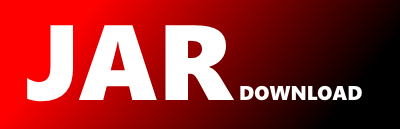
com.amazonaws.services.pi.AWSPIAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pi Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pi;
import javax.annotation.Generated;
import com.amazonaws.services.pi.model.*;
/**
* Interface for accessing AWS PI asynchronously. Each asynchronous method will return a Java Future object representing
* the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive notification when
* an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.pi.AbstractAWSPIAsync} instead.
*
*
* Amazon RDS Performance Insights
*
* Amazon RDS Performance Insights enables you to monitor and explore different dimensions of database load based on
* data captured from a running DB instance. The guide provides detailed information about Performance Insights data
* types, parameters and errors.
*
*
* When Performance Insights is enabled, the Amazon RDS Performance Insights API provides visibility into the
* performance of your DB instance. Amazon CloudWatch provides the authoritative source for Amazon Web Services
* service-vended monitoring metrics. Performance Insights offers a domain-specific view of DB load.
*
*
* DB load is measured as average active sessions. Performance Insights provides the data to API consumers as a
* two-dimensional time-series dataset. The time dimension provides DB load data for each time point in the queried time
* range. Each time point decomposes overall load in relation to the requested dimensions, measured at that time point.
* Examples include SQL, Wait event, User, and Host.
*
*
* -
*
* To learn more about Performance Insights and Amazon Aurora DB instances, go to the Amazon Aurora User
* Guide .
*
*
* -
*
* To learn more about Performance Insights and Amazon RDS DB instances, go to the Amazon RDS User Guide .
*
*
* -
*
* To learn more about Performance Insights and Amazon DocumentDB clusters, go to the Amazon DocumentDB
* Developer Guide .
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSPIAsync extends AWSPI {
/**
*
* Creates a new performance analysis report for a specific time period for the DB instance.
*
*
* @param createPerformanceAnalysisReportRequest
* @return A Java Future containing the result of the CreatePerformanceAnalysisReport operation returned by the
* service.
* @sample AWSPIAsync.CreatePerformanceAnalysisReport
* @see AWS API Documentation
*/
java.util.concurrent.Future createPerformanceAnalysisReportAsync(
CreatePerformanceAnalysisReportRequest createPerformanceAnalysisReportRequest);
/**
*
* Creates a new performance analysis report for a specific time period for the DB instance.
*
*
* @param createPerformanceAnalysisReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePerformanceAnalysisReport operation returned by the
* service.
* @sample AWSPIAsyncHandler.CreatePerformanceAnalysisReport
* @see AWS API Documentation
*/
java.util.concurrent.Future createPerformanceAnalysisReportAsync(
CreatePerformanceAnalysisReportRequest createPerformanceAnalysisReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a performance analysis report.
*
*
* @param deletePerformanceAnalysisReportRequest
* @return A Java Future containing the result of the DeletePerformanceAnalysisReport operation returned by the
* service.
* @sample AWSPIAsync.DeletePerformanceAnalysisReport
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePerformanceAnalysisReportAsync(
DeletePerformanceAnalysisReportRequest deletePerformanceAnalysisReportRequest);
/**
*
* Deletes a performance analysis report.
*
*
* @param deletePerformanceAnalysisReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePerformanceAnalysisReport operation returned by the
* service.
* @sample AWSPIAsyncHandler.DeletePerformanceAnalysisReport
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePerformanceAnalysisReportAsync(
DeletePerformanceAnalysisReportRequest deletePerformanceAnalysisReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* For a specific time period, retrieve the top N
dimension keys for a metric.
*
*
*
* Each response element returns a maximum of 500 bytes. For larger elements, such as SQL statements, only the first
* 500 bytes are returned.
*
*
*
* @param describeDimensionKeysRequest
* @return A Java Future containing the result of the DescribeDimensionKeys operation returned by the service.
* @sample AWSPIAsync.DescribeDimensionKeys
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDimensionKeysAsync(DescribeDimensionKeysRequest describeDimensionKeysRequest);
/**
*
* For a specific time period, retrieve the top N
dimension keys for a metric.
*
*
*
* Each response element returns a maximum of 500 bytes. For larger elements, such as SQL statements, only the first
* 500 bytes are returned.
*
*
*
* @param describeDimensionKeysRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDimensionKeys operation returned by the service.
* @sample AWSPIAsyncHandler.DescribeDimensionKeys
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDimensionKeysAsync(DescribeDimensionKeysRequest describeDimensionKeysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the attributes of the specified dimension group for a DB instance or data source. For example, if you specify
* a SQL ID, GetDimensionKeyDetails
retrieves the full text of the dimension
* db.sql.statement
associated with this ID. This operation is useful because
* GetResourceMetrics
and DescribeDimensionKeys
don't support retrieval of large SQL
* statement text.
*
*
* @param getDimensionKeyDetailsRequest
* @return A Java Future containing the result of the GetDimensionKeyDetails operation returned by the service.
* @sample AWSPIAsync.GetDimensionKeyDetails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDimensionKeyDetailsAsync(GetDimensionKeyDetailsRequest getDimensionKeyDetailsRequest);
/**
*
* Get the attributes of the specified dimension group for a DB instance or data source. For example, if you specify
* a SQL ID, GetDimensionKeyDetails
retrieves the full text of the dimension
* db.sql.statement
associated with this ID. This operation is useful because
* GetResourceMetrics
and DescribeDimensionKeys
don't support retrieval of large SQL
* statement text.
*
*
* @param getDimensionKeyDetailsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDimensionKeyDetails operation returned by the service.
* @sample AWSPIAsyncHandler.GetDimensionKeyDetails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDimensionKeyDetailsAsync(GetDimensionKeyDetailsRequest getDimensionKeyDetailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the report including the report ID, status, time details, and the insights with recommendations. The
* report status can be RUNNING
, SUCCEEDED
, or FAILED
. The insights include
* the description
and recommendation
fields.
*
*
* @param getPerformanceAnalysisReportRequest
* @return A Java Future containing the result of the GetPerformanceAnalysisReport operation returned by the
* service.
* @sample AWSPIAsync.GetPerformanceAnalysisReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getPerformanceAnalysisReportAsync(
GetPerformanceAnalysisReportRequest getPerformanceAnalysisReportRequest);
/**
*
* Retrieves the report including the report ID, status, time details, and the insights with recommendations. The
* report status can be RUNNING
, SUCCEEDED
, or FAILED
. The insights include
* the description
and recommendation
fields.
*
*
* @param getPerformanceAnalysisReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPerformanceAnalysisReport operation returned by the
* service.
* @sample AWSPIAsyncHandler.GetPerformanceAnalysisReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getPerformanceAnalysisReportAsync(
GetPerformanceAnalysisReportRequest getPerformanceAnalysisReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve the metadata for different features. For example, the metadata might indicate that a feature is turned
* on or off on a specific DB instance.
*
*
* @param getResourceMetadataRequest
* @return A Java Future containing the result of the GetResourceMetadata operation returned by the service.
* @sample AWSPIAsync.GetResourceMetadata
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResourceMetadataAsync(GetResourceMetadataRequest getResourceMetadataRequest);
/**
*
* Retrieve the metadata for different features. For example, the metadata might indicate that a feature is turned
* on or off on a specific DB instance.
*
*
* @param getResourceMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetResourceMetadata operation returned by the service.
* @sample AWSPIAsyncHandler.GetResourceMetadata
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResourceMetadataAsync(GetResourceMetadataRequest getResourceMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve Performance Insights metrics for a set of data sources over a time period. You can provide specific
* dimension groups and dimensions, and provide aggregation and filtering criteria for each group.
*
*
*
* Each response element returns a maximum of 500 bytes. For larger elements, such as SQL statements, only the first
* 500 bytes are returned.
*
*
*
* @param getResourceMetricsRequest
* @return A Java Future containing the result of the GetResourceMetrics operation returned by the service.
* @sample AWSPIAsync.GetResourceMetrics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResourceMetricsAsync(GetResourceMetricsRequest getResourceMetricsRequest);
/**
*
* Retrieve Performance Insights metrics for a set of data sources over a time period. You can provide specific
* dimension groups and dimensions, and provide aggregation and filtering criteria for each group.
*
*
*
* Each response element returns a maximum of 500 bytes. For larger elements, such as SQL statements, only the first
* 500 bytes are returned.
*
*
*
* @param getResourceMetricsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetResourceMetrics operation returned by the service.
* @sample AWSPIAsyncHandler.GetResourceMetrics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResourceMetricsAsync(GetResourceMetricsRequest getResourceMetricsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve the dimensions that can be queried for each specified metric type on a specified DB instance.
*
*
* @param listAvailableResourceDimensionsRequest
* @return A Java Future containing the result of the ListAvailableResourceDimensions operation returned by the
* service.
* @sample AWSPIAsync.ListAvailableResourceDimensions
* @see AWS API Documentation
*/
java.util.concurrent.Future listAvailableResourceDimensionsAsync(
ListAvailableResourceDimensionsRequest listAvailableResourceDimensionsRequest);
/**
*
* Retrieve the dimensions that can be queried for each specified metric type on a specified DB instance.
*
*
* @param listAvailableResourceDimensionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAvailableResourceDimensions operation returned by the
* service.
* @sample AWSPIAsyncHandler.ListAvailableResourceDimensions
* @see AWS API Documentation
*/
java.util.concurrent.Future listAvailableResourceDimensionsAsync(
ListAvailableResourceDimensionsRequest listAvailableResourceDimensionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve metrics of the specified types that can be queried for a specified DB instance.
*
*
* @param listAvailableResourceMetricsRequest
* @return A Java Future containing the result of the ListAvailableResourceMetrics operation returned by the
* service.
* @sample AWSPIAsync.ListAvailableResourceMetrics
* @see AWS API Documentation
*/
java.util.concurrent.Future listAvailableResourceMetricsAsync(
ListAvailableResourceMetricsRequest listAvailableResourceMetricsRequest);
/**
*
* Retrieve metrics of the specified types that can be queried for a specified DB instance.
*
*
* @param listAvailableResourceMetricsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAvailableResourceMetrics operation returned by the
* service.
* @sample AWSPIAsyncHandler.ListAvailableResourceMetrics
* @see AWS API Documentation
*/
java.util.concurrent.Future listAvailableResourceMetricsAsync(
ListAvailableResourceMetricsRequest listAvailableResourceMetricsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the analysis reports created for the DB instance. The reports are sorted based on the start time of
* each report.
*
*
* @param listPerformanceAnalysisReportsRequest
* @return A Java Future containing the result of the ListPerformanceAnalysisReports operation returned by the
* service.
* @sample AWSPIAsync.ListPerformanceAnalysisReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listPerformanceAnalysisReportsAsync(
ListPerformanceAnalysisReportsRequest listPerformanceAnalysisReportsRequest);
/**
*
* Lists all the analysis reports created for the DB instance. The reports are sorted based on the start time of
* each report.
*
*
* @param listPerformanceAnalysisReportsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPerformanceAnalysisReports operation returned by the
* service.
* @sample AWSPIAsyncHandler.ListPerformanceAnalysisReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listPerformanceAnalysisReportsAsync(
ListPerformanceAnalysisReportsRequest listPerformanceAnalysisReportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves all the metadata tags associated with Amazon RDS Performance Insights resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSPIAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves all the metadata tags associated with Amazon RDS Performance Insights resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSPIAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds metadata tags to the Amazon RDS Performance Insights resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSPIAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds metadata tags to the Amazon RDS Performance Insights resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSPIAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the metadata tags from the Amazon RDS Performance Insights resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSPIAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Deletes the metadata tags from the Amazon RDS Performance Insights resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSPIAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}