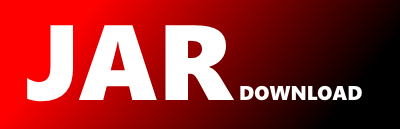
com.amazonaws.services.personalize.model.CreateSolutionRequest Maven / Gradle / Ivy
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.personalize.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateSolutionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name for the solution.
*
*/
private String name;
/**
*
* Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is
* false
.
*
*
* When performing AutoML, this parameter is always true
and you should not set it to
* false
.
*
*/
private Boolean performHPO;
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* Whether to perform automated machine learning (AutoML). The default is false
. For this case, you
* must specify recipeArn
.
*
*
* When set to true
, Amazon Personalize analyzes your training data and selects the optimal
* USER_PERSONALIZATION recipe and hyperparameters. In this case, you must omit recipeArn
. Amazon
* Personalize determines the optimal recipe by running tests with different values for the hyperparameters. AutoML
* lengthens the training process as compared to selecting a specific recipe.
*
*/
private Boolean performAutoML;
/**
*
* The ARN of the recipe to use for model training. Only specified when performAutoML
is false.
*
*/
private String recipeArn;
/**
*
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*
*/
private String datasetGroupArn;
/**
*
* When your have multiple event types (using an EVENT_TYPE
schema field), this parameter specifies
* which event type (for example, 'click' or 'like') is used for training the model.
*
*
* If you do not provide an eventType
, Amazon Personalize will use all interactions for training with
* equal weight regardless of type.
*
*/
private String eventType;
/**
*
* The configuration to use with the solution. When performAutoML
is set to true, Amazon Personalize
* only evaluates the autoMLConfig
section of the solution configuration.
*
*
*
* Amazon Personalize doesn't support configuring the hpoObjective
at this time.
*
*
*/
private SolutionConfig solutionConfig;
/**
*
* A list of tags to apply to
* the solution.
*
*/
private java.util.List tags;
/**
*
* The name for the solution.
*
*
* @param name
* The name for the solution.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name for the solution.
*
*
* @return The name for the solution.
*/
public String getName() {
return this.name;
}
/**
*
* The name for the solution.
*
*
* @param name
* The name for the solution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSolutionRequest withName(String name) {
setName(name);
return this;
}
/**
*
* Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is
* false
.
*
*
* When performing AutoML, this parameter is always true
and you should not set it to
* false
.
*
*
* @param performHPO
* Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is
* false
.
*
* When performing AutoML, this parameter is always true
and you should not set it to
* false
.
*/
public void setPerformHPO(Boolean performHPO) {
this.performHPO = performHPO;
}
/**
*
* Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is
* false
.
*
*
* When performing AutoML, this parameter is always true
and you should not set it to
* false
.
*
*
* @return Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is
* false
.
*
* When performing AutoML, this parameter is always true
and you should not set it to
* false
.
*/
public Boolean getPerformHPO() {
return this.performHPO;
}
/**
*
* Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is
* false
.
*
*
* When performing AutoML, this parameter is always true
and you should not set it to
* false
.
*
*
* @param performHPO
* Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is
* false
.
*
* When performing AutoML, this parameter is always true
and you should not set it to
* false
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSolutionRequest withPerformHPO(Boolean performHPO) {
setPerformHPO(performHPO);
return this;
}
/**
*
* Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is
* false
.
*
*
* When performing AutoML, this parameter is always true
and you should not set it to
* false
.
*
*
* @return Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is
* false
.
*
* When performing AutoML, this parameter is always true
and you should not set it to
* false
.
*/
public Boolean isPerformHPO() {
return this.performHPO;
}
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* Whether to perform automated machine learning (AutoML). The default is false
. For this case, you
* must specify recipeArn
.
*
*
* When set to true
, Amazon Personalize analyzes your training data and selects the optimal
* USER_PERSONALIZATION recipe and hyperparameters. In this case, you must omit recipeArn
. Amazon
* Personalize determines the optimal recipe by running tests with different values for the hyperparameters. AutoML
* lengthens the training process as compared to selecting a specific recipe.
*
*
* @param performAutoML
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available
* Amazon Personalize recipes. For more information, see Determining your use
* case.
*
*
*
* Whether to perform automated machine learning (AutoML). The default is false
. For this case,
* you must specify recipeArn
.
*
*
* When set to true
, Amazon Personalize analyzes your training data and selects the optimal
* USER_PERSONALIZATION recipe and hyperparameters. In this case, you must omit recipeArn
.
* Amazon Personalize determines the optimal recipe by running tests with different values for the
* hyperparameters. AutoML lengthens the training process as compared to selecting a specific recipe.
*/
public void setPerformAutoML(Boolean performAutoML) {
this.performAutoML = performAutoML;
}
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* Whether to perform automated machine learning (AutoML). The default is false
. For this case, you
* must specify recipeArn
.
*
*
* When set to true
, Amazon Personalize analyzes your training data and selects the optimal
* USER_PERSONALIZATION recipe and hyperparameters. In this case, you must omit recipeArn
. Amazon
* Personalize determines the optimal recipe by running tests with different values for the hyperparameters. AutoML
* lengthens the training process as compared to selecting a specific recipe.
*
*
* @return
* We don't recommend enabling automated machine learning. Instead, match your use case to the available
* Amazon Personalize recipes. For more information, see Determining your use
* case.
*
*
*
* Whether to perform automated machine learning (AutoML). The default is false
. For this case,
* you must specify recipeArn
.
*
*
* When set to true
, Amazon Personalize analyzes your training data and selects the optimal
* USER_PERSONALIZATION recipe and hyperparameters. In this case, you must omit recipeArn
.
* Amazon Personalize determines the optimal recipe by running tests with different values for the
* hyperparameters. AutoML lengthens the training process as compared to selecting a specific recipe.
*/
public Boolean getPerformAutoML() {
return this.performAutoML;
}
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* Whether to perform automated machine learning (AutoML). The default is false
. For this case, you
* must specify recipeArn
.
*
*
* When set to true
, Amazon Personalize analyzes your training data and selects the optimal
* USER_PERSONALIZATION recipe and hyperparameters. In this case, you must omit recipeArn
. Amazon
* Personalize determines the optimal recipe by running tests with different values for the hyperparameters. AutoML
* lengthens the training process as compared to selecting a specific recipe.
*
*
* @param performAutoML
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available
* Amazon Personalize recipes. For more information, see Determining your use
* case.
*
*
*
* Whether to perform automated machine learning (AutoML). The default is false
. For this case,
* you must specify recipeArn
.
*
*
* When set to true
, Amazon Personalize analyzes your training data and selects the optimal
* USER_PERSONALIZATION recipe and hyperparameters. In this case, you must omit recipeArn
.
* Amazon Personalize determines the optimal recipe by running tests with different values for the
* hyperparameters. AutoML lengthens the training process as compared to selecting a specific recipe.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSolutionRequest withPerformAutoML(Boolean performAutoML) {
setPerformAutoML(performAutoML);
return this;
}
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* Whether to perform automated machine learning (AutoML). The default is false
. For this case, you
* must specify recipeArn
.
*
*
* When set to true
, Amazon Personalize analyzes your training data and selects the optimal
* USER_PERSONALIZATION recipe and hyperparameters. In this case, you must omit recipeArn
. Amazon
* Personalize determines the optimal recipe by running tests with different values for the hyperparameters. AutoML
* lengthens the training process as compared to selecting a specific recipe.
*
*
* @return
* We don't recommend enabling automated machine learning. Instead, match your use case to the available
* Amazon Personalize recipes. For more information, see Determining your use
* case.
*
*
*
* Whether to perform automated machine learning (AutoML). The default is false
. For this case,
* you must specify recipeArn
.
*
*
* When set to true
, Amazon Personalize analyzes your training data and selects the optimal
* USER_PERSONALIZATION recipe and hyperparameters. In this case, you must omit recipeArn
.
* Amazon Personalize determines the optimal recipe by running tests with different values for the
* hyperparameters. AutoML lengthens the training process as compared to selecting a specific recipe.
*/
public Boolean isPerformAutoML() {
return this.performAutoML;
}
/**
*
* The ARN of the recipe to use for model training. Only specified when performAutoML
is false.
*
*
* @param recipeArn
* The ARN of the recipe to use for model training. Only specified when performAutoML
is false.
*/
public void setRecipeArn(String recipeArn) {
this.recipeArn = recipeArn;
}
/**
*
* The ARN of the recipe to use for model training. Only specified when performAutoML
is false.
*
*
* @return The ARN of the recipe to use for model training. Only specified when performAutoML
is false.
*/
public String getRecipeArn() {
return this.recipeArn;
}
/**
*
* The ARN of the recipe to use for model training. Only specified when performAutoML
is false.
*
*
* @param recipeArn
* The ARN of the recipe to use for model training. Only specified when performAutoML
is false.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSolutionRequest withRecipeArn(String recipeArn) {
setRecipeArn(recipeArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*
*
* @param datasetGroupArn
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*/
public void setDatasetGroupArn(String datasetGroupArn) {
this.datasetGroupArn = datasetGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*
*
* @return The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*/
public String getDatasetGroupArn() {
return this.datasetGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*
*
* @param datasetGroupArn
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSolutionRequest withDatasetGroupArn(String datasetGroupArn) {
setDatasetGroupArn(datasetGroupArn);
return this;
}
/**
*
* When your have multiple event types (using an EVENT_TYPE
schema field), this parameter specifies
* which event type (for example, 'click' or 'like') is used for training the model.
*
*
* If you do not provide an eventType
, Amazon Personalize will use all interactions for training with
* equal weight regardless of type.
*
*
* @param eventType
* When your have multiple event types (using an EVENT_TYPE
schema field), this parameter
* specifies which event type (for example, 'click' or 'like') is used for training the model.
*
* If you do not provide an eventType
, Amazon Personalize will use all interactions for training
* with equal weight regardless of type.
*/
public void setEventType(String eventType) {
this.eventType = eventType;
}
/**
*
* When your have multiple event types (using an EVENT_TYPE
schema field), this parameter specifies
* which event type (for example, 'click' or 'like') is used for training the model.
*
*
* If you do not provide an eventType
, Amazon Personalize will use all interactions for training with
* equal weight regardless of type.
*
*
* @return When your have multiple event types (using an EVENT_TYPE
schema field), this parameter
* specifies which event type (for example, 'click' or 'like') is used for training the model.
*
* If you do not provide an eventType
, Amazon Personalize will use all interactions for
* training with equal weight regardless of type.
*/
public String getEventType() {
return this.eventType;
}
/**
*
* When your have multiple event types (using an EVENT_TYPE
schema field), this parameter specifies
* which event type (for example, 'click' or 'like') is used for training the model.
*
*
* If you do not provide an eventType
, Amazon Personalize will use all interactions for training with
* equal weight regardless of type.
*
*
* @param eventType
* When your have multiple event types (using an EVENT_TYPE
schema field), this parameter
* specifies which event type (for example, 'click' or 'like') is used for training the model.
*
* If you do not provide an eventType
, Amazon Personalize will use all interactions for training
* with equal weight regardless of type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSolutionRequest withEventType(String eventType) {
setEventType(eventType);
return this;
}
/**
*
* The configuration to use with the solution. When performAutoML
is set to true, Amazon Personalize
* only evaluates the autoMLConfig
section of the solution configuration.
*
*
*
* Amazon Personalize doesn't support configuring the hpoObjective
at this time.
*
*
*
* @param solutionConfig
* The configuration to use with the solution. When performAutoML
is set to true, Amazon
* Personalize only evaluates the autoMLConfig
section of the solution configuration.
*
* Amazon Personalize doesn't support configuring the hpoObjective
at this time.
*
*/
public void setSolutionConfig(SolutionConfig solutionConfig) {
this.solutionConfig = solutionConfig;
}
/**
*
* The configuration to use with the solution. When performAutoML
is set to true, Amazon Personalize
* only evaluates the autoMLConfig
section of the solution configuration.
*
*
*
* Amazon Personalize doesn't support configuring the hpoObjective
at this time.
*
*
*
* @return The configuration to use with the solution. When performAutoML
is set to true, Amazon
* Personalize only evaluates the autoMLConfig
section of the solution configuration.
*
*
* Amazon Personalize doesn't support configuring the hpoObjective
at this time.
*
*/
public SolutionConfig getSolutionConfig() {
return this.solutionConfig;
}
/**
*
* The configuration to use with the solution. When performAutoML
is set to true, Amazon Personalize
* only evaluates the autoMLConfig
section of the solution configuration.
*
*
*
* Amazon Personalize doesn't support configuring the hpoObjective
at this time.
*
*
*
* @param solutionConfig
* The configuration to use with the solution. When performAutoML
is set to true, Amazon
* Personalize only evaluates the autoMLConfig
section of the solution configuration.
*
* Amazon Personalize doesn't support configuring the hpoObjective
at this time.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSolutionRequest withSolutionConfig(SolutionConfig solutionConfig) {
setSolutionConfig(solutionConfig);
return this;
}
/**
*
* A list of tags to apply to
* the solution.
*
*
* @return A list of tags to
* apply to the solution.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of tags to apply to
* the solution.
*
*
* @param tags
* A list of tags to
* apply to the solution.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of tags to apply to
* the solution.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags to
* apply to the solution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSolutionRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags to apply to
* the solution.
*
*
* @param tags
* A list of tags to
* apply to the solution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSolutionRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getPerformHPO() != null)
sb.append("PerformHPO: ").append(getPerformHPO()).append(",");
if (getPerformAutoML() != null)
sb.append("PerformAutoML: ").append(getPerformAutoML()).append(",");
if (getRecipeArn() != null)
sb.append("RecipeArn: ").append(getRecipeArn()).append(",");
if (getDatasetGroupArn() != null)
sb.append("DatasetGroupArn: ").append(getDatasetGroupArn()).append(",");
if (getEventType() != null)
sb.append("EventType: ").append(getEventType()).append(",");
if (getSolutionConfig() != null)
sb.append("SolutionConfig: ").append(getSolutionConfig()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateSolutionRequest == false)
return false;
CreateSolutionRequest other = (CreateSolutionRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getPerformHPO() == null ^ this.getPerformHPO() == null)
return false;
if (other.getPerformHPO() != null && other.getPerformHPO().equals(this.getPerformHPO()) == false)
return false;
if (other.getPerformAutoML() == null ^ this.getPerformAutoML() == null)
return false;
if (other.getPerformAutoML() != null && other.getPerformAutoML().equals(this.getPerformAutoML()) == false)
return false;
if (other.getRecipeArn() == null ^ this.getRecipeArn() == null)
return false;
if (other.getRecipeArn() != null && other.getRecipeArn().equals(this.getRecipeArn()) == false)
return false;
if (other.getDatasetGroupArn() == null ^ this.getDatasetGroupArn() == null)
return false;
if (other.getDatasetGroupArn() != null && other.getDatasetGroupArn().equals(this.getDatasetGroupArn()) == false)
return false;
if (other.getEventType() == null ^ this.getEventType() == null)
return false;
if (other.getEventType() != null && other.getEventType().equals(this.getEventType()) == false)
return false;
if (other.getSolutionConfig() == null ^ this.getSolutionConfig() == null)
return false;
if (other.getSolutionConfig() != null && other.getSolutionConfig().equals(this.getSolutionConfig()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getPerformHPO() == null) ? 0 : getPerformHPO().hashCode());
hashCode = prime * hashCode + ((getPerformAutoML() == null) ? 0 : getPerformAutoML().hashCode());
hashCode = prime * hashCode + ((getRecipeArn() == null) ? 0 : getRecipeArn().hashCode());
hashCode = prime * hashCode + ((getDatasetGroupArn() == null) ? 0 : getDatasetGroupArn().hashCode());
hashCode = prime * hashCode + ((getEventType() == null) ? 0 : getEventType().hashCode());
hashCode = prime * hashCode + ((getSolutionConfig() == null) ? 0 : getSolutionConfig().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateSolutionRequest clone() {
return (CreateSolutionRequest) super.clone();
}
}