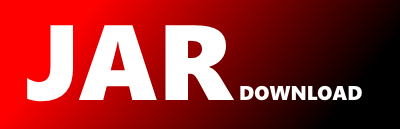
com.amazonaws.services.outposts.model.ListSitesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-outposts Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.outposts.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListSitesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
private String nextToken;
private Integer maxResults;
/**
*
* Filters the results by country code.
*
*/
private java.util.List operatingAddressCountryCodeFilter;
/**
*
* Filters the results by state or region.
*
*/
private java.util.List operatingAddressStateOrRegionFilter;
/**
*
* Filters the results by city.
*
*/
private java.util.List operatingAddressCityFilter;
/**
* @param nextToken
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
* @return
*/
public String getNextToken() {
return this.nextToken;
}
/**
* @param nextToken
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSitesRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
* @param maxResults
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
* @return
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
* @param maxResults
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSitesRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* Filters the results by country code.
*
*
* @return Filters the results by country code.
*/
public java.util.List getOperatingAddressCountryCodeFilter() {
return operatingAddressCountryCodeFilter;
}
/**
*
* Filters the results by country code.
*
*
* @param operatingAddressCountryCodeFilter
* Filters the results by country code.
*/
public void setOperatingAddressCountryCodeFilter(java.util.Collection operatingAddressCountryCodeFilter) {
if (operatingAddressCountryCodeFilter == null) {
this.operatingAddressCountryCodeFilter = null;
return;
}
this.operatingAddressCountryCodeFilter = new java.util.ArrayList(operatingAddressCountryCodeFilter);
}
/**
*
* Filters the results by country code.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOperatingAddressCountryCodeFilter(java.util.Collection)} or
* {@link #withOperatingAddressCountryCodeFilter(java.util.Collection)} if you want to override the existing values.
*
*
* @param operatingAddressCountryCodeFilter
* Filters the results by country code.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSitesRequest withOperatingAddressCountryCodeFilter(String... operatingAddressCountryCodeFilter) {
if (this.operatingAddressCountryCodeFilter == null) {
setOperatingAddressCountryCodeFilter(new java.util.ArrayList(operatingAddressCountryCodeFilter.length));
}
for (String ele : operatingAddressCountryCodeFilter) {
this.operatingAddressCountryCodeFilter.add(ele);
}
return this;
}
/**
*
* Filters the results by country code.
*
*
* @param operatingAddressCountryCodeFilter
* Filters the results by country code.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSitesRequest withOperatingAddressCountryCodeFilter(java.util.Collection operatingAddressCountryCodeFilter) {
setOperatingAddressCountryCodeFilter(operatingAddressCountryCodeFilter);
return this;
}
/**
*
* Filters the results by state or region.
*
*
* @return Filters the results by state or region.
*/
public java.util.List getOperatingAddressStateOrRegionFilter() {
return operatingAddressStateOrRegionFilter;
}
/**
*
* Filters the results by state or region.
*
*
* @param operatingAddressStateOrRegionFilter
* Filters the results by state or region.
*/
public void setOperatingAddressStateOrRegionFilter(java.util.Collection operatingAddressStateOrRegionFilter) {
if (operatingAddressStateOrRegionFilter == null) {
this.operatingAddressStateOrRegionFilter = null;
return;
}
this.operatingAddressStateOrRegionFilter = new java.util.ArrayList(operatingAddressStateOrRegionFilter);
}
/**
*
* Filters the results by state or region.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOperatingAddressStateOrRegionFilter(java.util.Collection)} or
* {@link #withOperatingAddressStateOrRegionFilter(java.util.Collection)} if you want to override the existing
* values.
*
*
* @param operatingAddressStateOrRegionFilter
* Filters the results by state or region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSitesRequest withOperatingAddressStateOrRegionFilter(String... operatingAddressStateOrRegionFilter) {
if (this.operatingAddressStateOrRegionFilter == null) {
setOperatingAddressStateOrRegionFilter(new java.util.ArrayList(operatingAddressStateOrRegionFilter.length));
}
for (String ele : operatingAddressStateOrRegionFilter) {
this.operatingAddressStateOrRegionFilter.add(ele);
}
return this;
}
/**
*
* Filters the results by state or region.
*
*
* @param operatingAddressStateOrRegionFilter
* Filters the results by state or region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSitesRequest withOperatingAddressStateOrRegionFilter(java.util.Collection operatingAddressStateOrRegionFilter) {
setOperatingAddressStateOrRegionFilter(operatingAddressStateOrRegionFilter);
return this;
}
/**
*
* Filters the results by city.
*
*
* @return Filters the results by city.
*/
public java.util.List getOperatingAddressCityFilter() {
return operatingAddressCityFilter;
}
/**
*
* Filters the results by city.
*
*
* @param operatingAddressCityFilter
* Filters the results by city.
*/
public void setOperatingAddressCityFilter(java.util.Collection operatingAddressCityFilter) {
if (operatingAddressCityFilter == null) {
this.operatingAddressCityFilter = null;
return;
}
this.operatingAddressCityFilter = new java.util.ArrayList(operatingAddressCityFilter);
}
/**
*
* Filters the results by city.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOperatingAddressCityFilter(java.util.Collection)} or
* {@link #withOperatingAddressCityFilter(java.util.Collection)} if you want to override the existing values.
*
*
* @param operatingAddressCityFilter
* Filters the results by city.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSitesRequest withOperatingAddressCityFilter(String... operatingAddressCityFilter) {
if (this.operatingAddressCityFilter == null) {
setOperatingAddressCityFilter(new java.util.ArrayList(operatingAddressCityFilter.length));
}
for (String ele : operatingAddressCityFilter) {
this.operatingAddressCityFilter.add(ele);
}
return this;
}
/**
*
* Filters the results by city.
*
*
* @param operatingAddressCityFilter
* Filters the results by city.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSitesRequest withOperatingAddressCityFilter(java.util.Collection operatingAddressCityFilter) {
setOperatingAddressCityFilter(operatingAddressCityFilter);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getOperatingAddressCountryCodeFilter() != null)
sb.append("OperatingAddressCountryCodeFilter: ").append(getOperatingAddressCountryCodeFilter()).append(",");
if (getOperatingAddressStateOrRegionFilter() != null)
sb.append("OperatingAddressStateOrRegionFilter: ").append(getOperatingAddressStateOrRegionFilter()).append(",");
if (getOperatingAddressCityFilter() != null)
sb.append("OperatingAddressCityFilter: ").append(getOperatingAddressCityFilter());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListSitesRequest == false)
return false;
ListSitesRequest other = (ListSitesRequest) obj;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getOperatingAddressCountryCodeFilter() == null ^ this.getOperatingAddressCountryCodeFilter() == null)
return false;
if (other.getOperatingAddressCountryCodeFilter() != null
&& other.getOperatingAddressCountryCodeFilter().equals(this.getOperatingAddressCountryCodeFilter()) == false)
return false;
if (other.getOperatingAddressStateOrRegionFilter() == null ^ this.getOperatingAddressStateOrRegionFilter() == null)
return false;
if (other.getOperatingAddressStateOrRegionFilter() != null
&& other.getOperatingAddressStateOrRegionFilter().equals(this.getOperatingAddressStateOrRegionFilter()) == false)
return false;
if (other.getOperatingAddressCityFilter() == null ^ this.getOperatingAddressCityFilter() == null)
return false;
if (other.getOperatingAddressCityFilter() != null && other.getOperatingAddressCityFilter().equals(this.getOperatingAddressCityFilter()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getOperatingAddressCountryCodeFilter() == null) ? 0 : getOperatingAddressCountryCodeFilter().hashCode());
hashCode = prime * hashCode + ((getOperatingAddressStateOrRegionFilter() == null) ? 0 : getOperatingAddressStateOrRegionFilter().hashCode());
hashCode = prime * hashCode + ((getOperatingAddressCityFilter() == null) ? 0 : getOperatingAddressCityFilter().hashCode());
return hashCode;
}
@Override
public ListSitesRequest clone() {
return (ListSitesRequest) super.clone();
}
}