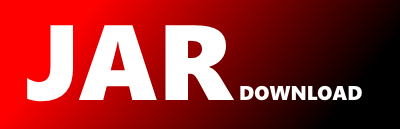
com.amazonaws.services.panorama.model.DescribeDeviceResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-panorama Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.panorama.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeDeviceResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* Beta software releases available for the device.
*
*/
private java.util.List alternateSoftwares;
/**
*
* The device's ARN.
*
*/
private String arn;
/**
*
* The device's maker.
*
*/
private String brand;
/**
*
* When the device was created.
*
*/
private java.util.Date createdTime;
/**
*
* The device's networking status.
*
*/
private NetworkStatus currentNetworkingStatus;
/**
*
* The device's current software version.
*
*/
private String currentSoftware;
/**
*
* The device's description.
*
*/
private String description;
/**
*
* A device's aggregated status. Including the device's connection status, provisioning status, and lease status.
*
*/
private String deviceAggregatedStatus;
/**
*
* The device's connection status.
*
*/
private String deviceConnectionStatus;
/**
*
* The device's ID.
*
*/
private String deviceId;
/**
*
* The most recent beta software release.
*
*/
private String latestAlternateSoftware;
/**
*
* A device's latest job. Includes the target image version, and the job status.
*
*/
private LatestDeviceJob latestDeviceJob;
/**
*
* The latest software version available for the device.
*
*/
private String latestSoftware;
/**
*
* The device's lease expiration time.
*
*/
private java.util.Date leaseExpirationTime;
/**
*
* The device's name.
*
*/
private String name;
/**
*
* The device's networking configuration.
*
*/
private NetworkPayload networkingConfiguration;
/**
*
* The device's provisioning status.
*
*/
private String provisioningStatus;
/**
*
* The device's serial number.
*
*/
private String serialNumber;
/**
*
* The device's tags.
*
*/
private java.util.Map tags;
/**
*
* The device's type.
*
*/
private String type;
/**
*
* Beta software releases available for the device.
*
*
* @return Beta software releases available for the device.
*/
public java.util.List getAlternateSoftwares() {
return alternateSoftwares;
}
/**
*
* Beta software releases available for the device.
*
*
* @param alternateSoftwares
* Beta software releases available for the device.
*/
public void setAlternateSoftwares(java.util.Collection alternateSoftwares) {
if (alternateSoftwares == null) {
this.alternateSoftwares = null;
return;
}
this.alternateSoftwares = new java.util.ArrayList(alternateSoftwares);
}
/**
*
* Beta software releases available for the device.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAlternateSoftwares(java.util.Collection)} or {@link #withAlternateSoftwares(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param alternateSoftwares
* Beta software releases available for the device.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withAlternateSoftwares(AlternateSoftwareMetadata... alternateSoftwares) {
if (this.alternateSoftwares == null) {
setAlternateSoftwares(new java.util.ArrayList(alternateSoftwares.length));
}
for (AlternateSoftwareMetadata ele : alternateSoftwares) {
this.alternateSoftwares.add(ele);
}
return this;
}
/**
*
* Beta software releases available for the device.
*
*
* @param alternateSoftwares
* Beta software releases available for the device.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withAlternateSoftwares(java.util.Collection alternateSoftwares) {
setAlternateSoftwares(alternateSoftwares);
return this;
}
/**
*
* The device's ARN.
*
*
* @param arn
* The device's ARN.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The device's ARN.
*
*
* @return The device's ARN.
*/
public String getArn() {
return this.arn;
}
/**
*
* The device's ARN.
*
*
* @param arn
* The device's ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The device's maker.
*
*
* @param brand
* The device's maker.
* @see DeviceBrand
*/
public void setBrand(String brand) {
this.brand = brand;
}
/**
*
* The device's maker.
*
*
* @return The device's maker.
* @see DeviceBrand
*/
public String getBrand() {
return this.brand;
}
/**
*
* The device's maker.
*
*
* @param brand
* The device's maker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceBrand
*/
public DescribeDeviceResult withBrand(String brand) {
setBrand(brand);
return this;
}
/**
*
* The device's maker.
*
*
* @param brand
* The device's maker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceBrand
*/
public DescribeDeviceResult withBrand(DeviceBrand brand) {
this.brand = brand.toString();
return this;
}
/**
*
* When the device was created.
*
*
* @param createdTime
* When the device was created.
*/
public void setCreatedTime(java.util.Date createdTime) {
this.createdTime = createdTime;
}
/**
*
* When the device was created.
*
*
* @return When the device was created.
*/
public java.util.Date getCreatedTime() {
return this.createdTime;
}
/**
*
* When the device was created.
*
*
* @param createdTime
* When the device was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withCreatedTime(java.util.Date createdTime) {
setCreatedTime(createdTime);
return this;
}
/**
*
* The device's networking status.
*
*
* @param currentNetworkingStatus
* The device's networking status.
*/
public void setCurrentNetworkingStatus(NetworkStatus currentNetworkingStatus) {
this.currentNetworkingStatus = currentNetworkingStatus;
}
/**
*
* The device's networking status.
*
*
* @return The device's networking status.
*/
public NetworkStatus getCurrentNetworkingStatus() {
return this.currentNetworkingStatus;
}
/**
*
* The device's networking status.
*
*
* @param currentNetworkingStatus
* The device's networking status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withCurrentNetworkingStatus(NetworkStatus currentNetworkingStatus) {
setCurrentNetworkingStatus(currentNetworkingStatus);
return this;
}
/**
*
* The device's current software version.
*
*
* @param currentSoftware
* The device's current software version.
*/
public void setCurrentSoftware(String currentSoftware) {
this.currentSoftware = currentSoftware;
}
/**
*
* The device's current software version.
*
*
* @return The device's current software version.
*/
public String getCurrentSoftware() {
return this.currentSoftware;
}
/**
*
* The device's current software version.
*
*
* @param currentSoftware
* The device's current software version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withCurrentSoftware(String currentSoftware) {
setCurrentSoftware(currentSoftware);
return this;
}
/**
*
* The device's description.
*
*
* @param description
* The device's description.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The device's description.
*
*
* @return The device's description.
*/
public String getDescription() {
return this.description;
}
/**
*
* The device's description.
*
*
* @param description
* The device's description.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* A device's aggregated status. Including the device's connection status, provisioning status, and lease status.
*
*
* @param deviceAggregatedStatus
* A device's aggregated status. Including the device's connection status, provisioning status, and lease
* status.
* @see DeviceAggregatedStatus
*/
public void setDeviceAggregatedStatus(String deviceAggregatedStatus) {
this.deviceAggregatedStatus = deviceAggregatedStatus;
}
/**
*
* A device's aggregated status. Including the device's connection status, provisioning status, and lease status.
*
*
* @return A device's aggregated status. Including the device's connection status, provisioning status, and lease
* status.
* @see DeviceAggregatedStatus
*/
public String getDeviceAggregatedStatus() {
return this.deviceAggregatedStatus;
}
/**
*
* A device's aggregated status. Including the device's connection status, provisioning status, and lease status.
*
*
* @param deviceAggregatedStatus
* A device's aggregated status. Including the device's connection status, provisioning status, and lease
* status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceAggregatedStatus
*/
public DescribeDeviceResult withDeviceAggregatedStatus(String deviceAggregatedStatus) {
setDeviceAggregatedStatus(deviceAggregatedStatus);
return this;
}
/**
*
* A device's aggregated status. Including the device's connection status, provisioning status, and lease status.
*
*
* @param deviceAggregatedStatus
* A device's aggregated status. Including the device's connection status, provisioning status, and lease
* status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceAggregatedStatus
*/
public DescribeDeviceResult withDeviceAggregatedStatus(DeviceAggregatedStatus deviceAggregatedStatus) {
this.deviceAggregatedStatus = deviceAggregatedStatus.toString();
return this;
}
/**
*
* The device's connection status.
*
*
* @param deviceConnectionStatus
* The device's connection status.
* @see DeviceConnectionStatus
*/
public void setDeviceConnectionStatus(String deviceConnectionStatus) {
this.deviceConnectionStatus = deviceConnectionStatus;
}
/**
*
* The device's connection status.
*
*
* @return The device's connection status.
* @see DeviceConnectionStatus
*/
public String getDeviceConnectionStatus() {
return this.deviceConnectionStatus;
}
/**
*
* The device's connection status.
*
*
* @param deviceConnectionStatus
* The device's connection status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceConnectionStatus
*/
public DescribeDeviceResult withDeviceConnectionStatus(String deviceConnectionStatus) {
setDeviceConnectionStatus(deviceConnectionStatus);
return this;
}
/**
*
* The device's connection status.
*
*
* @param deviceConnectionStatus
* The device's connection status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceConnectionStatus
*/
public DescribeDeviceResult withDeviceConnectionStatus(DeviceConnectionStatus deviceConnectionStatus) {
this.deviceConnectionStatus = deviceConnectionStatus.toString();
return this;
}
/**
*
* The device's ID.
*
*
* @param deviceId
* The device's ID.
*/
public void setDeviceId(String deviceId) {
this.deviceId = deviceId;
}
/**
*
* The device's ID.
*
*
* @return The device's ID.
*/
public String getDeviceId() {
return this.deviceId;
}
/**
*
* The device's ID.
*
*
* @param deviceId
* The device's ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withDeviceId(String deviceId) {
setDeviceId(deviceId);
return this;
}
/**
*
* The most recent beta software release.
*
*
* @param latestAlternateSoftware
* The most recent beta software release.
*/
public void setLatestAlternateSoftware(String latestAlternateSoftware) {
this.latestAlternateSoftware = latestAlternateSoftware;
}
/**
*
* The most recent beta software release.
*
*
* @return The most recent beta software release.
*/
public String getLatestAlternateSoftware() {
return this.latestAlternateSoftware;
}
/**
*
* The most recent beta software release.
*
*
* @param latestAlternateSoftware
* The most recent beta software release.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withLatestAlternateSoftware(String latestAlternateSoftware) {
setLatestAlternateSoftware(latestAlternateSoftware);
return this;
}
/**
*
* A device's latest job. Includes the target image version, and the job status.
*
*
* @param latestDeviceJob
* A device's latest job. Includes the target image version, and the job status.
*/
public void setLatestDeviceJob(LatestDeviceJob latestDeviceJob) {
this.latestDeviceJob = latestDeviceJob;
}
/**
*
* A device's latest job. Includes the target image version, and the job status.
*
*
* @return A device's latest job. Includes the target image version, and the job status.
*/
public LatestDeviceJob getLatestDeviceJob() {
return this.latestDeviceJob;
}
/**
*
* A device's latest job. Includes the target image version, and the job status.
*
*
* @param latestDeviceJob
* A device's latest job. Includes the target image version, and the job status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withLatestDeviceJob(LatestDeviceJob latestDeviceJob) {
setLatestDeviceJob(latestDeviceJob);
return this;
}
/**
*
* The latest software version available for the device.
*
*
* @param latestSoftware
* The latest software version available for the device.
*/
public void setLatestSoftware(String latestSoftware) {
this.latestSoftware = latestSoftware;
}
/**
*
* The latest software version available for the device.
*
*
* @return The latest software version available for the device.
*/
public String getLatestSoftware() {
return this.latestSoftware;
}
/**
*
* The latest software version available for the device.
*
*
* @param latestSoftware
* The latest software version available for the device.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withLatestSoftware(String latestSoftware) {
setLatestSoftware(latestSoftware);
return this;
}
/**
*
* The device's lease expiration time.
*
*
* @param leaseExpirationTime
* The device's lease expiration time.
*/
public void setLeaseExpirationTime(java.util.Date leaseExpirationTime) {
this.leaseExpirationTime = leaseExpirationTime;
}
/**
*
* The device's lease expiration time.
*
*
* @return The device's lease expiration time.
*/
public java.util.Date getLeaseExpirationTime() {
return this.leaseExpirationTime;
}
/**
*
* The device's lease expiration time.
*
*
* @param leaseExpirationTime
* The device's lease expiration time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withLeaseExpirationTime(java.util.Date leaseExpirationTime) {
setLeaseExpirationTime(leaseExpirationTime);
return this;
}
/**
*
* The device's name.
*
*
* @param name
* The device's name.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The device's name.
*
*
* @return The device's name.
*/
public String getName() {
return this.name;
}
/**
*
* The device's name.
*
*
* @param name
* The device's name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withName(String name) {
setName(name);
return this;
}
/**
*
* The device's networking configuration.
*
*
* @param networkingConfiguration
* The device's networking configuration.
*/
public void setNetworkingConfiguration(NetworkPayload networkingConfiguration) {
this.networkingConfiguration = networkingConfiguration;
}
/**
*
* The device's networking configuration.
*
*
* @return The device's networking configuration.
*/
public NetworkPayload getNetworkingConfiguration() {
return this.networkingConfiguration;
}
/**
*
* The device's networking configuration.
*
*
* @param networkingConfiguration
* The device's networking configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withNetworkingConfiguration(NetworkPayload networkingConfiguration) {
setNetworkingConfiguration(networkingConfiguration);
return this;
}
/**
*
* The device's provisioning status.
*
*
* @param provisioningStatus
* The device's provisioning status.
* @see DeviceStatus
*/
public void setProvisioningStatus(String provisioningStatus) {
this.provisioningStatus = provisioningStatus;
}
/**
*
* The device's provisioning status.
*
*
* @return The device's provisioning status.
* @see DeviceStatus
*/
public String getProvisioningStatus() {
return this.provisioningStatus;
}
/**
*
* The device's provisioning status.
*
*
* @param provisioningStatus
* The device's provisioning status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceStatus
*/
public DescribeDeviceResult withProvisioningStatus(String provisioningStatus) {
setProvisioningStatus(provisioningStatus);
return this;
}
/**
*
* The device's provisioning status.
*
*
* @param provisioningStatus
* The device's provisioning status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceStatus
*/
public DescribeDeviceResult withProvisioningStatus(DeviceStatus provisioningStatus) {
this.provisioningStatus = provisioningStatus.toString();
return this;
}
/**
*
* The device's serial number.
*
*
* @param serialNumber
* The device's serial number.
*/
public void setSerialNumber(String serialNumber) {
this.serialNumber = serialNumber;
}
/**
*
* The device's serial number.
*
*
* @return The device's serial number.
*/
public String getSerialNumber() {
return this.serialNumber;
}
/**
*
* The device's serial number.
*
*
* @param serialNumber
* The device's serial number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withSerialNumber(String serialNumber) {
setSerialNumber(serialNumber);
return this;
}
/**
*
* The device's tags.
*
*
* @return The device's tags.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The device's tags.
*
*
* @param tags
* The device's tags.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The device's tags.
*
*
* @param tags
* The device's tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see DescribeDeviceResult#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDeviceResult clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* The device's type.
*
*
* @param type
* The device's type.
* @see DeviceType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The device's type.
*
*
* @return The device's type.
* @see DeviceType
*/
public String getType() {
return this.type;
}
/**
*
* The device's type.
*
*
* @param type
* The device's type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceType
*/
public DescribeDeviceResult withType(String type) {
setType(type);
return this;
}
/**
*
* The device's type.
*
*
* @param type
* The device's type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeviceType
*/
public DescribeDeviceResult withType(DeviceType type) {
this.type = type.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAlternateSoftwares() != null)
sb.append("AlternateSoftwares: ").append(getAlternateSoftwares()).append(",");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getBrand() != null)
sb.append("Brand: ").append(getBrand()).append(",");
if (getCreatedTime() != null)
sb.append("CreatedTime: ").append(getCreatedTime()).append(",");
if (getCurrentNetworkingStatus() != null)
sb.append("CurrentNetworkingStatus: ").append(getCurrentNetworkingStatus()).append(",");
if (getCurrentSoftware() != null)
sb.append("CurrentSoftware: ").append(getCurrentSoftware()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getDeviceAggregatedStatus() != null)
sb.append("DeviceAggregatedStatus: ").append(getDeviceAggregatedStatus()).append(",");
if (getDeviceConnectionStatus() != null)
sb.append("DeviceConnectionStatus: ").append(getDeviceConnectionStatus()).append(",");
if (getDeviceId() != null)
sb.append("DeviceId: ").append(getDeviceId()).append(",");
if (getLatestAlternateSoftware() != null)
sb.append("LatestAlternateSoftware: ").append(getLatestAlternateSoftware()).append(",");
if (getLatestDeviceJob() != null)
sb.append("LatestDeviceJob: ").append(getLatestDeviceJob()).append(",");
if (getLatestSoftware() != null)
sb.append("LatestSoftware: ").append(getLatestSoftware()).append(",");
if (getLeaseExpirationTime() != null)
sb.append("LeaseExpirationTime: ").append(getLeaseExpirationTime()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getNetworkingConfiguration() != null)
sb.append("NetworkingConfiguration: ").append(getNetworkingConfiguration()).append(",");
if (getProvisioningStatus() != null)
sb.append("ProvisioningStatus: ").append(getProvisioningStatus()).append(",");
if (getSerialNumber() != null)
sb.append("SerialNumber: ").append(getSerialNumber()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeDeviceResult == false)
return false;
DescribeDeviceResult other = (DescribeDeviceResult) obj;
if (other.getAlternateSoftwares() == null ^ this.getAlternateSoftwares() == null)
return false;
if (other.getAlternateSoftwares() != null && other.getAlternateSoftwares().equals(this.getAlternateSoftwares()) == false)
return false;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getBrand() == null ^ this.getBrand() == null)
return false;
if (other.getBrand() != null && other.getBrand().equals(this.getBrand()) == false)
return false;
if (other.getCreatedTime() == null ^ this.getCreatedTime() == null)
return false;
if (other.getCreatedTime() != null && other.getCreatedTime().equals(this.getCreatedTime()) == false)
return false;
if (other.getCurrentNetworkingStatus() == null ^ this.getCurrentNetworkingStatus() == null)
return false;
if (other.getCurrentNetworkingStatus() != null && other.getCurrentNetworkingStatus().equals(this.getCurrentNetworkingStatus()) == false)
return false;
if (other.getCurrentSoftware() == null ^ this.getCurrentSoftware() == null)
return false;
if (other.getCurrentSoftware() != null && other.getCurrentSoftware().equals(this.getCurrentSoftware()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getDeviceAggregatedStatus() == null ^ this.getDeviceAggregatedStatus() == null)
return false;
if (other.getDeviceAggregatedStatus() != null && other.getDeviceAggregatedStatus().equals(this.getDeviceAggregatedStatus()) == false)
return false;
if (other.getDeviceConnectionStatus() == null ^ this.getDeviceConnectionStatus() == null)
return false;
if (other.getDeviceConnectionStatus() != null && other.getDeviceConnectionStatus().equals(this.getDeviceConnectionStatus()) == false)
return false;
if (other.getDeviceId() == null ^ this.getDeviceId() == null)
return false;
if (other.getDeviceId() != null && other.getDeviceId().equals(this.getDeviceId()) == false)
return false;
if (other.getLatestAlternateSoftware() == null ^ this.getLatestAlternateSoftware() == null)
return false;
if (other.getLatestAlternateSoftware() != null && other.getLatestAlternateSoftware().equals(this.getLatestAlternateSoftware()) == false)
return false;
if (other.getLatestDeviceJob() == null ^ this.getLatestDeviceJob() == null)
return false;
if (other.getLatestDeviceJob() != null && other.getLatestDeviceJob().equals(this.getLatestDeviceJob()) == false)
return false;
if (other.getLatestSoftware() == null ^ this.getLatestSoftware() == null)
return false;
if (other.getLatestSoftware() != null && other.getLatestSoftware().equals(this.getLatestSoftware()) == false)
return false;
if (other.getLeaseExpirationTime() == null ^ this.getLeaseExpirationTime() == null)
return false;
if (other.getLeaseExpirationTime() != null && other.getLeaseExpirationTime().equals(this.getLeaseExpirationTime()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getNetworkingConfiguration() == null ^ this.getNetworkingConfiguration() == null)
return false;
if (other.getNetworkingConfiguration() != null && other.getNetworkingConfiguration().equals(this.getNetworkingConfiguration()) == false)
return false;
if (other.getProvisioningStatus() == null ^ this.getProvisioningStatus() == null)
return false;
if (other.getProvisioningStatus() != null && other.getProvisioningStatus().equals(this.getProvisioningStatus()) == false)
return false;
if (other.getSerialNumber() == null ^ this.getSerialNumber() == null)
return false;
if (other.getSerialNumber() != null && other.getSerialNumber().equals(this.getSerialNumber()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAlternateSoftwares() == null) ? 0 : getAlternateSoftwares().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getBrand() == null) ? 0 : getBrand().hashCode());
hashCode = prime * hashCode + ((getCreatedTime() == null) ? 0 : getCreatedTime().hashCode());
hashCode = prime * hashCode + ((getCurrentNetworkingStatus() == null) ? 0 : getCurrentNetworkingStatus().hashCode());
hashCode = prime * hashCode + ((getCurrentSoftware() == null) ? 0 : getCurrentSoftware().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getDeviceAggregatedStatus() == null) ? 0 : getDeviceAggregatedStatus().hashCode());
hashCode = prime * hashCode + ((getDeviceConnectionStatus() == null) ? 0 : getDeviceConnectionStatus().hashCode());
hashCode = prime * hashCode + ((getDeviceId() == null) ? 0 : getDeviceId().hashCode());
hashCode = prime * hashCode + ((getLatestAlternateSoftware() == null) ? 0 : getLatestAlternateSoftware().hashCode());
hashCode = prime * hashCode + ((getLatestDeviceJob() == null) ? 0 : getLatestDeviceJob().hashCode());
hashCode = prime * hashCode + ((getLatestSoftware() == null) ? 0 : getLatestSoftware().hashCode());
hashCode = prime * hashCode + ((getLeaseExpirationTime() == null) ? 0 : getLeaseExpirationTime().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getNetworkingConfiguration() == null) ? 0 : getNetworkingConfiguration().hashCode());
hashCode = prime * hashCode + ((getProvisioningStatus() == null) ? 0 : getProvisioningStatus().hashCode());
hashCode = prime * hashCode + ((getSerialNumber() == null) ? 0 : getSerialNumber().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
return hashCode;
}
@Override
public DescribeDeviceResult clone() {
try {
return (DescribeDeviceResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}