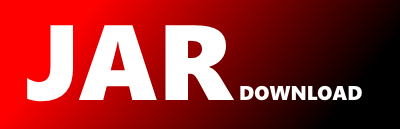
com.amazonaws.services.pinpointemail.AmazonPinpointEmailAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pinpointemail Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointemail;
import javax.annotation.Generated;
import com.amazonaws.services.pinpointemail.model.*;
/**
* Interface for accessing Pinpoint Email asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.pinpointemail.AbstractAmazonPinpointEmailAsync} instead.
*
*
* Amazon Pinpoint Email Service
*
* Welcome to the Amazon Pinpoint Email API Reference. This guide provides information about the Amazon Pinpoint
* Email API (version 1.0), including supported operations, data types, parameters, and schemas.
*
*
* Amazon Pinpoint is an AWS service that you can use to engage with your
* customers across multiple messaging channels. You can use Amazon Pinpoint to send email, SMS text messages, voice
* messages, and push notifications. The Amazon Pinpoint Email API provides programmatic access to options that are
* unique to the email channel and supplement the options provided by the Amazon Pinpoint API.
*
*
* If you're new to Amazon Pinpoint, you might find it helpful to also review the Amazon Pinpoint Developer Guide.
* The Amazon Pinpoint Developer Guide provides tutorials, code samples, and procedures that demonstrate how to
* use Amazon Pinpoint features programmatically and how to integrate Amazon Pinpoint functionality into mobile apps and
* other types of applications. The guide also provides information about key topics such as Amazon Pinpoint integration
* with other AWS services and the limits that apply to using the service.
*
*
* The Amazon Pinpoint Email API is available in several AWS Regions and it provides an endpoint for each of these
* Regions. For a list of all the Regions and endpoints where the API is currently available, see AWS Service Endpoints in the
* Amazon Web Services General Reference. To learn more about AWS Regions, see Managing AWS Regions in the Amazon Web
* Services General Reference.
*
*
* In each Region, AWS maintains multiple Availability Zones. These Availability Zones are physically isolated from each
* other, but are united by private, low-latency, high-throughput, and highly redundant network connections. These
* Availability Zones enable us to provide very high levels of availability and redundancy, while also minimizing
* latency. To learn more about the number of Availability Zones that are available in each Region, see AWS Global Infrastructure.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonPinpointEmailAsync extends AmazonPinpointEmail {
/**
*
* Create a configuration set. Configuration sets are groups of rules that you can apply to the emails you
* send using Amazon Pinpoint. You apply a configuration set to an email by including a reference to the
* configuration set in the headers of the email. When you apply a configuration set to an email, all of the rules
* in that configuration set are applied to the email.
*
*
* @param createConfigurationSetRequest
* A request to create a configuration set.
* @return A Java Future containing the result of the CreateConfigurationSet operation returned by the service.
* @sample AmazonPinpointEmailAsync.CreateConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createConfigurationSetAsync(CreateConfigurationSetRequest createConfigurationSetRequest);
/**
*
* Create a configuration set. Configuration sets are groups of rules that you can apply to the emails you
* send using Amazon Pinpoint. You apply a configuration set to an email by including a reference to the
* configuration set in the headers of the email. When you apply a configuration set to an email, all of the rules
* in that configuration set are applied to the email.
*
*
* @param createConfigurationSetRequest
* A request to create a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConfigurationSet operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.CreateConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createConfigurationSetAsync(CreateConfigurationSetRequest createConfigurationSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an event destination. In Amazon Pinpoint, events include message sends, deliveries, opens, clicks,
* bounces, and complaints. Event destinations are places that you can send information about these events
* to. For example, you can send event data to Amazon SNS to receive notifications when you receive bounces or
* complaints, or you can use Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* A single configuration set can include more than one event destination.
*
*
* @param createConfigurationSetEventDestinationRequest
* A request to add an event destination to a configuration set.
* @return A Java Future containing the result of the CreateConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonPinpointEmailAsync.CreateConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future createConfigurationSetEventDestinationAsync(
CreateConfigurationSetEventDestinationRequest createConfigurationSetEventDestinationRequest);
/**
*
* Create an event destination. In Amazon Pinpoint, events include message sends, deliveries, opens, clicks,
* bounces, and complaints. Event destinations are places that you can send information about these events
* to. For example, you can send event data to Amazon SNS to receive notifications when you receive bounces or
* complaints, or you can use Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* A single configuration set can include more than one event destination.
*
*
* @param createConfigurationSetEventDestinationRequest
* A request to add an event destination to a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonPinpointEmailAsyncHandler.CreateConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future createConfigurationSetEventDestinationAsync(
CreateConfigurationSetEventDestinationRequest createConfigurationSetEventDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new pool of dedicated IP addresses. A pool can include one or more dedicated IP addresses that are
* associated with your Amazon Pinpoint account. You can associate a pool with a configuration set. When you send an
* email that uses that configuration set, Amazon Pinpoint sends it using only the IP addresses in the associated
* pool.
*
*
* @param createDedicatedIpPoolRequest
* A request to create a new dedicated IP pool.
* @return A Java Future containing the result of the CreateDedicatedIpPool operation returned by the service.
* @sample AmazonPinpointEmailAsync.CreateDedicatedIpPool
* @see AWS API Documentation
*/
java.util.concurrent.Future createDedicatedIpPoolAsync(CreateDedicatedIpPoolRequest createDedicatedIpPoolRequest);
/**
*
* Create a new pool of dedicated IP addresses. A pool can include one or more dedicated IP addresses that are
* associated with your Amazon Pinpoint account. You can associate a pool with a configuration set. When you send an
* email that uses that configuration set, Amazon Pinpoint sends it using only the IP addresses in the associated
* pool.
*
*
* @param createDedicatedIpPoolRequest
* A request to create a new dedicated IP pool.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDedicatedIpPool operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.CreateDedicatedIpPool
* @see AWS API Documentation
*/
java.util.concurrent.Future createDedicatedIpPoolAsync(CreateDedicatedIpPoolRequest createDedicatedIpPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new predictive inbox placement test. Predictive inbox placement tests can help you predict how your
* messages will be handled by various email providers around the world. When you perform a predictive inbox
* placement test, you provide a sample message that contains the content that you plan to send to your customers.
* Amazon Pinpoint then sends that message to special email addresses spread across several major email providers.
* After about 24 hours, the test is complete, and you can use the GetDeliverabilityTestReport
* operation to view the results of the test.
*
*
* @param createDeliverabilityTestReportRequest
* A request to perform a predictive inbox placement test. Predictive inbox placement tests can help you
* predict how your messages will be handled by various email providers around the world. When you perform a
* predictive inbox placement test, you provide a sample message that contains the content that you plan to
* send to your customers. Amazon Pinpoint then sends that message to special email addresses spread across
* several major email providers. After about 24 hours, the test is complete, and you can use the
* GetDeliverabilityTestReport
operation to view the results of the test.
* @return A Java Future containing the result of the CreateDeliverabilityTestReport operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.CreateDeliverabilityTestReport
* @see AWS API Documentation
*/
java.util.concurrent.Future createDeliverabilityTestReportAsync(
CreateDeliverabilityTestReportRequest createDeliverabilityTestReportRequest);
/**
*
* Create a new predictive inbox placement test. Predictive inbox placement tests can help you predict how your
* messages will be handled by various email providers around the world. When you perform a predictive inbox
* placement test, you provide a sample message that contains the content that you plan to send to your customers.
* Amazon Pinpoint then sends that message to special email addresses spread across several major email providers.
* After about 24 hours, the test is complete, and you can use the GetDeliverabilityTestReport
* operation to view the results of the test.
*
*
* @param createDeliverabilityTestReportRequest
* A request to perform a predictive inbox placement test. Predictive inbox placement tests can help you
* predict how your messages will be handled by various email providers around the world. When you perform a
* predictive inbox placement test, you provide a sample message that contains the content that you plan to
* send to your customers. Amazon Pinpoint then sends that message to special email addresses spread across
* several major email providers. After about 24 hours, the test is complete, and you can use the
* GetDeliverabilityTestReport
operation to view the results of the test.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDeliverabilityTestReport operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.CreateDeliverabilityTestReport
* @see AWS API Documentation
*/
java.util.concurrent.Future createDeliverabilityTestReportAsync(
CreateDeliverabilityTestReportRequest createDeliverabilityTestReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Verifies an email identity for use with Amazon Pinpoint. In Amazon Pinpoint, an identity is an email address or
* domain that you use when you send email. Before you can use an identity to send email with Amazon Pinpoint, you
* first have to verify it. By verifying an address, you demonstrate that you're the owner of the address, and that
* you've given Amazon Pinpoint permission to send email from the address.
*
*
* When you verify an email address, Amazon Pinpoint sends an email to the address. Your email address is verified
* as soon as you follow the link in the verification email.
*
*
* When you verify a domain, this operation provides a set of DKIM tokens, which you can convert into CNAME tokens.
* You add these CNAME tokens to the DNS configuration for your domain. Your domain is verified when Amazon Pinpoint
* detects these records in the DNS configuration for your domain. It usually takes around 72 hours to complete the
* domain verification process.
*
*
* @param createEmailIdentityRequest
* A request to begin the verification process for an email identity (an email address or domain).
* @return A Java Future containing the result of the CreateEmailIdentity operation returned by the service.
* @sample AmazonPinpointEmailAsync.CreateEmailIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future createEmailIdentityAsync(CreateEmailIdentityRequest createEmailIdentityRequest);
/**
*
* Verifies an email identity for use with Amazon Pinpoint. In Amazon Pinpoint, an identity is an email address or
* domain that you use when you send email. Before you can use an identity to send email with Amazon Pinpoint, you
* first have to verify it. By verifying an address, you demonstrate that you're the owner of the address, and that
* you've given Amazon Pinpoint permission to send email from the address.
*
*
* When you verify an email address, Amazon Pinpoint sends an email to the address. Your email address is verified
* as soon as you follow the link in the verification email.
*
*
* When you verify a domain, this operation provides a set of DKIM tokens, which you can convert into CNAME tokens.
* You add these CNAME tokens to the DNS configuration for your domain. Your domain is verified when Amazon Pinpoint
* detects these records in the DNS configuration for your domain. It usually takes around 72 hours to complete the
* domain verification process.
*
*
* @param createEmailIdentityRequest
* A request to begin the verification process for an email identity (an email address or domain).
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEmailIdentity operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.CreateEmailIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future createEmailIdentityAsync(CreateEmailIdentityRequest createEmailIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an existing configuration set.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param deleteConfigurationSetRequest
* A request to delete a configuration set.
* @return A Java Future containing the result of the DeleteConfigurationSet operation returned by the service.
* @sample AmazonPinpointEmailAsync.DeleteConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetAsync(DeleteConfigurationSetRequest deleteConfigurationSetRequest);
/**
*
* Delete an existing configuration set.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param deleteConfigurationSetRequest
* A request to delete a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConfigurationSet operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.DeleteConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetAsync(DeleteConfigurationSetRequest deleteConfigurationSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an event destination.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param deleteConfigurationSetEventDestinationRequest
* A request to delete an event destination from a configuration set.
* @return A Java Future containing the result of the DeleteConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonPinpointEmailAsync.DeleteConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetEventDestinationAsync(
DeleteConfigurationSetEventDestinationRequest deleteConfigurationSetEventDestinationRequest);
/**
*
* Delete an event destination.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param deleteConfigurationSetEventDestinationRequest
* A request to delete an event destination from a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonPinpointEmailAsyncHandler.DeleteConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetEventDestinationAsync(
DeleteConfigurationSetEventDestinationRequest deleteConfigurationSetEventDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a dedicated IP pool.
*
*
* @param deleteDedicatedIpPoolRequest
* A request to delete a dedicated IP pool.
* @return A Java Future containing the result of the DeleteDedicatedIpPool operation returned by the service.
* @sample AmazonPinpointEmailAsync.DeleteDedicatedIpPool
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDedicatedIpPoolAsync(DeleteDedicatedIpPoolRequest deleteDedicatedIpPoolRequest);
/**
*
* Delete a dedicated IP pool.
*
*
* @param deleteDedicatedIpPoolRequest
* A request to delete a dedicated IP pool.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDedicatedIpPool operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.DeleteDedicatedIpPool
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDedicatedIpPoolAsync(DeleteDedicatedIpPoolRequest deleteDedicatedIpPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an email identity that you previously verified for use with Amazon Pinpoint. An identity can be either an
* email address or a domain name.
*
*
* @param deleteEmailIdentityRequest
* A request to delete an existing email identity. When you delete an identity, you lose the ability to use
* Amazon Pinpoint to send email from that identity. You can restore your ability to send email by completing
* the verification process for the identity again.
* @return A Java Future containing the result of the DeleteEmailIdentity operation returned by the service.
* @sample AmazonPinpointEmailAsync.DeleteEmailIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEmailIdentityAsync(DeleteEmailIdentityRequest deleteEmailIdentityRequest);
/**
*
* Deletes an email identity that you previously verified for use with Amazon Pinpoint. An identity can be either an
* email address or a domain name.
*
*
* @param deleteEmailIdentityRequest
* A request to delete an existing email identity. When you delete an identity, you lose the ability to use
* Amazon Pinpoint to send email from that identity. You can restore your ability to send email by completing
* the verification process for the identity again.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEmailIdentity operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.DeleteEmailIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEmailIdentityAsync(DeleteEmailIdentityRequest deleteEmailIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtain information about the email-sending status and capabilities of your Amazon Pinpoint account in the current
* AWS Region.
*
*
* @param getAccountRequest
* A request to obtain information about the email-sending capabilities of your Amazon Pinpoint account.
* @return A Java Future containing the result of the GetAccount operation returned by the service.
* @sample AmazonPinpointEmailAsync.GetAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountAsync(GetAccountRequest getAccountRequest);
/**
*
* Obtain information about the email-sending status and capabilities of your Amazon Pinpoint account in the current
* AWS Region.
*
*
* @param getAccountRequest
* A request to obtain information about the email-sending capabilities of your Amazon Pinpoint account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccount operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.GetAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountAsync(GetAccountRequest getAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve a list of the blacklists that your dedicated IP addresses appear on.
*
*
* @param getBlacklistReportsRequest
* A request to retrieve a list of the blacklists that your dedicated IP addresses appear on.
* @return A Java Future containing the result of the GetBlacklistReports operation returned by the service.
* @sample AmazonPinpointEmailAsync.GetBlacklistReports
* @see AWS API Documentation
*/
java.util.concurrent.Future getBlacklistReportsAsync(GetBlacklistReportsRequest getBlacklistReportsRequest);
/**
*
* Retrieve a list of the blacklists that your dedicated IP addresses appear on.
*
*
* @param getBlacklistReportsRequest
* A request to retrieve a list of the blacklists that your dedicated IP addresses appear on.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBlacklistReports operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.GetBlacklistReports
* @see AWS API Documentation
*/
java.util.concurrent.Future getBlacklistReportsAsync(GetBlacklistReportsRequest getBlacklistReportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get information about an existing configuration set, including the dedicated IP pool that it's associated with,
* whether or not it's enabled for sending email, and more.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param getConfigurationSetRequest
* A request to obtain information about a configuration set.
* @return A Java Future containing the result of the GetConfigurationSet operation returned by the service.
* @sample AmazonPinpointEmailAsync.GetConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future getConfigurationSetAsync(GetConfigurationSetRequest getConfigurationSetRequest);
/**
*
* Get information about an existing configuration set, including the dedicated IP pool that it's associated with,
* whether or not it's enabled for sending email, and more.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param getConfigurationSetRequest
* A request to obtain information about a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetConfigurationSet operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.GetConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future getConfigurationSetAsync(GetConfigurationSetRequest getConfigurationSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve a list of event destinations that are associated with a configuration set.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param getConfigurationSetEventDestinationsRequest
* A request to obtain information about the event destinations for a configuration set.
* @return A Java Future containing the result of the GetConfigurationSetEventDestinations operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.GetConfigurationSetEventDestinations
* @see AWS API Documentation
*/
java.util.concurrent.Future getConfigurationSetEventDestinationsAsync(
GetConfigurationSetEventDestinationsRequest getConfigurationSetEventDestinationsRequest);
/**
*
* Retrieve a list of event destinations that are associated with a configuration set.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param getConfigurationSetEventDestinationsRequest
* A request to obtain information about the event destinations for a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetConfigurationSetEventDestinations operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.GetConfigurationSetEventDestinations
* @see AWS API Documentation
*/
java.util.concurrent.Future getConfigurationSetEventDestinationsAsync(
GetConfigurationSetEventDestinationsRequest getConfigurationSetEventDestinationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get information about a dedicated IP address, including the name of the dedicated IP pool that it's associated
* with, as well information about the automatic warm-up process for the address.
*
*
* @param getDedicatedIpRequest
* A request to obtain more information about a dedicated IP address.
* @return A Java Future containing the result of the GetDedicatedIp operation returned by the service.
* @sample AmazonPinpointEmailAsync.GetDedicatedIp
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDedicatedIpAsync(GetDedicatedIpRequest getDedicatedIpRequest);
/**
*
* Get information about a dedicated IP address, including the name of the dedicated IP pool that it's associated
* with, as well information about the automatic warm-up process for the address.
*
*
* @param getDedicatedIpRequest
* A request to obtain more information about a dedicated IP address.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDedicatedIp operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.GetDedicatedIp
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDedicatedIpAsync(GetDedicatedIpRequest getDedicatedIpRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the dedicated IP addresses that are associated with your Amazon Pinpoint account.
*
*
* @param getDedicatedIpsRequest
* A request to obtain more information about dedicated IP pools.
* @return A Java Future containing the result of the GetDedicatedIps operation returned by the service.
* @sample AmazonPinpointEmailAsync.GetDedicatedIps
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDedicatedIpsAsync(GetDedicatedIpsRequest getDedicatedIpsRequest);
/**
*
* List the dedicated IP addresses that are associated with your Amazon Pinpoint account.
*
*
* @param getDedicatedIpsRequest
* A request to obtain more information about dedicated IP pools.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDedicatedIps operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.GetDedicatedIps
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDedicatedIpsAsync(GetDedicatedIpsRequest getDedicatedIpsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve information about the status of the Deliverability dashboard for your Amazon Pinpoint account. When the
* Deliverability dashboard is enabled, you gain access to reputation, deliverability, and other metrics for the
* domains that you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox
* placement tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon Pinpoint. For more information about the features and cost of a Deliverability
* dashboard subscription, see Amazon Pinpoint Pricing.
*
*
* @param getDeliverabilityDashboardOptionsRequest
* Retrieve information about the status of the Deliverability dashboard for your Amazon Pinpoint account.
* When the Deliverability dashboard is enabled, you gain access to reputation, deliverability, and other
* metrics for the domains that you use to send email using Amazon Pinpoint. You also gain the ability to
* perform predictive inbox placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon Pinpoint. For more information about the features and cost of a
* Deliverability dashboard subscription, see Amazon
* Pinpoint Pricing.
* @return A Java Future containing the result of the GetDeliverabilityDashboardOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.GetDeliverabilityDashboardOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeliverabilityDashboardOptionsAsync(
GetDeliverabilityDashboardOptionsRequest getDeliverabilityDashboardOptionsRequest);
/**
*
* Retrieve information about the status of the Deliverability dashboard for your Amazon Pinpoint account. When the
* Deliverability dashboard is enabled, you gain access to reputation, deliverability, and other metrics for the
* domains that you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox
* placement tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon Pinpoint. For more information about the features and cost of a Deliverability
* dashboard subscription, see Amazon Pinpoint Pricing.
*
*
* @param getDeliverabilityDashboardOptionsRequest
* Retrieve information about the status of the Deliverability dashboard for your Amazon Pinpoint account.
* When the Deliverability dashboard is enabled, you gain access to reputation, deliverability, and other
* metrics for the domains that you use to send email using Amazon Pinpoint. You also gain the ability to
* perform predictive inbox placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon Pinpoint. For more information about the features and cost of a
* Deliverability dashboard subscription, see Amazon
* Pinpoint Pricing.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeliverabilityDashboardOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.GetDeliverabilityDashboardOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeliverabilityDashboardOptionsAsync(
GetDeliverabilityDashboardOptionsRequest getDeliverabilityDashboardOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve the results of a predictive inbox placement test.
*
*
* @param getDeliverabilityTestReportRequest
* A request to retrieve the results of a predictive inbox placement test.
* @return A Java Future containing the result of the GetDeliverabilityTestReport operation returned by the service.
* @sample AmazonPinpointEmailAsync.GetDeliverabilityTestReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeliverabilityTestReportAsync(
GetDeliverabilityTestReportRequest getDeliverabilityTestReportRequest);
/**
*
* Retrieve the results of a predictive inbox placement test.
*
*
* @param getDeliverabilityTestReportRequest
* A request to retrieve the results of a predictive inbox placement test.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeliverabilityTestReport operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.GetDeliverabilityTestReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeliverabilityTestReportAsync(
GetDeliverabilityTestReportRequest getDeliverabilityTestReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only if the
* campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
*
*
* @param getDomainDeliverabilityCampaignRequest
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only
* if the campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
* @return A Java Future containing the result of the GetDomainDeliverabilityCampaign operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.GetDomainDeliverabilityCampaign
* @see AWS API Documentation
*/
java.util.concurrent.Future getDomainDeliverabilityCampaignAsync(
GetDomainDeliverabilityCampaignRequest getDomainDeliverabilityCampaignRequest);
/**
*
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only if the
* campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
*
*
* @param getDomainDeliverabilityCampaignRequest
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only
* if the campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDomainDeliverabilityCampaign operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.GetDomainDeliverabilityCampaign
* @see AWS API Documentation
*/
java.util.concurrent.Future getDomainDeliverabilityCampaignAsync(
GetDomainDeliverabilityCampaignRequest getDomainDeliverabilityCampaignRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve inbox placement and engagement rates for the domains that you use to send email.
*
*
* @param getDomainStatisticsReportRequest
* A request to obtain deliverability metrics for a domain.
* @return A Java Future containing the result of the GetDomainStatisticsReport operation returned by the service.
* @sample AmazonPinpointEmailAsync.GetDomainStatisticsReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getDomainStatisticsReportAsync(
GetDomainStatisticsReportRequest getDomainStatisticsReportRequest);
/**
*
* Retrieve inbox placement and engagement rates for the domains that you use to send email.
*
*
* @param getDomainStatisticsReportRequest
* A request to obtain deliverability metrics for a domain.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDomainStatisticsReport operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.GetDomainStatisticsReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getDomainStatisticsReportAsync(
GetDomainStatisticsReportRequest getDomainStatisticsReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about a specific identity associated with your Amazon Pinpoint account, including the
* identity's verification status, its DKIM authentication status, and its custom Mail-From settings.
*
*
* @param getEmailIdentityRequest
* A request to return details about an email identity.
* @return A Java Future containing the result of the GetEmailIdentity operation returned by the service.
* @sample AmazonPinpointEmailAsync.GetEmailIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future getEmailIdentityAsync(GetEmailIdentityRequest getEmailIdentityRequest);
/**
*
* Provides information about a specific identity associated with your Amazon Pinpoint account, including the
* identity's verification status, its DKIM authentication status, and its custom Mail-From settings.
*
*
* @param getEmailIdentityRequest
* A request to return details about an email identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEmailIdentity operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.GetEmailIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future getEmailIdentityAsync(GetEmailIdentityRequest getEmailIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all of the configuration sets associated with your Amazon Pinpoint account in the current region.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param listConfigurationSetsRequest
* A request to obtain a list of configuration sets for your Amazon Pinpoint account in the current AWS
* Region.
* @return A Java Future containing the result of the ListConfigurationSets operation returned by the service.
* @sample AmazonPinpointEmailAsync.ListConfigurationSets
* @see AWS API Documentation
*/
java.util.concurrent.Future listConfigurationSetsAsync(ListConfigurationSetsRequest listConfigurationSetsRequest);
/**
*
* List all of the configuration sets associated with your Amazon Pinpoint account in the current region.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param listConfigurationSetsRequest
* A request to obtain a list of configuration sets for your Amazon Pinpoint account in the current AWS
* Region.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListConfigurationSets operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.ListConfigurationSets
* @see AWS API Documentation
*/
java.util.concurrent.Future listConfigurationSetsAsync(ListConfigurationSetsRequest listConfigurationSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all of the dedicated IP pools that exist in your Amazon Pinpoint account in the current AWS Region.
*
*
* @param listDedicatedIpPoolsRequest
* A request to obtain a list of dedicated IP pools.
* @return A Java Future containing the result of the ListDedicatedIpPools operation returned by the service.
* @sample AmazonPinpointEmailAsync.ListDedicatedIpPools
* @see AWS API Documentation
*/
java.util.concurrent.Future listDedicatedIpPoolsAsync(ListDedicatedIpPoolsRequest listDedicatedIpPoolsRequest);
/**
*
* List all of the dedicated IP pools that exist in your Amazon Pinpoint account in the current AWS Region.
*
*
* @param listDedicatedIpPoolsRequest
* A request to obtain a list of dedicated IP pools.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDedicatedIpPools operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.ListDedicatedIpPools
* @see AWS API Documentation
*/
java.util.concurrent.Future listDedicatedIpPoolsAsync(ListDedicatedIpPoolsRequest listDedicatedIpPoolsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Show a list of the predictive inbox placement tests that you've performed, regardless of their statuses. For
* predictive inbox placement tests that are complete, you can use the GetDeliverabilityTestReport
* operation to view the results.
*
*
* @param listDeliverabilityTestReportsRequest
* A request to list all of the predictive inbox placement tests that you've performed.
* @return A Java Future containing the result of the ListDeliverabilityTestReports operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.ListDeliverabilityTestReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeliverabilityTestReportsAsync(
ListDeliverabilityTestReportsRequest listDeliverabilityTestReportsRequest);
/**
*
* Show a list of the predictive inbox placement tests that you've performed, regardless of their statuses. For
* predictive inbox placement tests that are complete, you can use the GetDeliverabilityTestReport
* operation to view the results.
*
*
* @param listDeliverabilityTestReportsRequest
* A request to list all of the predictive inbox placement tests that you've performed.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDeliverabilityTestReports operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.ListDeliverabilityTestReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeliverabilityTestReportsAsync(
ListDeliverabilityTestReportsRequest listDeliverabilityTestReportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a specified
* time range. This data is available for a domain only if you enabled the Deliverability dashboard (
* PutDeliverabilityDashboardOption
operation) for the domain.
*
*
* @param listDomainDeliverabilityCampaignsRequest
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a
* specified time range. This data is available for a domain only if you enabled the Deliverability dashboard
* (PutDeliverabilityDashboardOption
operation) for the domain.
* @return A Java Future containing the result of the ListDomainDeliverabilityCampaigns operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.ListDomainDeliverabilityCampaigns
* @see AWS API Documentation
*/
java.util.concurrent.Future listDomainDeliverabilityCampaignsAsync(
ListDomainDeliverabilityCampaignsRequest listDomainDeliverabilityCampaignsRequest);
/**
*
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a specified
* time range. This data is available for a domain only if you enabled the Deliverability dashboard (
* PutDeliverabilityDashboardOption
operation) for the domain.
*
*
* @param listDomainDeliverabilityCampaignsRequest
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a
* specified time range. This data is available for a domain only if you enabled the Deliverability dashboard
* (PutDeliverabilityDashboardOption
operation) for the domain.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDomainDeliverabilityCampaigns operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.ListDomainDeliverabilityCampaigns
* @see AWS API Documentation
*/
java.util.concurrent.Future listDomainDeliverabilityCampaignsAsync(
ListDomainDeliverabilityCampaignsRequest listDomainDeliverabilityCampaignsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all of the email identities that are associated with your Amazon Pinpoint account. An identity
* can be either an email address or a domain. This operation returns identities that are verified as well as those
* that aren't.
*
*
* @param listEmailIdentitiesRequest
* A request to list all of the email identities associated with your Amazon Pinpoint account. This list
* includes identities that you've already verified, identities that are unverified, and identities that were
* verified in the past, but are no longer verified.
* @return A Java Future containing the result of the ListEmailIdentities operation returned by the service.
* @sample AmazonPinpointEmailAsync.ListEmailIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listEmailIdentitiesAsync(ListEmailIdentitiesRequest listEmailIdentitiesRequest);
/**
*
* Returns a list of all of the email identities that are associated with your Amazon Pinpoint account. An identity
* can be either an email address or a domain. This operation returns identities that are verified as well as those
* that aren't.
*
*
* @param listEmailIdentitiesRequest
* A request to list all of the email identities associated with your Amazon Pinpoint account. This list
* includes identities that you've already verified, identities that are unverified, and identities that were
* verified in the past, but are no longer verified.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEmailIdentities operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.ListEmailIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listEmailIdentitiesAsync(ListEmailIdentitiesRequest listEmailIdentitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve a list of the tags (keys and values) that are associated with a specified resource. A tag is a
* label that you optionally define and associate with a resource in Amazon Pinpoint. Each tag consists of a
* required tag key and an optional associated tag value. A tag key is a general label that acts as a
* category for more specific tag values. A tag value acts as a descriptor within a tag key.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonPinpointEmailAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieve a list of the tags (keys and values) that are associated with a specified resource. A tag is a
* label that you optionally define and associate with a resource in Amazon Pinpoint. Each tag consists of a
* required tag key and an optional associated tag value. A tag key is a general label that acts as a
* category for more specific tag values. A tag value acts as a descriptor within a tag key.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable the automatic warm-up feature for dedicated IP addresses.
*
*
* @param putAccountDedicatedIpWarmupAttributesRequest
* A request to enable or disable the automatic IP address warm-up feature.
* @return A Java Future containing the result of the PutAccountDedicatedIpWarmupAttributes operation returned by
* the service.
* @sample AmazonPinpointEmailAsync.PutAccountDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountDedicatedIpWarmupAttributesAsync(
PutAccountDedicatedIpWarmupAttributesRequest putAccountDedicatedIpWarmupAttributesRequest);
/**
*
* Enable or disable the automatic warm-up feature for dedicated IP addresses.
*
*
* @param putAccountDedicatedIpWarmupAttributesRequest
* A request to enable or disable the automatic IP address warm-up feature.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAccountDedicatedIpWarmupAttributes operation returned by
* the service.
* @sample AmazonPinpointEmailAsyncHandler.PutAccountDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountDedicatedIpWarmupAttributesAsync(
PutAccountDedicatedIpWarmupAttributesRequest putAccountDedicatedIpWarmupAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable the ability of your account to send email.
*
*
* @param putAccountSendingAttributesRequest
* A request to change the ability of your account to send email.
* @return A Java Future containing the result of the PutAccountSendingAttributes operation returned by the service.
* @sample AmazonPinpointEmailAsync.PutAccountSendingAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountSendingAttributesAsync(
PutAccountSendingAttributesRequest putAccountSendingAttributesRequest);
/**
*
* Enable or disable the ability of your account to send email.
*
*
* @param putAccountSendingAttributesRequest
* A request to change the ability of your account to send email.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAccountSendingAttributes operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.PutAccountSendingAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountSendingAttributesAsync(
PutAccountSendingAttributesRequest putAccountSendingAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate a configuration set with a dedicated IP pool. You can use dedicated IP pools to create groups of
* dedicated IP addresses for sending specific types of email.
*
*
* @param putConfigurationSetDeliveryOptionsRequest
* A request to associate a configuration set with a dedicated IP pool.
* @return A Java Future containing the result of the PutConfigurationSetDeliveryOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.PutConfigurationSetDeliveryOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetDeliveryOptionsAsync(
PutConfigurationSetDeliveryOptionsRequest putConfigurationSetDeliveryOptionsRequest);
/**
*
* Associate a configuration set with a dedicated IP pool. You can use dedicated IP pools to create groups of
* dedicated IP addresses for sending specific types of email.
*
*
* @param putConfigurationSetDeliveryOptionsRequest
* A request to associate a configuration set with a dedicated IP pool.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfigurationSetDeliveryOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.PutConfigurationSetDeliveryOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetDeliveryOptionsAsync(
PutConfigurationSetDeliveryOptionsRequest putConfigurationSetDeliveryOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable collection of reputation metrics for emails that you send using a particular configuration set
* in a specific AWS Region.
*
*
* @param putConfigurationSetReputationOptionsRequest
* A request to enable or disable tracking of reputation metrics for a configuration set.
* @return A Java Future containing the result of the PutConfigurationSetReputationOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.PutConfigurationSetReputationOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetReputationOptionsAsync(
PutConfigurationSetReputationOptionsRequest putConfigurationSetReputationOptionsRequest);
/**
*
* Enable or disable collection of reputation metrics for emails that you send using a particular configuration set
* in a specific AWS Region.
*
*
* @param putConfigurationSetReputationOptionsRequest
* A request to enable or disable tracking of reputation metrics for a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfigurationSetReputationOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.PutConfigurationSetReputationOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetReputationOptionsAsync(
PutConfigurationSetReputationOptionsRequest putConfigurationSetReputationOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable email sending for messages that use a particular configuration set in a specific AWS Region.
*
*
* @param putConfigurationSetSendingOptionsRequest
* A request to enable or disable the ability of Amazon Pinpoint to send emails that use a specific
* configuration set.
* @return A Java Future containing the result of the PutConfigurationSetSendingOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.PutConfigurationSetSendingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetSendingOptionsAsync(
PutConfigurationSetSendingOptionsRequest putConfigurationSetSendingOptionsRequest);
/**
*
* Enable or disable email sending for messages that use a particular configuration set in a specific AWS Region.
*
*
* @param putConfigurationSetSendingOptionsRequest
* A request to enable or disable the ability of Amazon Pinpoint to send emails that use a specific
* configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfigurationSetSendingOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.PutConfigurationSetSendingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetSendingOptionsAsync(
PutConfigurationSetSendingOptionsRequest putConfigurationSetSendingOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specify a custom domain to use for open and click tracking elements in email that you send using Amazon Pinpoint.
*
*
* @param putConfigurationSetTrackingOptionsRequest
* A request to add a custom domain for tracking open and click events to a configuration set.
* @return A Java Future containing the result of the PutConfigurationSetTrackingOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.PutConfigurationSetTrackingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetTrackingOptionsAsync(
PutConfigurationSetTrackingOptionsRequest putConfigurationSetTrackingOptionsRequest);
/**
*
* Specify a custom domain to use for open and click tracking elements in email that you send using Amazon Pinpoint.
*
*
* @param putConfigurationSetTrackingOptionsRequest
* A request to add a custom domain for tracking open and click events to a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfigurationSetTrackingOptions operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.PutConfigurationSetTrackingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetTrackingOptionsAsync(
PutConfigurationSetTrackingOptionsRequest putConfigurationSetTrackingOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Move a dedicated IP address to an existing dedicated IP pool.
*
*
*
* The dedicated IP address that you specify must already exist, and must be associated with your Amazon Pinpoint
* account.
*
*
* The dedicated IP pool you specify must already exist. You can create a new pool by using the
* CreateDedicatedIpPool
operation.
*
*
*
* @param putDedicatedIpInPoolRequest
* A request to move a dedicated IP address to a dedicated IP pool.
* @return A Java Future containing the result of the PutDedicatedIpInPool operation returned by the service.
* @sample AmazonPinpointEmailAsync.PutDedicatedIpInPool
* @see AWS API Documentation
*/
java.util.concurrent.Future putDedicatedIpInPoolAsync(PutDedicatedIpInPoolRequest putDedicatedIpInPoolRequest);
/**
*
* Move a dedicated IP address to an existing dedicated IP pool.
*
*
*
* The dedicated IP address that you specify must already exist, and must be associated with your Amazon Pinpoint
* account.
*
*
* The dedicated IP pool you specify must already exist. You can create a new pool by using the
* CreateDedicatedIpPool
operation.
*
*
*
* @param putDedicatedIpInPoolRequest
* A request to move a dedicated IP address to a dedicated IP pool.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutDedicatedIpInPool operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.PutDedicatedIpInPool
* @see AWS API Documentation
*/
java.util.concurrent.Future putDedicatedIpInPoolAsync(PutDedicatedIpInPoolRequest putDedicatedIpInPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* @param putDedicatedIpWarmupAttributesRequest
* A request to change the warm-up attributes for a dedicated IP address. This operation is useful when you
* want to resume the warm-up process for an existing IP address.
* @return A Java Future containing the result of the PutDedicatedIpWarmupAttributes operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.PutDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putDedicatedIpWarmupAttributesAsync(
PutDedicatedIpWarmupAttributesRequest putDedicatedIpWarmupAttributesRequest);
/**
*
*
* @param putDedicatedIpWarmupAttributesRequest
* A request to change the warm-up attributes for a dedicated IP address. This operation is useful when you
* want to resume the warm-up process for an existing IP address.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutDedicatedIpWarmupAttributes operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.PutDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putDedicatedIpWarmupAttributesAsync(
PutDedicatedIpWarmupAttributesRequest putDedicatedIpWarmupAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable the Deliverability dashboard for your Amazon Pinpoint account. When you enable the
* Deliverability dashboard, you gain access to reputation, deliverability, and other metrics for the domains that
* you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox placement
* tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon Pinpoint. For more information about the features and cost of a Deliverability
* dashboard subscription, see Amazon Pinpoint Pricing.
*
*
* @param putDeliverabilityDashboardOptionRequest
* Enable or disable the Deliverability dashboard for your Amazon Pinpoint account. When you enable the
* Deliverability dashboard, you gain access to reputation, deliverability, and other metrics for the domains
* that you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox
* placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon Pinpoint. For more information about the features and cost of a
* Deliverability dashboard subscription, see Amazon
* Pinpoint Pricing.
* @return A Java Future containing the result of the PutDeliverabilityDashboardOption operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.PutDeliverabilityDashboardOption
* @see AWS API Documentation
*/
java.util.concurrent.Future putDeliverabilityDashboardOptionAsync(
PutDeliverabilityDashboardOptionRequest putDeliverabilityDashboardOptionRequest);
/**
*
* Enable or disable the Deliverability dashboard for your Amazon Pinpoint account. When you enable the
* Deliverability dashboard, you gain access to reputation, deliverability, and other metrics for the domains that
* you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox placement
* tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon Pinpoint. For more information about the features and cost of a Deliverability
* dashboard subscription, see Amazon Pinpoint Pricing.
*
*
* @param putDeliverabilityDashboardOptionRequest
* Enable or disable the Deliverability dashboard for your Amazon Pinpoint account. When you enable the
* Deliverability dashboard, you gain access to reputation, deliverability, and other metrics for the domains
* that you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox
* placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon Pinpoint. For more information about the features and cost of a
* Deliverability dashboard subscription, see Amazon
* Pinpoint Pricing.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutDeliverabilityDashboardOption operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.PutDeliverabilityDashboardOption
* @see AWS API Documentation
*/
java.util.concurrent.Future putDeliverabilityDashboardOptionAsync(
PutDeliverabilityDashboardOptionRequest putDeliverabilityDashboardOptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to enable or disable DKIM authentication for an email identity.
*
*
* @param putEmailIdentityDkimAttributesRequest
* A request to enable or disable DKIM signing of email that you send from an email identity.
* @return A Java Future containing the result of the PutEmailIdentityDkimAttributes operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.PutEmailIdentityDkimAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityDkimAttributesAsync(
PutEmailIdentityDkimAttributesRequest putEmailIdentityDkimAttributesRequest);
/**
*
* Used to enable or disable DKIM authentication for an email identity.
*
*
* @param putEmailIdentityDkimAttributesRequest
* A request to enable or disable DKIM signing of email that you send from an email identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEmailIdentityDkimAttributes operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.PutEmailIdentityDkimAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityDkimAttributesAsync(
PutEmailIdentityDkimAttributesRequest putEmailIdentityDkimAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to enable or disable feedback forwarding for an identity. This setting determines what happens when an
* identity is used to send an email that results in a bounce or complaint event.
*
*
* When you enable feedback forwarding, Amazon Pinpoint sends you email notifications when bounce or complaint
* events occur. Amazon Pinpoint sends this notification to the address that you specified in the Return-Path header
* of the original email.
*
*
* When you disable feedback forwarding, Amazon Pinpoint sends notifications through other mechanisms, such as by
* notifying an Amazon SNS topic. You're required to have a method of tracking bounces and complaints. If you
* haven't set up another mechanism for receiving bounce or complaint notifications, Amazon Pinpoint sends an email
* notification when these events occur (even if this setting is disabled).
*
*
* @param putEmailIdentityFeedbackAttributesRequest
* A request to set the attributes that control how bounce and complaint events are processed.
* @return A Java Future containing the result of the PutEmailIdentityFeedbackAttributes operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.PutEmailIdentityFeedbackAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityFeedbackAttributesAsync(
PutEmailIdentityFeedbackAttributesRequest putEmailIdentityFeedbackAttributesRequest);
/**
*
* Used to enable or disable feedback forwarding for an identity. This setting determines what happens when an
* identity is used to send an email that results in a bounce or complaint event.
*
*
* When you enable feedback forwarding, Amazon Pinpoint sends you email notifications when bounce or complaint
* events occur. Amazon Pinpoint sends this notification to the address that you specified in the Return-Path header
* of the original email.
*
*
* When you disable feedback forwarding, Amazon Pinpoint sends notifications through other mechanisms, such as by
* notifying an Amazon SNS topic. You're required to have a method of tracking bounces and complaints. If you
* haven't set up another mechanism for receiving bounce or complaint notifications, Amazon Pinpoint sends an email
* notification when these events occur (even if this setting is disabled).
*
*
* @param putEmailIdentityFeedbackAttributesRequest
* A request to set the attributes that control how bounce and complaint events are processed.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEmailIdentityFeedbackAttributes operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.PutEmailIdentityFeedbackAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityFeedbackAttributesAsync(
PutEmailIdentityFeedbackAttributesRequest putEmailIdentityFeedbackAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to enable or disable the custom Mail-From domain configuration for an email identity.
*
*
* @param putEmailIdentityMailFromAttributesRequest
* A request to configure the custom MAIL FROM domain for a verified identity.
* @return A Java Future containing the result of the PutEmailIdentityMailFromAttributes operation returned by the
* service.
* @sample AmazonPinpointEmailAsync.PutEmailIdentityMailFromAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityMailFromAttributesAsync(
PutEmailIdentityMailFromAttributesRequest putEmailIdentityMailFromAttributesRequest);
/**
*
* Used to enable or disable the custom Mail-From domain configuration for an email identity.
*
*
* @param putEmailIdentityMailFromAttributesRequest
* A request to configure the custom MAIL FROM domain for a verified identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEmailIdentityMailFromAttributes operation returned by the
* service.
* @sample AmazonPinpointEmailAsyncHandler.PutEmailIdentityMailFromAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityMailFromAttributesAsync(
PutEmailIdentityMailFromAttributesRequest putEmailIdentityMailFromAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends an email message. You can use the Amazon Pinpoint Email API to send two types of messages:
*
*
* -
*
* Simple – A standard email message. When you create this type of message, you specify the sender, the
* recipient, and the message body, and Amazon Pinpoint assembles the message for you.
*
*
* -
*
* Raw – A raw, MIME-formatted email message. When you send this type of email, you have to specify all of
* the message headers, as well as the message body. You can use this message type to send messages that contain
* attachments. The message that you specify has to be a valid MIME message.
*
*
*
*
* @param sendEmailRequest
* A request to send an email message.
* @return A Java Future containing the result of the SendEmail operation returned by the service.
* @sample AmazonPinpointEmailAsync.SendEmail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future sendEmailAsync(SendEmailRequest sendEmailRequest);
/**
*
* Sends an email message. You can use the Amazon Pinpoint Email API to send two types of messages:
*
*
* -
*
* Simple – A standard email message. When you create this type of message, you specify the sender, the
* recipient, and the message body, and Amazon Pinpoint assembles the message for you.
*
*
* -
*
* Raw – A raw, MIME-formatted email message. When you send this type of email, you have to specify all of
* the message headers, as well as the message body. You can use this message type to send messages that contain
* attachments. The message that you specify has to be a valid MIME message.
*
*
*
*
* @param sendEmailRequest
* A request to send an email message.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendEmail operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.SendEmail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future sendEmailAsync(SendEmailRequest sendEmailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Add one or more tags (keys and values) to a specified resource. A tag is a label that you optionally
* define and associate with a resource in Amazon Pinpoint. Tags can help you categorize and manage resources in
* different ways, such as by purpose, owner, environment, or other criteria. A resource can have as many as 50
* tags.
*
*
* Each tag consists of a required tag key and an associated tag value, both of which you define. A
* tag key is a general label that acts as a category for more specific tag values. A tag value acts as a descriptor
* within a tag key.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonPinpointEmailAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Add one or more tags (keys and values) to a specified resource. A tag is a label that you optionally
* define and associate with a resource in Amazon Pinpoint. Tags can help you categorize and manage resources in
* different ways, such as by purpose, owner, environment, or other criteria. A resource can have as many as 50
* tags.
*
*
* Each tag consists of a required tag key and an associated tag value, both of which you define. A
* tag key is a general label that acts as a category for more specific tag values. A tag value acts as a descriptor
* within a tag key.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove one or more tags (keys and values) from a specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonPinpointEmailAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Remove one or more tags (keys and values) from a specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonPinpointEmailAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update the configuration of an event destination for a configuration set.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param updateConfigurationSetEventDestinationRequest
* A request to change the settings for an event destination for a configuration set.
* @return A Java Future containing the result of the UpdateConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonPinpointEmailAsync.UpdateConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future updateConfigurationSetEventDestinationAsync(
UpdateConfigurationSetEventDestinationRequest updateConfigurationSetEventDestinationRequest);
/**
*
* Update the configuration of an event destination for a configuration set.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param updateConfigurationSetEventDestinationRequest
* A request to change the settings for an event destination for a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonPinpointEmailAsyncHandler.UpdateConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future updateConfigurationSetEventDestinationAsync(
UpdateConfigurationSetEventDestinationRequest updateConfigurationSetEventDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}