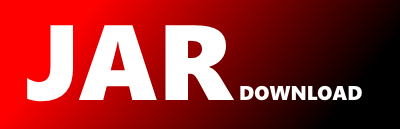
com.amazonaws.services.outposts.model.CatalogItem Maven / Gradle / Ivy
Show all versions of aws-java-sdk-outposts Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.outposts.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about a catalog item.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CatalogItem implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the catalog item.
*
*/
private String catalogItemId;
/**
*
* The status of a catalog item.
*
*/
private String itemStatus;
/**
*
* Information about the EC2 capacity of an item.
*
*/
private java.util.List eC2Capacities;
/**
*
* Information about the power draw of an item.
*
*/
private Float powerKva;
/**
*
* The weight of the item in pounds.
*
*/
private Integer weightLbs;
/**
*
* The uplink speed this catalog item requires for the connection to the Region.
*
*/
private java.util.List supportedUplinkGbps;
/**
*
* The supported storage options for the catalog item.
*
*/
private java.util.List supportedStorage;
/**
*
* The ID of the catalog item.
*
*
* @param catalogItemId
* The ID of the catalog item.
*/
public void setCatalogItemId(String catalogItemId) {
this.catalogItemId = catalogItemId;
}
/**
*
* The ID of the catalog item.
*
*
* @return The ID of the catalog item.
*/
public String getCatalogItemId() {
return this.catalogItemId;
}
/**
*
* The ID of the catalog item.
*
*
* @param catalogItemId
* The ID of the catalog item.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CatalogItem withCatalogItemId(String catalogItemId) {
setCatalogItemId(catalogItemId);
return this;
}
/**
*
* The status of a catalog item.
*
*
* @param itemStatus
* The status of a catalog item.
* @see CatalogItemStatus
*/
public void setItemStatus(String itemStatus) {
this.itemStatus = itemStatus;
}
/**
*
* The status of a catalog item.
*
*
* @return The status of a catalog item.
* @see CatalogItemStatus
*/
public String getItemStatus() {
return this.itemStatus;
}
/**
*
* The status of a catalog item.
*
*
* @param itemStatus
* The status of a catalog item.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CatalogItemStatus
*/
public CatalogItem withItemStatus(String itemStatus) {
setItemStatus(itemStatus);
return this;
}
/**
*
* The status of a catalog item.
*
*
* @param itemStatus
* The status of a catalog item.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CatalogItemStatus
*/
public CatalogItem withItemStatus(CatalogItemStatus itemStatus) {
this.itemStatus = itemStatus.toString();
return this;
}
/**
*
* Information about the EC2 capacity of an item.
*
*
* @return Information about the EC2 capacity of an item.
*/
public java.util.List getEC2Capacities() {
return eC2Capacities;
}
/**
*
* Information about the EC2 capacity of an item.
*
*
* @param eC2Capacities
* Information about the EC2 capacity of an item.
*/
public void setEC2Capacities(java.util.Collection eC2Capacities) {
if (eC2Capacities == null) {
this.eC2Capacities = null;
return;
}
this.eC2Capacities = new java.util.ArrayList(eC2Capacities);
}
/**
*
* Information about the EC2 capacity of an item.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEC2Capacities(java.util.Collection)} or {@link #withEC2Capacities(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param eC2Capacities
* Information about the EC2 capacity of an item.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CatalogItem withEC2Capacities(EC2Capacity... eC2Capacities) {
if (this.eC2Capacities == null) {
setEC2Capacities(new java.util.ArrayList(eC2Capacities.length));
}
for (EC2Capacity ele : eC2Capacities) {
this.eC2Capacities.add(ele);
}
return this;
}
/**
*
* Information about the EC2 capacity of an item.
*
*
* @param eC2Capacities
* Information about the EC2 capacity of an item.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CatalogItem withEC2Capacities(java.util.Collection eC2Capacities) {
setEC2Capacities(eC2Capacities);
return this;
}
/**
*
* Information about the power draw of an item.
*
*
* @param powerKva
* Information about the power draw of an item.
*/
public void setPowerKva(Float powerKva) {
this.powerKva = powerKva;
}
/**
*
* Information about the power draw of an item.
*
*
* @return Information about the power draw of an item.
*/
public Float getPowerKva() {
return this.powerKva;
}
/**
*
* Information about the power draw of an item.
*
*
* @param powerKva
* Information about the power draw of an item.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CatalogItem withPowerKva(Float powerKva) {
setPowerKva(powerKva);
return this;
}
/**
*
* The weight of the item in pounds.
*
*
* @param weightLbs
* The weight of the item in pounds.
*/
public void setWeightLbs(Integer weightLbs) {
this.weightLbs = weightLbs;
}
/**
*
* The weight of the item in pounds.
*
*
* @return The weight of the item in pounds.
*/
public Integer getWeightLbs() {
return this.weightLbs;
}
/**
*
* The weight of the item in pounds.
*
*
* @param weightLbs
* The weight of the item in pounds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CatalogItem withWeightLbs(Integer weightLbs) {
setWeightLbs(weightLbs);
return this;
}
/**
*
* The uplink speed this catalog item requires for the connection to the Region.
*
*
* @return The uplink speed this catalog item requires for the connection to the Region.
*/
public java.util.List getSupportedUplinkGbps() {
return supportedUplinkGbps;
}
/**
*
* The uplink speed this catalog item requires for the connection to the Region.
*
*
* @param supportedUplinkGbps
* The uplink speed this catalog item requires for the connection to the Region.
*/
public void setSupportedUplinkGbps(java.util.Collection supportedUplinkGbps) {
if (supportedUplinkGbps == null) {
this.supportedUplinkGbps = null;
return;
}
this.supportedUplinkGbps = new java.util.ArrayList(supportedUplinkGbps);
}
/**
*
* The uplink speed this catalog item requires for the connection to the Region.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedUplinkGbps(java.util.Collection)} or {@link #withSupportedUplinkGbps(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param supportedUplinkGbps
* The uplink speed this catalog item requires for the connection to the Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CatalogItem withSupportedUplinkGbps(Integer... supportedUplinkGbps) {
if (this.supportedUplinkGbps == null) {
setSupportedUplinkGbps(new java.util.ArrayList(supportedUplinkGbps.length));
}
for (Integer ele : supportedUplinkGbps) {
this.supportedUplinkGbps.add(ele);
}
return this;
}
/**
*
* The uplink speed this catalog item requires for the connection to the Region.
*
*
* @param supportedUplinkGbps
* The uplink speed this catalog item requires for the connection to the Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CatalogItem withSupportedUplinkGbps(java.util.Collection supportedUplinkGbps) {
setSupportedUplinkGbps(supportedUplinkGbps);
return this;
}
/**
*
* The supported storage options for the catalog item.
*
*
* @return The supported storage options for the catalog item.
* @see SupportedStorageEnum
*/
public java.util.List getSupportedStorage() {
return supportedStorage;
}
/**
*
* The supported storage options for the catalog item.
*
*
* @param supportedStorage
* The supported storage options for the catalog item.
* @see SupportedStorageEnum
*/
public void setSupportedStorage(java.util.Collection supportedStorage) {
if (supportedStorage == null) {
this.supportedStorage = null;
return;
}
this.supportedStorage = new java.util.ArrayList(supportedStorage);
}
/**
*
* The supported storage options for the catalog item.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedStorage(java.util.Collection)} or {@link #withSupportedStorage(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param supportedStorage
* The supported storage options for the catalog item.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SupportedStorageEnum
*/
public CatalogItem withSupportedStorage(String... supportedStorage) {
if (this.supportedStorage == null) {
setSupportedStorage(new java.util.ArrayList(supportedStorage.length));
}
for (String ele : supportedStorage) {
this.supportedStorage.add(ele);
}
return this;
}
/**
*
* The supported storage options for the catalog item.
*
*
* @param supportedStorage
* The supported storage options for the catalog item.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SupportedStorageEnum
*/
public CatalogItem withSupportedStorage(java.util.Collection supportedStorage) {
setSupportedStorage(supportedStorage);
return this;
}
/**
*
* The supported storage options for the catalog item.
*
*
* @param supportedStorage
* The supported storage options for the catalog item.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SupportedStorageEnum
*/
public CatalogItem withSupportedStorage(SupportedStorageEnum... supportedStorage) {
java.util.ArrayList supportedStorageCopy = new java.util.ArrayList(supportedStorage.length);
for (SupportedStorageEnum value : supportedStorage) {
supportedStorageCopy.add(value.toString());
}
if (getSupportedStorage() == null) {
setSupportedStorage(supportedStorageCopy);
} else {
getSupportedStorage().addAll(supportedStorageCopy);
}
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCatalogItemId() != null)
sb.append("CatalogItemId: ").append(getCatalogItemId()).append(",");
if (getItemStatus() != null)
sb.append("ItemStatus: ").append(getItemStatus()).append(",");
if (getEC2Capacities() != null)
sb.append("EC2Capacities: ").append(getEC2Capacities()).append(",");
if (getPowerKva() != null)
sb.append("PowerKva: ").append(getPowerKva()).append(",");
if (getWeightLbs() != null)
sb.append("WeightLbs: ").append(getWeightLbs()).append(",");
if (getSupportedUplinkGbps() != null)
sb.append("SupportedUplinkGbps: ").append(getSupportedUplinkGbps()).append(",");
if (getSupportedStorage() != null)
sb.append("SupportedStorage: ").append(getSupportedStorage());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CatalogItem == false)
return false;
CatalogItem other = (CatalogItem) obj;
if (other.getCatalogItemId() == null ^ this.getCatalogItemId() == null)
return false;
if (other.getCatalogItemId() != null && other.getCatalogItemId().equals(this.getCatalogItemId()) == false)
return false;
if (other.getItemStatus() == null ^ this.getItemStatus() == null)
return false;
if (other.getItemStatus() != null && other.getItemStatus().equals(this.getItemStatus()) == false)
return false;
if (other.getEC2Capacities() == null ^ this.getEC2Capacities() == null)
return false;
if (other.getEC2Capacities() != null && other.getEC2Capacities().equals(this.getEC2Capacities()) == false)
return false;
if (other.getPowerKva() == null ^ this.getPowerKva() == null)
return false;
if (other.getPowerKva() != null && other.getPowerKva().equals(this.getPowerKva()) == false)
return false;
if (other.getWeightLbs() == null ^ this.getWeightLbs() == null)
return false;
if (other.getWeightLbs() != null && other.getWeightLbs().equals(this.getWeightLbs()) == false)
return false;
if (other.getSupportedUplinkGbps() == null ^ this.getSupportedUplinkGbps() == null)
return false;
if (other.getSupportedUplinkGbps() != null && other.getSupportedUplinkGbps().equals(this.getSupportedUplinkGbps()) == false)
return false;
if (other.getSupportedStorage() == null ^ this.getSupportedStorage() == null)
return false;
if (other.getSupportedStorage() != null && other.getSupportedStorage().equals(this.getSupportedStorage()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCatalogItemId() == null) ? 0 : getCatalogItemId().hashCode());
hashCode = prime * hashCode + ((getItemStatus() == null) ? 0 : getItemStatus().hashCode());
hashCode = prime * hashCode + ((getEC2Capacities() == null) ? 0 : getEC2Capacities().hashCode());
hashCode = prime * hashCode + ((getPowerKva() == null) ? 0 : getPowerKva().hashCode());
hashCode = prime * hashCode + ((getWeightLbs() == null) ? 0 : getWeightLbs().hashCode());
hashCode = prime * hashCode + ((getSupportedUplinkGbps() == null) ? 0 : getSupportedUplinkGbps().hashCode());
hashCode = prime * hashCode + ((getSupportedStorage() == null) ? 0 : getSupportedStorage().hashCode());
return hashCode;
}
@Override
public CatalogItem clone() {
try {
return (CatalogItem) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.outposts.model.transform.CatalogItemMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}