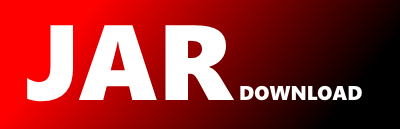
com.amazonaws.services.rds.AmazonRDS Maven / Gradle / Ivy
Show all versions of aws-java-sdk-osgi Show documentation
/*
* Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.rds;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.rds.model.*;
/**
* Interface for accessing AmazonRDS.
* Amazon Relational Database Service
* Amazon Relational Database Service (Amazon RDS) is a web service that
* makes it easier to set up, operate, and scale a relational database in
* the cloud. It provides cost-efficient, resizable capacity for an
* industry-standard relational database and manages common database
* administration tasks, freeing up developers to focus on what makes
* their applications and businesses unique.
*
*
* Amazon RDS gives you access to the capabilities of a MySQL,
* PostgreSQL, Microsoft SQL Server, Oracle, or Aurora database server.
* This means the code, applications, and tools you already use today
* with your existing databases work with Amazon RDS without
* modification. Amazon RDS automatically backs up your database and
* maintains the database software that powers your DB instance. Amazon
* RDS is flexible: you can scale your database instance's compute
* resources and storage capacity to meet your application's demand. As
* with all Amazon Web Services, there are no up-front investments, and
* you pay only for the resources you use.
*
*
* This is an interface reference for Amazon RDS. It contains
* documentation for a programming or command line interface you can use
* to manage Amazon RDS. Note that Amazon RDS is asynchronous, which
* means that some interfaces may require techniques such as polling or
* callback functions to determine when a command has been applied. In
* this reference, the parameter descriptions indicate whether a command
* is applied immediately, on the next instance reboot, or during the
* maintenance window. For a summary of the Amazon RDS interfaces, go to
* Available RDS Interfaces
* .
*
*/
public interface AmazonRDS {
/**
* Overrides the default endpoint for this client ("https://rds.amazonaws.com").
* Callers can use this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "rds.amazonaws.com") or a full
* URL, including the protocol (ex: "https://rds.amazonaws.com"). If the
* protocol is not specified here, the default protocol from this client's
* {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and
* a complete list of all available endpoints for all AWS services, see:
*
* http://developer.amazonwebservices.com/connect/entry.jspa?externalID=3912
*
* This method is not threadsafe. An endpoint should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "rds.amazonaws.com") or a full URL,
* including the protocol (ex: "https://rds.amazonaws.com") of
* the region specific AWS endpoint this client will communicate
* with.
*
* @throws IllegalArgumentException
* If any problems are detected with the specified endpoint.
*/
public void setEndpoint(String endpoint) throws java.lang.IllegalArgumentException;
/**
* An alternative to {@link AmazonRDS#setEndpoint(String)}, sets the
* regional endpoint for this client's service calls. Callers can use this
* method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol.
* To use http instead, specify it in the {@link ClientConfiguration}
* supplied at construction.
*
* This method is not threadsafe. A region should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param region
* The region this client will communicate with. See
* {@link Region#getRegion(com.amazonaws.regions.Regions)} for
* accessing a given region.
* @throws java.lang.IllegalArgumentException
* If the given region is null, or if this service isn't
* available in the given region. See
* {@link Region#isServiceSupported(String)}
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
*/
public void setRegion(Region region) throws java.lang.IllegalArgumentException;
/**
*
* Deletes a DB subnet group.
*
*
* NOTE:The specified database subnet group must not be
* associated with any DB instances.
*
*
* @param deleteDBSubnetGroupRequest Container for the necessary
* parameters to execute the DeleteDBSubnetGroup service method on
* AmazonRDS.
*
*
* @throws DBSubnetGroupNotFoundException
* @throws InvalidDBSubnetGroupStateException
* @throws InvalidDBSubnetStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteDBSubnetGroup(DeleteDBSubnetGroupRequest deleteDBSubnetGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Copies the specified option group.
*
*
* @param copyOptionGroupRequest Container for the necessary parameters
* to execute the CopyOptionGroup service method on AmazonRDS.
*
* @return The response from the CopyOptionGroup service method, as
* returned by AmazonRDS.
*
* @throws OptionGroupAlreadyExistsException
* @throws OptionGroupNotFoundException
* @throws OptionGroupQuotaExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public OptionGroup copyOptionGroup(CopyOptionGroupRequest copyOptionGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Restores a DB instance to an arbitrary point-in-time. Users can
* restore to any point in time before the LatestRestorableTime for up to
* BackupRetentionPeriod days. The target database is created from the
* source database with the same configuration as the original database
* except that the DB instance is created with the default DB security
* group.
*
*
* @param restoreDBInstanceToPointInTimeRequest Container for the
* necessary parameters to execute the RestoreDBInstanceToPointInTime
* service method on AmazonRDS.
*
* @return The response from the RestoreDBInstanceToPointInTime service
* method, as returned by AmazonRDS.
*
* @throws PointInTimeRestoreNotEnabledException
* @throws DBSubnetGroupNotFoundException
* @throws DBInstanceAlreadyExistsException
* @throws DBInstanceNotFoundException
* @throws InvalidVPCNetworkStateException
* @throws StorageTypeNotSupportedException
* @throws InvalidSubnetException
* @throws AuthorizationNotFoundException
* @throws KMSKeyNotAccessibleException
* @throws InvalidRestoreException
* @throws InstanceQuotaExceededException
* @throws StorageQuotaExceededException
* @throws InvalidDBInstanceStateException
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* @throws InsufficientDBInstanceCapacityException
* @throws OptionGroupNotFoundException
* @throws ProvisionedIopsNotAvailableInAZException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBInstance restoreDBInstanceToPointInTime(RestoreDBInstanceToPointInTimeRequest restoreDBInstanceToPointInTimeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Copies the specified DBSnapshot. The source DBSnapshot must be in the
* "available" state.
*
*
* @param copyDBSnapshotRequest Container for the necessary parameters to
* execute the CopyDBSnapshot service method on AmazonRDS.
*
* @return The response from the CopyDBSnapshot service method, as
* returned by AmazonRDS.
*
* @throws InvalidDBSnapshotStateException
* @throws SnapshotQuotaExceededException
* @throws DBSnapshotAlreadyExistsException
* @throws DBSnapshotNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBSnapshot copyDBSnapshot(CopyDBSnapshotRequest copyDBSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of resources (for example, DB instances) that have at
* least one pending maintenance action.
*
*
* @param describePendingMaintenanceActionsRequest Container for the
* necessary parameters to execute the DescribePendingMaintenanceActions
* service method on AmazonRDS.
*
* @return The response from the DescribePendingMaintenanceActions
* service method, as returned by AmazonRDS.
*
* @throws ResourceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribePendingMaintenanceActionsResult describePendingMaintenanceActions(DescribePendingMaintenanceActionsRequest describePendingMaintenanceActionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new DB instance.
*
*
* @param createDBInstanceRequest Container for the necessary parameters
* to execute the CreateDBInstance service method on AmazonRDS.
*
* @return The response from the CreateDBInstance service method, as
* returned by AmazonRDS.
*
* @throws DBParameterGroupNotFoundException
* @throws DBSubnetGroupNotFoundException
* @throws DBInstanceAlreadyExistsException
* @throws InvalidVPCNetworkStateException
* @throws StorageTypeNotSupportedException
* @throws DBSecurityGroupNotFoundException
* @throws InvalidSubnetException
* @throws AuthorizationNotFoundException
* @throws KMSKeyNotAccessibleException
* @throws InstanceQuotaExceededException
* @throws StorageQuotaExceededException
* @throws InsufficientDBInstanceCapacityException
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* @throws OptionGroupNotFoundException
* @throws ProvisionedIopsNotAvailableInAZException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBInstance createDBInstance(CreateDBInstanceRequest createDBInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new DB security group. DB security groups control access to
* a DB instance.
*
*
* @param createDBSecurityGroupRequest Container for the necessary
* parameters to execute the CreateDBSecurityGroup service method on
* AmazonRDS.
*
* @return The response from the CreateDBSecurityGroup service method, as
* returned by AmazonRDS.
*
* @throws DBSecurityGroupQuotaExceededException
* @throws DBSecurityGroupNotSupportedException
* @throws DBSecurityGroupAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBSecurityGroup createDBSecurityGroup(CreateDBSecurityGroupRequest createDBSecurityGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes the available option groups.
*
*
* @param describeOptionGroupsRequest Container for the necessary
* parameters to execute the DescribeOptionGroups service method on
* AmazonRDS.
*
* @return The response from the DescribeOptionGroups service method, as
* returned by AmazonRDS.
*
* @throws OptionGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeOptionGroupsResult describeOptionGroups(DescribeOptionGroupsRequest describeOptionGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about provisioned RDS instances. This API
* supports pagination.
*
*
* @param describeDBInstancesRequest Container for the necessary
* parameters to execute the DescribeDBInstances service method on
* AmazonRDS.
*
* @return The response from the DescribeDBInstances service method, as
* returned by AmazonRDS.
*
* @throws DBInstanceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBInstancesResult describeDBInstances(DescribeDBInstancesRequest describeDBInstancesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Removes a source identifier from an existing RDS event notification
* subscription.
*
*
* @param removeSourceIdentifierFromSubscriptionRequest Container for the
* necessary parameters to execute the
* RemoveSourceIdentifierFromSubscription service method on AmazonRDS.
*
* @return The response from the RemoveSourceIdentifierFromSubscription
* service method, as returned by AmazonRDS.
*
* @throws SourceNotFoundException
* @throws SubscriptionNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public EventSubscription removeSourceIdentifierFromSubscription(RemoveSourceIdentifierFromSubscriptionRequest removeSourceIdentifierFromSubscriptionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a DB security group.
*
*
* NOTE:The specified DB security group must not be associated
* with any DB instances.
*
*
* @param deleteDBSecurityGroupRequest Container for the necessary
* parameters to execute the DeleteDBSecurityGroup service method on
* AmazonRDS.
*
*
* @throws DBSecurityGroupNotFoundException
* @throws InvalidDBSecurityGroupStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteDBSecurityGroup(DeleteDBSecurityGroupRequest deleteDBSecurityGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Enables ingress to a DBSecurityGroup using one of two forms of
* authorization. First, EC2 or VPC security groups can be added to the
* DBSecurityGroup if the application using the database is running on
* EC2 or VPC instances. Second, IP ranges are available if the
* application accessing your database is running on the Internet.
* Required parameters for this API are one of CIDR range,
* EC2SecurityGroupId for VPC, or (EC2SecurityGroupOwnerId and either
* EC2SecurityGroupName or EC2SecurityGroupId for non-VPC).
*
*
* NOTE: You cannot authorize ingress from an EC2 security group
* in one Region to an Amazon RDS DB instance in another. You cannot
* authorize ingress from a VPC security group in one VPC to an Amazon
* RDS DB instance in another.
*
*
* For an overview of CIDR ranges, go to the
* Wikipedia Tutorial
* .
*
*
* @param authorizeDBSecurityGroupIngressRequest Container for the
* necessary parameters to execute the AuthorizeDBSecurityGroupIngress
* service method on AmazonRDS.
*
* @return The response from the AuthorizeDBSecurityGroupIngress service
* method, as returned by AmazonRDS.
*
* @throws DBSecurityGroupNotFoundException
* @throws InvalidDBSecurityGroupStateException
* @throws AuthorizationAlreadyExistsException
* @throws AuthorizationQuotaExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBSecurityGroup authorizeDBSecurityGroupIngress(AuthorizeDBSecurityGroupIngressRequest authorizeDBSecurityGroupIngressRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Applies a pending maintenance action to a resource (for example, a DB
* instance).
*
*
* @param applyPendingMaintenanceActionRequest Container for the
* necessary parameters to execute the ApplyPendingMaintenanceAction
* service method on AmazonRDS.
*
* @return The response from the ApplyPendingMaintenanceAction service
* method, as returned by AmazonRDS.
*
* @throws ResourceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public ResourcePendingMaintenanceActions applyPendingMaintenanceAction(ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modify settings for a DB instance. You can change one or more
* database configuration parameters by specifying these parameters and
* the new values in the request.
*
*
* @param modifyDBInstanceRequest Container for the necessary parameters
* to execute the ModifyDBInstance service method on AmazonRDS.
*
* @return The response from the ModifyDBInstance service method, as
* returned by AmazonRDS.
*
* @throws DBParameterGroupNotFoundException
* @throws CertificateNotFoundException
* @throws DBInstanceAlreadyExistsException
* @throws DBInstanceNotFoundException
* @throws InvalidVPCNetworkStateException
* @throws StorageTypeNotSupportedException
* @throws DBSecurityGroupNotFoundException
* @throws DBUpgradeDependencyFailureException
* @throws AuthorizationNotFoundException
* @throws StorageQuotaExceededException
* @throws InvalidDBInstanceStateException
* @throws InvalidDBSecurityGroupStateException
* @throws InsufficientDBInstanceCapacityException
* @throws OptionGroupNotFoundException
* @throws ProvisionedIopsNotAvailableInAZException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBInstance modifyDBInstance(ModifyDBInstanceRequest modifyDBInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes an RDS event notification subscription.
*
*
* @param deleteEventSubscriptionRequest Container for the necessary
* parameters to execute the DeleteEventSubscription service method on
* AmazonRDS.
*
* @return The response from the DeleteEventSubscription service method,
* as returned by AmazonRDS.
*
* @throws SubscriptionNotFoundException
* @throws InvalidEventSubscriptionStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public EventSubscription deleteEventSubscription(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Removes metadata tags from an Amazon RDS resource.
*
*
* For an overview on tagging an Amazon RDS resource, see
* Tagging Amazon RDS Resources
* .
*
*
* @param removeTagsFromResourceRequest Container for the necessary
* parameters to execute the RemoveTagsFromResource service method on
* AmazonRDS.
*
*
* @throws DBInstanceNotFoundException
* @throws DBSnapshotNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public void removeTagsFromResource(RemoveTagsFromResourceRequest removeTagsFromResourceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Displays a list of categories for all event source types, or, if
* specified, for a specified source type. You can see a list of the
* event categories and source types in the
* Events
* topic in the Amazon RDS User Guide.
*
*
* @param describeEventCategoriesRequest Container for the necessary
* parameters to execute the DescribeEventCategories service method on
* AmazonRDS.
*
* @return The response from the DescribeEventCategories service method,
* as returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventCategoriesResult describeEventCategories(DescribeEventCategoriesRequest describeEventCategoriesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Copies the specified DB parameter group.
*
*
* @param copyDBParameterGroupRequest Container for the necessary
* parameters to execute the CopyDBParameterGroup service method on
* AmazonRDS.
*
* @return The response from the CopyDBParameterGroup service method, as
* returned by AmazonRDS.
*
* @throws DBParameterGroupNotFoundException
* @throws DBParameterGroupQuotaExceededException
* @throws DBParameterGroupAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBParameterGroup copyDBParameterGroup(CopyDBParameterGroupRequest copyDBParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a DB instance that acts as a Read Replica of a source DB
* instance.
*
*
* All Read Replica DB instances are created as Single-AZ deployments
* with backups disabled. All other DB instance attributes (including DB
* security groups and DB parameter groups) are inherited from the source
* DB instance, except as specified below.
*
*
* IMPORTANT: The source DB instance must have backup retention
* enabled.
*
*
* @param createDBInstanceReadReplicaRequest Container for the necessary
* parameters to execute the CreateDBInstanceReadReplica service method
* on AmazonRDS.
*
* @return The response from the CreateDBInstanceReadReplica service
* method, as returned by AmazonRDS.
*
* @throws DBParameterGroupNotFoundException
* @throws DBSubnetGroupNotFoundException
* @throws DBInstanceAlreadyExistsException
* @throws DBInstanceNotFoundException
* @throws InvalidVPCNetworkStateException
* @throws StorageTypeNotSupportedException
* @throws InvalidDBSubnetGroupException
* @throws DBSecurityGroupNotFoundException
* @throws InvalidSubnetException
* @throws KMSKeyNotAccessibleException
* @throws InstanceQuotaExceededException
* @throws DBSubnetGroupNotAllowedException
* @throws StorageQuotaExceededException
* @throws InvalidDBInstanceStateException
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* @throws InsufficientDBInstanceCapacityException
* @throws OptionGroupNotFoundException
* @throws ProvisionedIopsNotAvailableInAZException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBInstance createDBInstanceReadReplica(CreateDBInstanceReadReplicaRequest createDBInstanceReadReplicaRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a DBSnapshot. If the snapshot is being copied, the copy
* operation is terminated.
*
*
* NOTE:The DBSnapshot must be in the available state to be
* deleted.
*
*
* @param deleteDBSnapshotRequest Container for the necessary parameters
* to execute the DeleteDBSnapshot service method on AmazonRDS.
*
* @return The response from the DeleteDBSnapshot service method, as
* returned by AmazonRDS.
*
* @throws InvalidDBSnapshotStateException
* @throws DBSnapshotNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBSnapshot deleteDBSnapshot(DeleteDBSnapshotRequest deleteDBSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Rebooting a DB instance restarts the database engine service. A
* reboot also applies to the DB instance any modifications to the
* associated DB parameter group that were pending. Rebooting a DB
* instance results in a momentary outage of the instance, during which
* the DB instance status is set to rebooting. If the RDS instance is
* configured for MultiAZ, it is possible that the reboot will be
* conducted through a failover. An Amazon RDS event is created when the
* reboot is completed.
*
*
* If your DB instance is deployed in multiple Availability Zones, you
* can force a failover from one AZ to the other during the reboot. You
* might force a failover to test the availability of your DB instance
* deployment or to restore operations to the original AZ after a
* failover occurs.
*
*
* The time required to reboot is a function of the specific database
* engine's crash recovery process. To improve the reboot time, we
* recommend that you reduce database activities as much as possible
* during the reboot process to reduce rollback activity for in-transit
* transactions.
*
*
* @param rebootDBInstanceRequest Container for the necessary parameters
* to execute the RebootDBInstance service method on AmazonRDS.
*
* @return The response from the RebootDBInstance service method, as
* returned by AmazonRDS.
*
* @throws DBInstanceNotFoundException
* @throws InvalidDBInstanceStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBInstance rebootDBInstance(RebootDBInstanceRequest rebootDBInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns events related to DB instances, DB security groups, DB
* snapshots, and DB parameter groups for the past 14 days. Events
* specific to a particular DB instance, DB security group, database
* snapshot, or DB parameter group can be obtained by providing the name
* as a parameter. By default, the past hour of events are returned.
*
*
* @param describeEventsRequest Container for the necessary parameters to
* execute the DescribeEvents service method on AmazonRDS.
*
* @return The response from the DescribeEvents service method, as
* returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventsResult describeEvents(DescribeEventsRequest describeEventsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Lists all tags on an Amazon RDS resource.
*
*
* For an overview on tagging an Amazon RDS resource, see
* Tagging Amazon RDS Resources
* .
*
*
* @param listTagsForResourceRequest Container for the necessary
* parameters to execute the ListTagsForResource service method on
* AmazonRDS.
*
* @return The response from the ListTagsForResource service method, as
* returned by AmazonRDS.
*
* @throws DBInstanceNotFoundException
* @throws DBSnapshotNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes all available options.
*
*
* @param describeOptionGroupOptionsRequest Container for the necessary
* parameters to execute the DescribeOptionGroupOptions service method on
* AmazonRDS.
*
* @return The response from the DescribeOptionGroupOptions service
* method, as returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeOptionGroupOptionsResult describeOptionGroupOptions(DescribeOptionGroupOptionsRequest describeOptionGroupOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the detailed parameter list for a particular DB parameter
* group.
*
*
* @param describeDBParametersRequest Container for the necessary
* parameters to execute the DescribeDBParameters service method on
* AmazonRDS.
*
* @return The response from the DescribeDBParameters service method, as
* returned by AmazonRDS.
*
* @throws DBParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBParametersResult describeDBParameters(DescribeDBParametersRequest describeDBParametersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Purchases a reserved DB instance offering.
*
*
* @param purchaseReservedDBInstancesOfferingRequest Container for the
* necessary parameters to execute the
* PurchaseReservedDBInstancesOffering service method on AmazonRDS.
*
* @return The response from the PurchaseReservedDBInstancesOffering
* service method, as returned by AmazonRDS.
*
* @throws ReservedDBInstancesOfferingNotFoundException
* @throws ReservedDBInstanceQuotaExceededException
* @throws ReservedDBInstanceAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public ReservedDBInstance purchaseReservedDBInstancesOffering(PurchaseReservedDBInstancesOfferingRequest purchaseReservedDBInstancesOfferingRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a
* DBSecurityGroupName
is specified, the list will contain
* only the descriptions of the specified DB security group.
*
*
* @param describeDBSecurityGroupsRequest Container for the necessary
* parameters to execute the DescribeDBSecurityGroups service method on
* AmazonRDS.
*
* @return The response from the DescribeDBSecurityGroups service method,
* as returned by AmazonRDS.
*
* @throws DBSecurityGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBSecurityGroupsResult describeDBSecurityGroups(DescribeDBSecurityGroupsRequest describeDBSecurityGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies an existing RDS event notification subscription. Note that
* you cannot modify the source identifiers using this call; to change
* source identifiers for a subscription, use the
* AddSourceIdentifierToSubscription and
* RemoveSourceIdentifierFromSubscription calls.
*
*
* You can see a list of the event categories for a given SourceType in
* the
* Events
* topic in the Amazon RDS User Guide or by using the
* DescribeEventCategories action.
*
*
* @param modifyEventSubscriptionRequest Container for the necessary
* parameters to execute the ModifyEventSubscription service method on
* AmazonRDS.
*
* @return The response from the ModifyEventSubscription service method,
* as returned by AmazonRDS.
*
* @throws SNSTopicArnNotFoundException
* @throws SNSInvalidTopicException
* @throws SubscriptionCategoryNotFoundException
* @throws SubscriptionNotFoundException
* @throws SNSNoAuthorizationException
* @throws EventSubscriptionQuotaExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public EventSubscription modifyEventSubscription(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteDBInstance action deletes a previously provisioned DB
* instance. A successful response from the web service indicates the
* request was received correctly. When you delete a DB instance, all
* automated backups for that instance are deleted and cannot be
* recovered. Manual DB snapshots of the DB instance to be deleted are
* not deleted.
*
*
* If a final DB snapshot is requested the status of the RDS instance
* will be "deleting" until the DB snapshot is created. The API action
* DescribeDBInstance
is used to monitor the status of this
* operation. The action cannot be canceled or reverted once submitted.
*
*
* @param deleteDBInstanceRequest Container for the necessary parameters
* to execute the DeleteDBInstance service method on AmazonRDS.
*
* @return The response from the DeleteDBInstance service method, as
* returned by AmazonRDS.
*
* @throws DBInstanceNotFoundException
* @throws InvalidDBInstanceStateException
* @throws SnapshotQuotaExceededException
* @throws DBSnapshotAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBInstance deleteDBInstance(DeleteDBInstanceRequest deleteDBInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new DB instance from a DB snapshot. The target database is
* created from the source database restore point with the same
* configuration as the original source database, except that the new RDS
* instance is created with the default security group.
*
*
* If your intent is to replace your original DB instance with the new,
* restored DB instance, then rename your original DB instance before you
* call the RestoreDBInstanceFromDBSnapshot action. RDS does not allow
* two DB instances with the same name. Once you have renamed your
* original DB instance with a different identifier, then you can pass
* the original name of the DB instance as the DBInstanceIdentifier in
* the call to the RestoreDBInstanceFromDBSnapshot action. The result is
* that you will replace the original DB instance with the DB instance
* created from the snapshot.
*
*
* @param restoreDBInstanceFromDBSnapshotRequest Container for the
* necessary parameters to execute the RestoreDBInstanceFromDBSnapshot
* service method on AmazonRDS.
*
* @return The response from the RestoreDBInstanceFromDBSnapshot service
* method, as returned by AmazonRDS.
*
* @throws DBSubnetGroupNotFoundException
* @throws DBInstanceAlreadyExistsException
* @throws InvalidVPCNetworkStateException
* @throws InvalidDBSnapshotStateException
* @throws StorageTypeNotSupportedException
* @throws InvalidSubnetException
* @throws AuthorizationNotFoundException
* @throws DBSnapshotNotFoundException
* @throws KMSKeyNotAccessibleException
* @throws InvalidRestoreException
* @throws InstanceQuotaExceededException
* @throws StorageQuotaExceededException
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* @throws InsufficientDBInstanceCapacityException
* @throws OptionGroupNotFoundException
* @throws ProvisionedIopsNotAvailableInAZException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBInstance restoreDBInstanceFromDBSnapshot(RestoreDBInstanceFromDBSnapshotRequest restoreDBInstanceFromDBSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new option group. You can create up to 20 option groups.
*
*
* @param createOptionGroupRequest Container for the necessary parameters
* to execute the CreateOptionGroup service method on AmazonRDS.
*
* @return The response from the CreateOptionGroup service method, as
* returned by AmazonRDS.
*
* @throws OptionGroupAlreadyExistsException
* @throws OptionGroupQuotaExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public OptionGroup createOptionGroup(CreateOptionGroupRequest createOptionGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies an existing option group.
*
*
* @param modifyOptionGroupRequest Container for the necessary parameters
* to execute the ModifyOptionGroup service method on AmazonRDS.
*
* @return The response from the ModifyOptionGroup service method, as
* returned by AmazonRDS.
*
* @throws InvalidOptionGroupStateException
* @throws OptionGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public OptionGroup modifyOptionGroup(ModifyOptionGroupRequest modifyOptionGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName
* is specified, the list will contain only the descriptions of the
* specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the
* Wikipedia Tutorial
* .
*
*
* @param describeDBSubnetGroupsRequest Container for the necessary
* parameters to execute the DescribeDBSubnetGroups service method on
* AmazonRDS.
*
* @return The response from the DescribeDBSubnetGroups service method,
* as returned by AmazonRDS.
*
* @throws DBSubnetGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBSubnetGroupsResult describeDBSubnetGroups(DescribeDBSubnetGroupsRequest describeDBSubnetGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of DBParameterGroup
descriptions. If a
* DBParameterGroupName
is specified, the list will contain
* only the description of the specified DB parameter group.
*
*
* @param describeDBParameterGroupsRequest Container for the necessary
* parameters to execute the DescribeDBParameterGroups service method on
* AmazonRDS.
*
* @return The response from the DescribeDBParameterGroups service
* method, as returned by AmazonRDS.
*
* @throws DBParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBParameterGroupsResult describeDBParameterGroups(DescribeDBParameterGroupsRequest describeDBParameterGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Lists all the subscription descriptions for a customer account. The
* description for a subscription includes SubscriptionName, SNSTopicARN,
* CustomerID, SourceType, SourceID, CreationTime, and Status.
*
*
* If you specify a SubscriptionName, lists the description for that
* subscription.
*
*
* @param describeEventSubscriptionsRequest Container for the necessary
* parameters to execute the DescribeEventSubscriptions service method on
* AmazonRDS.
*
* @return The response from the DescribeEventSubscriptions service
* method, as returned by AmazonRDS.
*
* @throws SubscriptionNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventSubscriptionsResult describeEventSubscriptions(DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies an existing DB subnet group. DB subnet groups must contain
* at least one subnet in at least two AZs in the region.
*
*
* @param modifyDBSubnetGroupRequest Container for the necessary
* parameters to execute the ModifyDBSubnetGroup service method on
* AmazonRDS.
*
* @return The response from the ModifyDBSubnetGroup service method, as
* returned by AmazonRDS.
*
* @throws DBSubnetGroupNotFoundException
* @throws DBSubnetQuotaExceededException
* @throws SubnetAlreadyInUseException
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* @throws InvalidSubnetException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBSubnetGroup modifyDBSubnetGroup(ModifyDBSubnetGroupRequest modifyDBSubnetGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about DB snapshots. This API supports pagination.
*
*
* @param describeDBSnapshotsRequest Container for the necessary
* parameters to execute the DescribeDBSnapshots service method on
* AmazonRDS.
*
* @return The response from the DescribeDBSnapshots service method, as
* returned by AmazonRDS.
*
* @throws DBSnapshotNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBSnapshotsResult describeDBSnapshots(DescribeDBSnapshotsRequest describeDBSnapshotsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new DB subnet group. DB subnet groups must contain at least
* one subnet in at least two AZs in the region.
*
*
* @param createDBSubnetGroupRequest Container for the necessary
* parameters to execute the CreateDBSubnetGroup service method on
* AmazonRDS.
*
* @return The response from the CreateDBSubnetGroup service method, as
* returned by AmazonRDS.
*
* @throws DBSubnetQuotaExceededException
* @throws DBSubnetGroupAlreadyExistsException
* @throws DBSubnetGroupQuotaExceededException
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* @throws InvalidSubnetException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBSubnetGroup createDBSubnetGroup(CreateDBSubnetGroupRequest createDBSubnetGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Lists all of the attributes for a customer account. The attributes
* include Amazon RDS quotas for the account, such as the number of DB
* instances allowed. The description for a quota includes the quota
* name, current usage toward that quota, and the quota's maximum value.
*
*
* This command does not take any parameters.
*
*
* @param describeAccountAttributesRequest Container for the necessary
* parameters to execute the DescribeAccountAttributes service method on
* AmazonRDS.
*
* @return The response from the DescribeAccountAttributes service
* method, as returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeAccountAttributesResult describeAccountAttributes(DescribeAccountAttributesRequest describeAccountAttributesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of the available DB engines.
*
*
* @param describeDBEngineVersionsRequest Container for the necessary
* parameters to execute the DescribeDBEngineVersions service method on
* AmazonRDS.
*
* @return The response from the DescribeDBEngineVersions service method,
* as returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBEngineVersionsResult describeDBEngineVersions(DescribeDBEngineVersionsRequest describeDBEngineVersionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies the parameters of a DB parameter group. To modify more than
* one parameter, submit a list of the following:
* ParameterName
, ParameterValue
, and
* ApplyMethod
. A maximum of 20 parameters can be modified
* in a single request.
*
*
* NOTE: Changes to dynamic parameters are applied immediately.
* Changes to static parameters require a reboot without failover to the
* DB instance associated with the parameter group before the change can
* take effect.
*
*
* IMPORTANT: After you modify a DB parameter group, you should
* wait at least 5 minutes before creating your first DB instance that
* uses that DB parameter group as the default parameter group. This
* allows Amazon RDS to fully complete the modify action before the
* parameter group is used as the default for a new DB instance. This is
* especially important for parameters that are critical when creating
* the default database for a DB instance, such as the character set for
* the default database defined by the character_set_database parameter.
* You can use the Parameter Groups option of the Amazon RDS console or
* the DescribeDBParameters command to verify that your DB parameter
* group has been created or modified.
*
*
* @param modifyDBParameterGroupRequest Container for the necessary
* parameters to execute the ModifyDBParameterGroup service method on
* AmazonRDS.
*
* @return The response from the ModifyDBParameterGroup service method,
* as returned by AmazonRDS.
*
* @throws DBParameterGroupNotFoundException
* @throws InvalidDBParameterGroupStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public ModifyDBParameterGroupResult modifyDBParameterGroup(ModifyDBParameterGroupRequest modifyDBParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the default engine and system parameter information for the
* specified database engine.
*
*
* @param describeEngineDefaultParametersRequest Container for the
* necessary parameters to execute the DescribeEngineDefaultParameters
* service method on AmazonRDS.
*
* @return The response from the DescribeEngineDefaultParameters service
* method, as returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public EngineDefaults describeEngineDefaultParameters(DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a DBSnapshot. The source DBInstance must be in "available"
* state.
*
*
* @param createDBSnapshotRequest Container for the necessary parameters
* to execute the CreateDBSnapshot service method on AmazonRDS.
*
* @return The response from the CreateDBSnapshot service method, as
* returned by AmazonRDS.
*
* @throws DBInstanceNotFoundException
* @throws InvalidDBInstanceStateException
* @throws SnapshotQuotaExceededException
* @throws DBSnapshotAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBSnapshot createDBSnapshot(CreateDBSnapshotRequest createDBSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Adds a source identifier to an existing RDS event notification
* subscription.
*
*
* @param addSourceIdentifierToSubscriptionRequest Container for the
* necessary parameters to execute the AddSourceIdentifierToSubscription
* service method on AmazonRDS.
*
* @return The response from the AddSourceIdentifierToSubscription
* service method, as returned by AmazonRDS.
*
* @throws SourceNotFoundException
* @throws SubscriptionNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public EventSubscription addSourceIdentifierToSubscription(AddSourceIdentifierToSubscriptionRequest addSourceIdentifierToSubscriptionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies the parameters of a DB parameter group to the engine/system
* default value. To reset specific parameters submit a list of the
* following: ParameterName
and ApplyMethod
.
* To reset the entire DB parameter group, specify the
* DBParameterGroup
name and ResetAllParameters
* parameters. When resetting the entire group, dynamic parameters are
* updated immediately and static parameters are set to
* pending-reboot
to take effect on the next DB instance
* restart or RebootDBInstance
request.
*
*
* @param resetDBParameterGroupRequest Container for the necessary
* parameters to execute the ResetDBParameterGroup service method on
* AmazonRDS.
*
* @return The response from the ResetDBParameterGroup service method, as
* returned by AmazonRDS.
*
* @throws DBParameterGroupNotFoundException
* @throws InvalidDBParameterGroupStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public ResetDBParameterGroupResult resetDBParameterGroup(ResetDBParameterGroupRequest resetDBParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a specified DBParameterGroup. The DBParameterGroup to be
* deleted cannot be associated with any DB instances.
*
*
* @param deleteDBParameterGroupRequest Container for the necessary
* parameters to execute the DeleteDBParameterGroup service method on
* AmazonRDS.
*
*
* @throws DBParameterGroupNotFoundException
* @throws InvalidDBParameterGroupStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteDBParameterGroup(DeleteDBParameterGroupRequest deleteDBParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of DB log files for the DB instance.
*
*
* @param describeDBLogFilesRequest Container for the necessary
* parameters to execute the DescribeDBLogFiles service method on
* AmazonRDS.
*
* @return The response from the DescribeDBLogFiles service method, as
* returned by AmazonRDS.
*
* @throws DBInstanceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBLogFilesResult describeDBLogFiles(DescribeDBLogFilesRequest describeDBLogFilesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about reserved DB instances for this account, or
* about a specified reserved DB instance.
*
*
* @param describeReservedDBInstancesRequest Container for the necessary
* parameters to execute the DescribeReservedDBInstances service method
* on AmazonRDS.
*
* @return The response from the DescribeReservedDBInstances service
* method, as returned by AmazonRDS.
*
* @throws ReservedDBInstanceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedDBInstancesResult describeReservedDBInstances(DescribeReservedDBInstancesRequest describeReservedDBInstancesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Promotes a Read Replica DB instance to a standalone DB instance.
*
*
* NOTE: We recommend that you enable automated backups on your
* Read Replica before promoting the Read Replica. This ensures that no
* backup is taken during the promotion process. Once the instance is
* promoted to a primary instance, backups are taken based on your backup
* settings.
*
*
* @param promoteReadReplicaRequest Container for the necessary
* parameters to execute the PromoteReadReplica service method on
* AmazonRDS.
*
* @return The response from the PromoteReadReplica service method, as
* returned by AmazonRDS.
*
* @throws DBInstanceNotFoundException
* @throws InvalidDBInstanceStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBInstance promoteReadReplica(PromoteReadReplicaRequest promoteReadReplicaRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Adds metadata tags to an Amazon RDS resource. These tags can also be
* used with cost allocation reporting to track cost associated with
* Amazon RDS resources, or used in Condition statement in IAM policy for
* Amazon RDS.
*
*
* For an overview on tagging Amazon RDS resources, see
* Tagging Amazon RDS Resources
* .
*
*
* @param addTagsToResourceRequest Container for the necessary parameters
* to execute the AddTagsToResource service method on AmazonRDS.
*
*
* @throws DBInstanceNotFoundException
* @throws DBSnapshotNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public void addTagsToResource(AddTagsToResourceRequest addTagsToResourceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Downloads all or a portion of the specified log file.
*
*
* @param downloadDBLogFilePortionRequest Container for the necessary
* parameters to execute the DownloadDBLogFilePortion service method on
* AmazonRDS.
*
* @return The response from the DownloadDBLogFilePortion service method,
* as returned by AmazonRDS.
*
* @throws DBInstanceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DownloadDBLogFilePortionResult downloadDBLogFilePortion(DownloadDBLogFilePortionRequest downloadDBLogFilePortionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an RDS event notification subscription. This action requires
* a topic ARN (Amazon Resource Name) created by either the RDS console,
* the SNS console, or the SNS API. To obtain an ARN with SNS, you must
* create a topic in Amazon SNS and subscribe to the topic. The ARN is
* displayed in the SNS console.
*
*
* You can specify the type of source (SourceType) you want to be
* notified of, provide a list of RDS sources (SourceIds) that triggers
* the events, and provide a list of event categories (EventCategories)
* for events you want to be notified of. For example, you can specify
* SourceType = db-instance, SourceIds = mydbinstance1, mydbinstance2 and
* EventCategories = Availability, Backup.
*
*
* If you specify both the SourceType and SourceIds, such as SourceType
* = db-instance and SourceIdentifier = myDBInstance1, you will be
* notified of all the db-instance events for the specified source. If
* you specify a SourceType but do not specify a SourceIdentifier, you
* will receive notice of the events for that source type for all your
* RDS sources. If you do not specify either the SourceType nor the
* SourceIdentifier, you will be notified of events generated from all
* RDS sources belonging to your customer account.
*
*
* @param createEventSubscriptionRequest Container for the necessary
* parameters to execute the CreateEventSubscription service method on
* AmazonRDS.
*
* @return The response from the CreateEventSubscription service method,
* as returned by AmazonRDS.
*
* @throws SourceNotFoundException
* @throws SNSTopicArnNotFoundException
* @throws SNSInvalidTopicException
* @throws SubscriptionCategoryNotFoundException
* @throws SNSNoAuthorizationException
* @throws EventSubscriptionQuotaExceededException
* @throws SubscriptionAlreadyExistException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public EventSubscription createEventSubscription(CreateEventSubscriptionRequest createEventSubscriptionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Revokes ingress from a DBSecurityGroup for previously authorized IP
* ranges or EC2 or VPC Security Groups. Required parameters for this API
* are one of CIDRIP, EC2SecurityGroupId for VPC, or
* (EC2SecurityGroupOwnerId and either EC2SecurityGroupName or
* EC2SecurityGroupId).
*
*
* @param revokeDBSecurityGroupIngressRequest Container for the necessary
* parameters to execute the RevokeDBSecurityGroupIngress service method
* on AmazonRDS.
*
* @return The response from the RevokeDBSecurityGroupIngress service
* method, as returned by AmazonRDS.
*
* @throws DBSecurityGroupNotFoundException
* @throws InvalidDBSecurityGroupStateException
* @throws AuthorizationNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBSecurityGroup revokeDBSecurityGroupIngress(RevokeDBSecurityGroupIngressRequest revokeDBSecurityGroupIngressRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of orderable DB instance options for the specified
* engine.
*
*
* @param describeOrderableDBInstanceOptionsRequest Container for the
* necessary parameters to execute the DescribeOrderableDBInstanceOptions
* service method on AmazonRDS.
*
* @return The response from the DescribeOrderableDBInstanceOptions
* service method, as returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeOrderableDBInstanceOptionsResult describeOrderableDBInstanceOptions(DescribeOrderableDBInstanceOptionsRequest describeOrderableDBInstanceOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new DB parameter group.
*
*
* A DB parameter group is initially created with the default parameters
* for the database engine used by the DB instance. To provide custom
* values for any of the parameters, you must modify the group after
* creating it using ModifyDBParameterGroup . Once you've created
* a DB parameter group, you need to associate it with your DB instance
* using ModifyDBInstance . When you associate a new DB parameter
* group with a running DB instance, you need to reboot the DB instance
* without failover for the new DB parameter group and associated
* settings to take effect.
*
*
* IMPORTANT: After you create a DB parameter group, you should
* wait at least 5 minutes before creating your first DB instance that
* uses that DB parameter group as the default parameter group. This
* allows Amazon RDS to fully complete the create action before the
* parameter group is used as the default for a new DB instance. This is
* especially important for parameters that are critical when creating
* the default database for a DB instance, such as the character set for
* the default database defined by the character_set_database parameter.
* You can use the Parameter Groups option of the Amazon RDS console or
* the DescribeDBParameters command to verify that your DB parameter
* group has been created or modified.
*
*
* @param createDBParameterGroupRequest Container for the necessary
* parameters to execute the CreateDBParameterGroup service method on
* AmazonRDS.
*
* @return The response from the CreateDBParameterGroup service method,
* as returned by AmazonRDS.
*
* @throws DBParameterGroupQuotaExceededException
* @throws DBParameterGroupAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DBParameterGroup createDBParameterGroup(CreateDBParameterGroupRequest createDBParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this AWS
* account.
*
*
* @param describeCertificatesRequest Container for the necessary
* parameters to execute the DescribeCertificates service method on
* AmazonRDS.
*
* @return The response from the DescribeCertificates service method, as
* returned by AmazonRDS.
*
* @throws CertificateNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeCertificatesResult describeCertificates(DescribeCertificatesRequest describeCertificatesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Lists available reserved DB instance offerings.
*
*
* @param describeReservedDBInstancesOfferingsRequest Container for the
* necessary parameters to execute the
* DescribeReservedDBInstancesOfferings service method on AmazonRDS.
*
* @return The response from the DescribeReservedDBInstancesOfferings
* service method, as returned by AmazonRDS.
*
* @throws ReservedDBInstancesOfferingNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedDBInstancesOfferingsResult describeReservedDBInstancesOfferings(DescribeReservedDBInstancesOfferingsRequest describeReservedDBInstancesOfferingsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes an existing option group.
*
*
* @param deleteOptionGroupRequest Container for the necessary parameters
* to execute the DeleteOptionGroup service method on AmazonRDS.
*
*
* @throws InvalidOptionGroupStateException
* @throws OptionGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteOptionGroup(DeleteOptionGroupRequest deleteOptionGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of resources (for example, DB instances) that have at
* least one pending maintenance action.
*
*
* @return The response from the DescribePendingMaintenanceActions
* service method, as returned by AmazonRDS.
*
* @throws ResourceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribePendingMaintenanceActionsResult describePendingMaintenanceActions() throws AmazonServiceException, AmazonClientException;
/**
*
* Describes the available option groups.
*
*
* @return The response from the DescribeOptionGroups service method, as
* returned by AmazonRDS.
*
* @throws OptionGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeOptionGroupsResult describeOptionGroups() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about provisioned RDS instances. This API
* supports pagination.
*
*
* @return The response from the DescribeDBInstances service method, as
* returned by AmazonRDS.
*
* @throws DBInstanceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBInstancesResult describeDBInstances() throws AmazonServiceException, AmazonClientException;
/**
*
* Displays a list of categories for all event source types, or, if
* specified, for a specified source type. You can see a list of the
* event categories and source types in the
* Events
* topic in the Amazon RDS User Guide.
*
*
* @return The response from the DescribeEventCategories service method,
* as returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventCategoriesResult describeEventCategories() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns events related to DB instances, DB security groups, DB
* snapshots, and DB parameter groups for the past 14 days. Events
* specific to a particular DB instance, DB security group, database
* snapshot, or DB parameter group can be obtained by providing the name
* as a parameter. By default, the past hour of events are returned.
*
*
* @return The response from the DescribeEvents service method, as
* returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventsResult describeEvents() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a
* DBSecurityGroupName
is specified, the list will contain
* only the descriptions of the specified DB security group.
*
*
* @return The response from the DescribeDBSecurityGroups service method,
* as returned by AmazonRDS.
*
* @throws DBSecurityGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBSecurityGroupsResult describeDBSecurityGroups() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName
* is specified, the list will contain only the descriptions of the
* specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the
* Wikipedia Tutorial
* .
*
*
* @return The response from the DescribeDBSubnetGroups service method,
* as returned by AmazonRDS.
*
* @throws DBSubnetGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBSubnetGroupsResult describeDBSubnetGroups() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of DBParameterGroup
descriptions. If a
* DBParameterGroupName
is specified, the list will contain
* only the description of the specified DB parameter group.
*
*
* @return The response from the DescribeDBParameterGroups service
* method, as returned by AmazonRDS.
*
* @throws DBParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBParameterGroupsResult describeDBParameterGroups() throws AmazonServiceException, AmazonClientException;
/**
*
* Lists all the subscription descriptions for a customer account. The
* description for a subscription includes SubscriptionName, SNSTopicARN,
* CustomerID, SourceType, SourceID, CreationTime, and Status.
*
*
* If you specify a SubscriptionName, lists the description for that
* subscription.
*
*
* @return The response from the DescribeEventSubscriptions service
* method, as returned by AmazonRDS.
*
* @throws SubscriptionNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventSubscriptionsResult describeEventSubscriptions() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about DB snapshots. This API supports pagination.
*
*
* @return The response from the DescribeDBSnapshots service method, as
* returned by AmazonRDS.
*
* @throws DBSnapshotNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBSnapshotsResult describeDBSnapshots() throws AmazonServiceException, AmazonClientException;
/**
*
* Lists all of the attributes for a customer account. The attributes
* include Amazon RDS quotas for the account, such as the number of DB
* instances allowed. The description for a quota includes the quota
* name, current usage toward that quota, and the quota's maximum value.
*
*
* This command does not take any parameters.
*
*
* @return The response from the DescribeAccountAttributes service
* method, as returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeAccountAttributesResult describeAccountAttributes() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of the available DB engines.
*
*
* @return The response from the DescribeDBEngineVersions service method,
* as returned by AmazonRDS.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDBEngineVersionsResult describeDBEngineVersions() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about reserved DB instances for this account, or
* about a specified reserved DB instance.
*
*
* @return The response from the DescribeReservedDBInstances service
* method, as returned by AmazonRDS.
*
* @throws ReservedDBInstanceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedDBInstancesResult describeReservedDBInstances() throws AmazonServiceException, AmazonClientException;
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this AWS
* account.
*
*
* @return The response from the DescribeCertificates service method, as
* returned by AmazonRDS.
*
* @throws CertificateNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeCertificatesResult describeCertificates() throws AmazonServiceException, AmazonClientException;
/**
*
* Lists available reserved DB instance offerings.
*
*
* @return The response from the DescribeReservedDBInstancesOfferings
* service method, as returned by AmazonRDS.
*
* @throws ReservedDBInstancesOfferingNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRDS indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedDBInstancesOfferingsResult describeReservedDBInstancesOfferings() throws AmazonServiceException, AmazonClientException;
/**
* Shuts down this client object, releasing any resources that might be held
* open. This is an optional method, and callers are not expected to call
* it, but can if they want to explicitly release any open resources. Once a
* client has been shutdown, it should not be used to make any more
* requests.
*/
public void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for
* debugging issues where a service isn't acting as expected. This data isn't considered part
* of the result data returned by an operation, so it's available through this separate,
* diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access
* this extra diagnostic information for an executed request, you should use this method
* to retrieve it as soon as possible after executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}