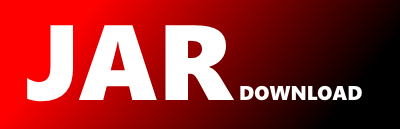
com.amazonaws.services.personalize.model.Solution Maven / Gradle / Ivy
Show all versions of aws-java-sdk-personalize Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.personalize.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
*
* After you create a solution, you can’t change its configuration. By default, all new solutions use automatic
* training. With automatic training, you incur training costs while your solution is active. You can't stop automatic
* training for a solution. To avoid unnecessary costs, make sure to delete the solution when you are finished. For
* information about training costs, see Amazon Personalize
* pricing.
*
*
*
* An object that provides information about a solution. A solution includes the custom recipe, customized parameters,
* and trained models (Solution Versions) that Amazon Personalize uses to generate recommendations.
*
*
* After you create a solution, you can’t change its configuration. If you need to make changes, you can clone the solution with the Amazon
* Personalize console or create a new one.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Solution implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the solution.
*
*/
private String name;
/**
*
* The ARN of the solution.
*
*/
private String solutionArn;
/**
*
* Whether to perform hyperparameter optimization (HPO) on the chosen recipe. The default is false
.
*
*/
private Boolean performHPO;
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* When true, Amazon Personalize performs a search for the best USER_PERSONALIZATION recipe from the list specified
* in the solution configuration (recipeArn
must not be specified). When false (the default), Amazon
* Personalize uses recipeArn
for training.
*
*/
private Boolean performAutoML;
/**
*
* Specifies whether the solution automatically creates solution versions. The default is True
and the
* solution automatically creates new solution versions every 7 days.
*
*
* For more information about auto training, see Creating and
* configuring a solution.
*
*/
private Boolean performAutoTraining;
/**
*
* The ARN of the recipe used to create the solution. This is required when performAutoML
is false.
*
*/
private String recipeArn;
/**
*
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*
*/
private String datasetGroupArn;
/**
*
* The event type (for example, 'click' or 'like') that is used for training the model. If no eventType
* is provided, Amazon Personalize uses all interactions for training with equal weight regardless of type.
*
*/
private String eventType;
/**
*
* Describes the configuration properties for the solution.
*
*/
private SolutionConfig solutionConfig;
/**
*
* When performAutoML
is true, specifies the best recipe found.
*
*/
private AutoMLResult autoMLResult;
/**
*
* The status of the solution.
*
*
* A solution can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*/
private String status;
/**
*
* The creation date and time (in Unix time) of the solution.
*
*/
private java.util.Date creationDateTime;
/**
*
* The date and time (in Unix time) that the solution was last updated.
*
*/
private java.util.Date lastUpdatedDateTime;
/**
*
* Describes the latest version of the solution, including the status and the ARN.
*
*/
private SolutionVersionSummary latestSolutionVersion;
/**
*
* The name of the solution.
*
*
* @param name
* The name of the solution.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the solution.
*
*
* @return The name of the solution.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the solution.
*
*
* @param name
* The name of the solution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withName(String name) {
setName(name);
return this;
}
/**
*
* The ARN of the solution.
*
*
* @param solutionArn
* The ARN of the solution.
*/
public void setSolutionArn(String solutionArn) {
this.solutionArn = solutionArn;
}
/**
*
* The ARN of the solution.
*
*
* @return The ARN of the solution.
*/
public String getSolutionArn() {
return this.solutionArn;
}
/**
*
* The ARN of the solution.
*
*
* @param solutionArn
* The ARN of the solution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withSolutionArn(String solutionArn) {
setSolutionArn(solutionArn);
return this;
}
/**
*
* Whether to perform hyperparameter optimization (HPO) on the chosen recipe. The default is false
.
*
*
* @param performHPO
* Whether to perform hyperparameter optimization (HPO) on the chosen recipe. The default is
* false
.
*/
public void setPerformHPO(Boolean performHPO) {
this.performHPO = performHPO;
}
/**
*
* Whether to perform hyperparameter optimization (HPO) on the chosen recipe. The default is false
.
*
*
* @return Whether to perform hyperparameter optimization (HPO) on the chosen recipe. The default is
* false
.
*/
public Boolean getPerformHPO() {
return this.performHPO;
}
/**
*
* Whether to perform hyperparameter optimization (HPO) on the chosen recipe. The default is false
.
*
*
* @param performHPO
* Whether to perform hyperparameter optimization (HPO) on the chosen recipe. The default is
* false
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withPerformHPO(Boolean performHPO) {
setPerformHPO(performHPO);
return this;
}
/**
*
* Whether to perform hyperparameter optimization (HPO) on the chosen recipe. The default is false
.
*
*
* @return Whether to perform hyperparameter optimization (HPO) on the chosen recipe. The default is
* false
.
*/
public Boolean isPerformHPO() {
return this.performHPO;
}
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* When true, Amazon Personalize performs a search for the best USER_PERSONALIZATION recipe from the list specified
* in the solution configuration (recipeArn
must not be specified). When false (the default), Amazon
* Personalize uses recipeArn
for training.
*
*
* @param performAutoML
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available
* Amazon Personalize recipes. For more information, see Determining your use
* case.
*
*
*
* When true, Amazon Personalize performs a search for the best USER_PERSONALIZATION recipe from the list
* specified in the solution configuration (recipeArn
must not be specified). When false (the
* default), Amazon Personalize uses recipeArn
for training.
*/
public void setPerformAutoML(Boolean performAutoML) {
this.performAutoML = performAutoML;
}
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* When true, Amazon Personalize performs a search for the best USER_PERSONALIZATION recipe from the list specified
* in the solution configuration (recipeArn
must not be specified). When false (the default), Amazon
* Personalize uses recipeArn
for training.
*
*
* @return
* We don't recommend enabling automated machine learning. Instead, match your use case to the available
* Amazon Personalize recipes. For more information, see Determining your use
* case.
*
*
*
* When true, Amazon Personalize performs a search for the best USER_PERSONALIZATION recipe from the list
* specified in the solution configuration (recipeArn
must not be specified). When false (the
* default), Amazon Personalize uses recipeArn
for training.
*/
public Boolean getPerformAutoML() {
return this.performAutoML;
}
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* When true, Amazon Personalize performs a search for the best USER_PERSONALIZATION recipe from the list specified
* in the solution configuration (recipeArn
must not be specified). When false (the default), Amazon
* Personalize uses recipeArn
for training.
*
*
* @param performAutoML
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available
* Amazon Personalize recipes. For more information, see Determining your use
* case.
*
*
*
* When true, Amazon Personalize performs a search for the best USER_PERSONALIZATION recipe from the list
* specified in the solution configuration (recipeArn
must not be specified). When false (the
* default), Amazon Personalize uses recipeArn
for training.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withPerformAutoML(Boolean performAutoML) {
setPerformAutoML(performAutoML);
return this;
}
/**
*
*
* We don't recommend enabling automated machine learning. Instead, match your use case to the available Amazon
* Personalize recipes. For more information, see Determining your use case.
*
*
*
* When true, Amazon Personalize performs a search for the best USER_PERSONALIZATION recipe from the list specified
* in the solution configuration (recipeArn
must not be specified). When false (the default), Amazon
* Personalize uses recipeArn
for training.
*
*
* @return
* We don't recommend enabling automated machine learning. Instead, match your use case to the available
* Amazon Personalize recipes. For more information, see Determining your use
* case.
*
*
*
* When true, Amazon Personalize performs a search for the best USER_PERSONALIZATION recipe from the list
* specified in the solution configuration (recipeArn
must not be specified). When false (the
* default), Amazon Personalize uses recipeArn
for training.
*/
public Boolean isPerformAutoML() {
return this.performAutoML;
}
/**
*
* Specifies whether the solution automatically creates solution versions. The default is True
and the
* solution automatically creates new solution versions every 7 days.
*
*
* For more information about auto training, see Creating and
* configuring a solution.
*
*
* @param performAutoTraining
* Specifies whether the solution automatically creates solution versions. The default is True
* and the solution automatically creates new solution versions every 7 days.
*
* For more information about auto training, see Creating and
* configuring a solution.
*/
public void setPerformAutoTraining(Boolean performAutoTraining) {
this.performAutoTraining = performAutoTraining;
}
/**
*
* Specifies whether the solution automatically creates solution versions. The default is True
and the
* solution automatically creates new solution versions every 7 days.
*
*
* For more information about auto training, see Creating and
* configuring a solution.
*
*
* @return Specifies whether the solution automatically creates solution versions. The default is True
* and the solution automatically creates new solution versions every 7 days.
*
* For more information about auto training, see Creating and
* configuring a solution.
*/
public Boolean getPerformAutoTraining() {
return this.performAutoTraining;
}
/**
*
* Specifies whether the solution automatically creates solution versions. The default is True
and the
* solution automatically creates new solution versions every 7 days.
*
*
* For more information about auto training, see Creating and
* configuring a solution.
*
*
* @param performAutoTraining
* Specifies whether the solution automatically creates solution versions. The default is True
* and the solution automatically creates new solution versions every 7 days.
*
* For more information about auto training, see Creating and
* configuring a solution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withPerformAutoTraining(Boolean performAutoTraining) {
setPerformAutoTraining(performAutoTraining);
return this;
}
/**
*
* Specifies whether the solution automatically creates solution versions. The default is True
and the
* solution automatically creates new solution versions every 7 days.
*
*
* For more information about auto training, see Creating and
* configuring a solution.
*
*
* @return Specifies whether the solution automatically creates solution versions. The default is True
* and the solution automatically creates new solution versions every 7 days.
*
* For more information about auto training, see Creating and
* configuring a solution.
*/
public Boolean isPerformAutoTraining() {
return this.performAutoTraining;
}
/**
*
* The ARN of the recipe used to create the solution. This is required when performAutoML
is false.
*
*
* @param recipeArn
* The ARN of the recipe used to create the solution. This is required when performAutoML
is
* false.
*/
public void setRecipeArn(String recipeArn) {
this.recipeArn = recipeArn;
}
/**
*
* The ARN of the recipe used to create the solution. This is required when performAutoML
is false.
*
*
* @return The ARN of the recipe used to create the solution. This is required when performAutoML
is
* false.
*/
public String getRecipeArn() {
return this.recipeArn;
}
/**
*
* The ARN of the recipe used to create the solution. This is required when performAutoML
is false.
*
*
* @param recipeArn
* The ARN of the recipe used to create the solution. This is required when performAutoML
is
* false.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withRecipeArn(String recipeArn) {
setRecipeArn(recipeArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*
*
* @param datasetGroupArn
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*/
public void setDatasetGroupArn(String datasetGroupArn) {
this.datasetGroupArn = datasetGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*
*
* @return The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*/
public String getDatasetGroupArn() {
return this.datasetGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
*
*
* @param datasetGroupArn
* The Amazon Resource Name (ARN) of the dataset group that provides the training data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withDatasetGroupArn(String datasetGroupArn) {
setDatasetGroupArn(datasetGroupArn);
return this;
}
/**
*
* The event type (for example, 'click' or 'like') that is used for training the model. If no eventType
* is provided, Amazon Personalize uses all interactions for training with equal weight regardless of type.
*
*
* @param eventType
* The event type (for example, 'click' or 'like') that is used for training the model. If no
* eventType
is provided, Amazon Personalize uses all interactions for training with equal
* weight regardless of type.
*/
public void setEventType(String eventType) {
this.eventType = eventType;
}
/**
*
* The event type (for example, 'click' or 'like') that is used for training the model. If no eventType
* is provided, Amazon Personalize uses all interactions for training with equal weight regardless of type.
*
*
* @return The event type (for example, 'click' or 'like') that is used for training the model. If no
* eventType
is provided, Amazon Personalize uses all interactions for training with equal
* weight regardless of type.
*/
public String getEventType() {
return this.eventType;
}
/**
*
* The event type (for example, 'click' or 'like') that is used for training the model. If no eventType
* is provided, Amazon Personalize uses all interactions for training with equal weight regardless of type.
*
*
* @param eventType
* The event type (for example, 'click' or 'like') that is used for training the model. If no
* eventType
is provided, Amazon Personalize uses all interactions for training with equal
* weight regardless of type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withEventType(String eventType) {
setEventType(eventType);
return this;
}
/**
*
* Describes the configuration properties for the solution.
*
*
* @param solutionConfig
* Describes the configuration properties for the solution.
*/
public void setSolutionConfig(SolutionConfig solutionConfig) {
this.solutionConfig = solutionConfig;
}
/**
*
* Describes the configuration properties for the solution.
*
*
* @return Describes the configuration properties for the solution.
*/
public SolutionConfig getSolutionConfig() {
return this.solutionConfig;
}
/**
*
* Describes the configuration properties for the solution.
*
*
* @param solutionConfig
* Describes the configuration properties for the solution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withSolutionConfig(SolutionConfig solutionConfig) {
setSolutionConfig(solutionConfig);
return this;
}
/**
*
* When performAutoML
is true, specifies the best recipe found.
*
*
* @param autoMLResult
* When performAutoML
is true, specifies the best recipe found.
*/
public void setAutoMLResult(AutoMLResult autoMLResult) {
this.autoMLResult = autoMLResult;
}
/**
*
* When performAutoML
is true, specifies the best recipe found.
*
*
* @return When performAutoML
is true, specifies the best recipe found.
*/
public AutoMLResult getAutoMLResult() {
return this.autoMLResult;
}
/**
*
* When performAutoML
is true, specifies the best recipe found.
*
*
* @param autoMLResult
* When performAutoML
is true, specifies the best recipe found.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withAutoMLResult(AutoMLResult autoMLResult) {
setAutoMLResult(autoMLResult);
return this;
}
/**
*
* The status of the solution.
*
*
* A solution can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* @param status
* The status of the solution.
*
* A solution can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the solution.
*
*
* A solution can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* @return The status of the solution.
*
* A solution can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the solution.
*
*
* A solution can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* @param status
* The status of the solution.
*
* A solution can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The creation date and time (in Unix time) of the solution.
*
*
* @param creationDateTime
* The creation date and time (in Unix time) of the solution.
*/
public void setCreationDateTime(java.util.Date creationDateTime) {
this.creationDateTime = creationDateTime;
}
/**
*
* The creation date and time (in Unix time) of the solution.
*
*
* @return The creation date and time (in Unix time) of the solution.
*/
public java.util.Date getCreationDateTime() {
return this.creationDateTime;
}
/**
*
* The creation date and time (in Unix time) of the solution.
*
*
* @param creationDateTime
* The creation date and time (in Unix time) of the solution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withCreationDateTime(java.util.Date creationDateTime) {
setCreationDateTime(creationDateTime);
return this;
}
/**
*
* The date and time (in Unix time) that the solution was last updated.
*
*
* @param lastUpdatedDateTime
* The date and time (in Unix time) that the solution was last updated.
*/
public void setLastUpdatedDateTime(java.util.Date lastUpdatedDateTime) {
this.lastUpdatedDateTime = lastUpdatedDateTime;
}
/**
*
* The date and time (in Unix time) that the solution was last updated.
*
*
* @return The date and time (in Unix time) that the solution was last updated.
*/
public java.util.Date getLastUpdatedDateTime() {
return this.lastUpdatedDateTime;
}
/**
*
* The date and time (in Unix time) that the solution was last updated.
*
*
* @param lastUpdatedDateTime
* The date and time (in Unix time) that the solution was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withLastUpdatedDateTime(java.util.Date lastUpdatedDateTime) {
setLastUpdatedDateTime(lastUpdatedDateTime);
return this;
}
/**
*
* Describes the latest version of the solution, including the status and the ARN.
*
*
* @param latestSolutionVersion
* Describes the latest version of the solution, including the status and the ARN.
*/
public void setLatestSolutionVersion(SolutionVersionSummary latestSolutionVersion) {
this.latestSolutionVersion = latestSolutionVersion;
}
/**
*
* Describes the latest version of the solution, including the status and the ARN.
*
*
* @return Describes the latest version of the solution, including the status and the ARN.
*/
public SolutionVersionSummary getLatestSolutionVersion() {
return this.latestSolutionVersion;
}
/**
*
* Describes the latest version of the solution, including the status and the ARN.
*
*
* @param latestSolutionVersion
* Describes the latest version of the solution, including the status and the ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Solution withLatestSolutionVersion(SolutionVersionSummary latestSolutionVersion) {
setLatestSolutionVersion(latestSolutionVersion);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getSolutionArn() != null)
sb.append("SolutionArn: ").append(getSolutionArn()).append(",");
if (getPerformHPO() != null)
sb.append("PerformHPO: ").append(getPerformHPO()).append(",");
if (getPerformAutoML() != null)
sb.append("PerformAutoML: ").append(getPerformAutoML()).append(",");
if (getPerformAutoTraining() != null)
sb.append("PerformAutoTraining: ").append(getPerformAutoTraining()).append(",");
if (getRecipeArn() != null)
sb.append("RecipeArn: ").append(getRecipeArn()).append(",");
if (getDatasetGroupArn() != null)
sb.append("DatasetGroupArn: ").append(getDatasetGroupArn()).append(",");
if (getEventType() != null)
sb.append("EventType: ").append(getEventType()).append(",");
if (getSolutionConfig() != null)
sb.append("SolutionConfig: ").append(getSolutionConfig()).append(",");
if (getAutoMLResult() != null)
sb.append("AutoMLResult: ").append(getAutoMLResult()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getCreationDateTime() != null)
sb.append("CreationDateTime: ").append(getCreationDateTime()).append(",");
if (getLastUpdatedDateTime() != null)
sb.append("LastUpdatedDateTime: ").append(getLastUpdatedDateTime()).append(",");
if (getLatestSolutionVersion() != null)
sb.append("LatestSolutionVersion: ").append(getLatestSolutionVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Solution == false)
return false;
Solution other = (Solution) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getSolutionArn() == null ^ this.getSolutionArn() == null)
return false;
if (other.getSolutionArn() != null && other.getSolutionArn().equals(this.getSolutionArn()) == false)
return false;
if (other.getPerformHPO() == null ^ this.getPerformHPO() == null)
return false;
if (other.getPerformHPO() != null && other.getPerformHPO().equals(this.getPerformHPO()) == false)
return false;
if (other.getPerformAutoML() == null ^ this.getPerformAutoML() == null)
return false;
if (other.getPerformAutoML() != null && other.getPerformAutoML().equals(this.getPerformAutoML()) == false)
return false;
if (other.getPerformAutoTraining() == null ^ this.getPerformAutoTraining() == null)
return false;
if (other.getPerformAutoTraining() != null && other.getPerformAutoTraining().equals(this.getPerformAutoTraining()) == false)
return false;
if (other.getRecipeArn() == null ^ this.getRecipeArn() == null)
return false;
if (other.getRecipeArn() != null && other.getRecipeArn().equals(this.getRecipeArn()) == false)
return false;
if (other.getDatasetGroupArn() == null ^ this.getDatasetGroupArn() == null)
return false;
if (other.getDatasetGroupArn() != null && other.getDatasetGroupArn().equals(this.getDatasetGroupArn()) == false)
return false;
if (other.getEventType() == null ^ this.getEventType() == null)
return false;
if (other.getEventType() != null && other.getEventType().equals(this.getEventType()) == false)
return false;
if (other.getSolutionConfig() == null ^ this.getSolutionConfig() == null)
return false;
if (other.getSolutionConfig() != null && other.getSolutionConfig().equals(this.getSolutionConfig()) == false)
return false;
if (other.getAutoMLResult() == null ^ this.getAutoMLResult() == null)
return false;
if (other.getAutoMLResult() != null && other.getAutoMLResult().equals(this.getAutoMLResult()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getCreationDateTime() == null ^ this.getCreationDateTime() == null)
return false;
if (other.getCreationDateTime() != null && other.getCreationDateTime().equals(this.getCreationDateTime()) == false)
return false;
if (other.getLastUpdatedDateTime() == null ^ this.getLastUpdatedDateTime() == null)
return false;
if (other.getLastUpdatedDateTime() != null && other.getLastUpdatedDateTime().equals(this.getLastUpdatedDateTime()) == false)
return false;
if (other.getLatestSolutionVersion() == null ^ this.getLatestSolutionVersion() == null)
return false;
if (other.getLatestSolutionVersion() != null && other.getLatestSolutionVersion().equals(this.getLatestSolutionVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getSolutionArn() == null) ? 0 : getSolutionArn().hashCode());
hashCode = prime * hashCode + ((getPerformHPO() == null) ? 0 : getPerformHPO().hashCode());
hashCode = prime * hashCode + ((getPerformAutoML() == null) ? 0 : getPerformAutoML().hashCode());
hashCode = prime * hashCode + ((getPerformAutoTraining() == null) ? 0 : getPerformAutoTraining().hashCode());
hashCode = prime * hashCode + ((getRecipeArn() == null) ? 0 : getRecipeArn().hashCode());
hashCode = prime * hashCode + ((getDatasetGroupArn() == null) ? 0 : getDatasetGroupArn().hashCode());
hashCode = prime * hashCode + ((getEventType() == null) ? 0 : getEventType().hashCode());
hashCode = prime * hashCode + ((getSolutionConfig() == null) ? 0 : getSolutionConfig().hashCode());
hashCode = prime * hashCode + ((getAutoMLResult() == null) ? 0 : getAutoMLResult().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getCreationDateTime() == null) ? 0 : getCreationDateTime().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedDateTime() == null) ? 0 : getLastUpdatedDateTime().hashCode());
hashCode = prime * hashCode + ((getLatestSolutionVersion() == null) ? 0 : getLatestSolutionVersion().hashCode());
return hashCode;
}
@Override
public Solution clone() {
try {
return (Solution) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.personalize.model.transform.SolutionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}