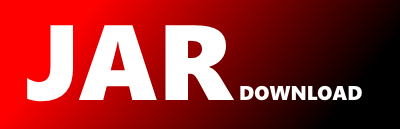
com.amazonaws.services.pinpointemail.AmazonPinpointEmailClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pinpointemail Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointemail;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.pinpointemail.AmazonPinpointEmailClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.pinpointemail.model.*;
import com.amazonaws.services.pinpointemail.model.transform.*;
/**
* Client for accessing Pinpoint Email. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
* Amazon Pinpoint Email Service
*
* Welcome to the Amazon Pinpoint Email API Reference. This guide provides information about the Amazon Pinpoint
* Email API (version 1.0), including supported operations, data types, parameters, and schemas.
*
*
* Amazon Pinpoint is an AWS service that you can use to engage with your
* customers across multiple messaging channels. You can use Amazon Pinpoint to send email, SMS text messages, voice
* messages, and push notifications. The Amazon Pinpoint Email API provides programmatic access to options that are
* unique to the email channel and supplement the options provided by the Amazon Pinpoint API.
*
*
* If you're new to Amazon Pinpoint, you might find it helpful to also review the Amazon Pinpoint Developer Guide.
* The Amazon Pinpoint Developer Guide provides tutorials, code samples, and procedures that demonstrate how to
* use Amazon Pinpoint features programmatically and how to integrate Amazon Pinpoint functionality into mobile apps and
* other types of applications. The guide also provides information about key topics such as Amazon Pinpoint integration
* with other AWS services and the limits that apply to using the service.
*
*
* The Amazon Pinpoint Email API is available in several AWS Regions and it provides an endpoint for each of these
* Regions. For a list of all the Regions and endpoints where the API is currently available, see AWS Service Endpoints in the
* Amazon Web Services General Reference. To learn more about AWS Regions, see Managing AWS Regions in the Amazon Web
* Services General Reference.
*
*
* In each Region, AWS maintains multiple Availability Zones. These Availability Zones are physically isolated from each
* other, but are united by private, low-latency, high-throughput, and highly redundant network connections. These
* Availability Zones enable us to provide very high levels of availability and redundancy, while also minimizing
* latency. To learn more about the number of Availability Zones that are available in each Region, see AWS Global Infrastructure.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonPinpointEmailClient extends AmazonWebServiceClient implements AmazonPinpointEmail {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonPinpointEmail.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "ses";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConcurrentModificationException").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.ConcurrentModificationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MessageRejected").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.MessageRejectedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.NotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("SendingPausedException").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.SendingPausedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccountSuspendedException").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.AccountSuspendedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.AlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyRequestsException").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.TooManyRequestsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MailFromDomainNotVerifiedException").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.MailFromDomainNotVerifiedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withExceptionUnmarshaller(
com.amazonaws.services.pinpointemail.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.pinpointemail.model.AmazonPinpointEmailException.class));
public static AmazonPinpointEmailClientBuilder builder() {
return AmazonPinpointEmailClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Pinpoint Email using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonPinpointEmailClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Pinpoint Email using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonPinpointEmailClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("email.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/pinpointemail/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/pinpointemail/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Create a configuration set. Configuration sets are groups of rules that you can apply to the emails you
* send using Amazon Pinpoint. You apply a configuration set to an email by including a reference to the
* configuration set in the headers of the email. When you apply a configuration set to an email, all of the rules
* in that configuration set are applied to the email.
*
*
* @param createConfigurationSetRequest
* A request to create a configuration set.
* @return Result of the CreateConfigurationSet operation returned by the service.
* @throws AlreadyExistsException
* The resource specified in your request already exists.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws LimitExceededException
* There are too many instances of the specified resource type.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ConcurrentModificationException
* The resource is being modified by another operation or thread.
* @sample AmazonPinpointEmail.CreateConfigurationSet
* @see AWS API Documentation
*/
@Override
public CreateConfigurationSetResult createConfigurationSet(CreateConfigurationSetRequest request) {
request = beforeClientExecution(request);
return executeCreateConfigurationSet(request);
}
@SdkInternalApi
final CreateConfigurationSetResult executeCreateConfigurationSet(CreateConfigurationSetRequest createConfigurationSetRequest) {
ExecutionContext executionContext = createExecutionContext(createConfigurationSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConfigurationSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createConfigurationSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateConfigurationSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateConfigurationSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create an event destination. In Amazon Pinpoint, events include message sends, deliveries, opens, clicks,
* bounces, and complaints. Event destinations are places that you can send information about these events
* to. For example, you can send event data to Amazon SNS to receive notifications when you receive bounces or
* complaints, or you can use Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* A single configuration set can include more than one event destination.
*
*
* @param createConfigurationSetEventDestinationRequest
* A request to add an event destination to a configuration set.
* @return Result of the CreateConfigurationSetEventDestination operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws AlreadyExistsException
* The resource specified in your request already exists.
* @throws LimitExceededException
* There are too many instances of the specified resource type.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.CreateConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public CreateConfigurationSetEventDestinationResult createConfigurationSetEventDestination(CreateConfigurationSetEventDestinationRequest request) {
request = beforeClientExecution(request);
return executeCreateConfigurationSetEventDestination(request);
}
@SdkInternalApi
final CreateConfigurationSetEventDestinationResult executeCreateConfigurationSetEventDestination(
CreateConfigurationSetEventDestinationRequest createConfigurationSetEventDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(createConfigurationSetEventDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConfigurationSetEventDestinationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createConfigurationSetEventDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateConfigurationSetEventDestination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateConfigurationSetEventDestinationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a new pool of dedicated IP addresses. A pool can include one or more dedicated IP addresses that are
* associated with your Amazon Pinpoint account. You can associate a pool with a configuration set. When you send an
* email that uses that configuration set, Amazon Pinpoint sends it using only the IP addresses in the associated
* pool.
*
*
* @param createDedicatedIpPoolRequest
* A request to create a new dedicated IP pool.
* @return Result of the CreateDedicatedIpPool operation returned by the service.
* @throws AlreadyExistsException
* The resource specified in your request already exists.
* @throws LimitExceededException
* There are too many instances of the specified resource type.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ConcurrentModificationException
* The resource is being modified by another operation or thread.
* @sample AmazonPinpointEmail.CreateDedicatedIpPool
* @see AWS API Documentation
*/
@Override
public CreateDedicatedIpPoolResult createDedicatedIpPool(CreateDedicatedIpPoolRequest request) {
request = beforeClientExecution(request);
return executeCreateDedicatedIpPool(request);
}
@SdkInternalApi
final CreateDedicatedIpPoolResult executeCreateDedicatedIpPool(CreateDedicatedIpPoolRequest createDedicatedIpPoolRequest) {
ExecutionContext executionContext = createExecutionContext(createDedicatedIpPoolRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDedicatedIpPoolRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDedicatedIpPoolRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDedicatedIpPool");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateDedicatedIpPoolResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a new predictive inbox placement test. Predictive inbox placement tests can help you predict how your
* messages will be handled by various email providers around the world. When you perform a predictive inbox
* placement test, you provide a sample message that contains the content that you plan to send to your customers.
* Amazon Pinpoint then sends that message to special email addresses spread across several major email providers.
* After about 24 hours, the test is complete, and you can use the GetDeliverabilityTestReport
* operation to view the results of the test.
*
*
* @param createDeliverabilityTestReportRequest
* A request to perform a predictive inbox placement test. Predictive inbox placement tests can help you
* predict how your messages will be handled by various email providers around the world. When you perform a
* predictive inbox placement test, you provide a sample message that contains the content that you plan to
* send to your customers. Amazon Pinpoint then sends that message to special email addresses spread across
* several major email providers. After about 24 hours, the test is complete, and you can use the
* GetDeliverabilityTestReport
operation to view the results of the test.
* @return Result of the CreateDeliverabilityTestReport operation returned by the service.
* @throws AccountSuspendedException
* The message can't be sent because the account's ability to send email has been permanently restricted.
* @throws SendingPausedException
* The message can't be sent because the account's ability to send email is currently paused.
* @throws MessageRejectedException
* The message can't be sent because it contains invalid content.
* @throws MailFromDomainNotVerifiedException
* The message can't be sent because the sending domain isn't verified.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws LimitExceededException
* There are too many instances of the specified resource type.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ConcurrentModificationException
* The resource is being modified by another operation or thread.
* @sample AmazonPinpointEmail.CreateDeliverabilityTestReport
* @see AWS API Documentation
*/
@Override
public CreateDeliverabilityTestReportResult createDeliverabilityTestReport(CreateDeliverabilityTestReportRequest request) {
request = beforeClientExecution(request);
return executeCreateDeliverabilityTestReport(request);
}
@SdkInternalApi
final CreateDeliverabilityTestReportResult executeCreateDeliverabilityTestReport(CreateDeliverabilityTestReportRequest createDeliverabilityTestReportRequest) {
ExecutionContext executionContext = createExecutionContext(createDeliverabilityTestReportRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDeliverabilityTestReportRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createDeliverabilityTestReportRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDeliverabilityTestReport");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateDeliverabilityTestReportResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Verifies an email identity for use with Amazon Pinpoint. In Amazon Pinpoint, an identity is an email address or
* domain that you use when you send email. Before you can use an identity to send email with Amazon Pinpoint, you
* first have to verify it. By verifying an address, you demonstrate that you're the owner of the address, and that
* you've given Amazon Pinpoint permission to send email from the address.
*
*
* When you verify an email address, Amazon Pinpoint sends an email to the address. Your email address is verified
* as soon as you follow the link in the verification email.
*
*
* When you verify a domain, this operation provides a set of DKIM tokens, which you can convert into CNAME tokens.
* You add these CNAME tokens to the DNS configuration for your domain. Your domain is verified when Amazon Pinpoint
* detects these records in the DNS configuration for your domain. It usually takes around 72 hours to complete the
* domain verification process.
*
*
* @param createEmailIdentityRequest
* A request to begin the verification process for an email identity (an email address or domain).
* @return Result of the CreateEmailIdentity operation returned by the service.
* @throws LimitExceededException
* There are too many instances of the specified resource type.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ConcurrentModificationException
* The resource is being modified by another operation or thread.
* @sample AmazonPinpointEmail.CreateEmailIdentity
* @see AWS API Documentation
*/
@Override
public CreateEmailIdentityResult createEmailIdentity(CreateEmailIdentityRequest request) {
request = beforeClientExecution(request);
return executeCreateEmailIdentity(request);
}
@SdkInternalApi
final CreateEmailIdentityResult executeCreateEmailIdentity(CreateEmailIdentityRequest createEmailIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(createEmailIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEmailIdentityRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createEmailIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEmailIdentity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateEmailIdentityResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an existing configuration set.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param deleteConfigurationSetRequest
* A request to delete a configuration set.
* @return Result of the DeleteConfigurationSet operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ConcurrentModificationException
* The resource is being modified by another operation or thread.
* @sample AmazonPinpointEmail.DeleteConfigurationSet
* @see AWS API Documentation
*/
@Override
public DeleteConfigurationSetResult deleteConfigurationSet(DeleteConfigurationSetRequest request) {
request = beforeClientExecution(request);
return executeDeleteConfigurationSet(request);
}
@SdkInternalApi
final DeleteConfigurationSetResult executeDeleteConfigurationSet(DeleteConfigurationSetRequest deleteConfigurationSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConfigurationSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConfigurationSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteConfigurationSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteConfigurationSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteConfigurationSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an event destination.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param deleteConfigurationSetEventDestinationRequest
* A request to delete an event destination from a configuration set.
* @return Result of the DeleteConfigurationSetEventDestination operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.DeleteConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public DeleteConfigurationSetEventDestinationResult deleteConfigurationSetEventDestination(DeleteConfigurationSetEventDestinationRequest request) {
request = beforeClientExecution(request);
return executeDeleteConfigurationSetEventDestination(request);
}
@SdkInternalApi
final DeleteConfigurationSetEventDestinationResult executeDeleteConfigurationSetEventDestination(
DeleteConfigurationSetEventDestinationRequest deleteConfigurationSetEventDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConfigurationSetEventDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConfigurationSetEventDestinationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteConfigurationSetEventDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteConfigurationSetEventDestination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteConfigurationSetEventDestinationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete a dedicated IP pool.
*
*
* @param deleteDedicatedIpPoolRequest
* A request to delete a dedicated IP pool.
* @return Result of the DeleteDedicatedIpPool operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ConcurrentModificationException
* The resource is being modified by another operation or thread.
* @sample AmazonPinpointEmail.DeleteDedicatedIpPool
* @see AWS API Documentation
*/
@Override
public DeleteDedicatedIpPoolResult deleteDedicatedIpPool(DeleteDedicatedIpPoolRequest request) {
request = beforeClientExecution(request);
return executeDeleteDedicatedIpPool(request);
}
@SdkInternalApi
final DeleteDedicatedIpPoolResult executeDeleteDedicatedIpPool(DeleteDedicatedIpPoolRequest deleteDedicatedIpPoolRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDedicatedIpPoolRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDedicatedIpPoolRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDedicatedIpPoolRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDedicatedIpPool");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteDedicatedIpPoolResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an email identity that you previously verified for use with Amazon Pinpoint. An identity can be either an
* email address or a domain name.
*
*
* @param deleteEmailIdentityRequest
* A request to delete an existing email identity. When you delete an identity, you lose the ability to use
* Amazon Pinpoint to send email from that identity. You can restore your ability to send email by completing
* the verification process for the identity again.
* @return Result of the DeleteEmailIdentity operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ConcurrentModificationException
* The resource is being modified by another operation or thread.
* @sample AmazonPinpointEmail.DeleteEmailIdentity
* @see AWS API Documentation
*/
@Override
public DeleteEmailIdentityResult deleteEmailIdentity(DeleteEmailIdentityRequest request) {
request = beforeClientExecution(request);
return executeDeleteEmailIdentity(request);
}
@SdkInternalApi
final DeleteEmailIdentityResult executeDeleteEmailIdentity(DeleteEmailIdentityRequest deleteEmailIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEmailIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEmailIdentityRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteEmailIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEmailIdentity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteEmailIdentityResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Obtain information about the email-sending status and capabilities of your Amazon Pinpoint account in the current
* AWS Region.
*
*
* @param getAccountRequest
* A request to obtain information about the email-sending capabilities of your Amazon Pinpoint account.
* @return Result of the GetAccount operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetAccount
* @see AWS API
* Documentation
*/
@Override
public GetAccountResult getAccount(GetAccountRequest request) {
request = beforeClientExecution(request);
return executeGetAccount(request);
}
@SdkInternalApi
final GetAccountResult executeGetAccount(GetAccountRequest getAccountRequest) {
ExecutionContext executionContext = createExecutionContext(getAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccountRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieve a list of the blacklists that your dedicated IP addresses appear on.
*
*
* @param getBlacklistReportsRequest
* A request to retrieve a list of the blacklists that your dedicated IP addresses appear on.
* @return Result of the GetBlacklistReports operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetBlacklistReports
* @see AWS API Documentation
*/
@Override
public GetBlacklistReportsResult getBlacklistReports(GetBlacklistReportsRequest request) {
request = beforeClientExecution(request);
return executeGetBlacklistReports(request);
}
@SdkInternalApi
final GetBlacklistReportsResult executeGetBlacklistReports(GetBlacklistReportsRequest getBlacklistReportsRequest) {
ExecutionContext executionContext = createExecutionContext(getBlacklistReportsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetBlacklistReportsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getBlacklistReportsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBlacklistReports");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetBlacklistReportsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get information about an existing configuration set, including the dedicated IP pool that it's associated with,
* whether or not it's enabled for sending email, and more.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param getConfigurationSetRequest
* A request to obtain information about a configuration set.
* @return Result of the GetConfigurationSet operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetConfigurationSet
* @see AWS API Documentation
*/
@Override
public GetConfigurationSetResult getConfigurationSet(GetConfigurationSetRequest request) {
request = beforeClientExecution(request);
return executeGetConfigurationSet(request);
}
@SdkInternalApi
final GetConfigurationSetResult executeGetConfigurationSet(GetConfigurationSetRequest getConfigurationSetRequest) {
ExecutionContext executionContext = createExecutionContext(getConfigurationSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetConfigurationSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getConfigurationSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetConfigurationSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetConfigurationSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieve a list of event destinations that are associated with a configuration set.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param getConfigurationSetEventDestinationsRequest
* A request to obtain information about the event destinations for a configuration set.
* @return Result of the GetConfigurationSetEventDestinations operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetConfigurationSetEventDestinations
* @see AWS API Documentation
*/
@Override
public GetConfigurationSetEventDestinationsResult getConfigurationSetEventDestinations(GetConfigurationSetEventDestinationsRequest request) {
request = beforeClientExecution(request);
return executeGetConfigurationSetEventDestinations(request);
}
@SdkInternalApi
final GetConfigurationSetEventDestinationsResult executeGetConfigurationSetEventDestinations(
GetConfigurationSetEventDestinationsRequest getConfigurationSetEventDestinationsRequest) {
ExecutionContext executionContext = createExecutionContext(getConfigurationSetEventDestinationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetConfigurationSetEventDestinationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getConfigurationSetEventDestinationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetConfigurationSetEventDestinations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetConfigurationSetEventDestinationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get information about a dedicated IP address, including the name of the dedicated IP pool that it's associated
* with, as well information about the automatic warm-up process for the address.
*
*
* @param getDedicatedIpRequest
* A request to obtain more information about a dedicated IP address.
* @return Result of the GetDedicatedIp operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetDedicatedIp
* @see AWS
* API Documentation
*/
@Override
public GetDedicatedIpResult getDedicatedIp(GetDedicatedIpRequest request) {
request = beforeClientExecution(request);
return executeGetDedicatedIp(request);
}
@SdkInternalApi
final GetDedicatedIpResult executeGetDedicatedIp(GetDedicatedIpRequest getDedicatedIpRequest) {
ExecutionContext executionContext = createExecutionContext(getDedicatedIpRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDedicatedIpRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDedicatedIpRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDedicatedIp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDedicatedIpResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List the dedicated IP addresses that are associated with your Amazon Pinpoint account.
*
*
* @param getDedicatedIpsRequest
* A request to obtain more information about dedicated IP pools.
* @return Result of the GetDedicatedIps operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetDedicatedIps
* @see AWS
* API Documentation
*/
@Override
public GetDedicatedIpsResult getDedicatedIps(GetDedicatedIpsRequest request) {
request = beforeClientExecution(request);
return executeGetDedicatedIps(request);
}
@SdkInternalApi
final GetDedicatedIpsResult executeGetDedicatedIps(GetDedicatedIpsRequest getDedicatedIpsRequest) {
ExecutionContext executionContext = createExecutionContext(getDedicatedIpsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDedicatedIpsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDedicatedIpsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDedicatedIps");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDedicatedIpsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieve information about the status of the Deliverability dashboard for your Amazon Pinpoint account. When the
* Deliverability dashboard is enabled, you gain access to reputation, deliverability, and other metrics for the
* domains that you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox
* placement tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon Pinpoint. For more information about the features and cost of a Deliverability
* dashboard subscription, see Amazon Pinpoint Pricing.
*
*
* @param getDeliverabilityDashboardOptionsRequest
* Retrieve information about the status of the Deliverability dashboard for your Amazon Pinpoint account.
* When the Deliverability dashboard is enabled, you gain access to reputation, deliverability, and other
* metrics for the domains that you use to send email using Amazon Pinpoint. You also gain the ability to
* perform predictive inbox placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon Pinpoint. For more information about the features and cost of a
* Deliverability dashboard subscription, see Amazon
* Pinpoint Pricing.
* @return Result of the GetDeliverabilityDashboardOptions operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws LimitExceededException
* There are too many instances of the specified resource type.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetDeliverabilityDashboardOptions
* @see AWS API Documentation
*/
@Override
public GetDeliverabilityDashboardOptionsResult getDeliverabilityDashboardOptions(GetDeliverabilityDashboardOptionsRequest request) {
request = beforeClientExecution(request);
return executeGetDeliverabilityDashboardOptions(request);
}
@SdkInternalApi
final GetDeliverabilityDashboardOptionsResult executeGetDeliverabilityDashboardOptions(
GetDeliverabilityDashboardOptionsRequest getDeliverabilityDashboardOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(getDeliverabilityDashboardOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDeliverabilityDashboardOptionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getDeliverabilityDashboardOptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDeliverabilityDashboardOptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetDeliverabilityDashboardOptionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieve the results of a predictive inbox placement test.
*
*
* @param getDeliverabilityTestReportRequest
* A request to retrieve the results of a predictive inbox placement test.
* @return Result of the GetDeliverabilityTestReport operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetDeliverabilityTestReport
* @see AWS API Documentation
*/
@Override
public GetDeliverabilityTestReportResult getDeliverabilityTestReport(GetDeliverabilityTestReportRequest request) {
request = beforeClientExecution(request);
return executeGetDeliverabilityTestReport(request);
}
@SdkInternalApi
final GetDeliverabilityTestReportResult executeGetDeliverabilityTestReport(GetDeliverabilityTestReportRequest getDeliverabilityTestReportRequest) {
ExecutionContext executionContext = createExecutionContext(getDeliverabilityTestReportRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDeliverabilityTestReportRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getDeliverabilityTestReportRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDeliverabilityTestReport");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetDeliverabilityTestReportResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only if the
* campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
*
*
* @param getDomainDeliverabilityCampaignRequest
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only
* if the campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
* @return Result of the GetDomainDeliverabilityCampaign operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @sample AmazonPinpointEmail.GetDomainDeliverabilityCampaign
* @see AWS API Documentation
*/
@Override
public GetDomainDeliverabilityCampaignResult getDomainDeliverabilityCampaign(GetDomainDeliverabilityCampaignRequest request) {
request = beforeClientExecution(request);
return executeGetDomainDeliverabilityCampaign(request);
}
@SdkInternalApi
final GetDomainDeliverabilityCampaignResult executeGetDomainDeliverabilityCampaign(
GetDomainDeliverabilityCampaignRequest getDomainDeliverabilityCampaignRequest) {
ExecutionContext executionContext = createExecutionContext(getDomainDeliverabilityCampaignRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDomainDeliverabilityCampaignRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getDomainDeliverabilityCampaignRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDomainDeliverabilityCampaign");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetDomainDeliverabilityCampaignResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieve inbox placement and engagement rates for the domains that you use to send email.
*
*
* @param getDomainStatisticsReportRequest
* A request to obtain deliverability metrics for a domain.
* @return Result of the GetDomainStatisticsReport operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetDomainStatisticsReport
* @see AWS API Documentation
*/
@Override
public GetDomainStatisticsReportResult getDomainStatisticsReport(GetDomainStatisticsReportRequest request) {
request = beforeClientExecution(request);
return executeGetDomainStatisticsReport(request);
}
@SdkInternalApi
final GetDomainStatisticsReportResult executeGetDomainStatisticsReport(GetDomainStatisticsReportRequest getDomainStatisticsReportRequest) {
ExecutionContext executionContext = createExecutionContext(getDomainStatisticsReportRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDomainStatisticsReportRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getDomainStatisticsReportRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDomainStatisticsReport");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetDomainStatisticsReportResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provides information about a specific identity associated with your Amazon Pinpoint account, including the
* identity's verification status, its DKIM authentication status, and its custom Mail-From settings.
*
*
* @param getEmailIdentityRequest
* A request to return details about an email identity.
* @return Result of the GetEmailIdentity operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.GetEmailIdentity
* @see AWS API Documentation
*/
@Override
public GetEmailIdentityResult getEmailIdentity(GetEmailIdentityRequest request) {
request = beforeClientExecution(request);
return executeGetEmailIdentity(request);
}
@SdkInternalApi
final GetEmailIdentityResult executeGetEmailIdentity(GetEmailIdentityRequest getEmailIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(getEmailIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEmailIdentityRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getEmailIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEmailIdentity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetEmailIdentityResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List all of the configuration sets associated with your Amazon Pinpoint account in the current region.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param listConfigurationSetsRequest
* A request to obtain a list of configuration sets for your Amazon Pinpoint account in the current AWS
* Region.
* @return Result of the ListConfigurationSets operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.ListConfigurationSets
* @see AWS API Documentation
*/
@Override
public ListConfigurationSetsResult listConfigurationSets(ListConfigurationSetsRequest request) {
request = beforeClientExecution(request);
return executeListConfigurationSets(request);
}
@SdkInternalApi
final ListConfigurationSetsResult executeListConfigurationSets(ListConfigurationSetsRequest listConfigurationSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listConfigurationSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListConfigurationSetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listConfigurationSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListConfigurationSets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListConfigurationSetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List all of the dedicated IP pools that exist in your Amazon Pinpoint account in the current AWS Region.
*
*
* @param listDedicatedIpPoolsRequest
* A request to obtain a list of dedicated IP pools.
* @return Result of the ListDedicatedIpPools operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.ListDedicatedIpPools
* @see AWS API Documentation
*/
@Override
public ListDedicatedIpPoolsResult listDedicatedIpPools(ListDedicatedIpPoolsRequest request) {
request = beforeClientExecution(request);
return executeListDedicatedIpPools(request);
}
@SdkInternalApi
final ListDedicatedIpPoolsResult executeListDedicatedIpPools(ListDedicatedIpPoolsRequest listDedicatedIpPoolsRequest) {
ExecutionContext executionContext = createExecutionContext(listDedicatedIpPoolsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDedicatedIpPoolsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDedicatedIpPoolsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDedicatedIpPools");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDedicatedIpPoolsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Show a list of the predictive inbox placement tests that you've performed, regardless of their statuses. For
* predictive inbox placement tests that are complete, you can use the GetDeliverabilityTestReport
* operation to view the results.
*
*
* @param listDeliverabilityTestReportsRequest
* A request to list all of the predictive inbox placement tests that you've performed.
* @return Result of the ListDeliverabilityTestReports operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.ListDeliverabilityTestReports
* @see AWS API Documentation
*/
@Override
public ListDeliverabilityTestReportsResult listDeliverabilityTestReports(ListDeliverabilityTestReportsRequest request) {
request = beforeClientExecution(request);
return executeListDeliverabilityTestReports(request);
}
@SdkInternalApi
final ListDeliverabilityTestReportsResult executeListDeliverabilityTestReports(ListDeliverabilityTestReportsRequest listDeliverabilityTestReportsRequest) {
ExecutionContext executionContext = createExecutionContext(listDeliverabilityTestReportsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDeliverabilityTestReportsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listDeliverabilityTestReportsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDeliverabilityTestReports");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListDeliverabilityTestReportsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a specified
* time range. This data is available for a domain only if you enabled the Deliverability dashboard (
* PutDeliverabilityDashboardOption
operation) for the domain.
*
*
* @param listDomainDeliverabilityCampaignsRequest
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a
* specified time range. This data is available for a domain only if you enabled the Deliverability dashboard
* (PutDeliverabilityDashboardOption
operation) for the domain.
* @return Result of the ListDomainDeliverabilityCampaigns operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @sample AmazonPinpointEmail.ListDomainDeliverabilityCampaigns
* @see AWS API Documentation
*/
@Override
public ListDomainDeliverabilityCampaignsResult listDomainDeliverabilityCampaigns(ListDomainDeliverabilityCampaignsRequest request) {
request = beforeClientExecution(request);
return executeListDomainDeliverabilityCampaigns(request);
}
@SdkInternalApi
final ListDomainDeliverabilityCampaignsResult executeListDomainDeliverabilityCampaigns(
ListDomainDeliverabilityCampaignsRequest listDomainDeliverabilityCampaignsRequest) {
ExecutionContext executionContext = createExecutionContext(listDomainDeliverabilityCampaignsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDomainDeliverabilityCampaignsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listDomainDeliverabilityCampaignsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDomainDeliverabilityCampaigns");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListDomainDeliverabilityCampaignsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of all of the email identities that are associated with your Amazon Pinpoint account. An identity
* can be either an email address or a domain. This operation returns identities that are verified as well as those
* that aren't.
*
*
* @param listEmailIdentitiesRequest
* A request to list all of the email identities associated with your Amazon Pinpoint account. This list
* includes identities that you've already verified, identities that are unverified, and identities that were
* verified in the past, but are no longer verified.
* @return Result of the ListEmailIdentities operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.ListEmailIdentities
* @see AWS API Documentation
*/
@Override
public ListEmailIdentitiesResult listEmailIdentities(ListEmailIdentitiesRequest request) {
request = beforeClientExecution(request);
return executeListEmailIdentities(request);
}
@SdkInternalApi
final ListEmailIdentitiesResult executeListEmailIdentities(ListEmailIdentitiesRequest listEmailIdentitiesRequest) {
ExecutionContext executionContext = createExecutionContext(listEmailIdentitiesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEmailIdentitiesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEmailIdentitiesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEmailIdentities");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListEmailIdentitiesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieve a list of the tags (keys and values) that are associated with a specified resource. A tag is a
* label that you optionally define and associate with a resource in Amazon Pinpoint. Each tag consists of a
* required tag key and an optional associated tag value. A tag key is a general label that acts as a
* category for more specific tag values. A tag value acts as a descriptor within a tag key.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @sample AmazonPinpointEmail.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enable or disable the automatic warm-up feature for dedicated IP addresses.
*
*
* @param putAccountDedicatedIpWarmupAttributesRequest
* A request to enable or disable the automatic IP address warm-up feature.
* @return Result of the PutAccountDedicatedIpWarmupAttributes operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutAccountDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
@Override
public PutAccountDedicatedIpWarmupAttributesResult putAccountDedicatedIpWarmupAttributes(PutAccountDedicatedIpWarmupAttributesRequest request) {
request = beforeClientExecution(request);
return executePutAccountDedicatedIpWarmupAttributes(request);
}
@SdkInternalApi
final PutAccountDedicatedIpWarmupAttributesResult executePutAccountDedicatedIpWarmupAttributes(
PutAccountDedicatedIpWarmupAttributesRequest putAccountDedicatedIpWarmupAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(putAccountDedicatedIpWarmupAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutAccountDedicatedIpWarmupAttributesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putAccountDedicatedIpWarmupAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutAccountDedicatedIpWarmupAttributes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutAccountDedicatedIpWarmupAttributesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enable or disable the ability of your account to send email.
*
*
* @param putAccountSendingAttributesRequest
* A request to change the ability of your account to send email.
* @return Result of the PutAccountSendingAttributes operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutAccountSendingAttributes
* @see AWS API Documentation
*/
@Override
public PutAccountSendingAttributesResult putAccountSendingAttributes(PutAccountSendingAttributesRequest request) {
request = beforeClientExecution(request);
return executePutAccountSendingAttributes(request);
}
@SdkInternalApi
final PutAccountSendingAttributesResult executePutAccountSendingAttributes(PutAccountSendingAttributesRequest putAccountSendingAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(putAccountSendingAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutAccountSendingAttributesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putAccountSendingAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutAccountSendingAttributes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutAccountSendingAttributesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associate a configuration set with a dedicated IP pool. You can use dedicated IP pools to create groups of
* dedicated IP addresses for sending specific types of email.
*
*
* @param putConfigurationSetDeliveryOptionsRequest
* A request to associate a configuration set with a dedicated IP pool.
* @return Result of the PutConfigurationSetDeliveryOptions operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutConfigurationSetDeliveryOptions
* @see AWS API Documentation
*/
@Override
public PutConfigurationSetDeliveryOptionsResult putConfigurationSetDeliveryOptions(PutConfigurationSetDeliveryOptionsRequest request) {
request = beforeClientExecution(request);
return executePutConfigurationSetDeliveryOptions(request);
}
@SdkInternalApi
final PutConfigurationSetDeliveryOptionsResult executePutConfigurationSetDeliveryOptions(
PutConfigurationSetDeliveryOptionsRequest putConfigurationSetDeliveryOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(putConfigurationSetDeliveryOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutConfigurationSetDeliveryOptionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putConfigurationSetDeliveryOptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutConfigurationSetDeliveryOptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutConfigurationSetDeliveryOptionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enable or disable collection of reputation metrics for emails that you send using a particular configuration set
* in a specific AWS Region.
*
*
* @param putConfigurationSetReputationOptionsRequest
* A request to enable or disable tracking of reputation metrics for a configuration set.
* @return Result of the PutConfigurationSetReputationOptions operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutConfigurationSetReputationOptions
* @see AWS API Documentation
*/
@Override
public PutConfigurationSetReputationOptionsResult putConfigurationSetReputationOptions(PutConfigurationSetReputationOptionsRequest request) {
request = beforeClientExecution(request);
return executePutConfigurationSetReputationOptions(request);
}
@SdkInternalApi
final PutConfigurationSetReputationOptionsResult executePutConfigurationSetReputationOptions(
PutConfigurationSetReputationOptionsRequest putConfigurationSetReputationOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(putConfigurationSetReputationOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutConfigurationSetReputationOptionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putConfigurationSetReputationOptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutConfigurationSetReputationOptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutConfigurationSetReputationOptionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enable or disable email sending for messages that use a particular configuration set in a specific AWS Region.
*
*
* @param putConfigurationSetSendingOptionsRequest
* A request to enable or disable the ability of Amazon Pinpoint to send emails that use a specific
* configuration set.
* @return Result of the PutConfigurationSetSendingOptions operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutConfigurationSetSendingOptions
* @see AWS API Documentation
*/
@Override
public PutConfigurationSetSendingOptionsResult putConfigurationSetSendingOptions(PutConfigurationSetSendingOptionsRequest request) {
request = beforeClientExecution(request);
return executePutConfigurationSetSendingOptions(request);
}
@SdkInternalApi
final PutConfigurationSetSendingOptionsResult executePutConfigurationSetSendingOptions(
PutConfigurationSetSendingOptionsRequest putConfigurationSetSendingOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(putConfigurationSetSendingOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutConfigurationSetSendingOptionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putConfigurationSetSendingOptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutConfigurationSetSendingOptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutConfigurationSetSendingOptionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Specify a custom domain to use for open and click tracking elements in email that you send using Amazon Pinpoint.
*
*
* @param putConfigurationSetTrackingOptionsRequest
* A request to add a custom domain for tracking open and click events to a configuration set.
* @return Result of the PutConfigurationSetTrackingOptions operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutConfigurationSetTrackingOptions
* @see AWS API Documentation
*/
@Override
public PutConfigurationSetTrackingOptionsResult putConfigurationSetTrackingOptions(PutConfigurationSetTrackingOptionsRequest request) {
request = beforeClientExecution(request);
return executePutConfigurationSetTrackingOptions(request);
}
@SdkInternalApi
final PutConfigurationSetTrackingOptionsResult executePutConfigurationSetTrackingOptions(
PutConfigurationSetTrackingOptionsRequest putConfigurationSetTrackingOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(putConfigurationSetTrackingOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutConfigurationSetTrackingOptionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putConfigurationSetTrackingOptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutConfigurationSetTrackingOptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutConfigurationSetTrackingOptionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Move a dedicated IP address to an existing dedicated IP pool.
*
*
*
* The dedicated IP address that you specify must already exist, and must be associated with your Amazon Pinpoint
* account.
*
*
* The dedicated IP pool you specify must already exist. You can create a new pool by using the
* CreateDedicatedIpPool
operation.
*
*
*
* @param putDedicatedIpInPoolRequest
* A request to move a dedicated IP address to a dedicated IP pool.
* @return Result of the PutDedicatedIpInPool operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutDedicatedIpInPool
* @see AWS API Documentation
*/
@Override
public PutDedicatedIpInPoolResult putDedicatedIpInPool(PutDedicatedIpInPoolRequest request) {
request = beforeClientExecution(request);
return executePutDedicatedIpInPool(request);
}
@SdkInternalApi
final PutDedicatedIpInPoolResult executePutDedicatedIpInPool(PutDedicatedIpInPoolRequest putDedicatedIpInPoolRequest) {
ExecutionContext executionContext = createExecutionContext(putDedicatedIpInPoolRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutDedicatedIpInPoolRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putDedicatedIpInPoolRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutDedicatedIpInPool");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutDedicatedIpInPoolResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* @param putDedicatedIpWarmupAttributesRequest
* A request to change the warm-up attributes for a dedicated IP address. This operation is useful when you
* want to resume the warm-up process for an existing IP address.
* @return Result of the PutDedicatedIpWarmupAttributes operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
@Override
public PutDedicatedIpWarmupAttributesResult putDedicatedIpWarmupAttributes(PutDedicatedIpWarmupAttributesRequest request) {
request = beforeClientExecution(request);
return executePutDedicatedIpWarmupAttributes(request);
}
@SdkInternalApi
final PutDedicatedIpWarmupAttributesResult executePutDedicatedIpWarmupAttributes(PutDedicatedIpWarmupAttributesRequest putDedicatedIpWarmupAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(putDedicatedIpWarmupAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutDedicatedIpWarmupAttributesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putDedicatedIpWarmupAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutDedicatedIpWarmupAttributes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutDedicatedIpWarmupAttributesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enable or disable the Deliverability dashboard for your Amazon Pinpoint account. When you enable the
* Deliverability dashboard, you gain access to reputation, deliverability, and other metrics for the domains that
* you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox placement
* tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon Pinpoint. For more information about the features and cost of a Deliverability
* dashboard subscription, see Amazon Pinpoint Pricing.
*
*
* @param putDeliverabilityDashboardOptionRequest
* Enable or disable the Deliverability dashboard for your Amazon Pinpoint account. When you enable the
* Deliverability dashboard, you gain access to reputation, deliverability, and other metrics for the domains
* that you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox
* placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon Pinpoint. For more information about the features and cost of a
* Deliverability dashboard subscription, see Amazon
* Pinpoint Pricing.
* @return Result of the PutDeliverabilityDashboardOption operation returned by the service.
* @throws AlreadyExistsException
* The resource specified in your request already exists.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws LimitExceededException
* There are too many instances of the specified resource type.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutDeliverabilityDashboardOption
* @see AWS API Documentation
*/
@Override
public PutDeliverabilityDashboardOptionResult putDeliverabilityDashboardOption(PutDeliverabilityDashboardOptionRequest request) {
request = beforeClientExecution(request);
return executePutDeliverabilityDashboardOption(request);
}
@SdkInternalApi
final PutDeliverabilityDashboardOptionResult executePutDeliverabilityDashboardOption(
PutDeliverabilityDashboardOptionRequest putDeliverabilityDashboardOptionRequest) {
ExecutionContext executionContext = createExecutionContext(putDeliverabilityDashboardOptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutDeliverabilityDashboardOptionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putDeliverabilityDashboardOptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutDeliverabilityDashboardOption");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutDeliverabilityDashboardOptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Used to enable or disable DKIM authentication for an email identity.
*
*
* @param putEmailIdentityDkimAttributesRequest
* A request to enable or disable DKIM signing of email that you send from an email identity.
* @return Result of the PutEmailIdentityDkimAttributes operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutEmailIdentityDkimAttributes
* @see AWS API Documentation
*/
@Override
public PutEmailIdentityDkimAttributesResult putEmailIdentityDkimAttributes(PutEmailIdentityDkimAttributesRequest request) {
request = beforeClientExecution(request);
return executePutEmailIdentityDkimAttributes(request);
}
@SdkInternalApi
final PutEmailIdentityDkimAttributesResult executePutEmailIdentityDkimAttributes(PutEmailIdentityDkimAttributesRequest putEmailIdentityDkimAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(putEmailIdentityDkimAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutEmailIdentityDkimAttributesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putEmailIdentityDkimAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutEmailIdentityDkimAttributes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutEmailIdentityDkimAttributesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Used to enable or disable feedback forwarding for an identity. This setting determines what happens when an
* identity is used to send an email that results in a bounce or complaint event.
*
*
* When you enable feedback forwarding, Amazon Pinpoint sends you email notifications when bounce or complaint
* events occur. Amazon Pinpoint sends this notification to the address that you specified in the Return-Path header
* of the original email.
*
*
* When you disable feedback forwarding, Amazon Pinpoint sends notifications through other mechanisms, such as by
* notifying an Amazon SNS topic. You're required to have a method of tracking bounces and complaints. If you
* haven't set up another mechanism for receiving bounce or complaint notifications, Amazon Pinpoint sends an email
* notification when these events occur (even if this setting is disabled).
*
*
* @param putEmailIdentityFeedbackAttributesRequest
* A request to set the attributes that control how bounce and complaint events are processed.
* @return Result of the PutEmailIdentityFeedbackAttributes operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutEmailIdentityFeedbackAttributes
* @see AWS API Documentation
*/
@Override
public PutEmailIdentityFeedbackAttributesResult putEmailIdentityFeedbackAttributes(PutEmailIdentityFeedbackAttributesRequest request) {
request = beforeClientExecution(request);
return executePutEmailIdentityFeedbackAttributes(request);
}
@SdkInternalApi
final PutEmailIdentityFeedbackAttributesResult executePutEmailIdentityFeedbackAttributes(
PutEmailIdentityFeedbackAttributesRequest putEmailIdentityFeedbackAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(putEmailIdentityFeedbackAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutEmailIdentityFeedbackAttributesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putEmailIdentityFeedbackAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutEmailIdentityFeedbackAttributes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutEmailIdentityFeedbackAttributesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Used to enable or disable the custom Mail-From domain configuration for an email identity.
*
*
* @param putEmailIdentityMailFromAttributesRequest
* A request to configure the custom MAIL FROM domain for a verified identity.
* @return Result of the PutEmailIdentityMailFromAttributes operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.PutEmailIdentityMailFromAttributes
* @see AWS API Documentation
*/
@Override
public PutEmailIdentityMailFromAttributesResult putEmailIdentityMailFromAttributes(PutEmailIdentityMailFromAttributesRequest request) {
request = beforeClientExecution(request);
return executePutEmailIdentityMailFromAttributes(request);
}
@SdkInternalApi
final PutEmailIdentityMailFromAttributesResult executePutEmailIdentityMailFromAttributes(
PutEmailIdentityMailFromAttributesRequest putEmailIdentityMailFromAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(putEmailIdentityMailFromAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutEmailIdentityMailFromAttributesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putEmailIdentityMailFromAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutEmailIdentityMailFromAttributes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutEmailIdentityMailFromAttributesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sends an email message. You can use the Amazon Pinpoint Email API to send two types of messages:
*
*
* -
*
* Simple – A standard email message. When you create this type of message, you specify the sender, the
* recipient, and the message body, and Amazon Pinpoint assembles the message for you.
*
*
* -
*
* Raw – A raw, MIME-formatted email message. When you send this type of email, you have to specify all of
* the message headers, as well as the message body. You can use this message type to send messages that contain
* attachments. The message that you specify has to be a valid MIME message.
*
*
*
*
* @param sendEmailRequest
* A request to send an email message.
* @return Result of the SendEmail operation returned by the service.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws LimitExceededException
* There are too many instances of the specified resource type.
* @throws AccountSuspendedException
* The message can't be sent because the account's ability to send email has been permanently restricted.
* @throws SendingPausedException
* The message can't be sent because the account's ability to send email is currently paused.
* @throws MessageRejectedException
* The message can't be sent because it contains invalid content.
* @throws MailFromDomainNotVerifiedException
* The message can't be sent because the sending domain isn't verified.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.SendEmail
* @see AWS API
* Documentation
*/
@Override
public SendEmailResult sendEmail(SendEmailRequest request) {
request = beforeClientExecution(request);
return executeSendEmail(request);
}
@SdkInternalApi
final SendEmailResult executeSendEmail(SendEmailRequest sendEmailRequest) {
ExecutionContext executionContext = createExecutionContext(sendEmailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SendEmailRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(sendEmailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SendEmail");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new SendEmailResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Add one or more tags (keys and values) to a specified resource. A tag is a label that you optionally
* define and associate with a resource in Amazon Pinpoint. Tags can help you categorize and manage resources in
* different ways, such as by purpose, owner, environment, or other criteria. A resource can have as many as 50
* tags.
*
*
* Each tag consists of a required tag key and an associated tag value, both of which you define. A
* tag key is a general label that acts as a category for more specific tag values. A tag value acts as a descriptor
* within a tag key.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ConcurrentModificationException
* The resource is being modified by another operation or thread.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @sample AmazonPinpointEmail.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Remove one or more tags (keys and values) from a specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ConcurrentModificationException
* The resource is being modified by another operation or thread.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @sample AmazonPinpointEmail.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update the configuration of an event destination for a configuration set.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param updateConfigurationSetEventDestinationRequest
* A request to change the settings for an event destination for a configuration set.
* @return Result of the UpdateConfigurationSetEventDestination operation returned by the service.
* @throws NotFoundException
* The resource you attempted to access doesn't exist.
* @throws TooManyRequestsException
* Too many requests have been made to the operation.
* @throws BadRequestException
* The input you provided is invalid.
* @sample AmazonPinpointEmail.UpdateConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public UpdateConfigurationSetEventDestinationResult updateConfigurationSetEventDestination(UpdateConfigurationSetEventDestinationRequest request) {
request = beforeClientExecution(request);
return executeUpdateConfigurationSetEventDestination(request);
}
@SdkInternalApi
final UpdateConfigurationSetEventDestinationResult executeUpdateConfigurationSetEventDestination(
UpdateConfigurationSetEventDestinationRequest updateConfigurationSetEventDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(updateConfigurationSetEventDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateConfigurationSetEventDestinationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateConfigurationSetEventDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Pinpoint Email");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateConfigurationSetEventDestination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateConfigurationSetEventDestinationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}