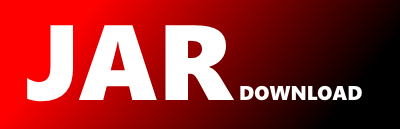
com.amazonaws.services.panorama.AWSPanoramaAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-panorama Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.panorama;
import javax.annotation.Generated;
import com.amazonaws.services.panorama.model.*;
/**
* Interface for accessing Panorama asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.panorama.AbstractAWSPanoramaAsync} instead.
*
*
*
* AWS Panorama
*
* Overview
*
*
* This is the AWS Panorama API Reference. For an introduction to the service, see What is AWS Panorama? in the AWS
* Panorama Developer Guide.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSPanoramaAsync extends AWSPanorama {
/**
*
* Creates an application instance and deploys it to a device.
*
*
* @param createApplicationInstanceRequest
* @return A Java Future containing the result of the CreateApplicationInstance operation returned by the service.
* @sample AWSPanoramaAsync.CreateApplicationInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationInstanceAsync(
CreateApplicationInstanceRequest createApplicationInstanceRequest);
/**
*
* Creates an application instance and deploys it to a device.
*
*
* @param createApplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApplicationInstance operation returned by the service.
* @sample AWSPanoramaAsyncHandler.CreateApplicationInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationInstanceAsync(
CreateApplicationInstanceRequest createApplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a job to run on a device. A job can update a device's software or reboot it.
*
*
* @param createJobForDevicesRequest
* @return A Java Future containing the result of the CreateJobForDevices operation returned by the service.
* @sample AWSPanoramaAsync.CreateJobForDevices
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createJobForDevicesAsync(CreateJobForDevicesRequest createJobForDevicesRequest);
/**
*
* Creates a job to run on a device. A job can update a device's software or reboot it.
*
*
* @param createJobForDevicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateJobForDevices operation returned by the service.
* @sample AWSPanoramaAsyncHandler.CreateJobForDevices
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createJobForDevicesAsync(CreateJobForDevicesRequest createJobForDevicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a camera stream node.
*
*
* @param createNodeFromTemplateJobRequest
* @return A Java Future containing the result of the CreateNodeFromTemplateJob operation returned by the service.
* @sample AWSPanoramaAsync.CreateNodeFromTemplateJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createNodeFromTemplateJobAsync(
CreateNodeFromTemplateJobRequest createNodeFromTemplateJobRequest);
/**
*
* Creates a camera stream node.
*
*
* @param createNodeFromTemplateJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNodeFromTemplateJob operation returned by the service.
* @sample AWSPanoramaAsyncHandler.CreateNodeFromTemplateJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createNodeFromTemplateJobAsync(
CreateNodeFromTemplateJobRequest createNodeFromTemplateJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a package and storage location in an Amazon S3 access point.
*
*
* @param createPackageRequest
* @return A Java Future containing the result of the CreatePackage operation returned by the service.
* @sample AWSPanoramaAsync.CreatePackage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPackageAsync(CreatePackageRequest createPackageRequest);
/**
*
* Creates a package and storage location in an Amazon S3 access point.
*
*
* @param createPackageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePackage operation returned by the service.
* @sample AWSPanoramaAsyncHandler.CreatePackage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPackageAsync(CreatePackageRequest createPackageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Imports a node package.
*
*
* @param createPackageImportJobRequest
* @return A Java Future containing the result of the CreatePackageImportJob operation returned by the service.
* @sample AWSPanoramaAsync.CreatePackageImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createPackageImportJobAsync(CreatePackageImportJobRequest createPackageImportJobRequest);
/**
*
* Imports a node package.
*
*
* @param createPackageImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePackageImportJob operation returned by the service.
* @sample AWSPanoramaAsyncHandler.CreatePackageImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createPackageImportJobAsync(CreatePackageImportJobRequest createPackageImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a device.
*
*
* @param deleteDeviceRequest
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* @sample AWSPanoramaAsync.DeleteDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDeviceAsync(DeleteDeviceRequest deleteDeviceRequest);
/**
*
* Deletes a device.
*
*
* @param deleteDeviceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DeleteDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDeviceAsync(DeleteDeviceRequest deleteDeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a package.
*
*
*
* To delete a package, you need permission to call s3:DeleteObject
in addition to permissions for the
* AWS Panorama API.
*
*
*
* @param deletePackageRequest
* @return A Java Future containing the result of the DeletePackage operation returned by the service.
* @sample AWSPanoramaAsync.DeletePackage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePackageAsync(DeletePackageRequest deletePackageRequest);
/**
*
* Deletes a package.
*
*
*
* To delete a package, you need permission to call s3:DeleteObject
in addition to permissions for the
* AWS Panorama API.
*
*
*
* @param deletePackageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePackage operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DeletePackage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePackageAsync(DeletePackageRequest deletePackageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters a package version.
*
*
* @param deregisterPackageVersionRequest
* @return A Java Future containing the result of the DeregisterPackageVersion operation returned by the service.
* @sample AWSPanoramaAsync.DeregisterPackageVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterPackageVersionAsync(DeregisterPackageVersionRequest deregisterPackageVersionRequest);
/**
*
* Deregisters a package version.
*
*
* @param deregisterPackageVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterPackageVersion operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DeregisterPackageVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterPackageVersionAsync(DeregisterPackageVersionRequest deregisterPackageVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about an application instance on a device.
*
*
* @param describeApplicationInstanceRequest
* @return A Java Future containing the result of the DescribeApplicationInstance operation returned by the service.
* @sample AWSPanoramaAsync.DescribeApplicationInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicationInstanceAsync(
DescribeApplicationInstanceRequest describeApplicationInstanceRequest);
/**
*
* Returns information about an application instance on a device.
*
*
* @param describeApplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeApplicationInstance operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DescribeApplicationInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicationInstanceAsync(
DescribeApplicationInstanceRequest describeApplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about an application instance's configuration manifest.
*
*
* @param describeApplicationInstanceDetailsRequest
* @return A Java Future containing the result of the DescribeApplicationInstanceDetails operation returned by the
* service.
* @sample AWSPanoramaAsync.DescribeApplicationInstanceDetails
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicationInstanceDetailsAsync(
DescribeApplicationInstanceDetailsRequest describeApplicationInstanceDetailsRequest);
/**
*
* Returns information about an application instance's configuration manifest.
*
*
* @param describeApplicationInstanceDetailsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeApplicationInstanceDetails operation returned by the
* service.
* @sample AWSPanoramaAsyncHandler.DescribeApplicationInstanceDetails
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicationInstanceDetailsAsync(
DescribeApplicationInstanceDetailsRequest describeApplicationInstanceDetailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a device.
*
*
* @param describeDeviceRequest
* @return A Java Future containing the result of the DescribeDevice operation returned by the service.
* @sample AWSPanoramaAsync.DescribeDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDeviceAsync(DescribeDeviceRequest describeDeviceRequest);
/**
*
* Returns information about a device.
*
*
* @param describeDeviceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDevice operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DescribeDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDeviceAsync(DescribeDeviceRequest describeDeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a device job.
*
*
* @param describeDeviceJobRequest
* @return A Java Future containing the result of the DescribeDeviceJob operation returned by the service.
* @sample AWSPanoramaAsync.DescribeDeviceJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDeviceJobAsync(DescribeDeviceJobRequest describeDeviceJobRequest);
/**
*
* Returns information about a device job.
*
*
* @param describeDeviceJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDeviceJob operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DescribeDeviceJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDeviceJobAsync(DescribeDeviceJobRequest describeDeviceJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a node.
*
*
* @param describeNodeRequest
* @return A Java Future containing the result of the DescribeNode operation returned by the service.
* @sample AWSPanoramaAsync.DescribeNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeNodeAsync(DescribeNodeRequest describeNodeRequest);
/**
*
* Returns information about a node.
*
*
* @param describeNodeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeNode operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DescribeNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeNodeAsync(DescribeNodeRequest describeNodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a job to create a camera stream node.
*
*
* @param describeNodeFromTemplateJobRequest
* @return A Java Future containing the result of the DescribeNodeFromTemplateJob operation returned by the service.
* @sample AWSPanoramaAsync.DescribeNodeFromTemplateJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeNodeFromTemplateJobAsync(
DescribeNodeFromTemplateJobRequest describeNodeFromTemplateJobRequest);
/**
*
* Returns information about a job to create a camera stream node.
*
*
* @param describeNodeFromTemplateJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeNodeFromTemplateJob operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DescribeNodeFromTemplateJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeNodeFromTemplateJobAsync(
DescribeNodeFromTemplateJobRequest describeNodeFromTemplateJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a package.
*
*
* @param describePackageRequest
* @return A Java Future containing the result of the DescribePackage operation returned by the service.
* @sample AWSPanoramaAsync.DescribePackage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describePackageAsync(DescribePackageRequest describePackageRequest);
/**
*
* Returns information about a package.
*
*
* @param describePackageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePackage operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DescribePackage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describePackageAsync(DescribePackageRequest describePackageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a package import job.
*
*
* @param describePackageImportJobRequest
* @return A Java Future containing the result of the DescribePackageImportJob operation returned by the service.
* @sample AWSPanoramaAsync.DescribePackageImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describePackageImportJobAsync(DescribePackageImportJobRequest describePackageImportJobRequest);
/**
*
* Returns information about a package import job.
*
*
* @param describePackageImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePackageImportJob operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DescribePackageImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describePackageImportJobAsync(DescribePackageImportJobRequest describePackageImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a package version.
*
*
* @param describePackageVersionRequest
* @return A Java Future containing the result of the DescribePackageVersion operation returned by the service.
* @sample AWSPanoramaAsync.DescribePackageVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future describePackageVersionAsync(DescribePackageVersionRequest describePackageVersionRequest);
/**
*
* Returns information about a package version.
*
*
* @param describePackageVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePackageVersion operation returned by the service.
* @sample AWSPanoramaAsyncHandler.DescribePackageVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future describePackageVersionAsync(DescribePackageVersionRequest describePackageVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of application instance dependencies.
*
*
* @param listApplicationInstanceDependenciesRequest
* @return A Java Future containing the result of the ListApplicationInstanceDependencies operation returned by the
* service.
* @sample AWSPanoramaAsync.ListApplicationInstanceDependencies
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationInstanceDependenciesAsync(
ListApplicationInstanceDependenciesRequest listApplicationInstanceDependenciesRequest);
/**
*
* Returns a list of application instance dependencies.
*
*
* @param listApplicationInstanceDependenciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplicationInstanceDependencies operation returned by the
* service.
* @sample AWSPanoramaAsyncHandler.ListApplicationInstanceDependencies
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationInstanceDependenciesAsync(
ListApplicationInstanceDependenciesRequest listApplicationInstanceDependenciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of application node instances.
*
*
* @param listApplicationInstanceNodeInstancesRequest
* @return A Java Future containing the result of the ListApplicationInstanceNodeInstances operation returned by the
* service.
* @sample AWSPanoramaAsync.ListApplicationInstanceNodeInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationInstanceNodeInstancesAsync(
ListApplicationInstanceNodeInstancesRequest listApplicationInstanceNodeInstancesRequest);
/**
*
* Returns a list of application node instances.
*
*
* @param listApplicationInstanceNodeInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplicationInstanceNodeInstances operation returned by the
* service.
* @sample AWSPanoramaAsyncHandler.ListApplicationInstanceNodeInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationInstanceNodeInstancesAsync(
ListApplicationInstanceNodeInstancesRequest listApplicationInstanceNodeInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of application instances.
*
*
* @param listApplicationInstancesRequest
* @return A Java Future containing the result of the ListApplicationInstances operation returned by the service.
* @sample AWSPanoramaAsync.ListApplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationInstancesAsync(ListApplicationInstancesRequest listApplicationInstancesRequest);
/**
*
* Returns a list of application instances.
*
*
* @param listApplicationInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplicationInstances operation returned by the service.
* @sample AWSPanoramaAsyncHandler.ListApplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationInstancesAsync(ListApplicationInstancesRequest listApplicationInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of devices.
*
*
* @param listDevicesRequest
* @return A Java Future containing the result of the ListDevices operation returned by the service.
* @sample AWSPanoramaAsync.ListDevices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDevicesAsync(ListDevicesRequest listDevicesRequest);
/**
*
* Returns a list of devices.
*
*
* @param listDevicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDevices operation returned by the service.
* @sample AWSPanoramaAsyncHandler.ListDevices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDevicesAsync(ListDevicesRequest listDevicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of jobs.
*
*
* @param listDevicesJobsRequest
* @return A Java Future containing the result of the ListDevicesJobs operation returned by the service.
* @sample AWSPanoramaAsync.ListDevicesJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDevicesJobsAsync(ListDevicesJobsRequest listDevicesJobsRequest);
/**
*
* Returns a list of jobs.
*
*
* @param listDevicesJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDevicesJobs operation returned by the service.
* @sample AWSPanoramaAsyncHandler.ListDevicesJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDevicesJobsAsync(ListDevicesJobsRequest listDevicesJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of camera stream node jobs.
*
*
* @param listNodeFromTemplateJobsRequest
* @return A Java Future containing the result of the ListNodeFromTemplateJobs operation returned by the service.
* @sample AWSPanoramaAsync.ListNodeFromTemplateJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listNodeFromTemplateJobsAsync(ListNodeFromTemplateJobsRequest listNodeFromTemplateJobsRequest);
/**
*
* Returns a list of camera stream node jobs.
*
*
* @param listNodeFromTemplateJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListNodeFromTemplateJobs operation returned by the service.
* @sample AWSPanoramaAsyncHandler.ListNodeFromTemplateJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listNodeFromTemplateJobsAsync(ListNodeFromTemplateJobsRequest listNodeFromTemplateJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of nodes.
*
*
* @param listNodesRequest
* @return A Java Future containing the result of the ListNodes operation returned by the service.
* @sample AWSPanoramaAsync.ListNodes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listNodesAsync(ListNodesRequest listNodesRequest);
/**
*
* Returns a list of nodes.
*
*
* @param listNodesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListNodes operation returned by the service.
* @sample AWSPanoramaAsyncHandler.ListNodes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listNodesAsync(ListNodesRequest listNodesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of package import jobs.
*
*
* @param listPackageImportJobsRequest
* @return A Java Future containing the result of the ListPackageImportJobs operation returned by the service.
* @sample AWSPanoramaAsync.ListPackageImportJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listPackageImportJobsAsync(ListPackageImportJobsRequest listPackageImportJobsRequest);
/**
*
* Returns a list of package import jobs.
*
*
* @param listPackageImportJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPackageImportJobs operation returned by the service.
* @sample AWSPanoramaAsyncHandler.ListPackageImportJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listPackageImportJobsAsync(ListPackageImportJobsRequest listPackageImportJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of packages.
*
*
* @param listPackagesRequest
* @return A Java Future containing the result of the ListPackages operation returned by the service.
* @sample AWSPanoramaAsync.ListPackages
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPackagesAsync(ListPackagesRequest listPackagesRequest);
/**
*
* Returns a list of packages.
*
*
* @param listPackagesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPackages operation returned by the service.
* @sample AWSPanoramaAsyncHandler.ListPackages
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPackagesAsync(ListPackagesRequest listPackagesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSPanoramaAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns a list of tags for a resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSPanoramaAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a device and returns a configuration archive. The configuration archive is a ZIP file that contains a
* provisioning certificate that is valid for 5 minutes. Name the configuration archive
* certificates-omni_device-name.zip
and transfer it to the device within 5 minutes. Use the
* included USB storage device and connect it to the USB 3.0 port next to the HDMI output.
*
*
* @param provisionDeviceRequest
* @return A Java Future containing the result of the ProvisionDevice operation returned by the service.
* @sample AWSPanoramaAsync.ProvisionDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future provisionDeviceAsync(ProvisionDeviceRequest provisionDeviceRequest);
/**
*
* Creates a device and returns a configuration archive. The configuration archive is a ZIP file that contains a
* provisioning certificate that is valid for 5 minutes. Name the configuration archive
* certificates-omni_device-name.zip
and transfer it to the device within 5 minutes. Use the
* included USB storage device and connect it to the USB 3.0 port next to the HDMI output.
*
*
* @param provisionDeviceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ProvisionDevice operation returned by the service.
* @sample AWSPanoramaAsyncHandler.ProvisionDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future provisionDeviceAsync(ProvisionDeviceRequest provisionDeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers a package version.
*
*
* @param registerPackageVersionRequest
* @return A Java Future containing the result of the RegisterPackageVersion operation returned by the service.
* @sample AWSPanoramaAsync.RegisterPackageVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future registerPackageVersionAsync(RegisterPackageVersionRequest registerPackageVersionRequest);
/**
*
* Registers a package version.
*
*
* @param registerPackageVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterPackageVersion operation returned by the service.
* @sample AWSPanoramaAsyncHandler.RegisterPackageVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future registerPackageVersionAsync(RegisterPackageVersionRequest registerPackageVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes an application instance.
*
*
* @param removeApplicationInstanceRequest
* @return A Java Future containing the result of the RemoveApplicationInstance operation returned by the service.
* @sample AWSPanoramaAsync.RemoveApplicationInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future removeApplicationInstanceAsync(
RemoveApplicationInstanceRequest removeApplicationInstanceRequest);
/**
*
* Removes an application instance.
*
*
* @param removeApplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveApplicationInstance operation returned by the service.
* @sample AWSPanoramaAsyncHandler.RemoveApplicationInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future removeApplicationInstanceAsync(
RemoveApplicationInstanceRequest removeApplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Signal camera nodes to stop or resume.
*
*
* @param signalApplicationInstanceNodeInstancesRequest
* @return A Java Future containing the result of the SignalApplicationInstanceNodeInstances operation returned by
* the service.
* @sample AWSPanoramaAsync.SignalApplicationInstanceNodeInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future signalApplicationInstanceNodeInstancesAsync(
SignalApplicationInstanceNodeInstancesRequest signalApplicationInstanceNodeInstancesRequest);
/**
*
* Signal camera nodes to stop or resume.
*
*
* @param signalApplicationInstanceNodeInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SignalApplicationInstanceNodeInstances operation returned by
* the service.
* @sample AWSPanoramaAsyncHandler.SignalApplicationInstanceNodeInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future signalApplicationInstanceNodeInstancesAsync(
SignalApplicationInstanceNodeInstancesRequest signalApplicationInstanceNodeInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tags a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSPanoramaAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Tags a resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSPanoramaAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSPanoramaAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSPanoramaAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a device's metadata.
*
*
* @param updateDeviceMetadataRequest
* @return A Java Future containing the result of the UpdateDeviceMetadata operation returned by the service.
* @sample AWSPanoramaAsync.UpdateDeviceMetadata
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDeviceMetadataAsync(UpdateDeviceMetadataRequest updateDeviceMetadataRequest);
/**
*
* Updates a device's metadata.
*
*
* @param updateDeviceMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDeviceMetadata operation returned by the service.
* @sample AWSPanoramaAsyncHandler.UpdateDeviceMetadata
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDeviceMetadataAsync(UpdateDeviceMetadataRequest updateDeviceMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}