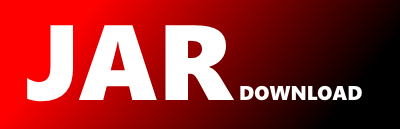
com.amazonaws.services.pi.model.GetResourceMetricsResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pi Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pi.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetResourceMetricsResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The start time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedStartTime
will be less than or equal to the value of the
* user-specified StartTime
.
*
*/
private java.util.Date alignedStartTime;
/**
*
* The end time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedEndTime
will be greater than or equal to the value of the
* user-specified Endtime
.
*
*/
private java.util.Date alignedEndTime;
/**
*
* An immutable identifier for a data source that is unique for an Amazon Web Services Region. Performance Insights
* gathers metrics from this data source. In the console, the identifier is shown as ResourceID. When you
* call DescribeDBInstances
, the identifier is returned as DbiResourceId
.
*
*/
private String identifier;
/**
*
* An array of metric results, where each array element contains all of the data points for a particular dimension.
*
*/
private java.util.List metricList;
/**
*
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*
*/
private String nextToken;
/**
*
* The start time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedStartTime
will be less than or equal to the value of the
* user-specified StartTime
.
*
*
* @param alignedStartTime
* The start time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedStartTime
will be less than or equal to the value of
* the user-specified StartTime
.
*/
public void setAlignedStartTime(java.util.Date alignedStartTime) {
this.alignedStartTime = alignedStartTime;
}
/**
*
* The start time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedStartTime
will be less than or equal to the value of the
* user-specified StartTime
.
*
*
* @return The start time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedStartTime
will be less than or equal to the value of
* the user-specified StartTime
.
*/
public java.util.Date getAlignedStartTime() {
return this.alignedStartTime;
}
/**
*
* The start time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedStartTime
will be less than or equal to the value of the
* user-specified StartTime
.
*
*
* @param alignedStartTime
* The start time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedStartTime
will be less than or equal to the value of
* the user-specified StartTime
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceMetricsResult withAlignedStartTime(java.util.Date alignedStartTime) {
setAlignedStartTime(alignedStartTime);
return this;
}
/**
*
* The end time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedEndTime
will be greater than or equal to the value of the
* user-specified Endtime
.
*
*
* @param alignedEndTime
* The end time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedEndTime
will be greater than or equal to the value of
* the user-specified Endtime
.
*/
public void setAlignedEndTime(java.util.Date alignedEndTime) {
this.alignedEndTime = alignedEndTime;
}
/**
*
* The end time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedEndTime
will be greater than or equal to the value of the
* user-specified Endtime
.
*
*
* @return The end time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedEndTime
will be greater than or equal to the value of
* the user-specified Endtime
.
*/
public java.util.Date getAlignedEndTime() {
return this.alignedEndTime;
}
/**
*
* The end time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedEndTime
will be greater than or equal to the value of the
* user-specified Endtime
.
*
*
* @param alignedEndTime
* The end time for the returned metrics, after alignment to a granular boundary (as specified by
* PeriodInSeconds
). AlignedEndTime
will be greater than or equal to the value of
* the user-specified Endtime
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceMetricsResult withAlignedEndTime(java.util.Date alignedEndTime) {
setAlignedEndTime(alignedEndTime);
return this;
}
/**
*
* An immutable identifier for a data source that is unique for an Amazon Web Services Region. Performance Insights
* gathers metrics from this data source. In the console, the identifier is shown as ResourceID. When you
* call DescribeDBInstances
, the identifier is returned as DbiResourceId
.
*
*
* @param identifier
* An immutable identifier for a data source that is unique for an Amazon Web Services Region. Performance
* Insights gathers metrics from this data source. In the console, the identifier is shown as
* ResourceID. When you call DescribeDBInstances
, the identifier is returned as
* DbiResourceId
.
*/
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
/**
*
* An immutable identifier for a data source that is unique for an Amazon Web Services Region. Performance Insights
* gathers metrics from this data source. In the console, the identifier is shown as ResourceID. When you
* call DescribeDBInstances
, the identifier is returned as DbiResourceId
.
*
*
* @return An immutable identifier for a data source that is unique for an Amazon Web Services Region. Performance
* Insights gathers metrics from this data source. In the console, the identifier is shown as
* ResourceID. When you call DescribeDBInstances
, the identifier is returned as
* DbiResourceId
.
*/
public String getIdentifier() {
return this.identifier;
}
/**
*
* An immutable identifier for a data source that is unique for an Amazon Web Services Region. Performance Insights
* gathers metrics from this data source. In the console, the identifier is shown as ResourceID. When you
* call DescribeDBInstances
, the identifier is returned as DbiResourceId
.
*
*
* @param identifier
* An immutable identifier for a data source that is unique for an Amazon Web Services Region. Performance
* Insights gathers metrics from this data source. In the console, the identifier is shown as
* ResourceID. When you call DescribeDBInstances
, the identifier is returned as
* DbiResourceId
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceMetricsResult withIdentifier(String identifier) {
setIdentifier(identifier);
return this;
}
/**
*
* An array of metric results, where each array element contains all of the data points for a particular dimension.
*
*
* @return An array of metric results, where each array element contains all of the data points for a particular
* dimension.
*/
public java.util.List getMetricList() {
return metricList;
}
/**
*
* An array of metric results, where each array element contains all of the data points for a particular dimension.
*
*
* @param metricList
* An array of metric results, where each array element contains all of the data points for a particular
* dimension.
*/
public void setMetricList(java.util.Collection metricList) {
if (metricList == null) {
this.metricList = null;
return;
}
this.metricList = new java.util.ArrayList(metricList);
}
/**
*
* An array of metric results, where each array element contains all of the data points for a particular dimension.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMetricList(java.util.Collection)} or {@link #withMetricList(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param metricList
* An array of metric results, where each array element contains all of the data points for a particular
* dimension.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceMetricsResult withMetricList(MetricKeyDataPoints... metricList) {
if (this.metricList == null) {
setMetricList(new java.util.ArrayList(metricList.length));
}
for (MetricKeyDataPoints ele : metricList) {
this.metricList.add(ele);
}
return this;
}
/**
*
* An array of metric results, where each array element contains all of the data points for a particular dimension.
*
*
* @param metricList
* An array of metric results, where each array element contains all of the data points for a particular
* dimension.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceMetricsResult withMetricList(java.util.Collection metricList) {
setMetricList(metricList);
return this;
}
/**
*
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*
*
* @param nextToken
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*
*
* @return An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*
*
* @param nextToken
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceMetricsResult withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAlignedStartTime() != null)
sb.append("AlignedStartTime: ").append(getAlignedStartTime()).append(",");
if (getAlignedEndTime() != null)
sb.append("AlignedEndTime: ").append(getAlignedEndTime()).append(",");
if (getIdentifier() != null)
sb.append("Identifier: ").append(getIdentifier()).append(",");
if (getMetricList() != null)
sb.append("MetricList: ").append(getMetricList()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetResourceMetricsResult == false)
return false;
GetResourceMetricsResult other = (GetResourceMetricsResult) obj;
if (other.getAlignedStartTime() == null ^ this.getAlignedStartTime() == null)
return false;
if (other.getAlignedStartTime() != null && other.getAlignedStartTime().equals(this.getAlignedStartTime()) == false)
return false;
if (other.getAlignedEndTime() == null ^ this.getAlignedEndTime() == null)
return false;
if (other.getAlignedEndTime() != null && other.getAlignedEndTime().equals(this.getAlignedEndTime()) == false)
return false;
if (other.getIdentifier() == null ^ this.getIdentifier() == null)
return false;
if (other.getIdentifier() != null && other.getIdentifier().equals(this.getIdentifier()) == false)
return false;
if (other.getMetricList() == null ^ this.getMetricList() == null)
return false;
if (other.getMetricList() != null && other.getMetricList().equals(this.getMetricList()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAlignedStartTime() == null) ? 0 : getAlignedStartTime().hashCode());
hashCode = prime * hashCode + ((getAlignedEndTime() == null) ? 0 : getAlignedEndTime().hashCode());
hashCode = prime * hashCode + ((getIdentifier() == null) ? 0 : getIdentifier().hashCode());
hashCode = prime * hashCode + ((getMetricList() == null) ? 0 : getMetricList().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
return hashCode;
}
@Override
public GetResourceMetricsResult clone() {
try {
return (GetResourceMetricsResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}