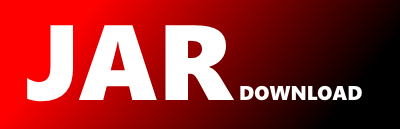
com.amazonaws.services.outposts.model.Order Maven / Gradle / Ivy
Show all versions of aws-java-sdk-outposts Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.outposts.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about an order.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Order implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the Outpost in the order.
*
*/
private String outpostId;
/**
*
* The ID of the order.
*
*/
private String orderId;
/**
*
* The status of the order.
*
*
* -
*
* PREPARING
- Order is received and being prepared.
*
*
* -
*
* IN_PROGRESS
- Order is either being built, shipped, or installed. To get more details, see the line
* item status.
*
*
* -
*
* COMPLETED
- Order is complete.
*
*
* -
*
* CANCELLED
- Order is cancelled.
*
*
* -
*
* ERROR
- Customer should contact support.
*
*
*
*
*
* The following status are deprecated: RECEIVED
, PENDING
, PROCESSING
,
* INSTALLING
, and FULFILLED
.
*
*
*/
private String status;
/**
*
* The line items for the order
*
*/
private java.util.List lineItems;
/**
*
* The payment option for the order.
*
*/
private String paymentOption;
/**
*
* The submission date for the order.
*
*/
private java.util.Date orderSubmissionDate;
/**
*
* The fulfillment date of the order.
*
*/
private java.util.Date orderFulfilledDate;
/**
*
* The payment term.
*
*/
private String paymentTerm;
/**
*
* The type of order.
*
*/
private String orderType;
/**
*
* The ID of the Outpost in the order.
*
*
* @param outpostId
* The ID of the Outpost in the order.
*/
public void setOutpostId(String outpostId) {
this.outpostId = outpostId;
}
/**
*
* The ID of the Outpost in the order.
*
*
* @return The ID of the Outpost in the order.
*/
public String getOutpostId() {
return this.outpostId;
}
/**
*
* The ID of the Outpost in the order.
*
*
* @param outpostId
* The ID of the Outpost in the order.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Order withOutpostId(String outpostId) {
setOutpostId(outpostId);
return this;
}
/**
*
* The ID of the order.
*
*
* @param orderId
* The ID of the order.
*/
public void setOrderId(String orderId) {
this.orderId = orderId;
}
/**
*
* The ID of the order.
*
*
* @return The ID of the order.
*/
public String getOrderId() {
return this.orderId;
}
/**
*
* The ID of the order.
*
*
* @param orderId
* The ID of the order.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Order withOrderId(String orderId) {
setOrderId(orderId);
return this;
}
/**
*
* The status of the order.
*
*
* -
*
* PREPARING
- Order is received and being prepared.
*
*
* -
*
* IN_PROGRESS
- Order is either being built, shipped, or installed. To get more details, see the line
* item status.
*
*
* -
*
* COMPLETED
- Order is complete.
*
*
* -
*
* CANCELLED
- Order is cancelled.
*
*
* -
*
* ERROR
- Customer should contact support.
*
*
*
*
*
* The following status are deprecated: RECEIVED
, PENDING
, PROCESSING
,
* INSTALLING
, and FULFILLED
.
*
*
*
* @param status
* The status of the order.
*
* -
*
* PREPARING
- Order is received and being prepared.
*
*
* -
*
* IN_PROGRESS
- Order is either being built, shipped, or installed. To get more details, see
* the line item status.
*
*
* -
*
* COMPLETED
- Order is complete.
*
*
* -
*
* CANCELLED
- Order is cancelled.
*
*
* -
*
* ERROR
- Customer should contact support.
*
*
*
*
*
* The following status are deprecated: RECEIVED
, PENDING
, PROCESSING
,
* INSTALLING
, and FULFILLED
.
*
* @see OrderStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the order.
*
*
* -
*
* PREPARING
- Order is received and being prepared.
*
*
* -
*
* IN_PROGRESS
- Order is either being built, shipped, or installed. To get more details, see the line
* item status.
*
*
* -
*
* COMPLETED
- Order is complete.
*
*
* -
*
* CANCELLED
- Order is cancelled.
*
*
* -
*
* ERROR
- Customer should contact support.
*
*
*
*
*
* The following status are deprecated: RECEIVED
, PENDING
, PROCESSING
,
* INSTALLING
, and FULFILLED
.
*
*
*
* @return The status of the order.
*
* -
*
* PREPARING
- Order is received and being prepared.
*
*
* -
*
* IN_PROGRESS
- Order is either being built, shipped, or installed. To get more details, see
* the line item status.
*
*
* -
*
* COMPLETED
- Order is complete.
*
*
* -
*
* CANCELLED
- Order is cancelled.
*
*
* -
*
* ERROR
- Customer should contact support.
*
*
*
*
*
* The following status are deprecated: RECEIVED
, PENDING
, PROCESSING
, INSTALLING
, and FULFILLED
.
*
* @see OrderStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the order.
*
*
* -
*
* PREPARING
- Order is received and being prepared.
*
*
* -
*
* IN_PROGRESS
- Order is either being built, shipped, or installed. To get more details, see the line
* item status.
*
*
* -
*
* COMPLETED
- Order is complete.
*
*
* -
*
* CANCELLED
- Order is cancelled.
*
*
* -
*
* ERROR
- Customer should contact support.
*
*
*
*
*
* The following status are deprecated: RECEIVED
, PENDING
, PROCESSING
,
* INSTALLING
, and FULFILLED
.
*
*
*
* @param status
* The status of the order.
*
* -
*
* PREPARING
- Order is received and being prepared.
*
*
* -
*
* IN_PROGRESS
- Order is either being built, shipped, or installed. To get more details, see
* the line item status.
*
*
* -
*
* COMPLETED
- Order is complete.
*
*
* -
*
* CANCELLED
- Order is cancelled.
*
*
* -
*
* ERROR
- Customer should contact support.
*
*
*
*
*
* The following status are deprecated: RECEIVED
, PENDING
, PROCESSING
,
* INSTALLING
, and FULFILLED
.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OrderStatus
*/
public Order withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the order.
*
*
* -
*
* PREPARING
- Order is received and being prepared.
*
*
* -
*
* IN_PROGRESS
- Order is either being built, shipped, or installed. To get more details, see the line
* item status.
*
*
* -
*
* COMPLETED
- Order is complete.
*
*
* -
*
* CANCELLED
- Order is cancelled.
*
*
* -
*
* ERROR
- Customer should contact support.
*
*
*
*
*
* The following status are deprecated: RECEIVED
, PENDING
, PROCESSING
,
* INSTALLING
, and FULFILLED
.
*
*
*
* @param status
* The status of the order.
*
* -
*
* PREPARING
- Order is received and being prepared.
*
*
* -
*
* IN_PROGRESS
- Order is either being built, shipped, or installed. To get more details, see
* the line item status.
*
*
* -
*
* COMPLETED
- Order is complete.
*
*
* -
*
* CANCELLED
- Order is cancelled.
*
*
* -
*
* ERROR
- Customer should contact support.
*
*
*
*
*
* The following status are deprecated: RECEIVED
, PENDING
, PROCESSING
,
* INSTALLING
, and FULFILLED
.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OrderStatus
*/
public Order withStatus(OrderStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The line items for the order
*
*
* @return The line items for the order
*/
public java.util.List getLineItems() {
return lineItems;
}
/**
*
* The line items for the order
*
*
* @param lineItems
* The line items for the order
*/
public void setLineItems(java.util.Collection lineItems) {
if (lineItems == null) {
this.lineItems = null;
return;
}
this.lineItems = new java.util.ArrayList(lineItems);
}
/**
*
* The line items for the order
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLineItems(java.util.Collection)} or {@link #withLineItems(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param lineItems
* The line items for the order
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Order withLineItems(LineItem... lineItems) {
if (this.lineItems == null) {
setLineItems(new java.util.ArrayList(lineItems.length));
}
for (LineItem ele : lineItems) {
this.lineItems.add(ele);
}
return this;
}
/**
*
* The line items for the order
*
*
* @param lineItems
* The line items for the order
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Order withLineItems(java.util.Collection lineItems) {
setLineItems(lineItems);
return this;
}
/**
*
* The payment option for the order.
*
*
* @param paymentOption
* The payment option for the order.
* @see PaymentOption
*/
public void setPaymentOption(String paymentOption) {
this.paymentOption = paymentOption;
}
/**
*
* The payment option for the order.
*
*
* @return The payment option for the order.
* @see PaymentOption
*/
public String getPaymentOption() {
return this.paymentOption;
}
/**
*
* The payment option for the order.
*
*
* @param paymentOption
* The payment option for the order.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PaymentOption
*/
public Order withPaymentOption(String paymentOption) {
setPaymentOption(paymentOption);
return this;
}
/**
*
* The payment option for the order.
*
*
* @param paymentOption
* The payment option for the order.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PaymentOption
*/
public Order withPaymentOption(PaymentOption paymentOption) {
this.paymentOption = paymentOption.toString();
return this;
}
/**
*
* The submission date for the order.
*
*
* @param orderSubmissionDate
* The submission date for the order.
*/
public void setOrderSubmissionDate(java.util.Date orderSubmissionDate) {
this.orderSubmissionDate = orderSubmissionDate;
}
/**
*
* The submission date for the order.
*
*
* @return The submission date for the order.
*/
public java.util.Date getOrderSubmissionDate() {
return this.orderSubmissionDate;
}
/**
*
* The submission date for the order.
*
*
* @param orderSubmissionDate
* The submission date for the order.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Order withOrderSubmissionDate(java.util.Date orderSubmissionDate) {
setOrderSubmissionDate(orderSubmissionDate);
return this;
}
/**
*
* The fulfillment date of the order.
*
*
* @param orderFulfilledDate
* The fulfillment date of the order.
*/
public void setOrderFulfilledDate(java.util.Date orderFulfilledDate) {
this.orderFulfilledDate = orderFulfilledDate;
}
/**
*
* The fulfillment date of the order.
*
*
* @return The fulfillment date of the order.
*/
public java.util.Date getOrderFulfilledDate() {
return this.orderFulfilledDate;
}
/**
*
* The fulfillment date of the order.
*
*
* @param orderFulfilledDate
* The fulfillment date of the order.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Order withOrderFulfilledDate(java.util.Date orderFulfilledDate) {
setOrderFulfilledDate(orderFulfilledDate);
return this;
}
/**
*
* The payment term.
*
*
* @param paymentTerm
* The payment term.
* @see PaymentTerm
*/
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
/**
*
* The payment term.
*
*
* @return The payment term.
* @see PaymentTerm
*/
public String getPaymentTerm() {
return this.paymentTerm;
}
/**
*
* The payment term.
*
*
* @param paymentTerm
* The payment term.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PaymentTerm
*/
public Order withPaymentTerm(String paymentTerm) {
setPaymentTerm(paymentTerm);
return this;
}
/**
*
* The payment term.
*
*
* @param paymentTerm
* The payment term.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PaymentTerm
*/
public Order withPaymentTerm(PaymentTerm paymentTerm) {
this.paymentTerm = paymentTerm.toString();
return this;
}
/**
*
* The type of order.
*
*
* @param orderType
* The type of order.
* @see OrderType
*/
public void setOrderType(String orderType) {
this.orderType = orderType;
}
/**
*
* The type of order.
*
*
* @return The type of order.
* @see OrderType
*/
public String getOrderType() {
return this.orderType;
}
/**
*
* The type of order.
*
*
* @param orderType
* The type of order.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OrderType
*/
public Order withOrderType(String orderType) {
setOrderType(orderType);
return this;
}
/**
*
* The type of order.
*
*
* @param orderType
* The type of order.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OrderType
*/
public Order withOrderType(OrderType orderType) {
this.orderType = orderType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOutpostId() != null)
sb.append("OutpostId: ").append(getOutpostId()).append(",");
if (getOrderId() != null)
sb.append("OrderId: ").append(getOrderId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getLineItems() != null)
sb.append("LineItems: ").append(getLineItems()).append(",");
if (getPaymentOption() != null)
sb.append("PaymentOption: ").append(getPaymentOption()).append(",");
if (getOrderSubmissionDate() != null)
sb.append("OrderSubmissionDate: ").append(getOrderSubmissionDate()).append(",");
if (getOrderFulfilledDate() != null)
sb.append("OrderFulfilledDate: ").append(getOrderFulfilledDate()).append(",");
if (getPaymentTerm() != null)
sb.append("PaymentTerm: ").append(getPaymentTerm()).append(",");
if (getOrderType() != null)
sb.append("OrderType: ").append(getOrderType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Order == false)
return false;
Order other = (Order) obj;
if (other.getOutpostId() == null ^ this.getOutpostId() == null)
return false;
if (other.getOutpostId() != null && other.getOutpostId().equals(this.getOutpostId()) == false)
return false;
if (other.getOrderId() == null ^ this.getOrderId() == null)
return false;
if (other.getOrderId() != null && other.getOrderId().equals(this.getOrderId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getLineItems() == null ^ this.getLineItems() == null)
return false;
if (other.getLineItems() != null && other.getLineItems().equals(this.getLineItems()) == false)
return false;
if (other.getPaymentOption() == null ^ this.getPaymentOption() == null)
return false;
if (other.getPaymentOption() != null && other.getPaymentOption().equals(this.getPaymentOption()) == false)
return false;
if (other.getOrderSubmissionDate() == null ^ this.getOrderSubmissionDate() == null)
return false;
if (other.getOrderSubmissionDate() != null && other.getOrderSubmissionDate().equals(this.getOrderSubmissionDate()) == false)
return false;
if (other.getOrderFulfilledDate() == null ^ this.getOrderFulfilledDate() == null)
return false;
if (other.getOrderFulfilledDate() != null && other.getOrderFulfilledDate().equals(this.getOrderFulfilledDate()) == false)
return false;
if (other.getPaymentTerm() == null ^ this.getPaymentTerm() == null)
return false;
if (other.getPaymentTerm() != null && other.getPaymentTerm().equals(this.getPaymentTerm()) == false)
return false;
if (other.getOrderType() == null ^ this.getOrderType() == null)
return false;
if (other.getOrderType() != null && other.getOrderType().equals(this.getOrderType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOutpostId() == null) ? 0 : getOutpostId().hashCode());
hashCode = prime * hashCode + ((getOrderId() == null) ? 0 : getOrderId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getLineItems() == null) ? 0 : getLineItems().hashCode());
hashCode = prime * hashCode + ((getPaymentOption() == null) ? 0 : getPaymentOption().hashCode());
hashCode = prime * hashCode + ((getOrderSubmissionDate() == null) ? 0 : getOrderSubmissionDate().hashCode());
hashCode = prime * hashCode + ((getOrderFulfilledDate() == null) ? 0 : getOrderFulfilledDate().hashCode());
hashCode = prime * hashCode + ((getPaymentTerm() == null) ? 0 : getPaymentTerm().hashCode());
hashCode = prime * hashCode + ((getOrderType() == null) ? 0 : getOrderType().hashCode());
return hashCode;
}
@Override
public Order clone() {
try {
return (Order) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.outposts.model.transform.OrderMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}