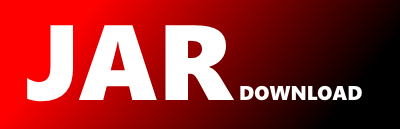
com.amazonaws.services.personalize.model.SolutionConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-personalize Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.personalize.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the configuration properties for the solution.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SolutionConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* Only events with a value greater than or equal to this threshold are used for training a model.
*
*/
private String eventValueThreshold;
/**
*
* Describes the properties for hyperparameter optimization (HPO).
*
*/
private HPOConfig hpoConfig;
/**
*
* Lists the algorithm hyperparameters and their values.
*
*/
private java.util.Map algorithmHyperParameters;
/**
*
* Lists the feature transformation parameters.
*
*/
private java.util.Map featureTransformationParameters;
/**
*
* The AutoMLConfig object
* containing a list of recipes to search when AutoML is performed.
*
*/
private AutoMLConfig autoMLConfig;
/**
*
* Describes the additional objective for the solution, such as maximizing streaming minutes or increasing revenue.
* For more information see Optimizing a
* solution.
*
*/
private OptimizationObjective optimizationObjective;
/**
*
* Specifies the training data configuration to use when creating a custom solution version (trained model).
*
*/
private TrainingDataConfig trainingDataConfig;
/**
*
* Specifies the automatic training configuration to use.
*
*/
private AutoTrainingConfig autoTrainingConfig;
/**
*
* Only events with a value greater than or equal to this threshold are used for training a model.
*
*
* @param eventValueThreshold
* Only events with a value greater than or equal to this threshold are used for training a model.
*/
public void setEventValueThreshold(String eventValueThreshold) {
this.eventValueThreshold = eventValueThreshold;
}
/**
*
* Only events with a value greater than or equal to this threshold are used for training a model.
*
*
* @return Only events with a value greater than or equal to this threshold are used for training a model.
*/
public String getEventValueThreshold() {
return this.eventValueThreshold;
}
/**
*
* Only events with a value greater than or equal to this threshold are used for training a model.
*
*
* @param eventValueThreshold
* Only events with a value greater than or equal to this threshold are used for training a model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig withEventValueThreshold(String eventValueThreshold) {
setEventValueThreshold(eventValueThreshold);
return this;
}
/**
*
* Describes the properties for hyperparameter optimization (HPO).
*
*
* @param hpoConfig
* Describes the properties for hyperparameter optimization (HPO).
*/
public void setHpoConfig(HPOConfig hpoConfig) {
this.hpoConfig = hpoConfig;
}
/**
*
* Describes the properties for hyperparameter optimization (HPO).
*
*
* @return Describes the properties for hyperparameter optimization (HPO).
*/
public HPOConfig getHpoConfig() {
return this.hpoConfig;
}
/**
*
* Describes the properties for hyperparameter optimization (HPO).
*
*
* @param hpoConfig
* Describes the properties for hyperparameter optimization (HPO).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig withHpoConfig(HPOConfig hpoConfig) {
setHpoConfig(hpoConfig);
return this;
}
/**
*
* Lists the algorithm hyperparameters and their values.
*
*
* @return Lists the algorithm hyperparameters and their values.
*/
public java.util.Map getAlgorithmHyperParameters() {
return algorithmHyperParameters;
}
/**
*
* Lists the algorithm hyperparameters and their values.
*
*
* @param algorithmHyperParameters
* Lists the algorithm hyperparameters and their values.
*/
public void setAlgorithmHyperParameters(java.util.Map algorithmHyperParameters) {
this.algorithmHyperParameters = algorithmHyperParameters;
}
/**
*
* Lists the algorithm hyperparameters and their values.
*
*
* @param algorithmHyperParameters
* Lists the algorithm hyperparameters and their values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig withAlgorithmHyperParameters(java.util.Map algorithmHyperParameters) {
setAlgorithmHyperParameters(algorithmHyperParameters);
return this;
}
/**
* Add a single AlgorithmHyperParameters entry
*
* @see SolutionConfig#withAlgorithmHyperParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig addAlgorithmHyperParametersEntry(String key, String value) {
if (null == this.algorithmHyperParameters) {
this.algorithmHyperParameters = new java.util.HashMap();
}
if (this.algorithmHyperParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.algorithmHyperParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into AlgorithmHyperParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig clearAlgorithmHyperParametersEntries() {
this.algorithmHyperParameters = null;
return this;
}
/**
*
* Lists the feature transformation parameters.
*
*
* @return Lists the feature transformation parameters.
*/
public java.util.Map getFeatureTransformationParameters() {
return featureTransformationParameters;
}
/**
*
* Lists the feature transformation parameters.
*
*
* @param featureTransformationParameters
* Lists the feature transformation parameters.
*/
public void setFeatureTransformationParameters(java.util.Map featureTransformationParameters) {
this.featureTransformationParameters = featureTransformationParameters;
}
/**
*
* Lists the feature transformation parameters.
*
*
* @param featureTransformationParameters
* Lists the feature transformation parameters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig withFeatureTransformationParameters(java.util.Map featureTransformationParameters) {
setFeatureTransformationParameters(featureTransformationParameters);
return this;
}
/**
* Add a single FeatureTransformationParameters entry
*
* @see SolutionConfig#withFeatureTransformationParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig addFeatureTransformationParametersEntry(String key, String value) {
if (null == this.featureTransformationParameters) {
this.featureTransformationParameters = new java.util.HashMap();
}
if (this.featureTransformationParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.featureTransformationParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into FeatureTransformationParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig clearFeatureTransformationParametersEntries() {
this.featureTransformationParameters = null;
return this;
}
/**
*
* The AutoMLConfig object
* containing a list of recipes to search when AutoML is performed.
*
*
* @param autoMLConfig
* The AutoMLConfig
* object containing a list of recipes to search when AutoML is performed.
*/
public void setAutoMLConfig(AutoMLConfig autoMLConfig) {
this.autoMLConfig = autoMLConfig;
}
/**
*
* The AutoMLConfig object
* containing a list of recipes to search when AutoML is performed.
*
*
* @return The AutoMLConfig
* object containing a list of recipes to search when AutoML is performed.
*/
public AutoMLConfig getAutoMLConfig() {
return this.autoMLConfig;
}
/**
*
* The AutoMLConfig object
* containing a list of recipes to search when AutoML is performed.
*
*
* @param autoMLConfig
* The AutoMLConfig
* object containing a list of recipes to search when AutoML is performed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig withAutoMLConfig(AutoMLConfig autoMLConfig) {
setAutoMLConfig(autoMLConfig);
return this;
}
/**
*
* Describes the additional objective for the solution, such as maximizing streaming minutes or increasing revenue.
* For more information see Optimizing a
* solution.
*
*
* @param optimizationObjective
* Describes the additional objective for the solution, such as maximizing streaming minutes or increasing
* revenue. For more information see Optimizing
* a solution.
*/
public void setOptimizationObjective(OptimizationObjective optimizationObjective) {
this.optimizationObjective = optimizationObjective;
}
/**
*
* Describes the additional objective for the solution, such as maximizing streaming minutes or increasing revenue.
* For more information see Optimizing a
* solution.
*
*
* @return Describes the additional objective for the solution, such as maximizing streaming minutes or increasing
* revenue. For more information see Optimizing a solution.
*/
public OptimizationObjective getOptimizationObjective() {
return this.optimizationObjective;
}
/**
*
* Describes the additional objective for the solution, such as maximizing streaming minutes or increasing revenue.
* For more information see Optimizing a
* solution.
*
*
* @param optimizationObjective
* Describes the additional objective for the solution, such as maximizing streaming minutes or increasing
* revenue. For more information see Optimizing
* a solution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig withOptimizationObjective(OptimizationObjective optimizationObjective) {
setOptimizationObjective(optimizationObjective);
return this;
}
/**
*
* Specifies the training data configuration to use when creating a custom solution version (trained model).
*
*
* @param trainingDataConfig
* Specifies the training data configuration to use when creating a custom solution version (trained model).
*/
public void setTrainingDataConfig(TrainingDataConfig trainingDataConfig) {
this.trainingDataConfig = trainingDataConfig;
}
/**
*
* Specifies the training data configuration to use when creating a custom solution version (trained model).
*
*
* @return Specifies the training data configuration to use when creating a custom solution version (trained model).
*/
public TrainingDataConfig getTrainingDataConfig() {
return this.trainingDataConfig;
}
/**
*
* Specifies the training data configuration to use when creating a custom solution version (trained model).
*
*
* @param trainingDataConfig
* Specifies the training data configuration to use when creating a custom solution version (trained model).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig withTrainingDataConfig(TrainingDataConfig trainingDataConfig) {
setTrainingDataConfig(trainingDataConfig);
return this;
}
/**
*
* Specifies the automatic training configuration to use.
*
*
* @param autoTrainingConfig
* Specifies the automatic training configuration to use.
*/
public void setAutoTrainingConfig(AutoTrainingConfig autoTrainingConfig) {
this.autoTrainingConfig = autoTrainingConfig;
}
/**
*
* Specifies the automatic training configuration to use.
*
*
* @return Specifies the automatic training configuration to use.
*/
public AutoTrainingConfig getAutoTrainingConfig() {
return this.autoTrainingConfig;
}
/**
*
* Specifies the automatic training configuration to use.
*
*
* @param autoTrainingConfig
* Specifies the automatic training configuration to use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SolutionConfig withAutoTrainingConfig(AutoTrainingConfig autoTrainingConfig) {
setAutoTrainingConfig(autoTrainingConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEventValueThreshold() != null)
sb.append("EventValueThreshold: ").append(getEventValueThreshold()).append(",");
if (getHpoConfig() != null)
sb.append("HpoConfig: ").append(getHpoConfig()).append(",");
if (getAlgorithmHyperParameters() != null)
sb.append("AlgorithmHyperParameters: ").append(getAlgorithmHyperParameters()).append(",");
if (getFeatureTransformationParameters() != null)
sb.append("FeatureTransformationParameters: ").append(getFeatureTransformationParameters()).append(",");
if (getAutoMLConfig() != null)
sb.append("AutoMLConfig: ").append(getAutoMLConfig()).append(",");
if (getOptimizationObjective() != null)
sb.append("OptimizationObjective: ").append(getOptimizationObjective()).append(",");
if (getTrainingDataConfig() != null)
sb.append("TrainingDataConfig: ").append(getTrainingDataConfig()).append(",");
if (getAutoTrainingConfig() != null)
sb.append("AutoTrainingConfig: ").append(getAutoTrainingConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SolutionConfig == false)
return false;
SolutionConfig other = (SolutionConfig) obj;
if (other.getEventValueThreshold() == null ^ this.getEventValueThreshold() == null)
return false;
if (other.getEventValueThreshold() != null && other.getEventValueThreshold().equals(this.getEventValueThreshold()) == false)
return false;
if (other.getHpoConfig() == null ^ this.getHpoConfig() == null)
return false;
if (other.getHpoConfig() != null && other.getHpoConfig().equals(this.getHpoConfig()) == false)
return false;
if (other.getAlgorithmHyperParameters() == null ^ this.getAlgorithmHyperParameters() == null)
return false;
if (other.getAlgorithmHyperParameters() != null && other.getAlgorithmHyperParameters().equals(this.getAlgorithmHyperParameters()) == false)
return false;
if (other.getFeatureTransformationParameters() == null ^ this.getFeatureTransformationParameters() == null)
return false;
if (other.getFeatureTransformationParameters() != null
&& other.getFeatureTransformationParameters().equals(this.getFeatureTransformationParameters()) == false)
return false;
if (other.getAutoMLConfig() == null ^ this.getAutoMLConfig() == null)
return false;
if (other.getAutoMLConfig() != null && other.getAutoMLConfig().equals(this.getAutoMLConfig()) == false)
return false;
if (other.getOptimizationObjective() == null ^ this.getOptimizationObjective() == null)
return false;
if (other.getOptimizationObjective() != null && other.getOptimizationObjective().equals(this.getOptimizationObjective()) == false)
return false;
if (other.getTrainingDataConfig() == null ^ this.getTrainingDataConfig() == null)
return false;
if (other.getTrainingDataConfig() != null && other.getTrainingDataConfig().equals(this.getTrainingDataConfig()) == false)
return false;
if (other.getAutoTrainingConfig() == null ^ this.getAutoTrainingConfig() == null)
return false;
if (other.getAutoTrainingConfig() != null && other.getAutoTrainingConfig().equals(this.getAutoTrainingConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEventValueThreshold() == null) ? 0 : getEventValueThreshold().hashCode());
hashCode = prime * hashCode + ((getHpoConfig() == null) ? 0 : getHpoConfig().hashCode());
hashCode = prime * hashCode + ((getAlgorithmHyperParameters() == null) ? 0 : getAlgorithmHyperParameters().hashCode());
hashCode = prime * hashCode + ((getFeatureTransformationParameters() == null) ? 0 : getFeatureTransformationParameters().hashCode());
hashCode = prime * hashCode + ((getAutoMLConfig() == null) ? 0 : getAutoMLConfig().hashCode());
hashCode = prime * hashCode + ((getOptimizationObjective() == null) ? 0 : getOptimizationObjective().hashCode());
hashCode = prime * hashCode + ((getTrainingDataConfig() == null) ? 0 : getTrainingDataConfig().hashCode());
hashCode = prime * hashCode + ((getAutoTrainingConfig() == null) ? 0 : getAutoTrainingConfig().hashCode());
return hashCode;
}
@Override
public SolutionConfig clone() {
try {
return (SolutionConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.personalize.model.transform.SolutionConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}