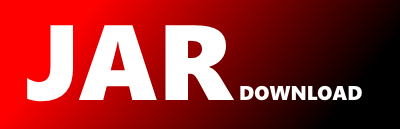
com.amazonaws.services.personalize.AmazonPersonalizeClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-personalize Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.personalize;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.personalize.AmazonPersonalizeClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.personalize.model.*;
import com.amazonaws.services.personalize.model.transform.*;
/**
* Client for accessing Amazon Personalize. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* Amazon Personalize is a machine learning service that makes it easy to add individualized recommendations to
* customers.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonPersonalizeClient extends AmazonWebServiceClient implements AmazonPersonalize {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonPersonalize.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "personalize";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyTagKeysException").withExceptionUnmarshaller(
com.amazonaws.services.personalize.model.transform.TooManyTagKeysExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidNextTokenException").withExceptionUnmarshaller(
com.amazonaws.services.personalize.model.transform.InvalidNextTokenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.personalize.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.personalize.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.personalize.model.transform.ResourceAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceInUseException").withExceptionUnmarshaller(
com.amazonaws.services.personalize.model.transform.ResourceInUseExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyTagsException").withExceptionUnmarshaller(
com.amazonaws.services.personalize.model.transform.TooManyTagsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidInputException").withExceptionUnmarshaller(
com.amazonaws.services.personalize.model.transform.InvalidInputExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.personalize.model.AmazonPersonalizeException.class));
public static AmazonPersonalizeClientBuilder builder() {
return AmazonPersonalizeClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Personalize using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonPersonalizeClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Personalize using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonPersonalizeClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("personalize.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/personalize/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/personalize/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Generates batch recommendations based on a list of items or users stored in Amazon S3 and exports the
* recommendations to an Amazon S3 bucket.
*
*
* To generate batch recommendations, specify the ARN of a solution version and an Amazon S3 URI for the input and
* output data. For user personalization, popular items, and personalized ranking solutions, the batch inference job
* generates a list of recommended items for each user ID in the input file. For related items solutions, the job
* generates a list of recommended items for each item ID in the input file.
*
*
* For more information, see Creating a batch
* inference job .
*
*
* If you use the Similar-Items recipe, Amazon Personalize can add descriptive themes to batch recommendations. To
* generate themes, set the job's mode to THEME_GENERATION
and specify the name of the field that
* contains item names in the input data.
*
*
* For more information about generating themes, see Batch recommendations
* with themes from Content Generator .
*
*
* You can't get batch recommendations with the Trending-Now or Next-Best-Action recipes.
*
*
* @param createBatchInferenceJobRequest
* @return Result of the CreateBatchInferenceJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateBatchInferenceJob
* @see AWS API Documentation
*/
@Override
public CreateBatchInferenceJobResult createBatchInferenceJob(CreateBatchInferenceJobRequest request) {
request = beforeClientExecution(request);
return executeCreateBatchInferenceJob(request);
}
@SdkInternalApi
final CreateBatchInferenceJobResult executeCreateBatchInferenceJob(CreateBatchInferenceJobRequest createBatchInferenceJobRequest) {
ExecutionContext executionContext = createExecutionContext(createBatchInferenceJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateBatchInferenceJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createBatchInferenceJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateBatchInferenceJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateBatchInferenceJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a batch segment job. The operation can handle up to 50 million records and the input file must be in JSON
* format. For more information, see Getting batch recommendations
* and user segments.
*
*
* @param createBatchSegmentJobRequest
* @return Result of the CreateBatchSegmentJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateBatchSegmentJob
* @see AWS API Documentation
*/
@Override
public CreateBatchSegmentJobResult createBatchSegmentJob(CreateBatchSegmentJobRequest request) {
request = beforeClientExecution(request);
return executeCreateBatchSegmentJob(request);
}
@SdkInternalApi
final CreateBatchSegmentJobResult executeCreateBatchSegmentJob(CreateBatchSegmentJobRequest createBatchSegmentJobRequest) {
ExecutionContext executionContext = createExecutionContext(createBatchSegmentJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateBatchSegmentJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createBatchSegmentJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateBatchSegmentJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateBatchSegmentJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* You incur campaign costs while it is active. To avoid unnecessary costs, make sure to delete the campaign when
* you are finished. For information about campaign costs, see Amazon Personalize pricing.
*
*
*
* Creates a campaign that deploys a solution version. When a client calls the GetRecommendations
* and
* GetPersonalizedRanking APIs, a campaign is specified in the request.
*
*
* Minimum Provisioned TPS and Auto-Scaling
*
*
*
* A high minProvisionedTPS
will increase your cost. We recommend starting with 1 for
* minProvisionedTPS
(the default). Track your usage using Amazon CloudWatch metrics, and increase the
* minProvisionedTPS
as necessary.
*
*
*
* When you create an Amazon Personalize campaign, you can specify the minimum provisioned transactions per second (
* minProvisionedTPS
) for the campaign. This is the baseline transaction throughput for the campaign
* provisioned by Amazon Personalize. It sets the minimum billing charge for the campaign while it is active. A
* transaction is a single GetRecommendations
or GetPersonalizedRanking
request. The
* default minProvisionedTPS
is 1.
*
*
* If your TPS increases beyond the minProvisionedTPS
, Amazon Personalize auto-scales the provisioned
* capacity up and down, but never below minProvisionedTPS
. There's a short time delay while the
* capacity is increased that might cause loss of transactions. When your traffic reduces, capacity returns to the
* minProvisionedTPS
.
*
*
* You are charged for the the minimum provisioned TPS or, if your requests exceed the
* minProvisionedTPS
, the actual TPS. The actual TPS is the total number of recommendation requests you
* make. We recommend starting with a low minProvisionedTPS
, track your usage using Amazon CloudWatch
* metrics, and then increase the minProvisionedTPS
as necessary.
*
*
* For more information about campaign costs, see Amazon
* Personalize pricing.
*
*
* Status
*
*
* A campaign can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the campaign status, call DescribeCampaign.
*
*
*
* Wait until the status
of the campaign is ACTIVE
before asking the campaign for
* recommendations.
*
*
*
* Related APIs
*
*
* -
*
* ListCampaigns
*
*
* -
*
* DescribeCampaign
*
*
* -
*
* UpdateCampaign
*
*
* -
*
* DeleteCampaign
*
*
*
*
* @param createCampaignRequest
* @return Result of the CreateCampaign operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateCampaign
* @see AWS API
* Documentation
*/
@Override
public CreateCampaignResult createCampaign(CreateCampaignRequest request) {
request = beforeClientExecution(request);
return executeCreateCampaign(request);
}
@SdkInternalApi
final CreateCampaignResult executeCreateCampaign(CreateCampaignRequest createCampaignRequest) {
ExecutionContext executionContext = createExecutionContext(createCampaignRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCampaignRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createCampaignRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCampaign");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateCampaignResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a batch job that deletes all references to specific users from an Amazon Personalize dataset group in
* batches. You specify the users to delete in a CSV file of userIds in an Amazon S3 bucket. After a job completes,
* Amazon Personalize no longer trains on the users’ data and no longer considers the users when generating user
* segments. For more information about creating a data deletion job, see Deleting users.
*
*
* -
*
* Your input file must be a CSV file with a single USER_ID column that lists the users IDs. For more information
* about preparing the CSV file, see Preparing your data
* deletion file and uploading it to Amazon S3.
*
*
* -
*
* To give Amazon Personalize permission to access your input CSV file of userIds, you must specify an IAM service
* role that has permission to read from the data source. This role needs GetObject
and
* ListBucket
permissions for the bucket and its content. These permissions are the same as importing
* data. For information on granting access to your Amazon S3 bucket, see Giving Amazon
* Personalize Access to Amazon S3 Resources.
*
*
*
*
* After you create a job, it can take up to a day to delete all references to the users from datasets and models.
* Until the job completes, Amazon Personalize continues to use the data when training. And if you use a User
* Segmentation recipe, the users might appear in user segments.
*
*
* Status
*
*
* A data deletion job can have one of the following statuses:
*
*
* -
*
* PENDING > IN_PROGRESS > COMPLETED -or- FAILED
*
*
*
*
* To get the status of the data deletion job, call DescribeDataDeletionJob API operation and specify the Amazon Resource Name (ARN) of the job. If the status
* is FAILED, the response includes a failureReason
key, which describes why the job failed.
*
*
* Related APIs
*
*
* -
*
*
* -
*
*
*
*
* @param createDataDeletionJobRequest
* @return Result of the CreateDataDeletionJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateDataDeletionJob
* @see AWS API Documentation
*/
@Override
public CreateDataDeletionJobResult createDataDeletionJob(CreateDataDeletionJobRequest request) {
request = beforeClientExecution(request);
return executeCreateDataDeletionJob(request);
}
@SdkInternalApi
final CreateDataDeletionJobResult executeCreateDataDeletionJob(CreateDataDeletionJobRequest createDataDeletionJobRequest) {
ExecutionContext executionContext = createExecutionContext(createDataDeletionJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDataDeletionJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDataDeletionJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDataDeletionJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateDataDeletionJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an empty dataset and adds it to the specified dataset group. Use CreateDatasetImportJob to import your training data to a dataset.
*
*
* There are 5 types of datasets:
*
*
* -
*
* Item interactions
*
*
* -
*
* Items
*
*
* -
*
* Users
*
*
* -
*
* Action interactions
*
*
* -
*
* Actions
*
*
*
*
* Each dataset type has an associated schema with required field types. Only the Item interactions
* dataset is required in order to train a model (also referred to as creating a solution).
*
*
* A dataset can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the status of the dataset, call DescribeDataset.
*
*
* Related APIs
*
*
* -
*
*
* -
*
* ListDatasets
*
*
* -
*
* DescribeDataset
*
*
* -
*
* DeleteDataset
*
*
*
*
* @param createDatasetRequest
* @return Result of the CreateDataset operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateDataset
* @see AWS API
* Documentation
*/
@Override
public CreateDatasetResult createDataset(CreateDatasetRequest request) {
request = beforeClientExecution(request);
return executeCreateDataset(request);
}
@SdkInternalApi
final CreateDatasetResult executeCreateDataset(CreateDatasetRequest createDatasetRequest) {
ExecutionContext executionContext = createExecutionContext(createDatasetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDatasetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDatasetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDataset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDatasetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a job that exports data from your dataset to an Amazon S3 bucket. To allow Amazon Personalize to export
* the training data, you must specify an service-linked IAM role that gives Amazon Personalize
* PutObject
permissions for your Amazon S3 bucket. For information, see Exporting a dataset in the Amazon
* Personalize developer guide.
*
*
* Status
*
*
* A dataset export job can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
*
*
* To get the status of the export job, call DescribeDatasetExportJob, and specify the Amazon Resource Name (ARN) of the dataset export job. The dataset
* export is complete when the status shows as ACTIVE. If the status shows as CREATE FAILED, the response includes a
* failureReason
key, which describes why the job failed.
*
*
* @param createDatasetExportJobRequest
* @return Result of the CreateDatasetExportJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateDatasetExportJob
* @see AWS API Documentation
*/
@Override
public CreateDatasetExportJobResult createDatasetExportJob(CreateDatasetExportJobRequest request) {
request = beforeClientExecution(request);
return executeCreateDatasetExportJob(request);
}
@SdkInternalApi
final CreateDatasetExportJobResult executeCreateDatasetExportJob(CreateDatasetExportJobRequest createDatasetExportJobRequest) {
ExecutionContext executionContext = createExecutionContext(createDatasetExportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDatasetExportJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDatasetExportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDatasetExportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateDatasetExportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an empty dataset group. A dataset group is a container for Amazon Personalize resources. A dataset group
* can contain at most three datasets, one for each type of dataset:
*
*
* -
*
* Item interactions
*
*
* -
*
* Items
*
*
* -
*
* Users
*
*
* -
*
* Actions
*
*
* -
*
* Action interactions
*
*
*
*
* A dataset group can be a Domain dataset group, where you specify a domain and use pre-configured resources like
* recommenders, or a Custom dataset group, where you use custom resources, such as a solution with a solution
* version, that you deploy with a campaign. If you start with a Domain dataset group, you can still add custom
* resources such as solutions and solution versions trained with recipes for custom use cases and deployed with
* campaigns.
*
*
* A dataset group can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING
*
*
*
*
* To get the status of the dataset group, call DescribeDatasetGroup.
* If the status shows as CREATE FAILED, the response includes a failureReason
key, which describes why
* the creation failed.
*
*
*
* You must wait until the status
of the dataset group is ACTIVE
before adding a dataset
* to the group.
*
*
*
* You can specify an Key Management Service (KMS) key to encrypt the datasets in the group. If you specify a KMS
* key, you must also include an Identity and Access Management (IAM) role that has permission to access the key.
*
*
* APIs that require a dataset group ARN in the request
*
*
* -
*
* CreateDataset
*
*
* -
*
*
* -
*
* CreateSolution
*
*
*
*
* Related APIs
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createDatasetGroupRequest
* @return Result of the CreateDatasetGroup operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateDatasetGroup
* @see AWS
* API Documentation
*/
@Override
public CreateDatasetGroupResult createDatasetGroup(CreateDatasetGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateDatasetGroup(request);
}
@SdkInternalApi
final CreateDatasetGroupResult executeCreateDatasetGroup(CreateDatasetGroupRequest createDatasetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createDatasetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDatasetGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDatasetGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDatasetGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDatasetGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a job that imports training data from your data source (an Amazon S3 bucket) to an Amazon Personalize
* dataset. To allow Amazon Personalize to ACTIVE -or- CREATE FAILED
*
*
*
* To get the status of the import job, call DescribeDatasetImportJob, providing the Amazon Resource Name (ARN) of the dataset import job. The dataset
* import is complete when the status shows as ACTIVE. If the status shows as CREATE FAILED, the response includes a
* failureReason
key, which describes why the job failed.
*
*
*
* Importing takes time. You must wait until the status shows as ACTIVE before training a model using the dataset.
*
*
*
* Related APIs
*
*
* -
*
*
* -
*
*
*
*
* @param createDatasetImportJobRequest
* @return Result of the CreateDatasetImportJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateDatasetImportJob
* @see AWS API Documentation
*/
@Override
public CreateDatasetImportJobResult createDatasetImportJob(CreateDatasetImportJobRequest request) {
request = beforeClientExecution(request);
return executeCreateDatasetImportJob(request);
}
@SdkInternalApi
final CreateDatasetImportJobResult executeCreateDatasetImportJob(CreateDatasetImportJobRequest createDatasetImportJobRequest) {
ExecutionContext executionContext = createExecutionContext(createDatasetImportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDatasetImportJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDatasetImportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDatasetImportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateDatasetImportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an event tracker that you use when adding event data to a specified dataset group using the PutEvents API.
*
*
*
* Only one event tracker can be associated with a dataset group. You will get an error if you call
* CreateEventTracker
using the same dataset group as an existing event tracker.
*
*
*
* When you create an event tracker, the response includes a tracking ID, which you pass as a parameter when you use
* the PutEvents operation.
* Amazon Personalize then appends the event data to the Item interactions dataset of the dataset group you specify
* in your event tracker.
*
*
* The event tracker can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the status of the event tracker, call DescribeEventTracker.
*
*
*
* The event tracker must be in the ACTIVE state before using the tracking ID.
*
*
*
* Related APIs
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createEventTrackerRequest
* @return Result of the CreateEventTracker operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateEventTracker
* @see AWS
* API Documentation
*/
@Override
public CreateEventTrackerResult createEventTracker(CreateEventTrackerRequest request) {
request = beforeClientExecution(request);
return executeCreateEventTracker(request);
}
@SdkInternalApi
final CreateEventTrackerResult executeCreateEventTracker(CreateEventTrackerRequest createEventTrackerRequest) {
ExecutionContext executionContext = createExecutionContext(createEventTrackerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEventTrackerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createEventTrackerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEventTracker");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateEventTrackerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a recommendation filter. For more information, see Filtering recommendations and user
* segments.
*
*
* @param createFilterRequest
* @return Result of the CreateFilter operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateFilter
* @see AWS API
* Documentation
*/
@Override
public CreateFilterResult createFilter(CreateFilterRequest request) {
request = beforeClientExecution(request);
return executeCreateFilter(request);
}
@SdkInternalApi
final CreateFilterResult executeCreateFilter(CreateFilterRequest createFilterRequest) {
ExecutionContext executionContext = createExecutionContext(createFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a metric attribution. A metric attribution creates reports on the data that you import into Amazon
* Personalize. Depending on how you imported the data, you can view reports in Amazon CloudWatch or Amazon S3. For
* more information, see Measuring impact of
* recommendations.
*
*
* @param createMetricAttributionRequest
* @return Result of the CreateMetricAttribution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @sample AmazonPersonalize.CreateMetricAttribution
* @see AWS API Documentation
*/
@Override
public CreateMetricAttributionResult createMetricAttribution(CreateMetricAttributionRequest request) {
request = beforeClientExecution(request);
return executeCreateMetricAttribution(request);
}
@SdkInternalApi
final CreateMetricAttributionResult executeCreateMetricAttribution(CreateMetricAttributionRequest createMetricAttributionRequest) {
ExecutionContext executionContext = createExecutionContext(createMetricAttributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMetricAttributionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMetricAttributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMetricAttribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMetricAttributionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a recommender with the recipe (a Domain dataset group use case) you specify. You create recommenders for
* a Domain dataset group and specify the recommender's Amazon Resource Name (ARN) when you make a GetRecommendations
* request.
*
*
* Minimum recommendation requests per second
*
*
*
* A high minRecommendationRequestsPerSecond
will increase your bill. We recommend starting with 1 for
* minRecommendationRequestsPerSecond
(the default). Track your usage using Amazon CloudWatch metrics,
* and increase the minRecommendationRequestsPerSecond
as necessary.
*
*
*
* When you create a recommender, you can configure the recommender's minimum recommendation requests per second.
* The minimum recommendation requests per second (minRecommendationRequestsPerSecond
) specifies the
* baseline recommendation request throughput provisioned by Amazon Personalize. The default
* minRecommendationRequestsPerSecond is 1
. A recommendation request is a single
* GetRecommendations
operation. Request throughput is measured in requests per second and Amazon
* Personalize uses your requests per second to derive your requests per hour and the price of your recommender
* usage.
*
*
* If your requests per second increases beyond minRecommendationRequestsPerSecond
, Amazon Personalize
* auto-scales the provisioned capacity up and down, but never below minRecommendationRequestsPerSecond
* . There's a short time delay while the capacity is increased that might cause loss of requests.
*
*
* Your bill is the greater of either the minimum requests per hour (based on minRecommendationRequestsPerSecond) or
* the actual number of requests. The actual request throughput used is calculated as the average requests/second
* within a one-hour window. We recommend starting with the default minRecommendationRequestsPerSecond
,
* track your usage using Amazon CloudWatch metrics, and then increase the
* minRecommendationRequestsPerSecond
as necessary.
*
*
* Status
*
*
* A recommender can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* STOP PENDING > STOP IN_PROGRESS > INACTIVE > START PENDING > START IN_PROGRESS > ACTIVE
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the recommender status, call DescribeRecommender.
*
*
*
* Wait until the status
of the recommender is ACTIVE
before asking the recommender for
* recommendations.
*
*
*
* Related APIs
*
*
* -
*
* ListRecommenders
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createRecommenderRequest
* @return Result of the CreateRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateRecommender
* @see AWS
* API Documentation
*/
@Override
public CreateRecommenderResult createRecommender(CreateRecommenderRequest request) {
request = beforeClientExecution(request);
return executeCreateRecommender(request);
}
@SdkInternalApi
final CreateRecommenderResult executeCreateRecommender(CreateRecommenderRequest createRecommenderRequest) {
ExecutionContext executionContext = createExecutionContext(createRecommenderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRecommenderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createRecommenderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRecommender");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateRecommenderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Personalize schema from the specified schema string. The schema you create must be in Avro JSON
* format.
*
*
* Amazon Personalize recognizes three schema variants. Each schema is associated with a dataset type and has a set
* of required field and keywords. If you are creating a schema for a dataset in a Domain dataset group, you provide
* the domain of the Domain dataset group. You specify a schema when you call CreateDataset.
*
*
* Related APIs
*
*
* -
*
* ListSchemas
*
*
* -
*
* DescribeSchema
*
*
* -
*
* DeleteSchema
*
*
*
*
* @param createSchemaRequest
* @return Result of the CreateSchema operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @sample AmazonPersonalize.CreateSchema
* @see AWS API
* Documentation
*/
@Override
public CreateSchemaResult createSchema(CreateSchemaRequest request) {
request = beforeClientExecution(request);
return executeCreateSchema(request);
}
@SdkInternalApi
final CreateSchemaResult executeCreateSchema(CreateSchemaRequest createSchemaRequest) {
ExecutionContext executionContext = createExecutionContext(createSchemaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSchemaRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSchemaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSchema");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSchemaResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* After you create a solution, you can’t change its configuration. By default, all new solutions use automatic
* training. With automatic training, you incur training costs while your solution is active. You can't stop
* automatic training for a solution. To avoid unnecessary costs, make sure to delete the solution when you are
* finished. For information about training costs, see Amazon
* Personalize pricing.
*
*
*
* Creates the configuration for training a model (creating a solution version). This configuration includes the
* recipe to use for model training and optional training configuration, such as columns to use in training and
* feature transformation parameters. For more information about configuring a solution, see Creating and
* configuring a solution.
*
*
* By default, new solutions use automatic training to create solution versions every 7 days. You can change the
* training frequency. Automatic solution version creation starts one hour after the solution is ACTIVE. If you
* manually create a solution version within the hour, the solution skips the first automatic training. For more
* information, see Configuring automatic
* training.
*
*
* To turn off automatic training, set performAutoTraining
to false. If you turn off automatic
* training, you must manually create a solution version by calling the CreateSolutionVersion
* operation.
*
*
* After training starts, you can get the solution version's Amazon Resource Name (ARN) with the ListSolutionVersions
* API operation. To get its status, use the DescribeSolutionVersion.
*
*
* After training completes you can evaluate model accuracy by calling GetSolutionMetrics. When
* you are satisfied with the solution version, you deploy it using CreateCampaign. The campaign
* provides recommendations to a client through the GetRecommendations
* API.
*
*
*
* Amazon Personalize doesn't support configuring the hpoObjective
for solution hyperparameter
* optimization at this time.
*
*
*
* Status
*
*
* A solution can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* To get the status of the solution, call DescribeSolution. If you
* use manual training, the status must be ACTIVE before you call CreateSolutionVersion
.
*
*
* Related APIs
*
*
* -
*
* ListSolutions
*
*
* -
*
*
* -
*
* DescribeSolution
*
*
* -
*
* DeleteSolution
*
*
*
*
* -
*
*
* -
*
*
*
*
* @param createSolutionRequest
* @return Result of the CreateSolution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @sample AmazonPersonalize.CreateSolution
* @see AWS API
* Documentation
*/
@Override
public CreateSolutionResult createSolution(CreateSolutionRequest request) {
request = beforeClientExecution(request);
return executeCreateSolution(request);
}
@SdkInternalApi
final CreateSolutionResult executeCreateSolution(CreateSolutionRequest createSolutionRequest) {
ExecutionContext executionContext = createExecutionContext(createSolutionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSolutionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSolutionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSolution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSolutionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Trains or retrains an active solution in a Custom dataset group. A solution is created using the CreateSolution operation and
* must be in the ACTIVE state before calling CreateSolutionVersion
. A new version of the solution is
* created every time you call this operation.
*
*
* Status
*
*
* A solution version can be in one of the following states:
*
*
* -
*
* CREATE PENDING
*
*
* -
*
* CREATE IN_PROGRESS
*
*
* -
*
* ACTIVE
*
*
* -
*
* CREATE FAILED
*
*
* -
*
* CREATE STOPPING
*
*
* -
*
* CREATE STOPPED
*
*
*
*
* To get the status of the version, call DescribeSolutionVersion. Wait until the status shows as ACTIVE before calling CreateCampaign
.
*
*
* If the status shows as CREATE FAILED, the response includes a failureReason
key, which describes why
* the job failed.
*
*
* Related APIs
*
*
* -
*
*
* -
*
*
* -
*
* ListSolutions
*
*
* -
*
* CreateSolution
*
*
* -
*
* DescribeSolution
*
*
* -
*
* DeleteSolution
*
*
*
*
* @param createSolutionVersionRequest
* @return Result of the CreateSolutionVersion operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @sample AmazonPersonalize.CreateSolutionVersion
* @see AWS API Documentation
*/
@Override
public CreateSolutionVersionResult createSolutionVersion(CreateSolutionVersionRequest request) {
request = beforeClientExecution(request);
return executeCreateSolutionVersion(request);
}
@SdkInternalApi
final CreateSolutionVersionResult executeCreateSolutionVersion(CreateSolutionVersionRequest createSolutionVersionRequest) {
ExecutionContext executionContext = createExecutionContext(createSolutionVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSolutionVersionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSolutionVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSolutionVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateSolutionVersionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes a campaign by deleting the solution deployment. The solution that the campaign is based on is not deleted
* and can be redeployed when needed. A deleted campaign can no longer be specified in a GetRecommendations
* request. For information on creating campaigns, see CreateCampaign.
*
*
* @param deleteCampaignRequest
* @return Result of the DeleteCampaign operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteCampaign
* @see AWS API
* Documentation
*/
@Override
public DeleteCampaignResult deleteCampaign(DeleteCampaignRequest request) {
request = beforeClientExecution(request);
return executeDeleteCampaign(request);
}
@SdkInternalApi
final DeleteCampaignResult executeDeleteCampaign(DeleteCampaignRequest deleteCampaignRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCampaignRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCampaignRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteCampaignRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCampaign");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteCampaignResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a dataset. You can't delete a dataset if an associated DatasetImportJob
or
* SolutionVersion
is in the CREATE PENDING or IN PROGRESS state. For more information on datasets, see
* CreateDataset.
*
*
* @param deleteDatasetRequest
* @return Result of the DeleteDataset operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteDataset
* @see AWS API
* Documentation
*/
@Override
public DeleteDatasetResult deleteDataset(DeleteDatasetRequest request) {
request = beforeClientExecution(request);
return executeDeleteDataset(request);
}
@SdkInternalApi
final DeleteDatasetResult executeDeleteDataset(DeleteDatasetRequest deleteDatasetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDatasetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDatasetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDatasetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDataset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDatasetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a dataset group. Before you delete a dataset group, you must delete the following:
*
*
* -
*
* All associated event trackers.
*
*
* -
*
* All associated solutions.
*
*
* -
*
* All datasets in the dataset group.
*
*
*
*
* @param deleteDatasetGroupRequest
* @return Result of the DeleteDatasetGroup operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteDatasetGroup
* @see AWS
* API Documentation
*/
@Override
public DeleteDatasetGroupResult deleteDatasetGroup(DeleteDatasetGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteDatasetGroup(request);
}
@SdkInternalApi
final DeleteDatasetGroupResult executeDeleteDatasetGroup(DeleteDatasetGroupRequest deleteDatasetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDatasetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDatasetGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDatasetGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDatasetGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDatasetGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the event tracker. Does not delete the dataset from the dataset group. For more information on event
* trackers, see CreateEventTracker.
*
*
* @param deleteEventTrackerRequest
* @return Result of the DeleteEventTracker operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteEventTracker
* @see AWS
* API Documentation
*/
@Override
public DeleteEventTrackerResult deleteEventTracker(DeleteEventTrackerRequest request) {
request = beforeClientExecution(request);
return executeDeleteEventTracker(request);
}
@SdkInternalApi
final DeleteEventTrackerResult executeDeleteEventTracker(DeleteEventTrackerRequest deleteEventTrackerRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEventTrackerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEventTrackerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteEventTrackerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEventTracker");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteEventTrackerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a filter.
*
*
* @param deleteFilterRequest
* @return Result of the DeleteFilter operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteFilter
* @see AWS API
* Documentation
*/
@Override
public DeleteFilterResult deleteFilter(DeleteFilterRequest request) {
request = beforeClientExecution(request);
return executeDeleteFilter(request);
}
@SdkInternalApi
final DeleteFilterResult executeDeleteFilter(DeleteFilterRequest deleteFilterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a metric attribution.
*
*
* @param deleteMetricAttributionRequest
* @return Result of the DeleteMetricAttribution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteMetricAttribution
* @see AWS API Documentation
*/
@Override
public DeleteMetricAttributionResult deleteMetricAttribution(DeleteMetricAttributionRequest request) {
request = beforeClientExecution(request);
return executeDeleteMetricAttribution(request);
}
@SdkInternalApi
final DeleteMetricAttributionResult executeDeleteMetricAttribution(DeleteMetricAttributionRequest deleteMetricAttributionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMetricAttributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMetricAttributionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteMetricAttributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMetricAttribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteMetricAttributionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deactivates and removes a recommender. A deleted recommender can no longer be specified in a GetRecommendations
* request.
*
*
* @param deleteRecommenderRequest
* @return Result of the DeleteRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteRecommender
* @see AWS
* API Documentation
*/
@Override
public DeleteRecommenderResult deleteRecommender(DeleteRecommenderRequest request) {
request = beforeClientExecution(request);
return executeDeleteRecommender(request);
}
@SdkInternalApi
final DeleteRecommenderResult executeDeleteRecommender(DeleteRecommenderRequest deleteRecommenderRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRecommenderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRecommenderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteRecommenderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRecommender");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteRecommenderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a schema. Before deleting a schema, you must delete all datasets referencing the schema. For more
* information on schemas, see CreateSchema.
*
*
* @param deleteSchemaRequest
* @return Result of the DeleteSchema operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteSchema
* @see AWS API
* Documentation
*/
@Override
public DeleteSchemaResult deleteSchema(DeleteSchemaRequest request) {
request = beforeClientExecution(request);
return executeDeleteSchema(request);
}
@SdkInternalApi
final DeleteSchemaResult executeDeleteSchema(DeleteSchemaRequest deleteSchemaRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSchemaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSchemaRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSchemaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSchema");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSchemaResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes all versions of a solution and the Solution
object itself. Before deleting a solution, you
* must delete all campaigns based on the solution. To determine what campaigns are using the solution, call ListCampaigns and supply the
* Amazon Resource Name (ARN) of the solution. You can't delete a solution if an associated
* SolutionVersion
is in the CREATE PENDING or IN PROGRESS state. For more information on solutions,
* see CreateSolution.
*
*
* @param deleteSolutionRequest
* @return Result of the DeleteSolution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.DeleteSolution
* @see AWS API
* Documentation
*/
@Override
public DeleteSolutionResult deleteSolution(DeleteSolutionRequest request) {
request = beforeClientExecution(request);
return executeDeleteSolution(request);
}
@SdkInternalApi
final DeleteSolutionResult executeDeleteSolution(DeleteSolutionRequest deleteSolutionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSolutionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSolutionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSolutionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSolution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSolutionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the given algorithm.
*
*
* @param describeAlgorithmRequest
* @return Result of the DescribeAlgorithm operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeAlgorithm
* @see AWS
* API Documentation
*/
@Override
public DescribeAlgorithmResult describeAlgorithm(DescribeAlgorithmRequest request) {
request = beforeClientExecution(request);
return executeDescribeAlgorithm(request);
}
@SdkInternalApi
final DescribeAlgorithmResult executeDescribeAlgorithm(DescribeAlgorithmRequest describeAlgorithmRequest) {
ExecutionContext executionContext = createExecutionContext(describeAlgorithmRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAlgorithmRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeAlgorithmRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAlgorithm");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeAlgorithmResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the properties of a batch inference job including name, Amazon Resource Name (ARN), status, input and output
* configurations, and the ARN of the solution version used to generate the recommendations.
*
*
* @param describeBatchInferenceJobRequest
* @return Result of the DescribeBatchInferenceJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeBatchInferenceJob
* @see AWS API Documentation
*/
@Override
public DescribeBatchInferenceJobResult describeBatchInferenceJob(DescribeBatchInferenceJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeBatchInferenceJob(request);
}
@SdkInternalApi
final DescribeBatchInferenceJobResult executeDescribeBatchInferenceJob(DescribeBatchInferenceJobRequest describeBatchInferenceJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeBatchInferenceJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeBatchInferenceJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeBatchInferenceJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeBatchInferenceJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeBatchInferenceJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the properties of a batch segment job including name, Amazon Resource Name (ARN), status, input and output
* configurations, and the ARN of the solution version used to generate segments.
*
*
* @param describeBatchSegmentJobRequest
* @return Result of the DescribeBatchSegmentJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeBatchSegmentJob
* @see AWS API Documentation
*/
@Override
public DescribeBatchSegmentJobResult describeBatchSegmentJob(DescribeBatchSegmentJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeBatchSegmentJob(request);
}
@SdkInternalApi
final DescribeBatchSegmentJobResult executeDescribeBatchSegmentJob(DescribeBatchSegmentJobRequest describeBatchSegmentJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeBatchSegmentJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeBatchSegmentJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeBatchSegmentJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeBatchSegmentJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeBatchSegmentJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the given campaign, including its status.
*
*
* A campaign can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* When the status
is CREATE FAILED
, the response includes the failureReason
* key, which describes why.
*
*
* For more information on campaigns, see CreateCampaign.
*
*
* @param describeCampaignRequest
* @return Result of the DescribeCampaign operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeCampaign
* @see AWS
* API Documentation
*/
@Override
public DescribeCampaignResult describeCampaign(DescribeCampaignRequest request) {
request = beforeClientExecution(request);
return executeDescribeCampaign(request);
}
@SdkInternalApi
final DescribeCampaignResult executeDescribeCampaign(DescribeCampaignRequest describeCampaignRequest) {
ExecutionContext executionContext = createExecutionContext(describeCampaignRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCampaignRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeCampaignRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeCampaign");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeCampaignResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the data deletion job created by CreateDataDeletionJob, including the job status.
*
*
* @param describeDataDeletionJobRequest
* @return Result of the DescribeDataDeletionJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeDataDeletionJob
* @see AWS API Documentation
*/
@Override
public DescribeDataDeletionJobResult describeDataDeletionJob(DescribeDataDeletionJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeDataDeletionJob(request);
}
@SdkInternalApi
final DescribeDataDeletionJobResult executeDescribeDataDeletionJob(DescribeDataDeletionJobRequest describeDataDeletionJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeDataDeletionJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDataDeletionJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeDataDeletionJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDataDeletionJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeDataDeletionJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the given dataset. For more information on datasets, see CreateDataset.
*
*
* @param describeDatasetRequest
* @return Result of the DescribeDataset operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeDataset
* @see AWS
* API Documentation
*/
@Override
public DescribeDatasetResult describeDataset(DescribeDatasetRequest request) {
request = beforeClientExecution(request);
return executeDescribeDataset(request);
}
@SdkInternalApi
final DescribeDatasetResult executeDescribeDataset(DescribeDatasetRequest describeDatasetRequest) {
ExecutionContext executionContext = createExecutionContext(describeDatasetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDatasetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeDatasetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDataset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeDatasetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the dataset export job created by CreateDatasetExportJob, including the export job status.
*
*
* @param describeDatasetExportJobRequest
* @return Result of the DescribeDatasetExportJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeDatasetExportJob
* @see AWS API Documentation
*/
@Override
public DescribeDatasetExportJobResult describeDatasetExportJob(DescribeDatasetExportJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeDatasetExportJob(request);
}
@SdkInternalApi
final DescribeDatasetExportJobResult executeDescribeDatasetExportJob(DescribeDatasetExportJobRequest describeDatasetExportJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeDatasetExportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDatasetExportJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeDatasetExportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDatasetExportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeDatasetExportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the given dataset group. For more information on dataset groups, see CreateDatasetGroup.
*
*
* @param describeDatasetGroupRequest
* @return Result of the DescribeDatasetGroup operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeDatasetGroup
* @see AWS API Documentation
*/
@Override
public DescribeDatasetGroupResult describeDatasetGroup(DescribeDatasetGroupRequest request) {
request = beforeClientExecution(request);
return executeDescribeDatasetGroup(request);
}
@SdkInternalApi
final DescribeDatasetGroupResult executeDescribeDatasetGroup(DescribeDatasetGroupRequest describeDatasetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(describeDatasetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDatasetGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeDatasetGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDatasetGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeDatasetGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the dataset import job created by CreateDatasetImportJob, including the import job status.
*
*
* @param describeDatasetImportJobRequest
* @return Result of the DescribeDatasetImportJob operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeDatasetImportJob
* @see AWS API Documentation
*/
@Override
public DescribeDatasetImportJobResult describeDatasetImportJob(DescribeDatasetImportJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeDatasetImportJob(request);
}
@SdkInternalApi
final DescribeDatasetImportJobResult executeDescribeDatasetImportJob(DescribeDatasetImportJobRequest describeDatasetImportJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeDatasetImportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDatasetImportJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeDatasetImportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDatasetImportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeDatasetImportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an event tracker. The response includes the trackingId
and status
of the
* event tracker. For more information on event trackers, see CreateEventTracker.
*
*
* @param describeEventTrackerRequest
* @return Result of the DescribeEventTracker operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeEventTracker
* @see AWS API Documentation
*/
@Override
public DescribeEventTrackerResult describeEventTracker(DescribeEventTrackerRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventTracker(request);
}
@SdkInternalApi
final DescribeEventTrackerResult executeDescribeEventTracker(DescribeEventTrackerRequest describeEventTrackerRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventTrackerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventTrackerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventTrackerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventTracker");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventTrackerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the given feature transformation.
*
*
* @param describeFeatureTransformationRequest
* @return Result of the DescribeFeatureTransformation operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeFeatureTransformation
* @see AWS API Documentation
*/
@Override
public DescribeFeatureTransformationResult describeFeatureTransformation(DescribeFeatureTransformationRequest request) {
request = beforeClientExecution(request);
return executeDescribeFeatureTransformation(request);
}
@SdkInternalApi
final DescribeFeatureTransformationResult executeDescribeFeatureTransformation(DescribeFeatureTransformationRequest describeFeatureTransformationRequest) {
ExecutionContext executionContext = createExecutionContext(describeFeatureTransformationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFeatureTransformationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeFeatureTransformationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFeatureTransformation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeFeatureTransformationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a filter's properties.
*
*
* @param describeFilterRequest
* @return Result of the DescribeFilter operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeFilter
* @see AWS API
* Documentation
*/
@Override
public DescribeFilterResult describeFilter(DescribeFilterRequest request) {
request = beforeClientExecution(request);
return executeDescribeFilter(request);
}
@SdkInternalApi
final DescribeFilterResult executeDescribeFilter(DescribeFilterRequest describeFilterRequest) {
ExecutionContext executionContext = createExecutionContext(describeFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a metric attribution.
*
*
* @param describeMetricAttributionRequest
* @return Result of the DescribeMetricAttribution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeMetricAttribution
* @see AWS API Documentation
*/
@Override
public DescribeMetricAttributionResult describeMetricAttribution(DescribeMetricAttributionRequest request) {
request = beforeClientExecution(request);
return executeDescribeMetricAttribution(request);
}
@SdkInternalApi
final DescribeMetricAttributionResult executeDescribeMetricAttribution(DescribeMetricAttributionRequest describeMetricAttributionRequest) {
ExecutionContext executionContext = createExecutionContext(describeMetricAttributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeMetricAttributionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeMetricAttributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeMetricAttribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeMetricAttributionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a recipe.
*
*
* A recipe contains three items:
*
*
* -
*
* An algorithm that trains a model.
*
*
* -
*
* Hyperparameters that govern the training.
*
*
* -
*
* Feature transformation information for modifying the input data before training.
*
*
*
*
* Amazon Personalize provides a set of predefined recipes. You specify a recipe when you create a solution with the
* CreateSolution API.
* CreateSolution
trains a model by using the algorithm in the specified recipe and a training dataset.
* The solution, when deployed as a campaign, can provide recommendations using the GetRecommendations
* API.
*
*
* @param describeRecipeRequest
* @return Result of the DescribeRecipe operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeRecipe
* @see AWS API
* Documentation
*/
@Override
public DescribeRecipeResult describeRecipe(DescribeRecipeRequest request) {
request = beforeClientExecution(request);
return executeDescribeRecipe(request);
}
@SdkInternalApi
final DescribeRecipeResult executeDescribeRecipe(DescribeRecipeRequest describeRecipeRequest) {
ExecutionContext executionContext = createExecutionContext(describeRecipeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRecipeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRecipeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRecipe");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeRecipeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the given recommender, including its status.
*
*
* A recommender can be in one of the following states:
*
*
* -
*
* CREATE PENDING > CREATE IN_PROGRESS > ACTIVE -or- CREATE FAILED
*
*
* -
*
* STOP PENDING > STOP IN_PROGRESS > INACTIVE > START PENDING > START IN_PROGRESS > ACTIVE
*
*
* -
*
* DELETE PENDING > DELETE IN_PROGRESS
*
*
*
*
* When the status
is CREATE FAILED
, the response includes the failureReason
* key, which describes why.
*
*
* The modelMetrics
key is null when the recommender is being created or deleted.
*
*
* For more information on recommenders, see CreateRecommender.
*
*
* @param describeRecommenderRequest
* @return Result of the DescribeRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeRecommender
* @see AWS API Documentation
*/
@Override
public DescribeRecommenderResult describeRecommender(DescribeRecommenderRequest request) {
request = beforeClientExecution(request);
return executeDescribeRecommender(request);
}
@SdkInternalApi
final DescribeRecommenderResult executeDescribeRecommender(DescribeRecommenderRequest describeRecommenderRequest) {
ExecutionContext executionContext = createExecutionContext(describeRecommenderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRecommenderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRecommenderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRecommender");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeRecommenderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a schema. For more information on schemas, see CreateSchema.
*
*
* @param describeSchemaRequest
* @return Result of the DescribeSchema operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeSchema
* @see AWS API
* Documentation
*/
@Override
public DescribeSchemaResult describeSchema(DescribeSchemaRequest request) {
request = beforeClientExecution(request);
return executeDescribeSchema(request);
}
@SdkInternalApi
final DescribeSchemaResult executeDescribeSchema(DescribeSchemaRequest describeSchemaRequest) {
ExecutionContext executionContext = createExecutionContext(describeSchemaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSchemaRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeSchemaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSchema");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeSchemaResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a solution. For more information on solutions, see CreateSolution.
*
*
* @param describeSolutionRequest
* @return Result of the DescribeSolution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeSolution
* @see AWS
* API Documentation
*/
@Override
public DescribeSolutionResult describeSolution(DescribeSolutionRequest request) {
request = beforeClientExecution(request);
return executeDescribeSolution(request);
}
@SdkInternalApi
final DescribeSolutionResult executeDescribeSolution(DescribeSolutionRequest describeSolutionRequest) {
ExecutionContext executionContext = createExecutionContext(describeSolutionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSolutionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeSolutionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSolution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeSolutionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a specific version of a solution. For more information on solutions, see CreateSolution
*
*
* @param describeSolutionVersionRequest
* @return Result of the DescribeSolutionVersion operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @sample AmazonPersonalize.DescribeSolutionVersion
* @see AWS API Documentation
*/
@Override
public DescribeSolutionVersionResult describeSolutionVersion(DescribeSolutionVersionRequest request) {
request = beforeClientExecution(request);
return executeDescribeSolutionVersion(request);
}
@SdkInternalApi
final DescribeSolutionVersionResult executeDescribeSolutionVersion(DescribeSolutionVersionRequest describeSolutionVersionRequest) {
ExecutionContext executionContext = createExecutionContext(describeSolutionVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSolutionVersionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeSolutionVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSolutionVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeSolutionVersionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the metrics for the specified solution version.
*
*
* @param getSolutionMetricsRequest
* @return Result of the GetSolutionMetrics operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.GetSolutionMetrics
* @see AWS
* API Documentation
*/
@Override
public GetSolutionMetricsResult getSolutionMetrics(GetSolutionMetricsRequest request) {
request = beforeClientExecution(request);
return executeGetSolutionMetrics(request);
}
@SdkInternalApi
final GetSolutionMetricsResult executeGetSolutionMetrics(GetSolutionMetricsRequest getSolutionMetricsRequest) {
ExecutionContext executionContext = createExecutionContext(getSolutionMetricsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSolutionMetricsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSolutionMetricsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSolutionMetrics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSolutionMetricsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of the batch inference jobs that have been performed off of a solution version.
*
*
* @param listBatchInferenceJobsRequest
* @return Result of the ListBatchInferenceJobs operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListBatchInferenceJobs
* @see AWS API Documentation
*/
@Override
public ListBatchInferenceJobsResult listBatchInferenceJobs(ListBatchInferenceJobsRequest request) {
request = beforeClientExecution(request);
return executeListBatchInferenceJobs(request);
}
@SdkInternalApi
final ListBatchInferenceJobsResult executeListBatchInferenceJobs(ListBatchInferenceJobsRequest listBatchInferenceJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listBatchInferenceJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBatchInferenceJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listBatchInferenceJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBatchInferenceJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListBatchInferenceJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of the batch segment jobs that have been performed off of a solution version that you specify.
*
*
* @param listBatchSegmentJobsRequest
* @return Result of the ListBatchSegmentJobs operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListBatchSegmentJobs
* @see AWS API Documentation
*/
@Override
public ListBatchSegmentJobsResult listBatchSegmentJobs(ListBatchSegmentJobsRequest request) {
request = beforeClientExecution(request);
return executeListBatchSegmentJobs(request);
}
@SdkInternalApi
final ListBatchSegmentJobsResult executeListBatchSegmentJobs(ListBatchSegmentJobsRequest listBatchSegmentJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listBatchSegmentJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBatchSegmentJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listBatchSegmentJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBatchSegmentJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListBatchSegmentJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of campaigns that use the given solution. When a solution is not specified, all the campaigns
* associated with the account are listed. The response provides the properties for each campaign, including the
* Amazon Resource Name (ARN). For more information on campaigns, see CreateCampaign.
*
*
* @param listCampaignsRequest
* @return Result of the ListCampaigns operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListCampaigns
* @see AWS API
* Documentation
*/
@Override
public ListCampaignsResult listCampaigns(ListCampaignsRequest request) {
request = beforeClientExecution(request);
return executeListCampaigns(request);
}
@SdkInternalApi
final ListCampaignsResult executeListCampaigns(ListCampaignsRequest listCampaignsRequest) {
ExecutionContext executionContext = createExecutionContext(listCampaignsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCampaignsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listCampaignsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCampaigns");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListCampaignsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of data deletion jobs for a dataset group ordered by creation time, with the most recent first.
* When a dataset group is not specified, all the data deletion jobs associated with the account are listed. The
* response provides the properties for each job, including the Amazon Resource Name (ARN). For more information on
* data deletion jobs, see Deleting
* users.
*
*
* @param listDataDeletionJobsRequest
* @return Result of the ListDataDeletionJobs operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListDataDeletionJobs
* @see AWS API Documentation
*/
@Override
public ListDataDeletionJobsResult listDataDeletionJobs(ListDataDeletionJobsRequest request) {
request = beforeClientExecution(request);
return executeListDataDeletionJobs(request);
}
@SdkInternalApi
final ListDataDeletionJobsResult executeListDataDeletionJobs(ListDataDeletionJobsRequest listDataDeletionJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listDataDeletionJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDataDeletionJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDataDeletionJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDataDeletionJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDataDeletionJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of dataset export jobs that use the given dataset. When a dataset is not specified, all the
* dataset export jobs associated with the account are listed. The response provides the properties for each dataset
* export job, including the Amazon Resource Name (ARN). For more information on dataset export jobs, see CreateDatasetExportJob. For more information on datasets, see CreateDataset.
*
*
* @param listDatasetExportJobsRequest
* @return Result of the ListDatasetExportJobs operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListDatasetExportJobs
* @see AWS API Documentation
*/
@Override
public ListDatasetExportJobsResult listDatasetExportJobs(ListDatasetExportJobsRequest request) {
request = beforeClientExecution(request);
return executeListDatasetExportJobs(request);
}
@SdkInternalApi
final ListDatasetExportJobsResult executeListDatasetExportJobs(ListDatasetExportJobsRequest listDatasetExportJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listDatasetExportJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDatasetExportJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDatasetExportJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDatasetExportJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListDatasetExportJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of dataset groups. The response provides the properties for each dataset group, including the
* Amazon Resource Name (ARN). For more information on dataset groups, see CreateDatasetGroup.
*
*
* @param listDatasetGroupsRequest
* @return Result of the ListDatasetGroups operation returned by the service.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListDatasetGroups
* @see AWS
* API Documentation
*/
@Override
public ListDatasetGroupsResult listDatasetGroups(ListDatasetGroupsRequest request) {
request = beforeClientExecution(request);
return executeListDatasetGroups(request);
}
@SdkInternalApi
final ListDatasetGroupsResult executeListDatasetGroups(ListDatasetGroupsRequest listDatasetGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listDatasetGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDatasetGroupsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDatasetGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDatasetGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDatasetGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of dataset import jobs that use the given dataset. When a dataset is not specified, all the
* dataset import jobs associated with the account are listed. The response provides the properties for each dataset
* import job, including the Amazon Resource Name (ARN). For more information on dataset import jobs, see CreateDatasetImportJob. For more information on datasets, see CreateDataset.
*
*
* @param listDatasetImportJobsRequest
* @return Result of the ListDatasetImportJobs operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListDatasetImportJobs
* @see AWS API Documentation
*/
@Override
public ListDatasetImportJobsResult listDatasetImportJobs(ListDatasetImportJobsRequest request) {
request = beforeClientExecution(request);
return executeListDatasetImportJobs(request);
}
@SdkInternalApi
final ListDatasetImportJobsResult executeListDatasetImportJobs(ListDatasetImportJobsRequest listDatasetImportJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listDatasetImportJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDatasetImportJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDatasetImportJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDatasetImportJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListDatasetImportJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the list of datasets contained in the given dataset group. The response provides the properties for each
* dataset, including the Amazon Resource Name (ARN). For more information on datasets, see CreateDataset.
*
*
* @param listDatasetsRequest
* @return Result of the ListDatasets operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListDatasets
* @see AWS API
* Documentation
*/
@Override
public ListDatasetsResult listDatasets(ListDatasetsRequest request) {
request = beforeClientExecution(request);
return executeListDatasets(request);
}
@SdkInternalApi
final ListDatasetsResult executeListDatasets(ListDatasetsRequest listDatasetsRequest) {
ExecutionContext executionContext = createExecutionContext(listDatasetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDatasetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDatasetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDatasets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDatasetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the list of event trackers associated with the account. The response provides the properties for each
* event tracker, including the Amazon Resource Name (ARN) and tracking ID. For more information on event trackers,
* see CreateEventTracker.
*
*
* @param listEventTrackersRequest
* @return Result of the ListEventTrackers operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListEventTrackers
* @see AWS
* API Documentation
*/
@Override
public ListEventTrackersResult listEventTrackers(ListEventTrackersRequest request) {
request = beforeClientExecution(request);
return executeListEventTrackers(request);
}
@SdkInternalApi
final ListEventTrackersResult executeListEventTrackers(ListEventTrackersRequest listEventTrackersRequest) {
ExecutionContext executionContext = createExecutionContext(listEventTrackersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEventTrackersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEventTrackersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEventTrackers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListEventTrackersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all filters that belong to a given dataset group.
*
*
* @param listFiltersRequest
* @return Result of the ListFilters operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListFilters
* @see AWS API
* Documentation
*/
@Override
public ListFiltersResult listFilters(ListFiltersRequest request) {
request = beforeClientExecution(request);
return executeListFilters(request);
}
@SdkInternalApi
final ListFiltersResult executeListFilters(ListFiltersRequest listFiltersRequest) {
ExecutionContext executionContext = createExecutionContext(listFiltersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFiltersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFiltersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFilters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFiltersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the metrics for the metric attribution.
*
*
* @param listMetricAttributionMetricsRequest
* @return Result of the ListMetricAttributionMetrics operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListMetricAttributionMetrics
* @see AWS API Documentation
*/
@Override
public ListMetricAttributionMetricsResult listMetricAttributionMetrics(ListMetricAttributionMetricsRequest request) {
request = beforeClientExecution(request);
return executeListMetricAttributionMetrics(request);
}
@SdkInternalApi
final ListMetricAttributionMetricsResult executeListMetricAttributionMetrics(ListMetricAttributionMetricsRequest listMetricAttributionMetricsRequest) {
ExecutionContext executionContext = createExecutionContext(listMetricAttributionMetricsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMetricAttributionMetricsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listMetricAttributionMetricsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMetricAttributionMetrics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListMetricAttributionMetricsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists metric attributions.
*
*
* @param listMetricAttributionsRequest
* @return Result of the ListMetricAttributions operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListMetricAttributions
* @see AWS API Documentation
*/
@Override
public ListMetricAttributionsResult listMetricAttributions(ListMetricAttributionsRequest request) {
request = beforeClientExecution(request);
return executeListMetricAttributions(request);
}
@SdkInternalApi
final ListMetricAttributionsResult executeListMetricAttributions(ListMetricAttributionsRequest listMetricAttributionsRequest) {
ExecutionContext executionContext = createExecutionContext(listMetricAttributionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMetricAttributionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listMetricAttributionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMetricAttributions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListMetricAttributionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of available recipes. The response provides the properties for each recipe, including the recipe's
* Amazon Resource Name (ARN).
*
*
* @param listRecipesRequest
* @return Result of the ListRecipes operation returned by the service.
* @throws InvalidNextTokenException
* The token is not valid.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @sample AmazonPersonalize.ListRecipes
* @see AWS API
* Documentation
*/
@Override
public ListRecipesResult listRecipes(ListRecipesRequest request) {
request = beforeClientExecution(request);
return executeListRecipes(request);
}
@SdkInternalApi
final ListRecipesResult executeListRecipes(ListRecipesRequest listRecipesRequest) {
ExecutionContext executionContext = createExecutionContext(listRecipesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRecipesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRecipesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRecipes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListRecipesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of recommenders in a given Domain dataset group. When a Domain dataset group is not specified, all
* the recommenders associated with the account are listed. The response provides the properties for each
* recommender, including the Amazon Resource Name (ARN). For more information on recommenders, see CreateRecommender.
*
*
* @param listRecommendersRequest
* @return Result of the ListRecommenders operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListRecommenders
* @see AWS
* API Documentation
*/
@Override
public ListRecommendersResult listRecommenders(ListRecommendersRequest request) {
request = beforeClientExecution(request);
return executeListRecommenders(request);
}
@SdkInternalApi
final ListRecommendersResult executeListRecommenders(ListRecommendersRequest listRecommendersRequest) {
ExecutionContext executionContext = createExecutionContext(listRecommendersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRecommendersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRecommendersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRecommenders");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListRecommendersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the list of schemas associated with the account. The response provides the properties for each schema,
* including the Amazon Resource Name (ARN). For more information on schemas, see CreateSchema.
*
*
* @param listSchemasRequest
* @return Result of the ListSchemas operation returned by the service.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListSchemas
* @see AWS API
* Documentation
*/
@Override
public ListSchemasResult listSchemas(ListSchemasRequest request) {
request = beforeClientExecution(request);
return executeListSchemas(request);
}
@SdkInternalApi
final ListSchemasResult executeListSchemas(ListSchemasRequest listSchemasRequest) {
ExecutionContext executionContext = createExecutionContext(listSchemasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSchemasRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSchemasRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSchemas");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSchemasResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of solution versions for the given solution. When a solution is not specified, all the solution
* versions associated with the account are listed. The response provides the properties for each solution version,
* including the Amazon Resource Name (ARN).
*
*
* @param listSolutionVersionsRequest
* @return Result of the ListSolutionVersions operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListSolutionVersions
* @see AWS API Documentation
*/
@Override
public ListSolutionVersionsResult listSolutionVersions(ListSolutionVersionsRequest request) {
request = beforeClientExecution(request);
return executeListSolutionVersions(request);
}
@SdkInternalApi
final ListSolutionVersionsResult executeListSolutionVersions(ListSolutionVersionsRequest listSolutionVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(listSolutionVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSolutionVersionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSolutionVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSolutionVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSolutionVersionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of solutions in a given dataset group. When a dataset group is not specified, all the solutions
* associated with the account are listed. The response provides the properties for each solution, including the
* Amazon Resource Name (ARN). For more information on solutions, see CreateSolution.
*
*
* @param listSolutionsRequest
* @return Result of the ListSolutions operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws InvalidNextTokenException
* The token is not valid.
* @sample AmazonPersonalize.ListSolutions
* @see AWS API
* Documentation
*/
@Override
public ListSolutionsResult listSolutions(ListSolutionsRequest request) {
request = beforeClientExecution(request);
return executeListSolutions(request);
}
@SdkInternalApi
final ListSolutionsResult executeListSolutions(ListSolutionsRequest listSolutionsRequest) {
ExecutionContext executionContext = createExecutionContext(listSolutionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSolutionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSolutionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSolutions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSolutionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get a list of tags
* attached to a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a recommender that is INACTIVE. Starting a recommender does not create any new models, but resumes billing
* and automatic retraining for the recommender.
*
*
* @param startRecommenderRequest
* @return Result of the StartRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.StartRecommender
* @see AWS
* API Documentation
*/
@Override
public StartRecommenderResult startRecommender(StartRecommenderRequest request) {
request = beforeClientExecution(request);
return executeStartRecommender(request);
}
@SdkInternalApi
final StartRecommenderResult executeStartRecommender(StartRecommenderRequest startRecommenderRequest) {
ExecutionContext executionContext = createExecutionContext(startRecommenderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartRecommenderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startRecommenderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartRecommender");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartRecommenderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops a recommender that is ACTIVE. Stopping a recommender halts billing and automatic retraining for the
* recommender.
*
*
* @param stopRecommenderRequest
* @return Result of the StopRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.StopRecommender
* @see AWS
* API Documentation
*/
@Override
public StopRecommenderResult stopRecommender(StopRecommenderRequest request) {
request = beforeClientExecution(request);
return executeStopRecommender(request);
}
@SdkInternalApi
final StopRecommenderResult executeStopRecommender(StopRecommenderRequest stopRecommenderRequest) {
ExecutionContext executionContext = createExecutionContext(stopRecommenderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopRecommenderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopRecommenderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopRecommender");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StopRecommenderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops creating a solution version that is in a state of CREATE_PENDING or CREATE IN_PROGRESS.
*
*
* Depending on the current state of the solution version, the solution version state changes as follows:
*
*
* -
*
* CREATE_PENDING > CREATE_STOPPED
*
*
* or
*
*
* -
*
* CREATE_IN_PROGRESS > CREATE_STOPPING > CREATE_STOPPED
*
*
*
*
* You are billed for all of the training completed up until you stop the solution version creation. You cannot
* resume creating a solution version once it has been stopped.
*
*
* @param stopSolutionVersionCreationRequest
* @return Result of the StopSolutionVersionCreation operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.StopSolutionVersionCreation
* @see AWS API Documentation
*/
@Override
public StopSolutionVersionCreationResult stopSolutionVersionCreation(StopSolutionVersionCreationRequest request) {
request = beforeClientExecution(request);
return executeStopSolutionVersionCreation(request);
}
@SdkInternalApi
final StopSolutionVersionCreationResult executeStopSolutionVersionCreation(StopSolutionVersionCreationRequest stopSolutionVersionCreationRequest) {
ExecutionContext executionContext = createExecutionContext(stopSolutionVersionCreationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopSolutionVersionCreationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(stopSolutionVersionCreationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopSolutionVersionCreation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopSolutionVersionCreationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Add a list of tags to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws TooManyTagsException
* You have exceeded the maximum number of tags you can apply to this resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws LimitExceededException
* The limit on the number of requests per second has been exceeded.
* @sample AmazonPersonalize.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified tags that are attached to a resource. For more information, see Removing tags from Amazon Personalize
* resources.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws TooManyTagKeysException
* The request contains more tag keys than can be associated with a resource (50 tag keys per resource).
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a campaign to deploy a retrained solution version with an existing campaign, change your campaign's
* minProvisionedTPS
, or modify your campaign's configuration. For example, you can set
* enableMetadataWithRecommendations
to true for an existing campaign.
*
*
* To update a campaign to start automatically using the latest solution version, specify the following:
*
*
* -
*
* For the SolutionVersionArn
parameter, specify the Amazon Resource Name (ARN) of your solution in
* SolutionArn/$LATEST
format.
*
*
* -
*
* In the campaignConfig
, set syncWithLatestSolutionVersion
to true
.
*
*
*
*
* To update a campaign, the campaign status must be ACTIVE or CREATE FAILED. Check the campaign status using the DescribeCampaign
* operation.
*
*
*
* You can still get recommendations from a campaign while an update is in progress. The campaign will use the
* previous solution version and campaign configuration to generate recommendations until the latest campaign update
* status is Active
.
*
*
*
* For more information about updating a campaign, including code samples, see Updating a campaign. For more
* information about campaigns, see Creating a campaign.
*
*
* @param updateCampaignRequest
* @return Result of the UpdateCampaign operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.UpdateCampaign
* @see AWS API
* Documentation
*/
@Override
public UpdateCampaignResult updateCampaign(UpdateCampaignRequest request) {
request = beforeClientExecution(request);
return executeUpdateCampaign(request);
}
@SdkInternalApi
final UpdateCampaignResult executeUpdateCampaign(UpdateCampaignRequest updateCampaignRequest) {
ExecutionContext executionContext = createExecutionContext(updateCampaignRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateCampaignRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateCampaignRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateCampaign");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateCampaignResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update a dataset to replace its schema with a new or existing one. For more information, see Replacing a dataset's
* schema.
*
*
* @param updateDatasetRequest
* @return Result of the UpdateDataset operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.UpdateDataset
* @see AWS API
* Documentation
*/
@Override
public UpdateDatasetResult updateDataset(UpdateDatasetRequest request) {
request = beforeClientExecution(request);
return executeUpdateDataset(request);
}
@SdkInternalApi
final UpdateDatasetResult executeUpdateDataset(UpdateDatasetRequest updateDatasetRequest) {
ExecutionContext executionContext = createExecutionContext(updateDatasetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDatasetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateDatasetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDataset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateDatasetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a metric attribution.
*
*
* @param updateMetricAttributionRequest
* @return Result of the UpdateMetricAttribution operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @sample AmazonPersonalize.UpdateMetricAttribution
* @see AWS API Documentation
*/
@Override
public UpdateMetricAttributionResult updateMetricAttribution(UpdateMetricAttributionRequest request) {
request = beforeClientExecution(request);
return executeUpdateMetricAttribution(request);
}
@SdkInternalApi
final UpdateMetricAttributionResult executeUpdateMetricAttribution(UpdateMetricAttributionRequest updateMetricAttributionRequest) {
ExecutionContext executionContext = createExecutionContext(updateMetricAttributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMetricAttributionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateMetricAttributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateMetricAttribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateMetricAttributionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the recommender to modify the recommender configuration. If you update the recommender to modify the
* columns used in training, Amazon Personalize automatically starts a full retraining of the models backing your
* recommender. While the update completes, you can still get recommendations from the recommender. The recommender
* uses the previous configuration until the update completes. To track the status of this update, use the
* latestRecommenderUpdate
returned in the DescribeRecommender
* operation.
*
*
* @param updateRecommenderRequest
* @return Result of the UpdateRecommender operation returned by the service.
* @throws InvalidInputException
* Provide a valid value for the field or parameter.
* @throws ResourceNotFoundException
* Could not find the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @sample AmazonPersonalize.UpdateRecommender
* @see AWS
* API Documentation
*/
@Override
public UpdateRecommenderResult updateRecommender(UpdateRecommenderRequest request) {
request = beforeClientExecution(request);
return executeUpdateRecommender(request);
}
@SdkInternalApi
final UpdateRecommenderResult executeUpdateRecommender(UpdateRecommenderRequest updateRecommenderRequest) {
ExecutionContext executionContext = createExecutionContext(updateRecommenderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateRecommenderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateRecommenderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Personalize");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateRecommender");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateRecommenderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}