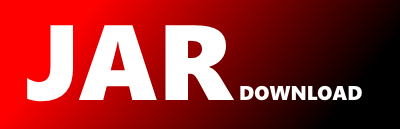
com.amazonaws.services.panorama.AWSPanorama Maven / Gradle / Ivy
Show all versions of aws-java-sdk-panorama Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.panorama;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.panorama.model.*;
/**
* Interface for accessing Panorama.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.panorama.AbstractAWSPanorama} instead.
*
*
*
* AWS Panorama
*
* Overview
*
*
* This is the AWS Panorama API Reference. For an introduction to the service, see What is AWS Panorama? in the AWS
* Panorama Developer Guide.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSPanorama {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "panorama";
/**
*
* Creates an application instance and deploys it to a device.
*
*
* @param createApplicationInstanceRequest
* @return Result of the CreateApplicationInstance operation returned by the service.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ServiceQuotaExceededException
* The request would cause a limit to be exceeded.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.CreateApplicationInstance
* @see AWS API Documentation
*/
CreateApplicationInstanceResult createApplicationInstance(CreateApplicationInstanceRequest createApplicationInstanceRequest);
/**
*
* Creates a job to run on a device. A job can update a device's software or reboot it.
*
*
* @param createJobForDevicesRequest
* @return Result of the CreateJobForDevices operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.CreateJobForDevices
* @see AWS
* API Documentation
*/
CreateJobForDevicesResult createJobForDevices(CreateJobForDevicesRequest createJobForDevicesRequest);
/**
*
* Creates a camera stream node.
*
*
* @param createNodeFromTemplateJobRequest
* @return Result of the CreateNodeFromTemplateJob operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.CreateNodeFromTemplateJob
* @see AWS API Documentation
*/
CreateNodeFromTemplateJobResult createNodeFromTemplateJob(CreateNodeFromTemplateJobRequest createNodeFromTemplateJobRequest);
/**
*
* Creates a package and storage location in an Amazon S3 access point.
*
*
* @param createPackageRequest
* @return Result of the CreatePackage operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.CreatePackage
* @see AWS API
* Documentation
*/
CreatePackageResult createPackage(CreatePackageRequest createPackageRequest);
/**
*
* Imports a node package.
*
*
* @param createPackageImportJobRequest
* @return Result of the CreatePackageImportJob operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.CreatePackageImportJob
* @see AWS API Documentation
*/
CreatePackageImportJobResult createPackageImportJob(CreatePackageImportJobRequest createPackageImportJobRequest);
/**
*
* Deletes a device.
*
*
* @param deleteDeviceRequest
* @return Result of the DeleteDevice operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DeleteDevice
* @see AWS API
* Documentation
*/
DeleteDeviceResult deleteDevice(DeleteDeviceRequest deleteDeviceRequest);
/**
*
* Deletes a package.
*
*
*
* To delete a package, you need permission to call s3:DeleteObject
in addition to permissions for the
* AWS Panorama API.
*
*
*
* @param deletePackageRequest
* @return Result of the DeletePackage operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DeletePackage
* @see AWS API
* Documentation
*/
DeletePackageResult deletePackage(DeletePackageRequest deletePackageRequest);
/**
*
* Deregisters a package version.
*
*
* @param deregisterPackageVersionRequest
* @return Result of the DeregisterPackageVersion operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DeregisterPackageVersion
* @see AWS API Documentation
*/
DeregisterPackageVersionResult deregisterPackageVersion(DeregisterPackageVersionRequest deregisterPackageVersionRequest);
/**
*
* Returns information about an application instance on a device.
*
*
* @param describeApplicationInstanceRequest
* @return Result of the DescribeApplicationInstance operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DescribeApplicationInstance
* @see AWS API Documentation
*/
DescribeApplicationInstanceResult describeApplicationInstance(DescribeApplicationInstanceRequest describeApplicationInstanceRequest);
/**
*
* Returns information about an application instance's configuration manifest.
*
*
* @param describeApplicationInstanceDetailsRequest
* @return Result of the DescribeApplicationInstanceDetails operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DescribeApplicationInstanceDetails
* @see AWS API Documentation
*/
DescribeApplicationInstanceDetailsResult describeApplicationInstanceDetails(
DescribeApplicationInstanceDetailsRequest describeApplicationInstanceDetailsRequest);
/**
*
* Returns information about a device.
*
*
* @param describeDeviceRequest
* @return Result of the DescribeDevice operation returned by the service.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DescribeDevice
* @see AWS API
* Documentation
*/
DescribeDeviceResult describeDevice(DescribeDeviceRequest describeDeviceRequest);
/**
*
* Returns information about a device job.
*
*
* @param describeDeviceJobRequest
* @return Result of the DescribeDeviceJob operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DescribeDeviceJob
* @see AWS API
* Documentation
*/
DescribeDeviceJobResult describeDeviceJob(DescribeDeviceJobRequest describeDeviceJobRequest);
/**
*
* Returns information about a node.
*
*
* @param describeNodeRequest
* @return Result of the DescribeNode operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DescribeNode
* @see AWS API
* Documentation
*/
DescribeNodeResult describeNode(DescribeNodeRequest describeNodeRequest);
/**
*
* Returns information about a job to create a camera stream node.
*
*
* @param describeNodeFromTemplateJobRequest
* @return Result of the DescribeNodeFromTemplateJob operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DescribeNodeFromTemplateJob
* @see AWS API Documentation
*/
DescribeNodeFromTemplateJobResult describeNodeFromTemplateJob(DescribeNodeFromTemplateJobRequest describeNodeFromTemplateJobRequest);
/**
*
* Returns information about a package.
*
*
* @param describePackageRequest
* @return Result of the DescribePackage operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DescribePackage
* @see AWS API
* Documentation
*/
DescribePackageResult describePackage(DescribePackageRequest describePackageRequest);
/**
*
* Returns information about a package import job.
*
*
* @param describePackageImportJobRequest
* @return Result of the DescribePackageImportJob operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DescribePackageImportJob
* @see AWS API Documentation
*/
DescribePackageImportJobResult describePackageImportJob(DescribePackageImportJobRequest describePackageImportJobRequest);
/**
*
* Returns information about a package version.
*
*
* @param describePackageVersionRequest
* @return Result of the DescribePackageVersion operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.DescribePackageVersion
* @see AWS API Documentation
*/
DescribePackageVersionResult describePackageVersion(DescribePackageVersionRequest describePackageVersionRequest);
/**
*
* Returns a list of application instance dependencies.
*
*
* @param listApplicationInstanceDependenciesRequest
* @return Result of the ListApplicationInstanceDependencies operation returned by the service.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListApplicationInstanceDependencies
* @see AWS API Documentation
*/
ListApplicationInstanceDependenciesResult listApplicationInstanceDependencies(
ListApplicationInstanceDependenciesRequest listApplicationInstanceDependenciesRequest);
/**
*
* Returns a list of application node instances.
*
*
* @param listApplicationInstanceNodeInstancesRequest
* @return Result of the ListApplicationInstanceNodeInstances operation returned by the service.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListApplicationInstanceNodeInstances
* @see AWS API Documentation
*/
ListApplicationInstanceNodeInstancesResult listApplicationInstanceNodeInstances(
ListApplicationInstanceNodeInstancesRequest listApplicationInstanceNodeInstancesRequest);
/**
*
* Returns a list of application instances.
*
*
* @param listApplicationInstancesRequest
* @return Result of the ListApplicationInstances operation returned by the service.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListApplicationInstances
* @see AWS API Documentation
*/
ListApplicationInstancesResult listApplicationInstances(ListApplicationInstancesRequest listApplicationInstancesRequest);
/**
*
* Returns a list of devices.
*
*
* @param listDevicesRequest
* @return Result of the ListDevices operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListDevices
* @see AWS API
* Documentation
*/
ListDevicesResult listDevices(ListDevicesRequest listDevicesRequest);
/**
*
* Returns a list of jobs.
*
*
* @param listDevicesJobsRequest
* @return Result of the ListDevicesJobs operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListDevicesJobs
* @see AWS API
* Documentation
*/
ListDevicesJobsResult listDevicesJobs(ListDevicesJobsRequest listDevicesJobsRequest);
/**
*
* Returns a list of camera stream node jobs.
*
*
* @param listNodeFromTemplateJobsRequest
* @return Result of the ListNodeFromTemplateJobs operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListNodeFromTemplateJobs
* @see AWS API Documentation
*/
ListNodeFromTemplateJobsResult listNodeFromTemplateJobs(ListNodeFromTemplateJobsRequest listNodeFromTemplateJobsRequest);
/**
*
* Returns a list of nodes.
*
*
* @param listNodesRequest
* @return Result of the ListNodes operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListNodes
* @see AWS API
* Documentation
*/
ListNodesResult listNodes(ListNodesRequest listNodesRequest);
/**
*
* Returns a list of package import jobs.
*
*
* @param listPackageImportJobsRequest
* @return Result of the ListPackageImportJobs operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListPackageImportJobs
* @see AWS
* API Documentation
*/
ListPackageImportJobsResult listPackageImportJobs(ListPackageImportJobsRequest listPackageImportJobsRequest);
/**
*
* Returns a list of packages.
*
*
* @param listPackagesRequest
* @return Result of the ListPackages operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListPackages
* @see AWS API
* Documentation
*/
ListPackagesResult listPackages(ListPackagesRequest listPackagesRequest);
/**
*
* Returns a list of tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Creates a device and returns a configuration archive. The configuration archive is a ZIP file that contains a
* provisioning certificate that is valid for 5 minutes. Name the configuration archive
* certificates-omni_device-name.zip
and transfer it to the device within 5 minutes. Use the
* included USB storage device and connect it to the USB 3.0 port next to the HDMI output.
*
*
* @param provisionDeviceRequest
* @return Result of the ProvisionDevice operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ServiceQuotaExceededException
* The request would cause a limit to be exceeded.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.ProvisionDevice
* @see AWS API
* Documentation
*/
ProvisionDeviceResult provisionDevice(ProvisionDeviceRequest provisionDeviceRequest);
/**
*
* Registers a package version.
*
*
* @param registerPackageVersionRequest
* @return Result of the RegisterPackageVersion operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.RegisterPackageVersion
* @see AWS API Documentation
*/
RegisterPackageVersionResult registerPackageVersion(RegisterPackageVersionRequest registerPackageVersionRequest);
/**
*
* Removes an application instance.
*
*
* @param removeApplicationInstanceRequest
* @return Result of the RemoveApplicationInstance operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.RemoveApplicationInstance
* @see AWS API Documentation
*/
RemoveApplicationInstanceResult removeApplicationInstance(RemoveApplicationInstanceRequest removeApplicationInstanceRequest);
/**
*
* Signal camera nodes to stop or resume.
*
*
* @param signalApplicationInstanceNodeInstancesRequest
* @return Result of the SignalApplicationInstanceNodeInstances operation returned by the service.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ServiceQuotaExceededException
* The request would cause a limit to be exceeded.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.SignalApplicationInstanceNodeInstances
* @see AWS API Documentation
*/
SignalApplicationInstanceNodeInstancesResult signalApplicationInstanceNodeInstances(
SignalApplicationInstanceNodeInstancesRequest signalApplicationInstanceNodeInstancesRequest);
/**
*
* Tags a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a device's metadata.
*
*
* @param updateDeviceMetadataRequest
* @return Result of the UpdateDeviceMetadata operation returned by the service.
* @throws ConflictException
* The target resource is in use.
* @throws ValidationException
* The request contains an invalid parameter value.
* @throws AccessDeniedException
* The requestor does not have permission to access the target action or resource.
* @throws ResourceNotFoundException
* The target resource was not found.
* @throws InternalServerException
* An internal error occurred.
* @sample AWSPanorama.UpdateDeviceMetadata
* @see AWS
* API Documentation
*/
UpdateDeviceMetadataResult updateDeviceMetadata(UpdateDeviceMetadataRequest updateDeviceMetadataRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}