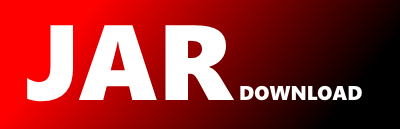
com.amazonaws.services.pi.model.Insight Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pi Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pi.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Retrieves the list of performance issues which are identified.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Insight implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique identifier for the insight. For example, insight-12345678901234567
.
*
*/
private String insightId;
/**
*
* The type of insight. For example, HighDBLoad
, HighCPU
, or DominatingSQLs
.
*
*/
private String insightType;
/**
*
* Indicates if the insight is causal or correlated insight.
*
*/
private String context;
/**
*
* The start time of the insight. For example, 2018-10-30T00:00:00Z
.
*
*/
private java.util.Date startTime;
/**
*
* The end time of the insight. For example, 2018-10-30T00:00:00Z
.
*
*/
private java.util.Date endTime;
/**
*
* The severity of the insight. The values are: Low
, Medium
, or High
.
*
*/
private String severity;
/**
*
* List of supporting insights that provide additional factors for the insight.
*
*/
private java.util.List supportingInsights;
/**
*
* Description of the insight. For example:
* A high severity Insight found between 02:00 to 02:30, where there was an unusually high DB load 600x above baseline. Likely performance impact
* .
*
*/
private String description;
/**
*
* List of recommendations for the insight. For example,
* Investigate the following SQLs that contributed to 100% of the total DBLoad during that time period: sql-id
* .
*
*/
private java.util.List recommendations;
/**
*
* List of data objects containing metrics and references from the time range while generating the insight.
*
*/
private java.util.List insightData;
/**
*
* Metric names and values from the timeframe used as baseline to generate the insight.
*
*/
private java.util.List baselineData;
/**
*
* The unique identifier for the insight. For example, insight-12345678901234567
.
*
*
* @param insightId
* The unique identifier for the insight. For example, insight-12345678901234567
.
*/
public void setInsightId(String insightId) {
this.insightId = insightId;
}
/**
*
* The unique identifier for the insight. For example, insight-12345678901234567
.
*
*
* @return The unique identifier for the insight. For example, insight-12345678901234567
.
*/
public String getInsightId() {
return this.insightId;
}
/**
*
* The unique identifier for the insight. For example, insight-12345678901234567
.
*
*
* @param insightId
* The unique identifier for the insight. For example, insight-12345678901234567
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withInsightId(String insightId) {
setInsightId(insightId);
return this;
}
/**
*
* The type of insight. For example, HighDBLoad
, HighCPU
, or DominatingSQLs
.
*
*
* @param insightType
* The type of insight. For example, HighDBLoad
, HighCPU
, or
* DominatingSQLs
.
*/
public void setInsightType(String insightType) {
this.insightType = insightType;
}
/**
*
* The type of insight. For example, HighDBLoad
, HighCPU
, or DominatingSQLs
.
*
*
* @return The type of insight. For example, HighDBLoad
, HighCPU
, or
* DominatingSQLs
.
*/
public String getInsightType() {
return this.insightType;
}
/**
*
* The type of insight. For example, HighDBLoad
, HighCPU
, or DominatingSQLs
.
*
*
* @param insightType
* The type of insight. For example, HighDBLoad
, HighCPU
, or
* DominatingSQLs
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withInsightType(String insightType) {
setInsightType(insightType);
return this;
}
/**
*
* Indicates if the insight is causal or correlated insight.
*
*
* @param context
* Indicates if the insight is causal or correlated insight.
* @see ContextType
*/
public void setContext(String context) {
this.context = context;
}
/**
*
* Indicates if the insight is causal or correlated insight.
*
*
* @return Indicates if the insight is causal or correlated insight.
* @see ContextType
*/
public String getContext() {
return this.context;
}
/**
*
* Indicates if the insight is causal or correlated insight.
*
*
* @param context
* Indicates if the insight is causal or correlated insight.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContextType
*/
public Insight withContext(String context) {
setContext(context);
return this;
}
/**
*
* Indicates if the insight is causal or correlated insight.
*
*
* @param context
* Indicates if the insight is causal or correlated insight.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContextType
*/
public Insight withContext(ContextType context) {
this.context = context.toString();
return this;
}
/**
*
* The start time of the insight. For example, 2018-10-30T00:00:00Z
.
*
*
* @param startTime
* The start time of the insight. For example, 2018-10-30T00:00:00Z
.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The start time of the insight. For example, 2018-10-30T00:00:00Z
.
*
*
* @return The start time of the insight. For example, 2018-10-30T00:00:00Z
.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The start time of the insight. For example, 2018-10-30T00:00:00Z
.
*
*
* @param startTime
* The start time of the insight. For example, 2018-10-30T00:00:00Z
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The end time of the insight. For example, 2018-10-30T00:00:00Z
.
*
*
* @param endTime
* The end time of the insight. For example, 2018-10-30T00:00:00Z
.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The end time of the insight. For example, 2018-10-30T00:00:00Z
.
*
*
* @return The end time of the insight. For example, 2018-10-30T00:00:00Z
.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The end time of the insight. For example, 2018-10-30T00:00:00Z
.
*
*
* @param endTime
* The end time of the insight. For example, 2018-10-30T00:00:00Z
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* The severity of the insight. The values are: Low
, Medium
, or High
.
*
*
* @param severity
* The severity of the insight. The values are: Low
, Medium
, or High
.
* @see Severity
*/
public void setSeverity(String severity) {
this.severity = severity;
}
/**
*
* The severity of the insight. The values are: Low
, Medium
, or High
.
*
*
* @return The severity of the insight. The values are: Low
, Medium
, or High
.
* @see Severity
*/
public String getSeverity() {
return this.severity;
}
/**
*
* The severity of the insight. The values are: Low
, Medium
, or High
.
*
*
* @param severity
* The severity of the insight. The values are: Low
, Medium
, or High
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Severity
*/
public Insight withSeverity(String severity) {
setSeverity(severity);
return this;
}
/**
*
* The severity of the insight. The values are: Low
, Medium
, or High
.
*
*
* @param severity
* The severity of the insight. The values are: Low
, Medium
, or High
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Severity
*/
public Insight withSeverity(Severity severity) {
this.severity = severity.toString();
return this;
}
/**
*
* List of supporting insights that provide additional factors for the insight.
*
*
* @return List of supporting insights that provide additional factors for the insight.
*/
public java.util.List getSupportingInsights() {
return supportingInsights;
}
/**
*
* List of supporting insights that provide additional factors for the insight.
*
*
* @param supportingInsights
* List of supporting insights that provide additional factors for the insight.
*/
public void setSupportingInsights(java.util.Collection supportingInsights) {
if (supportingInsights == null) {
this.supportingInsights = null;
return;
}
this.supportingInsights = new java.util.ArrayList(supportingInsights);
}
/**
*
* List of supporting insights that provide additional factors for the insight.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportingInsights(java.util.Collection)} or {@link #withSupportingInsights(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param supportingInsights
* List of supporting insights that provide additional factors for the insight.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withSupportingInsights(Insight... supportingInsights) {
if (this.supportingInsights == null) {
setSupportingInsights(new java.util.ArrayList(supportingInsights.length));
}
for (Insight ele : supportingInsights) {
this.supportingInsights.add(ele);
}
return this;
}
/**
*
* List of supporting insights that provide additional factors for the insight.
*
*
* @param supportingInsights
* List of supporting insights that provide additional factors for the insight.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withSupportingInsights(java.util.Collection supportingInsights) {
setSupportingInsights(supportingInsights);
return this;
}
/**
*
* Description of the insight. For example:
* A high severity Insight found between 02:00 to 02:30, where there was an unusually high DB load 600x above baseline. Likely performance impact
* .
*
*
* @param description
* Description of the insight. For example:
* A high severity Insight found between 02:00 to 02:30, where there was an unusually high DB load 600x above baseline. Likely performance impact
* .
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* Description of the insight. For example:
* A high severity Insight found between 02:00 to 02:30, where there was an unusually high DB load 600x above baseline. Likely performance impact
* .
*
*
* @return Description of the insight. For example:
* A high severity Insight found between 02:00 to 02:30, where there was an unusually high DB load 600x above baseline. Likely performance impact
* .
*/
public String getDescription() {
return this.description;
}
/**
*
* Description of the insight. For example:
* A high severity Insight found between 02:00 to 02:30, where there was an unusually high DB load 600x above baseline. Likely performance impact
* .
*
*
* @param description
* Description of the insight. For example:
* A high severity Insight found between 02:00 to 02:30, where there was an unusually high DB load 600x above baseline. Likely performance impact
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* List of recommendations for the insight. For example,
* Investigate the following SQLs that contributed to 100% of the total DBLoad during that time period: sql-id
* .
*
*
* @return List of recommendations for the insight. For example,
* Investigate the following SQLs that contributed to 100% of the total DBLoad during that time period: sql-id
* .
*/
public java.util.List getRecommendations() {
return recommendations;
}
/**
*
* List of recommendations for the insight. For example,
* Investigate the following SQLs that contributed to 100% of the total DBLoad during that time period: sql-id
* .
*
*
* @param recommendations
* List of recommendations for the insight. For example,
* Investigate the following SQLs that contributed to 100% of the total DBLoad during that time period: sql-id
* .
*/
public void setRecommendations(java.util.Collection recommendations) {
if (recommendations == null) {
this.recommendations = null;
return;
}
this.recommendations = new java.util.ArrayList(recommendations);
}
/**
*
* List of recommendations for the insight. For example,
* Investigate the following SQLs that contributed to 100% of the total DBLoad during that time period: sql-id
* .
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRecommendations(java.util.Collection)} or {@link #withRecommendations(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param recommendations
* List of recommendations for the insight. For example,
* Investigate the following SQLs that contributed to 100% of the total DBLoad during that time period: sql-id
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withRecommendations(Recommendation... recommendations) {
if (this.recommendations == null) {
setRecommendations(new java.util.ArrayList(recommendations.length));
}
for (Recommendation ele : recommendations) {
this.recommendations.add(ele);
}
return this;
}
/**
*
* List of recommendations for the insight. For example,
* Investigate the following SQLs that contributed to 100% of the total DBLoad during that time period: sql-id
* .
*
*
* @param recommendations
* List of recommendations for the insight. For example,
* Investigate the following SQLs that contributed to 100% of the total DBLoad during that time period: sql-id
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withRecommendations(java.util.Collection recommendations) {
setRecommendations(recommendations);
return this;
}
/**
*
* List of data objects containing metrics and references from the time range while generating the insight.
*
*
* @return List of data objects containing metrics and references from the time range while generating the insight.
*/
public java.util.List getInsightData() {
return insightData;
}
/**
*
* List of data objects containing metrics and references from the time range while generating the insight.
*
*
* @param insightData
* List of data objects containing metrics and references from the time range while generating the insight.
*/
public void setInsightData(java.util.Collection insightData) {
if (insightData == null) {
this.insightData = null;
return;
}
this.insightData = new java.util.ArrayList(insightData);
}
/**
*
* List of data objects containing metrics and references from the time range while generating the insight.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInsightData(java.util.Collection)} or {@link #withInsightData(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param insightData
* List of data objects containing metrics and references from the time range while generating the insight.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withInsightData(Data... insightData) {
if (this.insightData == null) {
setInsightData(new java.util.ArrayList(insightData.length));
}
for (Data ele : insightData) {
this.insightData.add(ele);
}
return this;
}
/**
*
* List of data objects containing metrics and references from the time range while generating the insight.
*
*
* @param insightData
* List of data objects containing metrics and references from the time range while generating the insight.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withInsightData(java.util.Collection insightData) {
setInsightData(insightData);
return this;
}
/**
*
* Metric names and values from the timeframe used as baseline to generate the insight.
*
*
* @return Metric names and values from the timeframe used as baseline to generate the insight.
*/
public java.util.List getBaselineData() {
return baselineData;
}
/**
*
* Metric names and values from the timeframe used as baseline to generate the insight.
*
*
* @param baselineData
* Metric names and values from the timeframe used as baseline to generate the insight.
*/
public void setBaselineData(java.util.Collection baselineData) {
if (baselineData == null) {
this.baselineData = null;
return;
}
this.baselineData = new java.util.ArrayList(baselineData);
}
/**
*
* Metric names and values from the timeframe used as baseline to generate the insight.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setBaselineData(java.util.Collection)} or {@link #withBaselineData(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param baselineData
* Metric names and values from the timeframe used as baseline to generate the insight.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withBaselineData(Data... baselineData) {
if (this.baselineData == null) {
setBaselineData(new java.util.ArrayList(baselineData.length));
}
for (Data ele : baselineData) {
this.baselineData.add(ele);
}
return this;
}
/**
*
* Metric names and values from the timeframe used as baseline to generate the insight.
*
*
* @param baselineData
* Metric names and values from the timeframe used as baseline to generate the insight.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Insight withBaselineData(java.util.Collection baselineData) {
setBaselineData(baselineData);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getInsightId() != null)
sb.append("InsightId: ").append(getInsightId()).append(",");
if (getInsightType() != null)
sb.append("InsightType: ").append(getInsightType()).append(",");
if (getContext() != null)
sb.append("Context: ").append(getContext()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getSeverity() != null)
sb.append("Severity: ").append(getSeverity()).append(",");
if (getSupportingInsights() != null)
sb.append("SupportingInsights: ").append(getSupportingInsights()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getRecommendations() != null)
sb.append("Recommendations: ").append(getRecommendations()).append(",");
if (getInsightData() != null)
sb.append("InsightData: ").append(getInsightData()).append(",");
if (getBaselineData() != null)
sb.append("BaselineData: ").append(getBaselineData());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Insight == false)
return false;
Insight other = (Insight) obj;
if (other.getInsightId() == null ^ this.getInsightId() == null)
return false;
if (other.getInsightId() != null && other.getInsightId().equals(this.getInsightId()) == false)
return false;
if (other.getInsightType() == null ^ this.getInsightType() == null)
return false;
if (other.getInsightType() != null && other.getInsightType().equals(this.getInsightType()) == false)
return false;
if (other.getContext() == null ^ this.getContext() == null)
return false;
if (other.getContext() != null && other.getContext().equals(this.getContext()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getSeverity() == null ^ this.getSeverity() == null)
return false;
if (other.getSeverity() != null && other.getSeverity().equals(this.getSeverity()) == false)
return false;
if (other.getSupportingInsights() == null ^ this.getSupportingInsights() == null)
return false;
if (other.getSupportingInsights() != null && other.getSupportingInsights().equals(this.getSupportingInsights()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getRecommendations() == null ^ this.getRecommendations() == null)
return false;
if (other.getRecommendations() != null && other.getRecommendations().equals(this.getRecommendations()) == false)
return false;
if (other.getInsightData() == null ^ this.getInsightData() == null)
return false;
if (other.getInsightData() != null && other.getInsightData().equals(this.getInsightData()) == false)
return false;
if (other.getBaselineData() == null ^ this.getBaselineData() == null)
return false;
if (other.getBaselineData() != null && other.getBaselineData().equals(this.getBaselineData()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getInsightId() == null) ? 0 : getInsightId().hashCode());
hashCode = prime * hashCode + ((getInsightType() == null) ? 0 : getInsightType().hashCode());
hashCode = prime * hashCode + ((getContext() == null) ? 0 : getContext().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getSeverity() == null) ? 0 : getSeverity().hashCode());
hashCode = prime * hashCode + ((getSupportingInsights() == null) ? 0 : getSupportingInsights().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getRecommendations() == null) ? 0 : getRecommendations().hashCode());
hashCode = prime * hashCode + ((getInsightData() == null) ? 0 : getInsightData().hashCode());
hashCode = prime * hashCode + ((getBaselineData() == null) ? 0 : getBaselineData().hashCode());
return hashCode;
}
@Override
public Insight clone() {
try {
return (Insight) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.pi.model.transform.InsightMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}