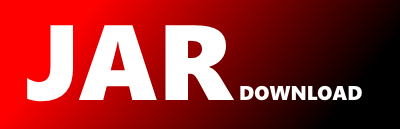
com.amazonaws.services.paymentcryptography.model.ExportTr34KeyBlock Maven / Gradle / Ivy
Show all versions of aws-java-sdk-paymentcryptography Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.paymentcryptography.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Parameter information for key material export using the asymmetric TR-34 key exchange method.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ExportTr34KeyBlock implements Serializable, Cloneable, StructuredPojo {
/**
*
* The KeyARN
of the certificate chain that signs the wrapping key certificate during TR-34 key export.
*
*/
private String certificateAuthorityPublicKeyIdentifier;
/**
*
* The KeyARN
of the wrapping key certificate. Amazon Web Services Payment Cryptography uses this
* certificate to wrap the key under export.
*
*/
private String wrappingKeyCertificate;
/**
*
* The export token to initiate key export from Amazon Web Services Payment Cryptography. It also contains the
* signing key certificate that will sign the wrapped key during TR-34 key block generation. Call GetParametersForExport to receive an export token. It expires after 7 days. You can use the same export
* token to export multiple keys from the same service account.
*
*/
private String exportToken;
/**
*
* The format of key block that Amazon Web Services Payment Cryptography will use during key export.
*
*/
private String keyBlockFormat;
/**
*
* A random number value that is unique to the TR-34 key block generated using 2 pass. The operation will fail, if a
* random nonce value is not provided for a TR-34 key block generated using 2 pass.
*
*/
private String randomNonce;
/**
*
* Optional metadata for export associated with the key material. This data is signed but transmitted in clear text.
*
*/
private KeyBlockHeaders keyBlockHeaders;
/**
*
* The KeyARN
of the certificate chain that signs the wrapping key certificate during TR-34 key export.
*
*
* @param certificateAuthorityPublicKeyIdentifier
* The KeyARN
of the certificate chain that signs the wrapping key certificate during TR-34 key
* export.
*/
public void setCertificateAuthorityPublicKeyIdentifier(String certificateAuthorityPublicKeyIdentifier) {
this.certificateAuthorityPublicKeyIdentifier = certificateAuthorityPublicKeyIdentifier;
}
/**
*
* The KeyARN
of the certificate chain that signs the wrapping key certificate during TR-34 key export.
*
*
* @return The KeyARN
of the certificate chain that signs the wrapping key certificate during TR-34 key
* export.
*/
public String getCertificateAuthorityPublicKeyIdentifier() {
return this.certificateAuthorityPublicKeyIdentifier;
}
/**
*
* The KeyARN
of the certificate chain that signs the wrapping key certificate during TR-34 key export.
*
*
* @param certificateAuthorityPublicKeyIdentifier
* The KeyARN
of the certificate chain that signs the wrapping key certificate during TR-34 key
* export.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ExportTr34KeyBlock withCertificateAuthorityPublicKeyIdentifier(String certificateAuthorityPublicKeyIdentifier) {
setCertificateAuthorityPublicKeyIdentifier(certificateAuthorityPublicKeyIdentifier);
return this;
}
/**
*
* The KeyARN
of the wrapping key certificate. Amazon Web Services Payment Cryptography uses this
* certificate to wrap the key under export.
*
*
* @param wrappingKeyCertificate
* The KeyARN
of the wrapping key certificate. Amazon Web Services Payment Cryptography uses
* this certificate to wrap the key under export.
*/
public void setWrappingKeyCertificate(String wrappingKeyCertificate) {
this.wrappingKeyCertificate = wrappingKeyCertificate;
}
/**
*
* The KeyARN
of the wrapping key certificate. Amazon Web Services Payment Cryptography uses this
* certificate to wrap the key under export.
*
*
* @return The KeyARN
of the wrapping key certificate. Amazon Web Services Payment Cryptography uses
* this certificate to wrap the key under export.
*/
public String getWrappingKeyCertificate() {
return this.wrappingKeyCertificate;
}
/**
*
* The KeyARN
of the wrapping key certificate. Amazon Web Services Payment Cryptography uses this
* certificate to wrap the key under export.
*
*
* @param wrappingKeyCertificate
* The KeyARN
of the wrapping key certificate. Amazon Web Services Payment Cryptography uses
* this certificate to wrap the key under export.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ExportTr34KeyBlock withWrappingKeyCertificate(String wrappingKeyCertificate) {
setWrappingKeyCertificate(wrappingKeyCertificate);
return this;
}
/**
*
* The export token to initiate key export from Amazon Web Services Payment Cryptography. It also contains the
* signing key certificate that will sign the wrapped key during TR-34 key block generation. Call GetParametersForExport to receive an export token. It expires after 7 days. You can use the same export
* token to export multiple keys from the same service account.
*
*
* @param exportToken
* The export token to initiate key export from Amazon Web Services Payment Cryptography. It also contains
* the signing key certificate that will sign the wrapped key during TR-34 key block generation. Call GetParametersForExport to receive an export token. It expires after 7 days. You can use the same
* export token to export multiple keys from the same service account.
*/
public void setExportToken(String exportToken) {
this.exportToken = exportToken;
}
/**
*
* The export token to initiate key export from Amazon Web Services Payment Cryptography. It also contains the
* signing key certificate that will sign the wrapped key during TR-34 key block generation. Call GetParametersForExport to receive an export token. It expires after 7 days. You can use the same export
* token to export multiple keys from the same service account.
*
*
* @return The export token to initiate key export from Amazon Web Services Payment Cryptography. It also contains
* the signing key certificate that will sign the wrapped key during TR-34 key block generation. Call GetParametersForExport to receive an export token. It expires after 7 days. You can use the same
* export token to export multiple keys from the same service account.
*/
public String getExportToken() {
return this.exportToken;
}
/**
*
* The export token to initiate key export from Amazon Web Services Payment Cryptography. It also contains the
* signing key certificate that will sign the wrapped key during TR-34 key block generation. Call GetParametersForExport to receive an export token. It expires after 7 days. You can use the same export
* token to export multiple keys from the same service account.
*
*
* @param exportToken
* The export token to initiate key export from Amazon Web Services Payment Cryptography. It also contains
* the signing key certificate that will sign the wrapped key during TR-34 key block generation. Call GetParametersForExport to receive an export token. It expires after 7 days. You can use the same
* export token to export multiple keys from the same service account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ExportTr34KeyBlock withExportToken(String exportToken) {
setExportToken(exportToken);
return this;
}
/**
*
* The format of key block that Amazon Web Services Payment Cryptography will use during key export.
*
*
* @param keyBlockFormat
* The format of key block that Amazon Web Services Payment Cryptography will use during key export.
* @see Tr34KeyBlockFormat
*/
public void setKeyBlockFormat(String keyBlockFormat) {
this.keyBlockFormat = keyBlockFormat;
}
/**
*
* The format of key block that Amazon Web Services Payment Cryptography will use during key export.
*
*
* @return The format of key block that Amazon Web Services Payment Cryptography will use during key export.
* @see Tr34KeyBlockFormat
*/
public String getKeyBlockFormat() {
return this.keyBlockFormat;
}
/**
*
* The format of key block that Amazon Web Services Payment Cryptography will use during key export.
*
*
* @param keyBlockFormat
* The format of key block that Amazon Web Services Payment Cryptography will use during key export.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Tr34KeyBlockFormat
*/
public ExportTr34KeyBlock withKeyBlockFormat(String keyBlockFormat) {
setKeyBlockFormat(keyBlockFormat);
return this;
}
/**
*
* The format of key block that Amazon Web Services Payment Cryptography will use during key export.
*
*
* @param keyBlockFormat
* The format of key block that Amazon Web Services Payment Cryptography will use during key export.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Tr34KeyBlockFormat
*/
public ExportTr34KeyBlock withKeyBlockFormat(Tr34KeyBlockFormat keyBlockFormat) {
this.keyBlockFormat = keyBlockFormat.toString();
return this;
}
/**
*
* A random number value that is unique to the TR-34 key block generated using 2 pass. The operation will fail, if a
* random nonce value is not provided for a TR-34 key block generated using 2 pass.
*
*
* @param randomNonce
* A random number value that is unique to the TR-34 key block generated using 2 pass. The operation will
* fail, if a random nonce value is not provided for a TR-34 key block generated using 2 pass.
*/
public void setRandomNonce(String randomNonce) {
this.randomNonce = randomNonce;
}
/**
*
* A random number value that is unique to the TR-34 key block generated using 2 pass. The operation will fail, if a
* random nonce value is not provided for a TR-34 key block generated using 2 pass.
*
*
* @return A random number value that is unique to the TR-34 key block generated using 2 pass. The operation will
* fail, if a random nonce value is not provided for a TR-34 key block generated using 2 pass.
*/
public String getRandomNonce() {
return this.randomNonce;
}
/**
*
* A random number value that is unique to the TR-34 key block generated using 2 pass. The operation will fail, if a
* random nonce value is not provided for a TR-34 key block generated using 2 pass.
*
*
* @param randomNonce
* A random number value that is unique to the TR-34 key block generated using 2 pass. The operation will
* fail, if a random nonce value is not provided for a TR-34 key block generated using 2 pass.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ExportTr34KeyBlock withRandomNonce(String randomNonce) {
setRandomNonce(randomNonce);
return this;
}
/**
*
* Optional metadata for export associated with the key material. This data is signed but transmitted in clear text.
*
*
* @param keyBlockHeaders
* Optional metadata for export associated with the key material. This data is signed but transmitted in
* clear text.
*/
public void setKeyBlockHeaders(KeyBlockHeaders keyBlockHeaders) {
this.keyBlockHeaders = keyBlockHeaders;
}
/**
*
* Optional metadata for export associated with the key material. This data is signed but transmitted in clear text.
*
*
* @return Optional metadata for export associated with the key material. This data is signed but transmitted in
* clear text.
*/
public KeyBlockHeaders getKeyBlockHeaders() {
return this.keyBlockHeaders;
}
/**
*
* Optional metadata for export associated with the key material. This data is signed but transmitted in clear text.
*
*
* @param keyBlockHeaders
* Optional metadata for export associated with the key material. This data is signed but transmitted in
* clear text.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ExportTr34KeyBlock withKeyBlockHeaders(KeyBlockHeaders keyBlockHeaders) {
setKeyBlockHeaders(keyBlockHeaders);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCertificateAuthorityPublicKeyIdentifier() != null)
sb.append("CertificateAuthorityPublicKeyIdentifier: ").append(getCertificateAuthorityPublicKeyIdentifier()).append(",");
if (getWrappingKeyCertificate() != null)
sb.append("WrappingKeyCertificate: ").append("***Sensitive Data Redacted***").append(",");
if (getExportToken() != null)
sb.append("ExportToken: ").append(getExportToken()).append(",");
if (getKeyBlockFormat() != null)
sb.append("KeyBlockFormat: ").append(getKeyBlockFormat()).append(",");
if (getRandomNonce() != null)
sb.append("RandomNonce: ").append(getRandomNonce()).append(",");
if (getKeyBlockHeaders() != null)
sb.append("KeyBlockHeaders: ").append(getKeyBlockHeaders());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ExportTr34KeyBlock == false)
return false;
ExportTr34KeyBlock other = (ExportTr34KeyBlock) obj;
if (other.getCertificateAuthorityPublicKeyIdentifier() == null ^ this.getCertificateAuthorityPublicKeyIdentifier() == null)
return false;
if (other.getCertificateAuthorityPublicKeyIdentifier() != null
&& other.getCertificateAuthorityPublicKeyIdentifier().equals(this.getCertificateAuthorityPublicKeyIdentifier()) == false)
return false;
if (other.getWrappingKeyCertificate() == null ^ this.getWrappingKeyCertificate() == null)
return false;
if (other.getWrappingKeyCertificate() != null && other.getWrappingKeyCertificate().equals(this.getWrappingKeyCertificate()) == false)
return false;
if (other.getExportToken() == null ^ this.getExportToken() == null)
return false;
if (other.getExportToken() != null && other.getExportToken().equals(this.getExportToken()) == false)
return false;
if (other.getKeyBlockFormat() == null ^ this.getKeyBlockFormat() == null)
return false;
if (other.getKeyBlockFormat() != null && other.getKeyBlockFormat().equals(this.getKeyBlockFormat()) == false)
return false;
if (other.getRandomNonce() == null ^ this.getRandomNonce() == null)
return false;
if (other.getRandomNonce() != null && other.getRandomNonce().equals(this.getRandomNonce()) == false)
return false;
if (other.getKeyBlockHeaders() == null ^ this.getKeyBlockHeaders() == null)
return false;
if (other.getKeyBlockHeaders() != null && other.getKeyBlockHeaders().equals(this.getKeyBlockHeaders()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCertificateAuthorityPublicKeyIdentifier() == null) ? 0 : getCertificateAuthorityPublicKeyIdentifier().hashCode());
hashCode = prime * hashCode + ((getWrappingKeyCertificate() == null) ? 0 : getWrappingKeyCertificate().hashCode());
hashCode = prime * hashCode + ((getExportToken() == null) ? 0 : getExportToken().hashCode());
hashCode = prime * hashCode + ((getKeyBlockFormat() == null) ? 0 : getKeyBlockFormat().hashCode());
hashCode = prime * hashCode + ((getRandomNonce() == null) ? 0 : getRandomNonce().hashCode());
hashCode = prime * hashCode + ((getKeyBlockHeaders() == null) ? 0 : getKeyBlockHeaders().hashCode());
return hashCode;
}
@Override
public ExportTr34KeyBlock clone() {
try {
return (ExportTr34KeyBlock) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.paymentcryptography.model.transform.ExportTr34KeyBlockMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}