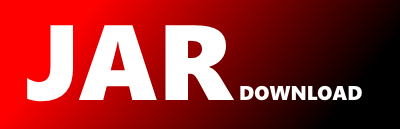
com.amazonaws.services.personalize.model.CreateDatasetImportJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-personalize Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.personalize.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateDatasetImportJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name for the dataset import job.
*
*/
private String jobName;
/**
*
* The ARN of the dataset that receives the imported data.
*
*/
private String datasetArn;
/**
*
* The Amazon S3 bucket that contains the training data to import.
*
*/
private DataSource dataSource;
/**
*
* The ARN of the IAM role that has permissions to read from the Amazon S3 data source.
*
*/
private String roleArn;
/**
*
* A list of tags to apply to
* the dataset import job.
*
*/
private java.util.List tags;
/**
*
* Specify how to add the new records to an existing dataset. The default import mode is FULL
. If you
* haven't imported bulk records into the dataset previously, you can only specify FULL
.
*
*
* -
*
* Specify FULL
to overwrite all existing bulk data in your dataset. Data you imported individually is
* not replaced.
*
*
* -
*
* Specify INCREMENTAL
to append the new records to the existing data in your dataset. Amazon
* Personalize replaces any record with the same ID with the new one.
*
*
*
*/
private String importMode;
/**
*
* If you created a metric attribution, specify whether to publish metrics for this import job to Amazon S3
*
*/
private Boolean publishAttributionMetricsToS3;
/**
*
* The name for the dataset import job.
*
*
* @param jobName
* The name for the dataset import job.
*/
public void setJobName(String jobName) {
this.jobName = jobName;
}
/**
*
* The name for the dataset import job.
*
*
* @return The name for the dataset import job.
*/
public String getJobName() {
return this.jobName;
}
/**
*
* The name for the dataset import job.
*
*
* @param jobName
* The name for the dataset import job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetImportJobRequest withJobName(String jobName) {
setJobName(jobName);
return this;
}
/**
*
* The ARN of the dataset that receives the imported data.
*
*
* @param datasetArn
* The ARN of the dataset that receives the imported data.
*/
public void setDatasetArn(String datasetArn) {
this.datasetArn = datasetArn;
}
/**
*
* The ARN of the dataset that receives the imported data.
*
*
* @return The ARN of the dataset that receives the imported data.
*/
public String getDatasetArn() {
return this.datasetArn;
}
/**
*
* The ARN of the dataset that receives the imported data.
*
*
* @param datasetArn
* The ARN of the dataset that receives the imported data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetImportJobRequest withDatasetArn(String datasetArn) {
setDatasetArn(datasetArn);
return this;
}
/**
*
* The Amazon S3 bucket that contains the training data to import.
*
*
* @param dataSource
* The Amazon S3 bucket that contains the training data to import.
*/
public void setDataSource(DataSource dataSource) {
this.dataSource = dataSource;
}
/**
*
* The Amazon S3 bucket that contains the training data to import.
*
*
* @return The Amazon S3 bucket that contains the training data to import.
*/
public DataSource getDataSource() {
return this.dataSource;
}
/**
*
* The Amazon S3 bucket that contains the training data to import.
*
*
* @param dataSource
* The Amazon S3 bucket that contains the training data to import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetImportJobRequest withDataSource(DataSource dataSource) {
setDataSource(dataSource);
return this;
}
/**
*
* The ARN of the IAM role that has permissions to read from the Amazon S3 data source.
*
*
* @param roleArn
* The ARN of the IAM role that has permissions to read from the Amazon S3 data source.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The ARN of the IAM role that has permissions to read from the Amazon S3 data source.
*
*
* @return The ARN of the IAM role that has permissions to read from the Amazon S3 data source.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The ARN of the IAM role that has permissions to read from the Amazon S3 data source.
*
*
* @param roleArn
* The ARN of the IAM role that has permissions to read from the Amazon S3 data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetImportJobRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* A list of tags to apply to
* the dataset import job.
*
*
* @return A list of tags to
* apply to the dataset import job.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of tags to apply to
* the dataset import job.
*
*
* @param tags
* A list of tags to
* apply to the dataset import job.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of tags to apply to
* the dataset import job.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags to
* apply to the dataset import job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetImportJobRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags to apply to
* the dataset import job.
*
*
* @param tags
* A list of tags to
* apply to the dataset import job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetImportJobRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Specify how to add the new records to an existing dataset. The default import mode is FULL
. If you
* haven't imported bulk records into the dataset previously, you can only specify FULL
.
*
*
* -
*
* Specify FULL
to overwrite all existing bulk data in your dataset. Data you imported individually is
* not replaced.
*
*
* -
*
* Specify INCREMENTAL
to append the new records to the existing data in your dataset. Amazon
* Personalize replaces any record with the same ID with the new one.
*
*
*
*
* @param importMode
* Specify how to add the new records to an existing dataset. The default import mode is FULL
.
* If you haven't imported bulk records into the dataset previously, you can only specify FULL
* .
*
* -
*
* Specify FULL
to overwrite all existing bulk data in your dataset. Data you imported
* individually is not replaced.
*
*
* -
*
* Specify INCREMENTAL
to append the new records to the existing data in your dataset. Amazon
* Personalize replaces any record with the same ID with the new one.
*
*
* @see ImportMode
*/
public void setImportMode(String importMode) {
this.importMode = importMode;
}
/**
*
* Specify how to add the new records to an existing dataset. The default import mode is FULL
. If you
* haven't imported bulk records into the dataset previously, you can only specify FULL
.
*
*
* -
*
* Specify FULL
to overwrite all existing bulk data in your dataset. Data you imported individually is
* not replaced.
*
*
* -
*
* Specify INCREMENTAL
to append the new records to the existing data in your dataset. Amazon
* Personalize replaces any record with the same ID with the new one.
*
*
*
*
* @return Specify how to add the new records to an existing dataset. The default import mode is FULL
.
* If you haven't imported bulk records into the dataset previously, you can only specify FULL
* .
*
* -
*
* Specify FULL
to overwrite all existing bulk data in your dataset. Data you imported
* individually is not replaced.
*
*
* -
*
* Specify INCREMENTAL
to append the new records to the existing data in your dataset. Amazon
* Personalize replaces any record with the same ID with the new one.
*
*
* @see ImportMode
*/
public String getImportMode() {
return this.importMode;
}
/**
*
* Specify how to add the new records to an existing dataset. The default import mode is FULL
. If you
* haven't imported bulk records into the dataset previously, you can only specify FULL
.
*
*
* -
*
* Specify FULL
to overwrite all existing bulk data in your dataset. Data you imported individually is
* not replaced.
*
*
* -
*
* Specify INCREMENTAL
to append the new records to the existing data in your dataset. Amazon
* Personalize replaces any record with the same ID with the new one.
*
*
*
*
* @param importMode
* Specify how to add the new records to an existing dataset. The default import mode is FULL
.
* If you haven't imported bulk records into the dataset previously, you can only specify FULL
* .
*
* -
*
* Specify FULL
to overwrite all existing bulk data in your dataset. Data you imported
* individually is not replaced.
*
*
* -
*
* Specify INCREMENTAL
to append the new records to the existing data in your dataset. Amazon
* Personalize replaces any record with the same ID with the new one.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ImportMode
*/
public CreateDatasetImportJobRequest withImportMode(String importMode) {
setImportMode(importMode);
return this;
}
/**
*
* Specify how to add the new records to an existing dataset. The default import mode is FULL
. If you
* haven't imported bulk records into the dataset previously, you can only specify FULL
.
*
*
* -
*
* Specify FULL
to overwrite all existing bulk data in your dataset. Data you imported individually is
* not replaced.
*
*
* -
*
* Specify INCREMENTAL
to append the new records to the existing data in your dataset. Amazon
* Personalize replaces any record with the same ID with the new one.
*
*
*
*
* @param importMode
* Specify how to add the new records to an existing dataset. The default import mode is FULL
.
* If you haven't imported bulk records into the dataset previously, you can only specify FULL
* .
*
* -
*
* Specify FULL
to overwrite all existing bulk data in your dataset. Data you imported
* individually is not replaced.
*
*
* -
*
* Specify INCREMENTAL
to append the new records to the existing data in your dataset. Amazon
* Personalize replaces any record with the same ID with the new one.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ImportMode
*/
public CreateDatasetImportJobRequest withImportMode(ImportMode importMode) {
this.importMode = importMode.toString();
return this;
}
/**
*
* If you created a metric attribution, specify whether to publish metrics for this import job to Amazon S3
*
*
* @param publishAttributionMetricsToS3
* If you created a metric attribution, specify whether to publish metrics for this import job to Amazon S3
*/
public void setPublishAttributionMetricsToS3(Boolean publishAttributionMetricsToS3) {
this.publishAttributionMetricsToS3 = publishAttributionMetricsToS3;
}
/**
*
* If you created a metric attribution, specify whether to publish metrics for this import job to Amazon S3
*
*
* @return If you created a metric attribution, specify whether to publish metrics for this import job to Amazon S3
*/
public Boolean getPublishAttributionMetricsToS3() {
return this.publishAttributionMetricsToS3;
}
/**
*
* If you created a metric attribution, specify whether to publish metrics for this import job to Amazon S3
*
*
* @param publishAttributionMetricsToS3
* If you created a metric attribution, specify whether to publish metrics for this import job to Amazon S3
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetImportJobRequest withPublishAttributionMetricsToS3(Boolean publishAttributionMetricsToS3) {
setPublishAttributionMetricsToS3(publishAttributionMetricsToS3);
return this;
}
/**
*
* If you created a metric attribution, specify whether to publish metrics for this import job to Amazon S3
*
*
* @return If you created a metric attribution, specify whether to publish metrics for this import job to Amazon S3
*/
public Boolean isPublishAttributionMetricsToS3() {
return this.publishAttributionMetricsToS3;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getJobName() != null)
sb.append("JobName: ").append(getJobName()).append(",");
if (getDatasetArn() != null)
sb.append("DatasetArn: ").append(getDatasetArn()).append(",");
if (getDataSource() != null)
sb.append("DataSource: ").append(getDataSource()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getImportMode() != null)
sb.append("ImportMode: ").append(getImportMode()).append(",");
if (getPublishAttributionMetricsToS3() != null)
sb.append("PublishAttributionMetricsToS3: ").append(getPublishAttributionMetricsToS3());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDatasetImportJobRequest == false)
return false;
CreateDatasetImportJobRequest other = (CreateDatasetImportJobRequest) obj;
if (other.getJobName() == null ^ this.getJobName() == null)
return false;
if (other.getJobName() != null && other.getJobName().equals(this.getJobName()) == false)
return false;
if (other.getDatasetArn() == null ^ this.getDatasetArn() == null)
return false;
if (other.getDatasetArn() != null && other.getDatasetArn().equals(this.getDatasetArn()) == false)
return false;
if (other.getDataSource() == null ^ this.getDataSource() == null)
return false;
if (other.getDataSource() != null && other.getDataSource().equals(this.getDataSource()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getImportMode() == null ^ this.getImportMode() == null)
return false;
if (other.getImportMode() != null && other.getImportMode().equals(this.getImportMode()) == false)
return false;
if (other.getPublishAttributionMetricsToS3() == null ^ this.getPublishAttributionMetricsToS3() == null)
return false;
if (other.getPublishAttributionMetricsToS3() != null
&& other.getPublishAttributionMetricsToS3().equals(this.getPublishAttributionMetricsToS3()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getJobName() == null) ? 0 : getJobName().hashCode());
hashCode = prime * hashCode + ((getDatasetArn() == null) ? 0 : getDatasetArn().hashCode());
hashCode = prime * hashCode + ((getDataSource() == null) ? 0 : getDataSource().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getImportMode() == null) ? 0 : getImportMode().hashCode());
hashCode = prime * hashCode + ((getPublishAttributionMetricsToS3() == null) ? 0 : getPublishAttributionMetricsToS3().hashCode());
return hashCode;
}
@Override
public CreateDatasetImportJobRequest clone() {
return (CreateDatasetImportJobRequest) super.clone();
}
}