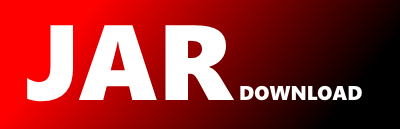
com.amazonaws.services.personalizeevents.model.ActionInteraction Maven / Gradle / Ivy
Show all versions of aws-java-sdk-personalizeevents Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.personalizeevents.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Represents an action interaction event sent using the PutActionInteractions
API.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ActionInteraction implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the action the user interacted with. This corresponds to the ACTION_ID
field of the Action
* interaction schema.
*
*/
private String actionId;
/**
*
* The ID of the user who interacted with the action. This corresponds to the USER_ID
field of the
* Action interaction schema.
*
*/
private String userId;
/**
*
* The ID associated with the user's visit. Your application generates a unique sessionId
when a user
* first visits your website or uses your application.
*
*/
private String sessionId;
/**
*
* The timestamp for when the action interaction event occurred. Timestamps must be in Unix epoch time format, in
* seconds.
*
*/
private java.util.Date timestamp;
/**
*
* The type of action interaction event. You can specify Viewed
, Taken
, and
* Not Taken
event types. For more information about action interaction event type data, see Event type
* data.
*
*/
private String eventType;
/**
*
* An ID associated with the event. If an event ID is not provided, Amazon Personalize generates a unique ID for the
* event. An event ID is not used as an input to the model. Amazon Personalize uses the event ID to distinguish
* unique events. Any subsequent events after the first with the same event ID are not used in model training.
*
*/
private String eventId;
/**
*
* The ID of the list of recommendations that contains the action the user interacted with.
*
*/
private String recommendationId;
/**
*
* A list of action IDs that represents the sequence of actions you have shown the user. For example,
* ["actionId1", "actionId2", "actionId3"]
. Amazon Personalize doesn't use impressions data from action
* interaction events. Instead, record multiple events for each action and use the Viewed
event type.
*
*/
private java.util.List impression;
/**
*
* A string map of event-specific data that you might choose to record. For example, if a user takes an action,
* other than the action ID, you might also send the number of actions taken by the user.
*
*
* Each item in the map consists of a key-value pair. For example,
*
*
* {"numberOfActions": "12"}
*
*
* The keys use camel case names that match the fields in the Action interactions schema. In the above example, the
* numberOfActions
would match the 'NUMBER_OF_ACTIONS' field defined in the Action interactions schema.
*
*
* The following can't be included as a keyword for properties (case insensitive).
*
*
* -
*
* userId
*
*
* -
*
* sessionId
*
*
* -
*
* eventType
*
*
* -
*
* timestamp
*
*
* -
*
* recommendationId
*
*
* -
*
* impression
*
*
*
*/
private String properties;
/**
*
* The ID of the action the user interacted with. This corresponds to the ACTION_ID
field of the Action
* interaction schema.
*
*
* @param actionId
* The ID of the action the user interacted with. This corresponds to the ACTION_ID
field of the
* Action interaction schema.
*/
public void setActionId(String actionId) {
this.actionId = actionId;
}
/**
*
* The ID of the action the user interacted with. This corresponds to the ACTION_ID
field of the Action
* interaction schema.
*
*
* @return The ID of the action the user interacted with. This corresponds to the ACTION_ID
field of
* the Action interaction schema.
*/
public String getActionId() {
return this.actionId;
}
/**
*
* The ID of the action the user interacted with. This corresponds to the ACTION_ID
field of the Action
* interaction schema.
*
*
* @param actionId
* The ID of the action the user interacted with. This corresponds to the ACTION_ID
field of the
* Action interaction schema.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withActionId(String actionId) {
setActionId(actionId);
return this;
}
/**
*
* The ID of the user who interacted with the action. This corresponds to the USER_ID
field of the
* Action interaction schema.
*
*
* @param userId
* The ID of the user who interacted with the action. This corresponds to the USER_ID
field of
* the Action interaction schema.
*/
public void setUserId(String userId) {
this.userId = userId;
}
/**
*
* The ID of the user who interacted with the action. This corresponds to the USER_ID
field of the
* Action interaction schema.
*
*
* @return The ID of the user who interacted with the action. This corresponds to the USER_ID
field of
* the Action interaction schema.
*/
public String getUserId() {
return this.userId;
}
/**
*
* The ID of the user who interacted with the action. This corresponds to the USER_ID
field of the
* Action interaction schema.
*
*
* @param userId
* The ID of the user who interacted with the action. This corresponds to the USER_ID
field of
* the Action interaction schema.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withUserId(String userId) {
setUserId(userId);
return this;
}
/**
*
* The ID associated with the user's visit. Your application generates a unique sessionId
when a user
* first visits your website or uses your application.
*
*
* @param sessionId
* The ID associated with the user's visit. Your application generates a unique sessionId
when a
* user first visits your website or uses your application.
*/
public void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
/**
*
* The ID associated with the user's visit. Your application generates a unique sessionId
when a user
* first visits your website or uses your application.
*
*
* @return The ID associated with the user's visit. Your application generates a unique sessionId
when
* a user first visits your website or uses your application.
*/
public String getSessionId() {
return this.sessionId;
}
/**
*
* The ID associated with the user's visit. Your application generates a unique sessionId
when a user
* first visits your website or uses your application.
*
*
* @param sessionId
* The ID associated with the user's visit. Your application generates a unique sessionId
when a
* user first visits your website or uses your application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withSessionId(String sessionId) {
setSessionId(sessionId);
return this;
}
/**
*
* The timestamp for when the action interaction event occurred. Timestamps must be in Unix epoch time format, in
* seconds.
*
*
* @param timestamp
* The timestamp for when the action interaction event occurred. Timestamps must be in Unix epoch time
* format, in seconds.
*/
public void setTimestamp(java.util.Date timestamp) {
this.timestamp = timestamp;
}
/**
*
* The timestamp for when the action interaction event occurred. Timestamps must be in Unix epoch time format, in
* seconds.
*
*
* @return The timestamp for when the action interaction event occurred. Timestamps must be in Unix epoch time
* format, in seconds.
*/
public java.util.Date getTimestamp() {
return this.timestamp;
}
/**
*
* The timestamp for when the action interaction event occurred. Timestamps must be in Unix epoch time format, in
* seconds.
*
*
* @param timestamp
* The timestamp for when the action interaction event occurred. Timestamps must be in Unix epoch time
* format, in seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withTimestamp(java.util.Date timestamp) {
setTimestamp(timestamp);
return this;
}
/**
*
* The type of action interaction event. You can specify Viewed
, Taken
, and
* Not Taken
event types. For more information about action interaction event type data, see Event type
* data.
*
*
* @param eventType
* The type of action interaction event. You can specify Viewed
, Taken
, and
* Not Taken
event types. For more information about action interaction event type data, see Event
* type data.
*/
public void setEventType(String eventType) {
this.eventType = eventType;
}
/**
*
* The type of action interaction event. You can specify Viewed
, Taken
, and
* Not Taken
event types. For more information about action interaction event type data, see Event type
* data.
*
*
* @return The type of action interaction event. You can specify Viewed
, Taken
, and
* Not Taken
event types. For more information about action interaction event type data, see Event
* type data.
*/
public String getEventType() {
return this.eventType;
}
/**
*
* The type of action interaction event. You can specify Viewed
, Taken
, and
* Not Taken
event types. For more information about action interaction event type data, see Event type
* data.
*
*
* @param eventType
* The type of action interaction event. You can specify Viewed
, Taken
, and
* Not Taken
event types. For more information about action interaction event type data, see Event
* type data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withEventType(String eventType) {
setEventType(eventType);
return this;
}
/**
*
* An ID associated with the event. If an event ID is not provided, Amazon Personalize generates a unique ID for the
* event. An event ID is not used as an input to the model. Amazon Personalize uses the event ID to distinguish
* unique events. Any subsequent events after the first with the same event ID are not used in model training.
*
*
* @param eventId
* An ID associated with the event. If an event ID is not provided, Amazon Personalize generates a unique ID
* for the event. An event ID is not used as an input to the model. Amazon Personalize uses the event ID to
* distinguish unique events. Any subsequent events after the first with the same event ID are not used in
* model training.
*/
public void setEventId(String eventId) {
this.eventId = eventId;
}
/**
*
* An ID associated with the event. If an event ID is not provided, Amazon Personalize generates a unique ID for the
* event. An event ID is not used as an input to the model. Amazon Personalize uses the event ID to distinguish
* unique events. Any subsequent events after the first with the same event ID are not used in model training.
*
*
* @return An ID associated with the event. If an event ID is not provided, Amazon Personalize generates a unique ID
* for the event. An event ID is not used as an input to the model. Amazon Personalize uses the event ID to
* distinguish unique events. Any subsequent events after the first with the same event ID are not used in
* model training.
*/
public String getEventId() {
return this.eventId;
}
/**
*
* An ID associated with the event. If an event ID is not provided, Amazon Personalize generates a unique ID for the
* event. An event ID is not used as an input to the model. Amazon Personalize uses the event ID to distinguish
* unique events. Any subsequent events after the first with the same event ID are not used in model training.
*
*
* @param eventId
* An ID associated with the event. If an event ID is not provided, Amazon Personalize generates a unique ID
* for the event. An event ID is not used as an input to the model. Amazon Personalize uses the event ID to
* distinguish unique events. Any subsequent events after the first with the same event ID are not used in
* model training.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withEventId(String eventId) {
setEventId(eventId);
return this;
}
/**
*
* The ID of the list of recommendations that contains the action the user interacted with.
*
*
* @param recommendationId
* The ID of the list of recommendations that contains the action the user interacted with.
*/
public void setRecommendationId(String recommendationId) {
this.recommendationId = recommendationId;
}
/**
*
* The ID of the list of recommendations that contains the action the user interacted with.
*
*
* @return The ID of the list of recommendations that contains the action the user interacted with.
*/
public String getRecommendationId() {
return this.recommendationId;
}
/**
*
* The ID of the list of recommendations that contains the action the user interacted with.
*
*
* @param recommendationId
* The ID of the list of recommendations that contains the action the user interacted with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withRecommendationId(String recommendationId) {
setRecommendationId(recommendationId);
return this;
}
/**
*
* A list of action IDs that represents the sequence of actions you have shown the user. For example,
* ["actionId1", "actionId2", "actionId3"]
. Amazon Personalize doesn't use impressions data from action
* interaction events. Instead, record multiple events for each action and use the Viewed
event type.
*
*
* @return A list of action IDs that represents the sequence of actions you have shown the user. For example,
* ["actionId1", "actionId2", "actionId3"]
. Amazon Personalize doesn't use impressions data
* from action interaction events. Instead, record multiple events for each action and use the
* Viewed
event type.
*/
public java.util.List getImpression() {
return impression;
}
/**
*
* A list of action IDs that represents the sequence of actions you have shown the user. For example,
* ["actionId1", "actionId2", "actionId3"]
. Amazon Personalize doesn't use impressions data from action
* interaction events. Instead, record multiple events for each action and use the Viewed
event type.
*
*
* @param impression
* A list of action IDs that represents the sequence of actions you have shown the user. For example,
* ["actionId1", "actionId2", "actionId3"]
. Amazon Personalize doesn't use impressions data from
* action interaction events. Instead, record multiple events for each action and use the Viewed
* event type.
*/
public void setImpression(java.util.Collection impression) {
if (impression == null) {
this.impression = null;
return;
}
this.impression = new java.util.ArrayList(impression);
}
/**
*
* A list of action IDs that represents the sequence of actions you have shown the user. For example,
* ["actionId1", "actionId2", "actionId3"]
. Amazon Personalize doesn't use impressions data from action
* interaction events. Instead, record multiple events for each action and use the Viewed
event type.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setImpression(java.util.Collection)} or {@link #withImpression(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param impression
* A list of action IDs that represents the sequence of actions you have shown the user. For example,
* ["actionId1", "actionId2", "actionId3"]
. Amazon Personalize doesn't use impressions data from
* action interaction events. Instead, record multiple events for each action and use the Viewed
* event type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withImpression(String... impression) {
if (this.impression == null) {
setImpression(new java.util.ArrayList(impression.length));
}
for (String ele : impression) {
this.impression.add(ele);
}
return this;
}
/**
*
* A list of action IDs that represents the sequence of actions you have shown the user. For example,
* ["actionId1", "actionId2", "actionId3"]
. Amazon Personalize doesn't use impressions data from action
* interaction events. Instead, record multiple events for each action and use the Viewed
event type.
*
*
* @param impression
* A list of action IDs that represents the sequence of actions you have shown the user. For example,
* ["actionId1", "actionId2", "actionId3"]
. Amazon Personalize doesn't use impressions data from
* action interaction events. Instead, record multiple events for each action and use the Viewed
* event type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withImpression(java.util.Collection impression) {
setImpression(impression);
return this;
}
/**
*
* A string map of event-specific data that you might choose to record. For example, if a user takes an action,
* other than the action ID, you might also send the number of actions taken by the user.
*
*
* Each item in the map consists of a key-value pair. For example,
*
*
* {"numberOfActions": "12"}
*
*
* The keys use camel case names that match the fields in the Action interactions schema. In the above example, the
* numberOfActions
would match the 'NUMBER_OF_ACTIONS' field defined in the Action interactions schema.
*
*
* The following can't be included as a keyword for properties (case insensitive).
*
*
* -
*
* userId
*
*
* -
*
* sessionId
*
*
* -
*
* eventType
*
*
* -
*
* timestamp
*
*
* -
*
* recommendationId
*
*
* -
*
* impression
*
*
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param properties
* A string map of event-specific data that you might choose to record. For example, if a user takes an
* action, other than the action ID, you might also send the number of actions taken by the user.
*
* Each item in the map consists of a key-value pair. For example,
*
*
* {"numberOfActions": "12"}
*
*
* The keys use camel case names that match the fields in the Action interactions schema. In the above
* example, the numberOfActions
would match the 'NUMBER_OF_ACTIONS' field defined in the Action
* interactions schema.
*
*
* The following can't be included as a keyword for properties (case insensitive).
*
*
* -
*
* userId
*
*
* -
*
* sessionId
*
*
* -
*
* eventType
*
*
* -
*
* timestamp
*
*
* -
*
* recommendationId
*
*
* -
*
* impression
*
*
*/
public void setProperties(String properties) {
this.properties = properties;
}
/**
*
* A string map of event-specific data that you might choose to record. For example, if a user takes an action,
* other than the action ID, you might also send the number of actions taken by the user.
*
*
* Each item in the map consists of a key-value pair. For example,
*
*
* {"numberOfActions": "12"}
*
*
* The keys use camel case names that match the fields in the Action interactions schema. In the above example, the
* numberOfActions
would match the 'NUMBER_OF_ACTIONS' field defined in the Action interactions schema.
*
*
* The following can't be included as a keyword for properties (case insensitive).
*
*
* -
*
* userId
*
*
* -
*
* sessionId
*
*
* -
*
* eventType
*
*
* -
*
* timestamp
*
*
* -
*
* recommendationId
*
*
* -
*
* impression
*
*
*
*
* This field's value will be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* @return A string map of event-specific data that you might choose to record. For example, if a user takes an
* action, other than the action ID, you might also send the number of actions taken by the user.
*
* Each item in the map consists of a key-value pair. For example,
*
*
* {"numberOfActions": "12"}
*
*
* The keys use camel case names that match the fields in the Action interactions schema. In the above
* example, the numberOfActions
would match the 'NUMBER_OF_ACTIONS' field defined in the Action
* interactions schema.
*
*
* The following can't be included as a keyword for properties (case insensitive).
*
*
* -
*
* userId
*
*
* -
*
* sessionId
*
*
* -
*
* eventType
*
*
* -
*
* timestamp
*
*
* -
*
* recommendationId
*
*
* -
*
* impression
*
*
*/
public String getProperties() {
return this.properties;
}
/**
*
* A string map of event-specific data that you might choose to record. For example, if a user takes an action,
* other than the action ID, you might also send the number of actions taken by the user.
*
*
* Each item in the map consists of a key-value pair. For example,
*
*
* {"numberOfActions": "12"}
*
*
* The keys use camel case names that match the fields in the Action interactions schema. In the above example, the
* numberOfActions
would match the 'NUMBER_OF_ACTIONS' field defined in the Action interactions schema.
*
*
* The following can't be included as a keyword for properties (case insensitive).
*
*
* -
*
* userId
*
*
* -
*
* sessionId
*
*
* -
*
* eventType
*
*
* -
*
* timestamp
*
*
* -
*
* recommendationId
*
*
* -
*
* impression
*
*
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param properties
* A string map of event-specific data that you might choose to record. For example, if a user takes an
* action, other than the action ID, you might also send the number of actions taken by the user.
*
* Each item in the map consists of a key-value pair. For example,
*
*
* {"numberOfActions": "12"}
*
*
* The keys use camel case names that match the fields in the Action interactions schema. In the above
* example, the numberOfActions
would match the 'NUMBER_OF_ACTIONS' field defined in the Action
* interactions schema.
*
*
* The following can't be included as a keyword for properties (case insensitive).
*
*
* -
*
* userId
*
*
* -
*
* sessionId
*
*
* -
*
* eventType
*
*
* -
*
* timestamp
*
*
* -
*
* recommendationId
*
*
* -
*
* impression
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ActionInteraction withProperties(String properties) {
setProperties(properties);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getActionId() != null)
sb.append("ActionId: ").append("***Sensitive Data Redacted***").append(",");
if (getUserId() != null)
sb.append("UserId: ").append("***Sensitive Data Redacted***").append(",");
if (getSessionId() != null)
sb.append("SessionId: ").append(getSessionId()).append(",");
if (getTimestamp() != null)
sb.append("Timestamp: ").append(getTimestamp()).append(",");
if (getEventType() != null)
sb.append("EventType: ").append(getEventType()).append(",");
if (getEventId() != null)
sb.append("EventId: ").append(getEventId()).append(",");
if (getRecommendationId() != null)
sb.append("RecommendationId: ").append(getRecommendationId()).append(",");
if (getImpression() != null)
sb.append("Impression: ").append("***Sensitive Data Redacted***").append(",");
if (getProperties() != null)
sb.append("Properties: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ActionInteraction == false)
return false;
ActionInteraction other = (ActionInteraction) obj;
if (other.getActionId() == null ^ this.getActionId() == null)
return false;
if (other.getActionId() != null && other.getActionId().equals(this.getActionId()) == false)
return false;
if (other.getUserId() == null ^ this.getUserId() == null)
return false;
if (other.getUserId() != null && other.getUserId().equals(this.getUserId()) == false)
return false;
if (other.getSessionId() == null ^ this.getSessionId() == null)
return false;
if (other.getSessionId() != null && other.getSessionId().equals(this.getSessionId()) == false)
return false;
if (other.getTimestamp() == null ^ this.getTimestamp() == null)
return false;
if (other.getTimestamp() != null && other.getTimestamp().equals(this.getTimestamp()) == false)
return false;
if (other.getEventType() == null ^ this.getEventType() == null)
return false;
if (other.getEventType() != null && other.getEventType().equals(this.getEventType()) == false)
return false;
if (other.getEventId() == null ^ this.getEventId() == null)
return false;
if (other.getEventId() != null && other.getEventId().equals(this.getEventId()) == false)
return false;
if (other.getRecommendationId() == null ^ this.getRecommendationId() == null)
return false;
if (other.getRecommendationId() != null && other.getRecommendationId().equals(this.getRecommendationId()) == false)
return false;
if (other.getImpression() == null ^ this.getImpression() == null)
return false;
if (other.getImpression() != null && other.getImpression().equals(this.getImpression()) == false)
return false;
if (other.getProperties() == null ^ this.getProperties() == null)
return false;
if (other.getProperties() != null && other.getProperties().equals(this.getProperties()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getActionId() == null) ? 0 : getActionId().hashCode());
hashCode = prime * hashCode + ((getUserId() == null) ? 0 : getUserId().hashCode());
hashCode = prime * hashCode + ((getSessionId() == null) ? 0 : getSessionId().hashCode());
hashCode = prime * hashCode + ((getTimestamp() == null) ? 0 : getTimestamp().hashCode());
hashCode = prime * hashCode + ((getEventType() == null) ? 0 : getEventType().hashCode());
hashCode = prime * hashCode + ((getEventId() == null) ? 0 : getEventId().hashCode());
hashCode = prime * hashCode + ((getRecommendationId() == null) ? 0 : getRecommendationId().hashCode());
hashCode = prime * hashCode + ((getImpression() == null) ? 0 : getImpression().hashCode());
hashCode = prime * hashCode + ((getProperties() == null) ? 0 : getProperties().hashCode());
return hashCode;
}
@Override
public ActionInteraction clone() {
try {
return (ActionInteraction) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.personalizeevents.model.transform.ActionInteractionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}