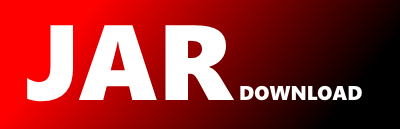
com.amazonaws.services.pi.model.DescribeDimensionKeysRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pi Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pi.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeDimensionKeysRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Web Services service for which Performance Insights will return metrics. Valid values are as follows:
*
*
* -
*
* RDS
*
*
* -
*
* DOCDB
*
*
*
*/
private String serviceType;
/**
*
* An immutable, Amazon Web Services Region-unique identifier for a data source. Performance Insights gathers
* metrics from this data source.
*
*
* To use an Amazon RDS instance as a data source, you specify its DbiResourceId
value. For example,
* specify db-FAIHNTYBKTGAUSUZQYPDS2GW4A
.
*
*/
private String identifier;
/**
*
* The date and time specifying the beginning of the requested time series data. You must specify a
* StartTime
within the past 7 days. The value specified is inclusive, which means that data
* points equal to or greater than StartTime
are returned.
*
*
* The value for StartTime
must be earlier than the value for EndTime
.
*
*/
private java.util.Date startTime;
/**
*
* The date and time specifying the end of the requested time series data. The value specified is exclusive,
* which means that data points less than (but not equal to) EndTime
are returned.
*
*
* The value for EndTime
must be later than the value for StartTime
.
*
*/
private java.util.Date endTime;
/**
*
* The name of a Performance Insights metric to be measured.
*
*
* Valid values for Metric
are:
*
*
* -
*
* db.load.avg
- A scaled representation of the number of active sessions for the database engine.
*
*
* -
*
* db.sampledload.avg
- The raw number of active sessions for the database engine.
*
*
*
*
* If the number of active sessions is less than an internal Performance Insights threshold,
* db.load.avg
and db.sampledload.avg
are the same value. If the number of active sessions
* is greater than the internal threshold, Performance Insights samples the active sessions, with
* db.load.avg
showing the scaled values, db.sampledload.avg
showing the raw values, and
* db.sampledload.avg
less than db.load.avg
. For most use cases, you can query
* db.load.avg
only.
*
*/
private String metric;
/**
*
* The granularity, in seconds, of the data points returned from Performance Insights. A period can be as short as
* one second, or as long as one day (86400 seconds). Valid values are:
*
*
* -
*
* 1
(one second)
*
*
* -
*
* 60
(one minute)
*
*
* -
*
* 300
(five minutes)
*
*
* -
*
* 3600
(one hour)
*
*
* -
*
* 86400
(twenty-four hours)
*
*
*
*
* If you don't specify PeriodInSeconds
, then Performance Insights chooses a value for you, with a goal
* of returning roughly 100-200 data points in the response.
*
*/
private Integer periodInSeconds;
/**
*
* A specification for how to aggregate the data points from a query result. You must specify a valid dimension
* group. Performance Insights returns all dimensions within this group, unless you provide the names of specific
* dimensions within this group. You can also request that Performance Insights return a limited number of values
* for a dimension.
*
*/
private DimensionGroup groupBy;
/**
*
* Additional metrics for the top N
dimension keys. If the specified dimension group in the
* GroupBy
parameter is db.sql_tokenized
, you can specify per-SQL metrics to get the
* values for the top N
SQL digests. The response syntax is as follows:
* "AdditionalMetrics" : { "string" : "string" }
.
*
*/
private java.util.List additionalMetrics;
/**
*
* For each dimension specified in GroupBy
, specify a secondary dimension to further subdivide the
* partition keys in the response.
*
*/
private DimensionGroup partitionBy;
/**
*
* One or more filters to apply in the request. Restrictions:
*
*
* -
*
* Any number of filters by the same dimension, as specified in the GroupBy
or Partition
* parameters.
*
*
* -
*
* A single filter for any other dimension in this dimension group.
*
*
*
*/
private java.util.Map filter;
/**
*
* The maximum number of items to return in the response. If more items exist than the specified
* MaxRecords
value, a pagination token is included in the response so that the remaining results can
* be retrieved.
*
*/
private Integer maxResults;
/**
*
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*
*/
private String nextToken;
/**
*
* The Amazon Web Services service for which Performance Insights will return metrics. Valid values are as follows:
*
*
* -
*
* RDS
*
*
* -
*
* DOCDB
*
*
*
*
* @param serviceType
* The Amazon Web Services service for which Performance Insights will return metrics. Valid values are as
* follows:
*
* -
*
* RDS
*
*
* -
*
* DOCDB
*
*
* @see ServiceType
*/
public void setServiceType(String serviceType) {
this.serviceType = serviceType;
}
/**
*
* The Amazon Web Services service for which Performance Insights will return metrics. Valid values are as follows:
*
*
* -
*
* RDS
*
*
* -
*
* DOCDB
*
*
*
*
* @return The Amazon Web Services service for which Performance Insights will return metrics. Valid values are as
* follows:
*
* -
*
* RDS
*
*
* -
*
* DOCDB
*
*
* @see ServiceType
*/
public String getServiceType() {
return this.serviceType;
}
/**
*
* The Amazon Web Services service for which Performance Insights will return metrics. Valid values are as follows:
*
*
* -
*
* RDS
*
*
* -
*
* DOCDB
*
*
*
*
* @param serviceType
* The Amazon Web Services service for which Performance Insights will return metrics. Valid values are as
* follows:
*
* -
*
* RDS
*
*
* -
*
* DOCDB
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceType
*/
public DescribeDimensionKeysRequest withServiceType(String serviceType) {
setServiceType(serviceType);
return this;
}
/**
*
* The Amazon Web Services service for which Performance Insights will return metrics. Valid values are as follows:
*
*
* -
*
* RDS
*
*
* -
*
* DOCDB
*
*
*
*
* @param serviceType
* The Amazon Web Services service for which Performance Insights will return metrics. Valid values are as
* follows:
*
* -
*
* RDS
*
*
* -
*
* DOCDB
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceType
*/
public DescribeDimensionKeysRequest withServiceType(ServiceType serviceType) {
this.serviceType = serviceType.toString();
return this;
}
/**
*
* An immutable, Amazon Web Services Region-unique identifier for a data source. Performance Insights gathers
* metrics from this data source.
*
*
* To use an Amazon RDS instance as a data source, you specify its DbiResourceId
value. For example,
* specify db-FAIHNTYBKTGAUSUZQYPDS2GW4A
.
*
*
* @param identifier
* An immutable, Amazon Web Services Region-unique identifier for a data source. Performance Insights gathers
* metrics from this data source.
*
* To use an Amazon RDS instance as a data source, you specify its DbiResourceId
value. For
* example, specify db-FAIHNTYBKTGAUSUZQYPDS2GW4A
.
*/
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
/**
*
* An immutable, Amazon Web Services Region-unique identifier for a data source. Performance Insights gathers
* metrics from this data source.
*
*
* To use an Amazon RDS instance as a data source, you specify its DbiResourceId
value. For example,
* specify db-FAIHNTYBKTGAUSUZQYPDS2GW4A
.
*
*
* @return An immutable, Amazon Web Services Region-unique identifier for a data source. Performance Insights
* gathers metrics from this data source.
*
* To use an Amazon RDS instance as a data source, you specify its DbiResourceId
value. For
* example, specify db-FAIHNTYBKTGAUSUZQYPDS2GW4A
.
*/
public String getIdentifier() {
return this.identifier;
}
/**
*
* An immutable, Amazon Web Services Region-unique identifier for a data source. Performance Insights gathers
* metrics from this data source.
*
*
* To use an Amazon RDS instance as a data source, you specify its DbiResourceId
value. For example,
* specify db-FAIHNTYBKTGAUSUZQYPDS2GW4A
.
*
*
* @param identifier
* An immutable, Amazon Web Services Region-unique identifier for a data source. Performance Insights gathers
* metrics from this data source.
*
* To use an Amazon RDS instance as a data source, you specify its DbiResourceId
value. For
* example, specify db-FAIHNTYBKTGAUSUZQYPDS2GW4A
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withIdentifier(String identifier) {
setIdentifier(identifier);
return this;
}
/**
*
* The date and time specifying the beginning of the requested time series data. You must specify a
* StartTime
within the past 7 days. The value specified is inclusive, which means that data
* points equal to or greater than StartTime
are returned.
*
*
* The value for StartTime
must be earlier than the value for EndTime
.
*
*
* @param startTime
* The date and time specifying the beginning of the requested time series data. You must specify a
* StartTime
within the past 7 days. The value specified is inclusive, which means that
* data points equal to or greater than StartTime
are returned.
*
* The value for StartTime
must be earlier than the value for EndTime
.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The date and time specifying the beginning of the requested time series data. You must specify a
* StartTime
within the past 7 days. The value specified is inclusive, which means that data
* points equal to or greater than StartTime
are returned.
*
*
* The value for StartTime
must be earlier than the value for EndTime
.
*
*
* @return The date and time specifying the beginning of the requested time series data. You must specify a
* StartTime
within the past 7 days. The value specified is inclusive, which means that
* data points equal to or greater than StartTime
are returned.
*
* The value for StartTime
must be earlier than the value for EndTime
.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The date and time specifying the beginning of the requested time series data. You must specify a
* StartTime
within the past 7 days. The value specified is inclusive, which means that data
* points equal to or greater than StartTime
are returned.
*
*
* The value for StartTime
must be earlier than the value for EndTime
.
*
*
* @param startTime
* The date and time specifying the beginning of the requested time series data. You must specify a
* StartTime
within the past 7 days. The value specified is inclusive, which means that
* data points equal to or greater than StartTime
are returned.
*
* The value for StartTime
must be earlier than the value for EndTime
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The date and time specifying the end of the requested time series data. The value specified is exclusive,
* which means that data points less than (but not equal to) EndTime
are returned.
*
*
* The value for EndTime
must be later than the value for StartTime
.
*
*
* @param endTime
* The date and time specifying the end of the requested time series data. The value specified is
* exclusive, which means that data points less than (but not equal to) EndTime
are
* returned.
*
* The value for EndTime
must be later than the value for StartTime
.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The date and time specifying the end of the requested time series data. The value specified is exclusive,
* which means that data points less than (but not equal to) EndTime
are returned.
*
*
* The value for EndTime
must be later than the value for StartTime
.
*
*
* @return The date and time specifying the end of the requested time series data. The value specified is
* exclusive, which means that data points less than (but not equal to) EndTime
are
* returned.
*
* The value for EndTime
must be later than the value for StartTime
.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The date and time specifying the end of the requested time series data. The value specified is exclusive,
* which means that data points less than (but not equal to) EndTime
are returned.
*
*
* The value for EndTime
must be later than the value for StartTime
.
*
*
* @param endTime
* The date and time specifying the end of the requested time series data. The value specified is
* exclusive, which means that data points less than (but not equal to) EndTime
are
* returned.
*
* The value for EndTime
must be later than the value for StartTime
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* The name of a Performance Insights metric to be measured.
*
*
* Valid values for Metric
are:
*
*
* -
*
* db.load.avg
- A scaled representation of the number of active sessions for the database engine.
*
*
* -
*
* db.sampledload.avg
- The raw number of active sessions for the database engine.
*
*
*
*
* If the number of active sessions is less than an internal Performance Insights threshold,
* db.load.avg
and db.sampledload.avg
are the same value. If the number of active sessions
* is greater than the internal threshold, Performance Insights samples the active sessions, with
* db.load.avg
showing the scaled values, db.sampledload.avg
showing the raw values, and
* db.sampledload.avg
less than db.load.avg
. For most use cases, you can query
* db.load.avg
only.
*
*
* @param metric
* The name of a Performance Insights metric to be measured.
*
* Valid values for Metric
are:
*
*
* -
*
* db.load.avg
- A scaled representation of the number of active sessions for the database
* engine.
*
*
* -
*
* db.sampledload.avg
- The raw number of active sessions for the database engine.
*
*
*
*
* If the number of active sessions is less than an internal Performance Insights threshold,
* db.load.avg
and db.sampledload.avg
are the same value. If the number of active
* sessions is greater than the internal threshold, Performance Insights samples the active sessions, with
* db.load.avg
showing the scaled values, db.sampledload.avg
showing the raw
* values, and db.sampledload.avg
less than db.load.avg
. For most use cases, you
* can query db.load.avg
only.
*/
public void setMetric(String metric) {
this.metric = metric;
}
/**
*
* The name of a Performance Insights metric to be measured.
*
*
* Valid values for Metric
are:
*
*
* -
*
* db.load.avg
- A scaled representation of the number of active sessions for the database engine.
*
*
* -
*
* db.sampledload.avg
- The raw number of active sessions for the database engine.
*
*
*
*
* If the number of active sessions is less than an internal Performance Insights threshold,
* db.load.avg
and db.sampledload.avg
are the same value. If the number of active sessions
* is greater than the internal threshold, Performance Insights samples the active sessions, with
* db.load.avg
showing the scaled values, db.sampledload.avg
showing the raw values, and
* db.sampledload.avg
less than db.load.avg
. For most use cases, you can query
* db.load.avg
only.
*
*
* @return The name of a Performance Insights metric to be measured.
*
* Valid values for Metric
are:
*
*
* -
*
* db.load.avg
- A scaled representation of the number of active sessions for the database
* engine.
*
*
* -
*
* db.sampledload.avg
- The raw number of active sessions for the database engine.
*
*
*
*
* If the number of active sessions is less than an internal Performance Insights threshold,
* db.load.avg
and db.sampledload.avg
are the same value. If the number of active
* sessions is greater than the internal threshold, Performance Insights samples the active sessions, with
* db.load.avg
showing the scaled values, db.sampledload.avg
showing the raw
* values, and db.sampledload.avg
less than db.load.avg
. For most use cases, you
* can query db.load.avg
only.
*/
public String getMetric() {
return this.metric;
}
/**
*
* The name of a Performance Insights metric to be measured.
*
*
* Valid values for Metric
are:
*
*
* -
*
* db.load.avg
- A scaled representation of the number of active sessions for the database engine.
*
*
* -
*
* db.sampledload.avg
- The raw number of active sessions for the database engine.
*
*
*
*
* If the number of active sessions is less than an internal Performance Insights threshold,
* db.load.avg
and db.sampledload.avg
are the same value. If the number of active sessions
* is greater than the internal threshold, Performance Insights samples the active sessions, with
* db.load.avg
showing the scaled values, db.sampledload.avg
showing the raw values, and
* db.sampledload.avg
less than db.load.avg
. For most use cases, you can query
* db.load.avg
only.
*
*
* @param metric
* The name of a Performance Insights metric to be measured.
*
* Valid values for Metric
are:
*
*
* -
*
* db.load.avg
- A scaled representation of the number of active sessions for the database
* engine.
*
*
* -
*
* db.sampledload.avg
- The raw number of active sessions for the database engine.
*
*
*
*
* If the number of active sessions is less than an internal Performance Insights threshold,
* db.load.avg
and db.sampledload.avg
are the same value. If the number of active
* sessions is greater than the internal threshold, Performance Insights samples the active sessions, with
* db.load.avg
showing the scaled values, db.sampledload.avg
showing the raw
* values, and db.sampledload.avg
less than db.load.avg
. For most use cases, you
* can query db.load.avg
only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withMetric(String metric) {
setMetric(metric);
return this;
}
/**
*
* The granularity, in seconds, of the data points returned from Performance Insights. A period can be as short as
* one second, or as long as one day (86400 seconds). Valid values are:
*
*
* -
*
* 1
(one second)
*
*
* -
*
* 60
(one minute)
*
*
* -
*
* 300
(five minutes)
*
*
* -
*
* 3600
(one hour)
*
*
* -
*
* 86400
(twenty-four hours)
*
*
*
*
* If you don't specify PeriodInSeconds
, then Performance Insights chooses a value for you, with a goal
* of returning roughly 100-200 data points in the response.
*
*
* @param periodInSeconds
* The granularity, in seconds, of the data points returned from Performance Insights. A period can be as
* short as one second, or as long as one day (86400 seconds). Valid values are:
*
* -
*
* 1
(one second)
*
*
* -
*
* 60
(one minute)
*
*
* -
*
* 300
(five minutes)
*
*
* -
*
* 3600
(one hour)
*
*
* -
*
* 86400
(twenty-four hours)
*
*
*
*
* If you don't specify PeriodInSeconds
, then Performance Insights chooses a value for you, with
* a goal of returning roughly 100-200 data points in the response.
*/
public void setPeriodInSeconds(Integer periodInSeconds) {
this.periodInSeconds = periodInSeconds;
}
/**
*
* The granularity, in seconds, of the data points returned from Performance Insights. A period can be as short as
* one second, or as long as one day (86400 seconds). Valid values are:
*
*
* -
*
* 1
(one second)
*
*
* -
*
* 60
(one minute)
*
*
* -
*
* 300
(five minutes)
*
*
* -
*
* 3600
(one hour)
*
*
* -
*
* 86400
(twenty-four hours)
*
*
*
*
* If you don't specify PeriodInSeconds
, then Performance Insights chooses a value for you, with a goal
* of returning roughly 100-200 data points in the response.
*
*
* @return The granularity, in seconds, of the data points returned from Performance Insights. A period can be as
* short as one second, or as long as one day (86400 seconds). Valid values are:
*
* -
*
* 1
(one second)
*
*
* -
*
* 60
(one minute)
*
*
* -
*
* 300
(five minutes)
*
*
* -
*
* 3600
(one hour)
*
*
* -
*
* 86400
(twenty-four hours)
*
*
*
*
* If you don't specify PeriodInSeconds
, then Performance Insights chooses a value for you,
* with a goal of returning roughly 100-200 data points in the response.
*/
public Integer getPeriodInSeconds() {
return this.periodInSeconds;
}
/**
*
* The granularity, in seconds, of the data points returned from Performance Insights. A period can be as short as
* one second, or as long as one day (86400 seconds). Valid values are:
*
*
* -
*
* 1
(one second)
*
*
* -
*
* 60
(one minute)
*
*
* -
*
* 300
(five minutes)
*
*
* -
*
* 3600
(one hour)
*
*
* -
*
* 86400
(twenty-four hours)
*
*
*
*
* If you don't specify PeriodInSeconds
, then Performance Insights chooses a value for you, with a goal
* of returning roughly 100-200 data points in the response.
*
*
* @param periodInSeconds
* The granularity, in seconds, of the data points returned from Performance Insights. A period can be as
* short as one second, or as long as one day (86400 seconds). Valid values are:
*
* -
*
* 1
(one second)
*
*
* -
*
* 60
(one minute)
*
*
* -
*
* 300
(five minutes)
*
*
* -
*
* 3600
(one hour)
*
*
* -
*
* 86400
(twenty-four hours)
*
*
*
*
* If you don't specify PeriodInSeconds
, then Performance Insights chooses a value for you, with
* a goal of returning roughly 100-200 data points in the response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withPeriodInSeconds(Integer periodInSeconds) {
setPeriodInSeconds(periodInSeconds);
return this;
}
/**
*
* A specification for how to aggregate the data points from a query result. You must specify a valid dimension
* group. Performance Insights returns all dimensions within this group, unless you provide the names of specific
* dimensions within this group. You can also request that Performance Insights return a limited number of values
* for a dimension.
*
*
* @param groupBy
* A specification for how to aggregate the data points from a query result. You must specify a valid
* dimension group. Performance Insights returns all dimensions within this group, unless you provide the
* names of specific dimensions within this group. You can also request that Performance Insights return a
* limited number of values for a dimension.
*/
public void setGroupBy(DimensionGroup groupBy) {
this.groupBy = groupBy;
}
/**
*
* A specification for how to aggregate the data points from a query result. You must specify a valid dimension
* group. Performance Insights returns all dimensions within this group, unless you provide the names of specific
* dimensions within this group. You can also request that Performance Insights return a limited number of values
* for a dimension.
*
*
* @return A specification for how to aggregate the data points from a query result. You must specify a valid
* dimension group. Performance Insights returns all dimensions within this group, unless you provide the
* names of specific dimensions within this group. You can also request that Performance Insights return a
* limited number of values for a dimension.
*/
public DimensionGroup getGroupBy() {
return this.groupBy;
}
/**
*
* A specification for how to aggregate the data points from a query result. You must specify a valid dimension
* group. Performance Insights returns all dimensions within this group, unless you provide the names of specific
* dimensions within this group. You can also request that Performance Insights return a limited number of values
* for a dimension.
*
*
* @param groupBy
* A specification for how to aggregate the data points from a query result. You must specify a valid
* dimension group. Performance Insights returns all dimensions within this group, unless you provide the
* names of specific dimensions within this group. You can also request that Performance Insights return a
* limited number of values for a dimension.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withGroupBy(DimensionGroup groupBy) {
setGroupBy(groupBy);
return this;
}
/**
*
* Additional metrics for the top N
dimension keys. If the specified dimension group in the
* GroupBy
parameter is db.sql_tokenized
, you can specify per-SQL metrics to get the
* values for the top N
SQL digests. The response syntax is as follows:
* "AdditionalMetrics" : { "string" : "string" }
.
*
*
* @return Additional metrics for the top N
dimension keys. If the specified dimension group in the
* GroupBy
parameter is db.sql_tokenized
, you can specify per-SQL metrics to get
* the values for the top N
SQL digests. The response syntax is as follows:
* "AdditionalMetrics" : { "string" : "string" }
.
*/
public java.util.List getAdditionalMetrics() {
return additionalMetrics;
}
/**
*
* Additional metrics for the top N
dimension keys. If the specified dimension group in the
* GroupBy
parameter is db.sql_tokenized
, you can specify per-SQL metrics to get the
* values for the top N
SQL digests. The response syntax is as follows:
* "AdditionalMetrics" : { "string" : "string" }
.
*
*
* @param additionalMetrics
* Additional metrics for the top N
dimension keys. If the specified dimension group in the
* GroupBy
parameter is db.sql_tokenized
, you can specify per-SQL metrics to get
* the values for the top N
SQL digests. The response syntax is as follows:
* "AdditionalMetrics" : { "string" : "string" }
.
*/
public void setAdditionalMetrics(java.util.Collection additionalMetrics) {
if (additionalMetrics == null) {
this.additionalMetrics = null;
return;
}
this.additionalMetrics = new java.util.ArrayList(additionalMetrics);
}
/**
*
* Additional metrics for the top N
dimension keys. If the specified dimension group in the
* GroupBy
parameter is db.sql_tokenized
, you can specify per-SQL metrics to get the
* values for the top N
SQL digests. The response syntax is as follows:
* "AdditionalMetrics" : { "string" : "string" }
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdditionalMetrics(java.util.Collection)} or {@link #withAdditionalMetrics(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param additionalMetrics
* Additional metrics for the top N
dimension keys. If the specified dimension group in the
* GroupBy
parameter is db.sql_tokenized
, you can specify per-SQL metrics to get
* the values for the top N
SQL digests. The response syntax is as follows:
* "AdditionalMetrics" : { "string" : "string" }
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withAdditionalMetrics(String... additionalMetrics) {
if (this.additionalMetrics == null) {
setAdditionalMetrics(new java.util.ArrayList(additionalMetrics.length));
}
for (String ele : additionalMetrics) {
this.additionalMetrics.add(ele);
}
return this;
}
/**
*
* Additional metrics for the top N
dimension keys. If the specified dimension group in the
* GroupBy
parameter is db.sql_tokenized
, you can specify per-SQL metrics to get the
* values for the top N
SQL digests. The response syntax is as follows:
* "AdditionalMetrics" : { "string" : "string" }
.
*
*
* @param additionalMetrics
* Additional metrics for the top N
dimension keys. If the specified dimension group in the
* GroupBy
parameter is db.sql_tokenized
, you can specify per-SQL metrics to get
* the values for the top N
SQL digests. The response syntax is as follows:
* "AdditionalMetrics" : { "string" : "string" }
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withAdditionalMetrics(java.util.Collection additionalMetrics) {
setAdditionalMetrics(additionalMetrics);
return this;
}
/**
*
* For each dimension specified in GroupBy
, specify a secondary dimension to further subdivide the
* partition keys in the response.
*
*
* @param partitionBy
* For each dimension specified in GroupBy
, specify a secondary dimension to further subdivide
* the partition keys in the response.
*/
public void setPartitionBy(DimensionGroup partitionBy) {
this.partitionBy = partitionBy;
}
/**
*
* For each dimension specified in GroupBy
, specify a secondary dimension to further subdivide the
* partition keys in the response.
*
*
* @return For each dimension specified in GroupBy
, specify a secondary dimension to further subdivide
* the partition keys in the response.
*/
public DimensionGroup getPartitionBy() {
return this.partitionBy;
}
/**
*
* For each dimension specified in GroupBy
, specify a secondary dimension to further subdivide the
* partition keys in the response.
*
*
* @param partitionBy
* For each dimension specified in GroupBy
, specify a secondary dimension to further subdivide
* the partition keys in the response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withPartitionBy(DimensionGroup partitionBy) {
setPartitionBy(partitionBy);
return this;
}
/**
*
* One or more filters to apply in the request. Restrictions:
*
*
* -
*
* Any number of filters by the same dimension, as specified in the GroupBy
or Partition
* parameters.
*
*
* -
*
* A single filter for any other dimension in this dimension group.
*
*
*
*
* @return One or more filters to apply in the request. Restrictions:
*
* -
*
* Any number of filters by the same dimension, as specified in the GroupBy
or
* Partition
parameters.
*
*
* -
*
* A single filter for any other dimension in this dimension group.
*
*
*/
public java.util.Map getFilter() {
return filter;
}
/**
*
* One or more filters to apply in the request. Restrictions:
*
*
* -
*
* Any number of filters by the same dimension, as specified in the GroupBy
or Partition
* parameters.
*
*
* -
*
* A single filter for any other dimension in this dimension group.
*
*
*
*
* @param filter
* One or more filters to apply in the request. Restrictions:
*
* -
*
* Any number of filters by the same dimension, as specified in the GroupBy
or
* Partition
parameters.
*
*
* -
*
* A single filter for any other dimension in this dimension group.
*
*
*/
public void setFilter(java.util.Map filter) {
this.filter = filter;
}
/**
*
* One or more filters to apply in the request. Restrictions:
*
*
* -
*
* Any number of filters by the same dimension, as specified in the GroupBy
or Partition
* parameters.
*
*
* -
*
* A single filter for any other dimension in this dimension group.
*
*
*
*
* @param filter
* One or more filters to apply in the request. Restrictions:
*
* -
*
* Any number of filters by the same dimension, as specified in the GroupBy
or
* Partition
parameters.
*
*
* -
*
* A single filter for any other dimension in this dimension group.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withFilter(java.util.Map filter) {
setFilter(filter);
return this;
}
/**
* Add a single Filter entry
*
* @see DescribeDimensionKeysRequest#withFilter
* @returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest addFilterEntry(String key, String value) {
if (null == this.filter) {
this.filter = new java.util.HashMap();
}
if (this.filter.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.filter.put(key, value);
return this;
}
/**
* Removes all the entries added into Filter.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest clearFilterEntries() {
this.filter = null;
return this;
}
/**
*
* The maximum number of items to return in the response. If more items exist than the specified
* MaxRecords
value, a pagination token is included in the response so that the remaining results can
* be retrieved.
*
*
* @param maxResults
* The maximum number of items to return in the response. If more items exist than the specified
* MaxRecords
value, a pagination token is included in the response so that the remaining
* results can be retrieved.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of items to return in the response. If more items exist than the specified
* MaxRecords
value, a pagination token is included in the response so that the remaining results can
* be retrieved.
*
*
* @return The maximum number of items to return in the response. If more items exist than the specified
* MaxRecords
value, a pagination token is included in the response so that the remaining
* results can be retrieved.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of items to return in the response. If more items exist than the specified
* MaxRecords
value, a pagination token is included in the response so that the remaining results can
* be retrieved.
*
*
* @param maxResults
* The maximum number of items to return in the response. If more items exist than the specified
* MaxRecords
value, a pagination token is included in the response so that the remaining
* results can be retrieved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*
*
* @param nextToken
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*
*
* @return An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
*
*
* @param nextToken
* An optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the token, up to the value specified by MaxRecords
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDimensionKeysRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServiceType() != null)
sb.append("ServiceType: ").append(getServiceType()).append(",");
if (getIdentifier() != null)
sb.append("Identifier: ").append(getIdentifier()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getMetric() != null)
sb.append("Metric: ").append(getMetric()).append(",");
if (getPeriodInSeconds() != null)
sb.append("PeriodInSeconds: ").append(getPeriodInSeconds()).append(",");
if (getGroupBy() != null)
sb.append("GroupBy: ").append(getGroupBy()).append(",");
if (getAdditionalMetrics() != null)
sb.append("AdditionalMetrics: ").append(getAdditionalMetrics()).append(",");
if (getPartitionBy() != null)
sb.append("PartitionBy: ").append(getPartitionBy()).append(",");
if (getFilter() != null)
sb.append("Filter: ").append(getFilter()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeDimensionKeysRequest == false)
return false;
DescribeDimensionKeysRequest other = (DescribeDimensionKeysRequest) obj;
if (other.getServiceType() == null ^ this.getServiceType() == null)
return false;
if (other.getServiceType() != null && other.getServiceType().equals(this.getServiceType()) == false)
return false;
if (other.getIdentifier() == null ^ this.getIdentifier() == null)
return false;
if (other.getIdentifier() != null && other.getIdentifier().equals(this.getIdentifier()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getMetric() == null ^ this.getMetric() == null)
return false;
if (other.getMetric() != null && other.getMetric().equals(this.getMetric()) == false)
return false;
if (other.getPeriodInSeconds() == null ^ this.getPeriodInSeconds() == null)
return false;
if (other.getPeriodInSeconds() != null && other.getPeriodInSeconds().equals(this.getPeriodInSeconds()) == false)
return false;
if (other.getGroupBy() == null ^ this.getGroupBy() == null)
return false;
if (other.getGroupBy() != null && other.getGroupBy().equals(this.getGroupBy()) == false)
return false;
if (other.getAdditionalMetrics() == null ^ this.getAdditionalMetrics() == null)
return false;
if (other.getAdditionalMetrics() != null && other.getAdditionalMetrics().equals(this.getAdditionalMetrics()) == false)
return false;
if (other.getPartitionBy() == null ^ this.getPartitionBy() == null)
return false;
if (other.getPartitionBy() != null && other.getPartitionBy().equals(this.getPartitionBy()) == false)
return false;
if (other.getFilter() == null ^ this.getFilter() == null)
return false;
if (other.getFilter() != null && other.getFilter().equals(this.getFilter()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServiceType() == null) ? 0 : getServiceType().hashCode());
hashCode = prime * hashCode + ((getIdentifier() == null) ? 0 : getIdentifier().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getMetric() == null) ? 0 : getMetric().hashCode());
hashCode = prime * hashCode + ((getPeriodInSeconds() == null) ? 0 : getPeriodInSeconds().hashCode());
hashCode = prime * hashCode + ((getGroupBy() == null) ? 0 : getGroupBy().hashCode());
hashCode = prime * hashCode + ((getAdditionalMetrics() == null) ? 0 : getAdditionalMetrics().hashCode());
hashCode = prime * hashCode + ((getPartitionBy() == null) ? 0 : getPartitionBy().hashCode());
hashCode = prime * hashCode + ((getFilter() == null) ? 0 : getFilter().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
return hashCode;
}
@Override
public DescribeDimensionKeysRequest clone() {
return (DescribeDimensionKeysRequest) super.clone();
}
}