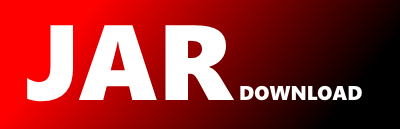
com.amazonaws.services.outposts.AWSOutpostsAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-outposts Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.outposts;
import javax.annotation.Generated;
import com.amazonaws.services.outposts.model.*;
/**
* Interface for accessing Outposts asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.outposts.AbstractAWSOutpostsAsync} instead.
*
*
*
* Amazon Web Services Outposts is a fully managed service that extends Amazon Web Services infrastructure, APIs, and
* tools to customer premises. By providing local access to Amazon Web Services managed infrastructure, Amazon Web
* Services Outposts enables customers to build and run applications on premises using the same programming interfaces
* as in Amazon Web Services Regions, while using local compute and storage resources for lower latency and local data
* processing needs.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSOutpostsAsync extends AWSOutposts {
/**
*
* Cancels the capacity task.
*
*
* @param cancelCapacityTaskRequest
* @return A Java Future containing the result of the CancelCapacityTask operation returned by the service.
* @sample AWSOutpostsAsync.CancelCapacityTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelCapacityTaskAsync(CancelCapacityTaskRequest cancelCapacityTaskRequest);
/**
*
* Cancels the capacity task.
*
*
* @param cancelCapacityTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelCapacityTask operation returned by the service.
* @sample AWSOutpostsAsyncHandler.CancelCapacityTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelCapacityTaskAsync(CancelCapacityTaskRequest cancelCapacityTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified order for an Outpost.
*
*
* @param cancelOrderRequest
* @return A Java Future containing the result of the CancelOrder operation returned by the service.
* @sample AWSOutpostsAsync.CancelOrder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelOrderAsync(CancelOrderRequest cancelOrderRequest);
/**
*
* Cancels the specified order for an Outpost.
*
*
* @param cancelOrderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelOrder operation returned by the service.
* @sample AWSOutpostsAsyncHandler.CancelOrder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelOrderAsync(CancelOrderRequest cancelOrderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an order for an Outpost.
*
*
* @param createOrderRequest
* @return A Java Future containing the result of the CreateOrder operation returned by the service.
* @sample AWSOutpostsAsync.CreateOrder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOrderAsync(CreateOrderRequest createOrderRequest);
/**
*
* Creates an order for an Outpost.
*
*
* @param createOrderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateOrder operation returned by the service.
* @sample AWSOutpostsAsyncHandler.CreateOrder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOrderAsync(CreateOrderRequest createOrderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Outpost.
*
*
* You can specify either an Availability one or an AZ ID.
*
*
* @param createOutpostRequest
* @return A Java Future containing the result of the CreateOutpost operation returned by the service.
* @sample AWSOutpostsAsync.CreateOutpost
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOutpostAsync(CreateOutpostRequest createOutpostRequest);
/**
*
* Creates an Outpost.
*
*
* You can specify either an Availability one or an AZ ID.
*
*
* @param createOutpostRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateOutpost operation returned by the service.
* @sample AWSOutpostsAsyncHandler.CreateOutpost
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOutpostAsync(CreateOutpostRequest createOutpostRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a site for an Outpost.
*
*
* @param createSiteRequest
* @return A Java Future containing the result of the CreateSite operation returned by the service.
* @sample AWSOutpostsAsync.CreateSite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSiteAsync(CreateSiteRequest createSiteRequest);
/**
*
* Creates a site for an Outpost.
*
*
* @param createSiteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSite operation returned by the service.
* @sample AWSOutpostsAsyncHandler.CreateSite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSiteAsync(CreateSiteRequest createSiteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Outpost.
*
*
* @param deleteOutpostRequest
* @return A Java Future containing the result of the DeleteOutpost operation returned by the service.
* @sample AWSOutpostsAsync.DeleteOutpost
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteOutpostAsync(DeleteOutpostRequest deleteOutpostRequest);
/**
*
* Deletes the specified Outpost.
*
*
* @param deleteOutpostRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOutpost operation returned by the service.
* @sample AWSOutpostsAsyncHandler.DeleteOutpost
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteOutpostAsync(DeleteOutpostRequest deleteOutpostRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified site.
*
*
* @param deleteSiteRequest
* @return A Java Future containing the result of the DeleteSite operation returned by the service.
* @sample AWSOutpostsAsync.DeleteSite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSiteAsync(DeleteSiteRequest deleteSiteRequest);
/**
*
* Deletes the specified site.
*
*
* @param deleteSiteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSite operation returned by the service.
* @sample AWSOutpostsAsyncHandler.DeleteSite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSiteAsync(DeleteSiteRequest deleteSiteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets details of the specified capacity task.
*
*
* @param getCapacityTaskRequest
* @return A Java Future containing the result of the GetCapacityTask operation returned by the service.
* @sample AWSOutpostsAsync.GetCapacityTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCapacityTaskAsync(GetCapacityTaskRequest getCapacityTaskRequest);
/**
*
* Gets details of the specified capacity task.
*
*
* @param getCapacityTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCapacityTask operation returned by the service.
* @sample AWSOutpostsAsyncHandler.GetCapacityTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCapacityTaskAsync(GetCapacityTaskRequest getCapacityTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified catalog item.
*
*
* @param getCatalogItemRequest
* @return A Java Future containing the result of the GetCatalogItem operation returned by the service.
* @sample AWSOutpostsAsync.GetCatalogItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCatalogItemAsync(GetCatalogItemRequest getCatalogItemRequest);
/**
*
* Gets information about the specified catalog item.
*
*
* @param getCatalogItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCatalogItem operation returned by the service.
* @sample AWSOutpostsAsyncHandler.GetCatalogItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCatalogItemAsync(GetCatalogItemRequest getCatalogItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* Amazon Web Services uses this action to install Outpost servers.
*
*
*
* Gets information about the specified connection.
*
*
* Use CloudTrail to monitor this action or Amazon Web Services managed policy for Amazon Web Services Outposts to
* secure it. For more information, see Amazon Web Services
* managed policies for Amazon Web Services Outposts and Logging Amazon Web
* Services Outposts API calls with Amazon Web Services CloudTrail in the Amazon Web Services Outposts User
* Guide.
*
*
* @param getConnectionRequest
* @return A Java Future containing the result of the GetConnection operation returned by the service.
* @sample AWSOutpostsAsync.GetConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getConnectionAsync(GetConnectionRequest getConnectionRequest);
/**
*
*
* Amazon Web Services uses this action to install Outpost servers.
*
*
*
* Gets information about the specified connection.
*
*
* Use CloudTrail to monitor this action or Amazon Web Services managed policy for Amazon Web Services Outposts to
* secure it. For more information, see Amazon Web Services
* managed policies for Amazon Web Services Outposts and Logging Amazon Web
* Services Outposts API calls with Amazon Web Services CloudTrail in the Amazon Web Services Outposts User
* Guide.
*
*
* @param getConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetConnection operation returned by the service.
* @sample AWSOutpostsAsyncHandler.GetConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getConnectionAsync(GetConnectionRequest getConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified order.
*
*
* @param getOrderRequest
* @return A Java Future containing the result of the GetOrder operation returned by the service.
* @sample AWSOutpostsAsync.GetOrder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOrderAsync(GetOrderRequest getOrderRequest);
/**
*
* Gets information about the specified order.
*
*
* @param getOrderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOrder operation returned by the service.
* @sample AWSOutpostsAsyncHandler.GetOrder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOrderAsync(GetOrderRequest getOrderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified Outpost.
*
*
* @param getOutpostRequest
* @return A Java Future containing the result of the GetOutpost operation returned by the service.
* @sample AWSOutpostsAsync.GetOutpost
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOutpostAsync(GetOutpostRequest getOutpostRequest);
/**
*
* Gets information about the specified Outpost.
*
*
* @param getOutpostRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOutpost operation returned by the service.
* @sample AWSOutpostsAsyncHandler.GetOutpost
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOutpostAsync(GetOutpostRequest getOutpostRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the instance types for the specified Outpost.
*
*
* @param getOutpostInstanceTypesRequest
* @return A Java Future containing the result of the GetOutpostInstanceTypes operation returned by the service.
* @sample AWSOutpostsAsync.GetOutpostInstanceTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future getOutpostInstanceTypesAsync(GetOutpostInstanceTypesRequest getOutpostInstanceTypesRequest);
/**
*
* Gets the instance types for the specified Outpost.
*
*
* @param getOutpostInstanceTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOutpostInstanceTypes operation returned by the service.
* @sample AWSOutpostsAsyncHandler.GetOutpostInstanceTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future getOutpostInstanceTypesAsync(GetOutpostInstanceTypesRequest getOutpostInstanceTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the instance types that an Outpost can support in InstanceTypeCapacity
. This will generally
* include instance types that are not currently configured and therefore cannot be launched with the current
* Outpost capacity configuration.
*
*
* @param getOutpostSupportedInstanceTypesRequest
* @return A Java Future containing the result of the GetOutpostSupportedInstanceTypes operation returned by the
* service.
* @sample AWSOutpostsAsync.GetOutpostSupportedInstanceTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future getOutpostSupportedInstanceTypesAsync(
GetOutpostSupportedInstanceTypesRequest getOutpostSupportedInstanceTypesRequest);
/**
*
* Gets the instance types that an Outpost can support in InstanceTypeCapacity
. This will generally
* include instance types that are not currently configured and therefore cannot be launched with the current
* Outpost capacity configuration.
*
*
* @param getOutpostSupportedInstanceTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOutpostSupportedInstanceTypes operation returned by the
* service.
* @sample AWSOutpostsAsyncHandler.GetOutpostSupportedInstanceTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future getOutpostSupportedInstanceTypesAsync(
GetOutpostSupportedInstanceTypesRequest getOutpostSupportedInstanceTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified Outpost site.
*
*
* @param getSiteRequest
* @return A Java Future containing the result of the GetSite operation returned by the service.
* @sample AWSOutpostsAsync.GetSite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSiteAsync(GetSiteRequest getSiteRequest);
/**
*
* Gets information about the specified Outpost site.
*
*
* @param getSiteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSite operation returned by the service.
* @sample AWSOutpostsAsyncHandler.GetSite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSiteAsync(GetSiteRequest getSiteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the site address of the specified site.
*
*
* @param getSiteAddressRequest
* @return A Java Future containing the result of the GetSiteAddress operation returned by the service.
* @sample AWSOutpostsAsync.GetSiteAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSiteAddressAsync(GetSiteAddressRequest getSiteAddressRequest);
/**
*
* Gets the site address of the specified site.
*
*
* @param getSiteAddressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSiteAddress operation returned by the service.
* @sample AWSOutpostsAsyncHandler.GetSiteAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSiteAddressAsync(GetSiteAddressRequest getSiteAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the hardware assets for the specified Outpost.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listAssetsRequest
* @return A Java Future containing the result of the ListAssets operation returned by the service.
* @sample AWSOutpostsAsync.ListAssets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAssetsAsync(ListAssetsRequest listAssetsRequest);
/**
*
* Lists the hardware assets for the specified Outpost.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listAssetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssets operation returned by the service.
* @sample AWSOutpostsAsyncHandler.ListAssets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAssetsAsync(ListAssetsRequest listAssetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the capacity tasks for your Amazon Web Services account.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listCapacityTasksRequest
* @return A Java Future containing the result of the ListCapacityTasks operation returned by the service.
* @sample AWSOutpostsAsync.ListCapacityTasks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCapacityTasksAsync(ListCapacityTasksRequest listCapacityTasksRequest);
/**
*
* Lists the capacity tasks for your Amazon Web Services account.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listCapacityTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCapacityTasks operation returned by the service.
* @sample AWSOutpostsAsyncHandler.ListCapacityTasks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCapacityTasksAsync(ListCapacityTasksRequest listCapacityTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the items in the catalog.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listCatalogItemsRequest
* @return A Java Future containing the result of the ListCatalogItems operation returned by the service.
* @sample AWSOutpostsAsync.ListCatalogItems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCatalogItemsAsync(ListCatalogItemsRequest listCatalogItemsRequest);
/**
*
* Lists the items in the catalog.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listCatalogItemsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCatalogItems operation returned by the service.
* @sample AWSOutpostsAsyncHandler.ListCatalogItems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCatalogItemsAsync(ListCatalogItemsRequest listCatalogItemsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Outpost orders for your Amazon Web Services account.
*
*
* @param listOrdersRequest
* @return A Java Future containing the result of the ListOrders operation returned by the service.
* @sample AWSOutpostsAsync.ListOrders
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOrdersAsync(ListOrdersRequest listOrdersRequest);
/**
*
* Lists the Outpost orders for your Amazon Web Services account.
*
*
* @param listOrdersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOrders operation returned by the service.
* @sample AWSOutpostsAsyncHandler.ListOrders
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOrdersAsync(ListOrdersRequest listOrdersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Outposts for your Amazon Web Services account.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listOutpostsRequest
* @return A Java Future containing the result of the ListOutposts operation returned by the service.
* @sample AWSOutpostsAsync.ListOutposts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOutpostsAsync(ListOutpostsRequest listOutpostsRequest);
/**
*
* Lists the Outposts for your Amazon Web Services account.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listOutpostsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOutposts operation returned by the service.
* @sample AWSOutpostsAsyncHandler.ListOutposts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOutpostsAsync(ListOutpostsRequest listOutpostsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Outpost sites for your Amazon Web Services account. Use filters to return specific results.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listSitesRequest
* @return A Java Future containing the result of the ListSites operation returned by the service.
* @sample AWSOutpostsAsync.ListSites
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSitesAsync(ListSitesRequest listSitesRequest);
/**
*
* Lists the Outpost sites for your Amazon Web Services account. Use filters to return specific results.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listSitesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSites operation returned by the service.
* @sample AWSOutpostsAsyncHandler.ListSites
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSitesAsync(ListSitesRequest listSitesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSOutpostsAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSOutpostsAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the specified capacity task. You can have one active capacity task for an order.
*
*
* @param startCapacityTaskRequest
* @return A Java Future containing the result of the StartCapacityTask operation returned by the service.
* @sample AWSOutpostsAsync.StartCapacityTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startCapacityTaskAsync(StartCapacityTaskRequest startCapacityTaskRequest);
/**
*
* Starts the specified capacity task. You can have one active capacity task for an order.
*
*
* @param startCapacityTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartCapacityTask operation returned by the service.
* @sample AWSOutpostsAsyncHandler.StartCapacityTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startCapacityTaskAsync(StartCapacityTaskRequest startCapacityTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* Amazon Web Services uses this action to install Outpost servers.
*
*
*
* Starts the connection required for Outpost server installation.
*
*
* Use CloudTrail to monitor this action or Amazon Web Services managed policy for Amazon Web Services Outposts to
* secure it. For more information, see Amazon Web Services
* managed policies for Amazon Web Services Outposts and Logging Amazon Web
* Services Outposts API calls with Amazon Web Services CloudTrail in the Amazon Web Services Outposts User
* Guide.
*
*
* @param startConnectionRequest
* @return A Java Future containing the result of the StartConnection operation returned by the service.
* @sample AWSOutpostsAsync.StartConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startConnectionAsync(StartConnectionRequest startConnectionRequest);
/**
*
*
* Amazon Web Services uses this action to install Outpost servers.
*
*
*
* Starts the connection required for Outpost server installation.
*
*
* Use CloudTrail to monitor this action or Amazon Web Services managed policy for Amazon Web Services Outposts to
* secure it. For more information, see Amazon Web Services
* managed policies for Amazon Web Services Outposts and Logging Amazon Web
* Services Outposts API calls with Amazon Web Services CloudTrail in the Amazon Web Services Outposts User
* Guide.
*
*
* @param startConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartConnection operation returned by the service.
* @sample AWSOutpostsAsyncHandler.StartConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startConnectionAsync(StartConnectionRequest startConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds tags to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSOutpostsAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds tags to the specified resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSOutpostsAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSOutpostsAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from the specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSOutpostsAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an Outpost.
*
*
* @param updateOutpostRequest
* @return A Java Future containing the result of the UpdateOutpost operation returned by the service.
* @sample AWSOutpostsAsync.UpdateOutpost
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateOutpostAsync(UpdateOutpostRequest updateOutpostRequest);
/**
*
* Updates an Outpost.
*
*
* @param updateOutpostRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateOutpost operation returned by the service.
* @sample AWSOutpostsAsyncHandler.UpdateOutpost
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateOutpostAsync(UpdateOutpostRequest updateOutpostRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified site.
*
*
* @param updateSiteRequest
* @return A Java Future containing the result of the UpdateSite operation returned by the service.
* @sample AWSOutpostsAsync.UpdateSite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSiteAsync(UpdateSiteRequest updateSiteRequest);
/**
*
* Updates the specified site.
*
*
* @param updateSiteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSite operation returned by the service.
* @sample AWSOutpostsAsyncHandler.UpdateSite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSiteAsync(UpdateSiteRequest updateSiteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the address of the specified site.
*
*
* You can't update a site address if there is an order in progress. You must wait for the order to complete or
* cancel the order.
*
*
* You can update the operating address before you place an order at the site, or after all Outposts that belong to
* the site have been deactivated.
*
*
* @param updateSiteAddressRequest
* @return A Java Future containing the result of the UpdateSiteAddress operation returned by the service.
* @sample AWSOutpostsAsync.UpdateSiteAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSiteAddressAsync(UpdateSiteAddressRequest updateSiteAddressRequest);
/**
*
* Updates the address of the specified site.
*
*
* You can't update a site address if there is an order in progress. You must wait for the order to complete or
* cancel the order.
*
*
* You can update the operating address before you place an order at the site, or after all Outposts that belong to
* the site have been deactivated.
*
*
* @param updateSiteAddressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSiteAddress operation returned by the service.
* @sample AWSOutpostsAsyncHandler.UpdateSiteAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSiteAddressAsync(UpdateSiteAddressRequest updateSiteAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update the physical and logistical details for a rack at a site. For more information about hardware requirements
* for racks, see Network
* readiness checklist in the Amazon Web Services Outposts User Guide.
*
*
* To update a rack at a site with an order of IN_PROGRESS
, you must wait for the order to complete or
* cancel the order.
*
*
* @param updateSiteRackPhysicalPropertiesRequest
* @return A Java Future containing the result of the UpdateSiteRackPhysicalProperties operation returned by the
* service.
* @sample AWSOutpostsAsync.UpdateSiteRackPhysicalProperties
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSiteRackPhysicalPropertiesAsync(
UpdateSiteRackPhysicalPropertiesRequest updateSiteRackPhysicalPropertiesRequest);
/**
*
* Update the physical and logistical details for a rack at a site. For more information about hardware requirements
* for racks, see Network
* readiness checklist in the Amazon Web Services Outposts User Guide.
*
*
* To update a rack at a site with an order of IN_PROGRESS
, you must wait for the order to complete or
* cancel the order.
*
*
* @param updateSiteRackPhysicalPropertiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSiteRackPhysicalProperties operation returned by the
* service.
* @sample AWSOutpostsAsyncHandler.UpdateSiteRackPhysicalProperties
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSiteRackPhysicalPropertiesAsync(
UpdateSiteRackPhysicalPropertiesRequest updateSiteRackPhysicalPropertiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}