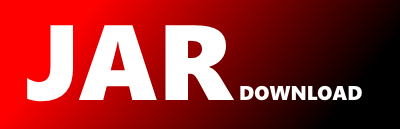
com.amazonaws.services.paymentcryptography.model.WrappedKey Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.paymentcryptography.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Parameter information for generating a WrappedKeyBlock for key exchange.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class WrappedKey implements Serializable, Cloneable, StructuredPojo {
/**
*
* The KeyARN
of the wrapped key.
*
*/
private String wrappingKeyArn;
/**
*
* The key block format of a wrapped key.
*
*/
private String wrappedKeyMaterialFormat;
/**
*
* Parameter information for generating a wrapped key using TR-31 or TR-34 skey exchange method.
*
*/
private String keyMaterial;
/**
*
* The key check value (KCV) is used to check if all parties holding a given key have the same key or to detect that
* a key has changed.
*
*/
private String keyCheckValue;
/**
*
* The algorithm that Amazon Web Services Payment Cryptography uses to calculate the key check value (KCV). It is
* used to validate the key integrity.
*
*
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be checked and
* retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is computed using a CMAC
* algorithm where the input data is 16 bytes of zero and retaining the 3 highest order bytes of the encrypted
* result.
*
*/
private String keyCheckValueAlgorithm;
/**
*
* The KeyARN
of the wrapped key.
*
*
* @param wrappingKeyArn
* The KeyARN
of the wrapped key.
*/
public void setWrappingKeyArn(String wrappingKeyArn) {
this.wrappingKeyArn = wrappingKeyArn;
}
/**
*
* The KeyARN
of the wrapped key.
*
*
* @return The KeyARN
of the wrapped key.
*/
public String getWrappingKeyArn() {
return this.wrappingKeyArn;
}
/**
*
* The KeyARN
of the wrapped key.
*
*
* @param wrappingKeyArn
* The KeyARN
of the wrapped key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WrappedKey withWrappingKeyArn(String wrappingKeyArn) {
setWrappingKeyArn(wrappingKeyArn);
return this;
}
/**
*
* The key block format of a wrapped key.
*
*
* @param wrappedKeyMaterialFormat
* The key block format of a wrapped key.
* @see WrappedKeyMaterialFormat
*/
public void setWrappedKeyMaterialFormat(String wrappedKeyMaterialFormat) {
this.wrappedKeyMaterialFormat = wrappedKeyMaterialFormat;
}
/**
*
* The key block format of a wrapped key.
*
*
* @return The key block format of a wrapped key.
* @see WrappedKeyMaterialFormat
*/
public String getWrappedKeyMaterialFormat() {
return this.wrappedKeyMaterialFormat;
}
/**
*
* The key block format of a wrapped key.
*
*
* @param wrappedKeyMaterialFormat
* The key block format of a wrapped key.
* @return Returns a reference to this object so that method calls can be chained together.
* @see WrappedKeyMaterialFormat
*/
public WrappedKey withWrappedKeyMaterialFormat(String wrappedKeyMaterialFormat) {
setWrappedKeyMaterialFormat(wrappedKeyMaterialFormat);
return this;
}
/**
*
* The key block format of a wrapped key.
*
*
* @param wrappedKeyMaterialFormat
* The key block format of a wrapped key.
* @return Returns a reference to this object so that method calls can be chained together.
* @see WrappedKeyMaterialFormat
*/
public WrappedKey withWrappedKeyMaterialFormat(WrappedKeyMaterialFormat wrappedKeyMaterialFormat) {
this.wrappedKeyMaterialFormat = wrappedKeyMaterialFormat.toString();
return this;
}
/**
*
* Parameter information for generating a wrapped key using TR-31 or TR-34 skey exchange method.
*
*
* @param keyMaterial
* Parameter information for generating a wrapped key using TR-31 or TR-34 skey exchange method.
*/
public void setKeyMaterial(String keyMaterial) {
this.keyMaterial = keyMaterial;
}
/**
*
* Parameter information for generating a wrapped key using TR-31 or TR-34 skey exchange method.
*
*
* @return Parameter information for generating a wrapped key using TR-31 or TR-34 skey exchange method.
*/
public String getKeyMaterial() {
return this.keyMaterial;
}
/**
*
* Parameter information for generating a wrapped key using TR-31 or TR-34 skey exchange method.
*
*
* @param keyMaterial
* Parameter information for generating a wrapped key using TR-31 or TR-34 skey exchange method.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WrappedKey withKeyMaterial(String keyMaterial) {
setKeyMaterial(keyMaterial);
return this;
}
/**
*
* The key check value (KCV) is used to check if all parties holding a given key have the same key or to detect that
* a key has changed.
*
*
* @param keyCheckValue
* The key check value (KCV) is used to check if all parties holding a given key have the same key or to
* detect that a key has changed.
*/
public void setKeyCheckValue(String keyCheckValue) {
this.keyCheckValue = keyCheckValue;
}
/**
*
* The key check value (KCV) is used to check if all parties holding a given key have the same key or to detect that
* a key has changed.
*
*
* @return The key check value (KCV) is used to check if all parties holding a given key have the same key or to
* detect that a key has changed.
*/
public String getKeyCheckValue() {
return this.keyCheckValue;
}
/**
*
* The key check value (KCV) is used to check if all parties holding a given key have the same key or to detect that
* a key has changed.
*
*
* @param keyCheckValue
* The key check value (KCV) is used to check if all parties holding a given key have the same key or to
* detect that a key has changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WrappedKey withKeyCheckValue(String keyCheckValue) {
setKeyCheckValue(keyCheckValue);
return this;
}
/**
*
* The algorithm that Amazon Web Services Payment Cryptography uses to calculate the key check value (KCV). It is
* used to validate the key integrity.
*
*
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be checked and
* retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is computed using a CMAC
* algorithm where the input data is 16 bytes of zero and retaining the 3 highest order bytes of the encrypted
* result.
*
*
* @param keyCheckValueAlgorithm
* The algorithm that Amazon Web Services Payment Cryptography uses to calculate the key check value (KCV).
* It is used to validate the key integrity.
*
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be
* checked and retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is computed
* using a CMAC algorithm where the input data is 16 bytes of zero and retaining the 3 highest order bytes of
* the encrypted result.
* @see KeyCheckValueAlgorithm
*/
public void setKeyCheckValueAlgorithm(String keyCheckValueAlgorithm) {
this.keyCheckValueAlgorithm = keyCheckValueAlgorithm;
}
/**
*
* The algorithm that Amazon Web Services Payment Cryptography uses to calculate the key check value (KCV). It is
* used to validate the key integrity.
*
*
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be checked and
* retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is computed using a CMAC
* algorithm where the input data is 16 bytes of zero and retaining the 3 highest order bytes of the encrypted
* result.
*
*
* @return The algorithm that Amazon Web Services Payment Cryptography uses to calculate the key check value (KCV).
* It is used to validate the key integrity.
*
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be
* checked and retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is
* computed using a CMAC algorithm where the input data is 16 bytes of zero and retaining the 3 highest
* order bytes of the encrypted result.
* @see KeyCheckValueAlgorithm
*/
public String getKeyCheckValueAlgorithm() {
return this.keyCheckValueAlgorithm;
}
/**
*
* The algorithm that Amazon Web Services Payment Cryptography uses to calculate the key check value (KCV). It is
* used to validate the key integrity.
*
*
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be checked and
* retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is computed using a CMAC
* algorithm where the input data is 16 bytes of zero and retaining the 3 highest order bytes of the encrypted
* result.
*
*
* @param keyCheckValueAlgorithm
* The algorithm that Amazon Web Services Payment Cryptography uses to calculate the key check value (KCV).
* It is used to validate the key integrity.
*
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be
* checked and retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is computed
* using a CMAC algorithm where the input data is 16 bytes of zero and retaining the 3 highest order bytes of
* the encrypted result.
* @return Returns a reference to this object so that method calls can be chained together.
* @see KeyCheckValueAlgorithm
*/
public WrappedKey withKeyCheckValueAlgorithm(String keyCheckValueAlgorithm) {
setKeyCheckValueAlgorithm(keyCheckValueAlgorithm);
return this;
}
/**
*
* The algorithm that Amazon Web Services Payment Cryptography uses to calculate the key check value (KCV). It is
* used to validate the key integrity.
*
*
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be checked and
* retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is computed using a CMAC
* algorithm where the input data is 16 bytes of zero and retaining the 3 highest order bytes of the encrypted
* result.
*
*
* @param keyCheckValueAlgorithm
* The algorithm that Amazon Web Services Payment Cryptography uses to calculate the key check value (KCV).
* It is used to validate the key integrity.
*
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be
* checked and retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is computed
* using a CMAC algorithm where the input data is 16 bytes of zero and retaining the 3 highest order bytes of
* the encrypted result.
* @return Returns a reference to this object so that method calls can be chained together.
* @see KeyCheckValueAlgorithm
*/
public WrappedKey withKeyCheckValueAlgorithm(KeyCheckValueAlgorithm keyCheckValueAlgorithm) {
this.keyCheckValueAlgorithm = keyCheckValueAlgorithm.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWrappingKeyArn() != null)
sb.append("WrappingKeyArn: ").append(getWrappingKeyArn()).append(",");
if (getWrappedKeyMaterialFormat() != null)
sb.append("WrappedKeyMaterialFormat: ").append(getWrappedKeyMaterialFormat()).append(",");
if (getKeyMaterial() != null)
sb.append("KeyMaterial: ").append("***Sensitive Data Redacted***").append(",");
if (getKeyCheckValue() != null)
sb.append("KeyCheckValue: ").append(getKeyCheckValue()).append(",");
if (getKeyCheckValueAlgorithm() != null)
sb.append("KeyCheckValueAlgorithm: ").append(getKeyCheckValueAlgorithm());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof WrappedKey == false)
return false;
WrappedKey other = (WrappedKey) obj;
if (other.getWrappingKeyArn() == null ^ this.getWrappingKeyArn() == null)
return false;
if (other.getWrappingKeyArn() != null && other.getWrappingKeyArn().equals(this.getWrappingKeyArn()) == false)
return false;
if (other.getWrappedKeyMaterialFormat() == null ^ this.getWrappedKeyMaterialFormat() == null)
return false;
if (other.getWrappedKeyMaterialFormat() != null && other.getWrappedKeyMaterialFormat().equals(this.getWrappedKeyMaterialFormat()) == false)
return false;
if (other.getKeyMaterial() == null ^ this.getKeyMaterial() == null)
return false;
if (other.getKeyMaterial() != null && other.getKeyMaterial().equals(this.getKeyMaterial()) == false)
return false;
if (other.getKeyCheckValue() == null ^ this.getKeyCheckValue() == null)
return false;
if (other.getKeyCheckValue() != null && other.getKeyCheckValue().equals(this.getKeyCheckValue()) == false)
return false;
if (other.getKeyCheckValueAlgorithm() == null ^ this.getKeyCheckValueAlgorithm() == null)
return false;
if (other.getKeyCheckValueAlgorithm() != null && other.getKeyCheckValueAlgorithm().equals(this.getKeyCheckValueAlgorithm()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWrappingKeyArn() == null) ? 0 : getWrappingKeyArn().hashCode());
hashCode = prime * hashCode + ((getWrappedKeyMaterialFormat() == null) ? 0 : getWrappedKeyMaterialFormat().hashCode());
hashCode = prime * hashCode + ((getKeyMaterial() == null) ? 0 : getKeyMaterial().hashCode());
hashCode = prime * hashCode + ((getKeyCheckValue() == null) ? 0 : getKeyCheckValue().hashCode());
hashCode = prime * hashCode + ((getKeyCheckValueAlgorithm() == null) ? 0 : getKeyCheckValueAlgorithm().hashCode());
return hashCode;
}
@Override
public WrappedKey clone() {
try {
return (WrappedKey) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.paymentcryptography.model.transform.WrappedKeyMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}