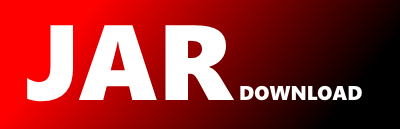
com.amazonaws.services.outposts.model.UpdateSiteRackPhysicalPropertiesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-outposts Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.outposts.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateSiteRackPhysicalPropertiesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID or the Amazon Resource Name (ARN) of the site.
*
*/
private String siteId;
/**
*
* The power draw, in kVA, available at the hardware placement position for the rack.
*
*/
private String powerDrawKva;
/**
*
* The power option that you can provide for hardware.
*
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
*
*/
private String powerPhase;
/**
*
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note the
* correlation between PowerPhase
and PowerConnector
.
*
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
*
*/
private String powerConnector;
/**
*
* Indicates whether the power feed comes above or below the rack.
*
*/
private String powerFeedDrop;
/**
*
* The uplink speed the rack should support for the connection to the Region.
*
*/
private String uplinkGbps;
/**
*
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the Outpost
* network devices provide a variable number of uplinks. Specify the number of uplinks for each Outpost network
* device that you intend to use to connect the rack to your network. Note the correlation between
* UplinkGbps
and UplinkCount
.
*
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
*
*/
private String uplinkCount;
/**
*
* The type of fiber that you will use to attach the Outpost to your network.
*
*/
private String fiberOpticCableType;
/**
*
* The type of optical standard that you will use to attach the Outpost to your network. This field is dependent on
* uplink speed, fiber type, and distance to the upstream device. For more information about networking requirements
* for racks, see Network in the Amazon Web Services Outposts User Guide.
*
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
*
*/
private String opticalStandard;
/**
*
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
*
*/
private String maximumSupportedWeightLbs;
/**
*
* The ID or the Amazon Resource Name (ARN) of the site.
*
*
* @param siteId
* The ID or the Amazon Resource Name (ARN) of the site.
*/
public void setSiteId(String siteId) {
this.siteId = siteId;
}
/**
*
* The ID or the Amazon Resource Name (ARN) of the site.
*
*
* @return The ID or the Amazon Resource Name (ARN) of the site.
*/
public String getSiteId() {
return this.siteId;
}
/**
*
* The ID or the Amazon Resource Name (ARN) of the site.
*
*
* @param siteId
* The ID or the Amazon Resource Name (ARN) of the site.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSiteRackPhysicalPropertiesRequest withSiteId(String siteId) {
setSiteId(siteId);
return this;
}
/**
*
* The power draw, in kVA, available at the hardware placement position for the rack.
*
*
* @param powerDrawKva
* The power draw, in kVA, available at the hardware placement position for the rack.
* @see PowerDrawKva
*/
public void setPowerDrawKva(String powerDrawKva) {
this.powerDrawKva = powerDrawKva;
}
/**
*
* The power draw, in kVA, available at the hardware placement position for the rack.
*
*
* @return The power draw, in kVA, available at the hardware placement position for the rack.
* @see PowerDrawKva
*/
public String getPowerDrawKva() {
return this.powerDrawKva;
}
/**
*
* The power draw, in kVA, available at the hardware placement position for the rack.
*
*
* @param powerDrawKva
* The power draw, in kVA, available at the hardware placement position for the rack.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PowerDrawKva
*/
public UpdateSiteRackPhysicalPropertiesRequest withPowerDrawKva(String powerDrawKva) {
setPowerDrawKva(powerDrawKva);
return this;
}
/**
*
* The power draw, in kVA, available at the hardware placement position for the rack.
*
*
* @param powerDrawKva
* The power draw, in kVA, available at the hardware placement position for the rack.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PowerDrawKva
*/
public UpdateSiteRackPhysicalPropertiesRequest withPowerDrawKva(PowerDrawKva powerDrawKva) {
this.powerDrawKva = powerDrawKva.toString();
return this;
}
/**
*
* The power option that you can provide for hardware.
*
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
*
*
* @param powerPhase
* The power option that you can provide for hardware.
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
* @see PowerPhase
*/
public void setPowerPhase(String powerPhase) {
this.powerPhase = powerPhase;
}
/**
*
* The power option that you can provide for hardware.
*
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
*
*
* @return The power option that you can provide for hardware.
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
* @see PowerPhase
*/
public String getPowerPhase() {
return this.powerPhase;
}
/**
*
* The power option that you can provide for hardware.
*
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
*
*
* @param powerPhase
* The power option that you can provide for hardware.
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PowerPhase
*/
public UpdateSiteRackPhysicalPropertiesRequest withPowerPhase(String powerPhase) {
setPowerPhase(powerPhase);
return this;
}
/**
*
* The power option that you can provide for hardware.
*
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
*
*
* @param powerPhase
* The power option that you can provide for hardware.
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PowerPhase
*/
public UpdateSiteRackPhysicalPropertiesRequest withPowerPhase(PowerPhase powerPhase) {
this.powerPhase = powerPhase.toString();
return this;
}
/**
*
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note the
* correlation between PowerPhase
and PowerConnector
.
*
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
*
*
* @param powerConnector
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note
* the correlation between PowerPhase
and PowerConnector
.
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
* @see PowerConnector
*/
public void setPowerConnector(String powerConnector) {
this.powerConnector = powerConnector;
}
/**
*
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note the
* correlation between PowerPhase
and PowerConnector
.
*
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
*
*
* @return The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note
* the correlation between PowerPhase
and PowerConnector
.
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
* @see PowerConnector
*/
public String getPowerConnector() {
return this.powerConnector;
}
/**
*
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note the
* correlation between PowerPhase
and PowerConnector
.
*
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
*
*
* @param powerConnector
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note
* the correlation between PowerPhase
and PowerConnector
.
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PowerConnector
*/
public UpdateSiteRackPhysicalPropertiesRequest withPowerConnector(String powerConnector) {
setPowerConnector(powerConnector);
return this;
}
/**
*
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note the
* correlation between PowerPhase
and PowerConnector
.
*
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
*
*
* @param powerConnector
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note
* the correlation between PowerPhase
and PowerConnector
.
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PowerConnector
*/
public UpdateSiteRackPhysicalPropertiesRequest withPowerConnector(PowerConnector powerConnector) {
this.powerConnector = powerConnector.toString();
return this;
}
/**
*
* Indicates whether the power feed comes above or below the rack.
*
*
* @param powerFeedDrop
* Indicates whether the power feed comes above or below the rack.
* @see PowerFeedDrop
*/
public void setPowerFeedDrop(String powerFeedDrop) {
this.powerFeedDrop = powerFeedDrop;
}
/**
*
* Indicates whether the power feed comes above or below the rack.
*
*
* @return Indicates whether the power feed comes above or below the rack.
* @see PowerFeedDrop
*/
public String getPowerFeedDrop() {
return this.powerFeedDrop;
}
/**
*
* Indicates whether the power feed comes above or below the rack.
*
*
* @param powerFeedDrop
* Indicates whether the power feed comes above or below the rack.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PowerFeedDrop
*/
public UpdateSiteRackPhysicalPropertiesRequest withPowerFeedDrop(String powerFeedDrop) {
setPowerFeedDrop(powerFeedDrop);
return this;
}
/**
*
* Indicates whether the power feed comes above or below the rack.
*
*
* @param powerFeedDrop
* Indicates whether the power feed comes above or below the rack.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PowerFeedDrop
*/
public UpdateSiteRackPhysicalPropertiesRequest withPowerFeedDrop(PowerFeedDrop powerFeedDrop) {
this.powerFeedDrop = powerFeedDrop.toString();
return this;
}
/**
*
* The uplink speed the rack should support for the connection to the Region.
*
*
* @param uplinkGbps
* The uplink speed the rack should support for the connection to the Region.
* @see UplinkGbps
*/
public void setUplinkGbps(String uplinkGbps) {
this.uplinkGbps = uplinkGbps;
}
/**
*
* The uplink speed the rack should support for the connection to the Region.
*
*
* @return The uplink speed the rack should support for the connection to the Region.
* @see UplinkGbps
*/
public String getUplinkGbps() {
return this.uplinkGbps;
}
/**
*
* The uplink speed the rack should support for the connection to the Region.
*
*
* @param uplinkGbps
* The uplink speed the rack should support for the connection to the Region.
* @return Returns a reference to this object so that method calls can be chained together.
* @see UplinkGbps
*/
public UpdateSiteRackPhysicalPropertiesRequest withUplinkGbps(String uplinkGbps) {
setUplinkGbps(uplinkGbps);
return this;
}
/**
*
* The uplink speed the rack should support for the connection to the Region.
*
*
* @param uplinkGbps
* The uplink speed the rack should support for the connection to the Region.
* @return Returns a reference to this object so that method calls can be chained together.
* @see UplinkGbps
*/
public UpdateSiteRackPhysicalPropertiesRequest withUplinkGbps(UplinkGbps uplinkGbps) {
this.uplinkGbps = uplinkGbps.toString();
return this;
}
/**
*
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the Outpost
* network devices provide a variable number of uplinks. Specify the number of uplinks for each Outpost network
* device that you intend to use to connect the rack to your network. Note the correlation between
* UplinkGbps
and UplinkCount
.
*
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
*
*
* @param uplinkCount
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the
* Outpost network devices provide a variable number of uplinks. Specify the number of uplinks for each
* Outpost network device that you intend to use to connect the rack to your network. Note the correlation
* between UplinkGbps
and UplinkCount
.
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
* @see UplinkCount
*/
public void setUplinkCount(String uplinkCount) {
this.uplinkCount = uplinkCount;
}
/**
*
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the Outpost
* network devices provide a variable number of uplinks. Specify the number of uplinks for each Outpost network
* device that you intend to use to connect the rack to your network. Note the correlation between
* UplinkGbps
and UplinkCount
.
*
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
*
*
* @return Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the
* Outpost network devices provide a variable number of uplinks. Specify the number of uplinks for each
* Outpost network device that you intend to use to connect the rack to your network. Note the correlation
* between UplinkGbps
and UplinkCount
.
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
* @see UplinkCount
*/
public String getUplinkCount() {
return this.uplinkCount;
}
/**
*
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the Outpost
* network devices provide a variable number of uplinks. Specify the number of uplinks for each Outpost network
* device that you intend to use to connect the rack to your network. Note the correlation between
* UplinkGbps
and UplinkCount
.
*
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
*
*
* @param uplinkCount
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the
* Outpost network devices provide a variable number of uplinks. Specify the number of uplinks for each
* Outpost network device that you intend to use to connect the rack to your network. Note the correlation
* between UplinkGbps
and UplinkCount
.
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see UplinkCount
*/
public UpdateSiteRackPhysicalPropertiesRequest withUplinkCount(String uplinkCount) {
setUplinkCount(uplinkCount);
return this;
}
/**
*
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the Outpost
* network devices provide a variable number of uplinks. Specify the number of uplinks for each Outpost network
* device that you intend to use to connect the rack to your network. Note the correlation between
* UplinkGbps
and UplinkCount
.
*
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
*
*
* @param uplinkCount
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the
* Outpost network devices provide a variable number of uplinks. Specify the number of uplinks for each
* Outpost network device that you intend to use to connect the rack to your network. Note the correlation
* between UplinkGbps
and UplinkCount
.
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see UplinkCount
*/
public UpdateSiteRackPhysicalPropertiesRequest withUplinkCount(UplinkCount uplinkCount) {
this.uplinkCount = uplinkCount.toString();
return this;
}
/**
*
* The type of fiber that you will use to attach the Outpost to your network.
*
*
* @param fiberOpticCableType
* The type of fiber that you will use to attach the Outpost to your network.
* @see FiberOpticCableType
*/
public void setFiberOpticCableType(String fiberOpticCableType) {
this.fiberOpticCableType = fiberOpticCableType;
}
/**
*
* The type of fiber that you will use to attach the Outpost to your network.
*
*
* @return The type of fiber that you will use to attach the Outpost to your network.
* @see FiberOpticCableType
*/
public String getFiberOpticCableType() {
return this.fiberOpticCableType;
}
/**
*
* The type of fiber that you will use to attach the Outpost to your network.
*
*
* @param fiberOpticCableType
* The type of fiber that you will use to attach the Outpost to your network.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FiberOpticCableType
*/
public UpdateSiteRackPhysicalPropertiesRequest withFiberOpticCableType(String fiberOpticCableType) {
setFiberOpticCableType(fiberOpticCableType);
return this;
}
/**
*
* The type of fiber that you will use to attach the Outpost to your network.
*
*
* @param fiberOpticCableType
* The type of fiber that you will use to attach the Outpost to your network.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FiberOpticCableType
*/
public UpdateSiteRackPhysicalPropertiesRequest withFiberOpticCableType(FiberOpticCableType fiberOpticCableType) {
this.fiberOpticCableType = fiberOpticCableType.toString();
return this;
}
/**
*
* The type of optical standard that you will use to attach the Outpost to your network. This field is dependent on
* uplink speed, fiber type, and distance to the upstream device. For more information about networking requirements
* for racks, see Network in the Amazon Web Services Outposts User Guide.
*
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
*
*
* @param opticalStandard
* The type of optical standard that you will use to attach the Outpost to your network. This field is
* dependent on uplink speed, fiber type, and distance to the upstream device. For more information about
* networking requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
* @see OpticalStandard
*/
public void setOpticalStandard(String opticalStandard) {
this.opticalStandard = opticalStandard;
}
/**
*
* The type of optical standard that you will use to attach the Outpost to your network. This field is dependent on
* uplink speed, fiber type, and distance to the upstream device. For more information about networking requirements
* for racks, see Network in the Amazon Web Services Outposts User Guide.
*
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
*
*
* @return The type of optical standard that you will use to attach the Outpost to your network. This field is
* dependent on uplink speed, fiber type, and distance to the upstream device. For more information about
* networking requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
* @see OpticalStandard
*/
public String getOpticalStandard() {
return this.opticalStandard;
}
/**
*
* The type of optical standard that you will use to attach the Outpost to your network. This field is dependent on
* uplink speed, fiber type, and distance to the upstream device. For more information about networking requirements
* for racks, see Network in the Amazon Web Services Outposts User Guide.
*
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
*
*
* @param opticalStandard
* The type of optical standard that you will use to attach the Outpost to your network. This field is
* dependent on uplink speed, fiber type, and distance to the upstream device. For more information about
* networking requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OpticalStandard
*/
public UpdateSiteRackPhysicalPropertiesRequest withOpticalStandard(String opticalStandard) {
setOpticalStandard(opticalStandard);
return this;
}
/**
*
* The type of optical standard that you will use to attach the Outpost to your network. This field is dependent on
* uplink speed, fiber type, and distance to the upstream device. For more information about networking requirements
* for racks, see Network in the Amazon Web Services Outposts User Guide.
*
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
*
*
* @param opticalStandard
* The type of optical standard that you will use to attach the Outpost to your network. This field is
* dependent on uplink speed, fiber type, and distance to the upstream device. For more information about
* networking requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OpticalStandard
*/
public UpdateSiteRackPhysicalPropertiesRequest withOpticalStandard(OpticalStandard opticalStandard) {
this.opticalStandard = opticalStandard.toString();
return this;
}
/**
*
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
*
*
* @param maximumSupportedWeightLbs
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
* @see MaximumSupportedWeightLbs
*/
public void setMaximumSupportedWeightLbs(String maximumSupportedWeightLbs) {
this.maximumSupportedWeightLbs = maximumSupportedWeightLbs;
}
/**
*
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
*
*
* @return The maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
* @see MaximumSupportedWeightLbs
*/
public String getMaximumSupportedWeightLbs() {
return this.maximumSupportedWeightLbs;
}
/**
*
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
*
*
* @param maximumSupportedWeightLbs
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaximumSupportedWeightLbs
*/
public UpdateSiteRackPhysicalPropertiesRequest withMaximumSupportedWeightLbs(String maximumSupportedWeightLbs) {
setMaximumSupportedWeightLbs(maximumSupportedWeightLbs);
return this;
}
/**
*
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
*
*
* @param maximumSupportedWeightLbs
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaximumSupportedWeightLbs
*/
public UpdateSiteRackPhysicalPropertiesRequest withMaximumSupportedWeightLbs(MaximumSupportedWeightLbs maximumSupportedWeightLbs) {
this.maximumSupportedWeightLbs = maximumSupportedWeightLbs.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSiteId() != null)
sb.append("SiteId: ").append(getSiteId()).append(",");
if (getPowerDrawKva() != null)
sb.append("PowerDrawKva: ").append(getPowerDrawKva()).append(",");
if (getPowerPhase() != null)
sb.append("PowerPhase: ").append(getPowerPhase()).append(",");
if (getPowerConnector() != null)
sb.append("PowerConnector: ").append(getPowerConnector()).append(",");
if (getPowerFeedDrop() != null)
sb.append("PowerFeedDrop: ").append(getPowerFeedDrop()).append(",");
if (getUplinkGbps() != null)
sb.append("UplinkGbps: ").append(getUplinkGbps()).append(",");
if (getUplinkCount() != null)
sb.append("UplinkCount: ").append(getUplinkCount()).append(",");
if (getFiberOpticCableType() != null)
sb.append("FiberOpticCableType: ").append(getFiberOpticCableType()).append(",");
if (getOpticalStandard() != null)
sb.append("OpticalStandard: ").append(getOpticalStandard()).append(",");
if (getMaximumSupportedWeightLbs() != null)
sb.append("MaximumSupportedWeightLbs: ").append(getMaximumSupportedWeightLbs());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateSiteRackPhysicalPropertiesRequest == false)
return false;
UpdateSiteRackPhysicalPropertiesRequest other = (UpdateSiteRackPhysicalPropertiesRequest) obj;
if (other.getSiteId() == null ^ this.getSiteId() == null)
return false;
if (other.getSiteId() != null && other.getSiteId().equals(this.getSiteId()) == false)
return false;
if (other.getPowerDrawKva() == null ^ this.getPowerDrawKva() == null)
return false;
if (other.getPowerDrawKva() != null && other.getPowerDrawKva().equals(this.getPowerDrawKva()) == false)
return false;
if (other.getPowerPhase() == null ^ this.getPowerPhase() == null)
return false;
if (other.getPowerPhase() != null && other.getPowerPhase().equals(this.getPowerPhase()) == false)
return false;
if (other.getPowerConnector() == null ^ this.getPowerConnector() == null)
return false;
if (other.getPowerConnector() != null && other.getPowerConnector().equals(this.getPowerConnector()) == false)
return false;
if (other.getPowerFeedDrop() == null ^ this.getPowerFeedDrop() == null)
return false;
if (other.getPowerFeedDrop() != null && other.getPowerFeedDrop().equals(this.getPowerFeedDrop()) == false)
return false;
if (other.getUplinkGbps() == null ^ this.getUplinkGbps() == null)
return false;
if (other.getUplinkGbps() != null && other.getUplinkGbps().equals(this.getUplinkGbps()) == false)
return false;
if (other.getUplinkCount() == null ^ this.getUplinkCount() == null)
return false;
if (other.getUplinkCount() != null && other.getUplinkCount().equals(this.getUplinkCount()) == false)
return false;
if (other.getFiberOpticCableType() == null ^ this.getFiberOpticCableType() == null)
return false;
if (other.getFiberOpticCableType() != null && other.getFiberOpticCableType().equals(this.getFiberOpticCableType()) == false)
return false;
if (other.getOpticalStandard() == null ^ this.getOpticalStandard() == null)
return false;
if (other.getOpticalStandard() != null && other.getOpticalStandard().equals(this.getOpticalStandard()) == false)
return false;
if (other.getMaximumSupportedWeightLbs() == null ^ this.getMaximumSupportedWeightLbs() == null)
return false;
if (other.getMaximumSupportedWeightLbs() != null && other.getMaximumSupportedWeightLbs().equals(this.getMaximumSupportedWeightLbs()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSiteId() == null) ? 0 : getSiteId().hashCode());
hashCode = prime * hashCode + ((getPowerDrawKva() == null) ? 0 : getPowerDrawKva().hashCode());
hashCode = prime * hashCode + ((getPowerPhase() == null) ? 0 : getPowerPhase().hashCode());
hashCode = prime * hashCode + ((getPowerConnector() == null) ? 0 : getPowerConnector().hashCode());
hashCode = prime * hashCode + ((getPowerFeedDrop() == null) ? 0 : getPowerFeedDrop().hashCode());
hashCode = prime * hashCode + ((getUplinkGbps() == null) ? 0 : getUplinkGbps().hashCode());
hashCode = prime * hashCode + ((getUplinkCount() == null) ? 0 : getUplinkCount().hashCode());
hashCode = prime * hashCode + ((getFiberOpticCableType() == null) ? 0 : getFiberOpticCableType().hashCode());
hashCode = prime * hashCode + ((getOpticalStandard() == null) ? 0 : getOpticalStandard().hashCode());
hashCode = prime * hashCode + ((getMaximumSupportedWeightLbs() == null) ? 0 : getMaximumSupportedWeightLbs().hashCode());
return hashCode;
}
@Override
public UpdateSiteRackPhysicalPropertiesRequest clone() {
return (UpdateSiteRackPhysicalPropertiesRequest) super.clone();
}
}