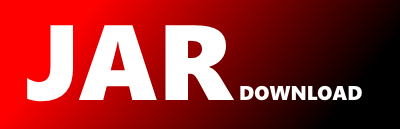
com.amazonaws.services.paymentcryptography.model.TrustedCertificatePublicKey Maven / Gradle / Ivy
Show all versions of aws-java-sdk-paymentcryptography Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.paymentcryptography.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Parameter information for trusted public key certificate import.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TrustedCertificatePublicKey implements Serializable, Cloneable, StructuredPojo {
/**
*
* The role of the key, the algorithm it supports, and the cryptographic operations allowed with the key. This data
* is immutable after a trusted public key is imported.
*
*/
private KeyAttributes keyAttributes;
/**
*
* Parameter information for trusted public key certificate import.
*
*/
private String publicKeyCertificate;
/**
*
* The KeyARN
of the root public key certificate or certificate chain that signs the trusted public key
* certificate import.
*
*/
private String certificateAuthorityPublicKeyIdentifier;
/**
*
* The role of the key, the algorithm it supports, and the cryptographic operations allowed with the key. This data
* is immutable after a trusted public key is imported.
*
*
* @param keyAttributes
* The role of the key, the algorithm it supports, and the cryptographic operations allowed with the key.
* This data is immutable after a trusted public key is imported.
*/
public void setKeyAttributes(KeyAttributes keyAttributes) {
this.keyAttributes = keyAttributes;
}
/**
*
* The role of the key, the algorithm it supports, and the cryptographic operations allowed with the key. This data
* is immutable after a trusted public key is imported.
*
*
* @return The role of the key, the algorithm it supports, and the cryptographic operations allowed with the key.
* This data is immutable after a trusted public key is imported.
*/
public KeyAttributes getKeyAttributes() {
return this.keyAttributes;
}
/**
*
* The role of the key, the algorithm it supports, and the cryptographic operations allowed with the key. This data
* is immutable after a trusted public key is imported.
*
*
* @param keyAttributes
* The role of the key, the algorithm it supports, and the cryptographic operations allowed with the key.
* This data is immutable after a trusted public key is imported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TrustedCertificatePublicKey withKeyAttributes(KeyAttributes keyAttributes) {
setKeyAttributes(keyAttributes);
return this;
}
/**
*
* Parameter information for trusted public key certificate import.
*
*
* @param publicKeyCertificate
* Parameter information for trusted public key certificate import.
*/
public void setPublicKeyCertificate(String publicKeyCertificate) {
this.publicKeyCertificate = publicKeyCertificate;
}
/**
*
* Parameter information for trusted public key certificate import.
*
*
* @return Parameter information for trusted public key certificate import.
*/
public String getPublicKeyCertificate() {
return this.publicKeyCertificate;
}
/**
*
* Parameter information for trusted public key certificate import.
*
*
* @param publicKeyCertificate
* Parameter information for trusted public key certificate import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TrustedCertificatePublicKey withPublicKeyCertificate(String publicKeyCertificate) {
setPublicKeyCertificate(publicKeyCertificate);
return this;
}
/**
*
* The KeyARN
of the root public key certificate or certificate chain that signs the trusted public key
* certificate import.
*
*
* @param certificateAuthorityPublicKeyIdentifier
* The KeyARN
of the root public key certificate or certificate chain that signs the trusted
* public key certificate import.
*/
public void setCertificateAuthorityPublicKeyIdentifier(String certificateAuthorityPublicKeyIdentifier) {
this.certificateAuthorityPublicKeyIdentifier = certificateAuthorityPublicKeyIdentifier;
}
/**
*
* The KeyARN
of the root public key certificate or certificate chain that signs the trusted public key
* certificate import.
*
*
* @return The KeyARN
of the root public key certificate or certificate chain that signs the trusted
* public key certificate import.
*/
public String getCertificateAuthorityPublicKeyIdentifier() {
return this.certificateAuthorityPublicKeyIdentifier;
}
/**
*
* The KeyARN
of the root public key certificate or certificate chain that signs the trusted public key
* certificate import.
*
*
* @param certificateAuthorityPublicKeyIdentifier
* The KeyARN
of the root public key certificate or certificate chain that signs the trusted
* public key certificate import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TrustedCertificatePublicKey withCertificateAuthorityPublicKeyIdentifier(String certificateAuthorityPublicKeyIdentifier) {
setCertificateAuthorityPublicKeyIdentifier(certificateAuthorityPublicKeyIdentifier);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getKeyAttributes() != null)
sb.append("KeyAttributes: ").append(getKeyAttributes()).append(",");
if (getPublicKeyCertificate() != null)
sb.append("PublicKeyCertificate: ").append("***Sensitive Data Redacted***").append(",");
if (getCertificateAuthorityPublicKeyIdentifier() != null)
sb.append("CertificateAuthorityPublicKeyIdentifier: ").append(getCertificateAuthorityPublicKeyIdentifier());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TrustedCertificatePublicKey == false)
return false;
TrustedCertificatePublicKey other = (TrustedCertificatePublicKey) obj;
if (other.getKeyAttributes() == null ^ this.getKeyAttributes() == null)
return false;
if (other.getKeyAttributes() != null && other.getKeyAttributes().equals(this.getKeyAttributes()) == false)
return false;
if (other.getPublicKeyCertificate() == null ^ this.getPublicKeyCertificate() == null)
return false;
if (other.getPublicKeyCertificate() != null && other.getPublicKeyCertificate().equals(this.getPublicKeyCertificate()) == false)
return false;
if (other.getCertificateAuthorityPublicKeyIdentifier() == null ^ this.getCertificateAuthorityPublicKeyIdentifier() == null)
return false;
if (other.getCertificateAuthorityPublicKeyIdentifier() != null
&& other.getCertificateAuthorityPublicKeyIdentifier().equals(this.getCertificateAuthorityPublicKeyIdentifier()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getKeyAttributes() == null) ? 0 : getKeyAttributes().hashCode());
hashCode = prime * hashCode + ((getPublicKeyCertificate() == null) ? 0 : getPublicKeyCertificate().hashCode());
hashCode = prime * hashCode + ((getCertificateAuthorityPublicKeyIdentifier() == null) ? 0 : getCertificateAuthorityPublicKeyIdentifier().hashCode());
return hashCode;
}
@Override
public TrustedCertificatePublicKey clone() {
try {
return (TrustedCertificatePublicKey) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.paymentcryptography.model.transform.TrustedCertificatePublicKeyMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}