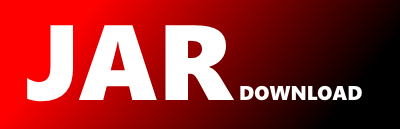
com.amazonaws.services.personalize.AmazonPersonalize Maven / Gradle / Ivy
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.personalize;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.personalize.model.*;
/**
* Interface for accessing Amazon Personalize.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.personalize.AbstractAmazonPersonalize} instead.
*
*
*
* Amazon Personalize is a machine learning service that makes it easy to add individualized recommendations to
* customers.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonPersonalize {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "personalize";
/**
*
* Creates a batch inference job. The operation can handle up to 50 million records and the input file must be in
* JSON format. For more information, see Creating a batch
* inference job.
*
*
* @param createBatchInferenceJobRequest
* @return Result of the CreateBatchInferenceJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateBatchInferenceJob
* @see AWS API Documentation
*/
CreateBatchInferenceJobResult createBatchInferenceJob(CreateBatchInferenceJobRequest createBatchInferenceJobRequest);
/**
*
* Creates a batch segment job. The operation can handle up to 50 million records and the input file must be in JSON
* format. For more information, see Getting batch recommendations
* and user segments.
*
*
* @param createBatchSegmentJobRequest
* @return Result of the CreateBatchSegmentJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateBatchSegmentJob
* @see AWS API Documentation
*/
CreateBatchSegmentJobResult createBatchSegmentJob(CreateBatchSegmentJobRequest createBatchSegmentJobRequest);
/**
*
* Creates a campaign that deploys a solution version. When a client calls the GetRecommendations
* and
* GetPersonalizedRanking APIs, a campaign is specified in the request.
*
*
* Minimum Provisioned TPS and Auto-Scaling
*
*
*
* A high minProvisionedTPS
will increase your bill. We recommend starting with 1 for
* minProvisionedTPS
(the default). Track your usage using Amazon CloudWatch metrics, and increase the
* minProvisionedTPS
as necessary.
*
*
*
* A transaction is a single GetRecommendations
or GetPersonalizedRanking
call.
* Transactions per second (TPS) is the throughput and unit of billing for Amazon Personalize. The minimum
* provisioned TPS (minProvisionedTPS
) specifies the baseline throughput provisioned by Amazon
* Personalize, and thus, the minimum billing charge.
*
*
* If your TPS increases beyond minProvisionedTPS
, Amazon Personalize auto-scales the provisioned
* capacity up and down, but never below minProvisionedTPS
. There's a short time delay while the
* capacity is increased that might cause loss of transactions.
*
*
* The actual TPS used is calculated as the average requests/second within a 5-minute window. You pay for maximum of
* either the minimum provisioned TPS or the actual TPS. We recommend starting with a low
* minProvisionedTPS
, track your usage using Amazon CloudWatch metrics, and then increase the
* minProvisionedTPS
as necessary.
*
*
* Status
*
*
* A campaign can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the campaign status, call DescribeCampaign.
*
*
*
* Wait until the status
of the campaign is ACTIVE
before asking the campaign for
* recommendations.
*
*
*
* Related APIs
*
*
* -
*
* ListCampaigns
*
*
* -
*
* DescribeCampaign
*
*
* -
*
* UpdateCampaign
*
*
* -
*
* DeleteCampaign
*
*
*
*
* @param createCampaignRequest
* @return Result of the CreateCampaign operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateCampaign
* @see AWS API
* Documentation
*/
CreateCampaignResult createCampaign(CreateCampaignRequest createCampaignRequest);
/**
*
* Creates an empty dataset and adds it to the specified dataset group. Use CreateDatasetImportJob to import your training data to a dataset.
*
*
* There are three types of datasets:
*
*
* -
*
* Interactions
*
*
* -
*
* Items
*
*
* -
*
* Users
*
*
*
*
* Each dataset type has an associated schema with required field types. Only the Interactions
dataset
* is required in order to train a model (also referred to as creating a solution).
*
*
* A dataset can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the status of the dataset, call DescribeDataset.
*
*
* Related APIs
*
*
* -
*
*
* -
*
* ListDatasets
*
*
* -
*
* DescribeDataset
*
*
* -
*
* DeleteDataset
*
*
*
*
* @param createDatasetRequest
* @return Result of the CreateDataset operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateDataset
* @see AWS API
* Documentation
*/
CreateDatasetResult createDataset(CreateDatasetRequest createDatasetRequest);
/**
*
* Creates a job that exports data from your dataset to an Amazon S3 bucket. To allow Amazon Personalize to export
* the training data, you must specify an service-linked IAM role that gives Amazon Personalize
* PutObject
permissions for your Amazon S3 bucket. For information, see Exporting a dataset in the Amazon
* Personalize developer guide.
*
*
* Status
*
*
* A dataset export job can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
*
*
* To get the status of the export job, call DescribeDatasetExportJob, and specify the Amazon Resource Name (ARN) of the dataset export job. The dataset
* export is complete when the status shows as ACTIVE. If the status shows as CREATE FAILED, the response includes a
* failureReason
key, which describes why the job failed.
*
*
* @param createDatasetExportJobRequest
* @return Result of the CreateDatasetExportJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateDatasetExportJob
* @see AWS API Documentation
*/
CreateDatasetExportJobResult createDatasetExportJob(CreateDatasetExportJobRequest createDatasetExportJobRequest);
/**
*
* Creates an empty dataset group. A dataset group is a container for Amazon Personalize resources. A dataset group
* can contain at most three datasets, one for each type of dataset:
*
*
* -
*
* Interactions
*
*
* -
*
* Items
*
*
* -
*
* Users
*
*
*
*
* A dataset group can be a Domain dataset group, where you specify a domain and use pre-configured resources like
* recommenders, or a Custom dataset group, where you use custom resources, such as a solution with a solution
* version, that you deploy with a campaign. If you start with a Domain dataset group, you can still add custom
* resources such as solutions and solution versions trained with recipes for custom use cases and deployed with
* campaigns.
*
*
* A dataset group can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING
*
*
*
*
* To get the status of the dataset group, call DescribeDatasetGroup.
* If the status shows as CREATE FAILED, the response includes a failureReason
key, which describes why
* the creation failed.
*
*
*
* You must wait until the status
of the dataset group is ACTIVE
before adding a dataset
* to the group.
*
*
*
* You can specify an Key Management Service (KMS) key to encrypt the datasets in the group. If you specify a KMS
* key, you must also include an Identity and Access Management (IAM) role that has permission to access the key.
*
*
* APIs that require a dataset group ARN in the request
*
*
* -
*
* CreateDataset
*
*
* -
*
*
* -
*
* CreateSolution
*
*
*
*
* Related APIs
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createDatasetGroupRequest
* @return Result of the CreateDatasetGroup operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateDatasetGroup
* @see AWS
* API Documentation
*/
CreateDatasetGroupResult createDatasetGroup(CreateDatasetGroupRequest createDatasetGroupRequest);
/**
*
* Creates a job that imports training data from your data source (an Amazon S3 bucket) to an Amazon Personalize
* dataset. To allow Amazon Personalize to ACTIVE -or- CREATE FAILED
*
*
*
* To get the status of the import job, call DescribeDatasetImportJob, providing the Amazon Resource Name (ARN) of the dataset import job. The dataset
* import is complete when the status shows as ACTIVE. If the status shows as CREATE FAILED, the response includes a
* failureReason
key, which describes why the job failed.
*
*
*
* Importing takes time. You must wait until the status shows as ACTIVE before training a model using the dataset.
*
*
*
* Related APIs
*
*
* -
*
*
* -
*
*
*
*
* @param createDatasetImportJobRequest
* @return Result of the CreateDatasetImportJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateDatasetImportJob
* @see AWS API Documentation
*/
CreateDatasetImportJobResult createDatasetImportJob(CreateDatasetImportJobRequest createDatasetImportJobRequest);
/**
*
* Creates an event tracker that you use when adding event data to a specified dataset group using the PutEvents API.
*
*
*
* Only one event tracker can be associated with a dataset group. You will get an error if you call
* CreateEventTracker
using the same dataset group as an existing event tracker.
*
*
*
* When you create an event tracker, the response includes a tracking ID, which you pass as a parameter when you use
* the PutEvents operation.
* Amazon Personalize then appends the event data to the Interactions dataset of the dataset group you specify in
* your event tracker.
*
*
* The event tracker can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the status of the event tracker, call DescribeEventTracker.
*
*
*
* The event tracker must be in the ACTIVE state before using the tracking ID.
*
*
*
* Related APIs
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createEventTrackerRequest
* @return Result of the CreateEventTracker operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateEventTracker
* @see AWS
* API Documentation
*/
CreateEventTrackerResult createEventTracker(CreateEventTrackerRequest createEventTrackerRequest);
/**
*
* Creates a recommendation filter. For more information, see Filtering recommendations and user
* segments.
*
*
* @param createFilterRequest
* @return Result of the CreateFilter operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateFilter
* @see AWS API
* Documentation
*/
CreateFilterResult createFilter(CreateFilterRequest createFilterRequest);
/**
*
* Creates a metric attribution. A metric attribution creates reports on the data that you import into Amazon
* Personalize. Depending on how you imported the data, you can view reports in Amazon CloudWatch or Amazon S3. For
* more information, see Measuring impact of
* recommendations.
*
*
* @param createMetricAttributionRequest
* @return Result of the CreateMetricAttribution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @sample AmazonPersonalize.CreateMetricAttribution
* @see AWS API Documentation
*/
CreateMetricAttributionResult createMetricAttribution(CreateMetricAttributionRequest createMetricAttributionRequest);
/**
*
* Creates a recommender with the recipe (a Domain dataset group use case) you specify. You create recommenders for
* a Domain dataset group and specify the recommender's Amazon Resource Name (ARN) when you make a GetRecommendations
* request.
*
*
* Minimum recommendation requests per second
*
*
*
* A high minRecommendationRequestsPerSecond
will increase your bill. We recommend starting with 1 for
* minRecommendationRequestsPerSecond
(the default). Track your usage using Amazon CloudWatch metrics,
* and increase the minRecommendationRequestsPerSecond
as necessary.
*
*
*
* When you create a recommender, you can configure the recommender's minimum recommendation requests per second.
* The minimum recommendation requests per second (minRecommendationRequestsPerSecond
) specifies the
* baseline recommendation request throughput provisioned by Amazon Personalize. The default
* minRecommendationRequestsPerSecond is 1
. A recommendation request is a single
* GetRecommendations
operation. Request throughput is measured in requests per second and Amazon
* Personalize uses your requests per second to derive your requests per hour and the price of your recommender
* usage.
*
*
* If your requests per second increases beyond minRecommendationRequestsPerSecond
, Amazon Personalize
* auto-scales the provisioned capacity up and down, but never below minRecommendationRequestsPerSecond
* . There's a short time delay while the capacity is increased that might cause loss of requests.
*
*
* Your bill is the greater of either the minimum requests per hour (based on minRecommendationRequestsPerSecond) or
* the actual number of requests. The actual request throughput used is calculated as the average requests/second
* within a one-hour window. We recommend starting with the default minRecommendationRequestsPerSecond
,
* track your usage using Amazon CloudWatch metrics, and then increase the
* minRecommendationRequestsPerSecond
as necessary.
*
*
* Status
*
*
* A recommender can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* STOP PENDING > STOP IN_PROGRESS > INACTIVE > START PENDING > START IN_PROGRESS > ACTIVE
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the recommender status, call DescribeRecommender.
*
*
*
* Wait until the status
of the recommender is ACTIVE
before asking the recommender for
* recommendations.
*
*
*
* Related APIs
*
*
* -
*
* ListRecommenders
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createRecommenderRequest
* @return Result of the CreateRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateRecommender
* @see AWS
* API Documentation
*/
CreateRecommenderResult createRecommender(CreateRecommenderRequest createRecommenderRequest);
/**
*
* Creates an Amazon Personalize schema from the specified schema string. The schema you create must be in Avro JSON
* format.
*
*
* Amazon Personalize recognizes three schema variants. Each schema is associated with a dataset type and has a set
* of required field and keywords. If you are creating a schema for a dataset in a Domain dataset group, you provide
* the domain of the Domain dataset group. You specify a schema when you call CreateDataset.
*
*
* Related APIs
*
*
* -
*
* ListSchemas
*
*
* -
*
* DescribeSchema
*
*
* -
*
* DeleteSchema
*
*
*
*
* @param createSchemaRequest
* @return Result of the CreateSchema operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @sample AmazonPersonalize.CreateSchema
* @see AWS API
* Documentation
*/
CreateSchemaResult createSchema(CreateSchemaRequest createSchemaRequest);
/**
*
* Creates the configuration for training a model. A trained model is known as a solution version. After the
* configuration is created, you train the model (create a solution version) by calling the CreateSolutionVersion
* operation. Every time you call CreateSolutionVersion
, a new version of the solution is created.
*
*
* After creating a solution version, you check its accuracy by calling GetSolutionMetrics. When
* you are satisfied with the version, you deploy it using CreateCampaign. The campaign
* provides recommendations to a client through the GetRecommendations
* API.
*
*
* To train a model, Amazon Personalize requires training data and a recipe. The training data comes from the
* dataset group that you provide in the request. A recipe specifies the training algorithm and a feature
* transformation. You can specify one of the predefined recipes provided by Amazon Personalize.
*
*
*
* Amazon Personalize doesn't support configuring the hpoObjective
for solution hyperparameter
* optimization at this time.
*
*
*
* Status
*
*
* A solution can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the status of the solution, call DescribeSolution. Wait
* until the status shows as ACTIVE before calling CreateSolutionVersion
.
*
*
* Related APIs
*
*
* -
*
* ListSolutions
*
*
* -
*
*
* -
*
* DescribeSolution
*
*
* -
*
* DeleteSolution
*
*
*
*
* -
*
*
* -
*
*
*
*
* @param createSolutionRequest
* @return Result of the CreateSolution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateSolution
* @see AWS API
* Documentation
*/
CreateSolutionResult createSolution(CreateSolutionRequest createSolutionRequest);
/**
*
* Trains or retrains an active solution in a Custom dataset group. A solution is created using the CreateSolution operation and
* must be in the ACTIVE state before calling CreateSolutionVersion
. A new version of the solution is
* created every time you call this operation.
*
*
* Status
*
*
* A solution version can be in one of the following states:
*
*
* -
*
* CREATE PENDING
*
*
* -
*
* CREATE IN_PROGRESS
*
*
* -
*
* ACTIVE
*
*
* -
*
* CREATE FAILED
*
*
* -
*
* CREATE STOPPING
*
*
* -
*
* CREATE STOPPED
*
*
*
*
* To get the status of the version, call DescribeSolutionVersion. Wait until the status shows as ACTIVE before calling CreateCampaign
.
*
*
* If the status shows as CREATE FAILED, the response includes a failureReason
key, which describes why
* the job failed.
*
*
* Related APIs
*
*
* -
*
*
* -
*
*
* -
*
* ListSolutions
*
*
* -
*
* CreateSolution
*
*
* -
*
* DescribeSolution
*
*
* -
*
* DeleteSolution
*
*
*
*
* @param createSolutionVersionRequest
* @return Result of the CreateSolutionVersion operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @sample AmazonPersonalize.CreateSolutionVersion
* @see AWS API Documentation
*/
CreateSolutionVersionResult createSolutionVersion(CreateSolutionVersionRequest createSolutionVersionRequest);
/**
*
* Removes a campaign by deleting the solution deployment. The solution that the campaign is based on is not deleted
* and can be redeployed when needed. A deleted campaign can no longer be specified in a GetRecommendations
* request. For information on creating campaigns, see CreateCampaign.
*
*
* @param deleteCampaignRequest
* @return Result of the DeleteCampaign operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteCampaign
* @see AWS API
* Documentation
*/
DeleteCampaignResult deleteCampaign(DeleteCampaignRequest deleteCampaignRequest);
/**
*
* Deletes a dataset. You can't delete a dataset if an associated DatasetImportJob
or
* SolutionVersion
is in the CREATE PENDING or IN PROGRESS state. For more information on datasets, see
* CreateDataset.
*
*
* @param deleteDatasetRequest
* @return Result of the DeleteDataset operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteDataset
* @see AWS API
* Documentation
*/
DeleteDatasetResult deleteDataset(DeleteDatasetRequest deleteDatasetRequest);
/**
*
* Deletes a dataset group. Before you delete a dataset group, you must delete the following:
*
*
* -
*
* All associated event trackers.
*
*
* -
*
* All associated solutions.
*
*
* -
*
* All datasets in the dataset group.
*
*
*
*
* @param deleteDatasetGroupRequest
* @return Result of the DeleteDatasetGroup operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteDatasetGroup
* @see AWS
* API Documentation
*/
DeleteDatasetGroupResult deleteDatasetGroup(DeleteDatasetGroupRequest deleteDatasetGroupRequest);
/**
*
* Deletes the event tracker. Does not delete the event-interactions dataset from the associated dataset group. For
* more information on event trackers, see CreateEventTracker.
*
*
* @param deleteEventTrackerRequest
* @return Result of the DeleteEventTracker operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteEventTracker
* @see AWS
* API Documentation
*/
DeleteEventTrackerResult deleteEventTracker(DeleteEventTrackerRequest deleteEventTrackerRequest);
/**
*
* Deletes a filter.
*
*
* @param deleteFilterRequest
* @return Result of the DeleteFilter operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteFilter
* @see AWS API
* Documentation
*/
DeleteFilterResult deleteFilter(DeleteFilterRequest deleteFilterRequest);
/**
*
* Deletes a metric attribution.
*
*
* @param deleteMetricAttributionRequest
* @return Result of the DeleteMetricAttribution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteMetricAttribution
* @see AWS API Documentation
*/
DeleteMetricAttributionResult deleteMetricAttribution(DeleteMetricAttributionRequest deleteMetricAttributionRequest);
/**
*
* Deactivates and removes a recommender. A deleted recommender can no longer be specified in a GetRecommendations
* request.
*
*
* @param deleteRecommenderRequest
* @return Result of the DeleteRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteRecommender
* @see AWS
* API Documentation
*/
DeleteRecommenderResult deleteRecommender(DeleteRecommenderRequest deleteRecommenderRequest);
/**
*
* Deletes a schema. Before deleting a schema, you must delete all datasets referencing the schema. For more
* information on schemas, see CreateSchema.
*
*
* @param deleteSchemaRequest
* @return Result of the DeleteSchema operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteSchema
* @see AWS API
* Documentation
*/
DeleteSchemaResult deleteSchema(DeleteSchemaRequest deleteSchemaRequest);
/**
*
* Deletes all versions of a solution and the Solution
object itself. Before deleting a solution, you
* must delete all campaigns based on the solution. To determine what campaigns are using the solution, call ListCampaigns and supply the
* Amazon Resource Name (ARN) of the solution. You can't delete a solution if an associated
* SolutionVersion
is in the CREATE PENDING or IN PROGRESS state. For more information on solutions,
* see CreateSolution.
*
*
* @param deleteSolutionRequest
* @return Result of the DeleteSolution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteSolution
* @see AWS API
* Documentation
*/
DeleteSolutionResult deleteSolution(DeleteSolutionRequest deleteSolutionRequest);
/**
*
* Describes the given algorithm.
*
*
* @param describeAlgorithmRequest
* @return Result of the DescribeAlgorithm operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeAlgorithm
* @see AWS
* API Documentation
*/
DescribeAlgorithmResult describeAlgorithm(DescribeAlgorithmRequest describeAlgorithmRequest);
/**
*
* Gets the properties of a batch inference job including name, Amazon Resource Name (ARN), status, input and output
* configurations, and the ARN of the solution version used to generate the recommendations.
*
*
* @param describeBatchInferenceJobRequest
* @return Result of the DescribeBatchInferenceJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeBatchInferenceJob
* @see AWS API Documentation
*/
DescribeBatchInferenceJobResult describeBatchInferenceJob(DescribeBatchInferenceJobRequest describeBatchInferenceJobRequest);
/**
*
* Gets the properties of a batch segment job including name, Amazon Resource Name (ARN), status, input and output
* configurations, and the ARN of the solution version used to generate segments.
*
*
* @param describeBatchSegmentJobRequest
* @return Result of the DescribeBatchSegmentJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeBatchSegmentJob
* @see AWS API Documentation
*/
DescribeBatchSegmentJobResult describeBatchSegmentJob(DescribeBatchSegmentJobRequest describeBatchSegmentJobRequest);
/**
*
* Describes the given campaign, including its status.
*
*
* A campaign can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* When the status
is CREATE FAILED
, the response includes the failureReason
* key, which describes why.
*
*
* For more information on campaigns, see CreateCampaign.
*
*
* @param describeCampaignRequest
* @return Result of the DescribeCampaign operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeCampaign
* @see AWS
* API Documentation
*/
DescribeCampaignResult describeCampaign(DescribeCampaignRequest describeCampaignRequest);
/**
*
* Describes the given dataset. For more information on datasets, see CreateDataset.
*
*
* @param describeDatasetRequest
* @return Result of the DescribeDataset operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeDataset
* @see AWS
* API Documentation
*/
DescribeDatasetResult describeDataset(DescribeDatasetRequest describeDatasetRequest);
/**
*
* Describes the dataset export job created by CreateDatasetExportJob, including the export job status.
*
*
* @param describeDatasetExportJobRequest
* @return Result of the DescribeDatasetExportJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeDatasetExportJob
* @see AWS API Documentation
*/
DescribeDatasetExportJobResult describeDatasetExportJob(DescribeDatasetExportJobRequest describeDatasetExportJobRequest);
/**
*
* Describes the given dataset group. For more information on dataset groups, see CreateDatasetGroup.
*
*
* @param describeDatasetGroupRequest
* @return Result of the DescribeDatasetGroup operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeDatasetGroup
* @see AWS API Documentation
*/
DescribeDatasetGroupResult describeDatasetGroup(DescribeDatasetGroupRequest describeDatasetGroupRequest);
/**
*
* Describes the dataset import job created by CreateDatasetImportJob, including the import job status.
*
*
* @param describeDatasetImportJobRequest
* @return Result of the DescribeDatasetImportJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeDatasetImportJob
* @see AWS API Documentation
*/
DescribeDatasetImportJobResult describeDatasetImportJob(DescribeDatasetImportJobRequest describeDatasetImportJobRequest);
/**
*
* Describes an event tracker. The response includes the trackingId
and status
of the
* event tracker. For more information on event trackers, see CreateEventTracker.
*
*
* @param describeEventTrackerRequest
* @return Result of the DescribeEventTracker operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeEventTracker
* @see AWS API Documentation
*/
DescribeEventTrackerResult describeEventTracker(DescribeEventTrackerRequest describeEventTrackerRequest);
/**
*
* Describes the given feature transformation.
*
*
* @param describeFeatureTransformationRequest
* @return Result of the DescribeFeatureTransformation operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeFeatureTransformation
* @see AWS API Documentation
*/
DescribeFeatureTransformationResult describeFeatureTransformation(DescribeFeatureTransformationRequest describeFeatureTransformationRequest);
/**
*
* Describes a filter's properties.
*
*
* @param describeFilterRequest
* @return Result of the DescribeFilter operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeFilter
* @see AWS API
* Documentation
*/
DescribeFilterResult describeFilter(DescribeFilterRequest describeFilterRequest);
/**
*
* Describes a metric attribution.
*
*
* @param describeMetricAttributionRequest
* @return Result of the DescribeMetricAttribution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeMetricAttribution
* @see AWS API Documentation
*/
DescribeMetricAttributionResult describeMetricAttribution(DescribeMetricAttributionRequest describeMetricAttributionRequest);
/**
*
* Describes a recipe.
*
*
* A recipe contains three items:
*
*
* -
*
* An algorithm that trains a model.
*
*
* -
*
* Hyperparameters that govern the training.
*
*
* -
*
* Feature transformation information for modifying the input data before training.
*
*
*
*
* Amazon Personalize provides a set of predefined recipes. You specify a recipe when you create a solution with the
* CreateSolution API.
* CreateSolution
trains a model by using the algorithm in the specified recipe and a training dataset.
* The solution, when deployed as a campaign, can provide recommendations using the GetRecommendations
* API.
*
*
* @param describeRecipeRequest
* @return Result of the DescribeRecipe operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeRecipe
* @see AWS API
* Documentation
*/
DescribeRecipeResult describeRecipe(DescribeRecipeRequest describeRecipeRequest);
/**
*
* Describes the given recommender, including its status.
*
*
* A recommender can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* STOP PENDING > STOP IN_PROGRESS > INACTIVE > START PENDING > START IN_PROGRESS > ACTIVE
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* When the status
is CREATE FAILED
, the response includes the failureReason
* key, which describes why.
*
*
* The modelMetrics
key is null when the recommender is being created or deleted.
*
*
* For more information on recommenders, see CreateRecommender.
*
*
* @param describeRecommenderRequest
* @return Result of the DescribeRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeRecommender
* @see AWS API Documentation
*/
DescribeRecommenderResult describeRecommender(DescribeRecommenderRequest describeRecommenderRequest);
/**
*
* Describes a schema. For more information on schemas, see CreateSchema.
*
*
* @param describeSchemaRequest
* @return Result of the DescribeSchema operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeSchema
* @see AWS API
* Documentation
*/
DescribeSchemaResult describeSchema(DescribeSchemaRequest describeSchemaRequest);
/**
*
* Describes a solution. For more information on solutions, see CreateSolution.
*
*
* @param describeSolutionRequest
* @return Result of the DescribeSolution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeSolution
* @see AWS
* API Documentation
*/
DescribeSolutionResult describeSolution(DescribeSolutionRequest describeSolutionRequest);
/**
*
* Describes a specific version of a solution. For more information on solutions, see CreateSolution
*
*
* @param describeSolutionVersionRequest
* @return Result of the DescribeSolutionVersion operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeSolutionVersion
* @see AWS API Documentation
*/
DescribeSolutionVersionResult describeSolutionVersion(DescribeSolutionVersionRequest describeSolutionVersionRequest);
/**
*
* Gets the metrics for the specified solution version.
*
*
* @param getSolutionMetricsRequest
* @return Result of the GetSolutionMetrics operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.GetSolutionMetrics
* @see AWS
* API Documentation
*/
GetSolutionMetricsResult getSolutionMetrics(GetSolutionMetricsRequest getSolutionMetricsRequest);
/**
*
* Gets a list of the batch inference jobs that have been performed off of a solution version.
*
*
* @param listBatchInferenceJobsRequest
* @return Result of the ListBatchInferenceJobs operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListBatchInferenceJobs
* @see AWS API Documentation
*/
ListBatchInferenceJobsResult listBatchInferenceJobs(ListBatchInferenceJobsRequest listBatchInferenceJobsRequest);
/**
*
* Gets a list of the batch segment jobs that have been performed off of a solution version that you specify.
*
*
* @param listBatchSegmentJobsRequest
* @return Result of the ListBatchSegmentJobs operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListBatchSegmentJobs
* @see AWS API Documentation
*/
ListBatchSegmentJobsResult listBatchSegmentJobs(ListBatchSegmentJobsRequest listBatchSegmentJobsRequest);
/**
*
* Returns a list of campaigns that use the given solution. When a solution is not specified, all the campaigns
* associated with the account are listed. The response provides the properties for each campaign, including the
* Amazon Resource Name (ARN). For more information on campaigns, see CreateCampaign.
*
*
* @param listCampaignsRequest
* @return Result of the ListCampaigns operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListCampaigns
* @see AWS API
* Documentation
*/
ListCampaignsResult listCampaigns(ListCampaignsRequest listCampaignsRequest);
/**
*
* Returns a list of dataset export jobs that use the given dataset. When a dataset is not specified, all the
* dataset export jobs associated with the account are listed. The response provides the properties for each dataset
* export job, including the Amazon Resource Name (ARN). For more information on dataset export jobs, see CreateDatasetExportJob. For more information on datasets, see CreateDataset.
*
*
* @param listDatasetExportJobsRequest
* @return Result of the ListDatasetExportJobs operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListDatasetExportJobs
* @see AWS API Documentation
*/
ListDatasetExportJobsResult listDatasetExportJobs(ListDatasetExportJobsRequest listDatasetExportJobsRequest);
/**
*
* Returns a list of dataset groups. The response provides the properties for each dataset group, including the
* Amazon Resource Name (ARN). For more information on dataset groups, see CreateDatasetGroup.
*
*
* @param listDatasetGroupsRequest
* @return Result of the ListDatasetGroups operation returned by the service.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListDatasetGroups
* @see AWS
* API Documentation
*/
ListDatasetGroupsResult listDatasetGroups(ListDatasetGroupsRequest listDatasetGroupsRequest);
/**
*
* Returns a list of dataset import jobs that use the given dataset. When a dataset is not specified, all the
* dataset import jobs associated with the account are listed. The response provides the properties for each dataset
* import job, including the Amazon Resource Name (ARN). For more information on dataset import jobs, see CreateDatasetImportJob. For more information on datasets, see CreateDataset.
*
*
* @param listDatasetImportJobsRequest
* @return Result of the ListDatasetImportJobs operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListDatasetImportJobs
* @see AWS API Documentation
*/
ListDatasetImportJobsResult listDatasetImportJobs(ListDatasetImportJobsRequest listDatasetImportJobsRequest);
/**
*
* Returns the list of datasets contained in the given dataset group. The response provides the properties for each
* dataset, including the Amazon Resource Name (ARN). For more information on datasets, see CreateDataset.
*
*
* @param listDatasetsRequest
* @return Result of the ListDatasets operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListDatasets
* @see AWS API
* Documentation
*/
ListDatasetsResult listDatasets(ListDatasetsRequest listDatasetsRequest);
/**
*
* Returns the list of event trackers associated with the account. The response provides the properties for each
* event tracker, including the Amazon Resource Name (ARN) and tracking ID. For more information on event trackers,
* see CreateEventTracker.
*
*
* @param listEventTrackersRequest
* @return Result of the ListEventTrackers operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListEventTrackers
* @see AWS
* API Documentation
*/
ListEventTrackersResult listEventTrackers(ListEventTrackersRequest listEventTrackersRequest);
/**
*
* Lists all filters that belong to a given dataset group.
*
*
* @param listFiltersRequest
* @return Result of the ListFilters operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListFilters
* @see AWS API
* Documentation
*/
ListFiltersResult listFilters(ListFiltersRequest listFiltersRequest);
/**
*
* Lists the metrics for the metric attribution.
*
*
* @param listMetricAttributionMetricsRequest
* @return Result of the ListMetricAttributionMetrics operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListMetricAttributionMetrics
* @see AWS API Documentation
*/
ListMetricAttributionMetricsResult listMetricAttributionMetrics(ListMetricAttributionMetricsRequest listMetricAttributionMetricsRequest);
/**
*
* Lists metric attributions.
*
*
* @param listMetricAttributionsRequest
* @return Result of the ListMetricAttributions operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListMetricAttributions
* @see AWS API Documentation
*/
ListMetricAttributionsResult listMetricAttributions(ListMetricAttributionsRequest listMetricAttributionsRequest);
/**
*
* Returns a list of available recipes. The response provides the properties for each recipe, including the recipe's
* Amazon Resource Name (ARN).
*
*
* @param listRecipesRequest
* @return Result of the ListRecipes operation returned by the service.
* @throws InvalidNextTokenException
* The token is not valid.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @sample AmazonPersonalize.ListRecipes
* @see AWS API
* Documentation
*/
ListRecipesResult listRecipes(ListRecipesRequest listRecipesRequest);
/**
*
* Returns a list of recommenders in a given Domain dataset group. When a Domain dataset group is not specified, all
* the recommenders associated with the account are listed. The response provides the properties for each
* recommender, including the Amazon Resource Name (ARN). For more information on recommenders, see CreateRecommender.
*
*
* @param listRecommendersRequest
* @return Result of the ListRecommenders operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListRecommenders
* @see AWS
* API Documentation
*/
ListRecommendersResult listRecommenders(ListRecommendersRequest listRecommendersRequest);
/**
*
* Returns the list of schemas associated with the account. The response provides the properties for each schema,
* including the Amazon Resource Name (ARN). For more information on schemas, see CreateSchema.
*
*
* @param listSchemasRequest
* @return Result of the ListSchemas operation returned by the service.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListSchemas
* @see AWS API
* Documentation
*/
ListSchemasResult listSchemas(ListSchemasRequest listSchemasRequest);
/**
*
* Returns a list of solution versions for the given solution. When a solution is not specified, all the solution
* versions associated with the account are listed. The response provides the properties for each solution version,
* including the Amazon Resource Name (ARN).
*
*
* @param listSolutionVersionsRequest
* @return Result of the ListSolutionVersions operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListSolutionVersions
* @see AWS API Documentation
*/
ListSolutionVersionsResult listSolutionVersions(ListSolutionVersionsRequest listSolutionVersionsRequest);
/**
*
* Returns a list of solutions that use the given dataset group. When a dataset group is not specified, all the
* solutions associated with the account are listed. The response provides the properties for each solution,
* including the Amazon Resource Name (ARN). For more information on solutions, see CreateSolution.
*
*
* @param listSolutionsRequest
* @return Result of the ListSolutions operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListSolutions
* @see AWS API
* Documentation
*/
ListSolutionsResult listSolutions(ListSolutionsRequest listSolutionsRequest);
/**
*
* Get a list of tags
* attached to a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Starts a recommender that is INACTIVE. Starting a recommender does not create any new models, but resumes billing
* and automatic retraining for the recommender.
*
*
* @param startRecommenderRequest
* @return Result of the StartRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.StartRecommender
* @see AWS
* API Documentation
*/
StartRecommenderResult startRecommender(StartRecommenderRequest startRecommenderRequest);
/**
*
* Stops a recommender that is ACTIVE. Stopping a recommender halts billing and automatic retraining for the
* recommender.
*
*
* @param stopRecommenderRequest
* @return Result of the StopRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.StopRecommender
* @see AWS
* API Documentation
*/
StopRecommenderResult stopRecommender(StopRecommenderRequest stopRecommenderRequest);
/**
*
* Stops creating a solution version that is in a state of CREATE_PENDING or CREATE IN_PROGRESS.
*
*
* Depending on the current state of the solution version, the solution version state changes as follows:
*
*
* -
*
* CREATE_PENDING > CREATE_STOPPED
*
*
* or
*
*
* -
*
* CREATE_IN_PROGRESS > CREATE_STOPPING > CREATE_STOPPED
*
*
*
*
* You are billed for all of the training completed up until you stop the solution version creation. You cannot
* resume creating a solution version once it has been stopped.
*
*
* @param stopSolutionVersionCreationRequest
* @return Result of the StopSolutionVersionCreation operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.StopSolutionVersionCreation
* @see AWS API Documentation
*/
StopSolutionVersionCreationResult stopSolutionVersionCreation(StopSolutionVersionCreationRequest stopSolutionVersionCreationRequest);
/**
*
* Add a list of tags to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @sample AmazonPersonalize.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Remove tags that are
* attached to a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws TooManyTagKeysException
* The request contains more tag keys than can be associated with a resource (50 tag keys per resource).
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a campaign by either deploying a new solution or changing the value of the campaign's
* minProvisionedTPS
parameter.
*
*
* To update a campaign, the campaign status must be ACTIVE or CREATE FAILED. Check the campaign status using the DescribeCampaign
* operation.
*
*
*
* You can still get recommendations from a campaign while an update is in progress. The campaign will use the
* previous solution version and campaign configuration to generate recommendations until the latest campaign update
* status is Active
.
*
*
*
* For more information on campaigns, see CreateCampaign.
*
*
* @param updateCampaignRequest
* @return Result of the UpdateCampaign operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.UpdateCampaign
* @see AWS API
* Documentation
*/
UpdateCampaignResult updateCampaign(UpdateCampaignRequest updateCampaignRequest);
/**
*
* Updates a metric attribution.
*
*
* @param updateMetricAttributionRequest
* @return Result of the UpdateMetricAttribution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @sample AmazonPersonalize.UpdateMetricAttribution
* @see AWS API Documentation
*/
UpdateMetricAttributionResult updateMetricAttribution(UpdateMetricAttributionRequest updateMetricAttributionRequest);
/**
*
* Updates the recommender to modify the recommender configuration. If you update the recommender to modify the
* columns used in training, Amazon Personalize automatically starts a full retraining of the models backing your
* recommender. While the update completes, you can still get recommendations from the recommender. The recommender
* uses the previous configuration until the update completes. To track the status of this update, use the
* latestRecommenderUpdate
returned in the DescribeRecommender
* operation.
*
*
* @param updateRecommenderRequest
* @return Result of the UpdateRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.UpdateRecommender
* @see AWS
* API Documentation
*/
UpdateRecommenderResult updateRecommender(UpdateRecommenderRequest updateRecommenderRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}