeringId(peeringId);
return this;
}
/**
*
* The ID of the account owner.
*
*
* @param ownerAccountId
* The ID of the account owner.
*/
public void setOwnerAccountId(String ownerAccountId) {
this.ownerAccountId = ownerAccountId;
}
/**
*
* The ID of the account owner.
*
*
* @return The ID of the account owner.
*/
public String getOwnerAccountId() {
return this.ownerAccountId;
}
/**
*
* The ID of the account owner.
*
*
* @param ownerAccountId
* The ID of the account owner.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withOwnerAccountId(String ownerAccountId) {
setOwnerAccountId(ownerAccountId);
return this;
}
/**
*
* The type of peering. This will be TRANSIT_GATEWAY
.
*
*
* @param peeringType
* The type of peering. This will be TRANSIT_GATEWAY
.
* @see PeeringType
*/
public void setPeeringType(String peeringType) {
this.peeringType = peeringType;
}
/**
*
* The type of peering. This will be TRANSIT_GATEWAY
.
*
*
* @return The type of peering. This will be TRANSIT_GATEWAY
.
* @see PeeringType
*/
public String getPeeringType() {
return this.peeringType;
}
/**
*
* The type of peering. This will be TRANSIT_GATEWAY
.
*
*
* @param peeringType
* The type of peering. This will be TRANSIT_GATEWAY
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PeeringType
*/
public Peering withPeeringType(String peeringType) {
setPeeringType(peeringType);
return this;
}
/**
*
* The type of peering. This will be TRANSIT_GATEWAY
.
*
*
* @param peeringType
* The type of peering. This will be TRANSIT_GATEWAY
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PeeringType
*/
public Peering withPeeringType(PeeringType peeringType) {
this.peeringType = peeringType.toString();
return this;
}
/**
*
* The current state of the peering connection.
*
*
* @param state
* The current state of the peering connection.
* @see PeeringState
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The current state of the peering connection.
*
*
* @return The current state of the peering connection.
* @see PeeringState
*/
public String getState() {
return this.state;
}
/**
*
* The current state of the peering connection.
*
*
* @param state
* The current state of the peering connection.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PeeringState
*/
public Peering withState(String state) {
setState(state);
return this;
}
/**
*
* The current state of the peering connection.
*
*
* @param state
* The current state of the peering connection.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PeeringState
*/
public Peering withState(PeeringState state) {
this.state = state.toString();
return this;
}
/**
*
* The edge location for the peer.
*
*
* @param edgeLocation
* The edge location for the peer.
*/
public void setEdgeLocation(String edgeLocation) {
this.edgeLocation = edgeLocation;
}
/**
*
* The edge location for the peer.
*
*
* @return The edge location for the peer.
*/
public String getEdgeLocation() {
return this.edgeLocation;
}
/**
*
* The edge location for the peer.
*
*
* @param edgeLocation
* The edge location for the peer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withEdgeLocation(String edgeLocation) {
setEdgeLocation(edgeLocation);
return this;
}
/**
*
* The resource ARN of the peer.
*
*
* @param resourceArn
* The resource ARN of the peer.
*/
public void setResourceArn(String resourceArn) {
this.resourceArn = resourceArn;
}
/**
*
* The resource ARN of the peer.
*
*
* @return The resource ARN of the peer.
*/
public String getResourceArn() {
return this.resourceArn;
}
/**
*
* The resource ARN of the peer.
*
*
* @param resourceArn
* The resource ARN of the peer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withResourceArn(String resourceArn) {
setResourceArn(resourceArn);
return this;
}
/**
*
* The list of key-value tags associated with the peering.
*
*
* @return The list of key-value tags associated with the peering.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The list of key-value tags associated with the peering.
*
*
* @param tags
* The list of key-value tags associated with the peering.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The list of key-value tags associated with the peering.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The list of key-value tags associated with the peering.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The list of key-value tags associated with the peering.
*
*
* @param tags
* The list of key-value tags associated with the peering.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The timestamp when the attachment peer was created.
*
*
* @param createdAt
* The timestamp when the attachment peer was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The timestamp when the attachment peer was created.
*
*
* @return The timestamp when the attachment peer was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The timestamp when the attachment peer was created.
*
*
* @param createdAt
* The timestamp when the attachment peer was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* Describes the error associated with the Connect peer request.
*
*
* @return Describes the error associated with the Connect peer request.
*/
public java.util.List getLastModificationErrors() {
return lastModificationErrors;
}
/**
*
* Describes the error associated with the Connect peer request.
*
*
* @param lastModificationErrors
* Describes the error associated with the Connect peer request.
*/
public void setLastModificationErrors(java.util.Collection lastModificationErrors) {
if (lastModificationErrors == null) {
this.lastModificationErrors = null;
return;
}
this.lastModificationErrors = new java.util.ArrayList(lastModificationErrors);
}
/**
*
* Describes the error associated with the Connect peer request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLastModificationErrors(java.util.Collection)} or
* {@link #withLastModificationErrors(java.util.Collection)} if you want to override the existing values.
*
*
* @param lastModificationErrors
* Describes the error associated with the Connect peer request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withLastModificationErrors(PeeringError... lastModificationErrors) {
if (this.lastModificationErrors == null) {
setLastModificationErrors(new java.util.ArrayList(lastModificationErrors.length));
}
for (PeeringError ele : lastModificationErrors) {
this.lastModificationErrors.add(ele);
}
return this;
}
/**
*
* Describes the error associated with the Connect peer request.
*
*
* @param lastModificationErrors
* Describes the error associated with the Connect peer request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Peering withLastModificationErrors(java.util.Collection lastModificationErrors) {
setLastModificationErrors(lastModificationErrors);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCoreNetworkId() != null)
sb.append("CoreNetworkId: ").append(getCoreNetworkId()).append(",");
if (getCoreNetworkArn() != null)
sb.append("CoreNetworkArn: ").append(getCoreNetworkArn()).append(",");
if (getPeeringId() != null)
sb.append("PeeringId: ").append(getPeeringId()).append(",");
if (getOwnerAccountId() != null)
sb.append("OwnerAccountId: ").append(getOwnerAccountId()).append(",");
if (getPeeringType() != null)
sb.append("PeeringType: ").append(getPeeringType()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getEdgeLocation() != null)
sb.append("EdgeLocation: ").append(getEdgeLocation()).append(",");
if (getResourceArn() != null)
sb.append("ResourceArn: ").append(getResourceArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getLastModificationErrors() != null)
sb.append("LastModificationErrors: ").append(getLastModificationErrors());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Peering == false)
return false;
Peering other = (Peering) obj;
if (other.getCoreNetworkId() == null ^ this.getCoreNetworkId() == null)
return false;
if (other.getCoreNetworkId() != null && other.getCoreNetworkId().equals(this.getCoreNetworkId()) == false)
return false;
if (other.getCoreNetworkArn() == null ^ this.getCoreNetworkArn() == null)
return false;
if (other.getCoreNetworkArn() != null && other.getCoreNetworkArn().equals(this.getCoreNetworkArn()) == false)
return false;
if (other.getPeeringId() == null ^ this.getPeeringId() == null)
return false;
if (other.getPeeringId() != null && other.getPeeringId().equals(this.getPeeringId()) == false)
return false;
if (other.getOwnerAccountId() == null ^ this.getOwnerAccountId() == null)
return false;
if (other.getOwnerAccountId() != null && other.getOwnerAccountId().equals(this.getOwnerAccountId()) == false)
return false;
if (other.getPeeringType() == null ^ this.getPeeringType() == null)
return false;
if (other.getPeeringType() != null && other.getPeeringType().equals(this.getPeeringType()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getEdgeLocation() == null ^ this.getEdgeLocation() == null)
return false;
if (other.getEdgeLocation() != null && other.getEdgeLocation().equals(this.getEdgeLocation()) == false)
return false;
if (other.getResourceArn() == null ^ this.getResourceArn() == null)
return false;
if (other.getResourceArn() != null && other.getResourceArn().equals(this.getResourceArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getLastModificationErrors() == null ^ this.getLastModificationErrors() == null)
return false;
if (other.getLastModificationErrors() != null && other.getLastModificationErrors().equals(this.getLastModificationErrors()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCoreNetworkId() == null) ? 0 : getCoreNetworkId().hashCode());
hashCode = prime * hashCode + ((getCoreNetworkArn() == null) ? 0 : getCoreNetworkArn().hashCode());
hashCode = prime * hashCode + ((getPeeringId() == null) ? 0 : getPeeringId().hashCode());
hashCode = prime * hashCode + ((getOwnerAccountId() == null) ? 0 : getOwnerAccountId().hashCode());
hashCode = prime * hashCode + ((getPeeringType() == null) ? 0 : getPeeringType().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getEdgeLocation() == null) ? 0 : getEdgeLocation().hashCode());
hashCode = prime * hashCode + ((getResourceArn() == null) ? 0 : getResourceArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getLastModificationErrors() == null) ? 0 : getLastModificationErrors().hashCode());
return hashCode;
}
@Override
public Peering clone() {
try {
return (Peering) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.networkmanager.model.transform.PeeringMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}